The "cpp cis roadmap" outlines a structured progression for mastering C++ command syntax, guiding learners from foundational concepts to advanced programming techniques.
#include <iostream>
int main() {
std::cout << "Welcome to the CPP learning roadmap!" << std::endl;
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is a powerful, high-level programming language that builds upon the foundation laid by its predecessor, C. Known for its performance and efficiency, it has become a staple in software development for a variety of applications, including system software, game development, and mobile applications. Some key features of C++ include:
- Object-Oriented Programming: This paradigm allows developers to create reusable and modular code using classes and objects.
- Low-Level Manipulation: C++ allows close interaction with hardware, making it suitable for system-level programming.
- Standard Template Library (STL): A comprehensive library that offers algorithms and data structures, which speeds up the development process.
Setting Up Your C++ Environment
Before diving into coding, you need to set up your development environment. A few steps to consider include:
- Choosing an Integrated Development Environment (IDE): Popular choices are Visual Studio, Code::Blocks, or JetBrains CLion.
- Installing a Compiler: Familiarize yourself with relevant compilers like g++ for UNIX systems or Microsoft Visual C++ for Windows.
- Testing Your Setup: Run this simple code to ensure your environment is correctly configured:
#include <iostream> int main() { std::cout << "Hello, C++ World!" << std::endl; return 0; }
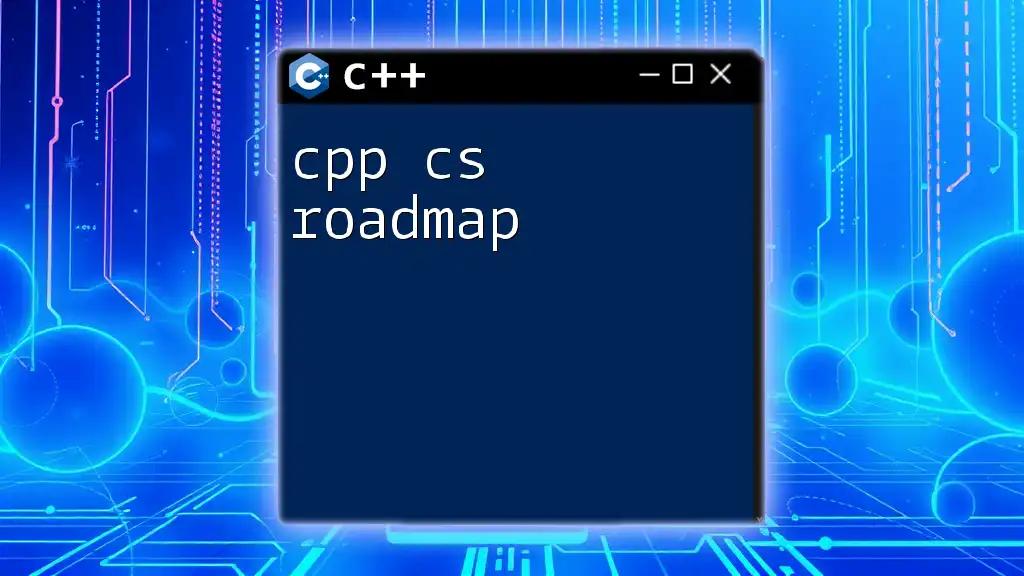
Core Concepts of C++ Programming
Variables and Data Types
In C++, variables are used to store data for processing. Understanding data types is crucial, as it defines how much memory is allocated and what operations can be performed. Major categories include:
- Fundamental Data Types: such as `int`, `float`, `char`, and `bool`.
- User-Defined Types: including `structs` and `classes` that allow you to tailor data structures to your needs.
For example, you can declare and initialize variables as follows:
int age = 30;
float salary = 60000.50f;
This snippet initializes an integer variable `age` and a float variable `salary`.
Control Structures
Control structures dictate the flow of execution in your program.
Conditional Statements
Conditional statements, such as `if`, `else if`, and `else`, allow you to execute code based on specified conditions. The `switch` statement serves as an alternate route for multiple branching paths.
Here’s an example of an `if` statement:
if (age > 18) {
std::cout << "Adult" << std::endl;
}
Loops
Loops enable the repeated execution of a block of code until a condition is met. The primary types of loops include:
- For Loop: Best for a known number of iterations.
- While Loop: Useful when the number of iterations isn’t predetermined.
- Do-While Loop: Executes at least once before checking the condition.
Functions in C++
Functions are reusable blocks of code that perform a specific task. Their utilization promotes code organization and reduces redundancy.
To define a simple function:
int add(int a, int b) {
return a + b;
}
In this example, the `add` function takes two integers as parameters and returns their sum, demonstrating both parameter passing and return values.
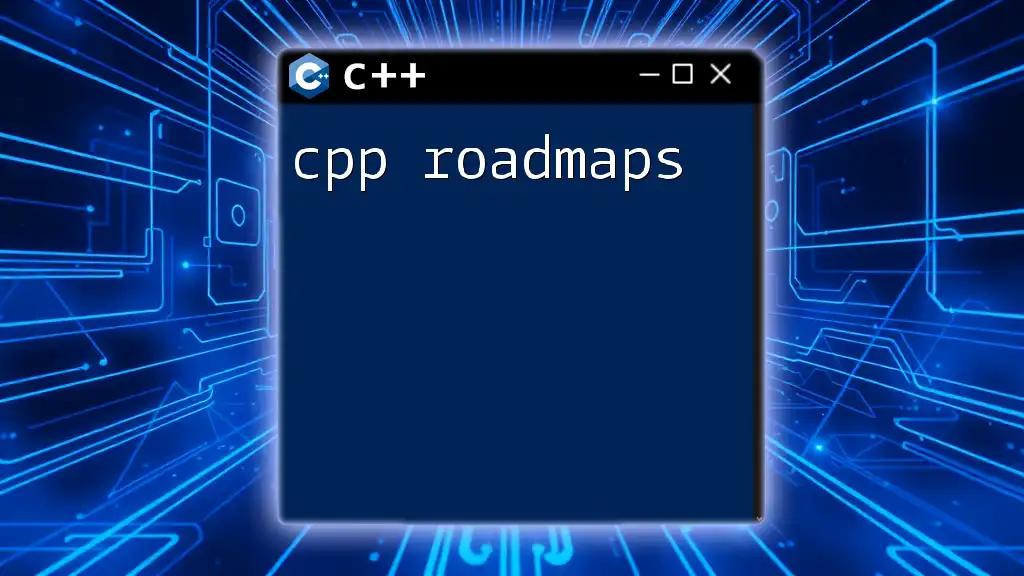
Object-Oriented Programming in C++
Introduction to OOP
Object-oriented programming (OOP) is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. The core principles of OOP are:
- Encapsulation: Bundling of data and methods that operate on that data.
- Inheritance: Allows new classes to inherit attributes and methods from existing classes.
- Polymorphism: Ability to call the same method on different objects, allowing different behaviors.
Classes and Objects
Classes serve as blueprints for creating objects. Here’s a basic structure of a class in C++:
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep beep!" << std::endl;
}
};
In this example, `Car` is a class with a public data member `brand` and a member function `honk()` to define behavior.
Inheritance and Polymorphism
Inheritance allows a class to derive attributes and behaviors from another class. C++ supports different types of inheritance, including public, protected, and private.
Polymorphism is achieved through function overriding:
class Vehicle {
public:
virtual void sound() {
std::cout << "Vehicle sound" << std::endl;
}
};
class Bike : public Vehicle {
public:
void sound() override {
std::cout << "Bike vroom" << std::endl;
}
};
In this example, the `Bike` class overrides the `sound` method of the `Vehicle` class, providing its own implementation.
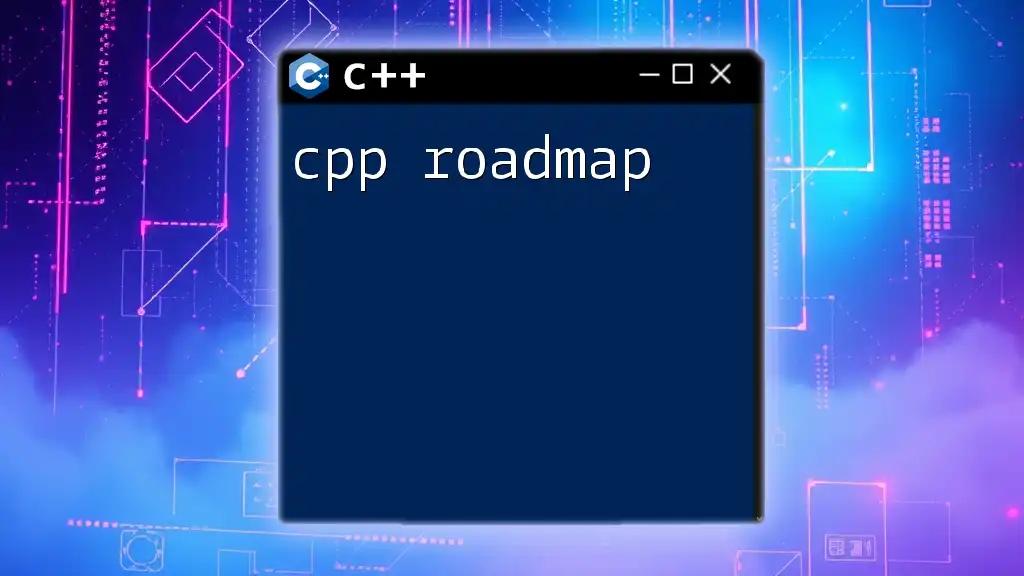
Advanced C++ Concepts
Templates and Generic Programming
Templates enable the creation of functions and classes that can operate with any data type, enhancing code reusability. Here’s a simple function template example:
template<typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
This template function can find the maximum of two values regardless of their data type.
Standard Template Library (STL)
The STL provides a rich set of generic classes and functions, including data structures like vectors, lists, and maps that simplify common programming tasks.
For example, initializing a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
This code snippet demonstrates how to create a vector that holds integers.
Exception Handling
Error management is essential for robust applications. C++ provides a mechanism known as exception handling to manage runtime errors gracefully.
The basic structure involves `try`, `catch`, and `throw` keywords:
try {
throw std::runtime_error("An error occurred");
} catch (std::exception &e) {
std::cout << e.what() << std::endl;
}
In this example, an exception is thrown, and a catch block handles it by printing the error message.
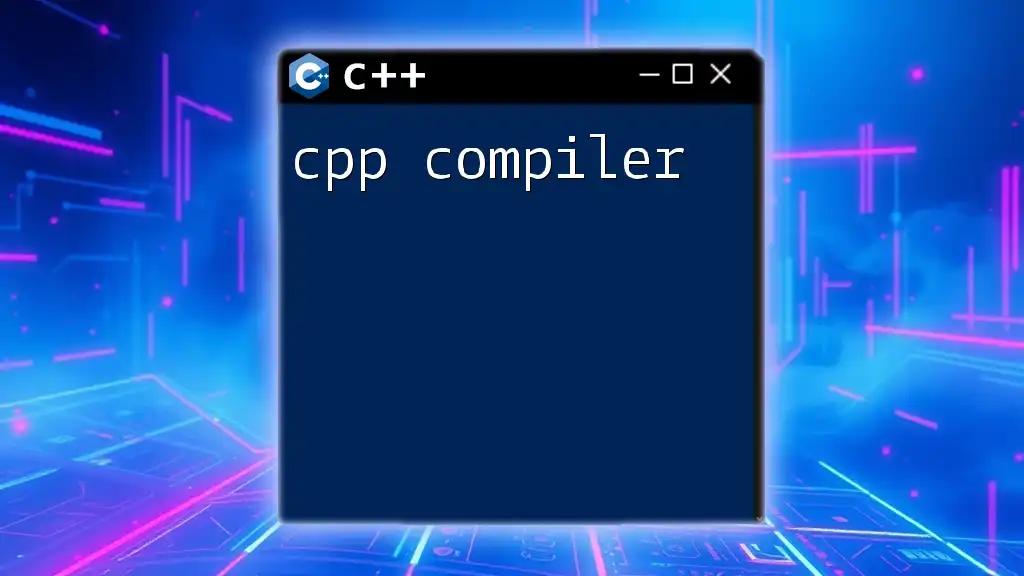
C++ Best Practices
Writing Clean Code
Writing clean code is essential for maintainability. Key practices include:
- Meaningful Naming: Choose descriptive variable and function names.
- Consistent Formatting: Use consistent indentation and control structure placement.
- Modular Design: Break down code into smaller, reusable functions.
Use of Comments
Comments play a significant role in enhancing code readability. Good commenting strategies include:
- Explain Why: Use comments to explain why a particular solution is used, rather than what is happening, which should be clear from well-named variables and code structure.
- Avoid Over-commenting: Redundant or obvious comments can clutter code and distract from understanding.
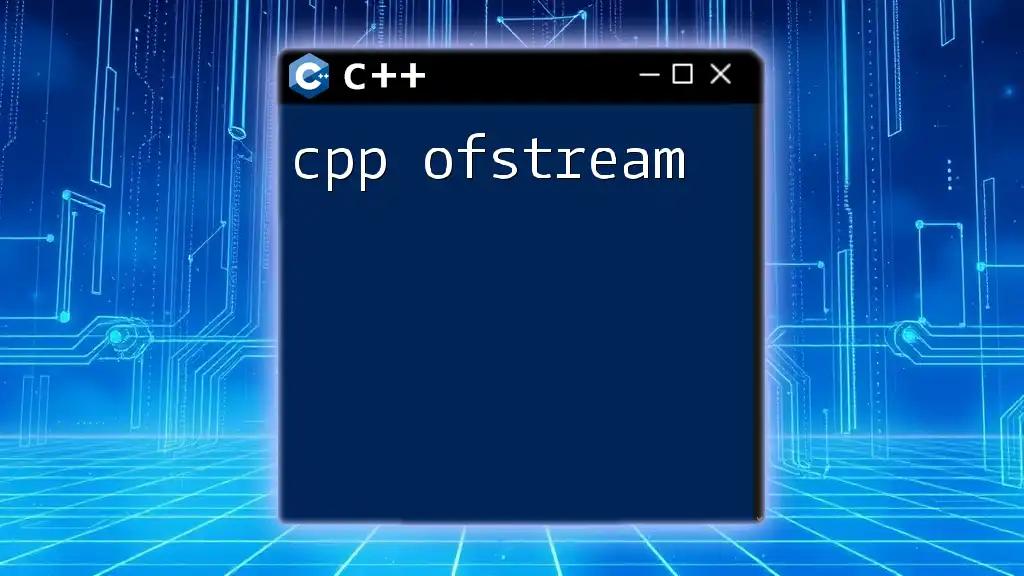
Resources for Further Learning
Recommended Books
A few notable books for enhancing your C++ skills include "Effective C++" by Scott Meyers and "C++ Primer" by Stanley B. Lippman. These resources offer in-depth knowledge that deepens understanding.
Online Courses and Tutorials
Platforms like Udemy and Coursera provide structured online courses designed for all skill levels, ensuring comprehensive coverage of C++ fundamentals and advanced topics.
Community and Forums
Engaging with the developer community can strengthen your knowledge. Consider participating in forums such as StackOverflow, Reddit's r/cpp, and the C++ section on GitHub to share insights and resolve programming challenges.
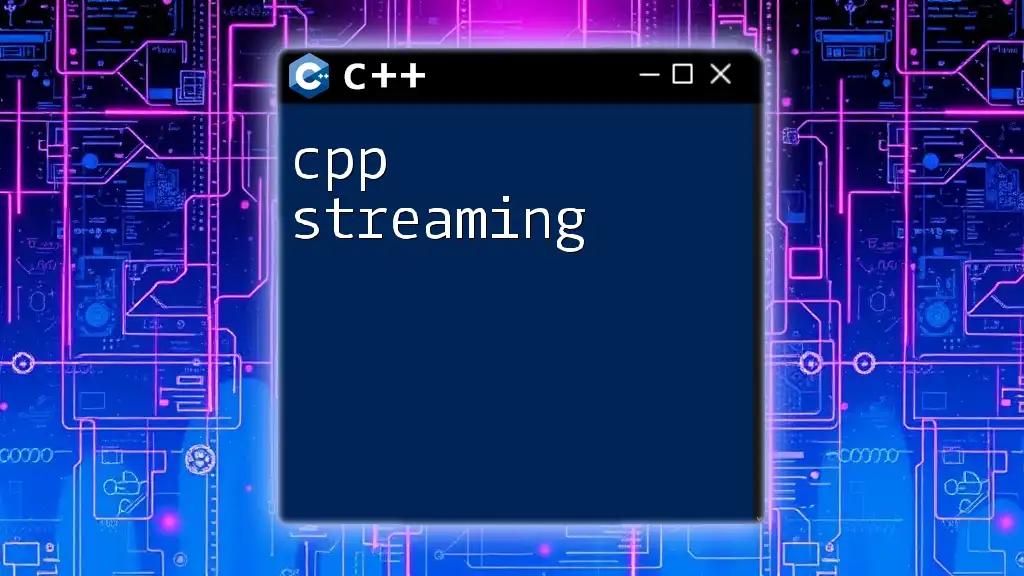
Conclusion
The C++ CIS roadmap serves as a systematic guide to mastering C++ commands and concepts efficiently. Whether you are a beginner or looking to enhance your skills, this roadmap encourages you to practice consistently and explore the vast capabilities of C++. Start your journey today, and unlock the potential of C++ programming!