In C++, the `min` and `max` functions are used to determine the smaller and larger of two values, respectively.
#include <iostream>
#include <algorithm> // Need to include this for min and max
int main() {
int a = 5, b = 10;
std::cout << "Min: " << std::min(a, b) << "\n"; // Outputs: Min: 5
std::cout << "Max: " << std::max(a, b) << "\n"; // Outputs: Max: 10
return 0;
}
Understanding Min and Max in C++
What Are Min and Max Functions?
In programming, especially in C++, the concepts of minimum and maximum values are essential for comparisons, sorting algorithms, and data analysis. These functions help us easily determine the smallest and largest values in a set or between two variables, making our code cleaner and more efficient.
Built-In Min and Max Functions
C++ provides built-in functions to find minimum and maximum values through `std::min` and `std::max`, which are defined in the `<algorithm>` header. These functions can simplify comparing values and retrieving the desired output without writing verbose code.
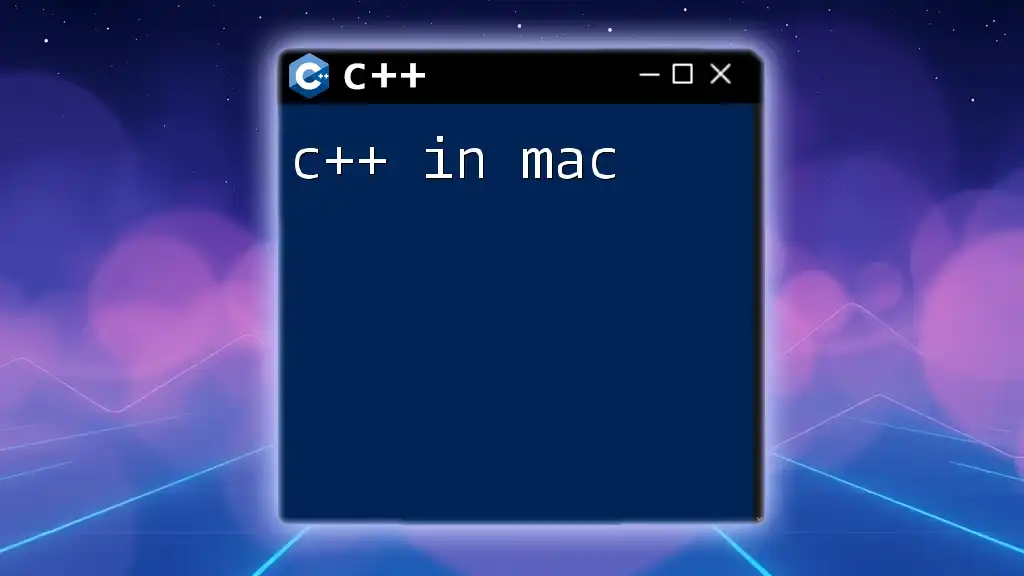
How To Use Min and Max in C++
Basic Syntax
Using `std::min` and `std::max` is straightforward. You call the functions by passing the values or variables for which you want to determine the minimum or maximum.
Here’s a simple example demonstrating how to use these functions:
#include <iostream>
#include <algorithm>
int main() {
int a = 5, b = 10;
std::cout << "Min: " << std::min(a, b) << ", Max: " << std::max(a, b) << std::endl;
return 0;
}
In this example, the program will output:
Min: 5, Max: 10
Function Overloading
`std::min` and `std::max` can handle various data types such as integers, floats, and even user-defined types. This flexibility allows them to be used broadly.
Here’s an example with floating-point numbers:
float x = 3.5, y = 2.2;
std::cout << "Min: " << std::min(x, y) << ", Max: " << std::max(x, y) << std::endl;
In this case, the output will be:
Min: 2.2, Max: 3.5
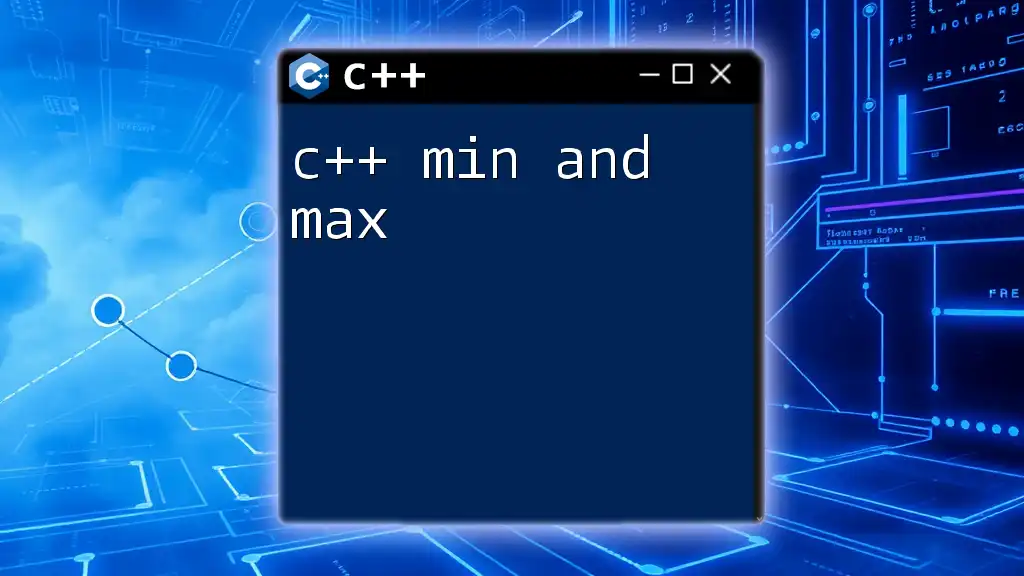
Using Min and Max with Containers
Using Min and Max with Vectors
C++ STL containers, like `std::vector`, allow us to store collections of data. To find the minimum and maximum values within a vector, we can use `std::min_element` and `std::max_element`.
Here’s how you can implement this:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> numbers = {5, 9, 3, 8};
auto minVal = *std::min_element(numbers.begin(), numbers.end());
auto maxVal = *std::max_element(numbers.begin(), numbers.end());
std::cout << "Min: " << minVal << ", Max: " << maxVal << std::endl;
return 0;
}
This code snippet produces the following output:
Min: 3, Max: 9
Finding Min and Max in Other STL Containers
Using `std::min` and `std::max` functions can also be applied to other STL containers, such as `std::list` or `std::set`. For example, to find the min and max values in a `std::set`, you would use the same `std::min_element` and `std::max_element` functions, taking into consideration that sets maintain unique elements.
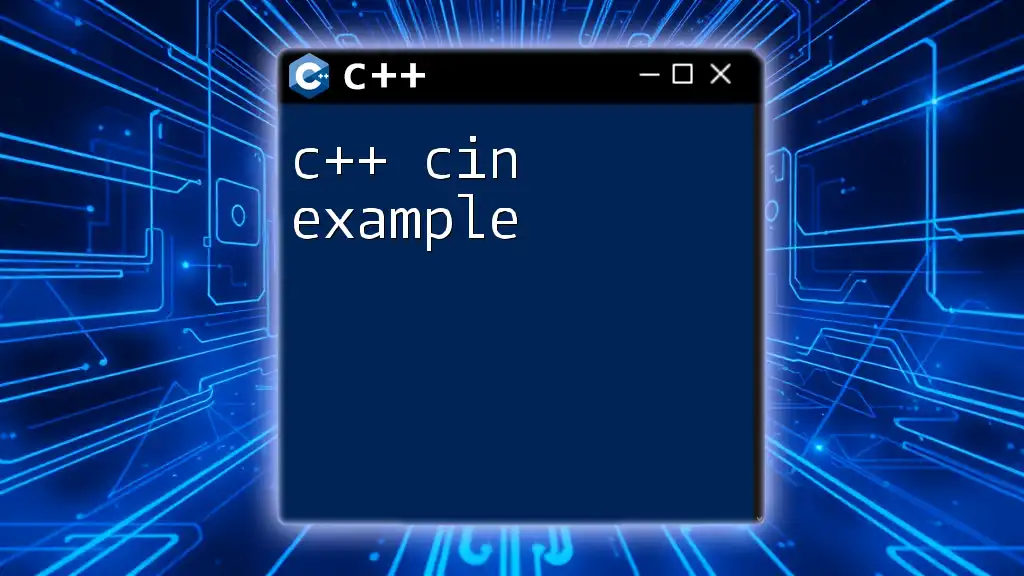
Advanced Use Cases
Lambda Functions with Min and Max
C++ allows the use of lambda functions, which are anonymous functions that can be defined in-place. This capability provides customization for how we determine minimums and maximums.
Here’s an example where we use a lambda function to find a custom minimum in a vector:
#include <vector>
#include <algorithm>
#include <iostream>
int main() {
std::vector<int> nums = {2, 4, 1, 6};
auto customMin = *std::min_element(nums.begin(), nums.end(),
[](int a, int b) { return a % 2 < b % 2; });
std::cout << "Custom Min: " << customMin << std::endl;
return 0;
}
In this code, the custom minimum is determined based on the parity of the numbers (even vs. odd).
Min and Max with Comparisons
For more complex data types, such as structures or classes, you can define your own comparison logic. The following example illustrates how to use custom objects with `std::max`:
#include <iostream>
#include <algorithm>
struct Object {
int value;
};
int main() {
Object obj1 {10};
Object obj2 {20};
auto maxObj = std::max(obj1, obj2, [](const Object& a, const Object& b) {
return a.value < b.value;
});
std::cout << "Max Object Value: " << maxObj.value << std::endl;
return 0;
}
In this case, the output will be:
Max Object Value: 20
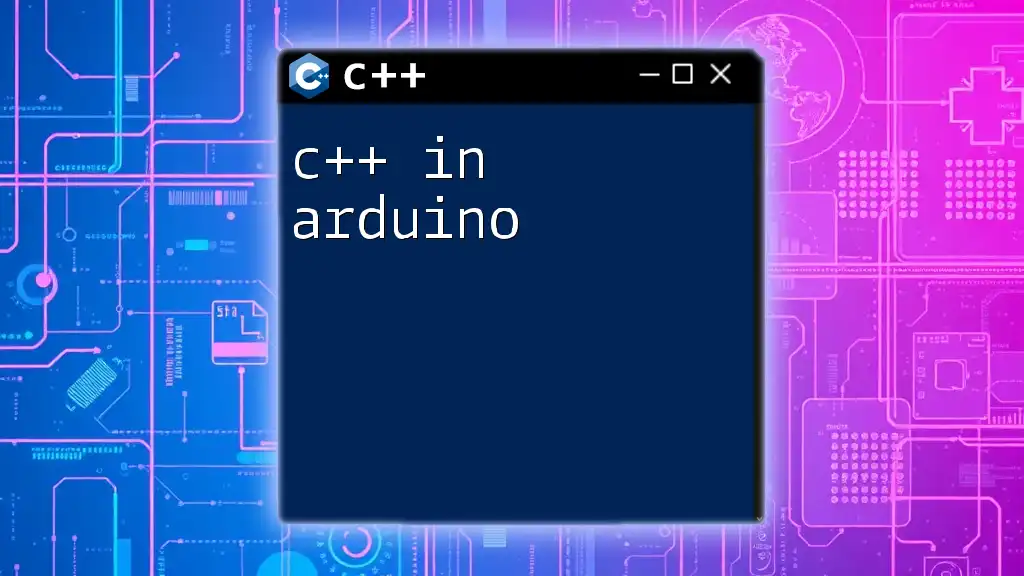
Best Practices
When to Use Min and Max
The built-in `std::min` and `std::max` functions are potent tools that should be employed when you need to compare two values quickly. Their usage simplifies code, enhances readability, and minimizes errors compared to manual comparisons.
However, be cautious about overusing these functions in performance-sensitive contexts. In cases where you call them within loops or time-critical functions, consider evaluating their impact on performance.
Error Handling
When using min and max, especially with containers, ensure that the container is not empty before calling `min_element` or `max_element`, as this can lead to undefined behavior. Check the size of the container first:
if (!numbers.empty()) {
auto minVal = *std::min_element(numbers.begin(), numbers.end());
// Further processing...
}
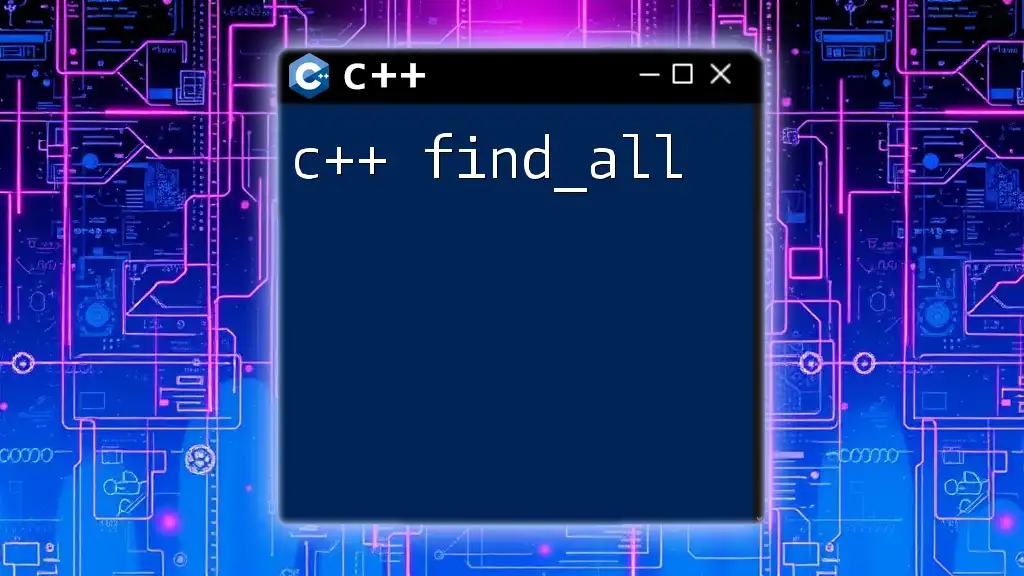
Conclusion
In this comprehensive guide about C++ min max, we explored the significance and usage of `std::min` and `std::max`, including how they operate with basic data types and STL containers. Additionally, we touched upon advanced usage with lambda functions and custom comparison logic. Understanding how to effectively use these functions will enhance your efficiency and coding style in C++. Practice applying these concepts in different scenarios to build your proficiency in C++ programming.