`std::shared_ptr` is a smart pointer that manages the lifetime of an object through reference counting, allowing multiple pointers to share ownership of the same object.
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> ptr1 = std::make_shared<int>(10);
std::shared_ptr<int> ptr2 = ptr1; // ptr2 shares ownership with ptr1
std::cout << "Value: " << *ptr1 << ", Use count: " << ptr1.use_count() << std::endl; // Outputs: Value: 10, Use count: 2
return 0;
}
Understanding shared_ptr
What is shared_ptr?
A shared_ptr in C++ is a type of smart pointer that allows multiple pointers to share ownership of a dynamically allocated object. This means that multiple shared_ptr instances can point to the same object in memory, and the object will be automatically deleted when the last shared_ptr pointing to it is destroyed or reset. This provides a robust solution to managing memory and reducing the risk of leaks or dangling pointers.
Shared_ptr is essential in modern C++ programming because it not only simplifies memory management but also enhances the safety and stability of the code.
Advantages of using shared_ptr
Using shared_ptr has several advantages over using raw pointers:
-
Automatic Memory Management: The memory allocated for the object is automatically deallocated when the last shared_ptr to that object is destroyed. This reduces the likelihood of memory leaks.
-
Shared Ownership Concept: This allows multiple parts of a program to manage the lifetime of an object without explicitly tracking its usage count.
-
Safety Against Dangling Pointers: Since shared_ptr keeps track of how many pointers are pointing to the same object, you don't have to worry about accessing memory that's already been freed.
-
Enhanced Code Readability: Using shared_ptr clearly indicates ownership semantics, making it easier for developers to understand the flow of memory management in the code.
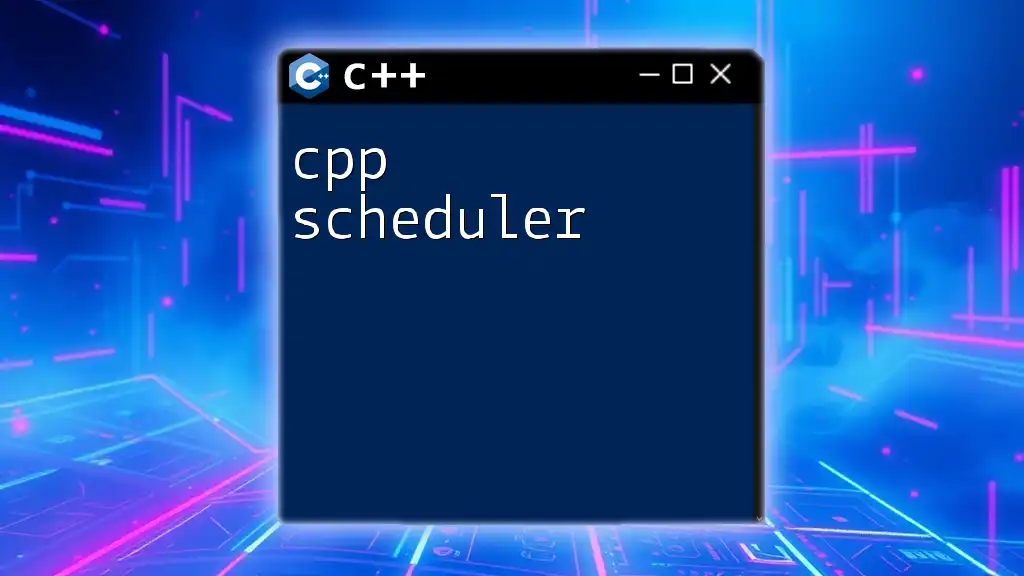
How to Use shared_ptr
Creating a shared_ptr
Creating a shared_ptr is straightforward. The standard way to create one is by using the `std::make_shared` function. This function creates an object and wraps it in a shared_ptr, ensuring efficient memory allocation.
Example Code Snippet:
#include <iostream>
#include <memory>
int main() {
std::shared_ptr<int> ptr = std::make_shared<int>(10);
std::cout << "Value: " << *ptr << std::endl;
return 0;
}
In the example above, `ptr` is a shared_ptr that points to an integer initialized to 10.
Initializing shared_ptr with raw pointers
You can also initialize a shared_ptr directly with a raw pointer. However, this approach is less common and comes with risks.
Code Example:
std::shared_ptr<int> ptr1(new int(20));
While this works, it’s essential to remember that if the raw pointer is not managed properly, it can lead to memory management issues.
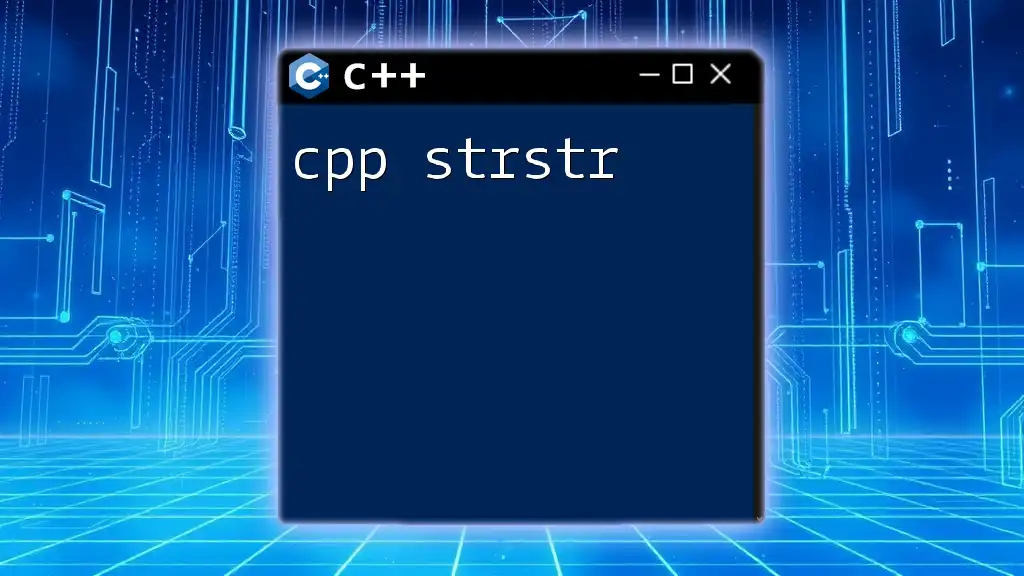
Shared Ownership Model
How shared_ptr manages ownership
One of the key features of shared_ptr is its reference counting mechanism. Each time a new shared_ptr is created pointing to the same object, the reference count increments. Conversely, when one is destroyed or reset, the reference count decrements.
You can check how many shared_ptr instances share ownership of the same object using the `use_count()` function.
Example:
auto ptr2 = ptr1;
std::cout << "Use count: " << ptr2.use_count() << std::endl;
When you run this code snippet, it will show the number of shared_ptrs currently sharing ownership of the same resource.
Transferring shared_ptr
You can transfer ownership of a shared_ptr using `std::move()`. This action transfers the resource from one shared_ptr to another while leaving the original shared_ptr in a null state.
Code Snippet:
std::shared_ptr<int> ptr3 = std::move(ptr1);
After this operation, `ptr1` will no longer own the resource, and attempting to dereference it will lead to undefined behavior.
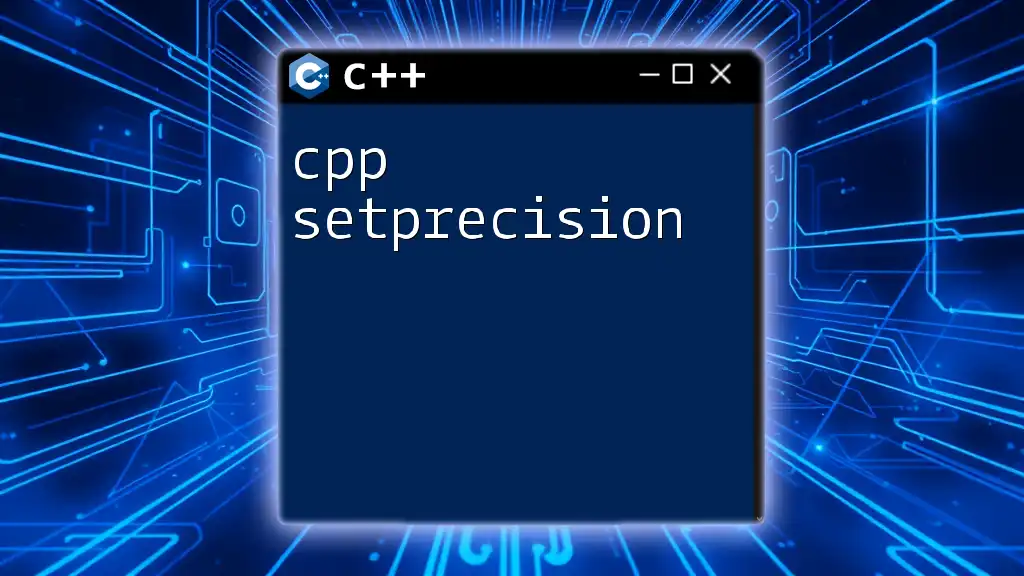
Practical Use Cases of shared_ptr
When to use shared_ptr
shared_ptr is ideal in scenarios where an object needs to be shared among multiple owners, like in a multithreaded application or when dealing with complex data structures (like trees or graphs) where multiple parts of your code need access to the same object without worrying about who should delete it.
Common pitfalls and best practices
While shared_ptr offers significant advantages, it also comes with its set of challenges. One common pitfall is circular references, which occur when two or more shared_ptr instances reference each other. This can lead to memory leaks because the reference count will never reach zero.
To avoid this, use weak_ptr for one of the pointers to break the circular ownership. This allows the shared_ptr to release the resource appropriately.
Example Case Study
Let’s consider a basic example involving a resource management class:
class Resource {
public:
Resource() { std::cout << "Resource acquired." << std::endl; }
~Resource() { std::cout << "Resource released." << std::endl; }
};
void createResource() {
std::shared_ptr<Resource> res1 = std::make_shared<Resource>();
// Do something with res1
}
In this example, a `Resource` object is created and managed by a shared_ptr. When `createResource()` completes, `res1` goes out of scope, and the Resource destructor is automatically called, demonstrating efficient memory management.
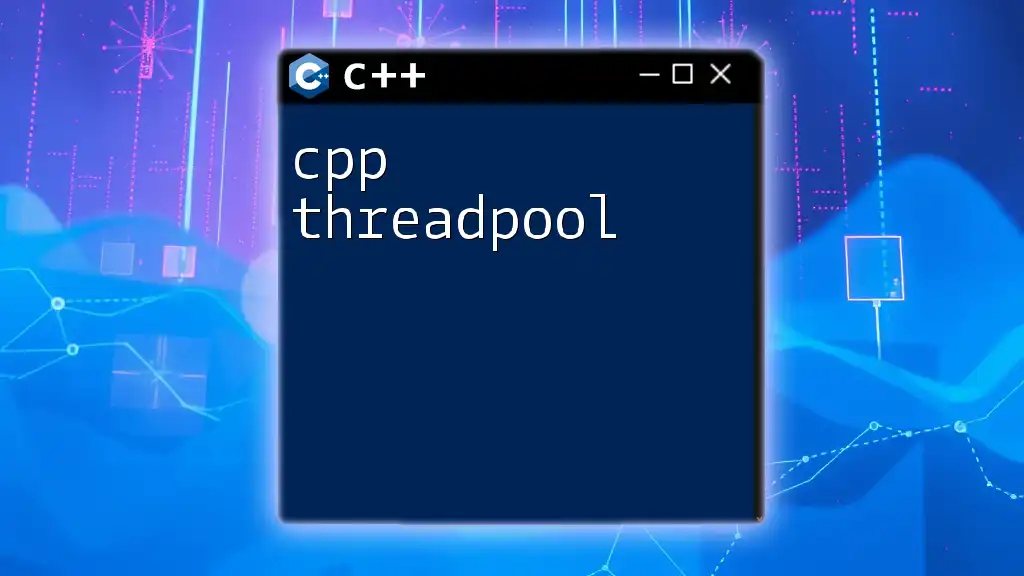
Comparing shared_ptr with other smart pointers
shared_ptr vs unique_ptr vs weak_ptr
Understanding the differences between smart pointers is crucial for effective memory management.
-
shared_ptr: Used for shared ownership of an object. Multiple shared_ptr instances can point to the same object.
-
unique_ptr: Holds exclusive ownership of an object, ensuring no other pointer shares the same instance. It cannot be copied but can be moved.
-
weak_ptr: Provides a non-owning reference to an object managed by a shared_ptr. This is particularly useful in breaking circular references and preventing memory leaks.
Performance comparison
When considering performance, weak_ptr has minimal overhead since it does not affect the reference count. However, both shared_ptr and unique_ptr involve some additional memory management overhead compared to raw pointers due to their reference counting and management features.
In scenarios where unique ownership is sufficient, prefer unique_ptr for its performance benefits. Use shared_ptr only when necessary to share ownership.
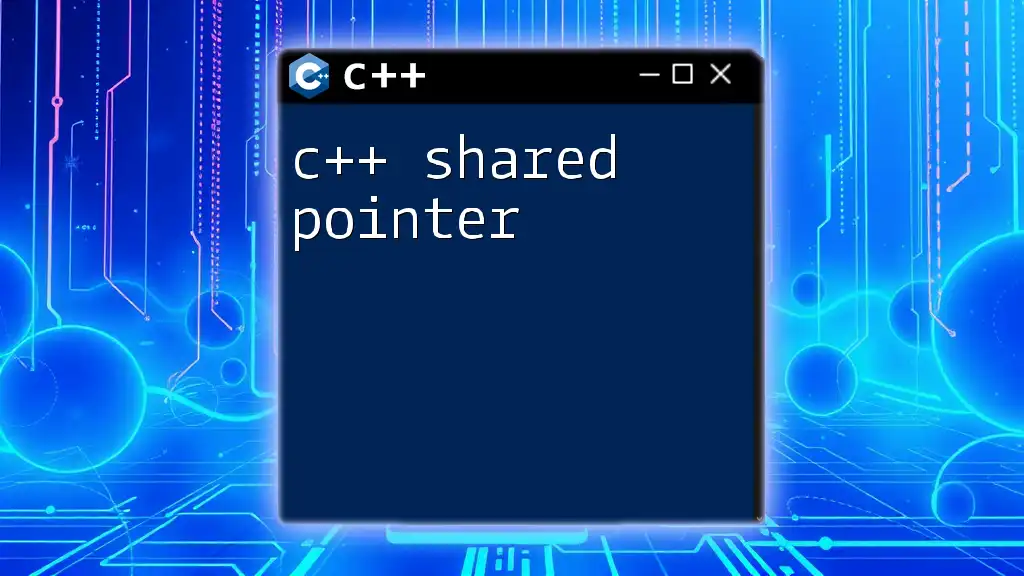
FAQs about shared_ptr
Common questions about shared_ptr
-
What happens when the last shared_ptr pointing to an object is destroyed? The object is automatically deallocated, ensuring no memory leaks.
-
Can I convert shared_ptr to unique_ptr? No, the ownership semantics differ. However, you can transfer ownership from shared_ptr to unique_ptr, but it requires a move operation.
-
What is use_count, and why is it important? The `use_count()` function indicates how many shared_ptr instances are currently owning the same object. This information can be critical for debugging and ensuring proper memory management.
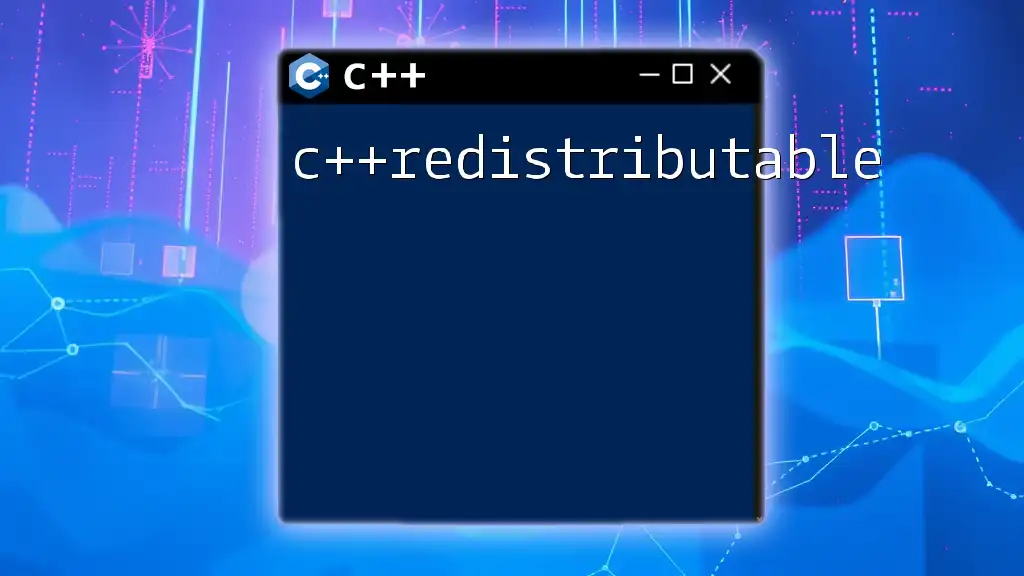
Conclusion
In conclusion, mastering cpp shared_ptr is vital for modern C++ development. By providing automatic memory management and safe shared ownership semantics, shared_ptr significantly enhances the safety and reliability of your software. Transitioning from raw pointers to smart pointers can greatly reduce the risks associated with manual memory management, make your code more readable, and keep your applications robust.
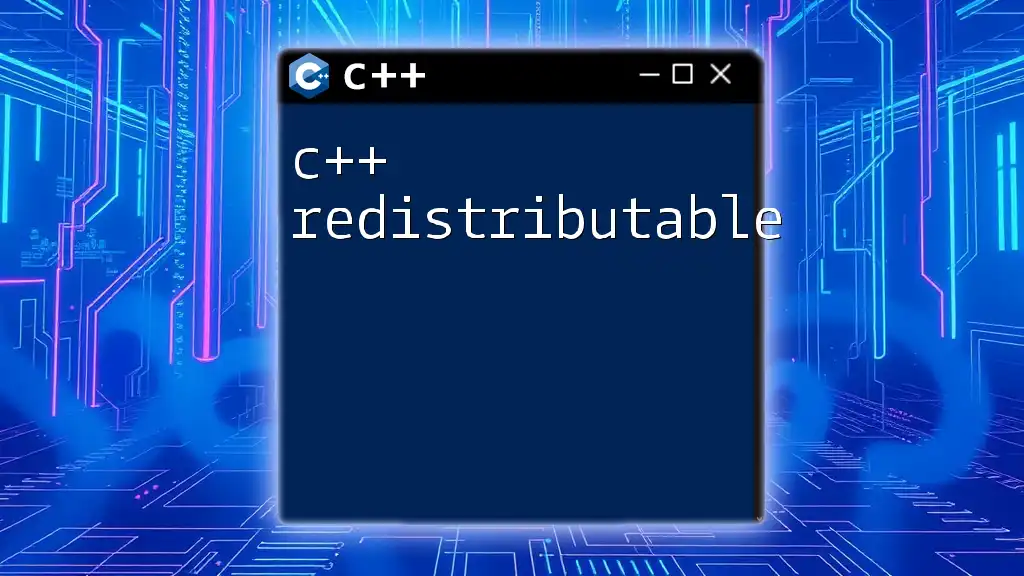
Call to Action
Try experimenting with the examples provided in this article to see how shared_ptr works in practice. To further your understanding of smart pointers and other C++ concepts, check out our tutorials and courses aimed at helping you master C++ programming quickly and effectively.