The `this_thread` namespace in C++ provides functionality to manage the execution of the current thread, including sleeping and yielding to allow other threads to run.
Here's a simple code snippet demonstrating how to use `this_thread::sleep_for` to pause execution for a specified duration:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Starting to sleep..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2)); // Sleep for 2 seconds
std::cout << "Woke up!" << std::endl;
return 0;
}
What is `this_thread`?
In C++, the `this_thread` namespace is a vital component of the threading library, introduced in C++11. It provides a set of functions that allow you to control the execution of threads within your application. The `this_thread` namespace includes functionalities for thread management, sleeping, and obtaining thread identifiers. This makes it easier to work with threads, enhancing the program's efficiency and responsiveness.
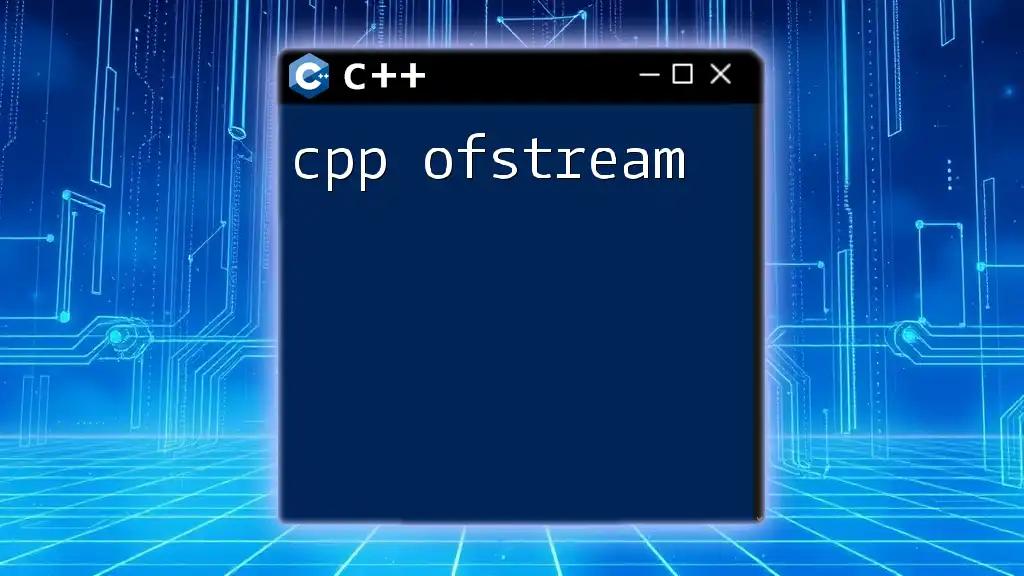
Understanding Threads in C++
Threads are the smallest units of processing that can be scheduled and executed by an operating system. Each thread represents a separate flow of control running concurrently with other threads.
Advantages of Using Threads
-
Concurrency: Threads can run simultaneously, allowing multiple operations to be performed at the same time. This can lead to performance improvements, especially in applications that require handling numerous tasks concurrently.
-
Responsiveness: In graphical user interfaces (GUIs), multithreading can enhance responsiveness by handling user input while performing background operations, preventing the UI from freezing.
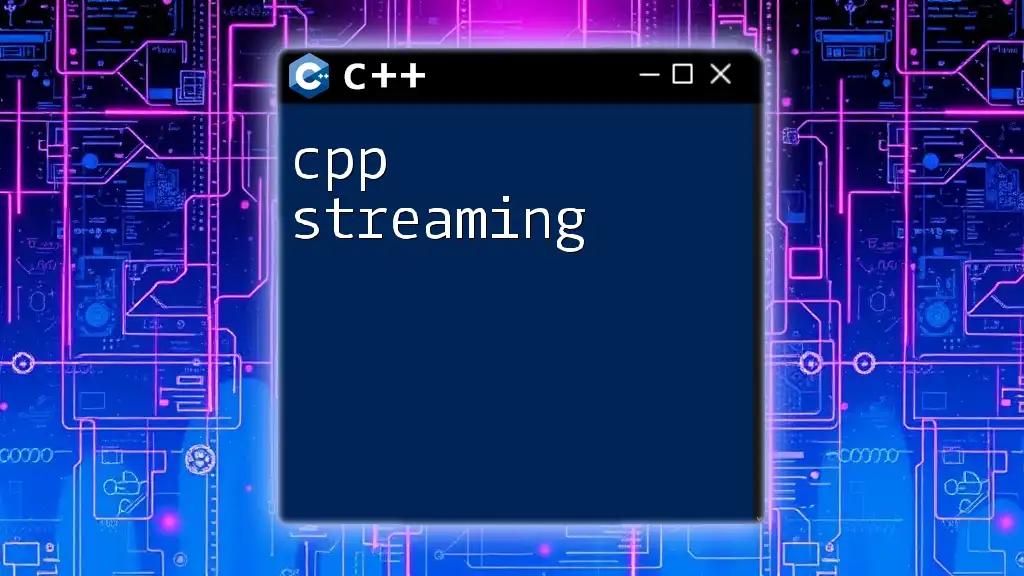
How to Use `this_thread`
To leverage the capabilities of `this_thread`, you need to include the relevant header file in your program:
#include <iostream>
#include <thread>
Basic Example of `this_thread`
Using `this_thread` is relatively straightforward. Below is an example that demonstrates how to create a simple thread:
void example_function() {
std::cout << "Hello from a new thread!" << std::endl;
}
int main() {
std::thread t(example_function); // Creating a new thread
t.join(); // Joining the thread to ensure it completes execution
return 0;
}
In this example, `example_function` runs in a separate thread from the `main` function. The `join()` method ensures that the `main` function waits for the thread to complete before it continues.
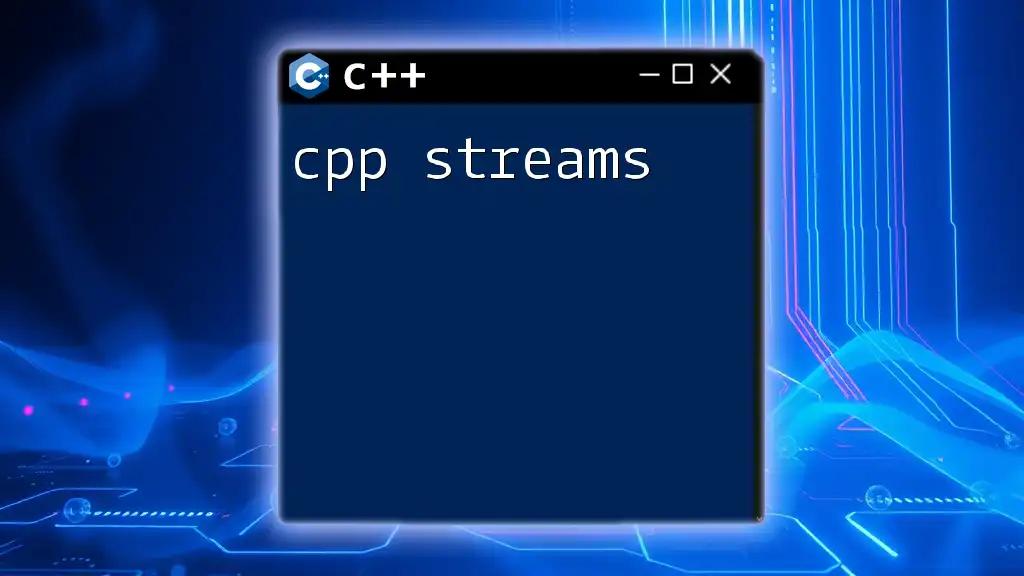
Key Functions in `this_thread`
Several critical functions in the `this_thread` namespace are essential for effective thread management.
sleep_for()
The `sleep_for` function is used to suspend the current thread for a specified duration. Here's how you can use it:
std::this_thread::sleep_for(std::chrono::milliseconds(1000)); // Sleeps for 1 second
std::cout << "Slept for 1 second." << std::endl;
This creates a delay in the executing thread, allowing other threads to run in the interim.
sleep_until()
Unlike `sleep_for`, which takes a duration as its parameter, `sleep_until` pauses the thread until a specific point in time. Here's an example:
auto now = std::chrono::system_clock::now();
std::this_thread::sleep_until(now + std::chrono::seconds(2)); // Sleeps until 2 seconds later
std::cout << "Slept until 2 seconds." << std::endl;
This can be particularly useful when you need precise timing for thread execution.
get_id()
The `get_id()` function returns the identifier of the thread. This can be particularly useful for debugging or logging purposes. Here’s how you can implement it:
std::thread t(example_function);
std::cout << "Thread ID: " << t.get_id() << std::endl; // Retrieves and prints the thread ID
t.join(); // Joins the thread
Using `get_id()` can be beneficial to trace which threads are active in a multithreaded application.
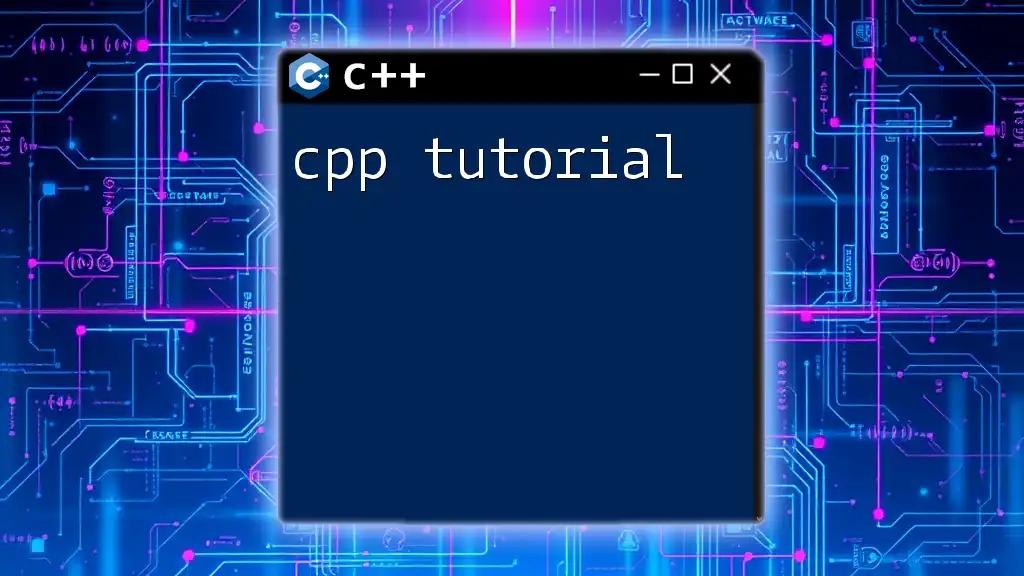
Thread Management with `this_thread`
Effective thread management is crucial in developing a stable and responsive application.
Joining and Detaching Threads
Thread management involves knowing when to join or detach threads.
Join: The `join()` method blocks the calling thread until the thread represented by the object completes its execution. It ensures that the program waits for the specific thread to finish.
std::thread t1(example_function); // Creating a thread
t1.join(); // Wait for t1 to finish
Detach: The `detach()` method allows a thread to execute independently. Once detached, the program does not need to wait for the thread to finish before it continues. Note that once a thread is detached, it cannot be joined.
std::thread t2(example_function);
t2.detach(); // Allow t2 to run independently
Understanding when to use `join` versus `detach` is vital for effective multithreaded programming to ensure proper resource management and avoid potential memory leaks.
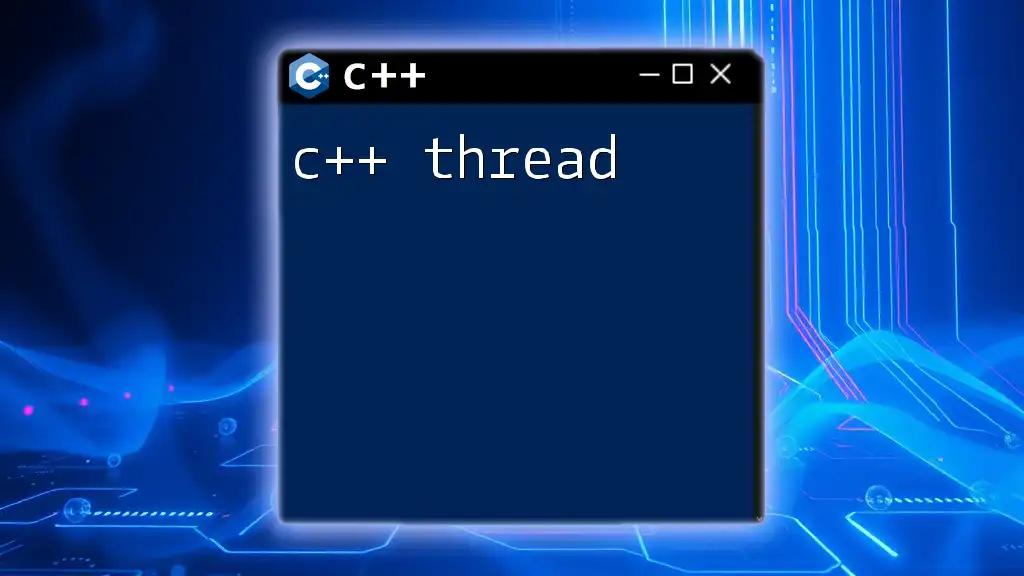
Best Practices in Multithreading
To develop effective multithreaded applications, consider the following tips:
-
Avoid Race Conditions: Ensure that shared resources are properly synchronized to prevent race conditions, where two threads access shared data simultaneously.
-
Use Locks: Utilize mutexes or other synchronization mechanisms to control access to shared resources.
-
Keep Threads Lightweight: Avoid heavyweight thread operations and strive to keep your threads focused on specific tasks to enhance efficiency.
-
Handle Exceptions: Be cautious of exceptions thrown by threads. Consider using `try-catch` blocks to handle errors gracefully.

Real-World Applications
The `this_thread` namespace provides functionality that's valuable in various domains:
-
Game Development: In games, threading can be used to update graphics while processing input in the background, resulting in smoother gameplay.
-
Servers: Multithreading enables servers to handle multiple client requests simultaneously, ensuring better performance and responsiveness.
-
Responsive GUIs: Applications with GUIs can use threading to perform heavy operations in the background while keeping the user interface responsive.

Conclusion
In summary, `cpp this_thread` enhances your ability to work with threads in C++, allowing for improved concurrency and performance. Understanding the key functions and their applications can significantly augment your programming capabilities in multi-threaded environments.
As you delve deeper into multithreading with `this_thread`, you will discover its potential to transform your programming and application development experience. For those looking to further expand their knowledge, consider exploring additional C++ resources and documentation that focus on threading and concurrent programming principles.