"A Tour of C++ (3rd Edition) provides a comprehensive overview of the C++ programming language, emphasizing modern practices and features while also guiding readers through practical examples and applications."
Here's a simple code snippet showcasing a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++: The Basics
What is C++?
C++ is a robust, high-level programming language that supports both procedural and object-oriented programming paradigms. Originally developed by Bjarne Stroustrup in the late 1970s, C++ has become a cornerstone in software development, providing the flexibility and power necessary for writing performance-critical applications.
When comparing C++ to other programming languages such as Python or Java, a few distinctions emerge:
- Performance: C++ is often faster than interpreted languages due to its compiled nature.
- Memory Control: Offers more control over system resources, allowing manual memory management.
- Versatility: Used in various domains, from system software and game development to high-frequency trading.
Example: Basic "Hello World" Program in C++
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Key Features of C++
Object-Oriented Programming (OOP)
C++ stands out with its rich support for object-oriented programming concepts. This paradigm allows developers to model real-world entities in code through:
- Classes and Objects: Blueprint for creating objects (instances) and containing data and methods.
- Abstraction: Hiding complex realities while exposing only the necessary parts.
- Encapsulation: Bundling data along with the methods that operate on that data.
- Inheritance: Creating new classes based on existing classes, promoting code reusability.
Standard Template Library (STL)
The Standard Template Library is a powerful feature of C++ that provides a set of generic classes and functions. With STL, developers can utilize pre-built algorithms and data structures, which significantly speeds up the development process.
By utilizing templates, developers can create classes or functions with a placeholder for the actual type, making code reusable and efficient.
The Structure of C++ Programs
Syntax and Semantics
C++ syntax is determined by a set of rules that defines the structure of the code. Key components include:
- Data Types: Fundamental types like `int`, `float`, and user-defined types like `class`.
- Operators: Symbols for operations, including arithmetic, relational, and logical operations.
- Control Structures: Statements for decision-making, looping, and branching like `if`, `for`, and `switch`.
Code Snippet: Simple Control Flow Example
#include <iostream>
int main() {
int number = 10;
if (number > 0) {
std::cout << "Positive number" << std::endl;
} else {
std::cout << "Negative number" << std::endl;
}
return 0;
}
Compiling and Executing C++ Programs
To run a C++ program, follow these steps:
- Compile the Code: Transform the code into an executable.
g++ filename.cpp -o output
- Execute the Program: Run the compiled output.
./output
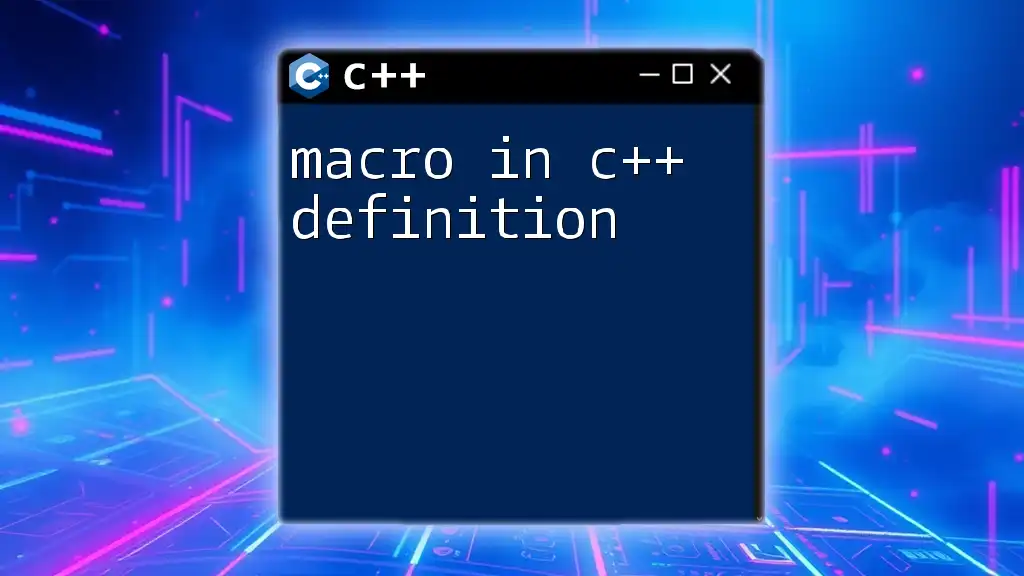
In-Depth Features of C++ 3rd Edition
Enhanced Standard Library
The 3rd edition of C++ introduced several new libraries and enhancements to existing frameworks. One notable addition is the `<algorithm>` header which includes a variety of data manipulation algorithms.
Code Example: Using `<algorithm>` for Sorting
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {5, 2, 8, 1, 3};
std::sort(nums.begin(), nums.end());
for (int num : nums) {
std::cout << num << " ";
}
return 0;
}
Exception Handling
Error management is a crucial aspect of programming. C++ provides a robust mechanism for handling exceptions to ensure that programs can continue running or fail gracefully.
Example: Try-Catch Block in C++
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
return 0;
}
Object-Oriented Enhancements
C++ has solidified its strengths in OOP with features such as virtual functions, which enable polymorphism. This allows methods to be redefined in derived classes.
Code Example:
#include <iostream>
class Base {
public:
virtual void show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class" << std::endl;
}
};
int main() {
Base* b = new Derived();
b->show(); // Outputs "Derived class"
delete b;
return 0;
}
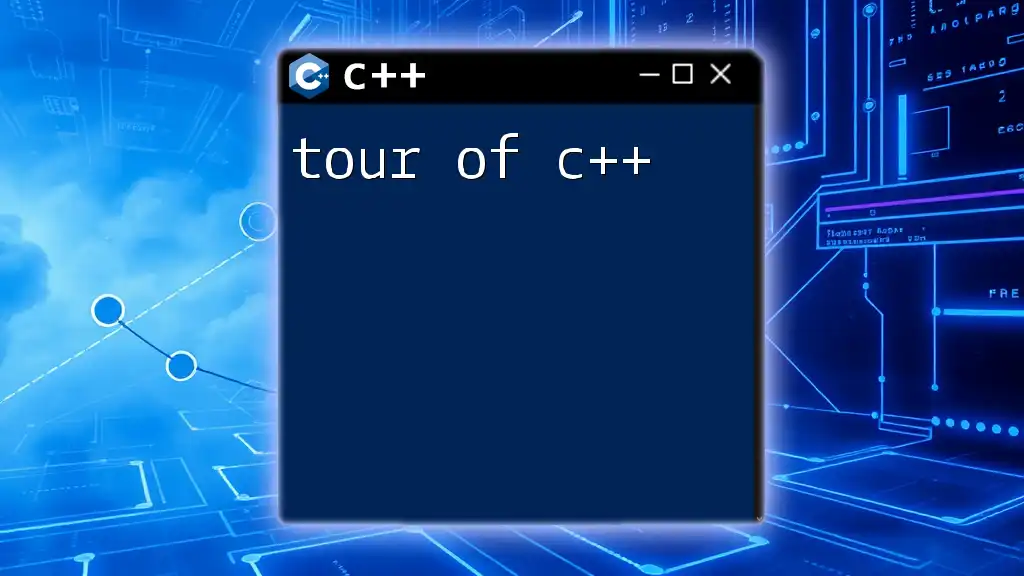
Advanced C++: Templates and Meta-programming
Introduction to Templates
Templates facilitate the creation of generic, reusable code. They allow for defining functions and classes that can operate with any data type.
Example: Creating a Function Template
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << add(5, 3) << std::endl; // Works with integers
std::cout << add(3.5, 2.5) << std::endl; // Works with doubles
return 0;
}
Meta-programming Basics
Meta-programming allows programmers to leverage the C++ type system for compiler-based programming. It serves to generate code that is optimized for performance.
This is especially useful for tasks such as type traits and SFINAE (Substitution Failure Is Not An Error), which help in creating flexible code that adapts to different types.
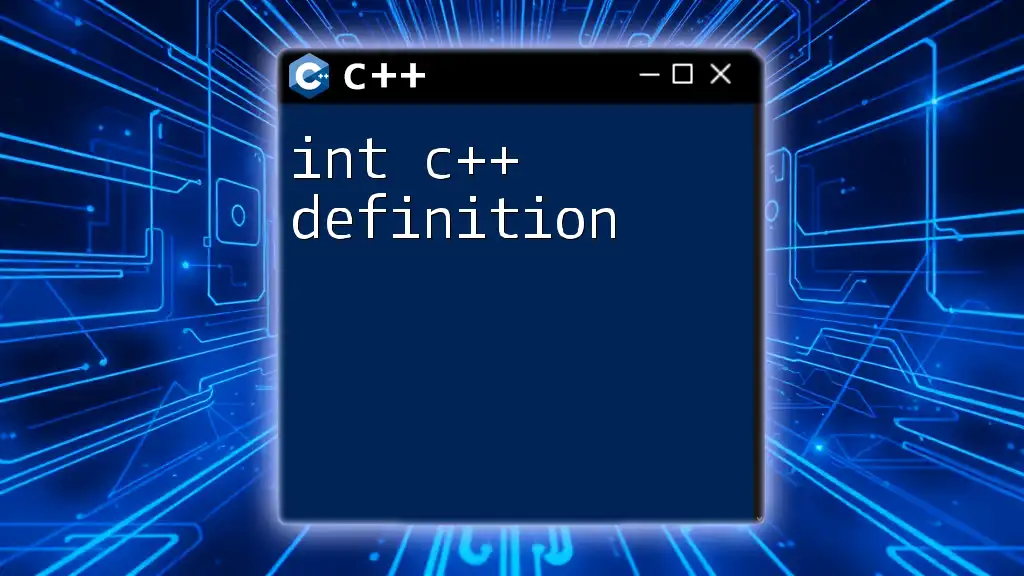
The Best Practices in C++ Programming
Coding Standards
Maintaining coding standards is vital for readability and manageability. Adhering to convention ensures that the code can be easily understood and maintained in future.
Key points include:
- Use descriptive names for variables and functions.
- Keep functions short and focused on a single task.
- Document code adequately with comments.
Memory Management
Efficient memory management is crucial in C++. Understanding dynamic versus static memory allocation can lead to significant performance improvements.
Example: Using Pointers and the `new` Operator
#include <iostream>
int main() {
int* ptr = new int; // dynamic allocation
*ptr = 42;
std::cout << *ptr << std::endl;
delete ptr; // free memory
return 0;
}
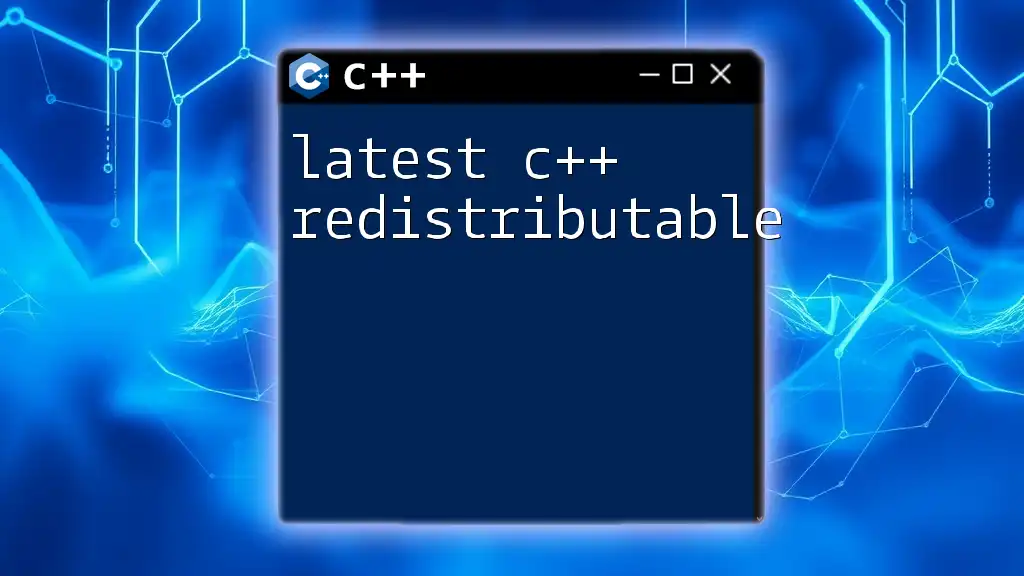
Common Pitfalls in C++
Mistakes to Avoid
While C++ is a powerful language, it can also lead to complications if not carefully managed. Common pitfalls include:
- Memory leaks due to forgotten `delete` statements.
- Undefined behavior from accessing uninitialized pointers.
- Mismanaging object lifetimes, leading to dangling references.
Debugging Techniques
Effective debugging is critical in C++. Tools such as GDB and Valgrind help developers identify issues in their code.
- GDB: A debugger for tracking down bugs in compiled code.
- Valgrind: Tool for memory debugging, detecting leaks and memory errors.
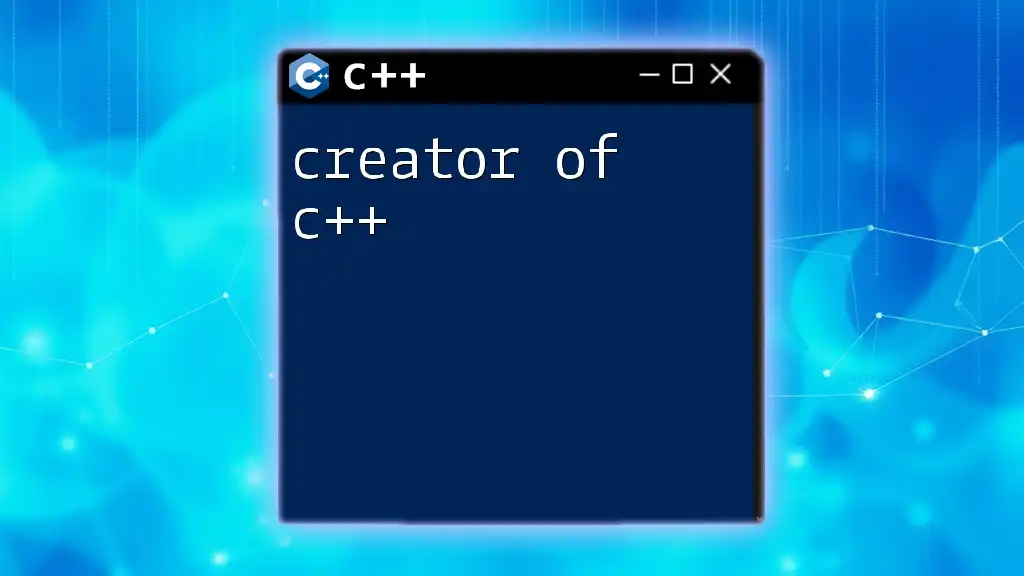
Conclusion
Exploring "a tour of C++ 3rd edition" reveals a language that, while steeped in tradition, continually evolves to meet modern programming challenges. Mastering its features—from object-oriented programming to advanced templates—opens up a landscape of possibilities for optimal performance in software development.
Harnessing these capabilities will not only enhance your coding skills but also allow you to tackle complex projects with confidence.