In C++, the `&` symbol refers to the "address-of" operator, which is used to obtain the memory address of a variable, as well as to define reference variables that allow multiple names to refer to the same object.
Here's an example code snippet demonstrating both uses:
#include <iostream>
int main() {
int x = 10; // a normal integer variable
int* ptr = &x; // using & to get the address of x
int& ref = x; // using & to create a reference to x
std::cout << "Value of x: " << x << std::endl;
std::cout << "Address of x: " << ptr << std::endl;
std::cout << "Reference to x: " << ref << std::endl;
return 0;
}
Understanding the Ampersand Symbol in C++
What is the Ampersand (&) in C++?
The ampersand symbol, &, is a versatile operator in C++ that plays a crucial role in memory management and data manipulation. Understanding its functions is essential for effective programming. It signifies two primary concepts: the reference operator and the address-of operator. While both are fundamentally linked through memory management, they operate quite differently and serve unique purposes in coding.
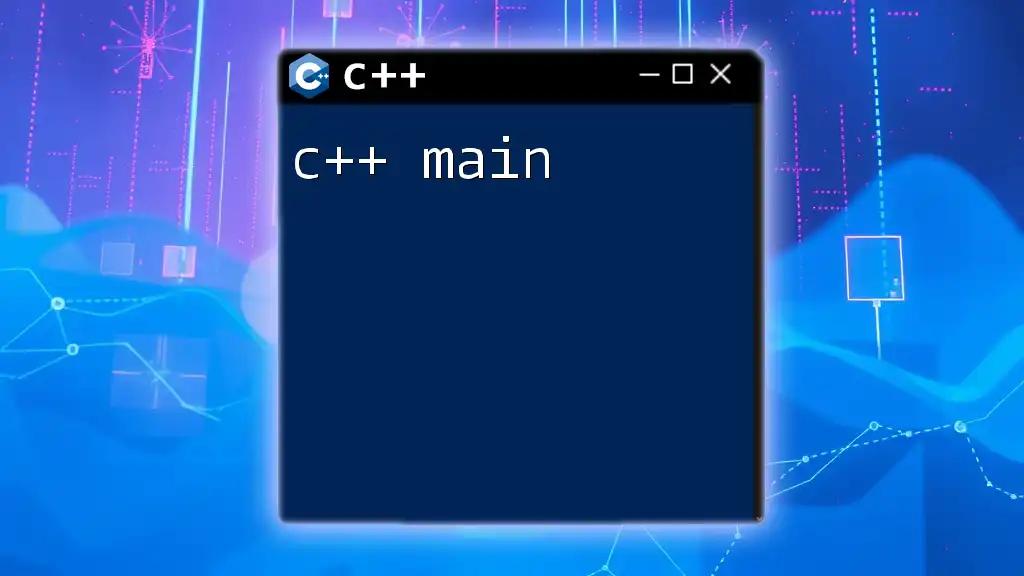
Uses of the Ampersand in C++
Reference Operator in C++
In C++, the ampersand is frequently used as a reference operator. This allows you to create an alias for a variable, enabling you to manipulate the original variable directly without creating a copy. This becomes particularly significant when dealing with complex data structures or large objects.
To use the ampersand as a reference operator, you would typically declare a function parameter like so:
void displayValue(int &ref) {
std::cout << "Value: " << ref << std::endl;
}
int main() {
int value = 5;
displayValue(value); // 'value' is passed by reference
return 0;
}
In this example, `value` is passed by reference, meaning any modifications inside `displayValue` will directly reflect on the `value` variable. This is an important feature, as it helps avoid unnecessary copying of large data objects, improving performance succinctly.
Explanations
Using references can dramatically simplify code by allowing for clean and maintainable function signatures. However, it comes with the responsibility of ensuring that the referenced variable remains valid throughout its usage.
Address-of Operator in C++
The second common use of the ampersand is as the address-of operator. This signifies that you can retrieve the memory address of a variable, which is vital for operations involving pointers.
Here’s a simple example:
int main() {
int var = 10;
std::cout << "Address of var: " << &var << std::endl; // Get address of var
return 0;
}
In this code snippet, `&var` is used to retrieve the memory address where `var` is stored. Understanding memory addresses is foundational to mastering pointers and dynamic memory allocation in C++.
Explanations
The ability to access a variable's address opens up various possibilities in programming, such as creating and managing dynamic data structures where memory needs to be allocated and freed explicitly.

Common Misconceptions about the Ampersand in C++
Misunderstanding References and Pointers
A common misconception involves confusing references and pointers. Both are essential concepts in C++, yet their roles and usage differ significantly.
References vs. Pointers:
Aspect | References | Pointers |
---|---|---|
Syntax | `int &ref` | `int *ptr` |
Initialization | Must be initialized at declaration | Can be initialized to `nullptr` |
Reassignment | Cannot be reassigned once initialized | Can be reassigned at any time |
Memory Safety | Generally safer; no null references | Can be null or point to invalid memory |
Code Example:
int a = 10;
int *p = &a; // Pointer to a
int &r = a; // Reference to a
Clarification
References provide a safer and more intuitive syntax for passing variables around, especially in function calls. Pointers, while also powerful, require a deeper understanding of memory management, which can introduce complexity and potential errors.
The Ampersand in Function Overloading
The ampersand also plays an important role in function overloading. When functions are overloaded, the ampersand can affect which version of the function is called based on how parameters are passed.
Code Example:
void func(int &x) {
std::cout << "Reference Function Called with: " << x << std::endl;
}
void func(int x) {
std::cout << "Value Function Called with: " << x << std::endl;
}
In this instance, when you pass an integer variable to `func()`, the version that corresponds to its type (value vs. reference) is called based on how you pass it.
Explanations
This behavior can lead to surprising results if not understood well, especially when dealing with function overloads that differ only in terms of reference usage. Understanding the nuances of how the ampersand operates in this context can help prevent unintended behavior in your programs.
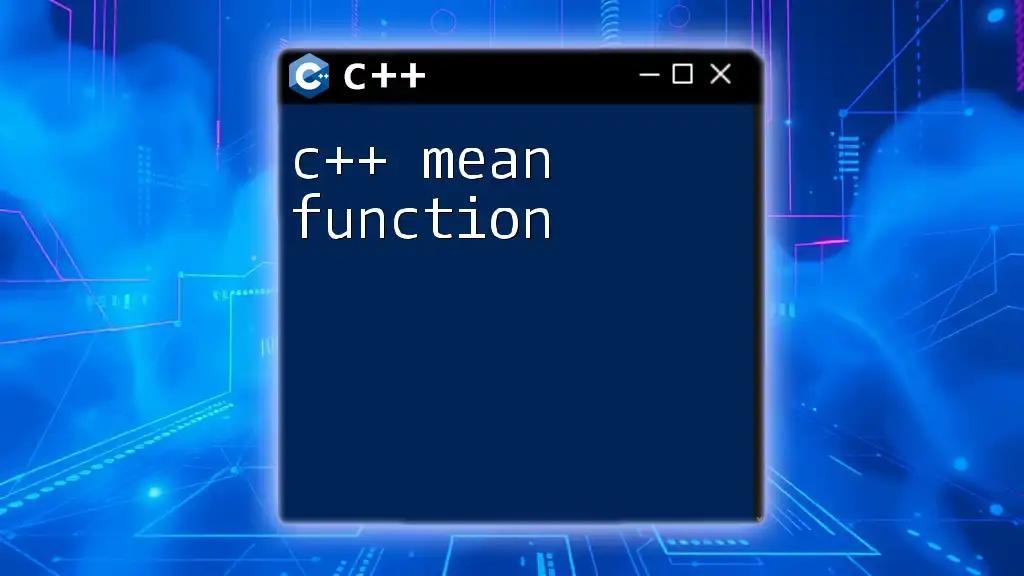
Best Practices for Using the Ampersand in C++
When to Use References
References are best utilized when you want to modify a variable without the cost of copying data, particularly with class types or large structures.
Considerations:
- Use references for parameters where the function should modify the passed variable.
- Favor references over pointers when you do not need to handle null cases.
Best Practices:
- Always use references when passing large objects.
- Clear naming conventions can help differentiate between reference and pointer parameters, improving readability.
Addressing Common Pitfalls
When using references, be cautious of dangling references, which occur when a reference points to a memory location that has been freed or is out of scope.
Example of Dangling Reference:
int *p = new int(10);
int &r = *p; // This is safe
delete p; // Now r refers to invalid memory
In the above example, after `p` is deleted, `r` becomes a dangling reference. Accessing it can result in undefined behavior.
Explanations
To mitigate this risk, ensure that references always point to valid memory locations. In situations where a reference might go out of scope, consider using smart pointers or checking the validity of the pointer before dereferencing it.
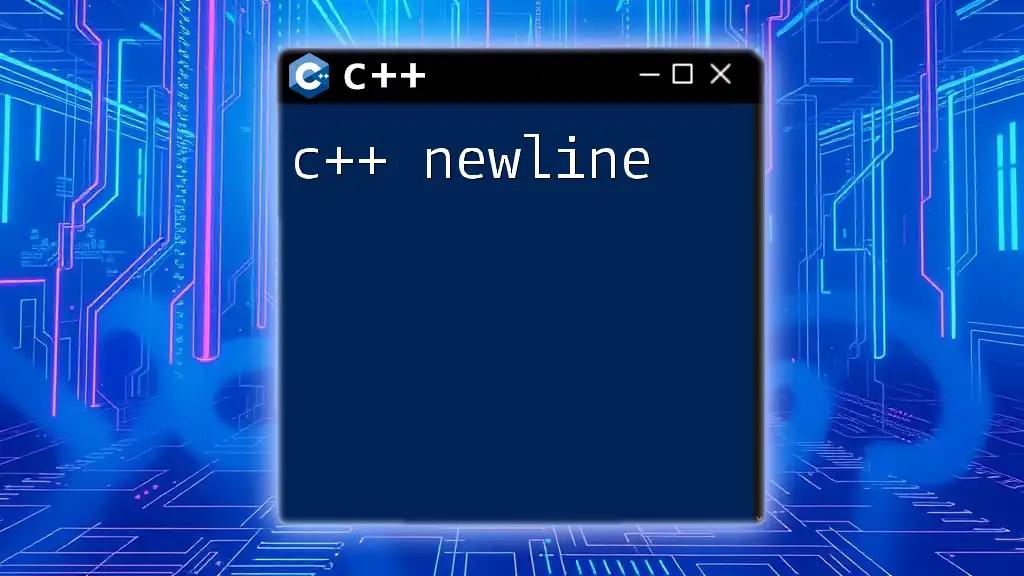
Conclusion
Recap of the Importance of Understanding "&" in C++
Understanding the ampersand (&) in C++ is pivotal for effective programming. From serving as a reference operator that allows direct manipulation of variables to acting as an address-of operator that unlocks the world of pointers, mastering its usage can significantly enhance your coding proficiency.
Call to Action
We encourage you to continue exploring the intricacies of C++. Engage with coding challenges or consider joining programming communities where you can discuss and share your experiences with ampersand usage and other C++ concepts. Your journey into the world of C++ programming has just begun!