Swift C++ interoperability allows developers to use C++ libraries and code within a Swift project, enabling high-performance computing capabilities alongside Swift's modern syntax.
Here’s a simple example of calling a C++ function from Swift using a bridging header:
// Example C++ code (example.cpp)
extern "C" {
int add(int a, int b) {
return a + b;
}
}
Make sure to create a bridging header to expose the C++ function to Swift.
Overview of Swift and C++
What is Swift?
Swift is a modern programming language developed by Apple, emphasizing safety, performance, and readability. It has gained widespread use in iOS and macOS development due to its clean syntax and robust nature. One of the standout features of Swift is its focus on eliminating common programming errors, thanks to its strong typing system and optional types. This allows developers to catch mistakes at compile time rather than at runtime, resulting in safer code.
What is C++?
C++ is a powerful, high-performance programming language that has been around since the 1980s. It is an extension of the C programming language, adding object-oriented features that facilitate code reuse and organization. C++ is renowned for its ability to deliver high-speed performance, making it a popular choice in systems programming, game development, and applications requiring intensive processing. Its rich set of libraries offers a wide range of functionality, which complements its performance advantages.
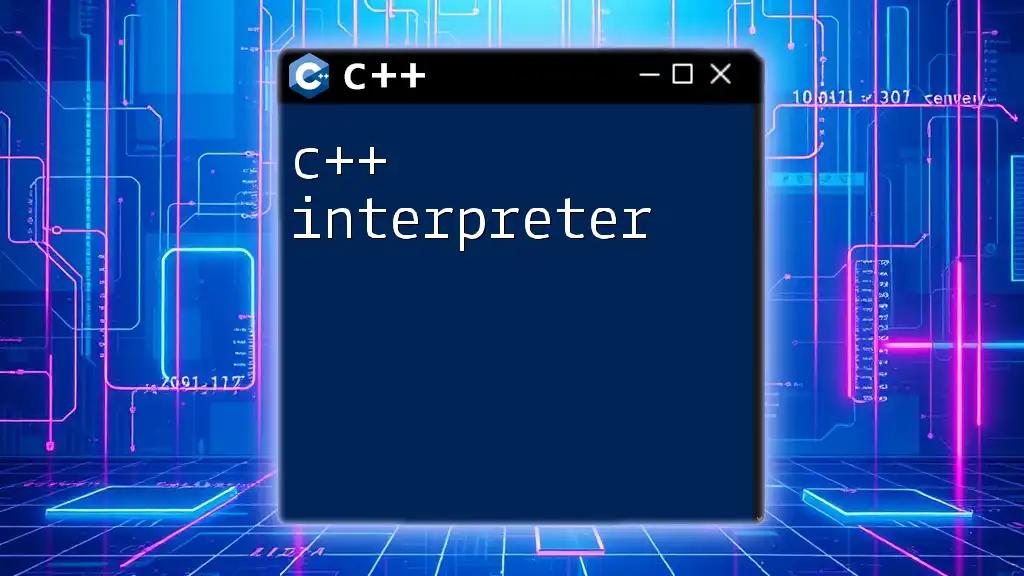
Setting Up Your Environment for Swift C++ Interop
Required Tools and Libraries
Before diving into swift c++ interoperability, you'll need the right tools to bridge the two languages effectively.
Xcode Setup
Xcode is Apple's integrated development environment (IDE) and is essential for Swift development. Make sure you have the latest version of Xcode installed on your macOS. Within Xcode, you'll want to ensure that you have enabled the necessary flags and configurations for working with C++ code.
CMake for C++ Projects
CMake is a cross-platform tool that automates the building process of software. It's especially useful when dealing with C++ projects because it handles the compilation process efficiently. To set up a CMake project for Swift interop, you would typically create a `CMakeLists.txt` file that outlines how to compile your C++ source files.
Creating a Basic Swift Package
To begin using Swift and C++, you’ll first need to initiate a Swift package. Here’s a straightforward process:
- Open the Terminal.
- Navigate to your desired directory and run:
swift package init --type executable
- Upon initialization, you will find a new folder structure that includes `Sources`, `Tests`, and `Package.swift`, which defines your package and its dependencies.

Bridging Swift and C++
Importing C++ Code into Swift
To facilitate the calling of C++ code within Swift, you can use Objective-C as an intermediary. This approach leverages Objective-C’s ability to communicate seamlessly with C++ code.
Using Objective-C as a Bridge
You will need to create a bridging header that imports your C++ code, allowing Swift to recognize and utilize it. For instance, create a file named `BridgingHeader.h`:
// BridgingHeader.h
#import "MyCPPClass.h"
By adding this bridging header to your Swift project, you enable your Swift files to access the C++ classes and functions defined in your C++ headers.
Creating a C++ Class
Example: Simple C++ Class
Imagine you need a simple C++ class that greets users. Define the C++ class in `MyCPPClass.h`:
// MyCPPClass.h
class MyCPPClass {
public:
void greet();
};
Next, implement the class methods in `MyCPPClass.cpp`:
// MyCPPClass.cpp
#include <iostream>
#include "MyCPPClass.h"
void MyCPPClass::greet() {
std::cout << "Hello from C++!" << std::endl;
}
This simple C++ class creates a `greet` function that outputs a message to the console.
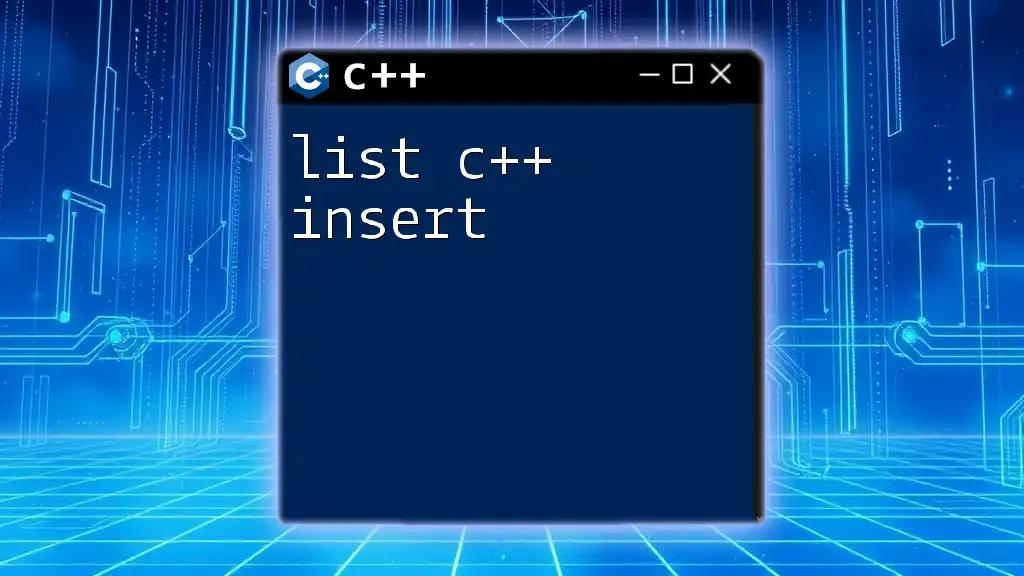
Calling C++ Code from Swift
Using the Bridging Header
Now that the bridging header is in place, you can call your C++ functions from Swift easily. Below is an example of how you can utilize the C++ class within a Swift class:
// MySwiftClass.swift
import Foundation
class MySwiftClass {
func callCPPFunction() {
let cppObject = MyCPPClass()
cppObject.greet()
}
}
In this Swift code, we create an instance of `MyCPPClass` and call the `greet` method, demonstrating the interoperability between Swift and C++.

Sending Data Between Swift and C++
Data Structures Compatibility
When working with swift c++ interoperability, understanding how to pass data back and forth can be crucial. Swift and C++ both support common data types, such as integers and strings; however, they have different representations.
Passing Complex Types
For scenarios requiring more complex types, you’ll need to modify your approach slightly. Here’s how to handle a custom class object:
// MyData.h
class MyData {
public:
int value;
MyData(int v) : value(v) {}
};
You would then create an interface in Swift to allow manipulation of this C++ object:
// MyDataInterface.swift
class MyDataInterface {
func manipulateData(data: MyData) {
print("Value from C++: \(data.value)")
}
}
This demonstrates how a C++ class can be utilized in Swift and how properties of C++ can be accessed within Swift code.

Error Handling in Swift C++ Interop
Managing Exceptions
One of the challenges in swift c++ interoperability is handling exceptions that arise from C++ code. Swift uses a `try-catch` model for error handling, while C++ employs structured exception handling (SEH). To capture C++ exceptions in Swift, you can wrap your C++ calls inside a blocking code segment and ensure any exceptions are appropriately converted into Swift errors.
Best Practices for Robust Interop
To create a robust interoperation environment, adhere to best practices such as:
- Validate inputs when passing data between Swift and C++.
- Always handle potential exceptions in C++ and ensure they don't propagate back into Swift.
- Modularize your code to keep Swift and C++ components organized and manageable.
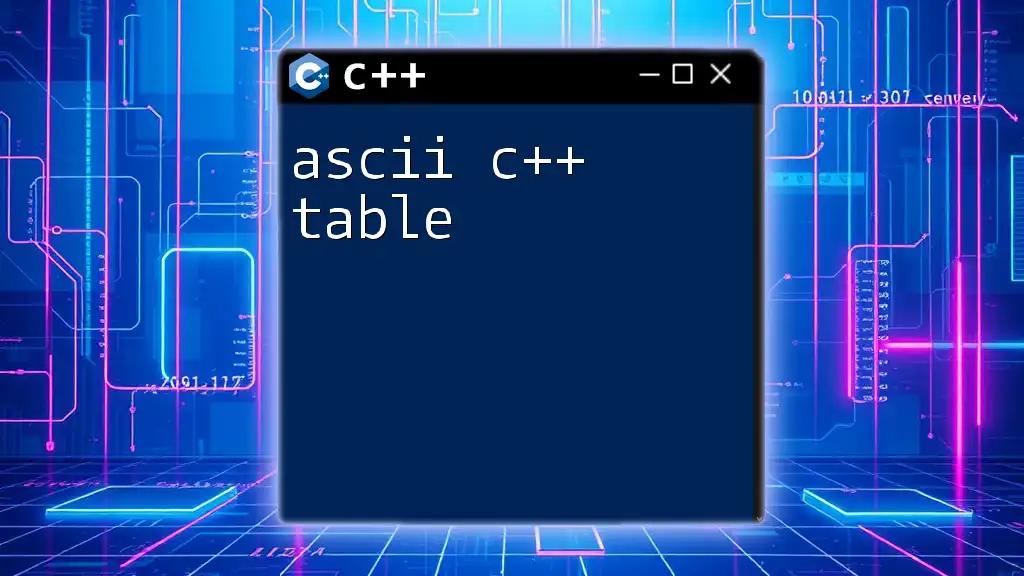
Performance Considerations
Benchmarking C++ Code from Swift
When integrating two languages, evaluating the performance impact is vital. You can employ tools like Xcode’s profiling instruments to measure the efficiency of C++ code called from Swift. Such assessments allow developers to pinpoint performance bottlenecks and adjust their code accordingly.
Memory Management Techniques
Swift's automatic reference counting (ARC) may clash with C++’s manual memory management. This necessitates careful monitoring of allocated resources to avoid memory leaks. Always ensure that C++ objects are deallocated properly, especially when they are created from Swift.
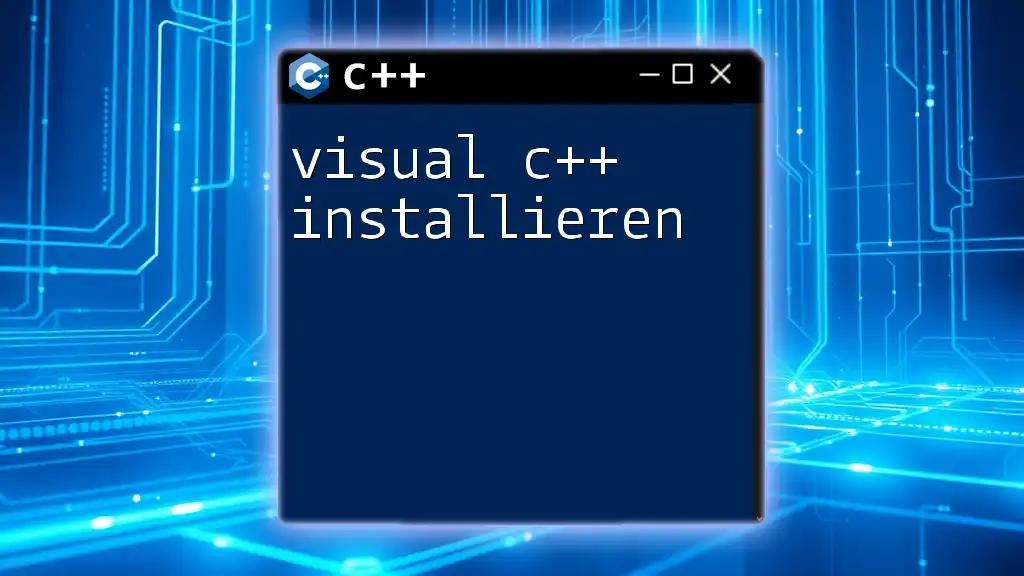
Conclusion
Throughout this exploration of swift c++ interoperability, we've delved into the requirements for setting up an effective environment, the methods of bridging code, and best practices for performance and error handling. The combination of Swift's modern capabilities and C++’s efficiency opens up a world of possibilities for developers looking to leverage the strengths of both languages in their applications.
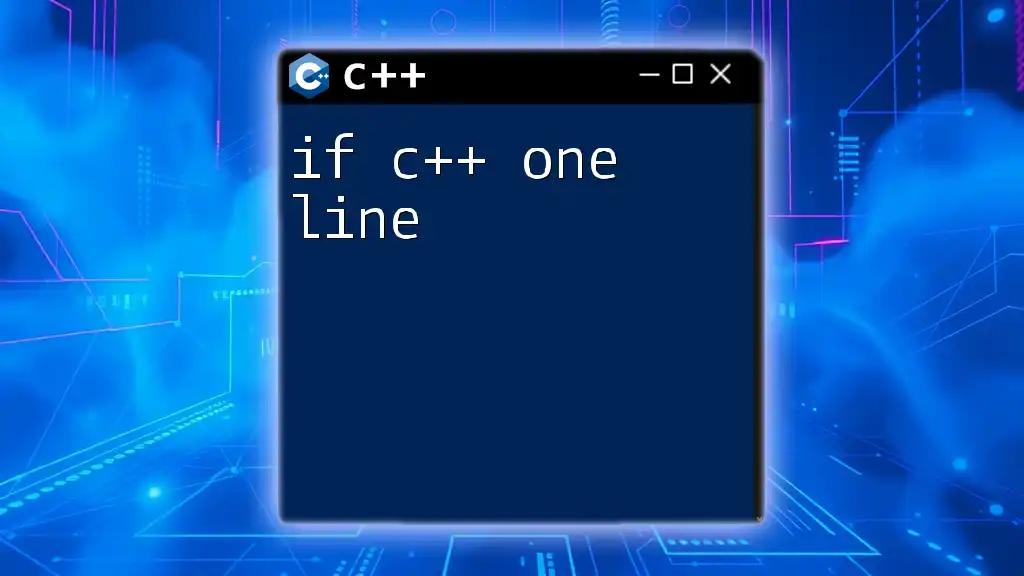
Additional Resources
Learning more about the intricacies of Swift and C++ interoperability can significantly enhance your development skills. You may consider diving into recommended books, online courses, or engaging with community forums where like-minded individuals share knowledge and solutions. These resources will be invaluable as you continue on your journey in mastering swift c++ interoperability.