C++ libraries are collections of pre-written code that provide functionality and tools to simplify programming tasks, allowing developers to leverage existing solutions instead of coding from scratch.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding C++ Libraries
What is a C++ Library?
A C++ library is a collection of precompiled code that can be reused in C++ programs. Libraries encapsulate frequently used functions, classes, and methods, allowing developers to write more efficient, maintainable, and modular code. By using libraries, you don’t need to reinvent the wheel; the code is already available, so you can focus on building unique functionalities.
Types of C++ Libraries
C++ libraries primarily come in two forms: static libraries and dynamic libraries.
Static Libraries
Static libraries are linked into the executable during the compilation process. Once compiled, the code within a static library becomes part of the program, which can lead to larger file sizes. However, static libraries do not require distribution alongside the program.
Example of static library compilation: To create a static library, you might use commands like:
g++ -c mylibrary.cpp
ar rcs libmylibrary.a mylibrary.o
This process compiles `mylibrary.cpp` into an object file and then archives it into a static library.
Dynamic Libraries (Shared Libraries)
Dynamic libraries are linked to the program at runtime. This means that the executable is smaller and that multiple programs can share the same library in memory, which saves resources. However, distributing your program requires ensuring the required dynamic libraries are available on the host system.
Example of dynamic library loading: Creating a shared library can be achieved with:
g++ -shared -o libmylibrary.so mylibrary.cpp
Why Use Libraries?
Utilizing libraries simplifies coding and enriches functionality and performance. Libraries enable code reuse, allowing developers to rely on thoroughly tested methods. This enhances productivity since writing new code always comes with the risk of bugs.
Comparison of writing from scratch vs. library usage:
- Writing from scratch can lead to longer development cycles and potential bugs.
- Using libraries provides you with ready-to-go solutions that are often community-tested.
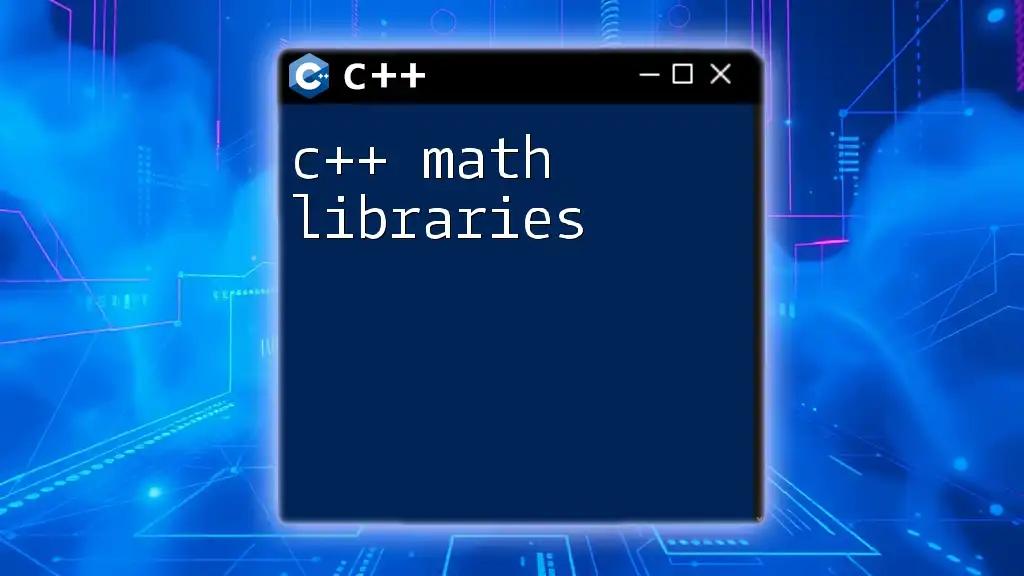
Common C++ Standard Libraries
Standard Template Library (STL)
Overview of STL
The Standard Template Library (STL) is a powerful C++ library that provides generic classes and functions. Its components include algorithms, containers (such as vectors and lists), and iterators, making it a cornerstone of modern C++ programming.
Example Usage of STL
Here's a simple example that demonstrates how to use vectors and the sort algorithm in the STL:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1, 2};
std::sort(numbers.begin(), numbers.end());
std::cout << "Sorted numbers: ";
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
This code snippet sorts a vector of integers in ascending order, showcasing how easy it is to utilize STL's functionalities.
Input/Output Libraries
iostream
The `iostream` library enables standard input and output in C++. It provides `cin`, `cout`, `cerr`, and `clog`, which are essential for reading from the console and writing to it.
Code example for input/output functions:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
This example captures user input and responds by greeting them, illustrating how to use `iostream` for console interactions.
String Libraries
string
The string library in C++ is vital for handling sequences of characters. It provides various functions for string manipulation, making it easy to work with text.
Example of string manipulation:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, ";
std::string name = "World";
std::string completeGreeting = greeting + name + "!";
std::cout << completeGreeting << std::endl;
return 0;
}
This code snippet shows string concatenation, which is simple and efficient with the `string` library.
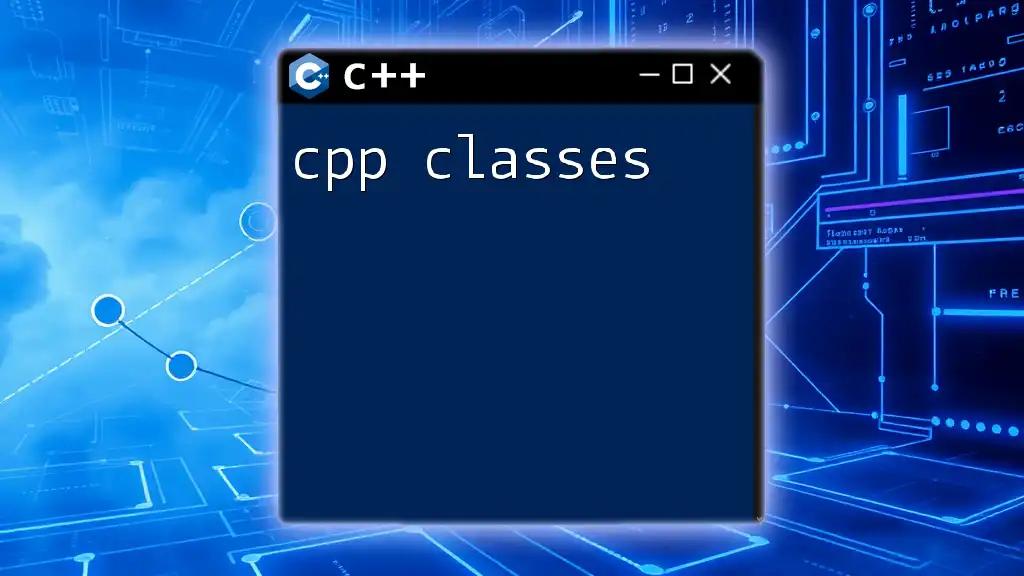
Third-Party C++ Libraries
Popular Third-Party Libraries
Boost
Boost is a widely recognized collection of peer-reviewed libraries that extends the functionality of C++. It includes modules for smart pointers, file systems, and multi-threading, among others.
Example code snippet using Boost:
#include <boost/shared_ptr.hpp>
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Boost Smart Pointer in Action!" << std::endl;
}
};
int main() {
boost::shared_ptr<MyClass> ptr(new MyClass());
ptr->display();
return 0;
}
In this example, the use of a smart pointer ensures proper memory management while maintaining simplicity.
Qt
Qt is a robust framework ideal for developing GUI applications in C++. Beyond GUI, it offers functionalities for networking, file handling, and more. The object-oriented nature of Qt simplifies complex tasks.
Example of creating a simple GUI using Qt:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, Qt!");
button.resize(200, 100);
button.show();
return app.exec();
}
This example initializes a basic GUI application that displays a button, highlighting how intuitive it is to create interfaces with Qt.
How to Find and Choose Libraries
When selecting libraries, consider factors such as performance, documentation, and community support. A well-documented library with an active community can significantly ease the learning curve.
Resources to locate libraries:
- GitHub: An excellent platform for discovering open-source libraries.
- C++ Package Managers (like vcpkg): Help in easily installing and managing libraries.
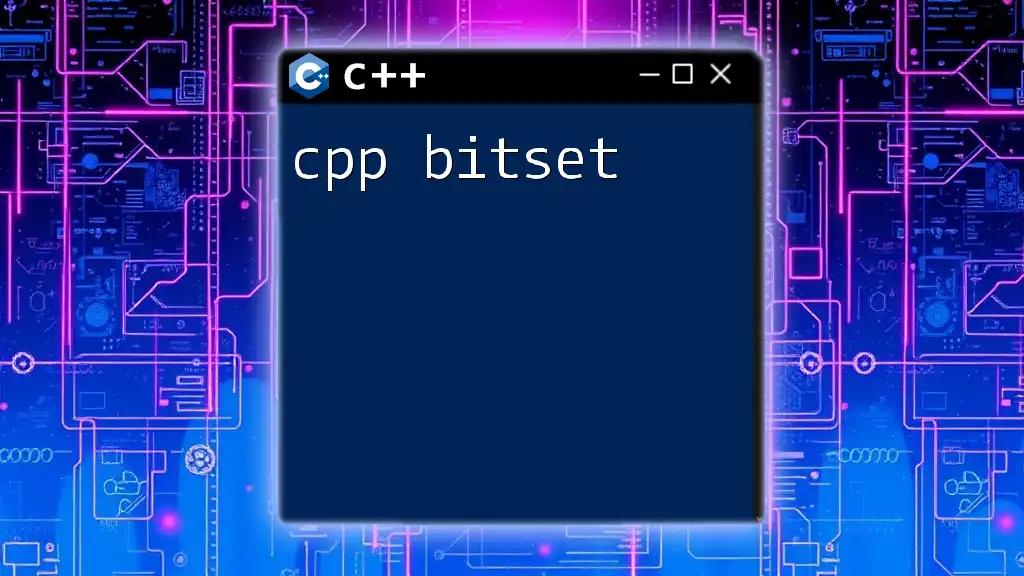
Creating Your Own C++ Library
Designing a Basic Library
To create a custom library, start by planning its structure and defining its purpose. Consider what functions or classes will be frequently used and how they can be organized logically.
Building and Compiling Your Library
Using commands in your terminal or command prompt, you can compile your library efficiently. Here's a simple example of how this might be done:
g++ -c mylibrary.cpp
ar rcs libmylibrary.a mylibrary.o
This set of commands compiles your source files and creates a static library that you can link against when building your applications.
Packaging and Distribution
Making your library available to others involves creating clear documentation and examples to demonstrate its use. Good documentation, including usage examples and function descriptions, is crucial for user adoption and understanding.
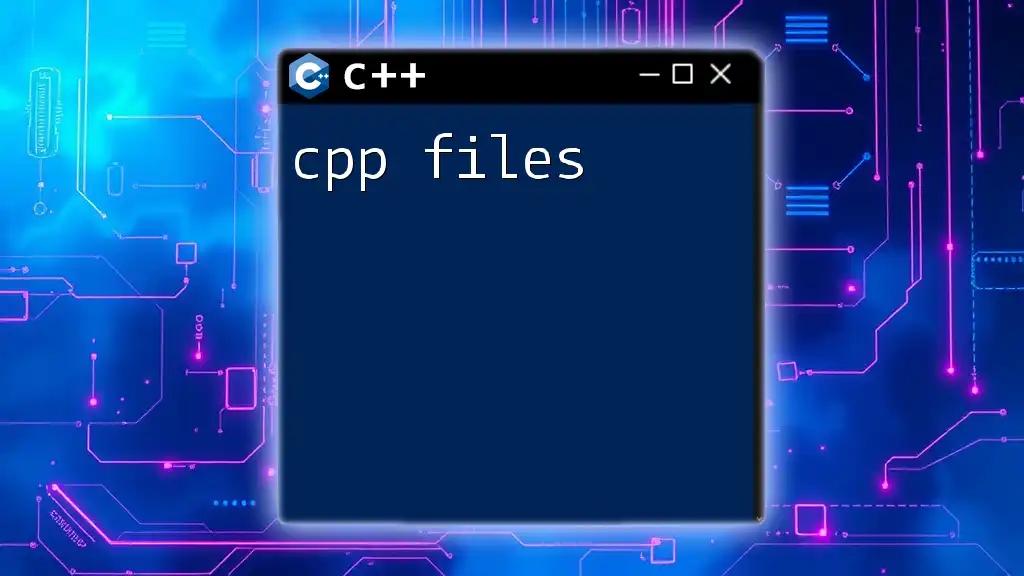
Best Practices for Using C++ Libraries
When to Use Libraries
Identify opportunities to leverage libraries based on the complexity of your task. Libraries often provide solutions to complex problems that would be time-consuming to implement from scratch. However, avoid over-reliance on libraries for trivial tasks, as this can lead to unnecessary complexity.
Managing Dependencies
Use tools like CMake to manage dependencies effectively. CMake simplifies the process of building projects that rely on different libraries. A typical CMakeLists.txt file may look like the following:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
find_package(Boost REQUIRED)
add_executable(MyApp main.cpp)
target_link_libraries(MyApp Boost::Boost)
Library Versioning
Maintaining compatible library versions is essential in ensuring that your application runs smoothly after updates. Follow semantic versioning principles: major versions for incompatible changes, minor versions for backward-compatible features, and patch versions for bug fixes.
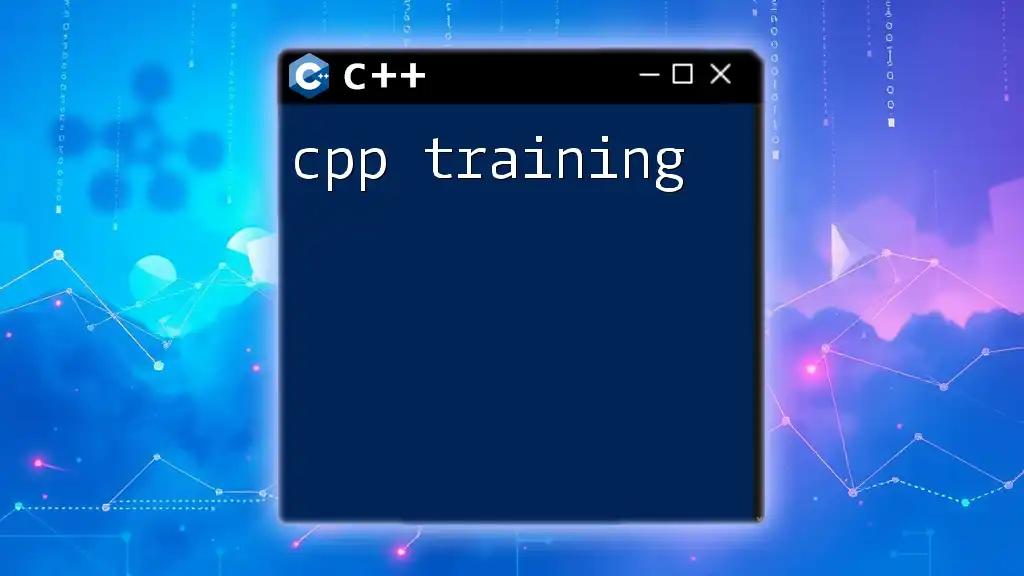
Conclusion
Recap of C++ Libraries
C++ libraries play a vital role in developing robust, efficient, and maintainable applications. By understanding and leveraging both standard and third-party libraries, developers can enhance their productivity and the overall quality of their code.
Call to Action
We encourage you to share your experiences with C++ libraries. Join workshops and courses to deepen your understanding and improve your coding skills. Explore how efficiently using C++ libraries can transform your programming journey.
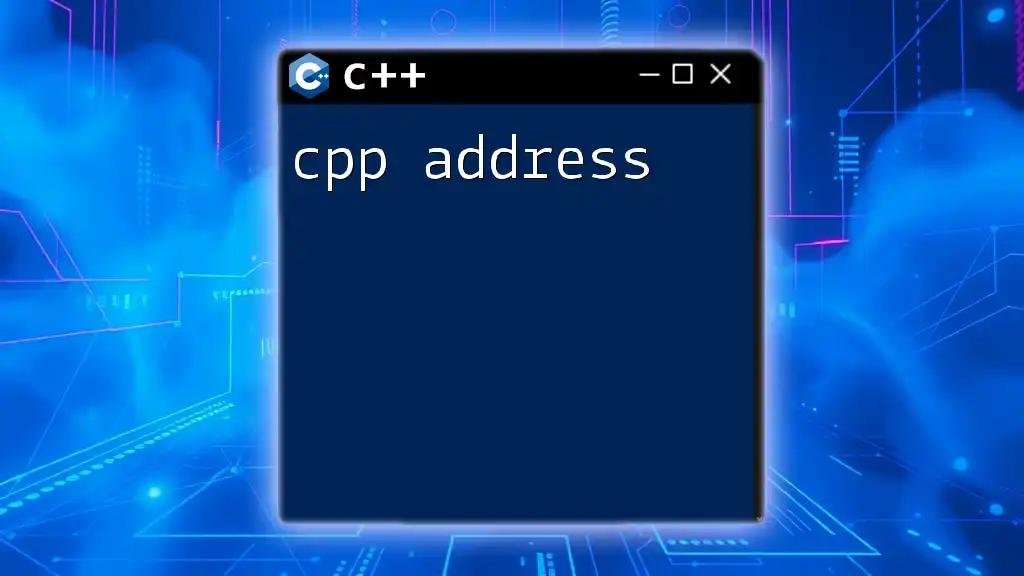
Additional Resources
Further Reading and Tutorials
Seek out books, websites, and online tutorials for comprehensive learning. Sites like cppreference.com and LearnCPP.com can be valuable resources.
Community Links
Participate in online forums and communities for C++ developers to exchange knowledge, ask questions, and collaborate. Engaging with others can expand your skill set and provide support as you learn.