In C++, `int&` denotes a reference to an integer variable, allowing you to create an alias for an existing integer variable without making a copy. Here's an example:
#include <iostream>
void increment(int& number) {
number++; // Increments the original integer
}
int main() {
int x = 5;
increment(x); // x is now 6
std::cout << x << std::endl; // Outputs: 6
return 0;
}
Understanding int& in C++
The `int&` in C++ denotes a reference type, specifically a reference to an integer. While it may seem simple, it plays a crucial role in how we work with variables and memory.
An int is a standard data type representing integer values, while int& (read as "int reference") provides a more dynamic link to that data without creating a copy. This distinction becomes crucial in various programming situations, particularly when efficiency and performance are paramount.
When to Use int& in Your Programs
Using `int&` can be particularly beneficial when you need to modify a variable directly without the overhead of copying data. This is especially useful in:
- Function parameters, where you want to change the input variable.
- Return types in functions when you want a function to provide direct access to a variable that may be stored elsewhere in memory.
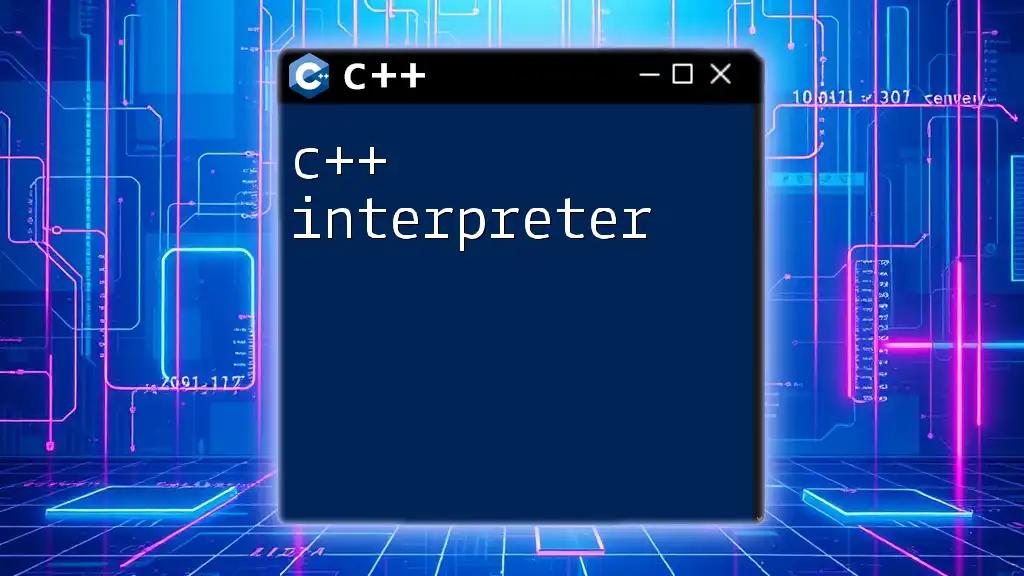
Creating and Using int& Variables
Declaring Reference Variables
To declare a reference variable, you simply follow the syntax:
int& referenceVariable = originalVariable;
For example:
int original = 10;
int& reference = original;
In this snippet, `reference` acts as an alias for `original`. Any changes made to `reference` will be reflected in `original` since they are two names for the same memory location.
Modifying Values via References
One of the most powerful features of `int&` is its ability to modify the original variable directly. For instance:
reference = 20; // This changes the value of 'original'
std::cout << original; // Outputs: 20
In this case, assigning a new value to `reference` automatically updates `original`. This direct connection can lead to more readable code, especially when passing variables around functions.
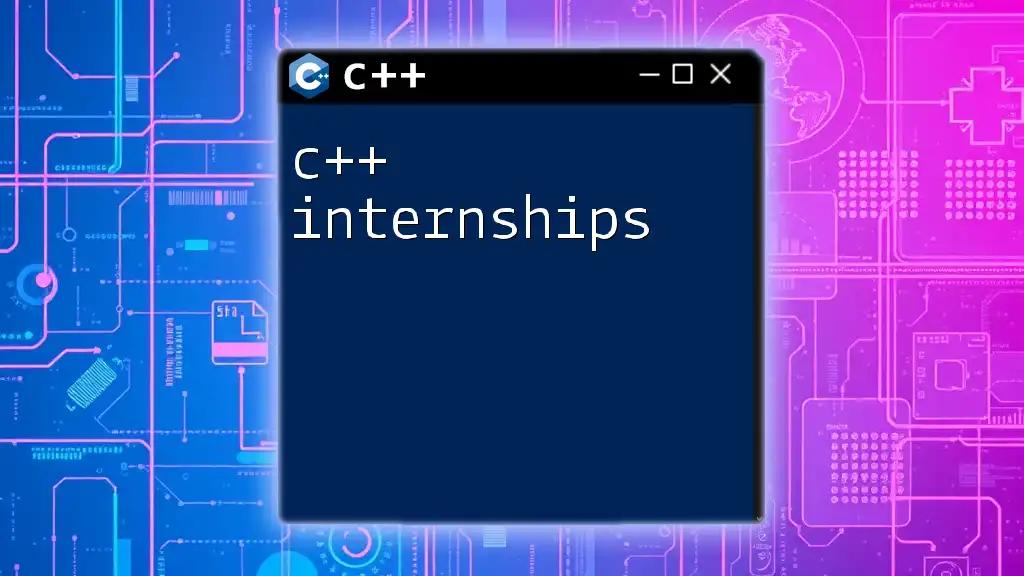
Benefits of Using int&
Using `int&` offers several advantages, including:
-
Memory Efficiency: When functions accept arguments by reference, they avoid creating copies of data, which can consume additional memory and time, especially with large structures.
-
Performance Considerations: Passing references allows for faster function calls, as the overhead of copying data is eliminated.
-
Code Clarity: By using references, your function signatures can indicate that a variable will be modified, leading to clearer and more maintainable code.

Common Use Cases for int&
Parameter Passing in Functions
An excellent way to utilize `int&` is when passing arguments to functions. Instead of passing by value, you can pass by reference:
void modifyValue(int& num) {
num += 5;
}
In this example, if we call `modifyValue(original)`, the value of `original` will increase by 5.
Returning References from Functions
While returning references can be powerful, caution is necessary to avoid potential pitfalls. Here’s an example:
int& getValue(int& num) {
return num; // Returns a reference to 'num'
}
This allows you to directly manipulate the original variable outside the function. However, be mindful when returning references to local variables since they become invalid once the function scope ends.

Potential Pitfalls of Using int&
While references are powerful, they can also lead to issues if not handled properly.
Dangling References
A dangling reference arises when you attempt to access a variable that has gone out of scope. For example:
int& danglingRef() {
int localVariable = 10;
return localVariable; // Dangerous! Local variable goes out of scope
}
In this situation, the reference returned will point to `localVariable`, but once the function exits, that memory is no longer valid, leading to undefined behavior.
Reference Binding Rules
Understanding reference binding rules is critical. For instance, you cannot bind a reference to a temporary value:
int& ref = 10; // Error! Cannot bind a reference to a temporary
This code results in a compilation error because literals (such as `10`) are temporary and cannot be referenced.
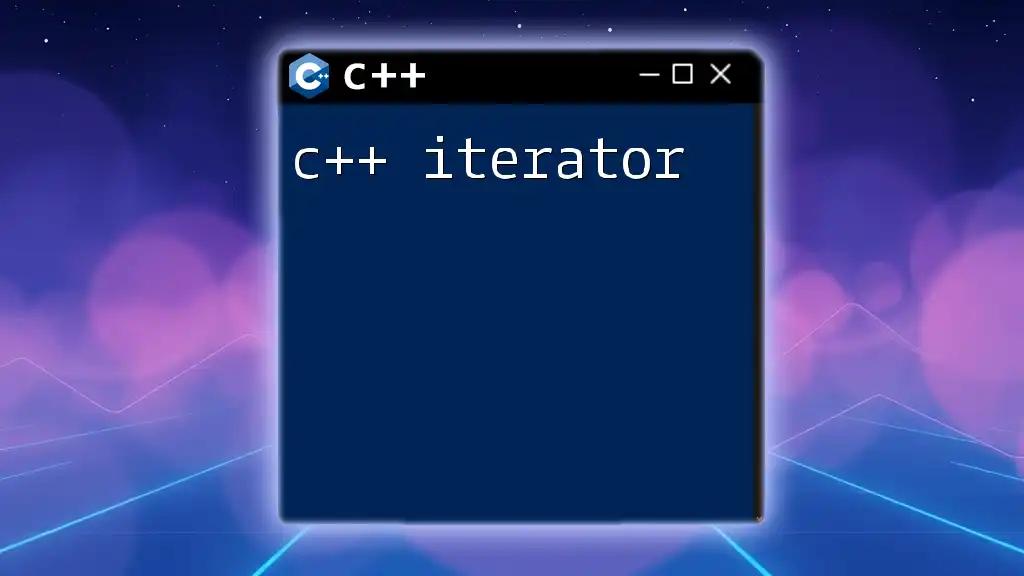
Best Practices for Using int&
To effectively utilize `int&`, follow these best practices:
-
Use `const int&` when you don’t need modification. It is beneficial for passing large data types, allowing you to avoid unnecessary copies while protecting the original data from being altered.
-
Ensure clarity in your functions. Use `int&` judiciously within loops and iterators to ensure that the code remains clear and purposeful.
-
Consider pointers for dynamic arrays or when handling optional arguments. It’s essential to know when a pointer might be more appropriate than a reference, especially when the possibility of null values exists.
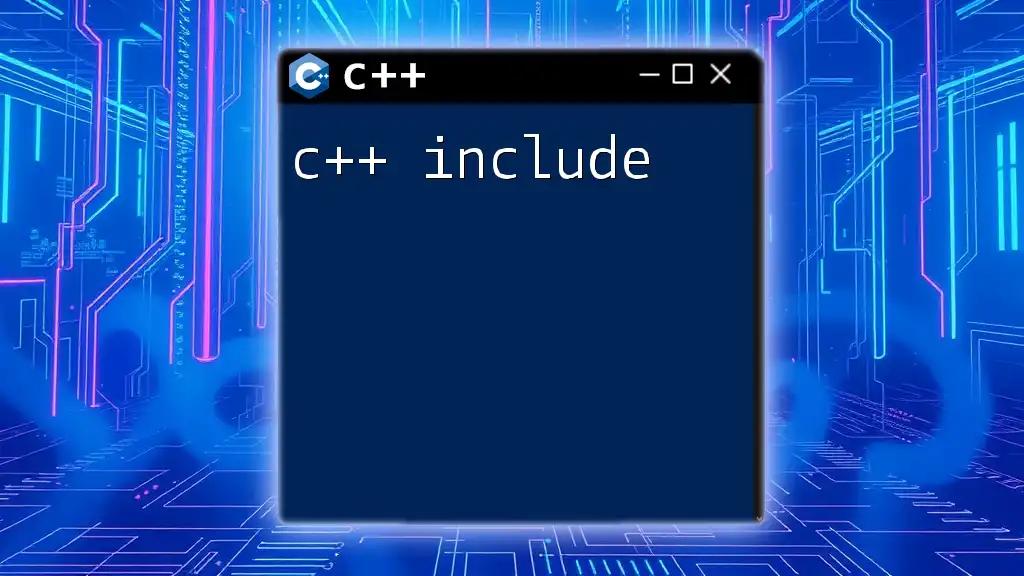
Advanced Concepts with int&
Reference Collapsing
When dealing with references, it’s important to understand reference collapsing rules. For instance, the interaction between lvalue references and rvalue references can lead to different behaviors.
The types `int&` (lvalue reference) and `int&&` (rvalue reference) behave differently, which can sometimes lead to confusion:
int a = 5;
int& b = a; // lvalue reference
int&& c = 10; // rvalue reference
This demonstrates how `int&&` can bind to temporary values (rvalues), while `int&` can only bind to existing objects (lvalues). This distinction becomes particularly important when working with more complex types and templates.
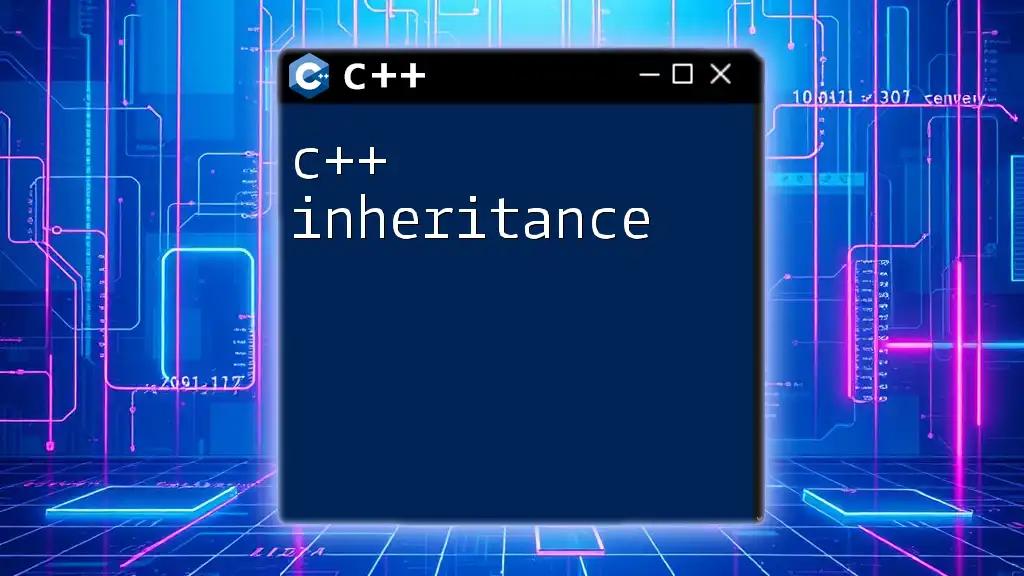
Conclusion
In conclusion, `c++ int&` provides an efficient and powerful way to manipulate variables while minimizing memory usage and enhancing performance. Having a clear understanding of references will not only improve your coding skills but also lead to more robust and maintainable C++ applications. Embrace the versatility of `int&`, and leverage it wisely in your programming endeavors.