In C++, multiple return values can be achieved using `std::tuple`, `std::pair`, or output parameters, allowing a function to return more than one value neatly.
Here's a simple example using `std::pair`:
#include <iostream>
#include <utility>
std::pair<int, double> getValues() {
return {42, 3.14};
}
int main() {
auto [integerValue, doubleValue] = getValues();
std::cout << "Integer: " << integerValue << ", Double: " << doubleValue << std::endl;
return 0;
}
Understanding Function Return Types
What is a Return Type?
A return type in C++ defines the type of value that a function will produce and return after execution. By default, functions can return only one value specified by their return type. However, there are methods to circumvent this limitation, allowing functions to return multiple values.
Why Return Multiple Values?
Returning multiple values can enhance the capability of functions, providing more information without requiring multiple function calls. This pattern is particularly useful in scenarios such as:
- Calculating both the quotient and remainder of a division operation.
- Returning both coordinates of a point from a mathematical computation.
- Packing necessary information for user-defined objects.
The ability to return multiple values can improve code clarity and efficiency, particularly in larger applications.
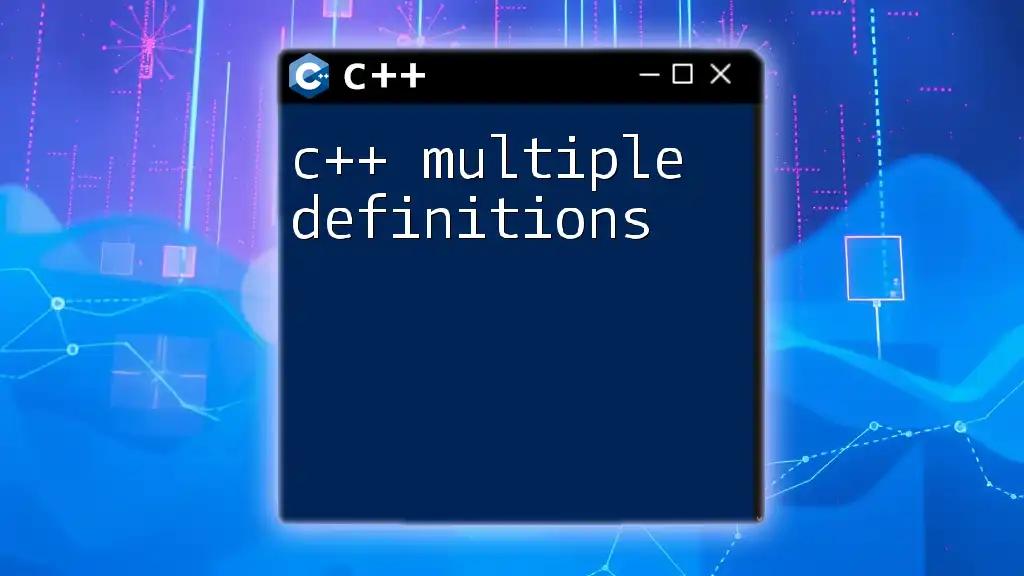
Common Methods for Returning Multiple Values in C++
Using Output Parameters
Defining Output Parameters
One common method of achieving c++ multiple return values is by using output parameters. This involves passing variables to a function by reference, allowing the function to modify these variables directly.
#include <iostream>
void calculateQuotientAndRemainder(int a, int b, int "ient, int &remainder) {
quotient = a / b;
remainder = a % b;
}
int main() {
int q, r;
calculateQuotientAndRemainder(10, 3, q, r);
std::cout << "Quotient: " << q << ", Remainder: " << r << std::endl;
return 0;
}
In this example, `calculateQuotientAndRemainder` takes integers `a` and `b` along with references to `quotient` and `remainder`. The function modifies these values before they are displayed in `main()`.
Advantages and Disadvantages
Using output parameters can be efficient, particularly if manipulating large data structures. However, they may make function signatures harder to read and track, reducing code maintainability if overused.
Returning Structs
Defining a Struct
Another approach is to define a struct that holds the multiple return values. Structs are an excellent way to package related data together succinctly.
#include <iostream>
struct DivisionResult {
int quotient;
int remainder;
};
DivisionResult calculateDivision(int a, int b) {
DivisionResult result;
result.quotient = a / b;
result.remainder = a % b;
return result;
}
int main() {
DivisionResult res = calculateDivision(10, 3);
std::cout << "Quotient: " << res.quotient << ", Remainder: " << res.remainder << std::endl;
return 0;
}
In this code, `DivisionResult` is a struct that encapsulates the results. The `calculateDivision` function returns an instance of this struct.
Using the Struct in Functions
The function call in `main()` creates a clear and strong connection between what is returned and its meaning. For related data, structs can enhance readability and structure.
Use cases for returning structs
Structs are particularly useful when returning multiple related values that logically belong together, such as coordinates in a 2D space or complex calculation results, making your code not just functional but also clean and understandable.
Using C++ Return Tuple
What is a Tuple?
C++ offers another robust method for returning multiple values through the use of std::tuple. A tuple is a fixed-size collection that can hold elements of different types, providing great flexibility.
Returning a Tuple from a Function
You can easily implement tuples in your functions like this:
#include <iostream>
#include <tuple>
std::tuple<int, int> calculateQuotientAndRemainder(int a, int b) {
return std::make_tuple(a / b, a % b);
}
int main() {
auto [q, r] = calculateQuotientAndRemainder(10, 3);
std::cout << "Quotient: " << q << ", Remainder: " << r << std::endl;
return 0;
}
Here, the `calculateQuotientAndRemainder` function returns a tuple containing both the quotient and the remainder. The use of structured binding in `main()` allows for a neat unpacking of the returned values.
When to Use Tuples
Tuples are best suited when you have a varying number of return values or when the return values are of different data types. They provide a high level of flexibility but can slightly sacrifice clarity if the tuple structure is not documented or understood by future developers.

Comparison of Methods for Returning Multiple Values
Output Parameters vs. Structs
Choosing between output parameters and structs boils down to readability and maintainability. Output parameters might clutter the function interface and complicate usage, while structs keep related data packaged together, which is easier to manage and understand.
Output Parameters vs. Tuples
While output parameters can be more straightforward for simple tasks, tuples allow for more flexible and concise return types. Choose tuples for varying return types and when you prefer simplicity in function calls.

Real-World Application and Examples
Using Multiple Return Values in a Program
Consider a function that calculates both the distance and the angle between two points in a coordinate system. Returning multiple values can efficiently capture the necessary information in one function call.
#include <iostream>
#include <cmath>
#include <tuple>
std::tuple<double, double> calculateDistanceAndAngle(double x1, double y1, double x2, double y2) {
double distance = std::sqrt(std::pow(x2 - x1, 2) + std::pow(y2 - y1, 2));
double angle = std::atan2(y2 - y1, x2 - x1) * (180.0 / M_PI);
return std::make_tuple(distance, angle);
}
int main() {
auto [dist, ang] = calculateDistanceAndAngle(0, 0, 3, 4);
std::cout << "Distance: " << dist << ", Angle: " << ang << " degrees" << std::endl;
return 0;
}
In this example, `calculateDistanceAndAngle` returns a tuple containing both distance and angle. This method provides clean and effective results with minimal overhead.
Benchmarking Performance of Different Methods
When considering performance, note that passing large data structures as reference outputs can be efficient. However, using tuples or structs may incur a slight overhead from object copying, especially when not returned optimally. Streamlining the return types and considering the larger impact on code efficiency is always essential.

Conclusion
Understanding how to manage c++ multiple return values can fundamentally change how you structure your code. By utilizing output parameters, structs, and tuples, developers can create more efficient, readable, and maintainable applications. Experiment with these examples, and explore which method suits your coding style best!

Additional Resources
For those looking to dig deeper into the world of C++, consider exploring resources like cppreference.com and various programming communities dedicated to advanced C++ features. These materials can broaden your knowledge and proficiency in C++ programming.

FAQs
What is the best way to return multiple values in C++? The best method varies by context. For related values, structs are often preferred; for flexibility, tuples may be the best choice.
Can I return more than two values effortlessly? Yes, functions can return any number of values through methods like structs or tuples, which can encapsulate multiple values cleanly.
Are there limitations when using tuples for returning values? One limitation of tuples is that they do not provide named access to their values, which could lead to confusion without proper documentation.
How does the choice of method affect readability and maintenance? Using structs often enhances readability for related data, while tuples provide flexibility. Balancing these considerations with project requirements is key.