In C++, multiple definitions occur when the same variable or function is declared in more than one translation unit, leading to a linker error; to avoid this, you should use `extern` for variables or include header guards for function definitions.
Here’s a simple example to demonstrate a variable definition issue:
// File: main.cpp
#include <iostream>
// Incorrectly defining the variable in multiple files
int globalVar = 5; // Definition
int main() {
std::cout << globalVar << std::endl;
return 0;
}
And another file that also incorrectly defines the same variable:
// File: another.cpp
int globalVar = 10; // Definition, which leads to multiple definitions error
To fix this, declare it in a header file (`globals.h`):
// File: globals.h
#ifndef GLOBALS_H
#define GLOBALS_H
extern int globalVar; // Declaration, not definition
#endif // GLOBALS_H
And then define it in one source file:
// File: globals.cpp
#include "globals.h"
int globalVar = 5; // Definition
Now you can include `globals.h` in any other source files without causing multiple definition errors.
Understanding C++ Multiple Definitions
What is a Multiple Definition in C++?
In C++, a multiple definition occurs when the same variable, function, or class is defined more than once within the same scope. This typically leads to compilation errors, as the compiler cannot ascertain which definition to use. Understanding the nuances of multiple definitions is essential for C++ developers to create clear, maintainable, and error-free code.
When Do Multiple Definitions Occur?
Multiple definitions can arise in various scenarios:
-
Global Variables: If a global variable is defined in multiple translation units (e.g., different .cpp files), the linker will produce an error stating that there are multiple definitions of that variable.
-
Function Definitions: Similar to global variables, defining a non-static function in more than one .cpp file will lead to a conflict during linking.
-
Class Definitions: If a class is defined multiple times without proper checks (such as guards), it can also trigger multiple definition errors.
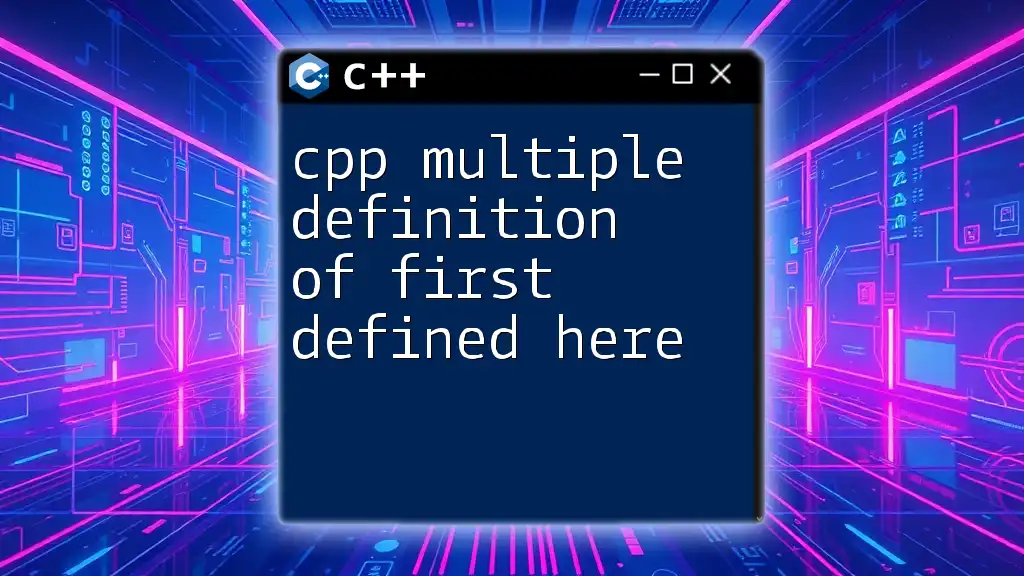
The C++ One Definition Rule (ODR)
Overview of the One Definition Rule
The One Definition Rule (ODR) states that a variable, function, class, or any other entity must have exactly one definition across the entire program. While you can have multiple declarations, the rule emphasizes that only one unique definition is allowed. Violating this rule can lead to multiple definition errors and unpredictable behavior in your application.
Scenarios Where ODR is Violated
-
Static vs. Non-static Functions: Static functions are limited to their translation unit and do not violate ODR, while defining non-static functions in multiple files does.
-
Inline Functions: These can be defined in multiple translation units, but if not handled properly (e.g., include guards or `static` keyword), they can lead to multiple definitions during linking.
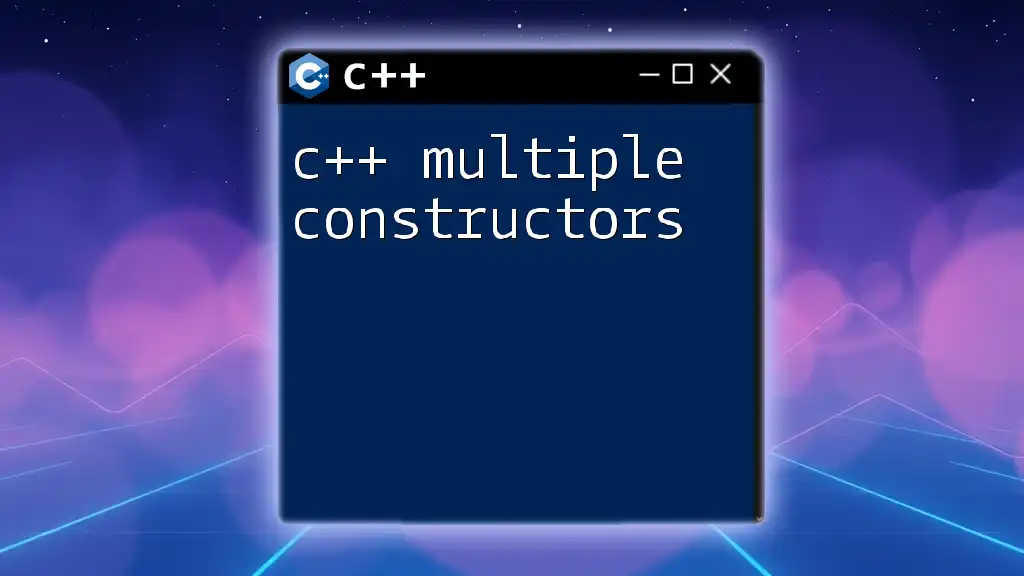
Example of Multiple Definitions in C++
Code Snippet Demonstration
Consider the following simple example where a global variable is unintentionally defined in multiple sources:
// file1.cpp
int value = 10; // Global variable
void printValue() {
std::cout << "Value in file1: " << value << std::endl;
}
// file2.cpp
int value = 10; // Global variable, defined again
void printValue() {
std::cout << "Value in file2: " << value << std::endl;
}
Analyzing the Error
When attempting to compile the above code, the linker will produce an error along the lines of "redefinition of 'value'". This indicates that the variable `value` has been defined in more than one compilation unit, thus violating the One Definition Rule.
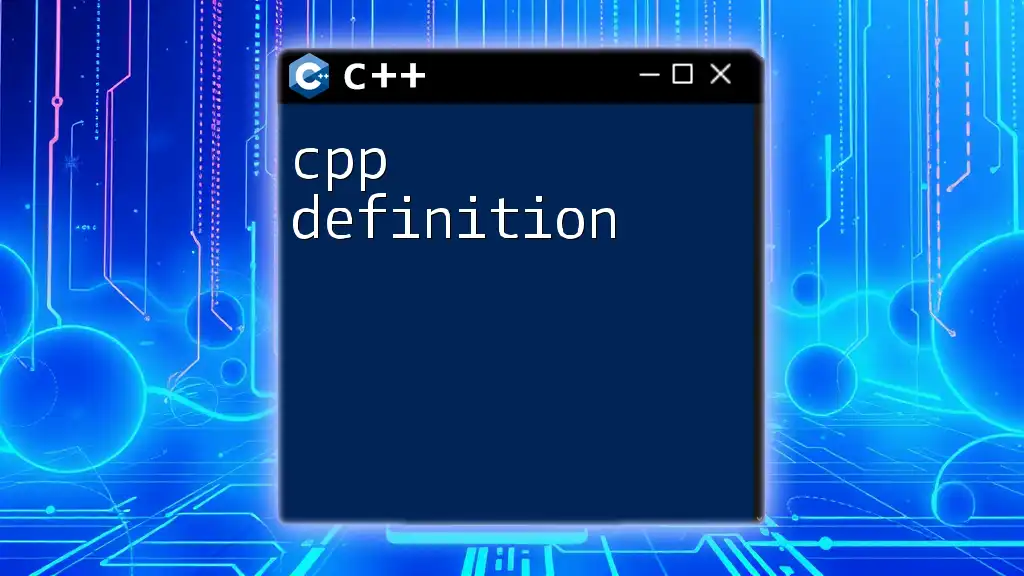
Fixing Multiple Definitions in C++
Using Header Guards
Header guards are preprocessor directives used to prevent a header file from being included multiple times, which can lead to multiple definitions. They should be used in every header file.
// my_header.h
#ifndef MY_HEADER_H
#define MY_HEADER_H
int value; // Just a declaration
#endif // MY_HEADER_H
By using header guards, the global variable `value` is declared in the header file but defined only once wherever necessary, avoiding any multiple definition errors.
Utilizing `extern` Keyword
The `extern` keyword informs the compiler that a variable exists but is defined in another translation unit. This allows you to declare global variables without defining them multiple times.
// my_header.h
extern int value; // Declared, but no definition
// my_source.cpp
int value = 10; // Defined only here.
This pattern assures that `value` is only defined once, while its declaration can be included across multiple files without causing conflicts.
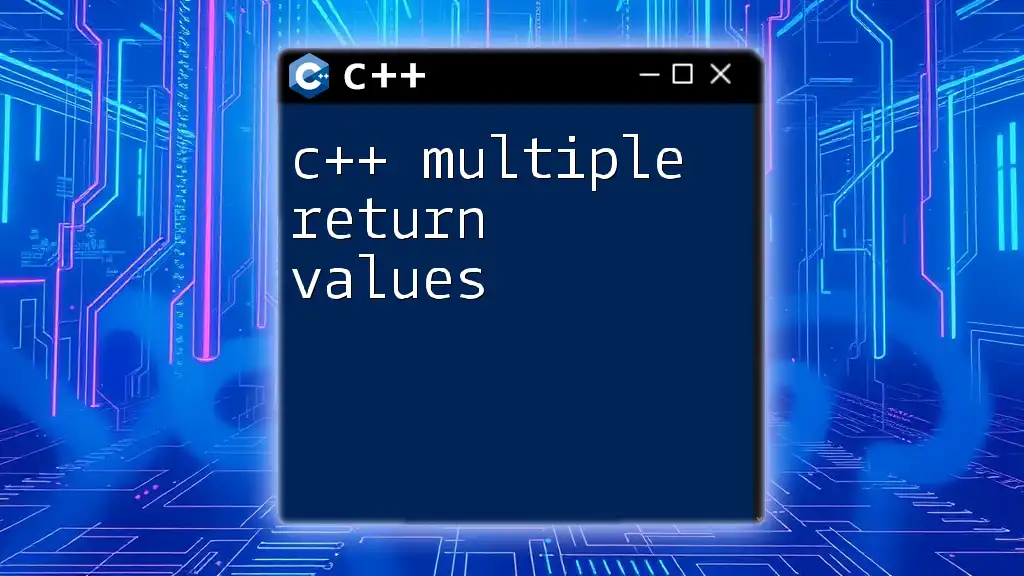
Best Practices to Avoid Multiple Definitions
Organizing Code with a Modular Approach
A modular approach encourages organizing code into separate files and folders, making definitions distinct and reducing the likelihood of unintended multiple definitions. Utilize header files for declarations and source files for definitions.
Using Inline Functions Wisely
Inline functions can be defined in header files and can mitigate multiple definitions due to C++'s inline specification. However, developers must ensure that the functions are indeed simple and should only be used in scenarios where performance is crucial.
inline void printValue() {
std::cout << "This is an inline function." << std::endl;
}
Inline functions can be defined in multiple translation units, but need to be straightforward to avoid complications.
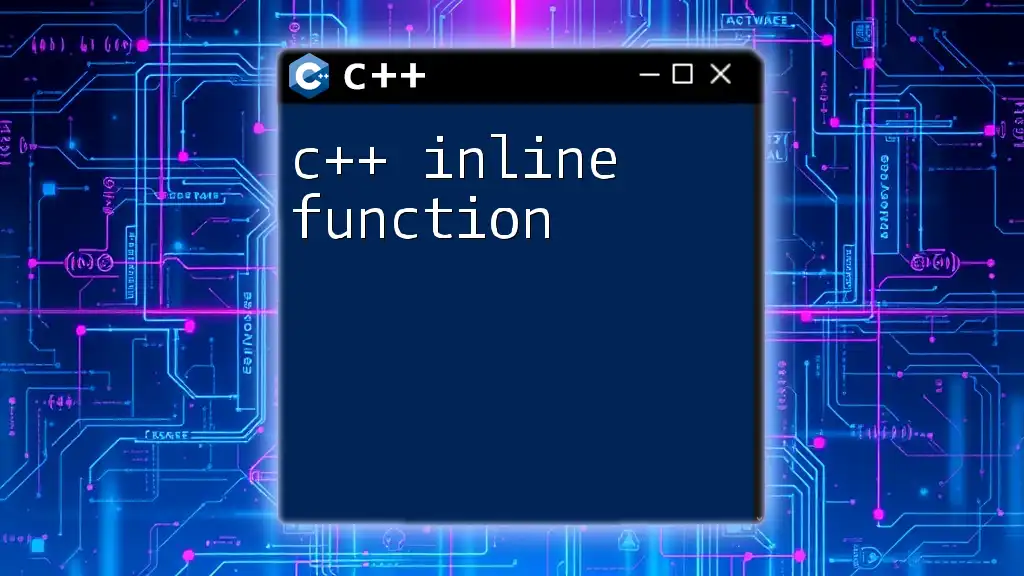
Conclusion
In summary, understanding C++ multiple definitions is crucial for any C++ developer. Recognizing the implications of the One Definition Rule and employing techniques like header guards and the `extern` keyword can significantly mitigate related errors in your programs. By adhering to best practices—like modular code organization and careful use of inline functions—you can develop cleaner, more robust applications, free of multiple definition headaches.
For more tips and insights on mastering C++, stay tuned for our upcoming posts, and join our community to further enhance your skills!