In C++, the median of a list of numbers can be calculated by sorting the list and then finding the middle element(s), as shown in the following code snippet:
#include <iostream>
#include <vector>
#include <algorithm>
double median(std::vector<int>& numbers) {
std::sort(numbers.begin(), numbers.end());
int n = numbers.size();
return n % 2 == 0 ? (numbers[n / 2 - 1] + numbers[n / 2]) / 2.0 : numbers[n / 2];
}
int main() {
std::vector<int> nums = {1, 3, 2, 5, 4};
std::cout << "Median: " << median(nums) << std::endl;
return 0;
}
What is the Median?
The median is a measure of central tendency that represents the middle value of a dataset when it is ordered. It serves as an important statistic, especially in cases where datasets may be skewed or contain extreme outlier values. Unlike the mean, which can be significantly affected by outliers, the median provides a clearer picture of the 'central' value of the data.
The median can be better understood in context with two other statistics: the mean and mode. The mean is the arithmetic average, while the mode represents the most frequently occurring value in a dataset. Each of these measures has its relevance and ideal use cases.
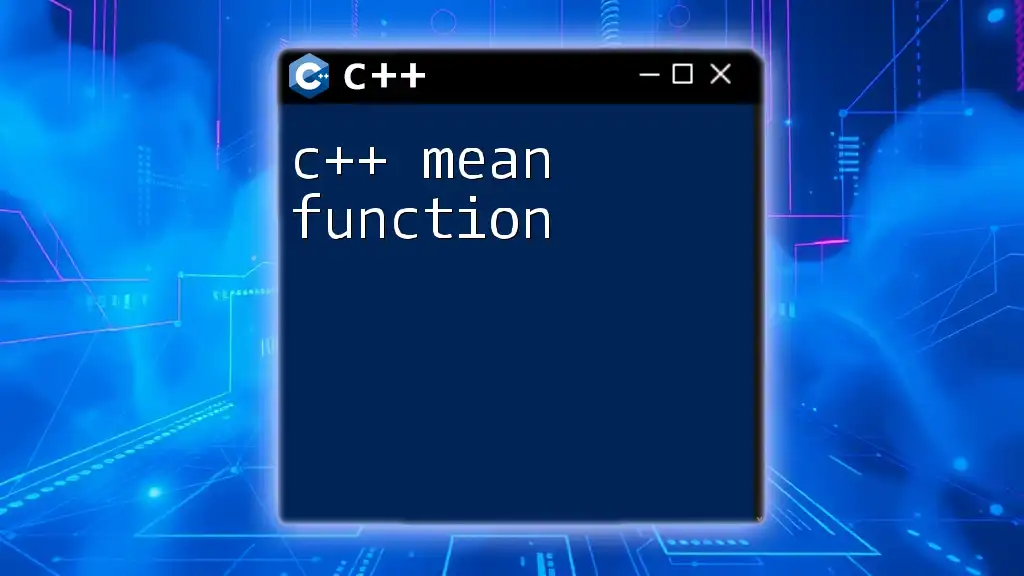
Why Use the Median?
Choosing the median over the mean is particularly advantageous in certain situations, especially when the dataset contains significant outliers. For instance, in income data analysis, where a few extremely high incomes can skew the average (mean) upwards, the median presents a more realistic view of what a 'typical' income might be.
Moreover, the median remains unaffected by the magnitude of variations in larger extreme values. Hence, it's an essential tool in fields such as finance, healthcare, and education, where accurately gauging the average scenario is critical.
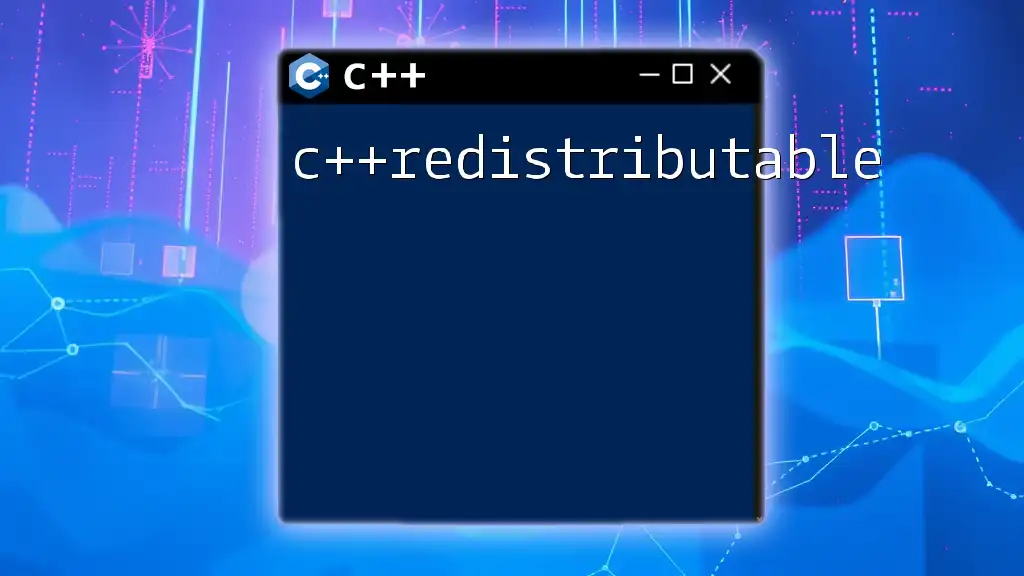
Methods to Calculate the Median in C++
Sorting the Data
To calculate the median, the first step is often to sort the data. This is essential, regardless of whether you are working with arrays, lists, or vectors in C++. Sorting can be accomplished using a variety of algorithms, from simple ones like bubble sort to more advanced methods like quicksort or mergesort.
Here is a sample code snippet demonstrating sorting an array using the C++ Standard Library:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {3, 1, 4, 1, 5, 9};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n);
std::cout << "Sorted array: ";
for (int i = 0; i < n; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
return 0;
}
Finding the Median of an Odd Set of Numbers
When the dataset length is odd, the median can be found simply by picking the middle element from the sorted array. The position of this element can be determined by dividing the total number of elements by two.
For example, consider the following code snippet for an odd-length array:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {7, 1, 3, 5, 9};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n);
// Median for odd-length array
int median = arr[n / 2];
std::cout << "Median: " << median << std::endl;
return 0;
}
Finding the Median of an Even Set of Numbers
In a dataset with an even number of values, the median is calculated as the average of the two middle numbers after sorting. This requires accessing the two central elements through indexing.
Here's an example code snippet demonstrating this:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {8, 3, 5, 7};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n);
// Median for even-length array
double median = (arr[n / 2 - 1] + arr[n / 2]) / 2.0;
std::cout << "Median: " << median << std::endl;
return 0;
}

Using Standard Library Functions
Introduction to STL
The Standard Template Library (STL) in C++ provides a variety of algorithms and data structures which can simplify the task of calculating the median. The STL lets developers utilize pre-existing functions, enhancing code efficiency and readability.
Using `std::vector`
Vectors are dynamic arrays in C++ that can be easily manipulated to store data. They are part of the STL and provide built-in sorting functions.
Here is an example of using a `std::vector` to calculate the median:
#include <iostream>
#include <vector>
#include <algorithm>
double calculateMedian(std::vector<int> &vec) {
std::sort(vec.begin(), vec.end());
int n = vec.size();
if (n % 2 != 0) // Odd length
return vec[n / 2];
else // Even length
return (vec[n / 2 - 1] + vec[n / 2]) / 2.0;
}
int main() {
std::vector<int> data = {3, 4, 1, 5, 2};
double median = calculateMedian(data);
std::cout << "Median: " << median << std::endl;
return 0;
}
Using `std::nth_element`
Another efficient method for finding the median is through `std::nth_element`, which partially sorts the data to position the nth element in its correct sorted position without fully sorting the entire dataset.
Here’s an example of how to use `std::nth_element`:
#include <iostream>
#include <vector>
#include <algorithm>
double calculateMedian(std::vector<int> vec) {
size_t n = vec.size();
std::nth_element(vec.begin(), vec.begin() + n / 2, vec.end());
if (n % 2 != 0) // Odd length
return vec[n / 2];
else {
int median1 = vec[n / 2];
std::nth_element(vec.begin(), vec.begin() + (n / 2 - 1), vec.end());
return (median1 + vec[n / 2 - 1]) / 2.0;
}
}
int main() {
std::vector<int> data = {7, 2, 1, 5, 4, 3, 6};
double median = calculateMedian(data);
std::cout << "Median: " << median << std::endl;
return 0;
}

Special Cases and Considerations
Handling Duplicates
When dealing with datasets that include duplicate values, the median calculation remains consistent, as the presence of duplicates will not change the middle value when the data is sorted. Keeping this in mind, when working with extensive datasets, performance optimization becomes incredibly relevant.
Performance Considerations
The time complexity of sorting an array is \(O(n \log n)\). However, if one uses `std::nth_element`, the complexity for finding the median can be reduced to \(O(n)\) on average. For very large datasets, this optimization can lead to significant performance improvements.
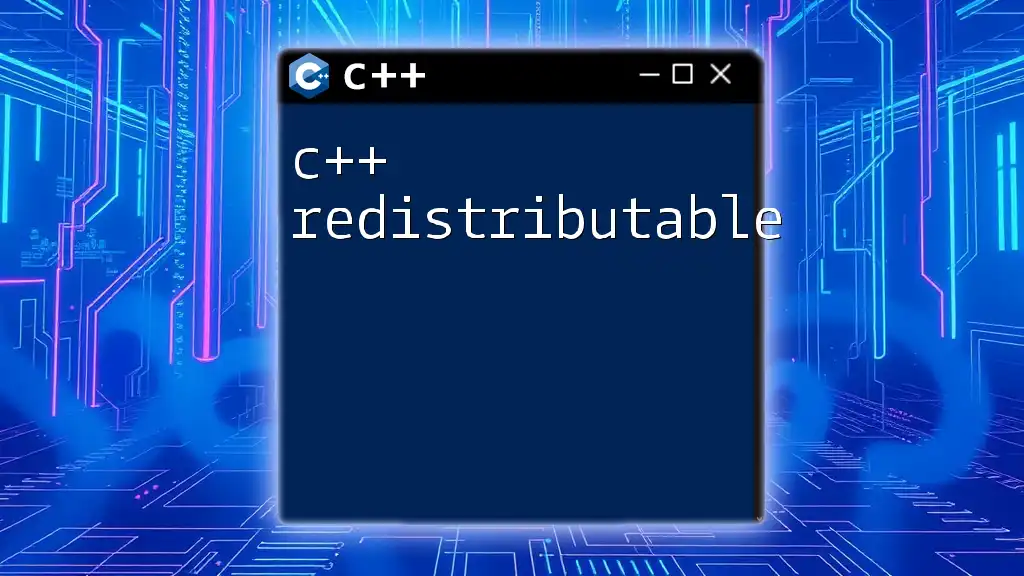
Practical Applications of the Median
Real-World Use Cases
The median is widely used in multiple sectors. In finance, it's often essential for analyzing income distributions, while in healthcare, median values provide clearer insights into patient data and health metrics without the distortion of outliers. In education, median test scores can give a more accurate representation of student performance compared to the mean.
User Input and Dynamic Data
Reading user input can create dynamic situations where the median needs to be calculated on-the-fly. Below is an example code snippet demonstrating how to calculate the median from user-entered data:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> data;
int number;
std::cout << "Enter numbers (type -1 to end): ";
while (std::cin >> number && number != -1) {
data.push_back(number);
}
double median = calculateMedian(data);
std::cout << "Median: " << median << std::endl;
return 0;
}
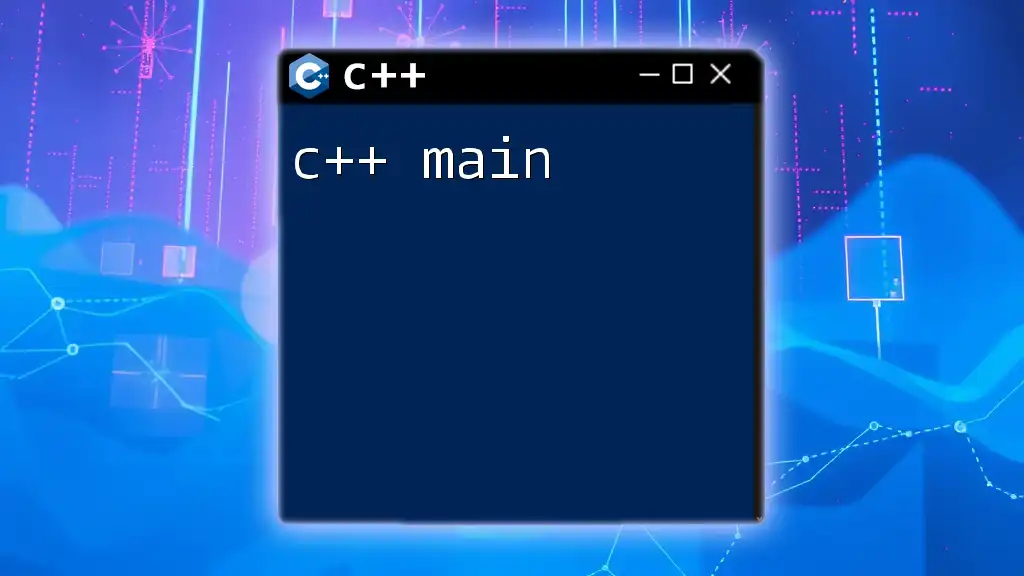
Conclusion
In conclusion, understanding how to calculate the C++ median is essential for anyone working with datasets. By combining various methods — from basic sorting to leveraging STL functions — developers can choose the most efficient approach for their specific application.
Summary of Key Points
We explored the definition and significance of the median, various methods to compute it in C++, STL functionalities, and various real-world applications. Each method presents unique advantages depending on the dataset's characteristics.
Encouragement for Further Learning
We encourage readers to explore more advanced topics in statistics and C++, including concepts like `percentiles`, `quartiles`, and data visualization techniques for deeper insights into data analysis. Unlocking these skills will greatly enhance your programming and analytical proficiency!
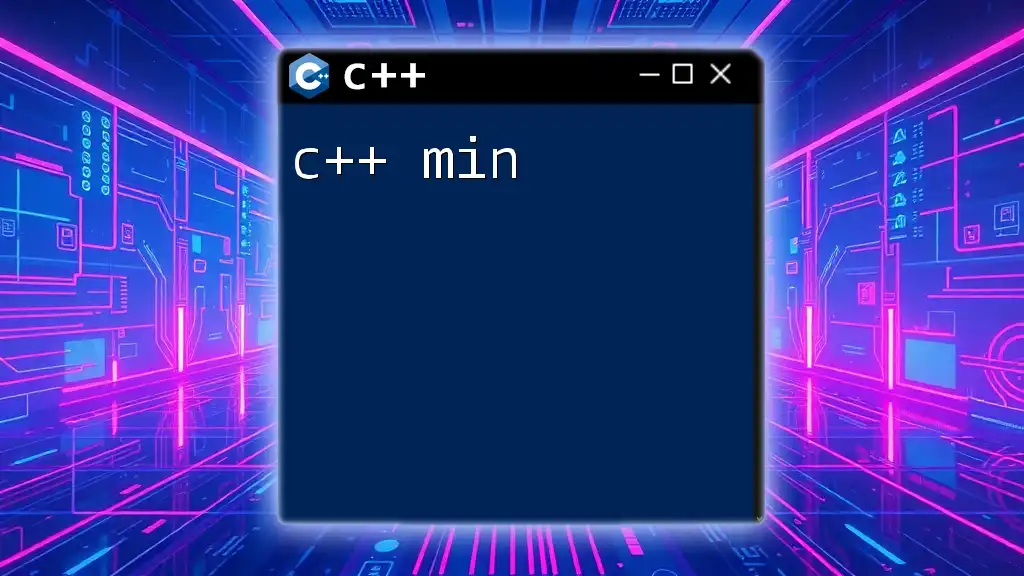
Additional Resources
For those eager to delve deeper, consider exploring books, articles, and online tutorials on C++ and statistical methods. Participating in online programming communities can also provide peer support and valuable discussions that can advance your knowledge and skills.