C++ math encompasses the various mathematical operations and functions you can perform using the C++ programming language, allowing developers to efficiently manipulate numerical data.
Here's a simple example using basic arithmetic operations:
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = 5;
cout << "Addition: " << (a + b) << endl;
cout << "Subtraction: " << (a - b) << endl;
cout << "Multiplication: " << (a * b) << endl;
cout << "Division: " << (a / b) << endl;
return 0;
}
Setting Up Your C++ Environment
Before diving into cpp math, it's essential to set up your development environment. This ensures that you can compile and run your C++ programs effectively.
Installing a C++ Compiler
A compiler is necessary to translate your C++ code into machine language that a computer can understand. Popular C++ compilers include `g++`, `clang`, and Microsoft's Visual C++. To get started, follow these steps:
- For g++: You can install it via package managers like `apt` on Ubuntu or `brew` on macOS.
- For clang: Installation is also typically done through a package manager or from official downloads.
- For Visual C++: You can install it through the Visual Studio Installer on Windows.
Choosing an Integrated Development Environment (IDE)
An IDE simplifies coding by providing features like syntax highlighting, auto-completion, and debugging tools. Popular options include:
- Visual Studio: Great for Windows users with a user-friendly interface.
- Code::Blocks: An open-source IDE that works on multiple platforms.
- Eclipse CDT: Known for extensibility and supporting various programming languages.
Configure your chosen IDE to support C++ file types and ensure the compiler is correctly linked.
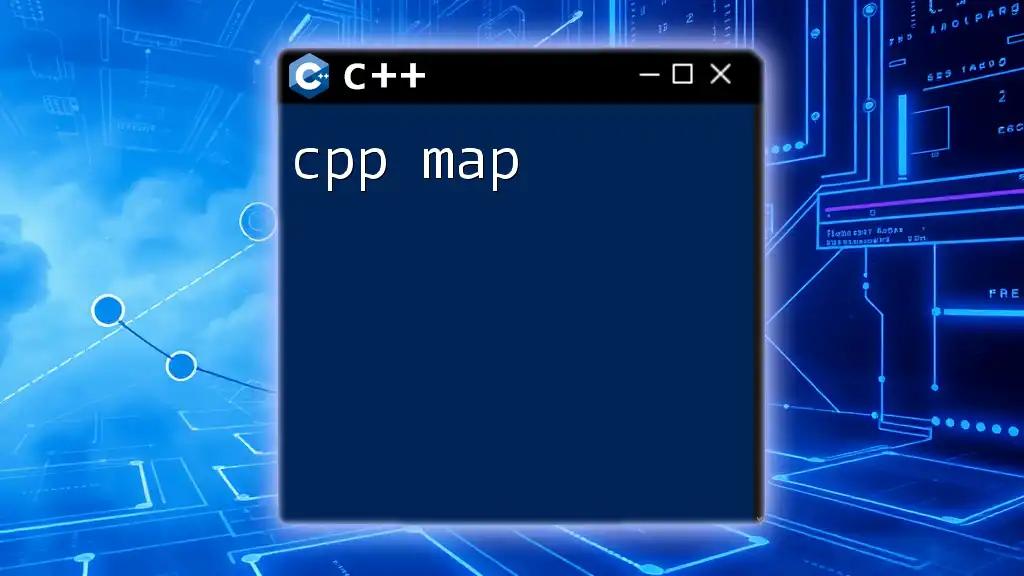
Basic Mathematical Operations in CPP
Mathematical operations are at the heart of programming in cpp math. Understanding the basic operators is crucial for performing calculations efficiently.
Addition, Subtraction, Multiplication, and Division
To perform these operations, C++ uses standard operators like `+`, `-`, `*`, and `/`. Here's a simple example:
int a = 5;
int b = 3;
int sum = a + b; // sum is 8
int difference = a - b; // difference is 2
int product = a * b; // product is 15
float quotient = (float)a / b; // quotient is approximately 1.67
Keep in mind that the division operator `/` will perform integer division if both operands are integers, which can lead to unexpected results.
Working with Modulus Operator
The modulus operator `%` is particularly useful in programming for finding the remainder of a division operation. It’s handy for various tasks, such as determining whether a number is even or odd:
int remainder = a % b; // returns 2, since 5 divided by 3 leaves a remainder of 2
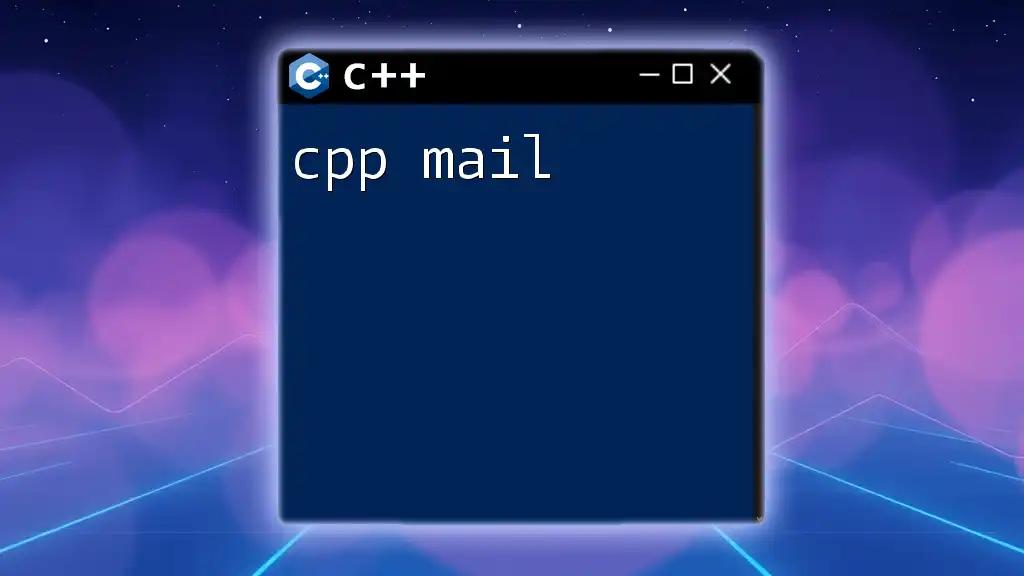
Advanced Math Functions in CPP
For more complex mathematical operations in cpp math, C++ provides a dedicated library called `<cmath>`. This library includes various functions for performing advanced calculations.
Using the `<cmath>` Library
The `<cmath>` library houses essential math functions, which can vastly extend your programming capabilities. Be sure to include it at the top of your program:
#include <cmath>
Common Math Functions
Among the many functions available, here are a few that you'll find particularly useful:
-
Square Root: The `sqrt()` function computes the square root of a number.
double root = sqrt(16); // returns 4.0
-
Power Function: The `pow()` function allows you to raise a number to a specific exponent.
double power = pow(2, 3); // returns 8.0
-
Trigonometric Functions: Functions like `sin()`, `cos()`, and `tan()` are essential for any calculations involving angles. Remember that these functions operate in radians, so conversion from degrees is necessary:
double angle = 30.0; // degrees double radians = angle * (M_PI / 180.0); // Convert to radians double sine = sin(radians); // returns 0.5
Rounding Functions
Rounding is another essential aspect of cpp math. C++ provides several functions for rounding numbers:
- Floor: Use `floor()` to round down to the nearest integer.
- Ceil: Use `ceil()` to round up to the nearest integer.
- Round: The `round()` function rounds to the nearest integer based on standard rounding rules.
double number = 3.7;
double rounded = round(number); // returns 4.0
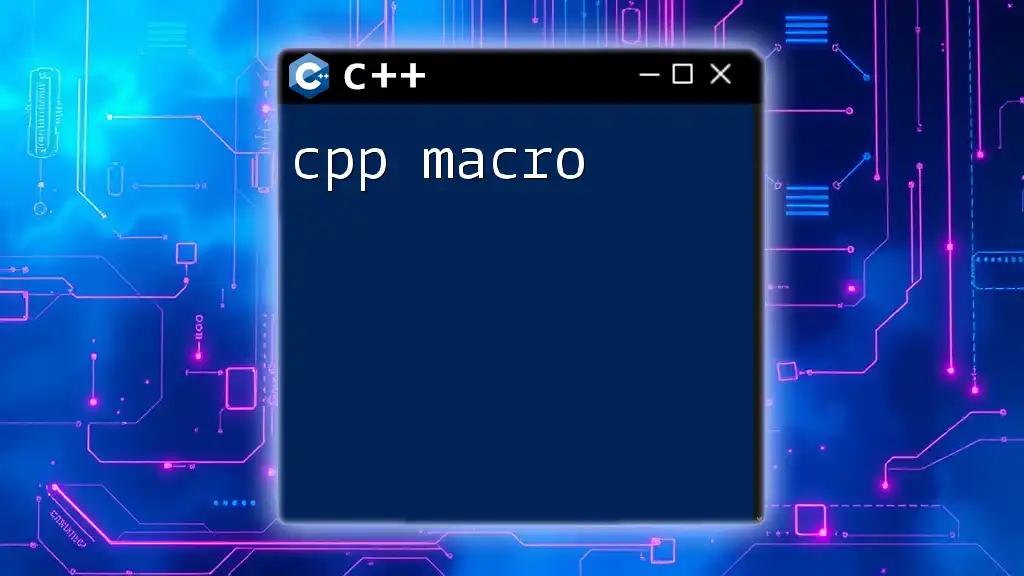
Complex Mathematical Operations
As your proficiency with cpp math grows, you may want to perform more complex calculations, such as operations on arrays and matrices.
Working with Arrays and Matrices
Arrays are fundamental for storing collections of data, allowing for efficient mathematical operations on multiple values. Here’s a straightforward example of how to calculate the sum of elements in an array:
int arr[] = {1, 2, 3, 4};
int arrSum = 0;
for (int i = 0; i < 4; i++) {
arrSum += arr[i]; // summing up values
}
Implementing User-Defined Functions for Math Operations
Custom functions are powerful because they allow you to encapsulate mathematical logic and reuse it throughout your code. For example, you could create a simple addition function:
double add(double x, double y) {
return x + y;
}
Using such functions makes it easier to maintain and understand your code.
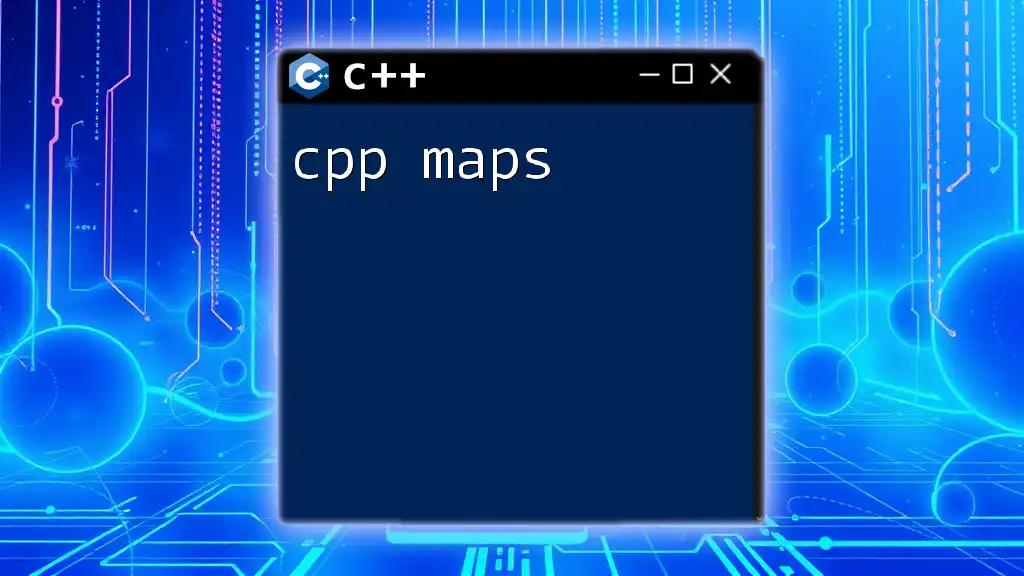
Error Handling in C++ Math Operations
In programming, handling errors, especially in mathematical operations, is crucial to ensure smooth execution and prevent crashes.
Managing Mathematical Errors
One common error is division by zero, which will lead to runtime exceptions. Always validate inputs to avoid this:
if (b != 0) {
quotient = (float)a / b;
} else {
std::cout << "Error: Division by zero!";
}
By implementing checks like this, you can make your programs more robust and user-friendly.
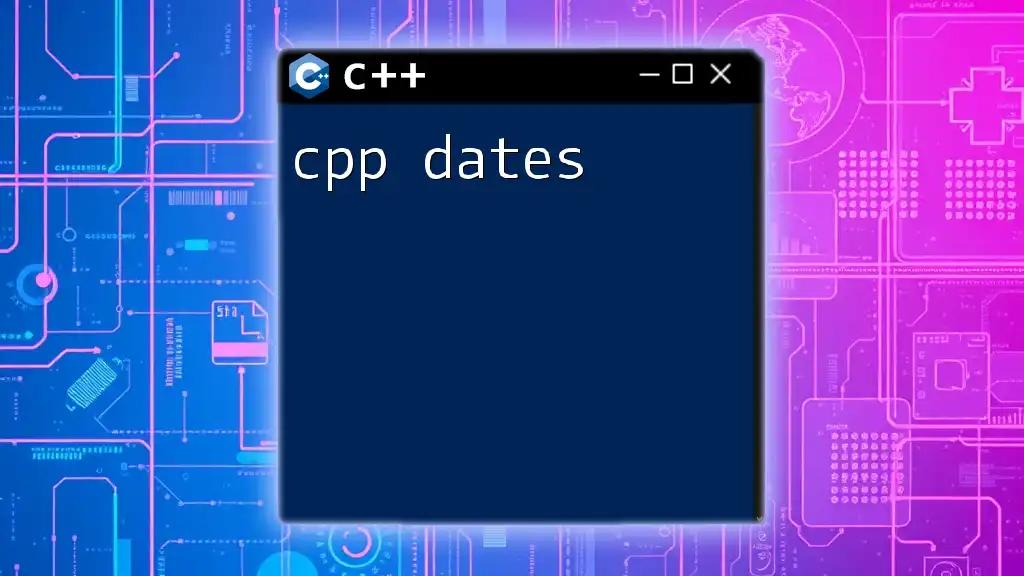
Conclusion
In this guide, we explored the fundamentals of cpp math, covering basic operations, advanced functions from the `<cmath>` library, and error handling strategies. Mastering these concepts is invaluable for any programmer looking to enhance their skills.
Remember, practice is key. Experiment with the examples provided and consider expanding your knowledge through additional resources. The world of C++ has much to offer, especially in the realm of mathematical computation.