The "C++ Ninja" program equips individuals with swift and effective techniques to master C++ commands, enhancing their coding skills and confidence.
Here's a simple code snippet demonstrating a basic use of a C++ command to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Ninja?
A C++ Ninja is not just someone who can write code but is a master in utilizing the full potential of C++. This term encapsulates developers who have honed their skills through practice, learning, and a problem-solving mindset. They possess the ability to tackle complex issues efficiently, write optimized code, and understand the inner workings of the language.
Characteristics of a Skilled C++ Developer
-
Deep Understanding of Core Concepts: Knowledge of variables, data types, control structures, functions, and object-oriented programming frameworks is paramount.
-
Mastery Over Advanced Topics: A truly skilled C++ Ninja is also familiar with advanced topics such as smart pointers, templates, and exception handling.
-
Problem Solving Approach: They possess an analytical mindset that allows them to break complex problems into manageable parts, applying the most efficient solutions.
The journey to becoming a C++ Ninja is paved with continuous learning, hands-on practice, and engagement with the development community.
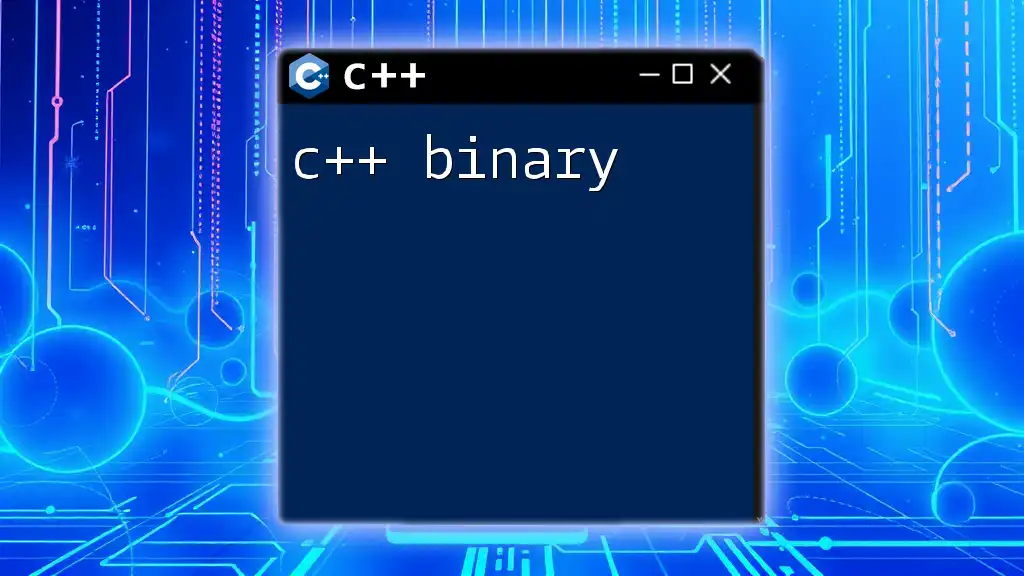
Core Concepts Every C++ Ninja Should Know
Variables and Data Types
In C++, variables serve as storage for data that your programs manipulate. Understanding the various data types available will empower you to store information efficiently.
Common data types include:
int age = 25; // Integer
double height = 5.9; // Floating-point
char grade = 'A'; // Character
bool isStudent = true; // Boolean
When developing software applications, choosing the right data type is essential for memory management and performance optimization.
Control Structures
Control structures provide a way to dictate the flow of program execution.
Conditional Statements
Conditional statements like `if`, `else if`, and `else` allow a program to take different actions based on conditions. Understanding how to utilize these effectively is a critical skill for any C++ Ninja.
Example:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
In this snippet, the program checks if the `age` variable meets a specific condition and prints a corresponding message.
Loops
Loops allow repetitive execution of code blocks as long as a specified condition is true. C++ offers various looping mechanisms such as `for`, `while`, and `do while`.
Example:
for (int i = 0; i < 5; i++) {
cout << i;
}
This loop prints the numbers 0 through 4, demonstrating how to control repetition in your code.
Functions
Functions are essential building blocks in C++. They encapsulate code so it can be reused, improving readability and maintainability.
Function Definition and Invocation
Defining a function means outlining its purpose and how it will be executed. Learning to define and call functions properly magnifies a developer's effectiveness.
Example:
void greet() {
cout << "Hello, C++ Ninja!";
}
By calling `greet();`, the message will be printed to the console. A C++ Ninja values functions for their ability to modularize code.
Object-Oriented Programming (OOP)
Object-Oriented Programming is pivotal to C++. It allows developers to create classes and objects, fostering code reusability and organization.
Classes and Objects
A class is a blueprint for creating objects, encapsulating properties and behaviors. Understanding how to implement classes is indispensable for a C++ Ninja.
Example:
class Ninja {
public:
void training() {
cout << "Training in C++!";
}
};
Here, the `Ninja` class has a method `training` that prints a message. Creating instances of this class allows diverse functionalities while maintaining a cohesive structure.
Inheritance and Polymorphism
Inheritance allows one class to inherit properties and methods from another, promoting code reuse and reducing redundancy.
Example of inheritance:
class AdvancedNinja : public Ninja { };
Polymorphism, on the other hand, enables methods to do different things based on the object calling them, adding flexibility to your code.
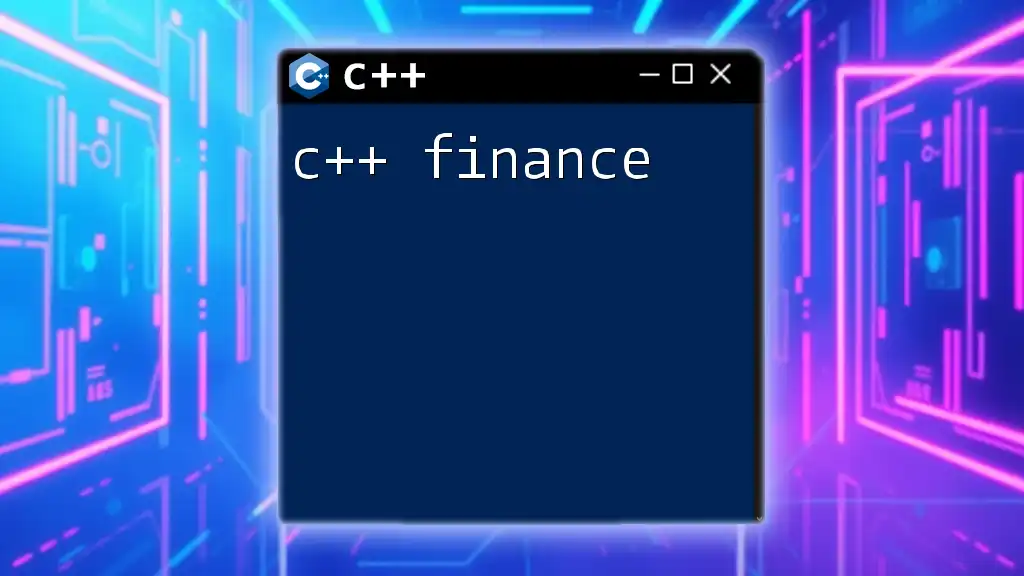
Advanced C++ Concepts
Smart Pointers
Memory management is crucial in C++. Smart pointers help mitigate memory leaks and dangling pointers by automatically managing memory allocation and deallocation.
Types of smart pointers include `unique_ptr`, `shared_ptr`, and `weak_ptr`. Here's an example using `unique_ptr`:
std::unique_ptr<int> ptr(new int(5));
By using smart pointers, you can ensure that memory is properly managed within your applications.
Templates
Templates are a feature that allows functions and classes to operate with generic types. They enable developers to write code that is more flexible and reusable.
Example of a template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
Here, the `add` function can accept different data types and still work seamlessly, showcasing the power of generic programming.
Exception Handling
Exception handling is essential for improving the robustness of your C++ code. It allows developers to manage unexpected events and errors without crashing the program.
Example of a try-catch block:
try {
// risky code
} catch (const std::exception& e) {
cout << e.what();
}
This notation ensures that if an exception occurs in the `try` block, it can be caught and handled gracefully.
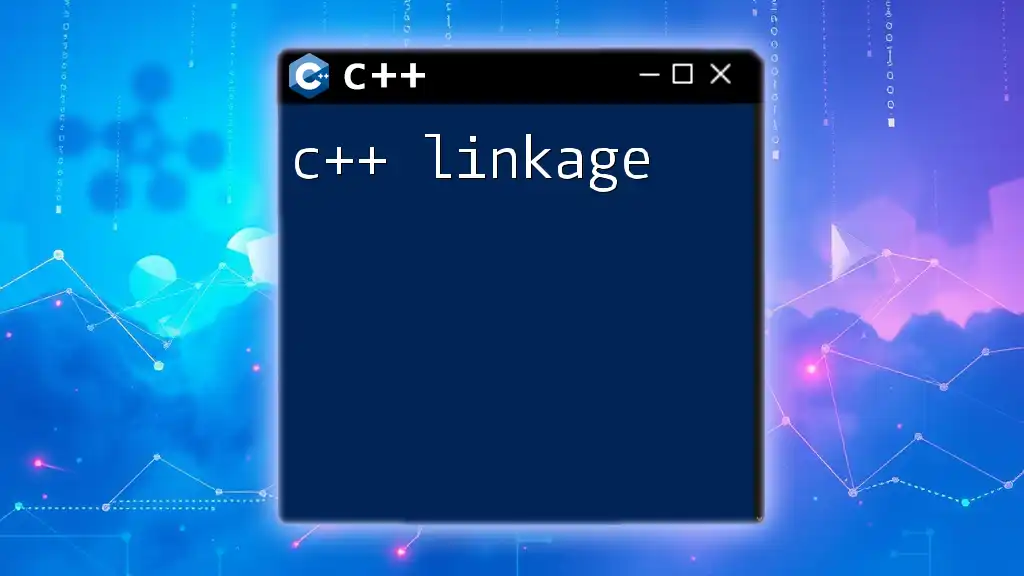
Coding Practices to Become a C++ Ninja
Code Readability and Organization
Writing clean, organized code should be a priority for any C++ Ninja. Maintainability becomes crucial over time, so ensure your code is easy to read and understand. Follow consistent naming conventions, comment on complex logic, and apply modular design principles.
Continuous Learning and Resources
In the rapidly evolving tech landscape, staying current is vital. Here are some popular resources for further learning:
- Books: Consider "The C++ Programming Language" by Bjarne Stroustrup or "Effective C++" by Scott Meyers.
- Websites: Engage in platforms like Codecademy, LeetCode, and Udacity for structured courses and challenges.
- Community: Join forums like Stack Overflow, Reddit's r/cpp, and GitHub repositories to connect and share knowledge with fellow developers.
Real-world Application and Projects
Hands-on experience is invaluable. Work on real-world projects to apply your skills practically. Consider contributing to open-source projects, developing applications, or creating personal projects. This practice not only sharpens your abilities but also builds your portfolio.
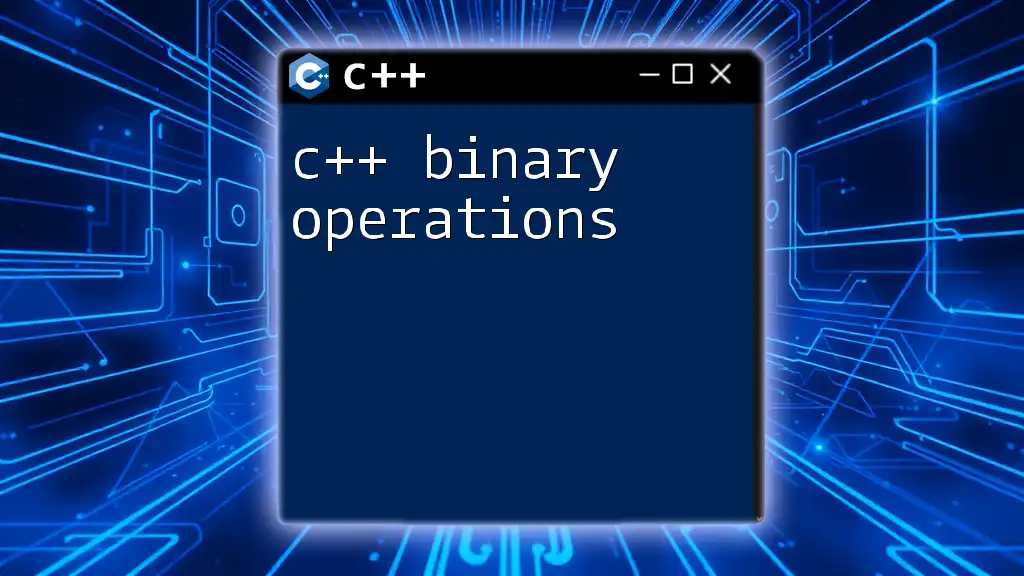
Conclusion
Becoming a C++ Ninja is a continuous journey of learning, practice, and engagement. Mastering the core concepts, delving into advanced topics, and consistently writing clean code will arm you with the tools necessary to excel in C++. Remember that every expert was once a beginner, so embrace the challenges along the way.
Join the community, share your insights, and immerse yourself in the skillful art of C++ programming!