C++ libraries are pre-written code collections that developers can use to perform common tasks more efficiently, enhancing productivity and enabling the creation of robust applications.
Here's an example code snippet showcasing the use of the Standard Template Library (STL) in C++:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1, 2};
std::sort(numbers.begin(), numbers.end());
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is a C++ Library?
A C++ library is a collection of precompiled reusable code that programmers can use to optimize their development process. Libraries provide a way to access existing functionalities without needing to write code from scratch.
There are two main types of C++ libraries:
- Static Libraries: These are linked to the program at compile time. They become part of the binary file, increasing the final size, but you don’t need to distribute the library separately.
- Dynamic Libraries: Also known as shared libraries, they are linked at runtime. They help in reducing the binary size and allow multiple programs to share the same library code in memory.
Leveraging libraries is crucial in software development as they can dramatically reduce development time while enhancing performance and maintainability.
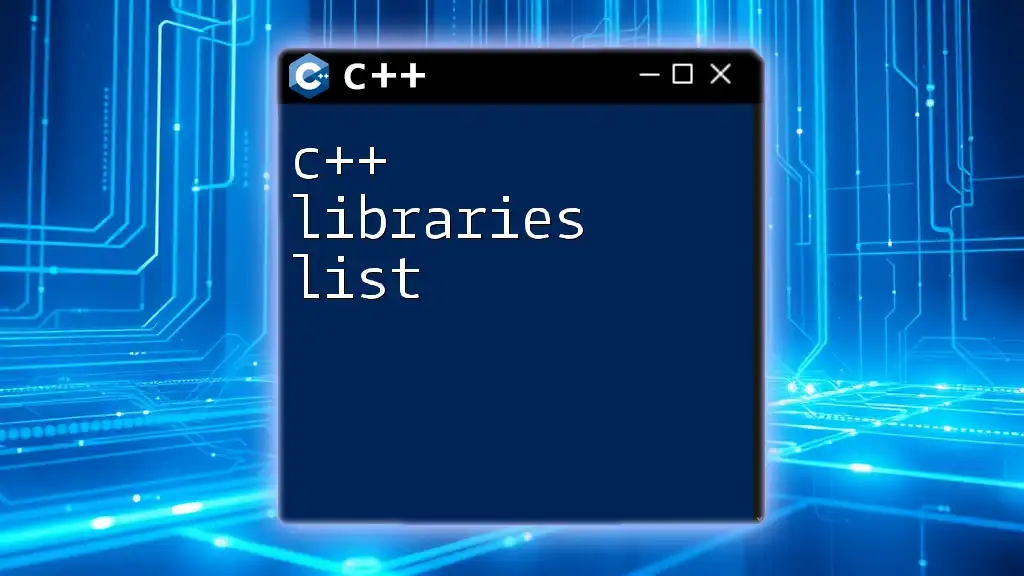
Criteria for Choosing a C++ Library
Performance
Performance is a critical aspect when selecting a library. A library with efficient algorithms and data structures can lead to significant time savings during execution. High-performance libraries, like Boost and STL, are well-optimized to handle various tasks effectively.
Usability
Usability refers to how easy it is to integrate and use a library in your project. A more user-friendly library often includes thorough documentation and clear examples that make it easier for developers to understand how to implement its functionalities. Code snippets demonstrating straightforward integration can ease the learning curve.
Community Support and Documentation
A vibrant community and comprehensive documentation greatly enhance a library's usability. Libraries with active support forums or resources are more likely to help troubleshoot problems or offer advice. Well-documented libraries reduce the time spent searching for solutions and foster a smoother learning experience.
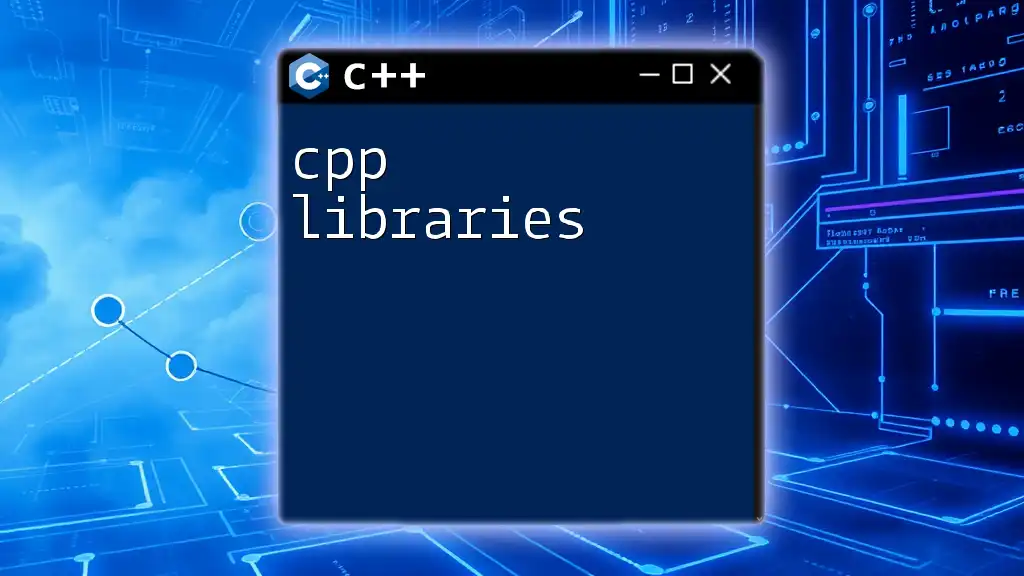
Top C++ Libraries to Consider
Boost
Boost is widely recognized as one of the best C++ libraries. It provides numerous components for tasks ranging from smart pointers to regex operations and even threading. This extensive collection ensures that developers can find optimized solutions for many common programming needs.
- Key Features: Boost offers powerful tools like:
- Smart pointers for safe memory management
- Various libraries, such as Boost.Regex for regular expressions
- Asynchronous programming with Boost.Asio
Example of Using Boost's Smart Pointers:
#include <boost/shared_ptr.hpp>
void example() {
boost::shared_ptr<int> p1(new int(10));
// Smart pointer automatically deletes memory when out of scope
}
Use Cases: Boost is ideal when you need high-performance solutions and advanced functionalities beyond the standard library, especially in complex applications.
Qt
Qt is a prominent C++ framework primarily focused on building Graphical User Interfaces (GUIs). It is cross-platform, allowing developers to write once and deploy on multiple OS platforms, such as Windows, macOS, and Linux.
- Key Features: Qt is revered for:
- Rich set of UI components and features, such as buttons, sliders, and menus
- Integrated development tools and support for 2D and 3D graphics
Simple Qt GUI Example:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello World!");
button.show();
return app.exec();
}
Use Cases: Use Qt when developing applications demanding a rich graphical interface, such as GUI applications, mobile apps, and even embedded systems.
STL (Standard Template Library)
The Standard Template Library (STL) is an essential component of modern C++. It provides a set of common classes and interfaces for data structures and algorithms.
- Key Components: STL includes:
- Containers (e.g., vectors, lists, maps)
- Algorithms (e.g., sort, search)
- Iterators that allow traversing data structures
Example of Using STL Vectors:
#include <vector>
#include <iostream>
int main() {
std::vector<int> v = {1, 2, 3, 4, 5};
for (int i : v) std::cout << i << " ";
return 0;
}
Use Cases: STL simplifies handling complex data structures and algorithms, making it suitable for general-purpose programming.
OpenCV
OpenCV is an open-source library mainly designed for real-time computer vision applications. It has a vast range of functionalities geared toward image processing and analysis.
- Key Features: OpenCV offers:
- Support for various image formats
- Image transformations, filtering, and shape detection
Basic Image Loading Example:
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.jpg");
cv::imshow("Display window", image);
cv::waitKey(0);
return 0;
}
Use Cases: OpenCV is a go-to library for projects involving computer vision, such as facial recognition systems, object detection, and image processing tasks.
PCL (Point Cloud Library)
The Point Cloud Library (PCL) is a key player for 3D data processing, especially in robotics and intelligent systems.
- Key Features: PCL provides a multitude of tools and algorithms for:
- 3D point cloud processing, filtering, and feature extraction
- It supports segmentation, registration, and visualization of 3D data.
Use Cases: Utilize PCL when developing applications that involve spatial data analysis or working with 3D sensor data.
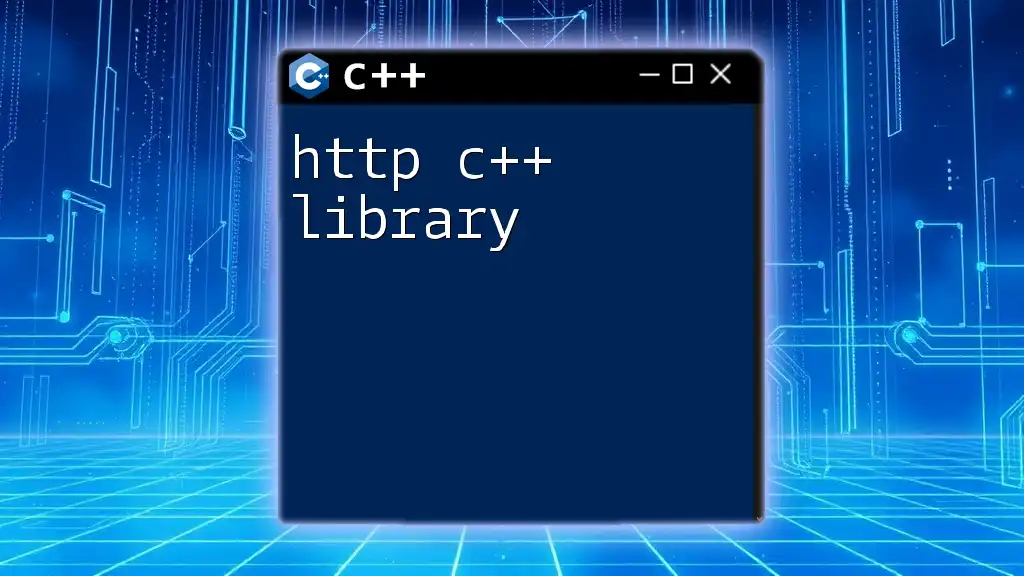
How to Integrate C++ Libraries into Your Projects
Setting Up Your Development Environment
To integrate libraries effectively, the initial setup is crucial. Use an IDE like Visual Studio, Code::Blocks, or CLion for a robust development experience. Alongside these IDEs, consider package managers like vcpkg or Conan for efficient library management.
Example Integration Process
Integrating a library can typically be done in a few straightforward steps. For example, to integrate Boost:
- Install Boost via your package manager. For vcpkg, you would run:
vcpkg install boost
- Include the relevant headers and link against the library in your project.
This simple integration approach saves time and ensures that you can focus on development rather than library setup.
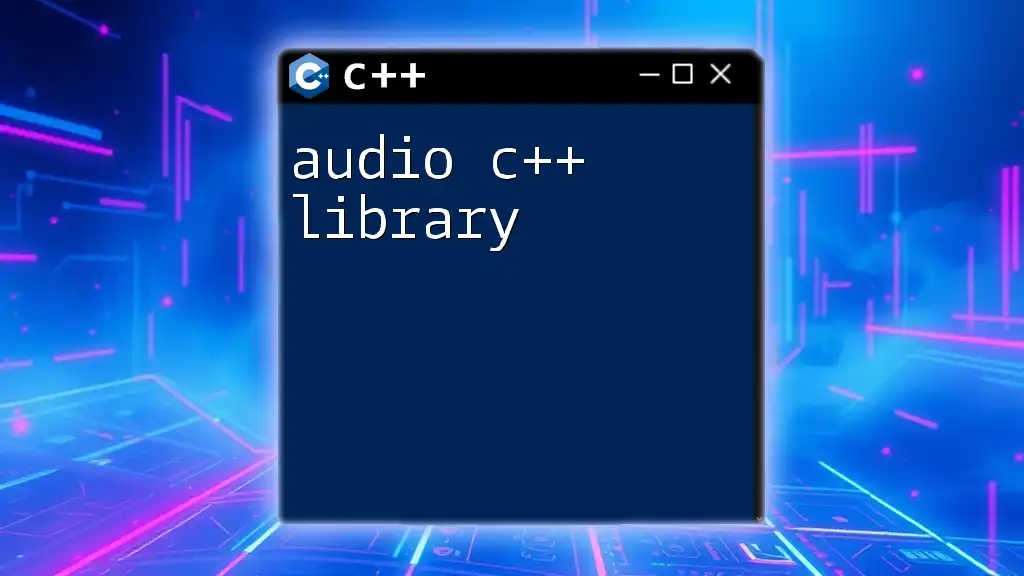
Conclusion
The discussion around the best C++ libraries reveals a multitude of options tailored for various programming needs. Whether you're looking for a comprehensive suite like Boost, a GUI framework like Qt, the fundamental STL, image processing capabilities from OpenCV, or 3D solutions from PCL, there’s something available to enhance productivity and performance.
Exploring these libraries can significantly empower your C++ programming skills and improve the quality and maintainability of your applications. Embrace the opportunity to experiment with these libraries, and transform your coding journey into an efficient and enjoyable experience.
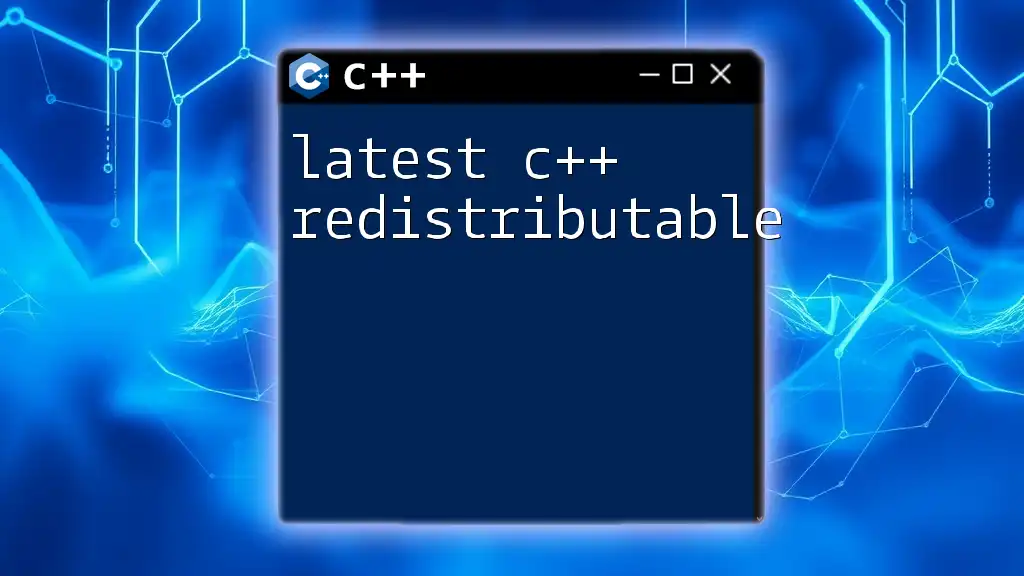
Additional Resources
To further your understanding and facilitate implementation, consult the following resources:
- Official documentation for each library
- Recommended tutorials and online courses
- Other useful tools and libraries worth documenting
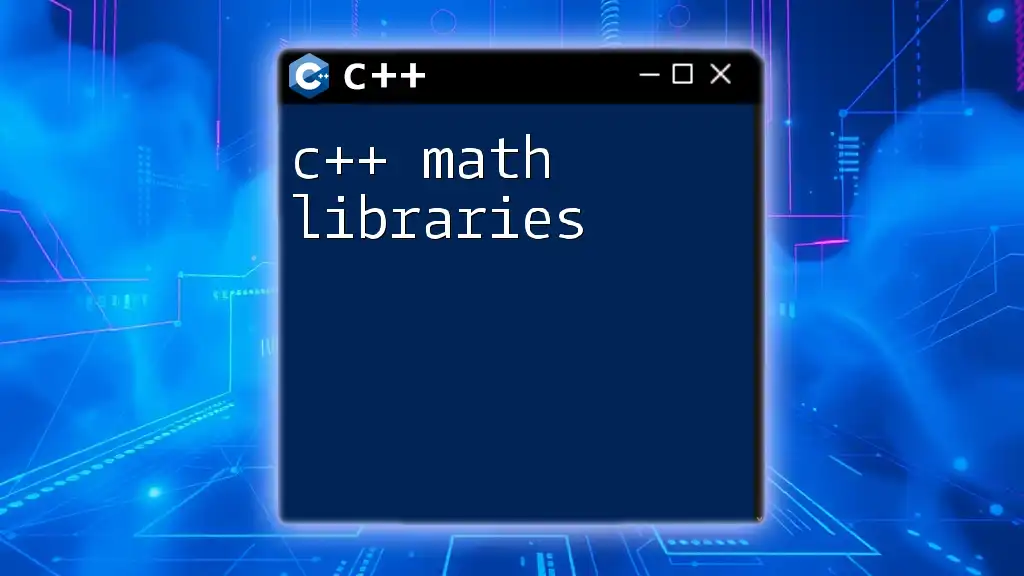
FAQ
Have questions about C++ libraries? Here are some common queries:
-
Q: What are the benefits of using libraries in C++?
-
A: Libraries allow developers to avoid reinventing the wheel, providing pre-tested functionalities that enhance productivity and reduce development time.
-
Q: How do I choose the right C++ library for my project?
-
A: Consider factors like performance, usability, community support, and your project requirements.
-
Q: Are C++ libraries platform-independent?
-
A: Many libraries, like Qt, are designed to be cross-platform, while others may have platform-specific limitations. Always check the documentation for details.