The `std::remove_if` function in C++ is used to remove elements from a container that satisfy a given predicate, effectively shifting them to the end of the container without actually altering its size.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5, 6};
nums.erase(std::remove_if(nums.begin(), nums.end(), [](int n) { return n % 2 == 0; }), nums.end());
for (int n : nums) {
std::cout << n << " ";
}
return 0;
}
Understanding the Basics of c++ remove_if
What is remove_if in C++?
The `remove_if` function is a part of the Standard Template Library (STL) in C++. It is used to remove elements from a collection based on a specified condition defined by a predicate function. While it doesn't actually erase elements from the container, it rearranges the elements so that the elements to be removed are placed at the end, and it returns an iterator pointing to the new end of the range.
Syntax
The syntax for `remove_if` is straightforward:
#include <algorithm>
template <class ForwardIterator, class UnaryPredicate>
ForwardIterator remove_if(ForwardIterator first, ForwardIterator last, UnaryPredicate p);
This indicates that `remove_if` can operate on any container that supports forward iteration.
Parameters
- ForwardIterator first: This parameter defines the start of the sequence to be processed.
- ForwardIterator last: This indicates the end of the sequence.
- UnaryPredicate p: A condition (function or lambda) that specifies which elements should be removed based on their 'truthiness' when passed to this function.
Return Value
The function returns a ForwardIterator that marks the end of the modified range. The elements that are considered "removed" are now in the latter part of the range, and you should use the `erase` method of the container to actually remove them.
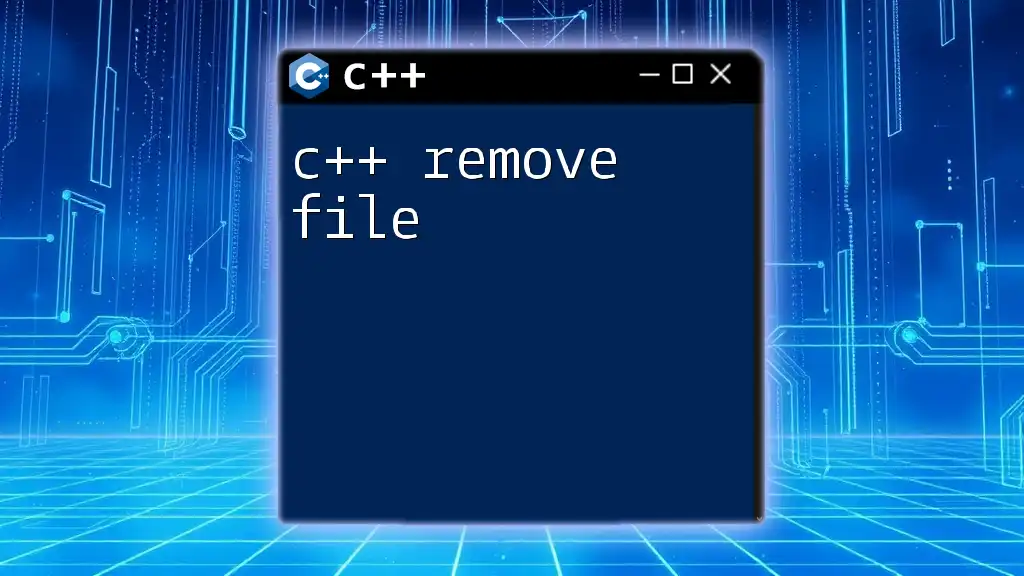
How c++ remove_if Works with Examples
Example 1: Removing Even Numbers
Let’s consider a practical example of how to use `remove_if` to remove even numbers from a vector.
#include <iostream>
#include <vector>
#include <algorithm>
bool isEven(int n) { return n % 2 == 0; }
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
auto newEnd = std::remove_if(numbers.begin(), numbers.end(), isEven);
numbers.erase(newEnd, numbers.end());
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this code snippet:
- We have a vector `numbers` containing integers.
- The `isEven` function returns `true` for even numbers.
- The `remove_if` algorithm processes this vector and moves all even numbers to the end.
- After that, we call `erase` to actually remove them from the vector.
- The output will be `1 3 5`, demonstrating that the even numbers have been effectively removed.
Example 2: Removing Strings Based on Length
Another example could involve strings where we want to remove words longer than three characters.
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
bool isLongerThanThree(const std::string& str) { return str.length() > 3; }
int main() {
std::vector<std::string> words = {"one", "two", "three", "four", "five"};
auto newEnd = std::remove_if(words.begin(), words.end(), isLongerThanThree);
words.erase(newEnd, words.end());
for (const auto& word : words) {
std::cout << word << " ";
}
return 0;
}
In this case:
- The `words` vector contains strings, and we aim to remove any string longer than three characters.
- The `isLongerThanThree` function acts as our predicate to guide `remove_if`.
- The final output will be `one two three`, showcasing the effectiveness of filtering.
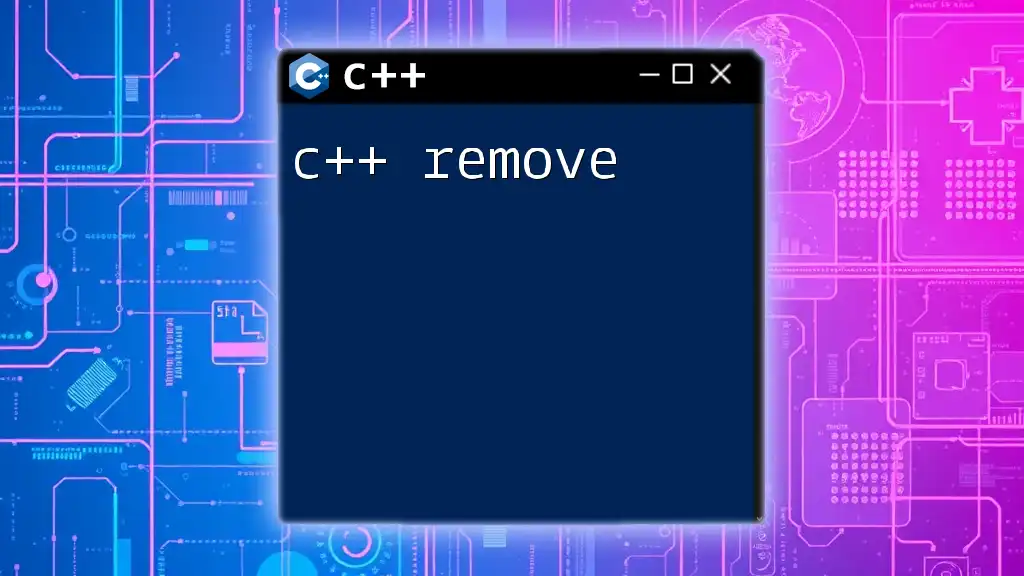
Practical Use Cases for c++ remove_if
Filtering Data in Applications
The `remove_if` function is highly useful in various data processing scenarios. For instance, it can be applied to filter user inputs in applications, ensuring only valid data remains in a data structure for further processing.
Enhancing Code Readability and Maintainability
Using `remove_if` can lead to cleaner and more maintainable code. Instead of handling element removal in intricate loops, you leverage the STL algorithm, thereby enhancing the clarity of your intentions to future readers of your code.
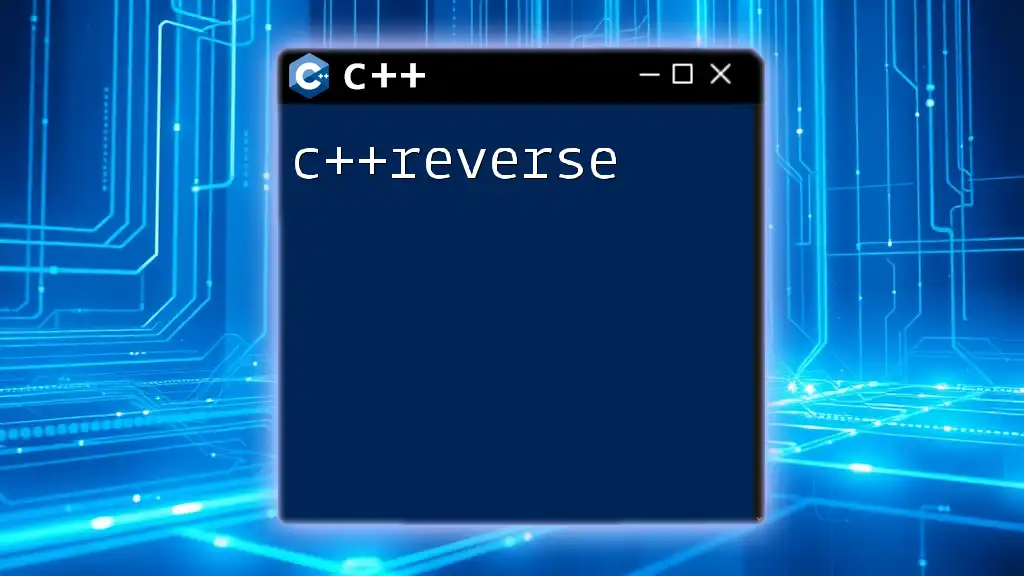
Common Mistakes and How to Avoid Them
Forgetting to Erase Elements
One of the most common pitfalls when using `remove_if` is neglecting to call `erase` afterwards. Since `remove_if` merely rearranges the elements, failing to invoke `erase` will leave the elements "removed" in place, making them accessible and potentially leading to logical errors in your program.
Inefficient Predicate Functions
Ensure that your predicate functions are efficient. Performance could significantly degrade if you use computationally expensive predicates, especially on larger datasets. Aim for simple and efficient checks to keep your code running smoothly.
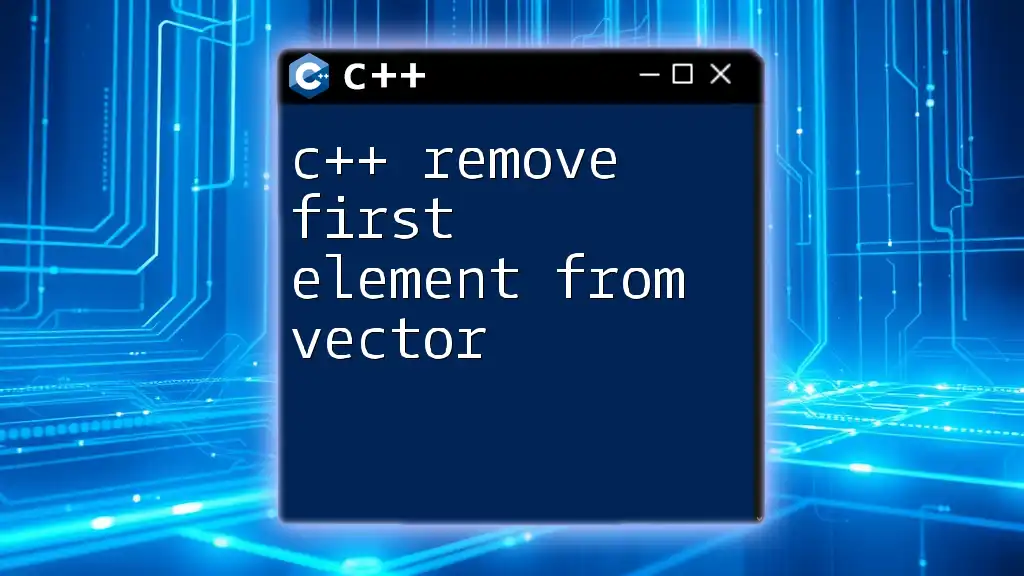
Tips and Best Practices
Using Lambda Functions
Using lambda expressions with `remove_if` can make your code more concise and readable. Here’s a brief example:
std::vector<int> numbers = {1, 2, 3, 4, 5, 6};
numbers.erase(std::remove_if(numbers.begin(), numbers.end(), [](int n) { return n % 2 == 0; }), numbers.end());
This eliminates the need for a separate function declaration and directly expresses the intended condition.
Performance Considerations
When employing `remove_if`, consider the performance implications when operating on large datasets. While `remove_if` performs in linear time, calling `erase` can potentially be costly due to the nature of the underlying container. Use the operations judiciously, and preprocess data when advantageous to optimize performance.
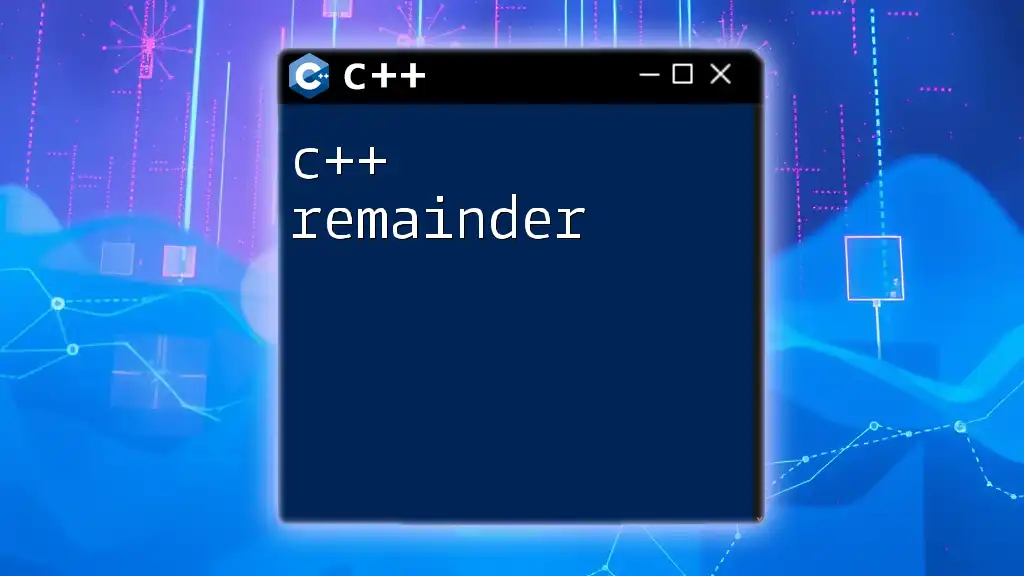
Conclusion
By now, you should have a robust understanding of how to utilize `c++ remove_if` effectively. From the syntax and working with examples to practical applications and common pitfalls, leveraging this STL algorithm can significantly enhance your coding efficiency and clarity.
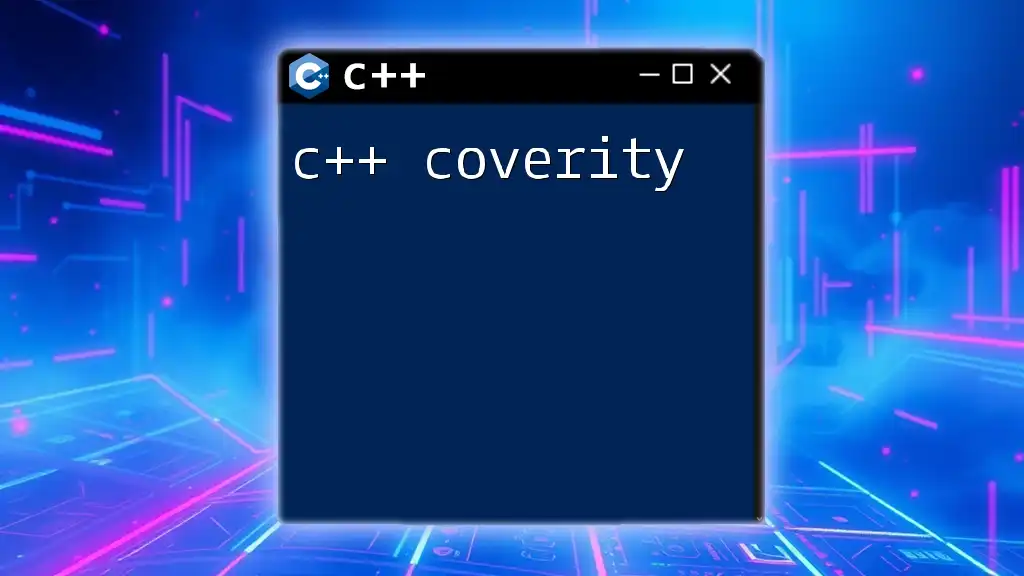
Additional Resources
For further exploration:
- Refer to the official C++ documentation for STL algorithms.
- Seek out literature that delves deeper into STL usages and best practices to refine your understanding and implementation skills.