In C++, a reference (`ref`) is an alias for another variable, allowing you to directly access and manipulate the original variable's value without copying it.
Here's a simple example:
#include <iostream>
using namespace std;
int main() {
int x = 10;
int &ref = x; // ref is a reference to x
ref = 20; // changes x to 20
cout << x; // Output: 20
return 0;
}
Introduction to C++ References
C++ references, often denoted as c++ ref, are a powerful feature that allows developers to create aliases for variables. They provide a way to access and manipulate existing variables without creating copies, thus enhancing performance and code readability. References are particularly beneficial in numerous programming scenarios, making them essential for any C++ developer.
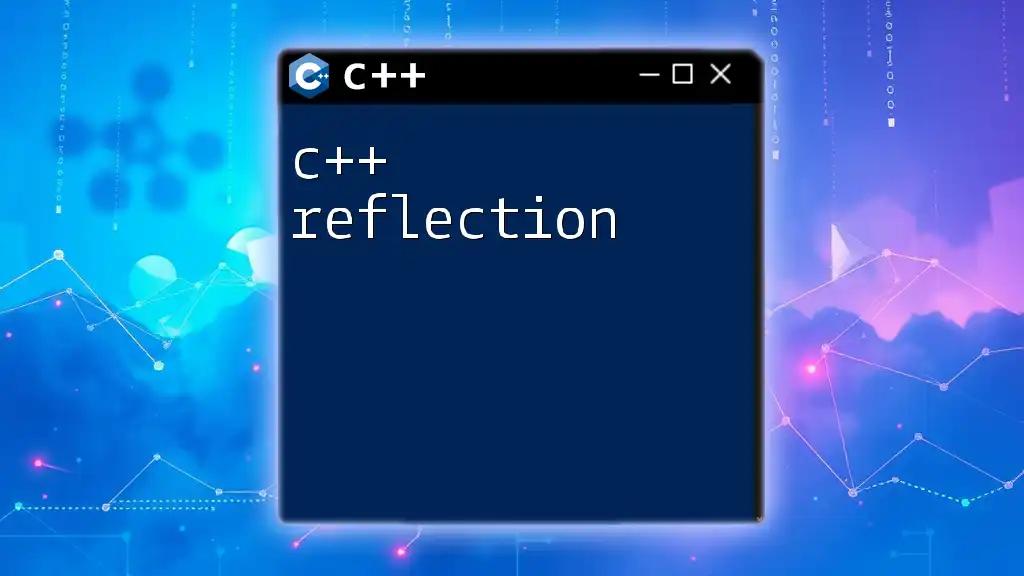
Understanding References in C++
What is a Reference?
A reference in C++ is essentially an alias for an existing variable. Declaring a reference requires the use of the ampersand symbol (`&`), which links the reference to the original variable.
For example, consider the following code snippet:
int original = 10;
int &ref = original; // ref is a reference to original
In this example, `ref` acts as a direct link to `original`. Any modification to `ref` will affect `original`, solidifying the notion that a reference does not occupy additional memory for a separate variable.
Differences Between References and Pointers
While both references and pointers can point to the same memory location, they possess critical differences. Here are key characteristics:
- Initialization: A reference must be initialized upon declaration, whereas a pointer can be declared without an initial value, potentially leading to null pointer dereferencing.
- Syntax: Accessing a reference is more intuitive as it uses a simple variable name, unlike pointers, which require dereferencing operators (`*` and `&`).
- Non-null: A reference cannot be null, providing a layer of safety that pointers don’t inherently possess.
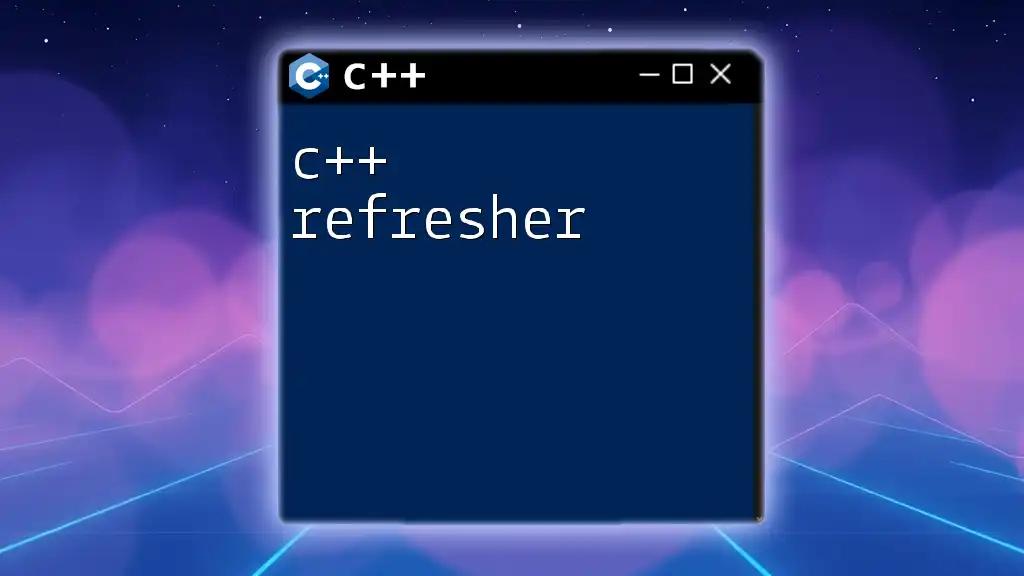
Types of References
Lvalue References
Lvalue references are the default type of reference in C++. They allow you to refer to variables that have a persistent memory address. Here’s an example:
int var = 5;
int &lref = var; // lvalue reference
In this instance, `lref` is an alias for `var`. Any changes made to `lref` will directly alter `var`.
Rvalue References
Introduced in C++11, rvalue references allow for more advanced memory management and optimization techniques, particularly move semantics. An rvalue reference can bind to temporary objects (rvalues), allowing developers to efficiently move resources. Here’s an example:
int &&rref = 5; // rvalue reference
This capability enables a more efficient transfer of resources during function calls instead of copying, which can be beneficial in resource-intensive applications.
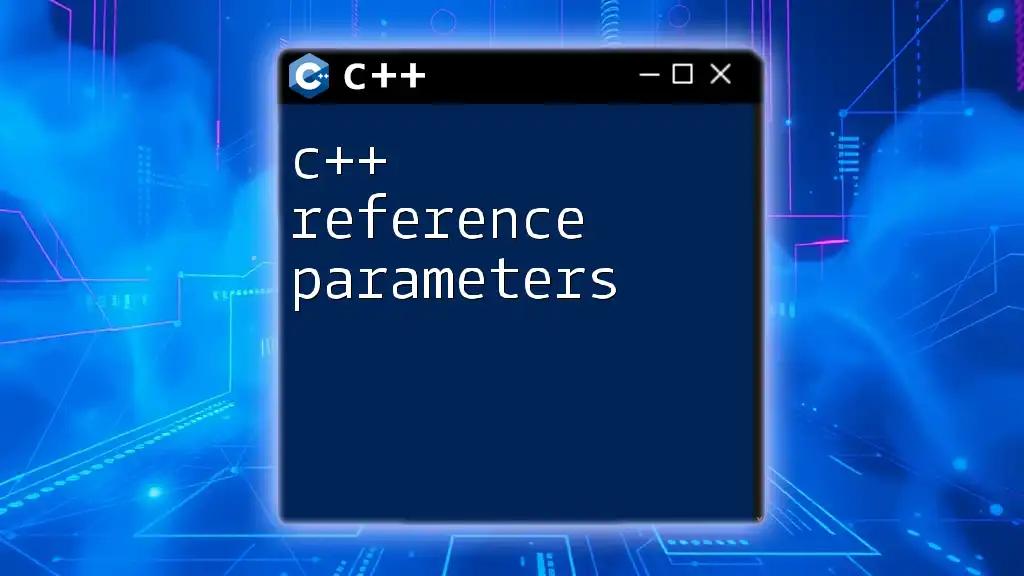
How to Use References Effectively
Using References as Function Parameters
One of the most common uses of references is in function parameters. By passing by reference instead of by value, you can avoid unnecessary copies of large objects. Here’s an example:
void increment(int &num) {
num += 1;
}
In this scenario, calling `increment(original)` modifies `original` directly, enhancing performance, especially in situations involving large data structures such as classes or structs.
Return Types and References
C++ also supports returning references from functions. This allows functions to provide direct access to variable data, eliminating the need for copies. Consider the following code:
int& findMax(int &a, int &b) {
return (a > b) ? a : b;
}
This function returns a reference to the larger of the two integer references, allowing the caller to modify the returned value directly. However, caution is required to avoid returning references to local variables.
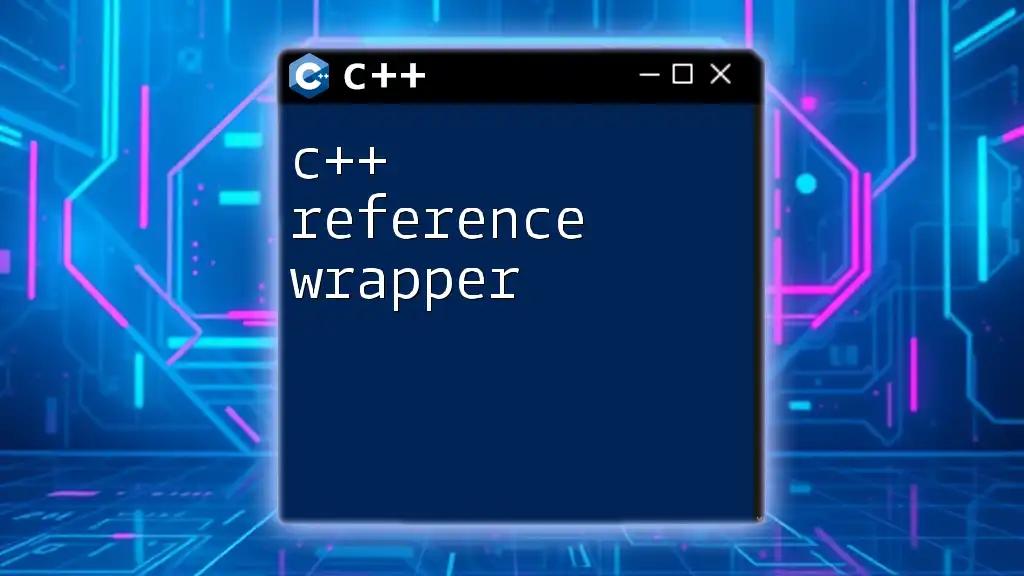
References in Object-Oriented Programming
Member Functions and References
In the realm of object-oriented programming, references can also simplify interactions with class member functions. By using references for method parameters, you can enhance efficiency and clarity.
Here’s a simple example:
class Point {
public:
void move(Point &p) { x += p.x; y += p.y; }
private:
int x, y;
};
Here, the `move` method alters the current object's position based on another `Point` object. Using a reference eliminates the overhead of copying the `Point` object, leading to more efficient code.
Copy Constructor and Assignment Operator
When defining custom types, implementing a copy constructor using references is crucial to proper resource management. Here’s how you can define a copy constructor:
class MyClass {
public:
MyClass(const MyClass &obj) {
// Perform deep copy
} // Copy constructor
};
This constructor utilizes a constant lvalue reference to ensure that the original object isn’t modified during copying.
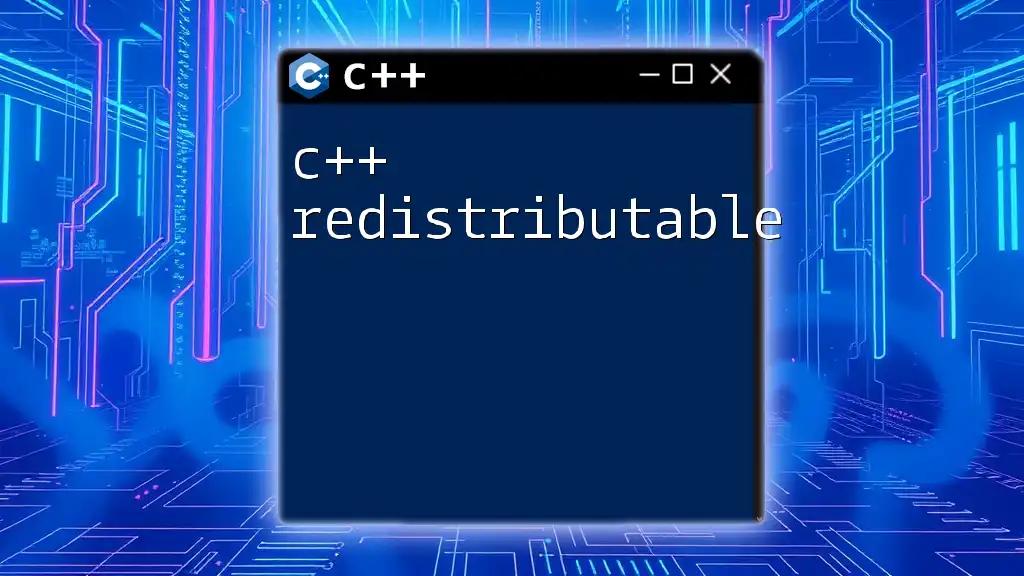
Best Practices with C++ References
When to Prefer References over Pointers
Choosing between references and pointers can significantly affect code readability and performance. Here are some cues for when to use references:
- Use a reference when you want to ensure the object being referenced will always exist.
- Prefer references for passing large objects to functions.
- Use pointers when dynamic memory allocation or the possibility of null references is required.
Common Pitfalls
While references are generally safe, developers should be mindful of potential pitfalls, particularly with dangling references. A dangling reference occurs when a reference points to a local variable that has gone out of scope. Avoiding this situation is critical to preventing undefined behavior in C++ applications.
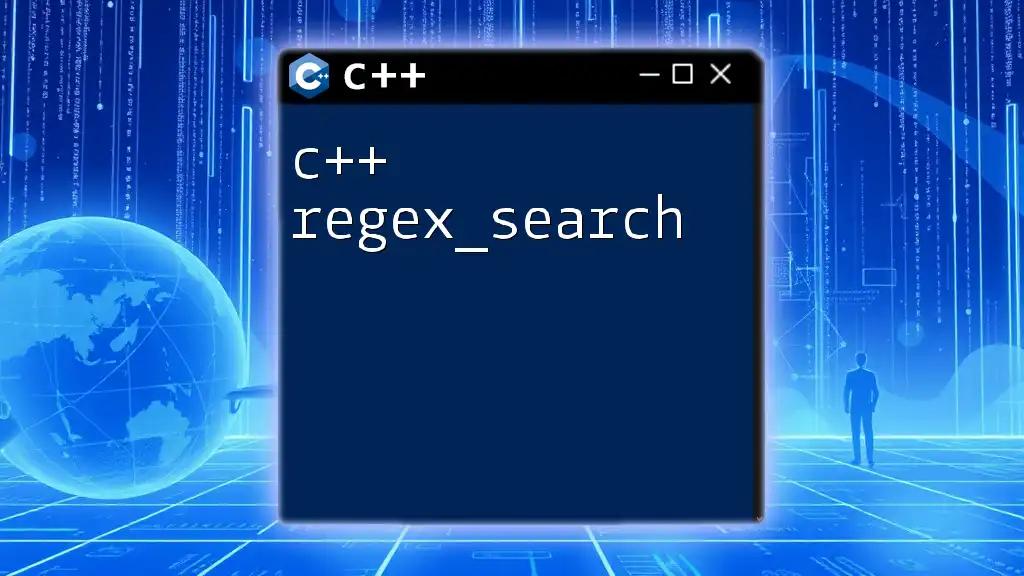
Advanced Topics
Move Semantics and Rvalue References
Understanding move semantics is pivotal for efficient resource management in modern C++. Rvalue references facilitate moving resources rather than copying, which boosts performance when handling temporary objects. For instance:
class A {
public:
A(A&& other) noexcept; // Move constructor
};
By leveraging move semantics, developers can optimize their applications, significantly reducing overhead during resource transfers.
Function Templates and Reference Collapsing
In templates, reference collapsing rules dictate how references interact. Understanding how to use forwarding references enhances the versatility of your template functions. For instance:
template <typename T>
void func(T &¶m); // Forwarding references
This technique allows the function to accept both lvalues and rvalues, granting greater flexibility in function design.
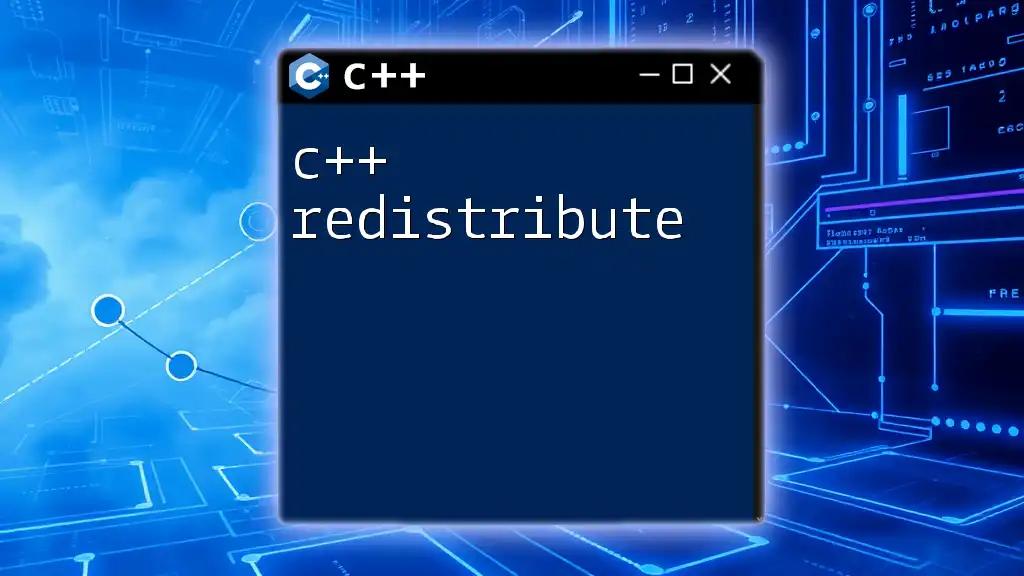
Conclusion
In summary, c++ ref plays a crucial role in the C++ programming language, enabling developers to write more efficient, clear, and maintainable code. By mastering references, you can optimize function performance, manage resources more effectively, and understand the nuances of modern C++ programming practices. As you continue your journey in C++, it's essential to deepen your understanding of references and apply them judiciously throughout your coding endeavors.
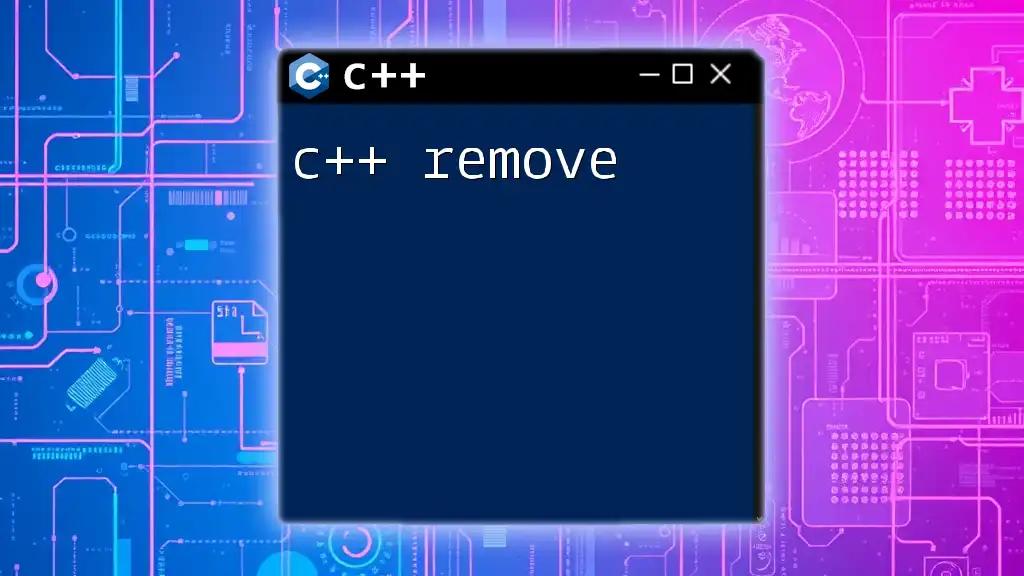
FAQs
What are the main advantages of using references in C++? References provide a safer alternative to pointers, eliminate the overhead of copying objects, and enhance code readability.
Can a reference be null? No, a reference must always refer to a valid object, making them inherently safer than pointers.
How do references fit into the bigger picture of C++ programming? References help reduce memory usage, improve performance, and simplify function interfaces, making them indispensable in modern C++ development.