A C++ thread pool is a design pattern that manages a collection of worker threads to efficiently execute tasks from a queue without the overhead of frequently creating and destroying threads.
Here's a simple example of a C++ thread pool implementation:
#include <iostream>
#include <vector>
#include <thread>
#include <queue>
#include <functional>
#include <condition_variable>
#include <future>
class ThreadPool {
public:
ThreadPool(size_t);
template<class F>
auto enqueue(F&& f) -> std::future<typename std::result_of<F()>::type>;
~ThreadPool();
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable cv;
bool stop;
};
ThreadPool::ThreadPool(size_t threads) : stop(false) {
for (size_t i = 0; i < threads; ++i)
workers.emplace_back([this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->queue_mutex);
this->cv.wait(lock, [this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
template<class F>
auto ThreadPool::enqueue(F&& f) -> std::future<typename std::result_of<F()>::type> {
using return_type = typename std::result_of<F()>::type;
auto task = std::make_shared<std::packaged_task<return_type()>>(std::forward<F>(f));
std::future<return_type> res = task->get_future();
{
std::unique_lock<std::mutex> lock(queue_mutex);
// Don't permit enqueueing after stopping the pool
if (stop)
throw std::runtime_error("enqueue on stopped ThreadPool");
tasks.emplace([task]() { (*task)(); });
}
cv.notify_one();
return res;
}
ThreadPool::~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
cv.notify_all();
for (std::thread &worker : workers)
worker.join();
}
Understanding Thread Pool Concepts
What is a Thread Pool?
A thread pool is a collection of worker threads that efficiently manage the execution of tasks, thereby preventing the overhead of thread creation and destruction for each individual task. By reusing existing threads, thread pools enable programs to run faster and more efficiently.
Benefits of using a thread pool include:
- Reduced overhead: Threads are created once and reused, which minimizes the cost of thread management.
- Simplicity: Code becomes cleaner and easier to maintain as the complexities of thread management are encapsulated in the thread pool.
- Flexible task scheduling: Multiple tasks can be queued and executed concurrently without blocking.
Key Terms Related to Thread Pool in C++
Understanding a few key terms can help clarify how thread pools function:
- Threads: Basic units of CPU usage that can execute tasks concurrently.
- Tasks: Units of work that are submitted to the thread pool for execution.
- Queue: A data structure that holds tasks waiting to be executed by threads.
- Worker Threads: Threads in the pool that pick up and execute tasks from the queue.
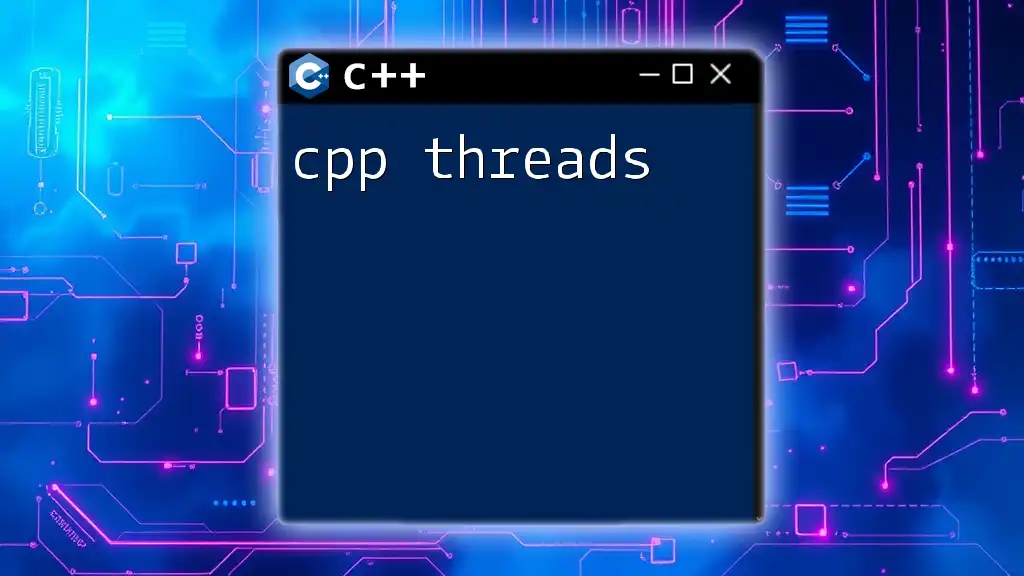
Advantages of Using a Thread Pool in C++
Performance Improvement
Thread pools significantly improve performance by minimizing the overhead that comes with dynamic thread creation. When tasks are offloaded to a thread pool, the application benefits from fast execution rates as threads remain in a ready state until needed. Consequently, the application can handle high loads without fearing long wait times for thread setup.
Efficient Resource Management
By maintaining a limited number of threads, a thread pool improves resource management. It only consumes the necessary resources, which conserves CPU and memory usage. This leads to reduced context switching, which occurs when the CPU switches from one thread to another. Context switching can be costly in terms of performance, making thread pools an attractive choice for developing efficient applications.
Scalability
Thread pools provide scalability by allowing applications to adapt to varying workloads. For instance, during peak times, additional tasks can be queued without risking resource exhaustion. This scalability makes thread pools a perfect choice for high-performance applications, such as servers handling numerous client connections.
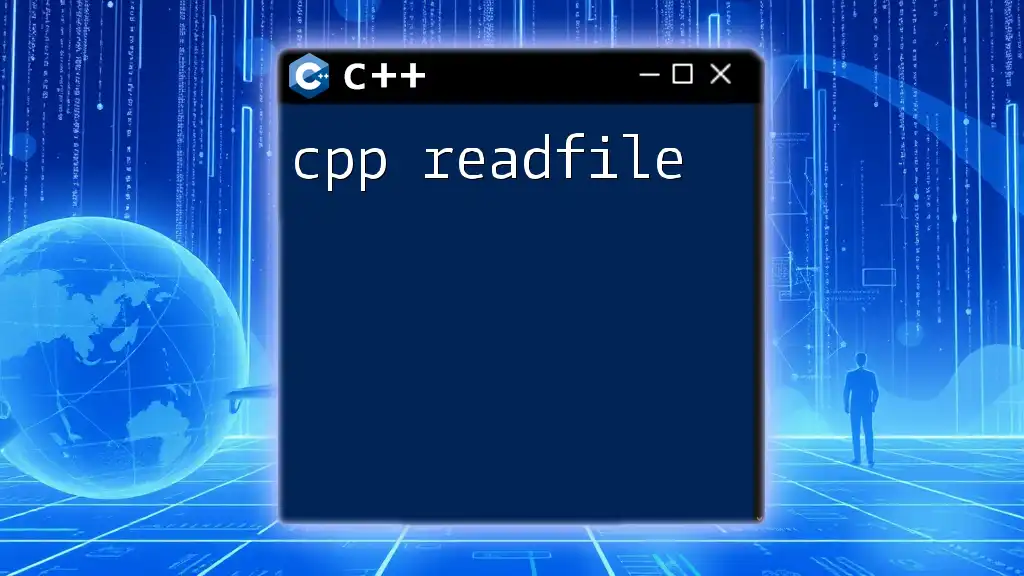
Implementing a C++ Thread Pool
Basic Structure of a C++ Thread Pool
A typical thread pool in C++ consists of the following components:
- A queue for tasks
- A collection of worker threads that execute the tasks
- Synchronization mechanisms to manage access to the queue safely
These components work together to implement the thread pool's functionality, ensuring that multiple tasks can be executed concurrently without notable interference.
Code Example: A Simple C++ Thread Pool
#include <iostream>
#include <vector>
#include <thread>
#include <queue>
#include <functional>
#include <condition_variable>
class ThreadPool {
public:
ThreadPool(size_t);
template<class F>
void enqueue(F&& f);
~ThreadPool();
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex queue_mutex;
std::condition_variable condition;
bool stop;
};
Explanation of the Code
- Constructor: Initializes the thread pool with the specified number of worker threads.
- A template method called `enqueue` allows users to submit tasks to the queue.
- Destructor: Ensures all tasks are completed and resources are cleaned up before the pool is destroyed.
Adding Tasks to the Pool
template<class F>
void ThreadPool::enqueue(F&& f) {
{
std::unique_lock<std::mutex> lock(queue_mutex);
tasks.emplace(std::forward<F>(f));
}
condition.notify_one();
}
Explanation of Task Management
This method handles task submission. It locks the queue mutex to ensure thread safety while adding the task. Once added, it notifies one waiting thread to pick up the new task from the queue.
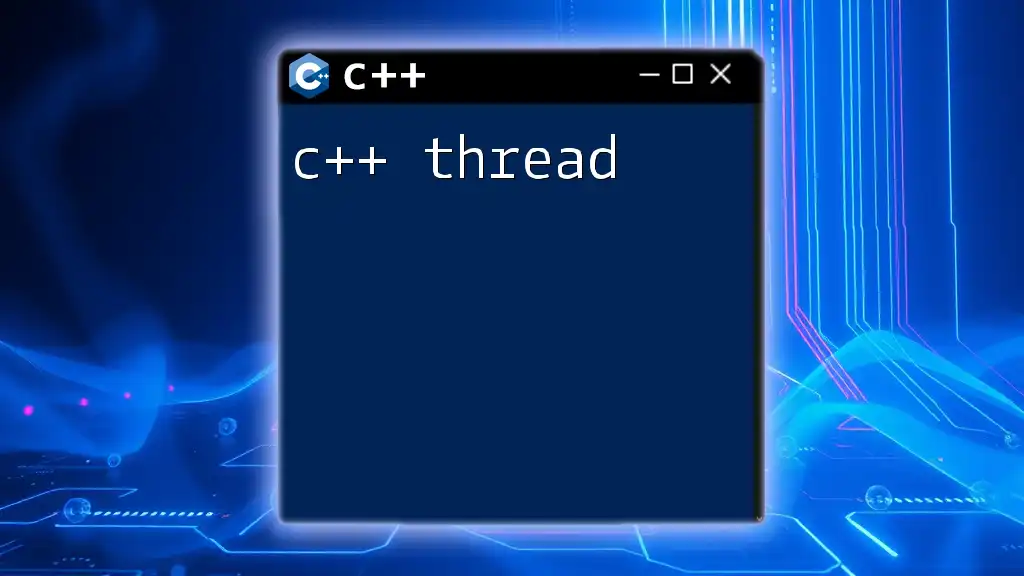
Using the Thread Pool in C++
Practical Example of a C++ Thread Pool
To demonstrate how to use the `ThreadPool`, let's implement a simple example:
// Example task
auto example_task = []() {
std::cout << "Task executed by thread ID: " << std::this_thread::get_id() << std::endl;
};
// Usage example
ThreadPool pool(4); // Create a thread pool with 4 threads
for(int i = 0; i < 10; ++i) {
pool.enqueue(example_task);
}
Explanation of Practical Example
In this scenario, a thread pool capable of executing four tasks concurrently is created. Ten example tasks are enqueued, and the thread pool manages their execution. Each thread executes a task independently, demonstrating concurrent behavior.
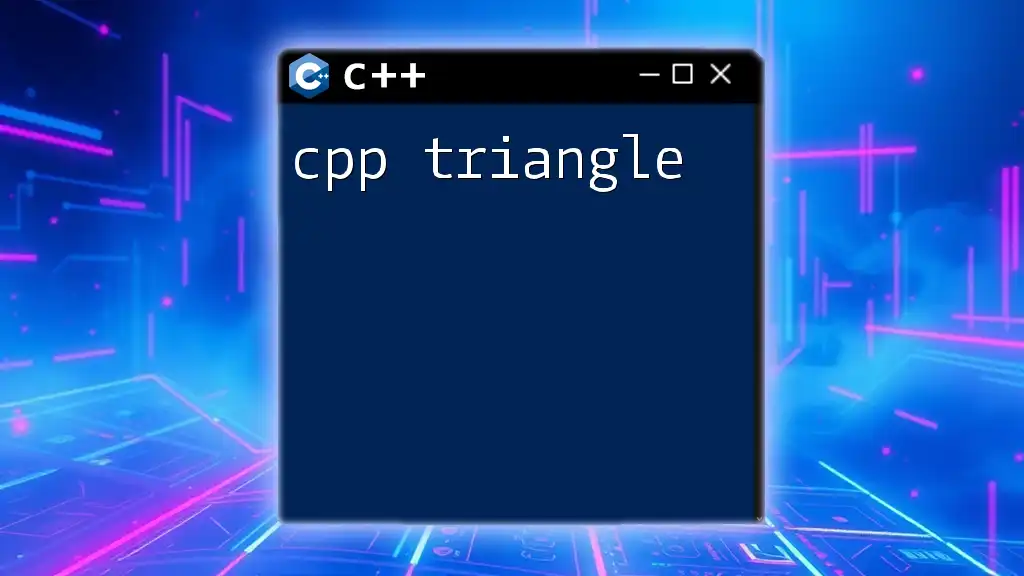
Thread Pool Best Practices
Choosing the Right Number of Threads
The ideal number of threads in a thread pool depends on factors like:
- The number of CPU cores: More cores typically allow for more threads.
- Task characteristics: If tasks are I/O bound, you might need more threads; if they are CPU-bound, stick to a number close to the number of cores.
As a general guideline, consider having one thread per core for CPU-bound tasks and a larger number for I/O-bound tasks.
Error Handling in Thread Pools
Proper error handling is essential in thread pools to ensure the stability of applications. A robust design might include:
- Task-specific error capturing: Allow each task to handle its exceptions without affecting the overall pool.
- Logging and reporting errors: Implement a logging mechanism to track issues for analysis.
Cleaning Up Resources
Graceful shutdown of a thread pool ensures that all resources are adequately managed. For instance:
ThreadPool::~ThreadPool() {
{
std::unique_lock<std::mutex> lock(queue_mutex);
stop = true;
}
condition.notify_all();
for (std::thread &worker: workers) {
worker.join();
}
}
Explanation of Resource Cleanup
The destructor locks the queue mutex to set a stop condition, notifies all waiting threads, and joins each worker thread back to the main thread to ensure clean termination. This process is crucial for efficient memory management.
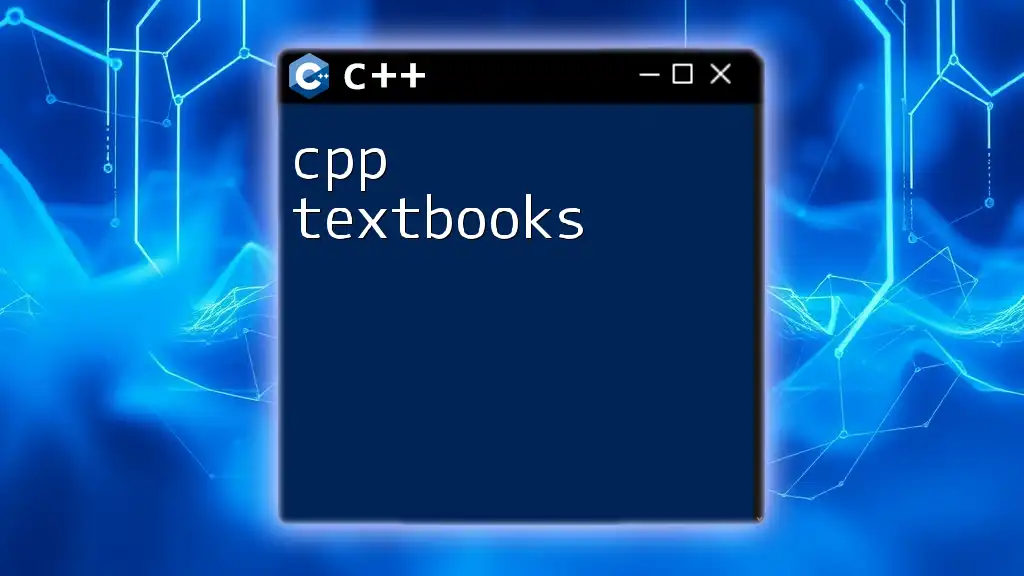
Thread Pool Libraries in C++
Overview of Popular C++ Thread Pool Libraries
Several established libraries offer thread pool implementations, including:
- Boost: A widely-used library that provides a comprehensive set of C++ libraries, including threading capabilities.
- Intel TBB (Threading Building Blocks): A library designed for scalable parallel programming, featuring a thread pool abstraction.
- C++11 Standard Libraries: Directly supports threading through features such as `std::async`, though not a strict thread pool.
Choosing a Library
Select a thread pool library based on factors like:
- Project requirements: Ensure the library meets your particular use case.
- Performance: Evaluate efficiency and ease of integration.
- Community and support: Strong community backing can help resolve issues that arise during development.
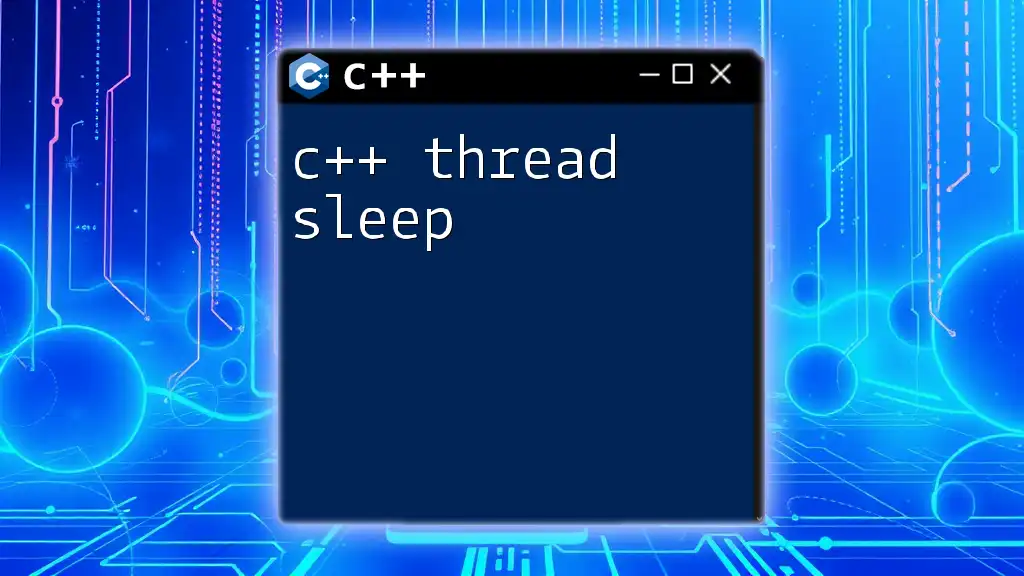
Conclusion
Thread pools are a powerful feature in C++ programming, providing efficient means to execute concurrent tasks. They improve performance by reducing overhead, enhance resource management, and enable scalability. Implementing a cpp threadpool can lead to cleaner, maintainable code while maximizing application efficiency.
Incorporate thread pools into your projects to take full advantage of concurrency in C++, and don't hesitate to reach out for further discussions or share your experiences with this topic!