The `min` function in C++ returns the smaller of two values, which can be utilized for comparing primitive data types or custom objects. Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <algorithm> // For std::min
int main() {
int a = 5;
int b = 10;
int minimum = std::min(a, b);
std::cout << "The minimum value is: " << minimum << std::endl;
return 0;
}
What is C++ min?
C++ provides a built-in function called `min` that helps to determine the smallest of two (or more) values. This function is particularly useful in scenarios involving comparisons, as it simplifies the code and enhances readability.
When programming, being able to quickly access the least of several values can make a significant difference in the efficiency and clarity of your code. The `min` function is a vital part of the C++ Standard Library, which is designed to facilitate a wide range of programming tasks, allowing developers to write more efficient and maintainable code.
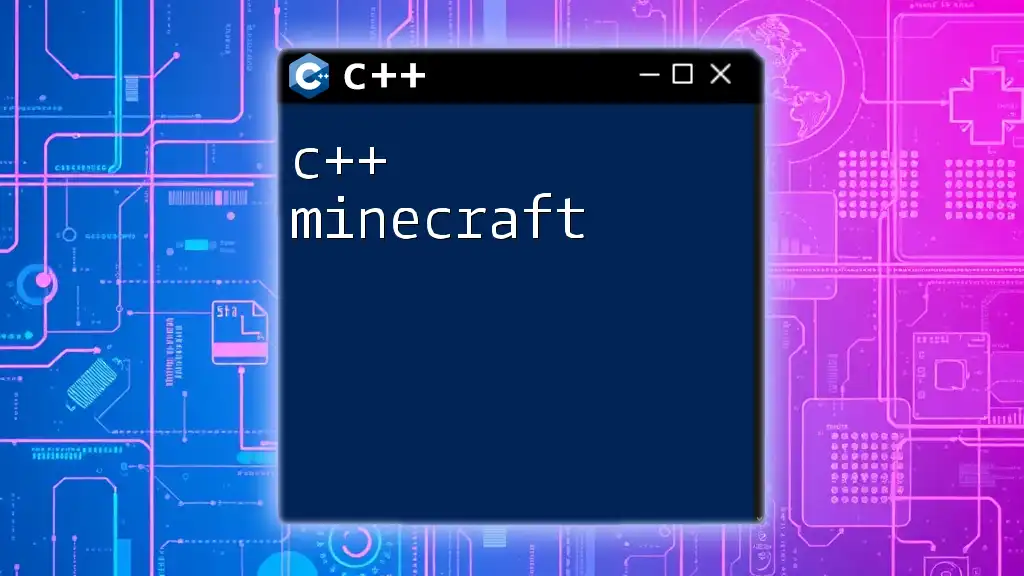
How to Use min in C++
Basic Syntax
The basic syntax of the `min` function is as follows:
T min(T a, T b)
In this syntax, T represents the data type of the values you want to compare. This can be any built-in type such as `int`, `float`, `double`, or `char`.
Example
Here’s a simple example of how to use `min` with two integer values:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 10;
cout << "Minimum is: " << min(a, b) << endl; // Output: 5
return 0;
}
In this code, the `min` function compares the values of `a` and `b`, outputting the smaller of the two.
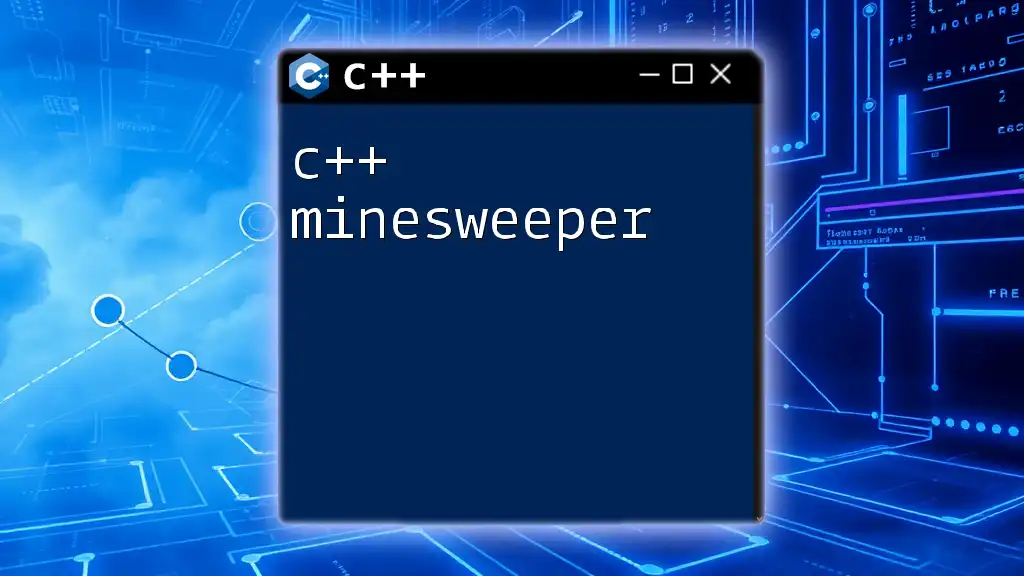
Features of C++ min
Overloading
The `min` function is overloaded, meaning it has the ability to accept different types or a different number of parameters. This flexibility allows developers to compare not just integers but also floating-point numbers, characters, and more.
Template Function
C++ utilizes template programming for the `min` function, allowing it to handle various data types seamlessly. This means that when you call `min` with any type, the C++ compiler automatically selects the correct function overload.
Example
Here’s how you can use `min` with float values:
float x = 5.5, y = 2.3;
cout << "Minimum is: " << min(x, y) << endl; // Output: 2.3
In this example, `min` correctly identifies the smallest floating-point number.
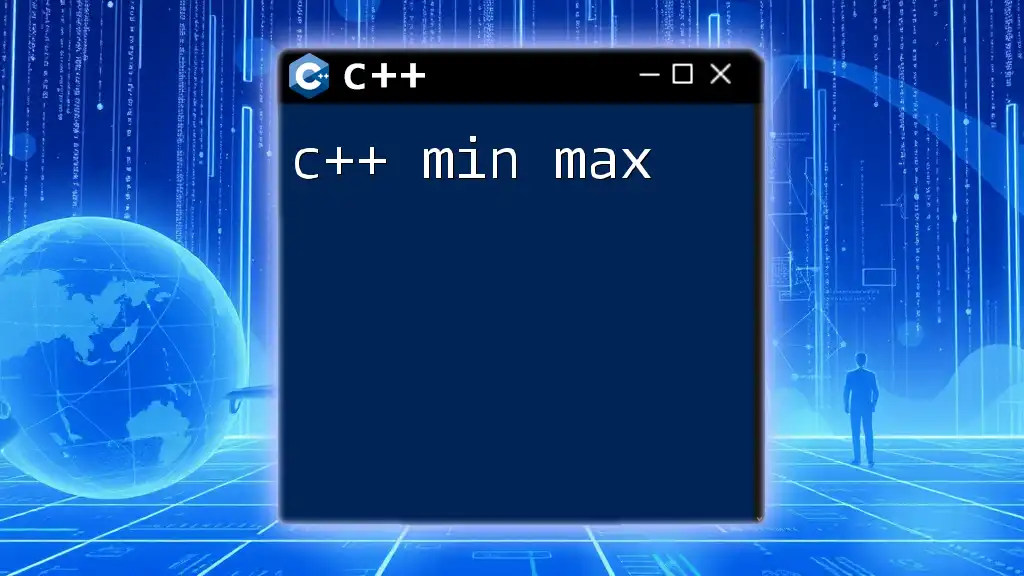
Practical Applications of min
One of the many practical applications of `min` is in comparisons within loops, where you might want to identify the smallest value in an array or a list of numbers. Instead of implementing lengthy conditional statements, `min` simplifies the process.
Example
Consider the following code snippet that finds the smallest number in an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {5, 10, 3, 8};
int smallest = arr[0];
for (int i = 1; i < 4; i++) {
smallest = min(smallest, arr[i]);
}
cout << "Smallest element is: " << smallest << endl; // Output: 3
return 0;
}
In this code, we loop through the array, continually updating the `smallest` variable with the smaller value using the `min` function.
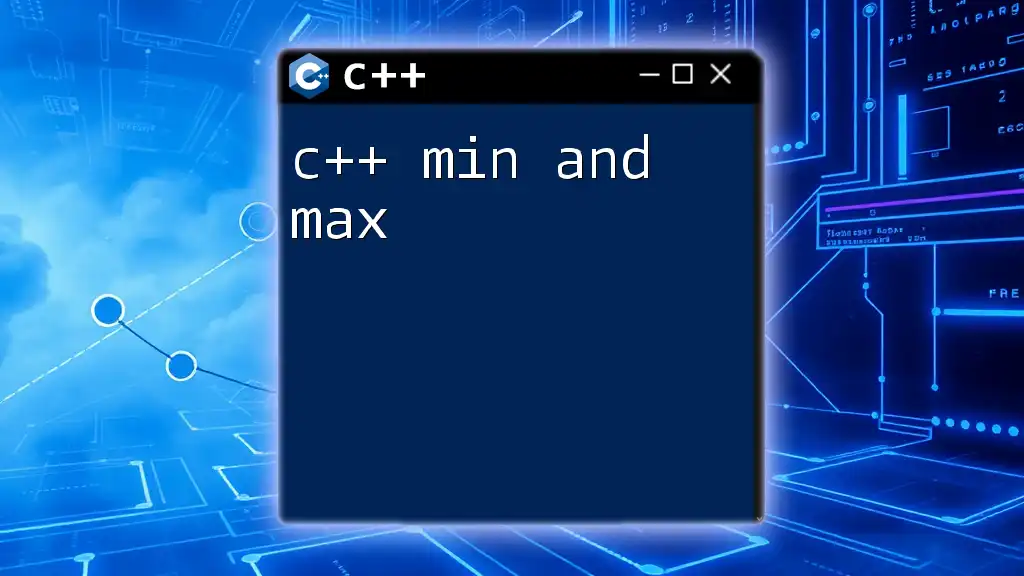
Common Mistakes and Troubleshooting
Understanding Data Types
One common mistake when using the `min` function is improper matching of data types. If you attempt to compare two variables of different types without explicit casting, you may encounter unexpected results or compilation errors.
Examples of Type Mismatch
For instance, trying to mix `int` with `char` or `float` with `string` can lead to issues. Always ensure that the types you are comparing are compatible to avoid unexpected behavior.
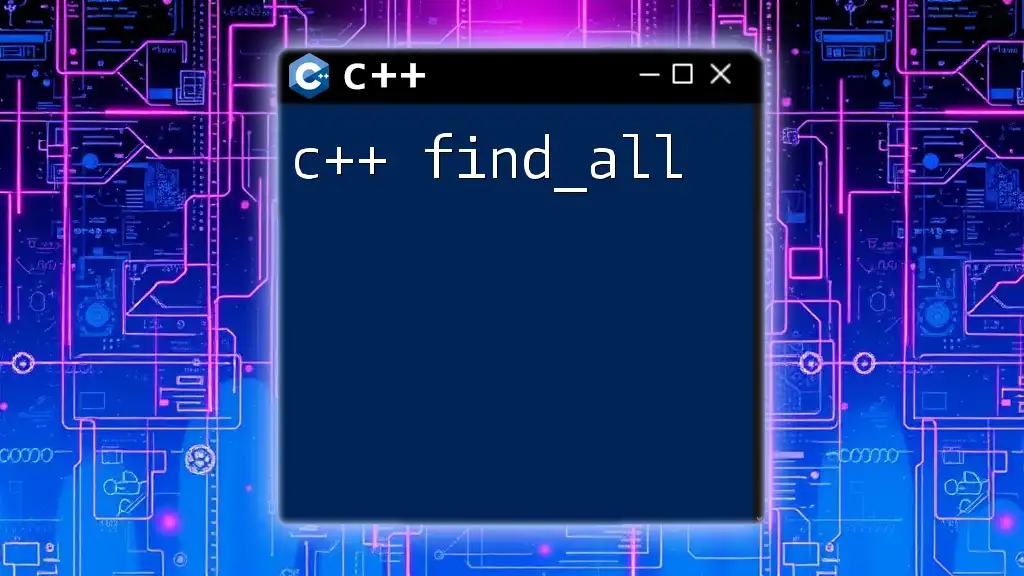
Performance Considerations
Efficiency of min Function
In the context of performance, the `min` function is generally efficient for small datasets. However, when dealing with larger collections or within nested loops, consider the overhead of repeatedly calling `min`.
When to Use and When to Avoid
While `min` is extremely useful, it’s also important to evaluate when it is appropriate to use it. In scenarios where performance is critical, and you frequently run comparisons, you might explore alternative methods like manual comparisons, especially if dealing with large arrays.
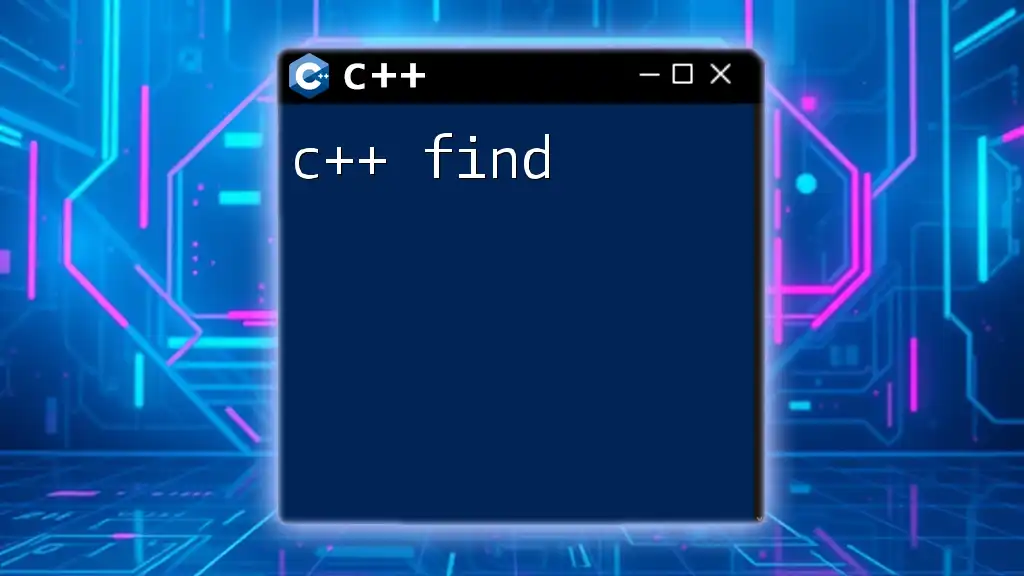
Alternative Approaches
Custom Min Function
If you find yourself needing more flexibility, you can easily implement your own version of `min`. Here’s an example of a custom min function using templates:
template <typename T>
T customMin(T a, T b) {
return (a < b) ? a : b;
}
// Usage
cout << "Custom Minimum: " << customMin(5, 10) << endl; // Output: 5
This custom function demonstrates how to return the smallest of two values while still maintaining type safety and flexibility.
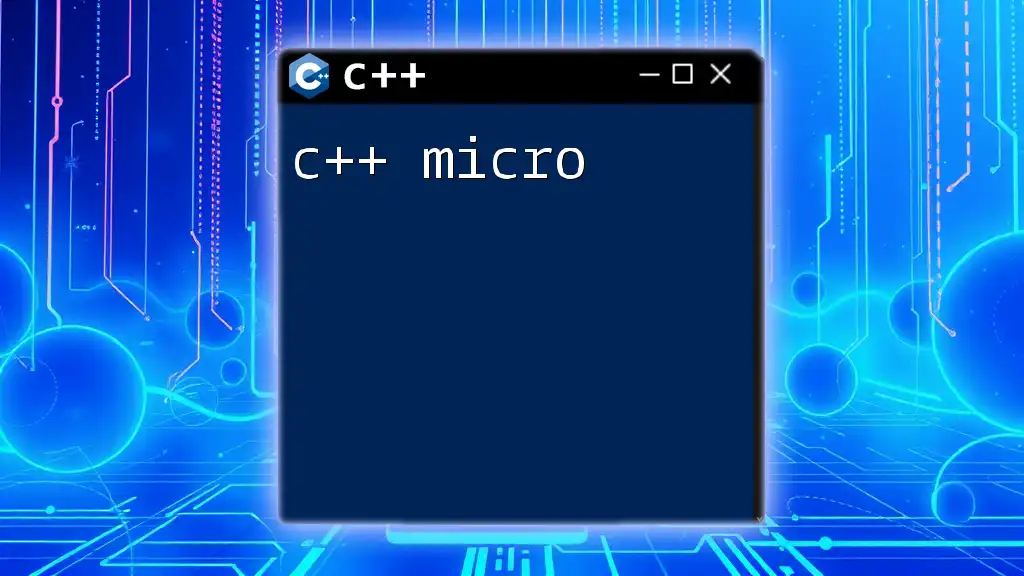
Conclusion
In summary, the `min` function in C++ is a powerful tool for simplifying comparisons in your code. Its overloaded nature and template support allow you to efficiently find the smallest value among integers, floats, and even user-defined types. Understanding and effectively utilizing `min` can enhance both the readability and performance of your C++ programs.
As you immerse yourself in programming with C++, I encourage you to experiment with `min` in varied scenarios. The more you practice, the more intuitive this versatile function will become in your programming toolkit.
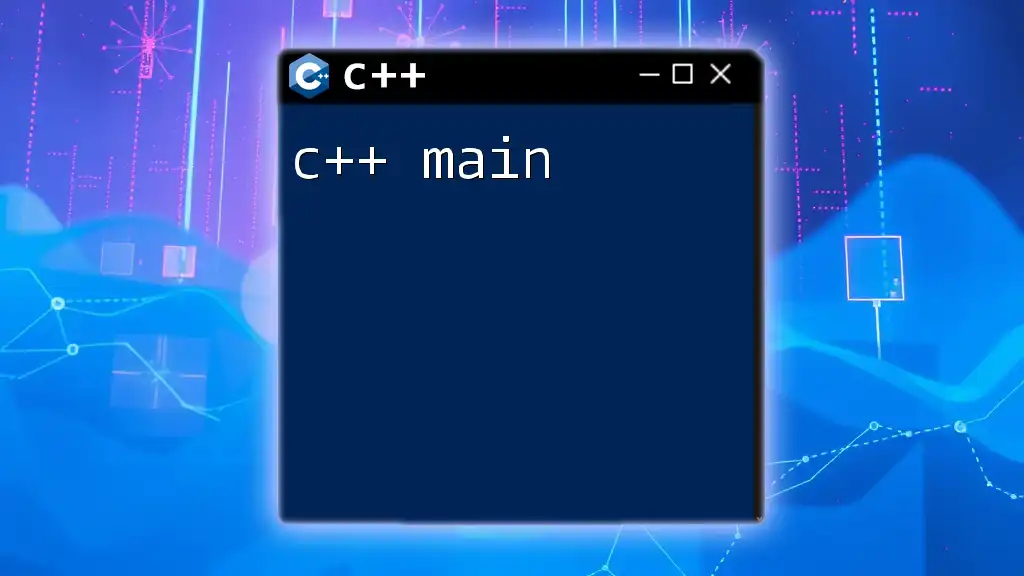
Additional Resources
To further expand your knowledge, consider exploring the following resources:
- C++ official documentation on Standard Libraries
- Books on C++ programming that delve into built-in functions and their applications
- Online tutorials and courses focusing on practical coding skills in C++
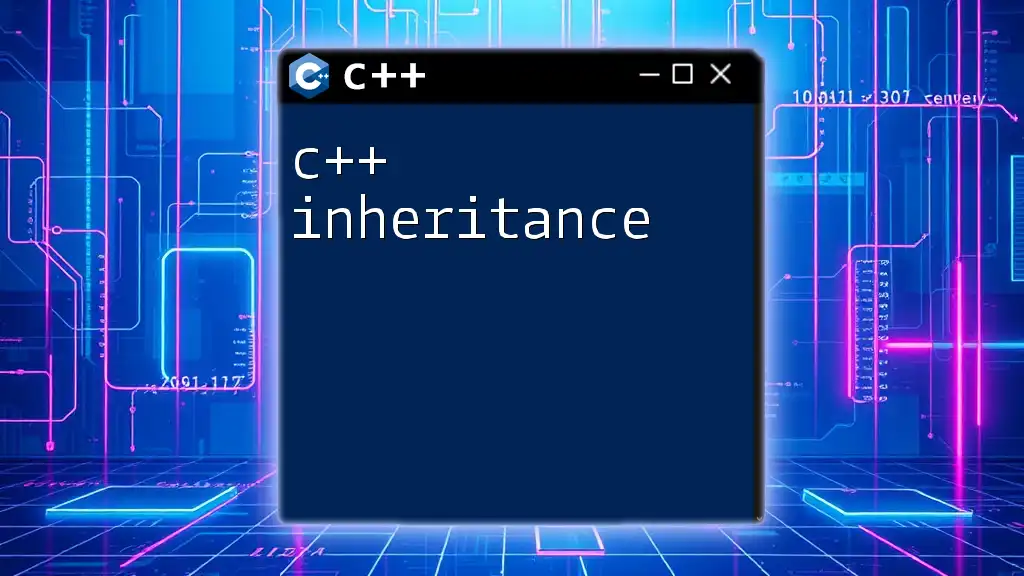
FAQs
What happens if I use min with mixed data types?
Using `min` with mixed data types typically leads to type promotion, where the smaller type is converted to the larger type. This can cause unexpected results; it's best to ensure that both values are of the same type.
Can I use min with more than two values?
While `min` is traditionally designed to compare two values, you can easily achieve this by calling the function several times or using algorithms from the Standard Library, such as `std::min_element`, when dealing with a collection of data.