The "cpp format" refers to the structured syntax and conventions used in C++ programming to create efficient and readable code.
Here’s a simple example of a C++ command:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Syntax
Key Syntax Elements
C++ syntax is fundamental to understanding how to write effective programs. It consists of a collection of keywords, symbols, and rules that dictate how code is structured. Essential keywords in C++ include `int`, `if`, `for`, `class`, among others. Each serves a distinct purpose, such as defining data types, making decisions, and creating loops.
When writing in C++, adhering to syntax rules is crucial. Here's a simple example of syntax in action:
int main() {
return 0;
}
In this snippet, `int` is a keyword that defines the return type of the function, while `main()` is the entry point of any C++ program. A common pitfall is forgetting to include semicolons at the end of statements, which can lead to syntax errors.
Code Blocks and Scope
Code blocks are areas of enclosed code that determine the scope of variables. In C++, curly braces `{}` are used to create these blocks. Understanding scope is vital, as it helps manage variable accessibility throughout the program. Variables defined within a block cannot be accessed from outside it, which helps prevent unintended side effects.
Here’s an example showcasing the use of code blocks:
#include <iostream>
int main() {
int x = 10; // x is accessible here
{
int y = 20; // y is only accessible within this block
std::cout << "y: " << y << std::endl; // prints 20
}
// std::cout << y; // This line would throw an error
return 0;
}
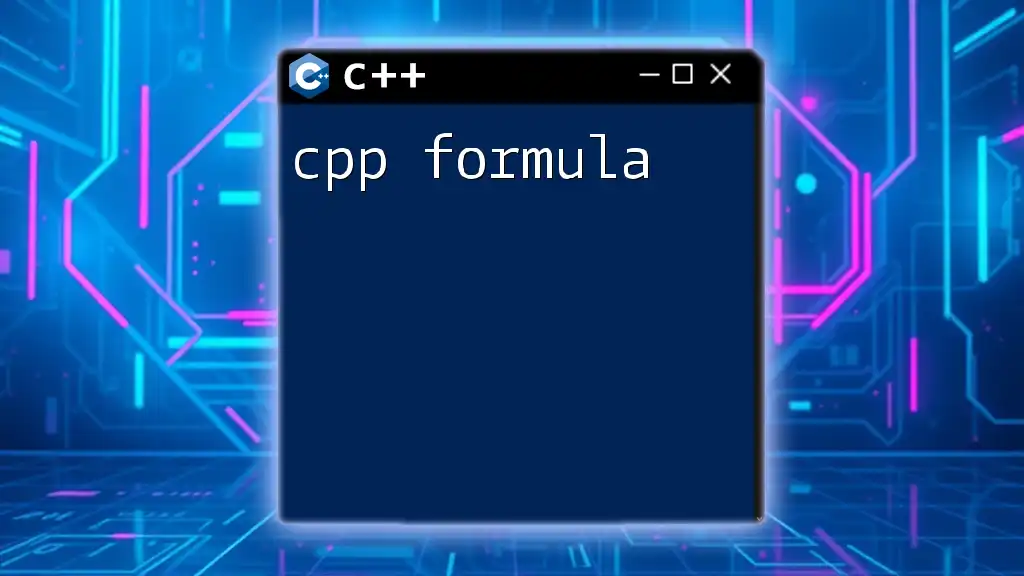
The Basics of C++ Formatting
Indentation Practices
Proper indentation serves as the foundation of readable code in C++. It visually represents the structure and flow of the program, allowing developers to quickly grasp the relationships between various code blocks. Consistent indentation helps avoid confusion, especially in complex programs.
For example, consider this poorly indented code:
int main(){int x=10;if(x>5){std::cout<<x;}}
This code is difficult to parse. Now look at the same code, well-indented:
int main() {
int x = 10;
if (x > 5) {
std::cout << x;
}
}
The second example is much clearer. Following best practices, it’s recommended to use either 4 spaces or a tab for indentation and to apply it uniformly throughout your project.
Line Length and Wrapping
Maintaining a reasonable line length is crucial in C++, with the typical recommendation being 80-120 characters per line. Long lines can make code harder to read and understand. When a line exceeds this limit, wrapping is necessary. Implementing this requires careful formatting.
Here's an example of a long line that should be wrapped:
std::cout << "This is an example of a very long line of code that exceeds the usual length limit.";
A better approach would be:
std::cout << "This is an example of a very long line of code "
<< "that exceeds the usual length limit.";
Utilizing concatenation or breaking lines at logical points enhances readability.
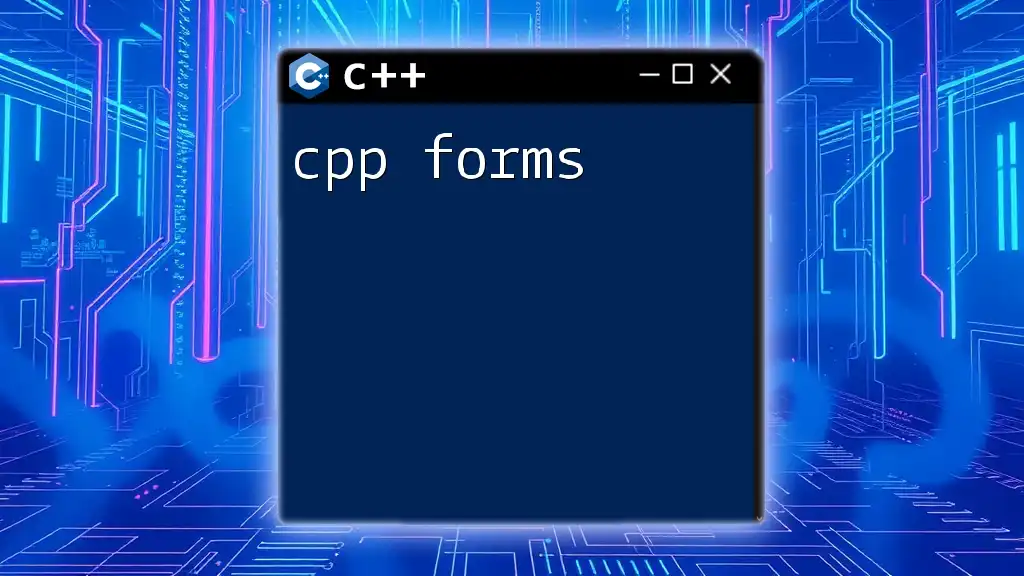
Naming Conventions
Variable and Function Naming
Naming conventions play a significant role in maintaining the readability and maintainability of your code. Using clear and descriptive names allows other developers to understand the purpose of a variable or function at a glance. Common practices include camelCase (e.g., `myVariable`) and snake_case (e.g., `my_variable`).
Here is an example that highlights good vs. bad naming:
int a; // bad
int customerAge; // good
Avoid ambiguous names; instead, opt for descriptive identifiers that express their intent.
Class and Method Naming
Classes and methods also follow specific naming conventions that enhance clarity. Typically, classes are named using PascalCase (e.g., `CustomerAccount`), while methods may use camelCase. Consistent naming for classes and methods clarifies their roles in the codebase.
Consider this effective naming strategy:
class ShoppingCart {
public:
void addItem(Item item);
void removeItem(Item item);
};
Clarity in class and method names builds a strong foundation for code comprehension, which simplifies collaboration and maintenance.
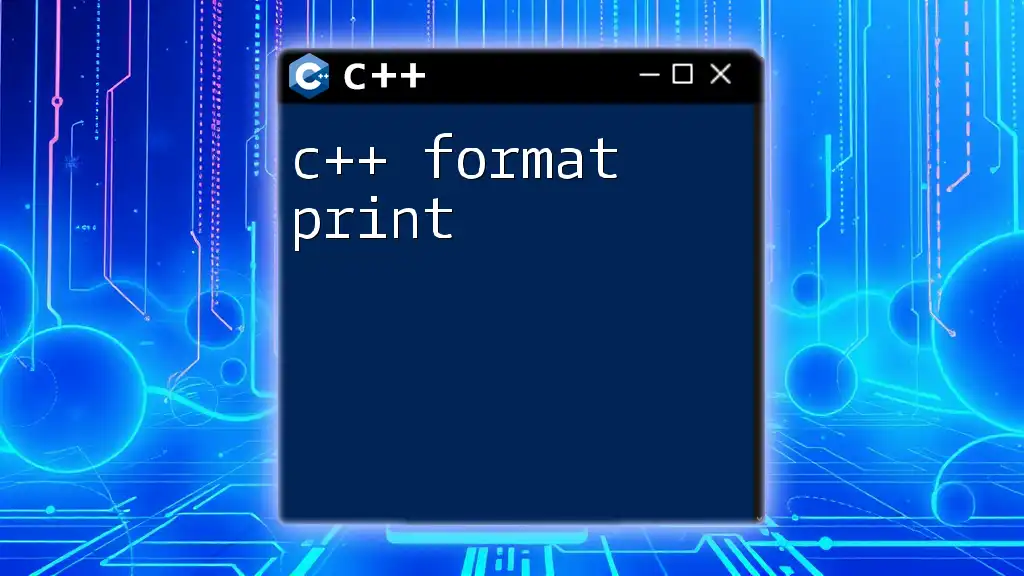
Commenting in C++
Importance of Comments
Comments are an essential element of C++ programming. They allow developers to leave notes for themselves and others about the purpose of specific sections of code. An insightful comment can drastically reduce the time required to understand a complex piece of logic.
Best Practices for Commenting
When writing comments, ensure they are meaningful and concise. Avoid stating the obvious - instead, clarify the intent behind complicated code. Here’s an example of effective commenting:
// Calculate the area of a rectangle
int area = length * width; // length and width are in meters
In contrast, this comment is unnecessary:
int x = 10; // set x to 10
Overall, aim for comments that explain why a piece of code exists rather than repeating what the code is doing.
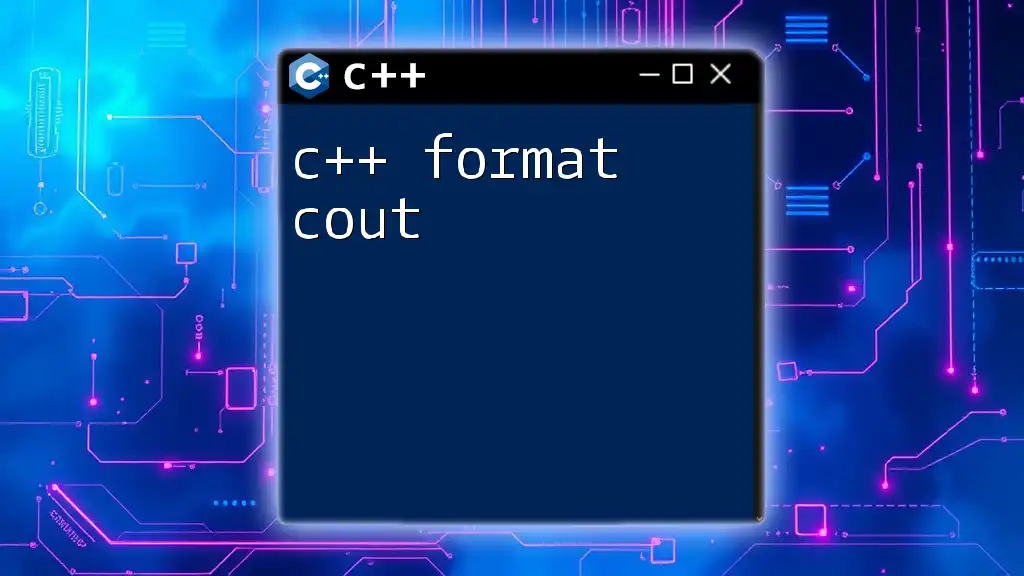
Structuring C++ Files
File Organization
Organizing your C++ files methodically enhances project manageability. A conventional approach includes separating header and source files. Header files typically contain declarations and are named with a `.h` extension, while source files include implementation and utilize a `.cpp` extension.
For instance, a common directory structure may look like this:
/my_cpp_project
|-- src/
| |-- main.cpp
| |-- utils.cpp
|-- include/
| |-- utils.h
Including Libraries and Dependencies
When utilizing external libraries, it's essential to include them properly. The `#include` directive is used for this purpose, which integrates library functionality into your program. Here’s how it can be done:
#include <iostream> // standard input-output library
#include "myfunctions.h" // user-defined functions
This approach streamlines the integration of additional features and enhances code functionality.
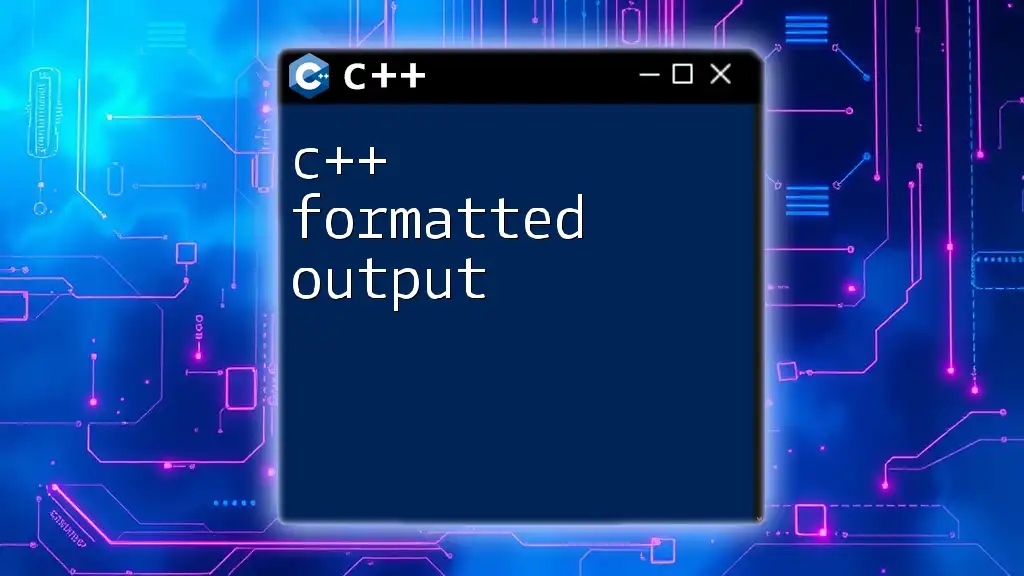
Advanced Formatting Techniques
Using Formatting Tools
Utilizing formatting tools can elevate your C++ code's quality without requiring extensive manual corrections. Tools like ClangFormat automatically format code according to your specified rules and styles, improving consistency across your codebase. Setting up formatting rules can typically be done in your IDE’s preferences or through configuration files.
Code Reviews and Consistency
Conducting regular code reviews is essential for maintaining formatting standards. Establishing conventions as a team ensures consistency. A checklist can be invaluable, focusing on aspects of formatting such as:
- Indentation style
- Line length compliance
- Comment usage
- Naming convention adherence
By fostering a culture of review and collaboration, teams can significantly diminish formatting issues and improve overall code quality.
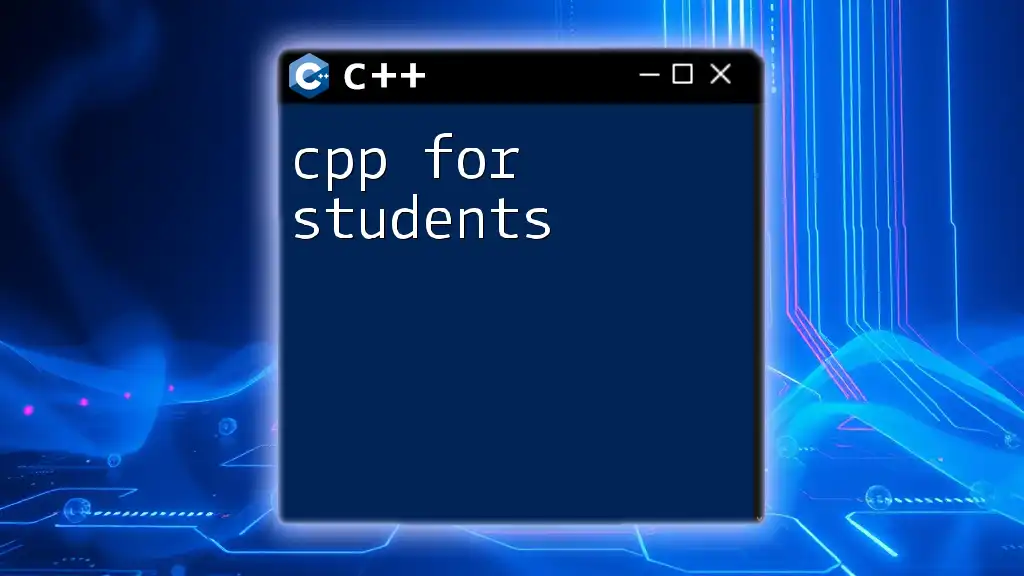
Conclusion
Adopting a well-defined cpp format enhances the overall quality and maintainability of your code. The guidelines outlined in this article serve as a solid foundation for writing clean, effective C++ programs. By practicing good formatting habits, you can become a more competent and efficient developer. Stay engaged with further resources and communities to continuously improve your coding skills.
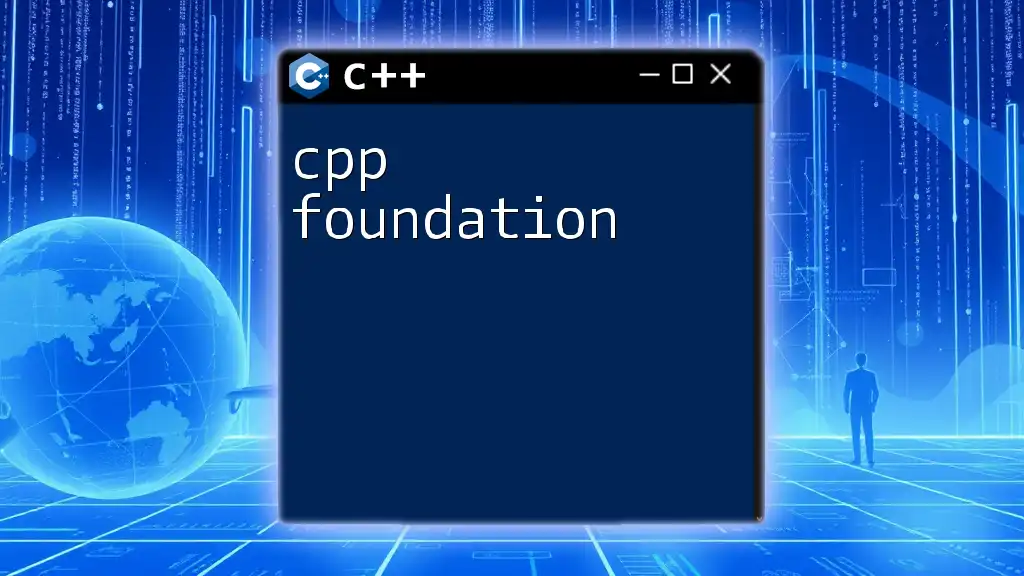
Additional Resources
For those eager to enhance their C++ knowledge, consider exploring recommended books, online courses, and community forums. Tapping into these resources can significantly broaden your understanding and capabilities in C++ programming.
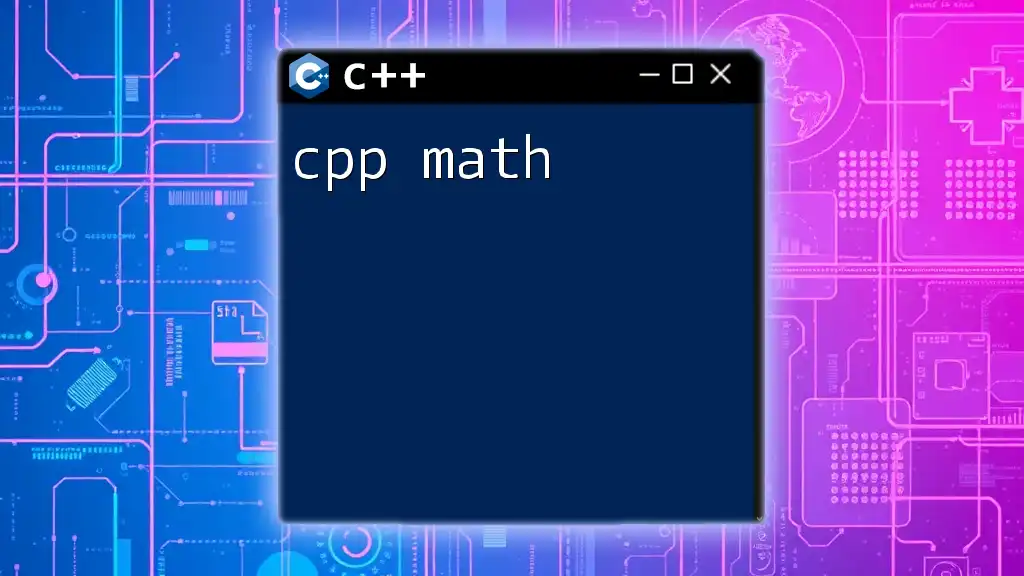
FAQs about C++ Formatting
Commonly asked questions concerning C++ format are prevalent among new developers. Addressing these queries clearly assists in fostering a deeper understanding of proper C++ coding practices.