A macro in C++ is a preprocessor directive that defines a shorthand for a specific code snippet, allowing for easier code maintenance and readability.
Here’s a simple example of a macro in C++:
#define SQUARE(x) ((x) * (x))
What is a Macro?
A macro in C++ is a fragment of code that has been given a name. Whenever the name is used, it is replaced by the contents of the macro. Macros are a powerful tool in programming, allowing code to be reused without rewriting it. They differ from functions in that they perform text substitutions before compilation, thus the replacement occurs during the preprocessing phase of compilation.
One significant advantage of macros is that they can reduce code duplication and enhance maintainability. However, they can also lead to complications, such as unexpected behaviors due to incorrect definitions or expansions.
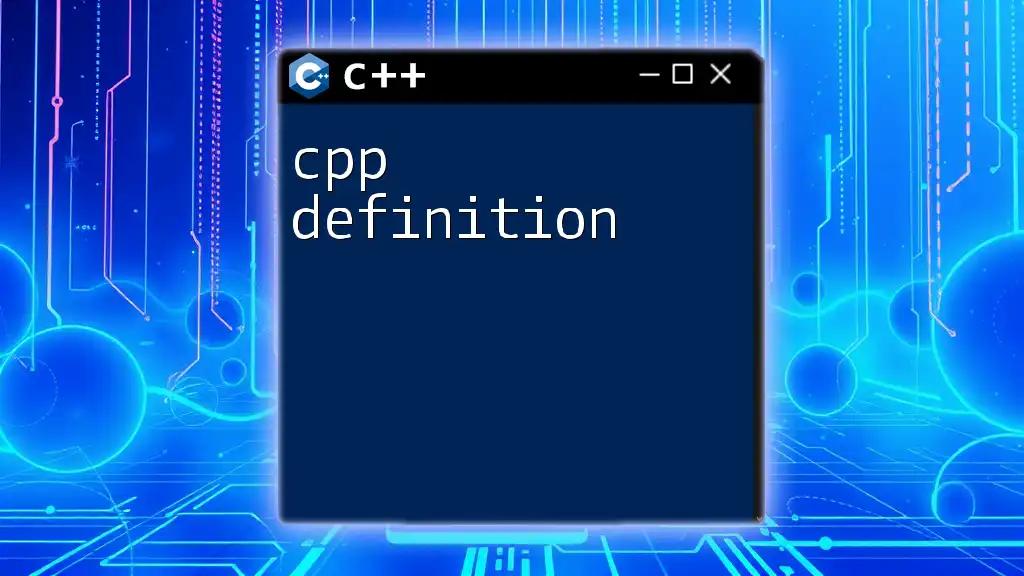
Syntax of Macros
The syntax for defining a macro is straightforward. A macro is defined using the `#define` directive, which is a preprocessor command.
An example of defining a simple macro is:
#define MACRO_NAME value
This creates a macro called `MACRO_NAME` that will be replaced by `value` throughout the code.
Understanding the process of token replacement is crucial. Macros can replace single tokens or more complex sequences, making them versatile yet potentially hazardous if not managed with care.
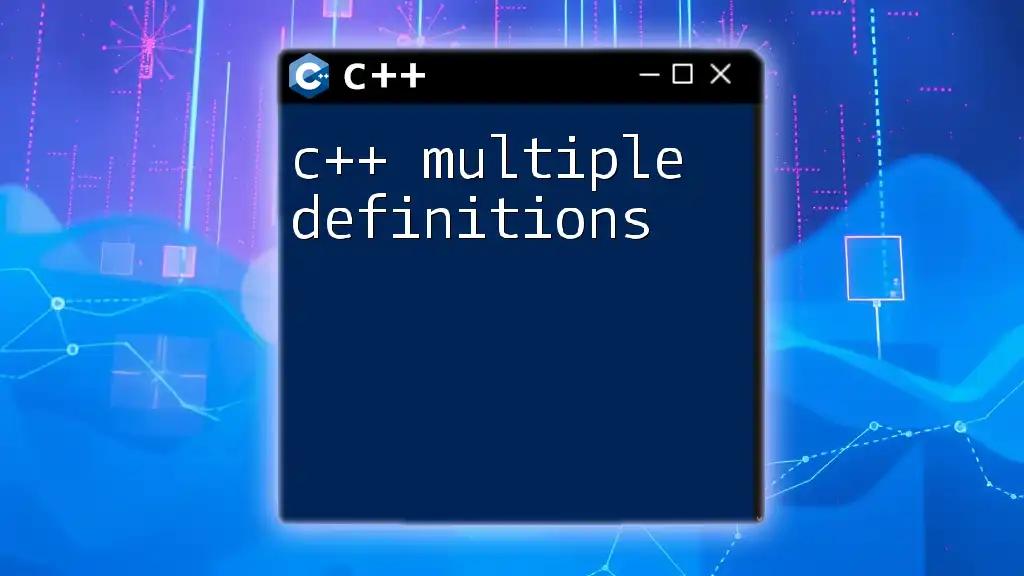
Types of Macros
Object-like Macros
Object-like macros are simple definitions that define a constant or a simple replacement. They do not take parameters, serving primarily as constants or predefined values.
For example:
#define PI 3.14
In this case, `PI` is a macro that will be replaced with `3.14` wherever it appears in the source code. Object-like macros are useful for defining constants in a centralized manner.
Function-like Macros
Function-like macros resemble functions but do not have the same overhead. They allow you to pass parameters but operate at the text substitution level.
Here's an example:
#define SQUARE(x) ((x) * (x))
When you use `SQUARE(4)`, the macro expands to `((4) * (4))`, resulting in `16`. While function-like macros can make code concise, using them can lead to unexpected behavior or hard-to-debug errors.
For instance, if you mistakenly call `SQUARE(i++)`, it may lead to multiple increments of `i`, which is something you would not see with a conventional function.
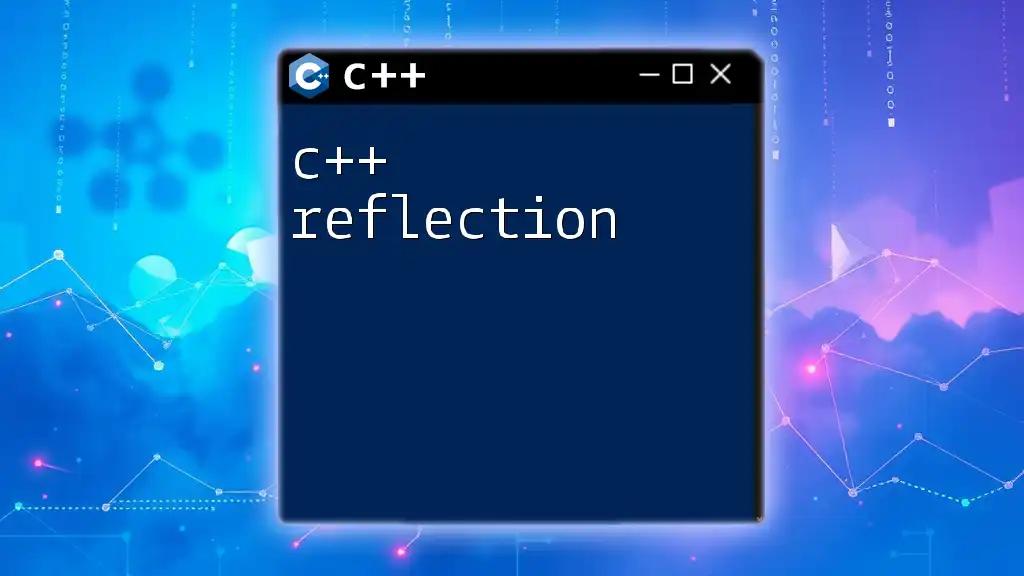
Common Use Cases for Macros
Conditional Compilation
Macros are frequently used for conditional compilation. This allows developers to include or exclude parts of the code based on specific conditions, such as platform compatibility or debugging needs.
Here’s an example:
#ifdef DEBUG
#define LOG(x) std::cout << x << std::endl;
#else
#define LOG(x)
#endif
In this scenario, if `DEBUG` is defined, the macro `LOG` will output logs, making it easier to trace the program's execution during debugging. If `DEBUG` is not defined, the macro does nothing, effectively preventing log output from compiling into the final binary.
Code Optimization
Macros can enhance code efficiency, especially for repeated calculations or settings that remain constant throughout the program. They streamline code and can prevent overhead from function calls.
For example, logging macros can significantly reduce code clutter and facilitate switchable logging layers.
Compatibility with Different Environments
For programs that need to run across various platforms, macros can adjust functionality to accommodate differences in system architectures or libraries.
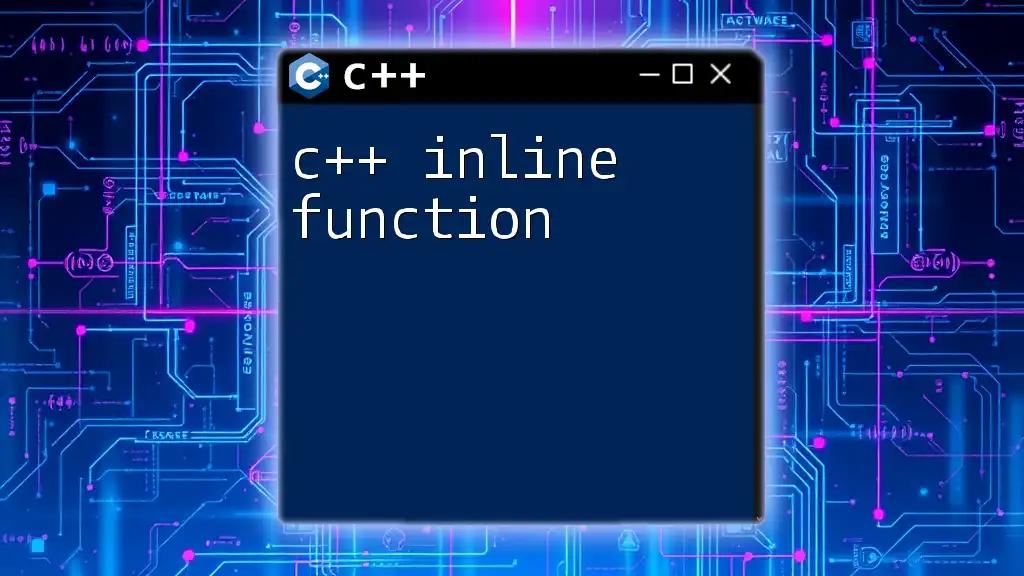
Using Macros Wisely
Potential Pitfalls
Despite their advantages, macros can lead to various issues if not utilized properly. Some common pitfalls include unexpected expansions and scope-related problems.
For example, consider this flawed macro definition:
#define DOUBLE(x) (x + x) // Pitfall
If you call `DOUBLE(a++)`, the expansion becomes `(a++ + a++)`, which increments `a` twice, potentially leading to unexpected results. Thus, it is crucial to be cautious with arguments passed to macros.
Best Practices
To ensure safe and effective macro usage, adhere to these guidelines:
- Limit Macro Use: Prefer constants (`const` or `constexpr`) or inline functions when possible. These alternatives offer type-safety and improved readability.
- Use Parentheses: When defining macros, always enclose parameters in parentheses to avoid precedence issues.
- Avoid Side Effects: Be extremely cautious if your macro arguments have side effects, such as incrementing or modifying variables.
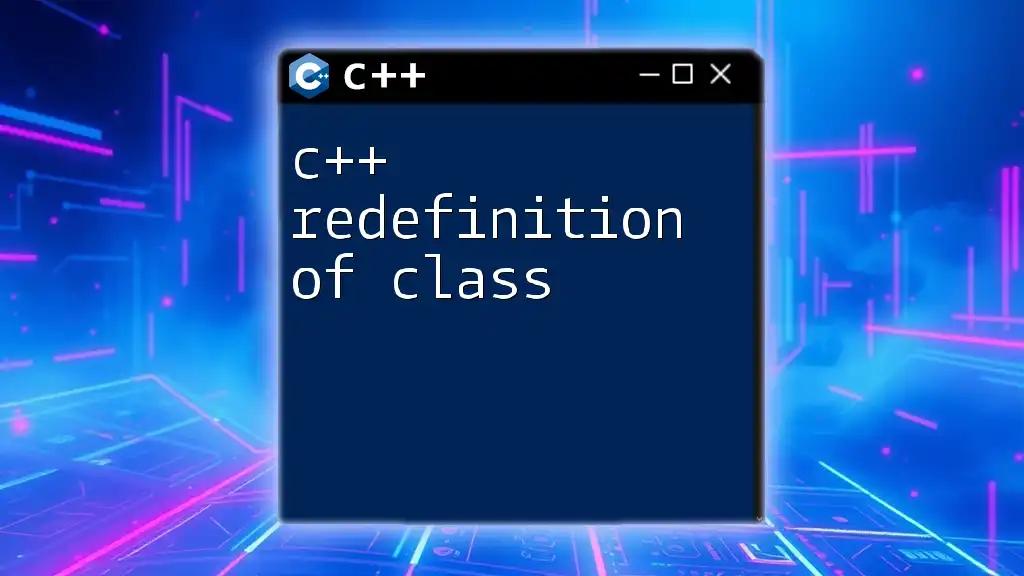
Alternatives to Macros
With advancements in C++, several reliable alternatives to macros emerged, such as:
- Inline Functions: Inline functions allow you to define small, reusable code snippets without the text substitution hazards that accompany macros.
- `constexpr`: For constants that can be computed at compile-time, using `constexpr` provides type safety and maintains code performance without the unpredictability of macros.
- Templates: Templates can offer generic behavior without the issues of macro expansions, enhancing both type safety and maintainability.

Conclusion
In summary, understanding the macro in C++ definition is essential for effective C++ programming. Macros significantly aid in code reusability and readability but come with risks that must be acknowledged. Employ them wisely and consider alternatives where they make more sense. Familiarity with macros will undoubtedly enhance your programming toolkit and allow for more sophisticated and efficient code structures.
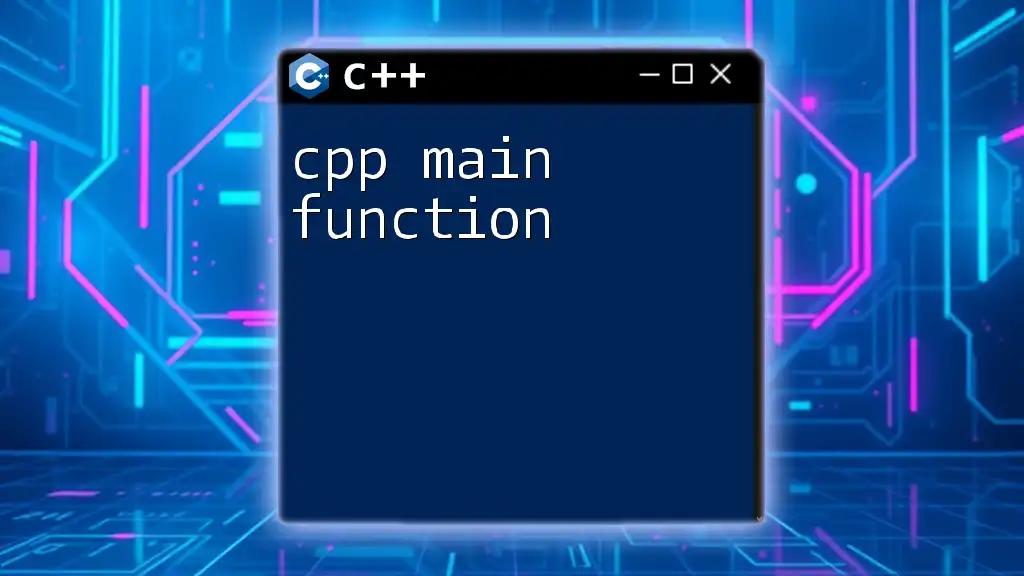
Additional Resources
To delve deeper into C++, consider accessing literature and online resources focused on macro usage, robust coding practices, and alternative strategies. Several tools and IDEs also support macro usage and can aid in efficient macro management.
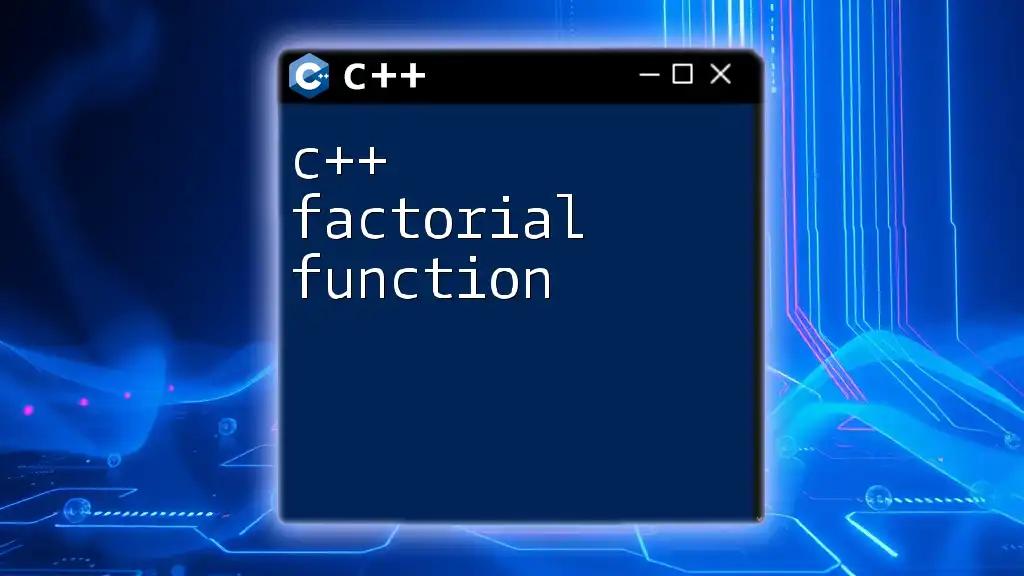
FAQs About Macros in C++
-
Q: Are macros type-safe?
A: No, macros are not type-safe as their expansion occurs at the preprocessor level, and they do not understand types. -
Q: When should I use inline functions instead of macros?
A: Use inline functions when you need type safety, while macros are better for simple substitutions. -
Q: What are the key differences between macros and functions?
A: The primary differences are in their behavior, with macros being expanded during the preprocessing phase and functions being invoked at runtime, showcasing type safety and scoping rules.
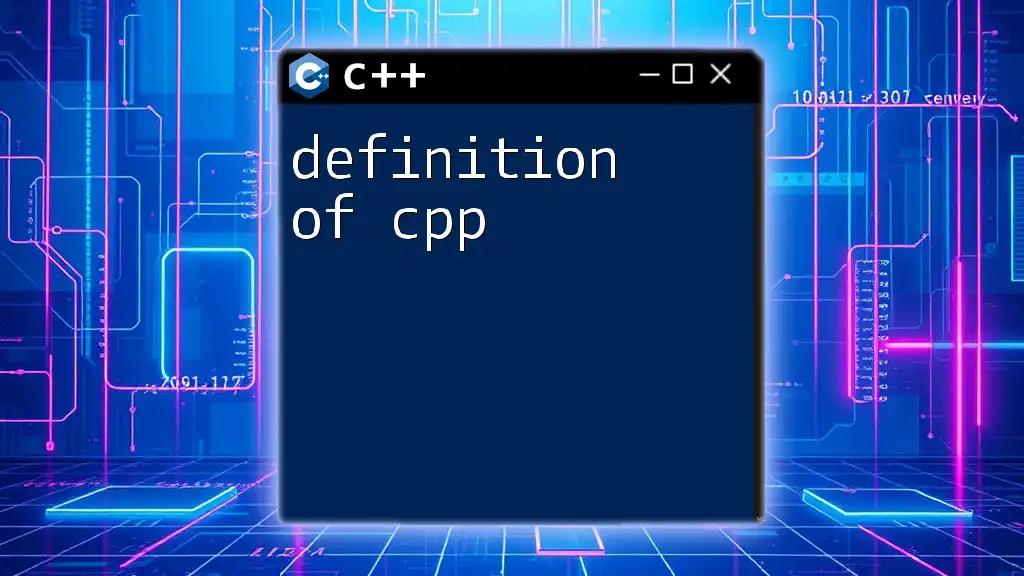
Code Playground
For hands-on experience with macros and experimentation, consider using online platforms like Repl.it or CodeSandbox where you can test your code and witness macros in action.