"Absolute C++ by Walter Savitch offers a comprehensive guide for mastering C++ programming through a focused, practical approach."
Here’s a simple code snippet demonstrating how to define and use a function in C++:
#include <iostream>
using namespace std;
// Function declaration
void greet() {
cout << "Hello, World!" << endl;
}
int main() {
greet(); // Function call
return 0;
}
Understanding C++: The Basics
What is C++?
C++ is a general-purpose programming language that was developed by Bjarne Stroustrup starting in 1979. It provides facilities for low-level memory manipulation and supports various programming paradigms, including procedural, object-oriented, and generic programming. Key features of C++ include strong type checking, a rich set of built-in functions, support for classes and objects, and the ability to seamlessly integrate with C code.
The Importance of Learning C++
Mastering C++ is fundamental for anyone interested in programming due to its influence on many other languages. It remains relevant for various applications, such as:
- Game Development: C++ is widely used in game engines like Unreal Engine due to its performance and control over system resources.
- Systems Software: Many operating systems and system-level applications are written in C++.
- Embedded Systems: C++ is suitable for developing software that operates on embedded systems, vital in IoT devices and automation.

Overview of "Absolute C++"
Book Structure and Key Themes
"Absolute C++" is structured for clarity and progressive learning. The book meticulously breaks down C++ concepts, starting from basic syntax to advanced topics like data structures and algorithms. Each chapter includes numerous examples, practice problems, and coding exercises designed to reinforce the material covered.
Target Audience
This book caters to a wide audience, making it suitable for:
- Beginners: Individuals new to programming can find accessible explanations to ease their learning curve.
- Intermediate Programmers: Those familiar with basic programming concepts can solidify and enhance their C++ knowledge.
- Educators: "Absolute C++" serves as an excellent resource for teaching programming in academic settings.
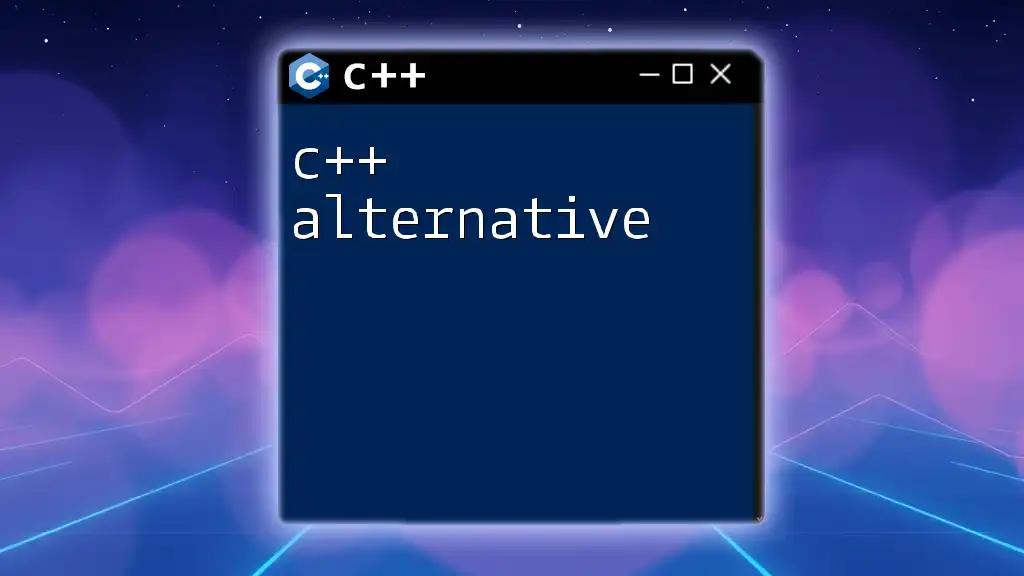
Core Concepts of "Absolute C++"
Data Types and Variables
C++ provides various data types to handle different kinds of data. Understanding these data types is crucial for effective programming. Commonly used data types include:
- int: Represents integer values.
- double: Used for floating-point values.
- char: For single characters.
For example, you can declare data types as follows:
int age = 30;
double salary = 85000.50;
char grade = 'A';
Employing the correct data type not only determines how data is stored but also helps in optimizing memory usage and program efficiency.
Control Structures
Conditional Statements
Conditional statements like `if-else` and `switch-case` allow your program to execute different codes based on certain conditions. This adds dynamism and decision-making capability to programs. An example of an `if-else` statement is:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Loops
Loops are essential for executing a set of instructions repeatedly. C++ supports different types of loops:
- For Loop: Ideal when the number of iterations is known.
- While Loop: Useful when the number of iterations is not predetermined.
Here’s a simple example using a for loop:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
Functions
Definition and Declaration
Functions in C++ are blocks of code designed to perform specific tasks. They help in breaking down complex problems into smaller, manageable parts. Here’s how to define a function:
int add(int a, int b) {
return a + b;
}
This function takes two integer arguments and returns their sum.
Function Overloading
C++ allows the creation of multiple functions with the same name but different parameter lists, known as function overloading. This enhances the readability of the code. For instance:
void print(int number);
void print(double number);
Both functions can coexist, and the correct one will be called based on the passed argument type.
Object-Oriented Programming Concepts
Classes and Objects
C++ supports Object-Oriented Programming (OOP), which is centered around the concept of "objects" that combine data and methods. Classes serve as blueprints for creating objects. For example:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Here, `Dog` is a class, and `bark()` is a method that defines a behavior of the Dog object.
Inheritance and Polymorphism
OOP principles like inheritance and polymorphism empower developers to build programs more efficiently. Inheritance enables one class to inherit properties and methods from another. Here's a concise example:
class Animal {}; // Base class
class Dog : public Animal {}; // Dog inherits from Animal
Polymorphism allows objects to be treated as instances of their parent class, especially useful in defining interfaces and implementing dynamic behavior.
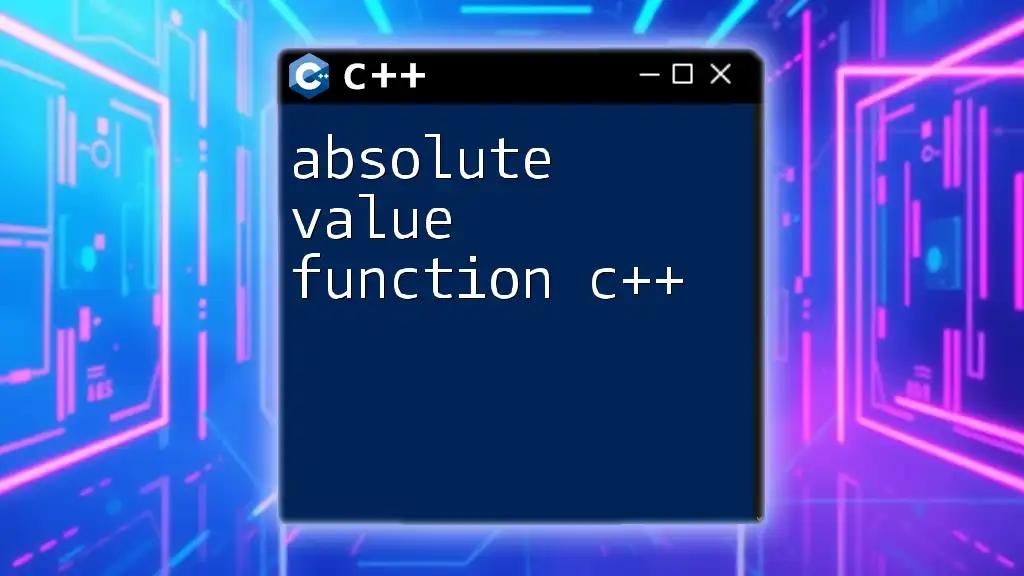
Practical Applications of C++ from "Absolute C++"
Real-World Examples
"Absolute C++" grounds theoretical concepts in practical application, presenting projects such as simple games or dynamic data structures. These examples highlight how the language can be employed in real-world scenarios.
Hands-On Exercises
To reinforce the concepts learned, the book includes a variety of hands-on exercises. For instance, students might undertake projects such as:
- Developing a basic calculator.
- Creating a simple text-based game.
- Implementing algorithms, such as sorting and searching.
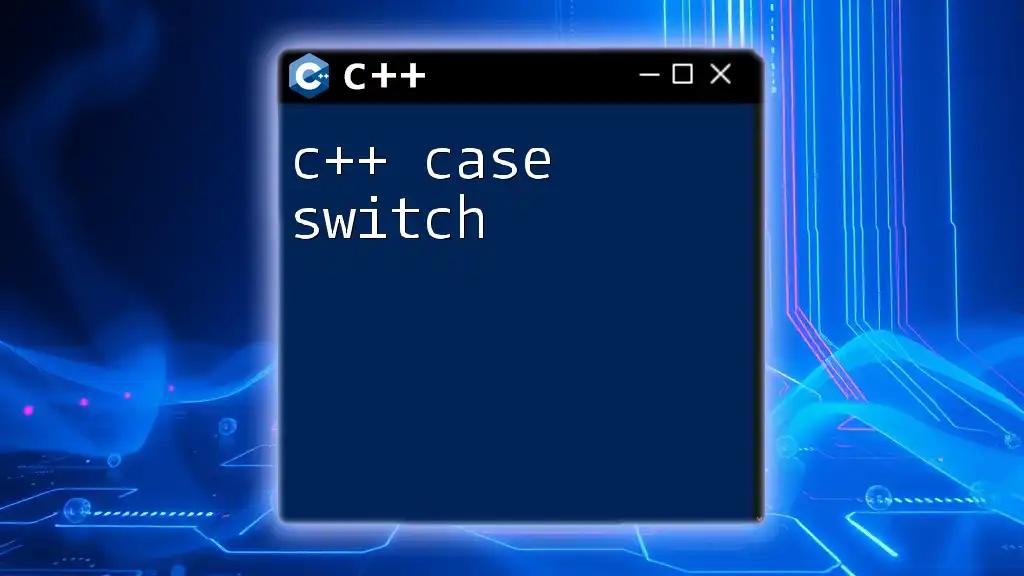
Supplementary Learning Resources
Online Platforms
To complement the teachings from "Absolute C++," online platforms like Codecademy, Coursera, and LeetCode offer additional C++ tutorials and practice problems.
Community and Forums
Engagement in programming communities such as Stack Overflow or the C++ subreddit can further enhance learning. Asking questions, sharing knowledge, and collaborating with others can greatly accelerate one’s understanding.

Tips for Effective Learning
Boosting Retention with Short Learning Sessions
It’s vital to structure your study time efficiently. Rather than marathon study sessions, focus on shorter but concentrated bursts of learning. This method enhances focus and retention, helping to solidify your understanding of complex concepts.
Utilizing Code Snippets Efficiently
Manipulating and experimenting with code snippets from "Absolute C++" fosters practical understanding. It encourages experimentation, allowing learners to see real-time effects of changes in the code.

Conclusion
"Absolute C++" by Walter Savitch stands as an exceptional resource for anyone keen on learning C++. It combines theoretical knowledge with practical exercises, assisting learners in building a strong foundation in programming principles. With committed practice and engagement, anyone can achieve proficiency in C++.
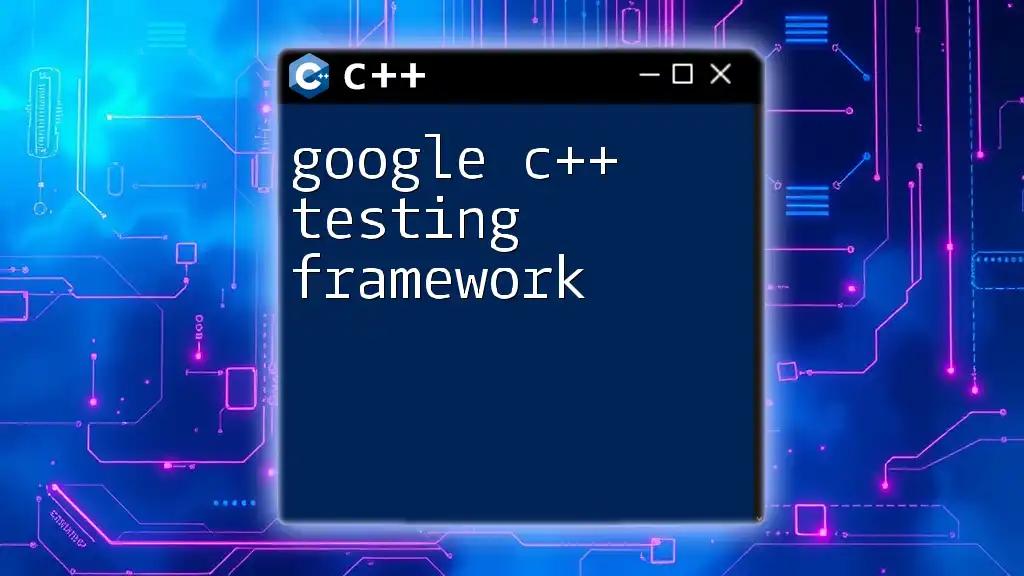
Call to Action
Are you ready to take your C++ skills to the next level? Join our C++ learning program today and transform your understanding of programming with guided support and resources.
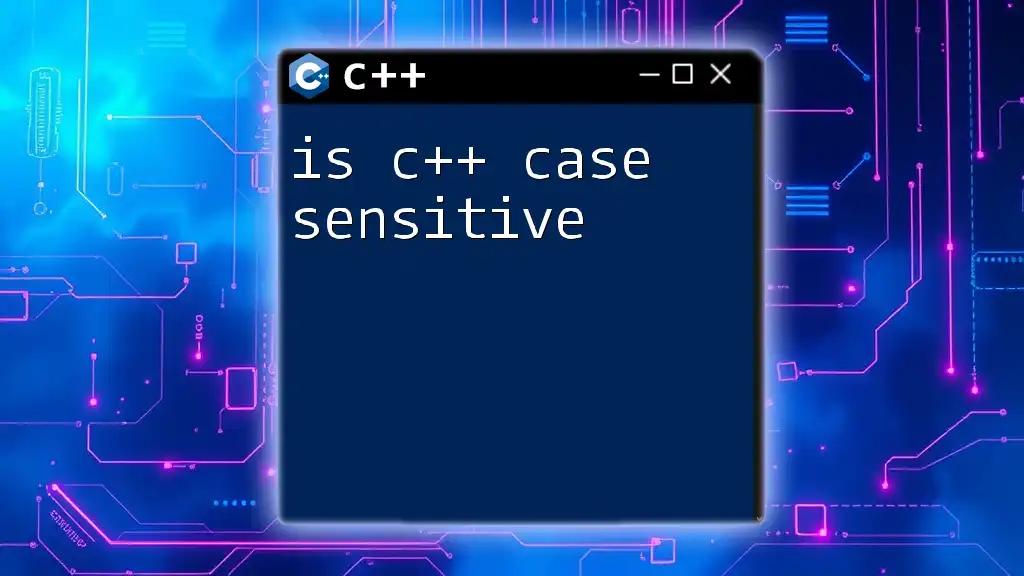
FAQs about "Absolute C++"
Common queries about "Absolute C++" often relate to its approach, the depth of coverage for various topics, and recommendations for supplementary resources to further enhance learning. Exploring these questions can deepen your understanding and clarify any uncertainties about the book and its materials.