The `mem` commands in C++ typically refer to memory management functions which allow you to allocate, deallocate, and manipulate memory directly, such as using `malloc` and `free` from the C Standard Library.
Here's a quick example of using `malloc` and `free`:
#include <iostream>
#include <cstdlib>
int main() {
int* array = (int*)malloc(5 * sizeof(int)); // Allocate memory for an array of 5 integers
if (array == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
return 1;
}
// Use the allocated memory
for(int i = 0; i < 5; ++i) {
array[i] = i * 10;
std::cout << array[i] << ' ';
}
std::cout << std::endl;
free(array); // Deallocate memory
return 0;
}
Understanding Memory in C++
Static vs Dynamic Memory Allocation
Static Memory Allocation refers to memory that is allocated at compile time. This type of memory allocation is fixed, which means that its size cannot be changed during runtime. Local variables typically use static allocation—allocated on the stack.
Here's an example of static memory allocation:
#include <iostream>
int main() {
int staticVar = 10; // Memory allocated on stack
std::cout << "Static Variable: " << staticVar << std::endl;
return 0;
}
On the other hand, Dynamic Memory Allocation allows for memory to be allocated during runtime. This is useful when the size of the data structure is not known beforehand. Memory allocated dynamically is done on the heap using operators like `new`.
Example of dynamic memory allocation:
#include <iostream>
int main() {
int* dynamicVar = new int; // Allocated on heap
*dynamicVar = 20;
std::cout << "Dynamic Variable: " << *dynamicVar << std::endl;
delete dynamicVar; // Always release memory
return 0;
}
The C++ Memory Model
The Stack is a region of memory that stores temporary variables created by each function (like local variables). The stack follows the Last In, First Out (LIFO) principle. Memory management is easy here as the memory is automatically managed when the function call ends.
The Heap, in contrast, is used for dynamically allocated memory. Unlike stack memory, you must manage heap memory manually with corresponding `new` and `delete` operators. This provides more flexibility but requires careful management to avoid memory leaks and errors.
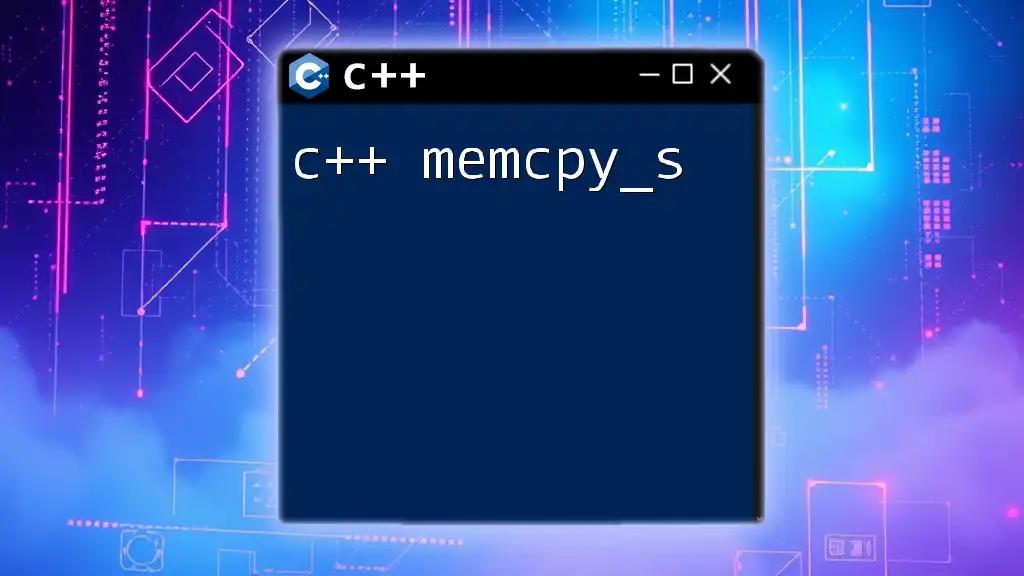
Memory Allocation Operators
Using `new` and `delete`
Allocating memory in C++ is straightforward with the `new` operator:
#include <iostream>
int main() {
int* num = new int(5); // Allocating an integer on the heap
std::cout << "The value is: " << *num << std::endl;
delete num; // Deallocate memory
return 0;
}
You can also allocate arrays using `new`:
#include <iostream>
int main() {
int* arr = new int[5]; // Allocating an array
for (int i = 0; i < 5; ++i) {
arr[i] = i + 1; // Initializing array
}
for (int i = 0; i < 5; ++i) {
std::cout << arr[i] << " "; // Outputting array
}
delete[] arr; // Properly deallocate array
return 0;
}
Deallocating memory should always be done using `delete` for single variables and `delete[]` for arrays:
delete num; // For single variable
delete[] arr; // For array
Smart Pointers: `unique_ptr`, `shared_ptr`, and `weak_ptr`
What are Smart Pointers? Smart pointers manage memory automatically and help prevent memory leaks. They are a safer alternative to raw pointers.
`unique_ptr`
`unique_ptr` represents exclusive ownership. When one `unique_ptr` is created, no other `unique_ptr` can point to the same object.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> uptr(new int(30));
std::cout << "Unique Pointer Value: " << *uptr << std::endl;
// uptr2 = uptr; // Error: can't copy unique_ptr
std::unique_ptr<int> uptr2 = std::move(uptr); // Ownership transfer
std::cout << "Unique Pointer Value after move: " << *uptr2 << std::endl;
return 0;
}
`shared_ptr`
`shared_ptr` allows multiple pointers to share ownership of the same object, which uses reference counting to manage memory.
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> sptr(new int(40));
std::cout << "Shared Pointer Value: " << *sptr << std::endl;
{
std::shared_ptr<int> sptr2 = sptr; // Both point to the same memory
std::cout << "Shared Pointer Value inside scope: " << *sptr2 << std::endl;
} // sptr2 goes out of scope, but memory is still valid
std::cout << "Shared Pointer Value after scope: " << *sptr << std::endl;
return 0;
}
`weak_ptr`
`weak_ptr` is used alongside `shared_ptr` to prevent circular references. It does not affect the reference count of `shared_ptr`.
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> sptr(new int(50));
std::weak_ptr<int> wptr = sptr; // Create weak pointer
if (auto shared_from_wptr = wptr.lock()) { // Check if memory is still valid
std::cout << "Weak Pointer Value: " << *shared_from_wptr << std::endl;
} else {
std::cout << "Memory is no longer accessible" << std::endl;
}
return 0;
}

Common Memory Management Issues
Memory Leaks
What is a Memory Leak? A memory leak occurs when the allocated memory is not properly deallocated, leading to wasted memory resources.
For example:
#include <iostream>
void createMemoryLeak() {
int* leak = new int(100); // Memory allocated but not deleted
// Memory leak occurs here
}
int main() {
createMemoryLeak();
// Memory allocated in createMemoryLeak is lost
return 0;
}
Detecting Memory Leaks: Tools like Valgrind are essential for identifying memory leaks in your programs effectively.
Dangling Pointers
Understanding Dangling Pointers: These are pointers that still reference a memory location that has been freed. This can lead to undefined behavior if you attempt to dereference them.
Here's an example:
#include <iostream>
int* createDanglingPointer() {
int* temp = new int(200);
delete temp; // Memory deallocated
return temp; // Dangling pointer returned
}
int main() {
int* danglingPtr = createDanglingPointer();
// std::cout << *danglingPtr; // Undefined behavior: accessing freed memory
return 0;
}
Double Deletion
What is Double Deletion? This happens when you attempt to delete the same memory location twice, leading to program crashes or undefined behavior.
Example:
#include <iostream>
int main() {
int* ptr = new int(300);
delete ptr; // Memory deallocated
delete ptr; // Error: attempting to deallocate memory twice
return 0;
}

Best Practices for Memory Management in C++
Always Initialize Pointers
Initializing pointers is crucial. If not initialized, a pointer may point to any arbitrary memory location which can lead to unpredictable behavior.
int* p = nullptr; // Initialized to nullptr
Use Smart Pointers Over Raw Pointers
Utilize smart pointers like `unique_ptr` and `shared_ptr`. They automatically manage memory for you, reducing the likelihood of leaks and dangling pointers.
Implementing RAII (Resource Acquisition Is Initialization)
What is RAII? RAII is a principle in C++ where resource allocation is tied to object lifetime. Resources are acquired during the creation phase and released during the destruction.
Example:
#include <iostream>
class Resource {
public:
Resource() { std::cout << "Resource acquired" << std::endl; }
~Resource() { std::cout << "Resource released" << std::endl; }
};
int main() {
Resource res; // Resource acquired here
// Resource automatically released when going out of scope
return 0;
}
Avoiding Manual Memory Management When Possible
Preference should be given to using STL containers (like `std::vector`, `std::string`, etc.) that handle memory management for you, which follows modern C++ practices.

Conclusion
Understanding c++ mem is critical for every C++ developer. By mastering memory management principles, utilizing the appropriate techniques, and adhering to best practices, you can write robust and efficient programs. We encourage readers to share their experiences and questions regarding memory management in C++. Together, we can enhance our understanding and mastery of this vital aspect of programming.

Additional Resources
For those looking to deepen their knowledge, consider exploring recommended books and courses, as well as joining online communities where you can engage with other C++ developers.