A C++ roadmap outlines the essential concepts and skills necessary for mastering C++, guiding learners from basic syntax to advanced programming techniques.
Here's a simple example of a C++ program that demonstrates the basic structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding CPP Fundamentals
Basic Syntax and Structure
Keywords and Data Types
Before you dive into writing C++ programs, it's crucial to familiarize yourself with its keywords and data types. C++ has a set of reserved words, like `int`, `float`, and `return`, which serve specific functions in the syntax.
Fundamental data types include:
- int: Represents integer values.
- float: Represents floating-point (decimal) numbers.
- char: Represents single characters.
Here's an example code snippet that demonstrates how to declare variables using these data types:
int age = 25;
float height = 5.9;
char initial = 'A';
Variables and Constants
Variables are storage locations that hold data and can change during the program's execution. Constants, on the other hand, are fixed values that should not be altered after their declaration.
Here’s how you declare a variable and a constant in C++:
int score = 100; // Variable
const float PI = 3.14; // Constant
Control Structures
Conditional Statements
Control structures, particularly conditional statements, allow your program to make decisions based on different conditions. The most common conditional statements are `if`, `else if`, and `switch`.
For example:
if (score > 50) {
cout << "Passed";
} else {
cout << "Failed";
}
Looping Constructs
Loops are essential for executing a block of code repeatedly. Familiarity with `for`, `while`, and `do-while` loops is vital.
A basic `for` loop looks like this:
for (int i = 0; i < 5; i++) {
cout << i;
}
And a `while` loop might appear as follows:
int count = 0;
while (count < 5) {
cout << count;
count++;
}
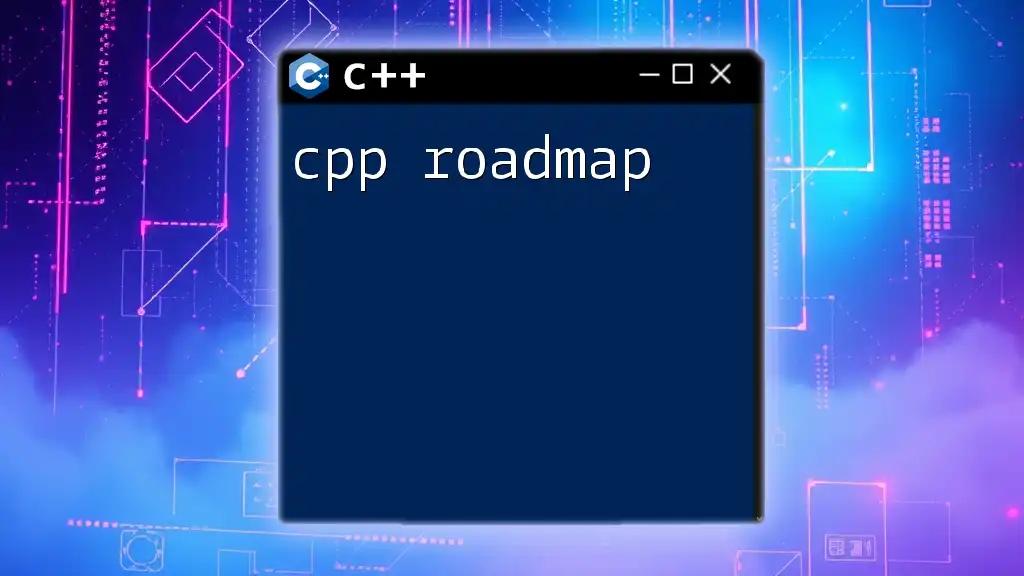
Intermediate Topics in C++
Functions
Defining and Calling Functions
Functions are reusable blocks of code that perform specific tasks. When you define a function, you should specify its return type, name, and parameters.
int add(int a, int b) {
return a + b;
}
// Calling the function
cout << add(5, 3);
Function Overloading
C++ allows multiple functions to have the same name as long as they differ in their parameter types or counts. This practice is known as function overloading.
Here’s an example:
int add(int a, int b) {
return a + b;
}
float add(float a, float b) {
return a + b;
}
Object-Oriented Programming (OOP) Concepts
Classes and Objects
C++ is an object-oriented language, making classes and objects crucial for developing applications. A class is a blueprint for creating objects, which are instances of the class.
Here's a simple definition of a class and how to create an object from it:
class Car {
public:
string model;
int year;
};
Car myCar;
myCar.model = "Toyota";
myCar.year = 2020;
Inheritance
Inheritance allows a new class to inherit properties and methods from an existing class, promoting code reusability.
Consider a base class `Vehicle` and a derived class `Car`:
class Vehicle {
public:
void start() {
cout << "Vehicle is starting";
}
};
class Car : public Vehicle {
public:
void honk() {
cout << "Car is honking";
}
};
Polymorphism
Polymorphism is the ability of different classes to be treated as objects of a common superclass. It is categorized into compile-time and runtime polymorphism. Overloading (method overloading) is compile-time, whereas overriding (method overriding) is runtime.
Example of method overriding:
class Animal {
public:
virtual void sound() {
cout << "Animal makes sound";
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Dog barks";
}
};
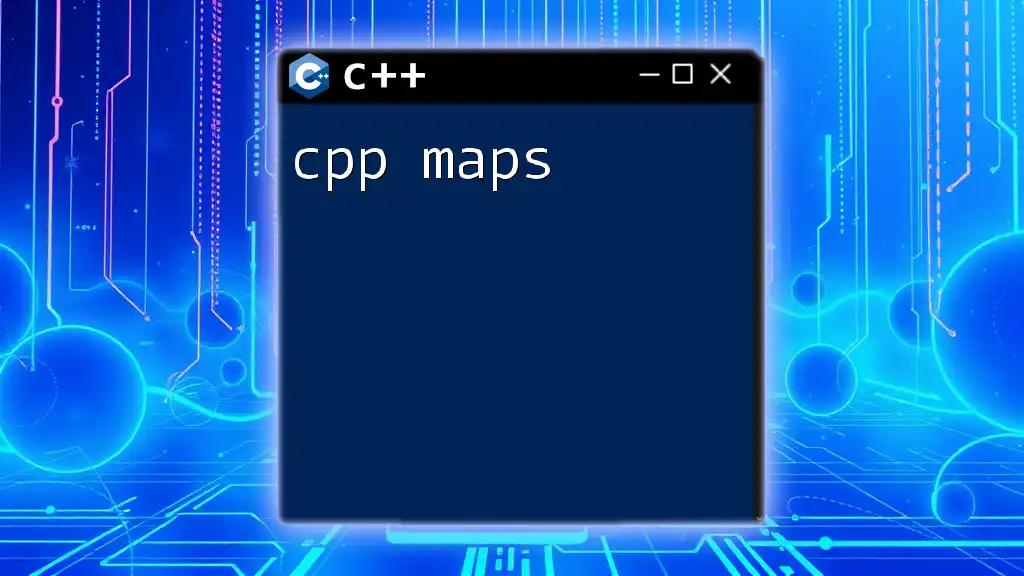
Advanced CPP Techniques
Templates and Generic Programming
Introduction to Templates
Templates allow you to write generic and reusable code. By using them, you can create functions and classes that work with any data type.
Here’s a simple function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
// Usage
cout << add<int>(5, 3);
cout << add<float>(5.5, 3.2);
Standard Template Library (STL)
Understanding STL
The Standard Template Library (STL) is a powerful set of C++ templates that provide general-purpose classes and functions. It includes data structures and algorithms, simplifying common operations.
Common STL Components
Familiarity with STL containers is highly beneficial. Important components include `vectors`, `stacks`, `queues`, and `maps`.
An example of using a vector:
#include <vector>
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num;
}
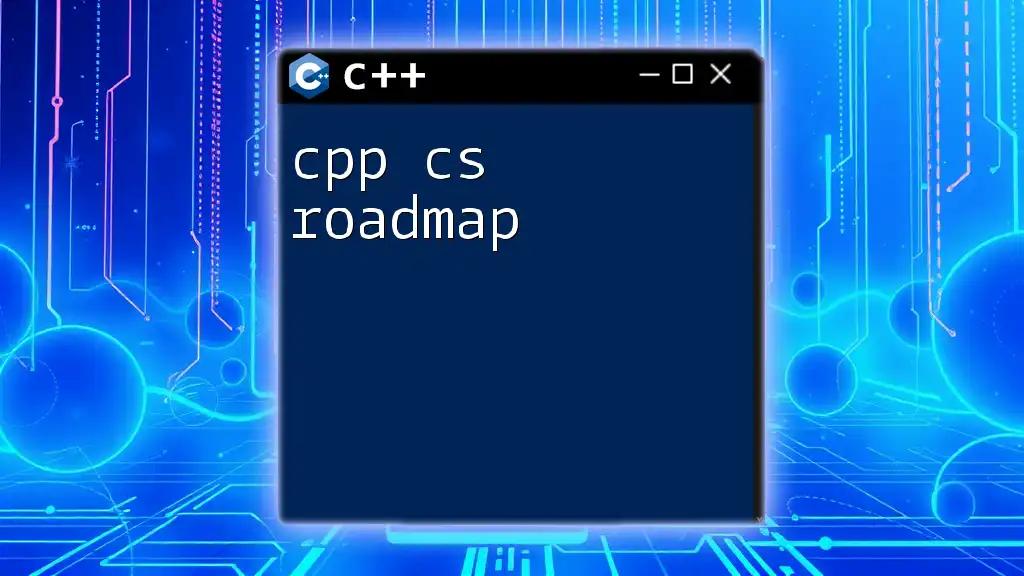
Best Practices and Tips
Coding Standards and Style
Importance of Coding Style
Maintaining a consistent coding style enhances readability and maintainability. Follow community conventions regarding indentation, naming conventions, and commenting.
Debugging and Optimization
Debugging Techniques
Effective debugging is crucial for identifying and resolving issues in your code. Leverage debugging tools and techniques, such as breakpoints and print statements, to trace program execution and inspect variable states.
Performance Optimization
How to Optimize C++ Code
Optimizing your C++ code enhances efficiency and reduces runtime. Consider the following tips for optimization:
- Use appropriate data structures for your algorithms.
- Minimize memory allocations to reduce overhead.
- Employ efficient algorithms to handle data processing.
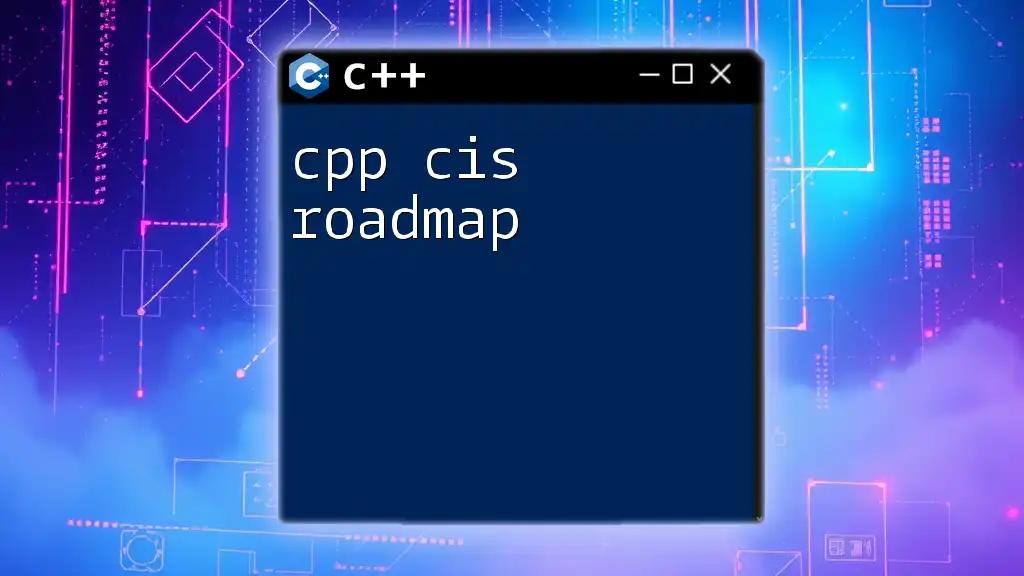
Path to Mastery
Resources for Learning C++
Online Courses and Tutorials
For structured learning, consider enrolling in online courses offered on platforms like Udemy, Coursera, or edX. These platforms provide comprehensive materials and exercises designed to enhance your understanding of C++.
Books and Documentation
Reading well-regarded books like "C++ Primer" or "Effective C++" can deepen your understanding. Additionally, always refer to the [official C++ documentation](https://en.cppreference.com/w/) for the most accurate and comprehensive information.
Joining the C++ Community
Forums and Discussion Groups
Engaging in communities like Stack Overflow or C++ subreddits can provide valuable insights and help you connect with fellow learners and experts.
Open Source Projects
Contributing to open source C++ projects is an excellent way to gain practical experience, interact with experienced developers, and reinforce your learning.
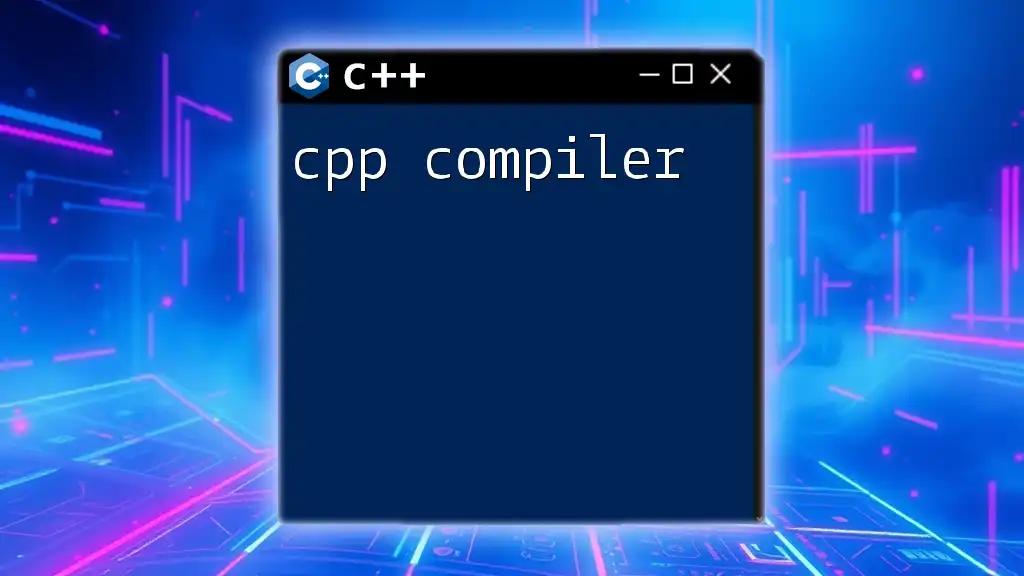
Conclusion
Having a clear roadmap for learning C++ is essential for acquiring proficiency in programming with this versatile language. Armed with the knowledge of its fundamentals, intermediate concepts, and advanced techniques, you are well-prepared to embark on your C++ journey. Embrace the process, keep practicing, and don't hesitate to leverage the resources available to you. Start your path toward becoming a C++ expert today, and remember: persistence is key!
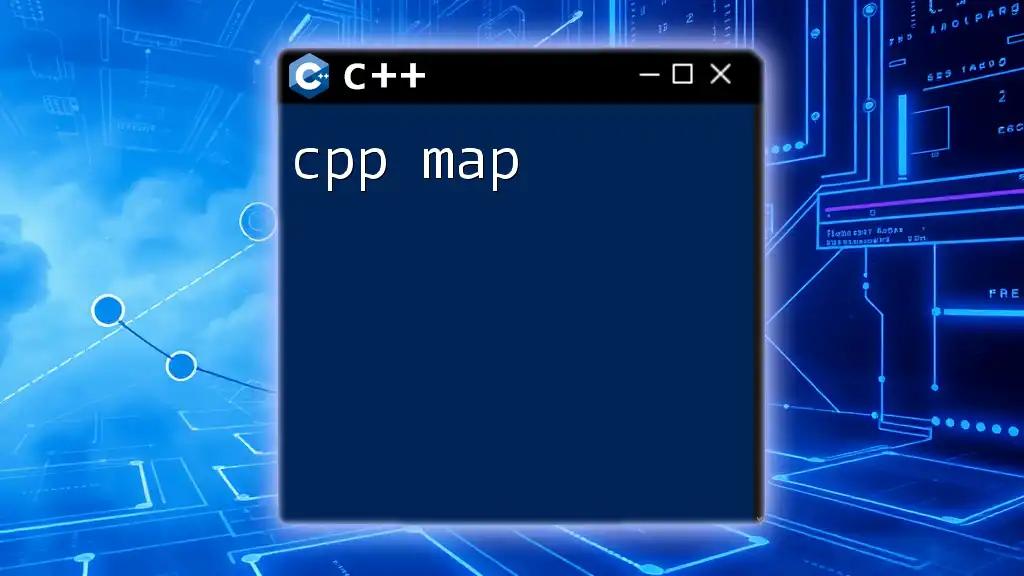
FAQs
Common Questions About Learning C++
Q: How long does it take to learn C++?
A: The time to learn C++ varies; with commitment, you can grasp the basics in a few weeks but mastering the language may take several months.
Q: What are the job opportunities available for C++ programmers?
A: C++ is widely used in software development for applications, game development, embedded systems, and high-performance computing, offering ample job opportunities.