In C++, you can retrieve a value from a `std::map` using the key with the `at()` or `[]` operators, which allows for efficient key-based access to the stored values.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap;
myMap["apple"] = 5;
myMap["banana"] = 10;
// Using at() method
std::cout << "Value for 'apple': " << myMap.at("apple") << std::endl;
// Using [] operator
std::cout << "Value for 'banana': " << myMap["banana"] << std::endl;
return 0;
}
Understanding C++ Maps
What is a C++ Map?
A C++ map is a part of the Standard Template Library (STL) that stores elements as key-value pairs. Each key must be unique, and each key is associated with exactly one value. Maps are implemented as binary search trees, which means they are automatically sorted by the keys. This structure allows for efficient searching, insertion, and deletion operations.
Maps are versatile and can store a variety of data types, such as integers, strings, and user-defined types, making them incredibly useful in a wide range of applications.
Why Use Maps?
Using maps comes with several advantages:
- Fast Lookup: Maps enable quick retrieval of values associated with a specific key, often achieving a time complexity of O(log n).
- Automatic Sorting: Maps automatically sort keys, making traversal and searching more intuitive.
- Memory Management: Maps manage memory efficiently, allowing for dynamic growth without the need for pre-allocation.
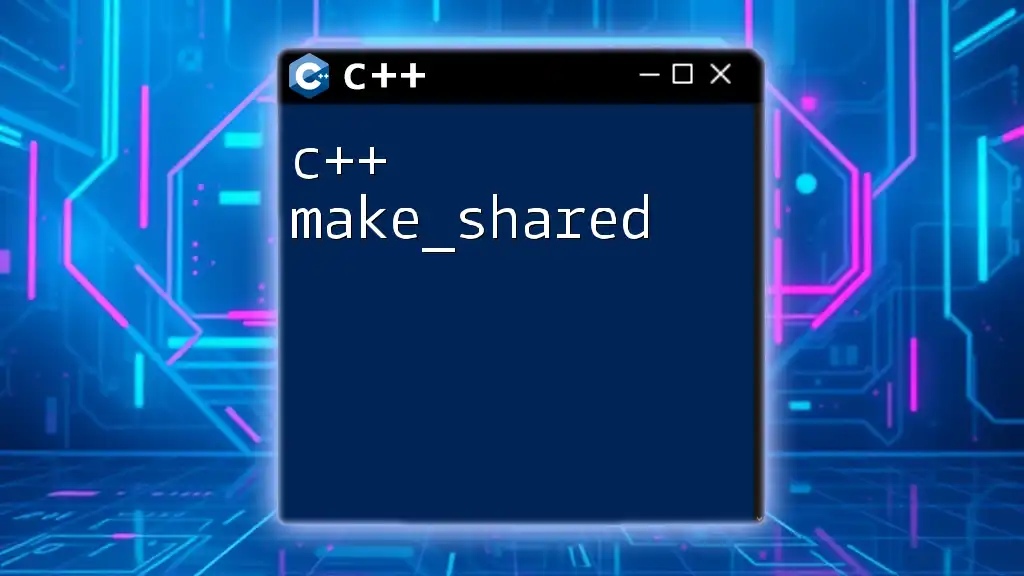
Getting Started with C++ Maps
How to Include Map Functionality
To use maps in C++, include the header file `<map>`:
#include <map>
You also need to operate within the `std` namespace unless you choose to prefix all items with `std::`.
Declaring a Map
Declaring a map requires specifying the data types for both the key and the value. The syntax is straightforward:
std::map<int, std::string> sampleMap;
In this example, `int` is the type of the key, while `std::string` is the type of the value.
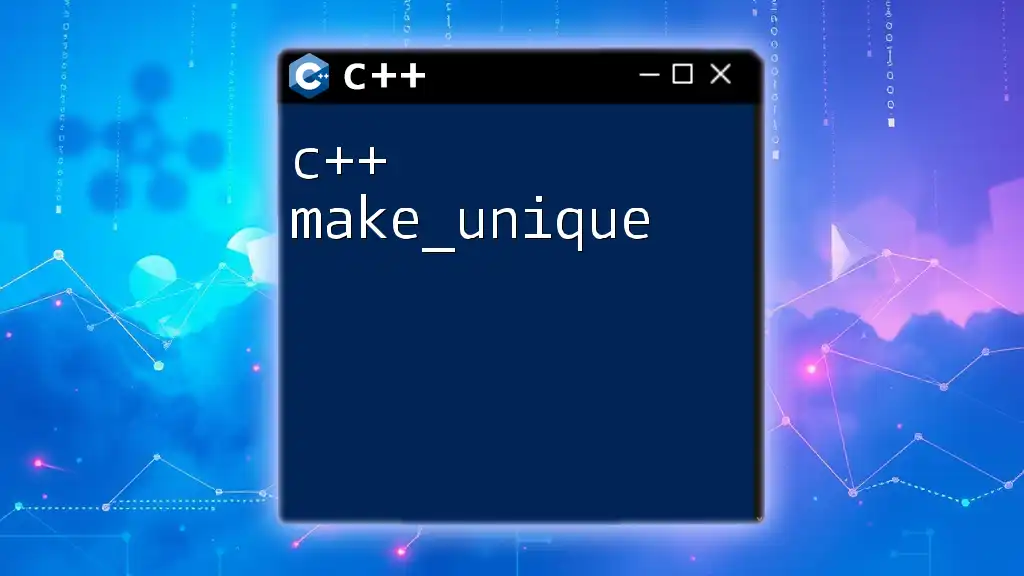
Adding Values to a Map
Inserting Key-Value Pairs
Inserting values into a map can be accomplished through multiple methods, prominently using `insert()` and the `operator[]`.
- Using `insert()`: You can insert values using the `insert()` method as follows:
sampleMap.insert(std::make_pair(1, "One"));
- Using `operator[]`: This method allows you to assign a value directly to a key. If the key doesn't already exist, it will be created.
sampleMap[2] = "Two";
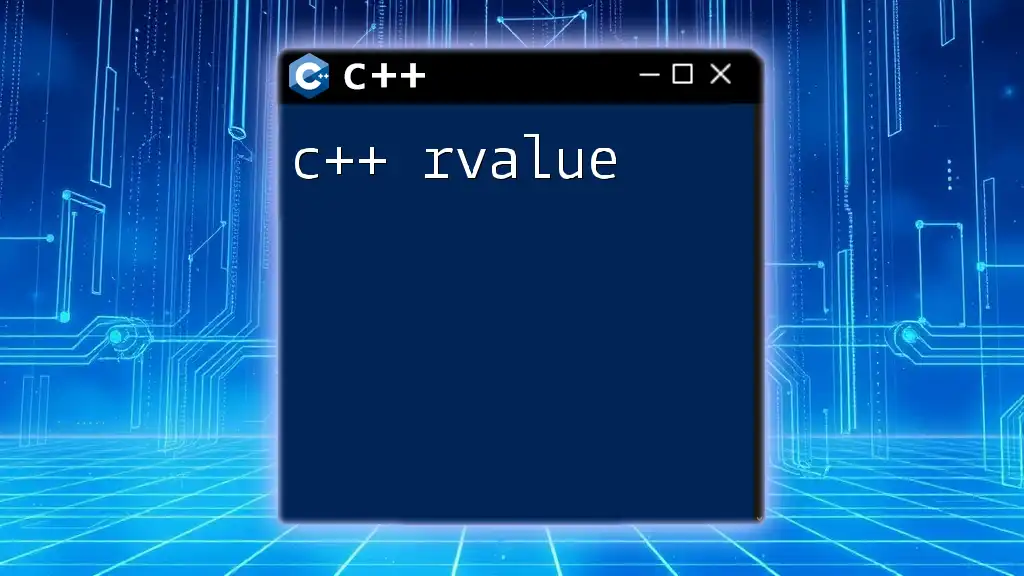
C++ Map Get Value by Key
Accessing Values
The primary method of accessing values in a map is by using the key associated with the value. Understanding this process is crucial for effectively managing data within maps.
One way to safely access values is by using the `at()` method. This method throws an exception if the key doesn’t exist:
std::string value = sampleMap.at(1); // Accessing a value safely
Using `operator[]` to Get Value
You can also retrieve values using the `operator[]`. It directly fetches the value associated with the specified key. However, if the key doesn't exist, `operator[]` will automatically add that key with a default value:
std::string value1 = sampleMap[1]; // Access using operator[]
This can lead to unintended consequences, especially if you fetch a value using a key that isn’t in the map.
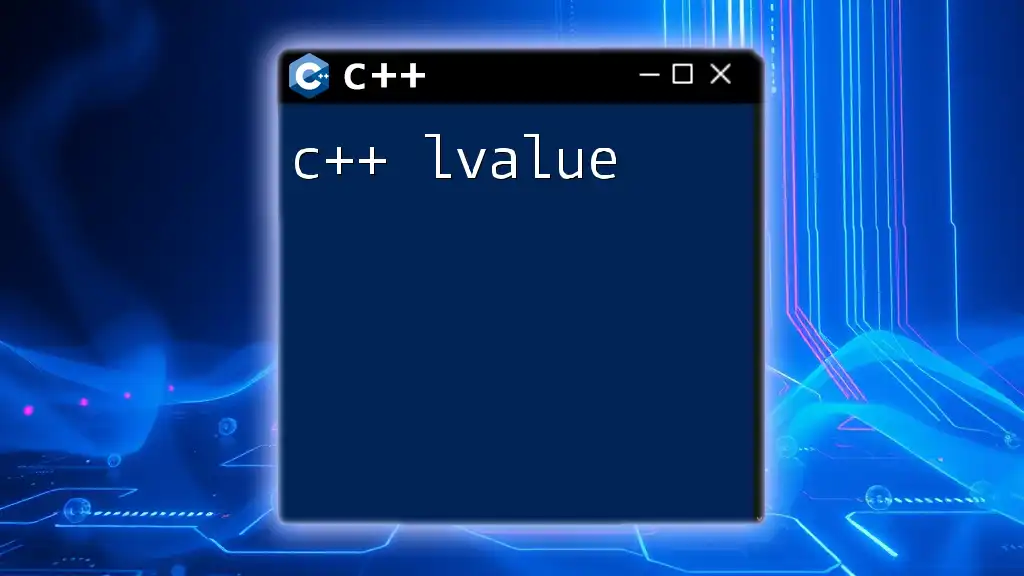
Handling Key Not Found Scenarios
Using `count()` Method
To avoid potential errors when accessing elements, you can use the `count()` method to check if a specific key exists in the map:
if (sampleMap.count(3)) {
std::string value2 = sampleMap[3];
} else {
std::cout << "Key not found." << std::endl;
}
This ensures that you only attempt to access keys that are present, preventing runtime errors.
Exception Handling with `at()`
When using the `at()` method, you can handle exceptions gracefully using try-catch blocks. This is particularly useful for managing scenarios where the key might not be present:
try {
std::string value3 = sampleMap.at(3);
} catch (const std::out_of_range& e) {
std::cerr << e.what() << std::endl; // Output the error message
}
This method provides a safer way to handle lookups, ensuring your program doesn’t crash unexpectedly.
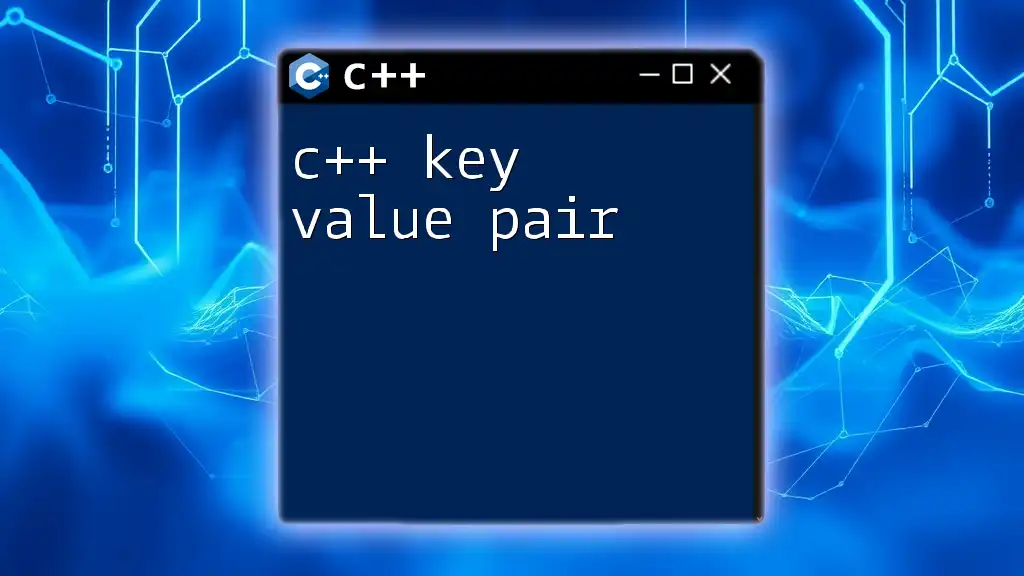
Iterating Through a Map
Using Iterators
To traverse a map's elements, you can use iterators. Iterators provide a powerful way to access elements efficiently:
for (auto it = sampleMap.begin(); it != sampleMap.end(); ++it) {
std::cout << it->first << " : " << it->second << std::endl;
}
Using Range-based For Loop
Another elegant approach to iterate through maps is by using range-based for loops. This method simplifies the syntax and enhances code readability:
for (const auto& pair : sampleMap) {
std::cout << pair.first << " : " << pair.second << std::endl;
}
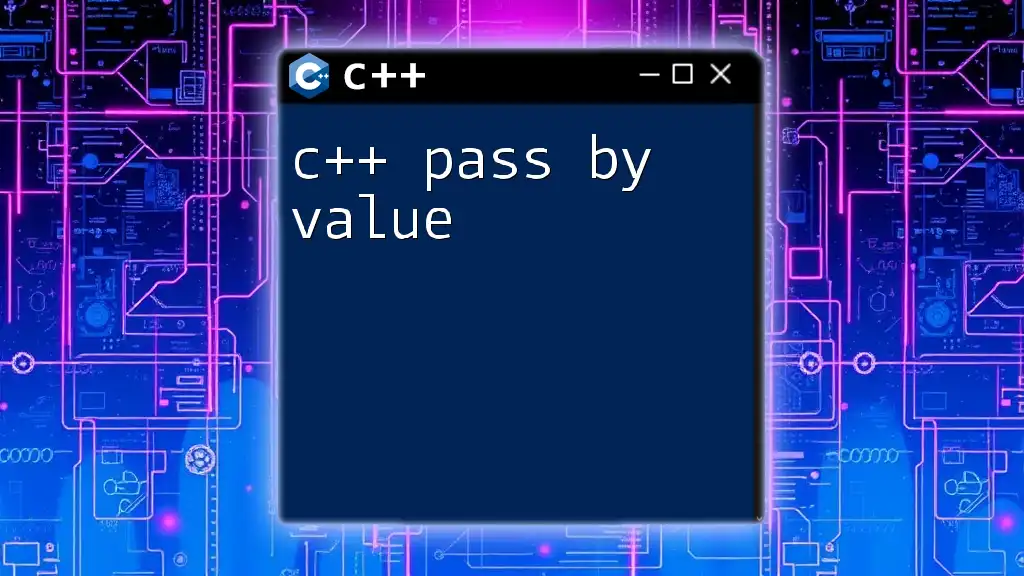
Best Practices for Using Maps in C++
Choosing the Right Map Type
C++ provides different types of maps, notably `std::map` and `std::unordered_map`.
- `std::map`: This is an ordered associative container that allows fast retrieval of data. It maintains elements in sorted order.
- `std::unordered_map`: This container uses hash tables for storage, providing faster access to values when order is not a concern.
Choosing between them involves understanding the specific needs of your application regarding data retrieval speed and order.
Memory Management Considerations
While maps are efficient in managing memory, it's essential to be mindful of the types of objects stored in maps. For example, storing large objects by value can lead to inefficient memory usage since each copy consumes additional resources. Instead, consider using smart pointers for complex objects.
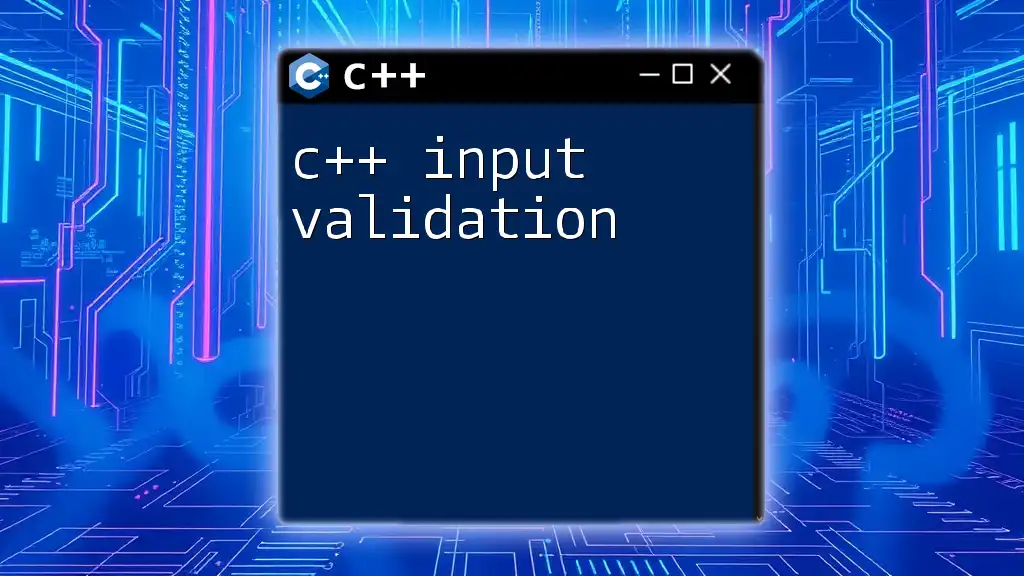
Conclusion
Understanding how to effectively use the C++ map to get values is a fundamental skill that enhances your programming toolkit. Maps are incredibly versatile, enabling efficient data management through key-value associations. Mastering the nuances of accessing, modifying, and iterating over maps will not only improve your code efficiency but will also prepare you for tackling more complex data structures in C++.
By utilizing the techniques outlined above, you can confidently navigate C++ maps and leverage their benefits in your programming endeavors.