A C++ roadmap outlines the essential steps and resources for learning C++ programming, from the basics to advanced concepts, enabling learners to navigate their journey efficiently.
Here's a basic example of a C++ command demonstrating a simple "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
What is C++?
C++ is a powerful, high-level programming language that has influenced many other languages due to its versatility and performance. Developed by Bjarne Stroustrup in the early 1980s, C++ extends the C programming language with features such as classes and object-oriented programming (OOP). Its ability to handle low-level memory manipulation, alongside high-level abstractions, makes it invaluable for systems programming, game development, and performance-critical applications.
Setting Up the Development Environment
Before diving into coding, it is crucial to set up the correct development environment for C++. This includes selecting an integrated development environment (IDE) and installing a C++ compiler.
Recommended IDEs:
- Visual Studio: Popular for Windows development, offering robust debugging and code management features.
- Code::Blocks: A free, open-source IDE that's easy to install and use across platforms.
- CLion: A commercial IDE that supports modern C++ features and is fully integrated with CMake.
Installing a C++ Compiler: To run C++ code, you’ll need a compiler. Common options include:
- GCC (GNU Compiler Collection): Widely used on Linux and supports multiple languages.
- Clang: A modern compiler with excellent diagnostics, preferred by some developers for its performance.
Make sure to follow specific installation steps for your operating system—Windows, macOS, or Linux—to ensure that your environment is ready for development.
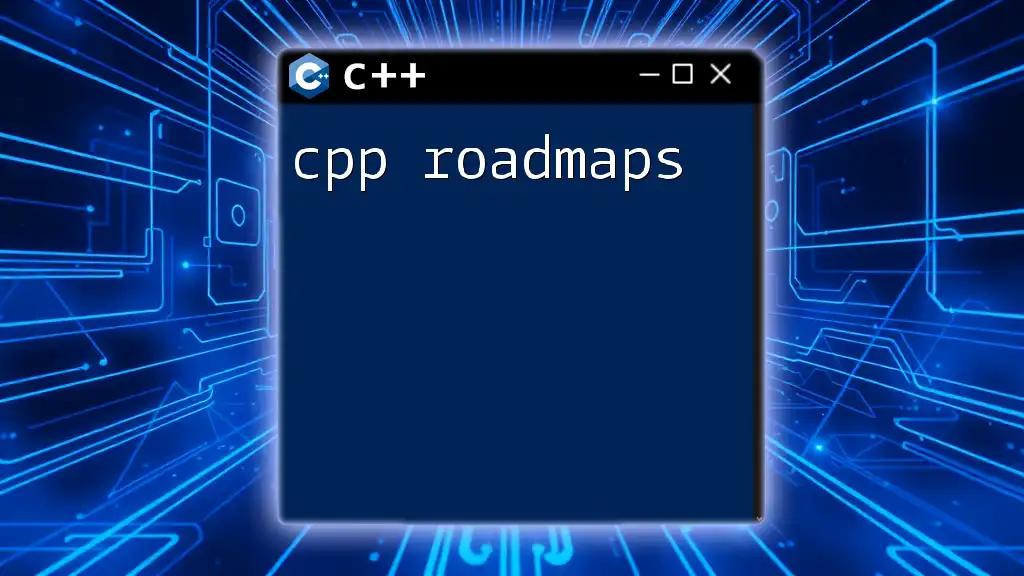
Basic C++ Concepts
Syntax and Structure
Understanding the basic syntax of C++ is essential for writing your first program. Every C++ program contains at least a `main` function, which serves as the entry point.
A simple C++ program looks like this:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This snippet includes the necessary library (`<iostream>` for input/output operations) and uses the `cout` function to display "Hello, World!" on the screen.
Data Types and Variables
C++ provides several data types, which can be categorized as follows:
- Fundamental Types: `int`, `float`, `double`, `char`, etc.
- Derived Types: arrays, pointers, etc.
- User-Defined Types: classes, structures, etc.
Variables must be declared with a specific data type before being used. Here’s an example of declaring and initializing different variables:
int age = 30;
float height = 5.9;
char initial = 'A';
Understanding data types is crucial since they dictate the kind of operations you can perform and the amount of memory your variables will occupy.
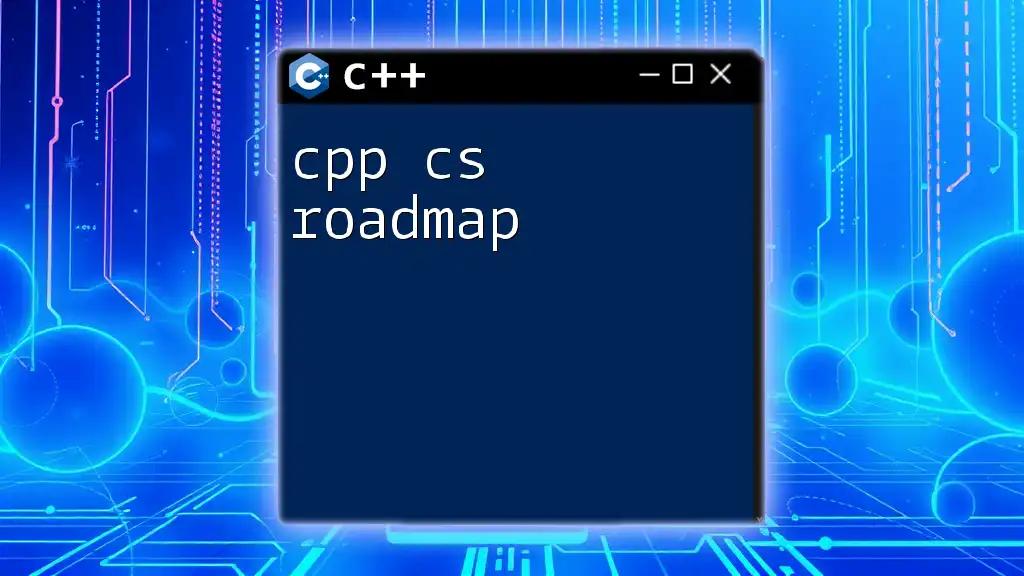
Control Structures
Conditional Statements
C++ provides various control structures to direct the flow of execution in your programs. The most commonly used conditional statements are `if`, `else if`, and `else`:
int number = 10;
if (number > 0) {
cout << "Positive" << endl;
} else {
cout << "Non-positive" << endl;
}
For scenarios requiring multiple conditions, the `switch` statement can be utilized:
char grade = 'A';
switch (grade) {
case 'A':
cout << "Excellent!" << endl;
break;
case 'B':
cout << "Well done!" << endl;
break;
default:
cout << "Grade not recognized!" << endl;
}
Loops
Loops allow you to execute a block of code multiple times based on a condition. The main types of loops in C++ are `for`, `while`, and `do-while`.
Here’s an example of a `for` loop that prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
In addition to `for` loops, you can use `while` loops for repeated execution based on dynamic conditions:
int i = 0;
while (i < 5) {
cout << i << endl;
i++;
}
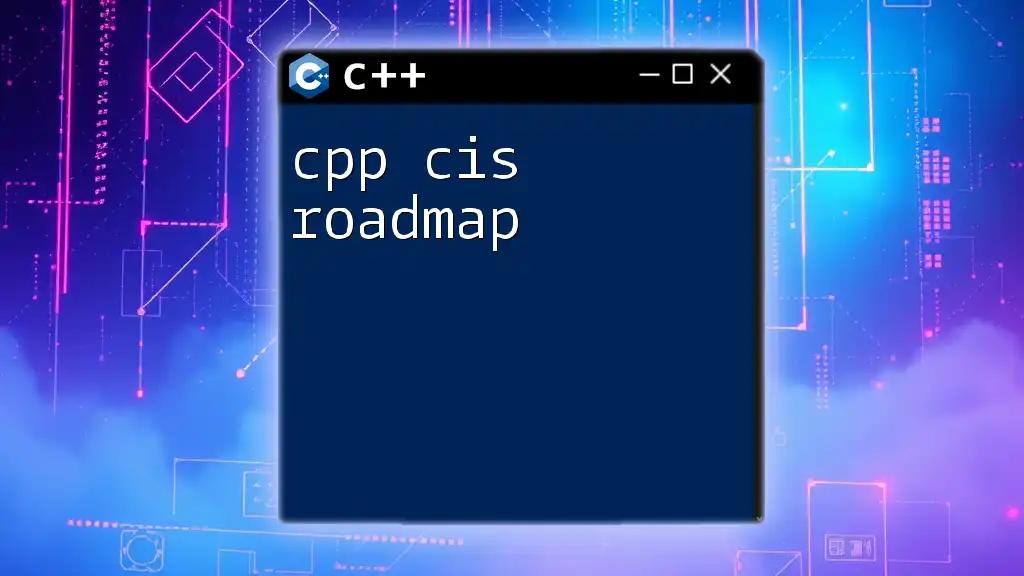
Functions in C++
Defining and Calling Functions
Functions are blocks of code designed to perform a specific task. They help in organizing code and enhancing reusability.
Here’s how you can define and call a function in C++:
int add(int a, int b) {
return a + b;
}
int main() {
cout << add(5, 3) << endl; // Outputs: 8
}
Function Overloading
C++ supports function overloading, which allows you to define multiple functions with the same name but different parameters.
An example of function overloading for the `add` function might look like this:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
With function overloading, C++ determines which function to call based on the argument types used.
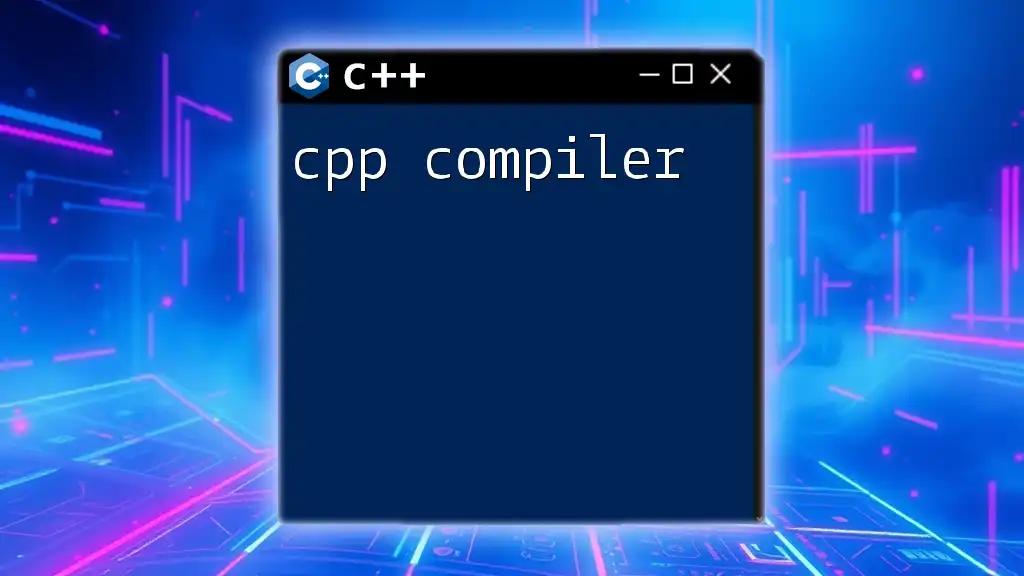
Object-Oriented Programming (OOP) in C++
Understanding OOP Concepts
Object-Oriented Programming is a programming paradigm based on the concept of "objects," which can contain data and functions. The fundamental concepts of OOP include:
- Classes and Objects: Classes define blueprints for objects. Objects are instances of classes.
- Encapsulation: Bundling data and methods that operate on that data within classes.
- Inheritance: Mechanism for a new class to inherit properties and behaviors from another class.
- Polymorphism: Ability to present the same interface for differing underlying forms (data types).
Creating Classes
Defining a class in C++ is straightforward. Here’s a simple example of a `Car` class:
class Car {
public:
string brand;
void honk() {
cout << "Honk!" << endl;
}
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.honk(); // Outputs: Honk!
}
Inheritance
Inheritance allows one class to inherit attributes and methods from another, facilitating code reuse. In C++, inheritance can be implemented as follows:
class Vehicle {
public:
void start() {
cout << "Vehicle starting..." << endl;
}
};
class Car : public Vehicle {
public:
void honk() {
cout << "Honk!" << endl;
}
};
In this example, `Car` inherits the `start()` method from `Vehicle`, allowing a `Car` object to invoke both `start()` and `honk()`.
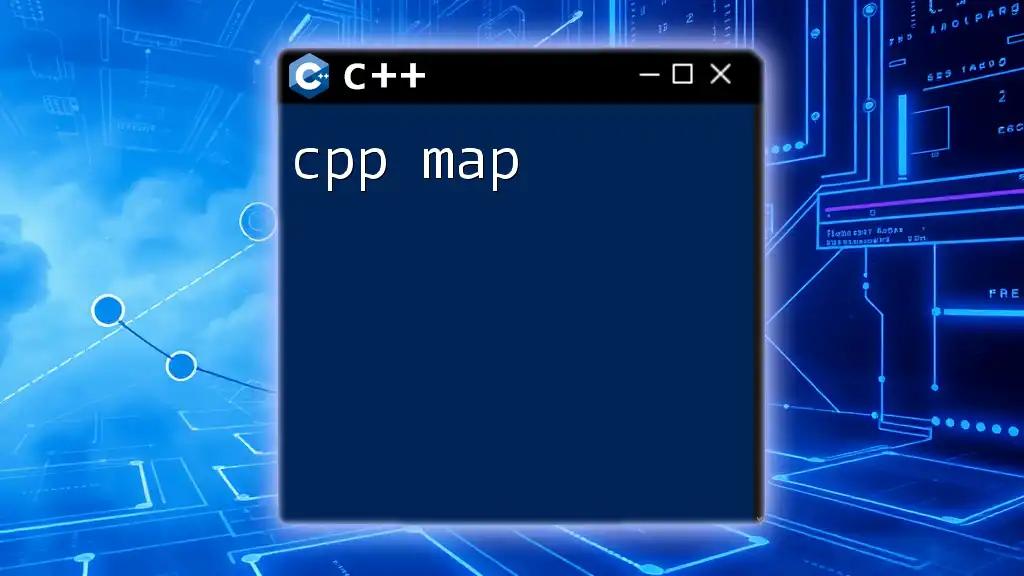
Advanced C++ Topics
Pointers and Dynamic Memory
Pointers in C++ are variables that store memory addresses of other variables, allowing for dynamic memory management:
int* ptr = new int; // dynamically allocate memory for an integer
*ptr = 10; // assign value
delete ptr; // free allocated memory
Using pointers correctly can lead to powerful programming techniques while also presenting challenges, such as memory leaks and dangling pointers.
Templates and Generic Programming
C++ supports templates that enable a function or class to operate with any data type, promoting code reuse. Here's an example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
In the above code, `add` can handle any data type, making your functions flexible and adaptable to various scenarios.
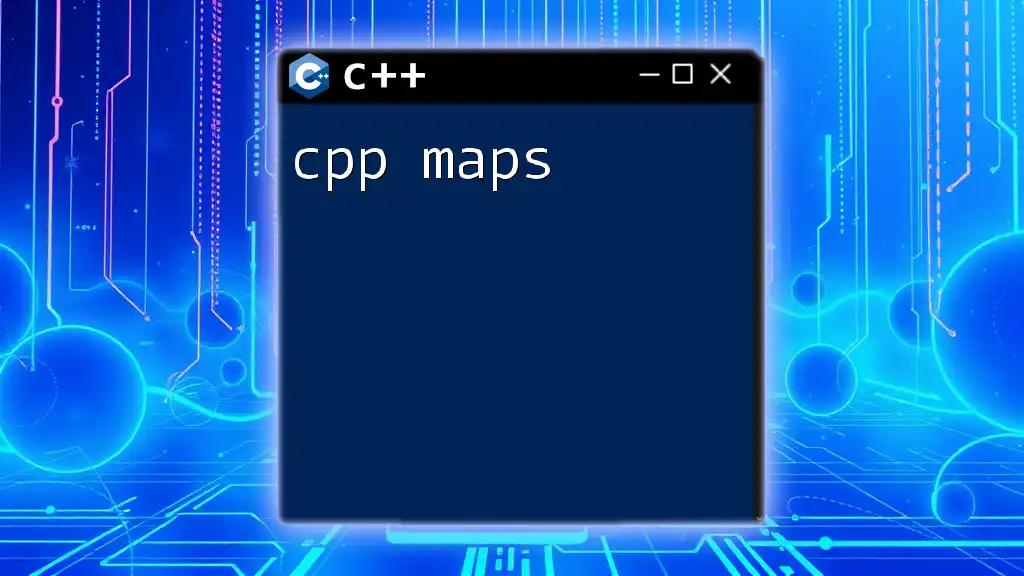
Best Practices in C++
Writing Clean and Maintainable Code
To ensure your code is easy to read and maintain, follow best practices such as:
- Using meaningful variable and function names.
- Properly commenting your code to explain complex logic.
- Organizing your code into manageable functions and classes.
Naming Conventions:
- Use camelCase for variables and functions (e.g., `myVariable`).
- Use PascalCase for class names (e.g., `CarModel`).
Error Handling
C++ provides robust error handling using exceptions. This helps manage and respond to runtime errors gracefully. Here’s how to use `try` and `catch` blocks:
try {
throw runtime_error("Sample exception");
} catch (const exception& e) {
cout << e.what() << endl; // Outputs: Sample exception
}
This example captures a thrown exception and executes the corresponding catch block, allowing for controlled management of errors.
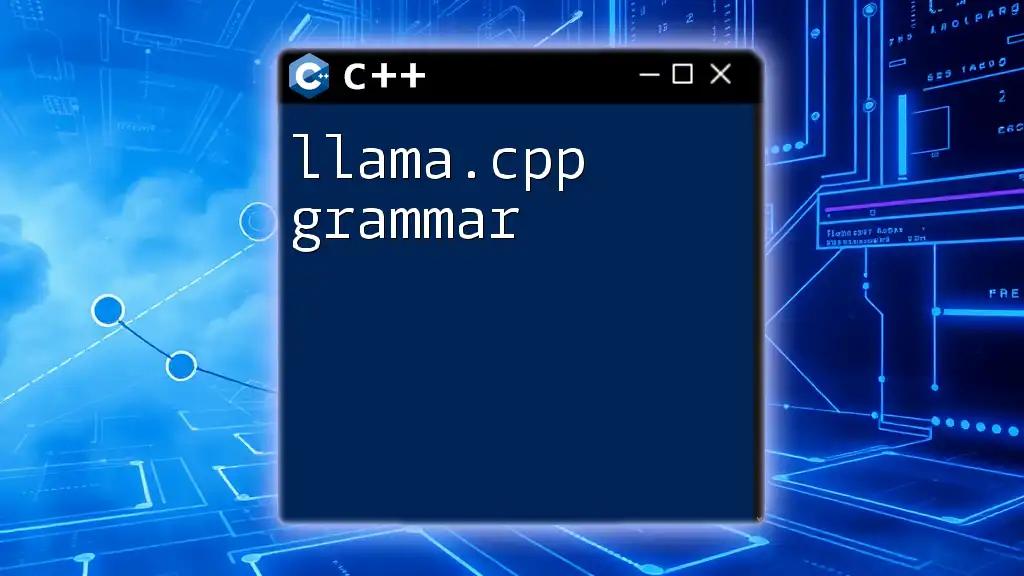
Resources and Tools
Recommended Books and Online Courses
To deepen your understanding of C++, consider the following resources:
- Books:
- "The C++ Programming Language" by Bjarne Stroustrup
- "Effective Modern C++" by Scott Meyers
- Online Courses:
- Platforms like Coursera, Udemy, and edX offer a range of courses tailored to various skill levels.
Community and Support
Engage with the C++ community through platforms like Stack Overflow or join forums and local coding groups. Sharing knowledge and experiences can significantly enhance your learning process.
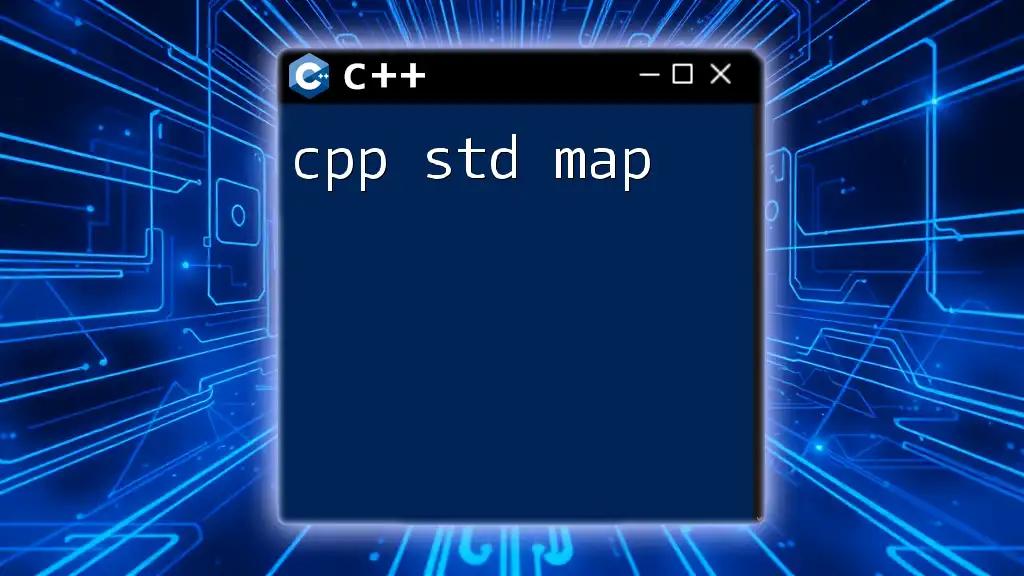
Conclusion
The C++ roadmap is more than just a guide; it's your pathway to mastering one of the most versatile programming languages available today. As you explore these concepts, remember that consistent practice is key. Don't hesitate to experiment with different features and paradigms. The journey may be challenging, but the rewards of becoming proficient in C++ are immeasurable.
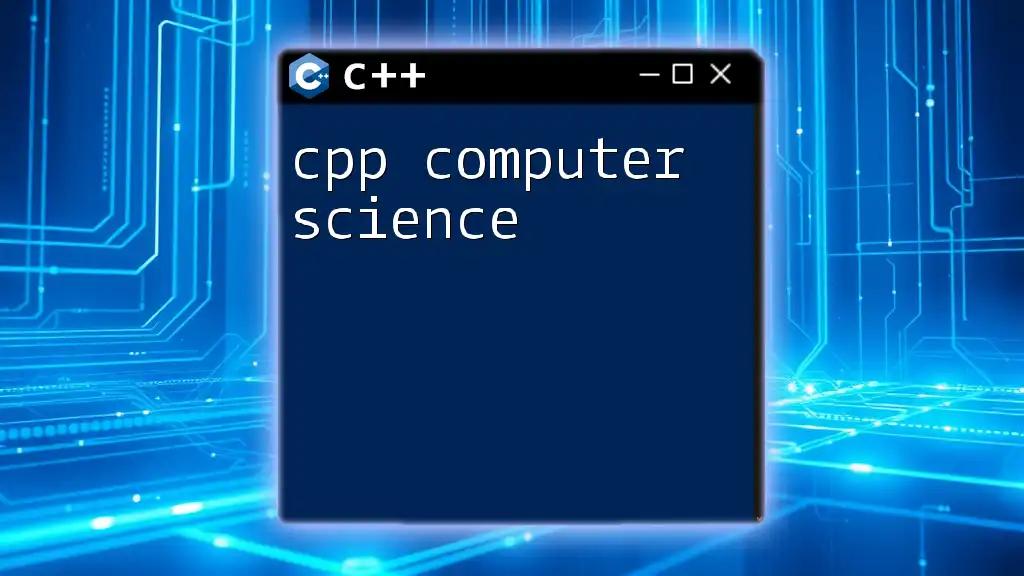
Call to Action
Ready to take your C++ skills to the next level? Sign up for our C++ courses and workshops today! Follow us on social media for regular updates and tips that will enhance your programming journey.