`memcpy_s` is a safe version of the `memcpy` function that copies a specified number of bytes from a source memory block to a destination memory block while ensuring that buffer overflows are prevented.
#include <string.h>
#include <stdio.h>
int main() {
char src[] = "Hello, World!";
char dest[50];
errno_t err = memcpy_s(dest, sizeof(dest), src, strlen(src) + 1);
if (err == 0) {
printf("Copied string: %s\n", dest);
} else {
printf("Error copying string\n");
}
return 0;
}
What is memcpy_s?
`memcpy_s` is a secure memory copy function introduced in the C Standard Library, specifically designed to enhance the safety of memory operations. It is part of the bounds-checking interfaces in the N2479 proposal for the C programming language. The primary goal of `memcpy_s` is to prevent common programming errors such as buffer overflows, offering a safeguard against corrupting adjacent memory locations.
Importance of Safety in Memory Operations
When performing memory operations, the risk of buffer overflows can lead to severe vulnerabilities, such as crashes or security exploits. Traditional functions like `memcpy` do not perform bounds checking, which can result in catastrophic failures if a programmer mistakenly writes outside the allocated memory. Using `memcpy_s` gives developers a reliable way to ensure their code does not inadvertently cause memory corruption.
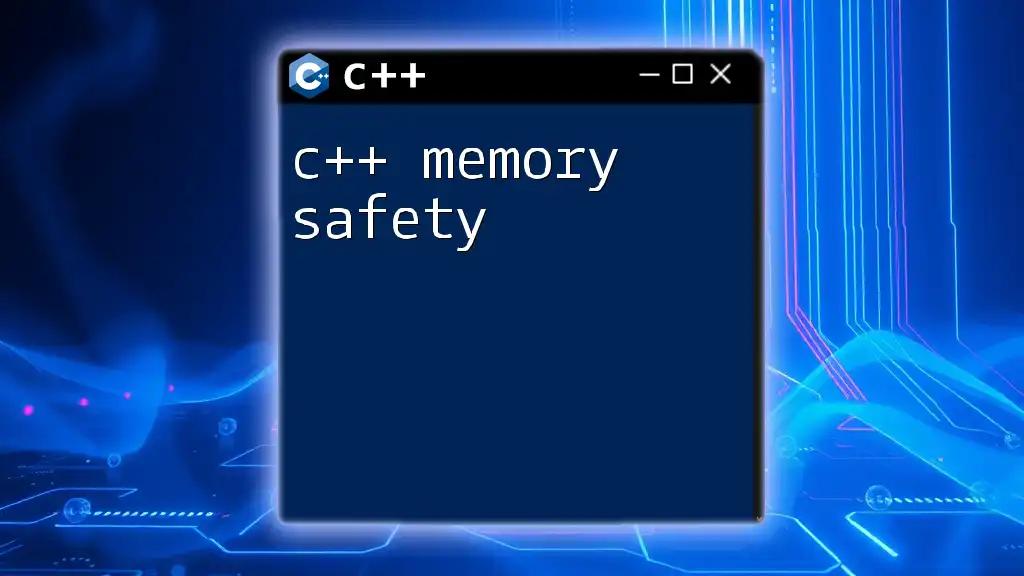
Syntax of memcpy_s
General Syntax
The syntax of `memcpy_s` is straightforward but requires attention to detail. Here is the general format:
errno_t memcpy_s(void *dest, rsize_t destsz, const void *src, rsize_t count);
This function takes four parameters:
- dest: A pointer to the destination memory buffer where data will be copied.
- destsz: The size of the destination buffer in bytes. This is crucial as it allows the function to check whether the buffer is large enough to hold the data.
- src: A pointer to the source memory buffer from which data will be copied.
- count: The number of bytes to copy from the source buffer.
Example of Syntax
Here's a simple example demonstrating the syntax of `memcpy_s`:
#include <cstdio>
#include <cstdlib>
#include <cstring>
void safeMemoryCopy() {
char dest[10];
const char* src = "Hello";
errno_t err = memcpy_s(dest, sizeof(dest), src, strlen(src) + 1);
// Additional code goes here
}
In this snippet, we define a destination buffer `dest` and a source string `src`. The function call attempts to safely copy the contents of `src` to `dest`, ensuring that no overflow occurs.
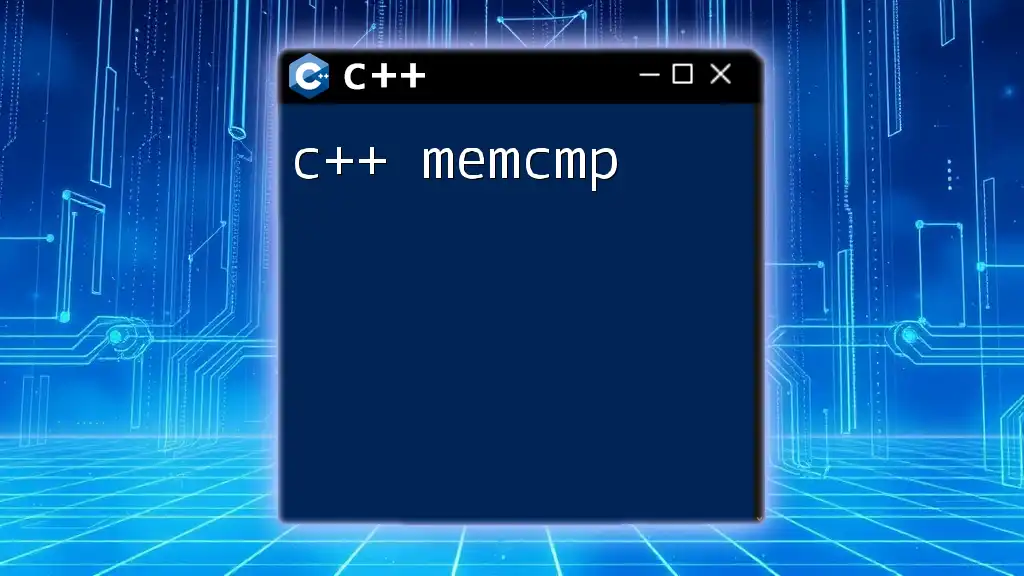
Key Features of memcpy_s
Bounds Checking
One of the standout features of `memcpy_s` is its built-in bounds checking. Before executing the memory copy operation, the function verifies that the destination buffer is sufficiently large to accommodate the bytes being copied. This check is critical in avoiding overflow situations that could lead to undefined behavior or security vulnerabilities.
Return Values
The function returns an error code (`errno_t`) to indicate the success or failure of the operation. A return value of `0` signifies a successful copy, while a non-zero value implies an error occurred. Developers should implement error handling every time `memcpy_s` is used, ensuring robustness in their applications.
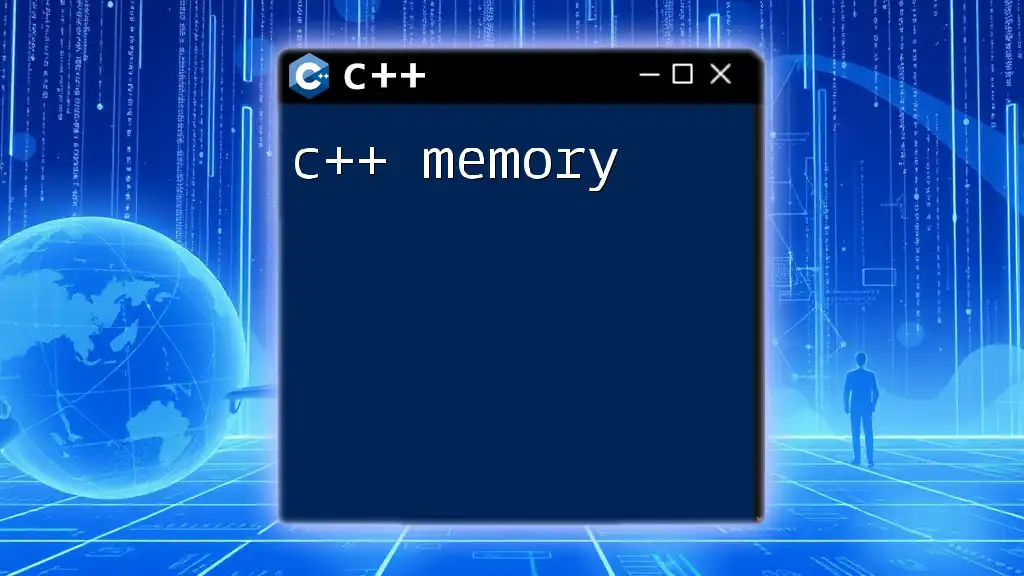
Usage Scenarios
When to Use memcpy_s
`memcpy_s` is particularly beneficial in environments where memory safety is paramount, such as system programming and applications dealing with sensitive data. Developers should choose `memcpy_s` over `memcpy` when:
- Copying data between fixed-size buffers.
- Working on applications that run in a security-sensitive context, where buffer overflows could lead to exploits.
Practical Example: Copying a String Safely
Here is a more extensive example where we employ `memcpy_s` to copy a string while ensuring safety:
#include <cstdio>
#include <cstdlib>
#include <cstring>
int main() {
char safeDest[20];
const char* safeSrc = "Safe String Example";
errno_t err = memcpy_s(safeDest, sizeof(safeDest), safeSrc, strlen(safeSrc) + 1);
if (err == 0) {
printf("Copied string: %s\n", safeDest);
} else {
printf("Memory copy failed with error code: %d\n", err);
}
return 0;
}
In this example, we define a larger destination buffer `safeDest` to accommodate the source string. After calling `memcpy_s`, we check the error code, allowing us to handle any issues gracefully.
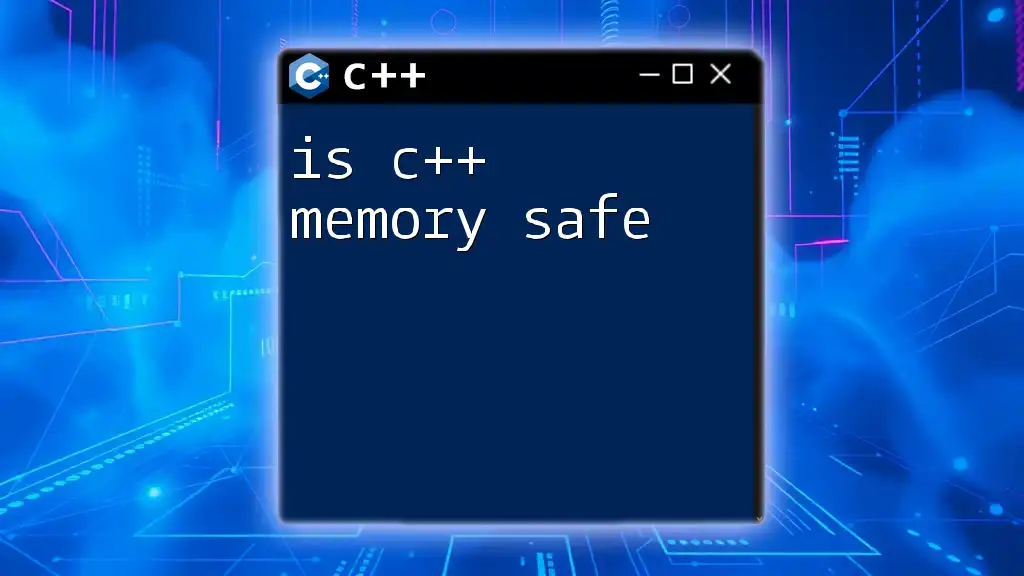
Error Handling with memcpy_s
Understanding Error Codes
Error handling is a critical aspect of using `memcpy_s`. The function can return several specific error codes, including:
- 0: Success - the operation completed successfully.
- EINVAL: Invalid argument - often due to a null pointer or invalid size.
- ERANGE: The destination buffer is too small to hold the copied data.
By understanding these error codes, developers can proactively handle potential issues and improve the robustness of their applications.
Example of Handling Errors
Consider the following error handling example:
errno_t err = memcpy_s(dest, sizeof(dest), src, bytesToCopy);
if (err != 0) {
// Handle error accordingly
fprintf(stderr, "Error encountered: %d\n", err);
}
In this example, we print an error message if the memory copy fails. By doing this, we ensure any potential problems do not go unnoticed, which could otherwise lead to hidden bugs in the application.
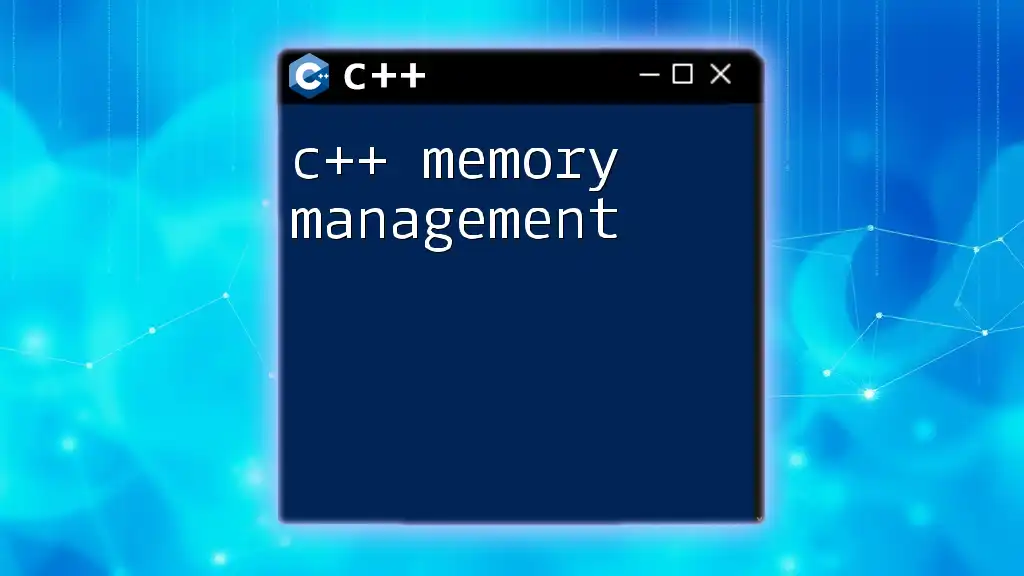
Best Practices for Using memcpy_s
General Guidelines
When utilizing `memcpy_s`, it's vital to adhere to certain best practices:
- Define buffer sizes adequately: Always ensure that your destination buffer is large enough to accommodate the incoming data, including space for a null terminator if copying strings.
- Use null-terminated strings: When working with string data, ensure that your source strings are null-terminated, which is vital for string operations.
Example of Best Practices
To encapsulate safe copying behavior, consider creating a utility function:
void safeCopy(char* dest, size_t destSize, const char* src) {
if (memcpy_s(dest, destSize, src, strlen(src) + 1) != 0) {
// Error handling
}
}
By wrapping `memcpy_s` in this function, we promote code reuse while maintaining safety and simplicity in our operations.
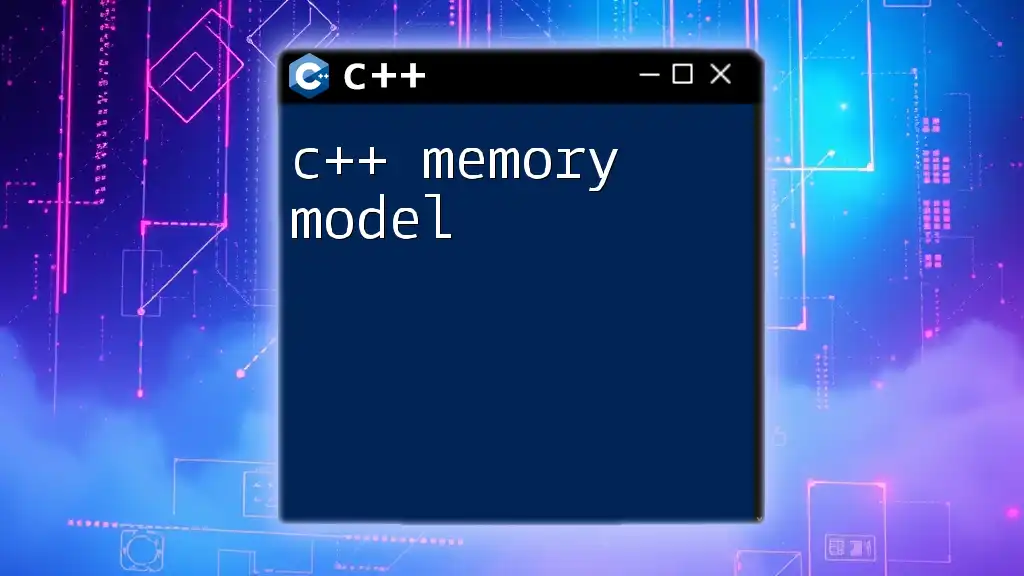
Common Mistakes and Misconceptions
Misunderstanding Parameter Requirements
One common mistake among developers is misunderstanding the parameters, particularly the destination size. Failing to provide the correct size may lead to invalid memory access, undermining the purpose of using `memcpy_s`.
Misusing the Return Value
Another frequent misconception involves ignoring the return value of `memcpy_s`. Neglecting to check this value may lead to vulnerabilities, as developers may unknowingly proceed with operations on invalid data.
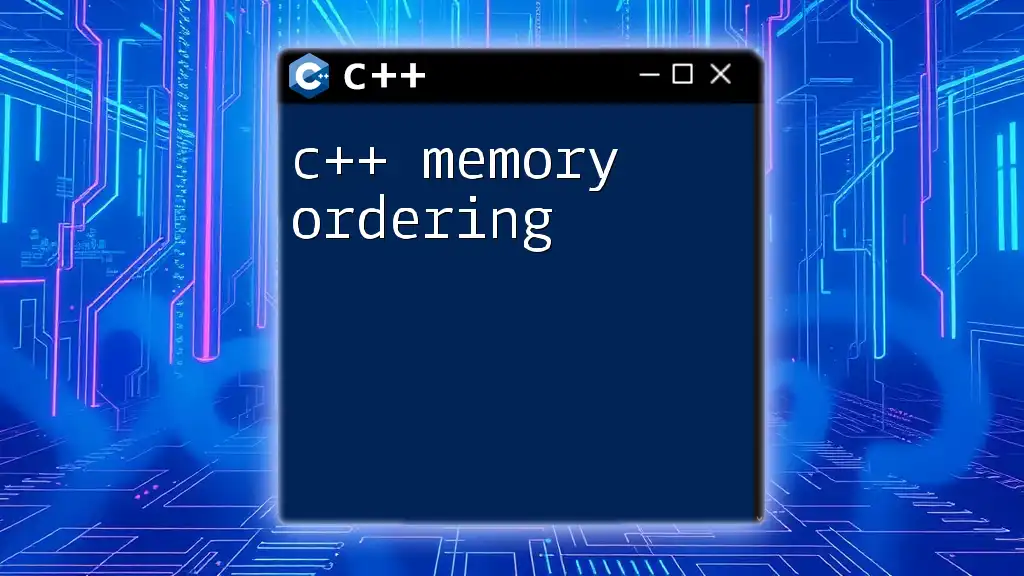
Conclusion
In summary, `memcpy_s` serves as a powerful tool for developers looking to enhance memory safety in their applications. Its bounds-checking functionality, along with the ability to gracefully handle errors, makes it a preferred choice over traditional memory copy operations. As you continue to refine your skills in C++, consider incorporating `memcpy_s` into your coding practices to foster a culture of safety and reliability in memory usage.
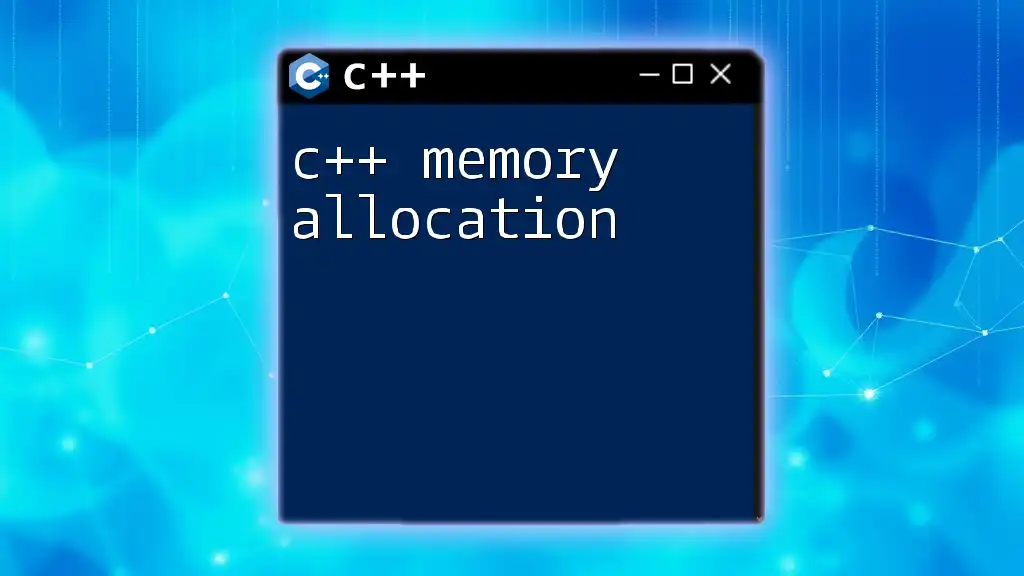
Additional Resources
For further reading on `memcpy_s`, refer to the official documentation and explore online tutorials or forums dedicated to C++ development. Engaging with a community can provide additional support and resources to overcome challenges encountered while using C++ commands.
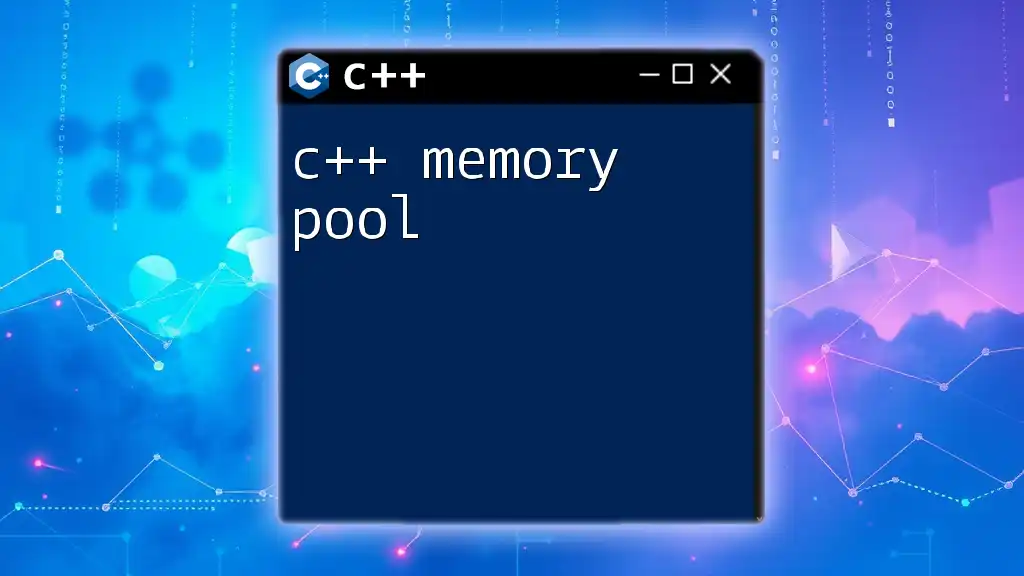
Call to Action
Join our learning community to dive deeper into the world of C++ programming. Our courses provide hands-on training in practical applications like `memcpy_s`, empowering you to write safer, more efficient code. Don’t miss out on becoming a proficient C++ developer!