In C++, an rvalue is a temporary object or a value that does not have a persistent memory address and typically appears on the right side of an assignment, often utilized in move semantics and perfect forwarding.
Here’s a simple example of an rvalue in use:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.push_back(42); // 42 is an rvalue
std::cout << "The value in vec is: " << vec[0] << std::endl;
return 0;
}
Understanding C++ Rvalue
Overview of C++ Values
In C++, values can be classified into two main categories: lvalues and rvalues. Understanding these categories is crucial for writing efficient code. While lvalues represent objects that occupy identifiable locations in memory and can be referenced, rvalues are temporary objects that do not persist beyond the expression that uses them.
What is an Rvalue?
An rvalue is defined as a temporary object that is created during the evaluation of an expression. Rvalues typically represent data that cannot be assigned a permanent address because they exist only for a short duration. Key characteristics include:
- They don’t have identifiable memory locations.
- They are often used as right-hand values in assignments.
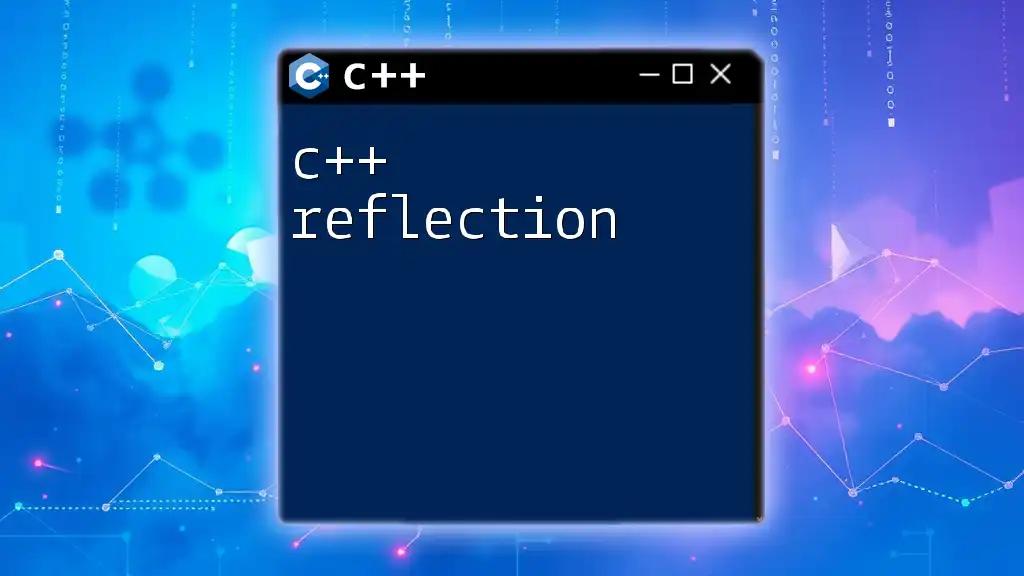
Rvalues vs Lvalues
Defining Lvalues
An lvalue denotes an object that occupies a specific location in memory. This allows lvalues to have identifiable addresses. For instance:
int x = 10; // x is an lvalue
In this example, `x` can be assigned a new value or referenced later in the code.
Comparing Rvalues and Lvalues
Characteristic | Lvalue | Rvalue |
---|---|---|
Memory Address | Has an identifiable address | Temporary, no persistent address |
Modifiability | Can be assigned a new value | Cannot be assigned |
Usage Context | Typically on the left side of assignment | Usually on the right side |
Examples
Consider the following code example:
int y = 10; // y is an lvalue
int&& z = 20; // 20 is an rvalue
In this case, `y` is an lvalue, whereas `20` represents an rvalue.
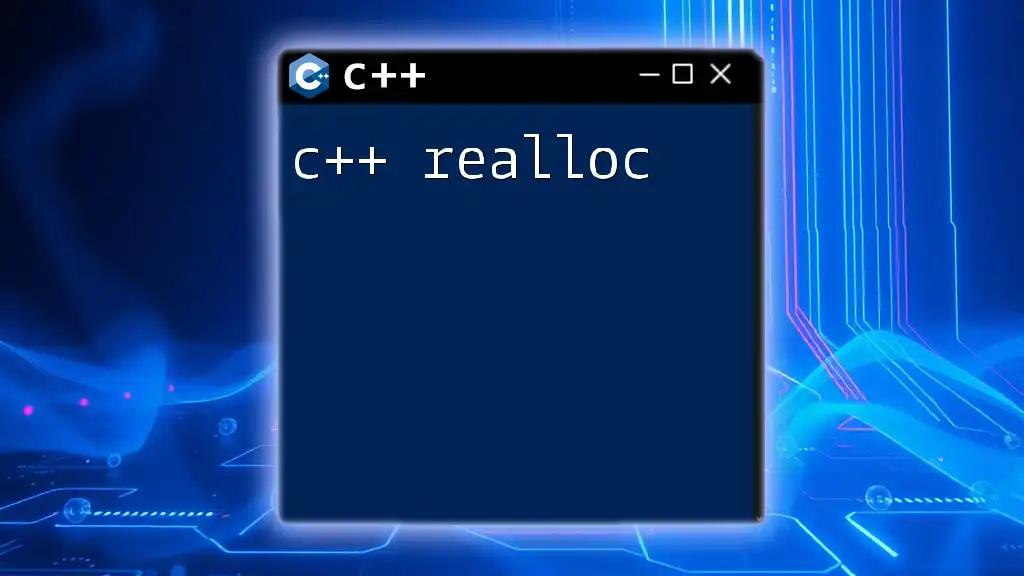
The Role of Rvalues in C++
Temporary Objects
Rvalues often take the form of temporary objects, which exist only during the execution of an expression. They are created when the compiler needs to evaluate expressions that do not have a persistent result. Understanding temporary objects and their lifecycle is vital because they often optimize memory usage and performance, especially in complex calculations.
Rvalues in Expressions
Rvalues are integral to various expressions in C++. They are prominently used during arithmetic operations or as return values from functions that generate a temporary result.
Code Examples
Here’s a simple demonstration of rvalues in use:
int add(int a, int b) {
return a + b; // The return value is an rvalue
}
int main() {
int result = add(5, 10); // add(5, 10) is an rvalue
}
In this scenario, the return value of the `add` function is an rvalue used for assignment to `result`.
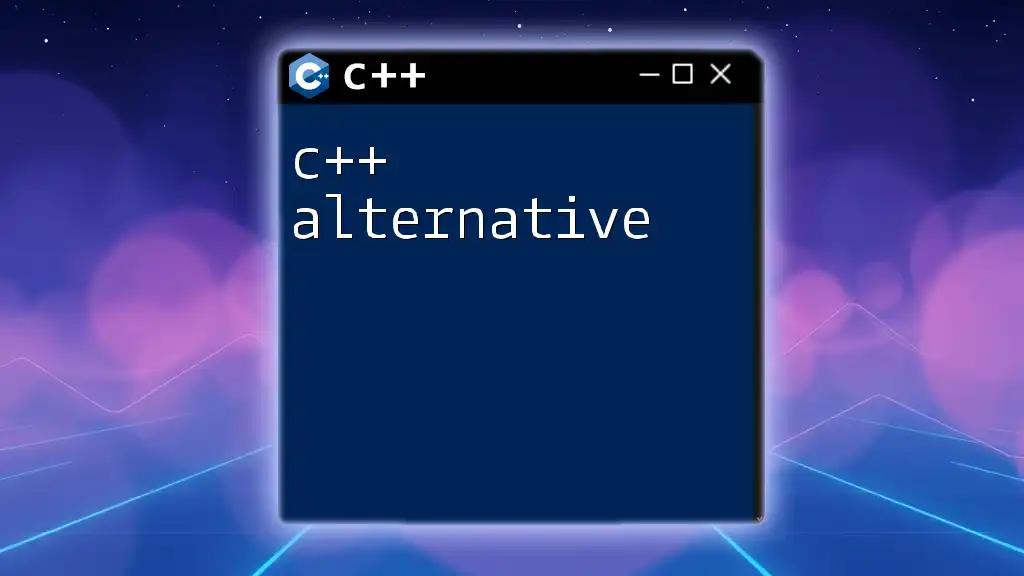
Rvalue References
Introduction to Rvalue References
Rvalue references are a recent addition to C++, introduced in C++11. They allow developers to bind rvalues to references using the `&&` syntax. This possess the ability to distinguish between lvalues and rvalues, making it possible to optimize resource management effectively.
Moving Semantics
Move semantics enables efficient transfer of resources from one object to another, especially for temporary objects. Instead of copying resources, which can be expensive, move semantics transfers ownership, significantly improving performance.
For example, consider the following class implementation:
class MyClass {
public:
MyClass() { /* resource acquisition */ }
MyClass(MyClass&& other) noexcept {
// Transfer ownership of resources
// other.resource = nullptr; // Prevent double deletion if needed
}
};
This class demonstrates how to implement rvalue references within the constructor to handle resource transfer efficiently.
Example of Move Semantics in Action
By utilizing move semantics, you reduce the overhead of unnecessary copies. The transfer of resources means that objects can be moved instead of copied, enhancing speed and performance.

Practical Use Cases of Rvalues
Utilizing Rvalues in Functions
In function overloads, you can design functions that gracefully accept rvalues, allowing for more flexible programming patterns.
void Process(MyClass&& obj) {
// Process obj directly, optimizing for speed
}
Such functions leverage rvalue references to enable a more efficient way to pass large objects without unnecessary copying, thus enhancing performance.
Resource Management with Rvalues
Using rvalues can lead to better resource management. By correctly implementing move semantics, C++ developers can drastically improve both memory efficiency and execution speed, particularly in standard template library (STL) containers like `std::vector` or `std::string`.
std::vector<MyClass> vec;
vec.push_back(MyClass()); // Temporary rvalue, efficiently moved

Advanced Rvalue Concepts
Perfect Forwarding
Perfect forwarding facilitates the use of rvalue references in function templates. It allows you to write generic functions that forward the arguments to another function while preserving their value category (lvalue or rvalue).
Example of perfect forwarding:
template<typename T>
void ForwardToFunction(T&& arg) {
RealFunction(std::forward<T>(arg));
}
In this example, `arg` is perfectly forwarded, maintaining its status as either an lvalue or rvalue as appropriate.
Rvalue Qualifiers in C++17 and Upwards
With C++17 and later, new features have been introduced around rvalues, further enhancing their utility. The `std::optional` and `std::variant` types are excellent examples that help manage resources more efficiently. Understanding these features can help in writing cleaner and more efficient modern C++ code.
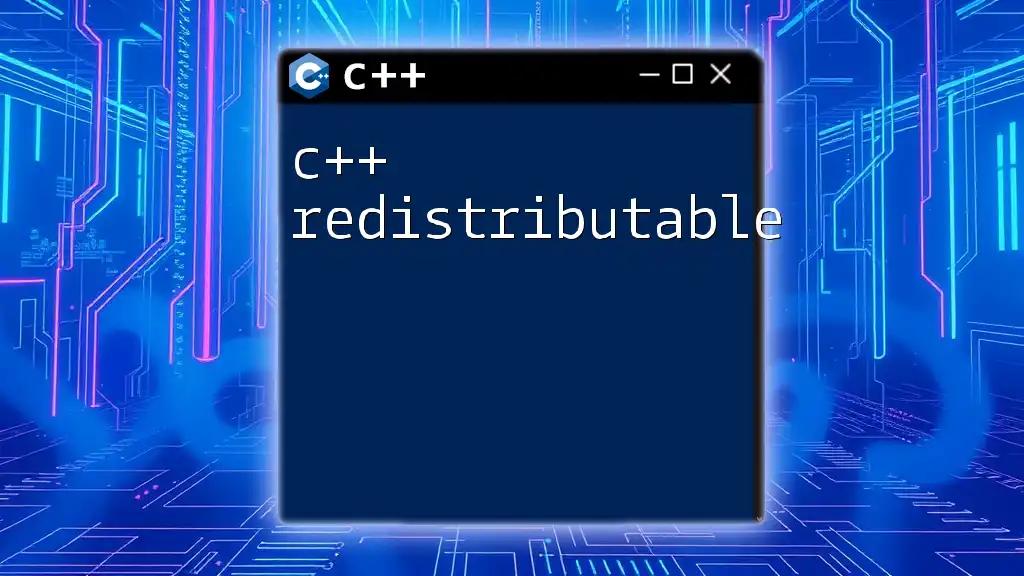
Common Patterns with Rvalues
Idiomatic Usage
Using rvalues effectively requires a good understanding of best practices. Always prefer rvalue references for classes that manage large resources or dynamically allocated memory, especially when implementing classes with copy and move constructors.
Common Pitfalls
A prevalent mistake among developers is failing to implement move constructors correctly, leading to resource leaks or double deletions. Carefully managing resources and understanding the timing of object lifetimes can help mitigate these issues.
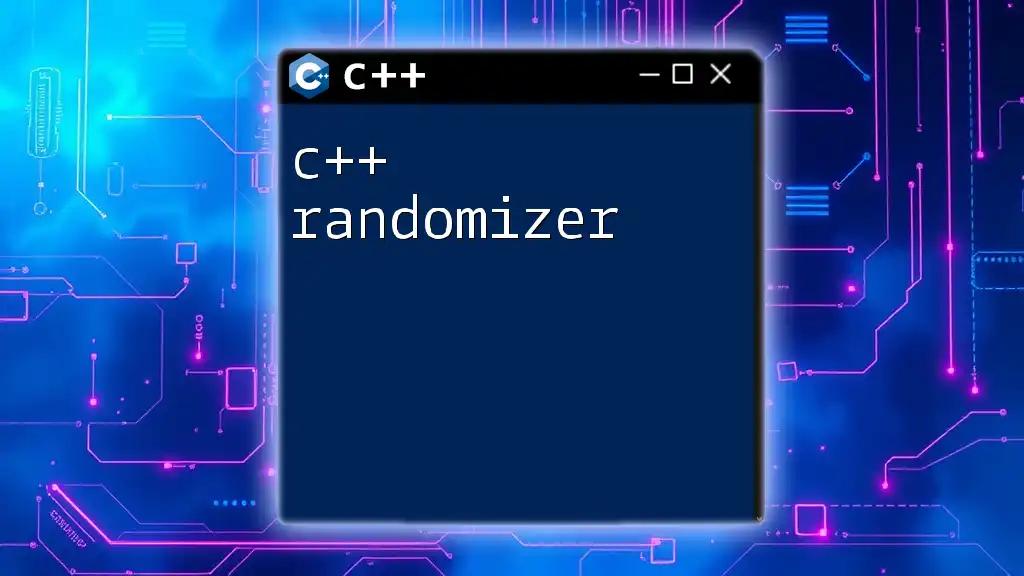
Conclusion
Understanding C++ rvalue concepts is not just an academic exercise; it’s essential for writing high-performance applications. By leveraging rvalue references and move semantics, developers can optimize memory usage and performance. The implications for modern C++ development are substantial, making this knowledge crucial for fresher developers and seasoned professionals alike.
Resources for Further Learning
To deepen your understanding, consider reading literature dedicated to modern C++, and exploring online resources that provide hands-on examples. Courses focused on C++ best practices, rvalue references, and move semantics can also significantly enhance your skills.
Call to Action
Start experimenting with rvalue references and move semantics in your coding projects. Embrace this powerful feature of C++ to achieve cleaner, faster, and more efficient code. Stay tuned for more articles exploring deeper C++ concepts!