In C++, the `std::ifstream` class is used to read from files in a straightforward manner, enabling you to extract data easily.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cout << "Unable to open file";
}
return 0;
}
Understanding File Streams in C++
What Are File Streams?
File streams in C++ are essential for handling input and output operations with files. They facilitate reading from and writing to disk files while abstracting the complexity involved in managing file data. There are three primary types of file streams available:
- `ifstream`: This is used for reading files.
- `ofstream`: This is utilized for writing files.
- `fstream`: This allows both reading and writing to files.
These streams provide a convenient interface to manipulate files using the same syntax and methods used for standard input and output.
Setting Up a Simple C++ Project
To get started with file operations in C++, you'll need to set up a basic project structure. Choose an integrated development environment (IDE) like Visual Studio, Code::Blocks, or any C++ compatible text editor. Ensure you have a compiler installed, like GCC or Clang.
In your project directory, keep the following structure:
/ProjectFolder
├── main.cpp
└── example.txt
Make sure to include the necessary headers at the beginning of your code:
#include <iostream>
#include <fstream>
#include <string>
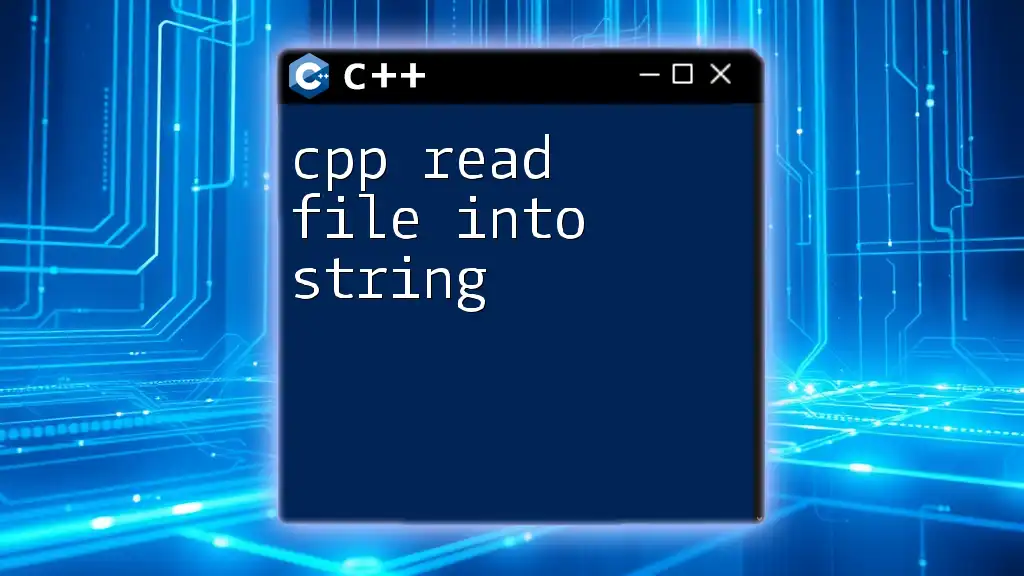
The Basics of Reading a File
Opening a File
To read from a file, you must first open it using `ifstream`. Here's a simple example:
std::ifstream inputFile("example.txt");
Error handling is crucial when opening files. If the file cannot be opened (such as when the file does not exist), you should handle this gracefully:
if (!inputFile) {
std::cerr << "File could not be opened!" << std::endl;
return 1; // Exit the program
}
Reading Data from a File
Using `std::getline()`
One of the most common ways to read data from a file is line-by-line using `std::getline()`. This method is particularly useful for reading text files where data is structured into lines. Here’s how to implement it:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl; // Print each line
}
Reading Word by Word
If you need to process data word by word, you can utilize the `operator>>`. This operator reads until it encounters whitespace, making it a good fit for space-separated data. Here’s an example:
std::string word;
while (inputFile >> word) {
std::cout << word << std::endl; // Print each word
}
Reading Character by Character
For more granular control over the file contents, you can read character by character using the `get()` method. This is often used in scenarios where processing each character is necessary, such as parsing or analyzing specific data formats:
char ch;
while (inputFile.get(ch)) {
std::cout << ch; // Print each character
}
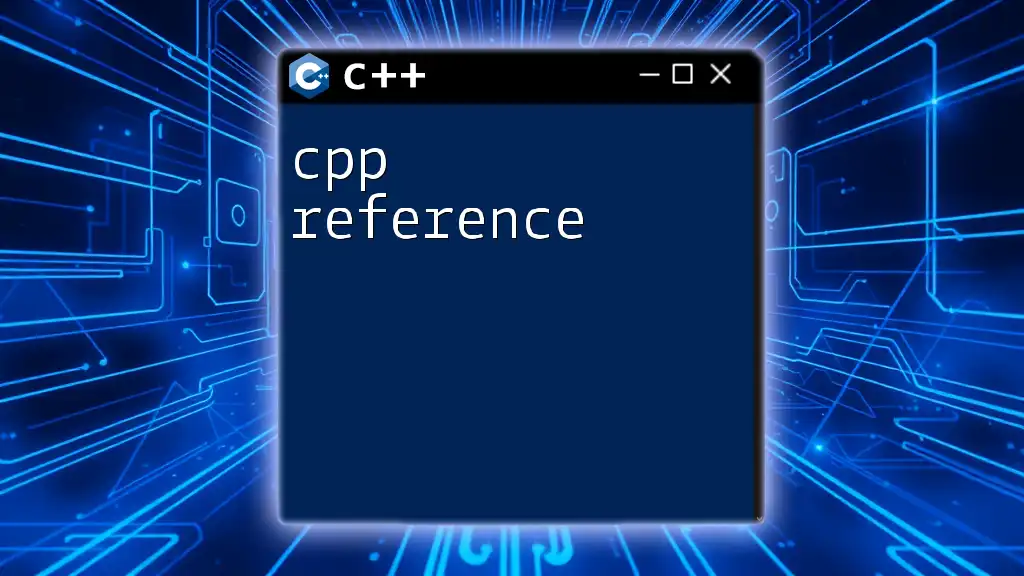
Handling Different File Formats
Reading Text Files
Text files are the most common file format for basic operations. They typically store data as plain text and can be easily handled by the methods mentioned above. Common use cases include configuration files, logs, and plain data storage.
Reading Binary Files
Binary files, on the other hand, store data in a format that is not meant to be human-readable. Reading binary files requires a specific approach. You will open the file using the `ios::binary` flag:
std::ifstream binaryFile("data.bin", std::ios::binary);
This ensures the file is read correctly, especially when dealing with non-textual data like images or executables.
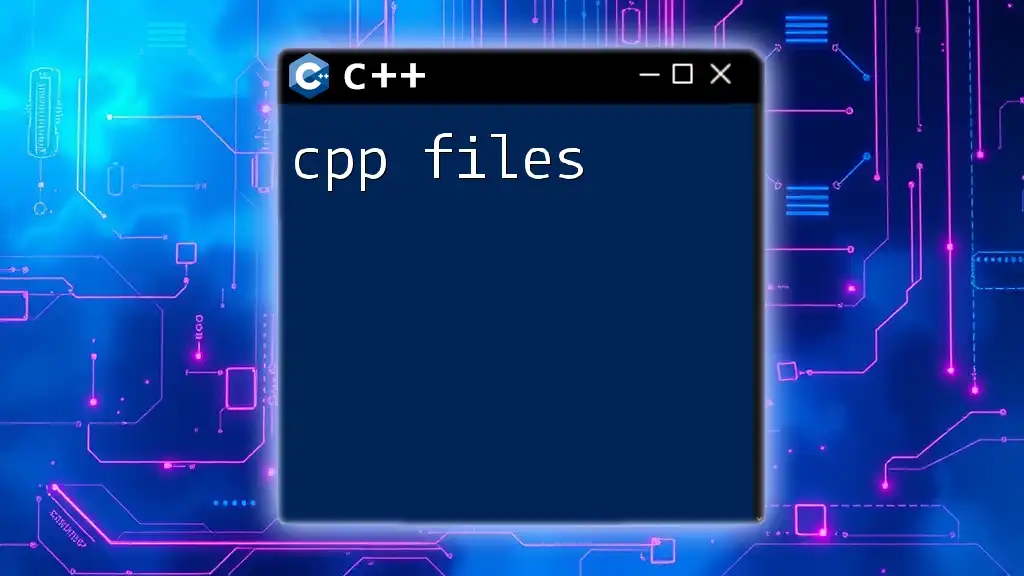
Advanced File Reading Techniques
Using Buffers for Efficient Reading
Using buffers can significantly enhance the efficiency of file reading operations, especially when dealing with large files. A buffer temporarily holds a block of data read from the file, reducing the number of read operations. Here’s how you can implement buffered reading:
char buffer[256];
while (inputFile.read(buffer, sizeof(buffer))) {
// Process buffer, such as printing it or parsing data
}
This method allows you to read chunks of data at a time, optimizing performance.
Error Handling and Validation
When working with file input, error handling is crucial to ensure data integrity and prevent runtime errors. After reading operations, check for conditions that indicate problems:
if (inputFile.bad()) {
std::cerr << "Error reading the file!" << std::endl;
}
Validating the data read from the file can prevent corrupt data from affecting your application. Implement checks after reading to confirm the data meets expected formats.
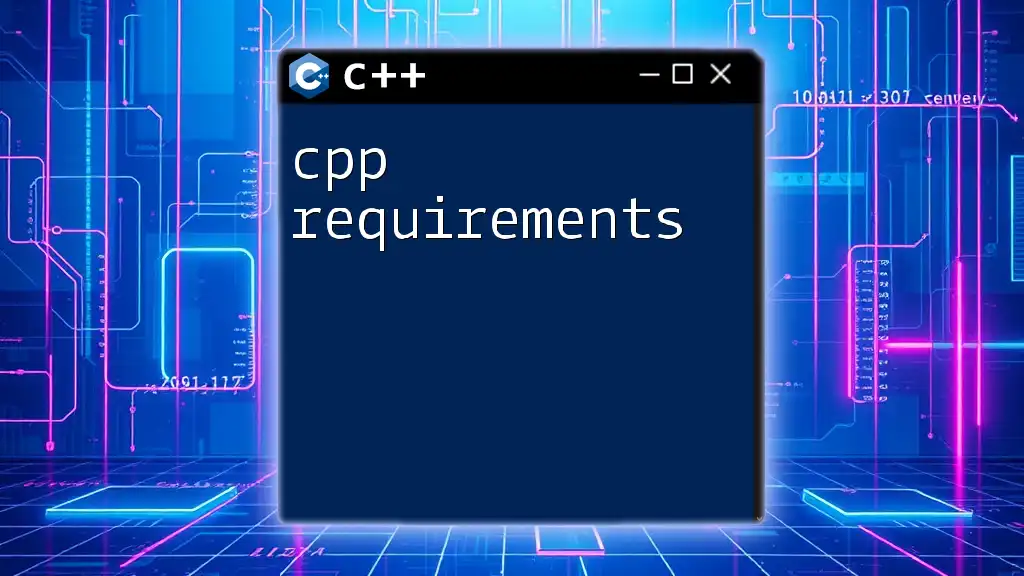
Closing a File
Once you are done reading a file, it is important to close it to free up system resources. This can be done using the `close()` method:
inputFile.close();
Not closing a file can lead to memory leaks or file corruption in some cases, so it should be considered a best practice.
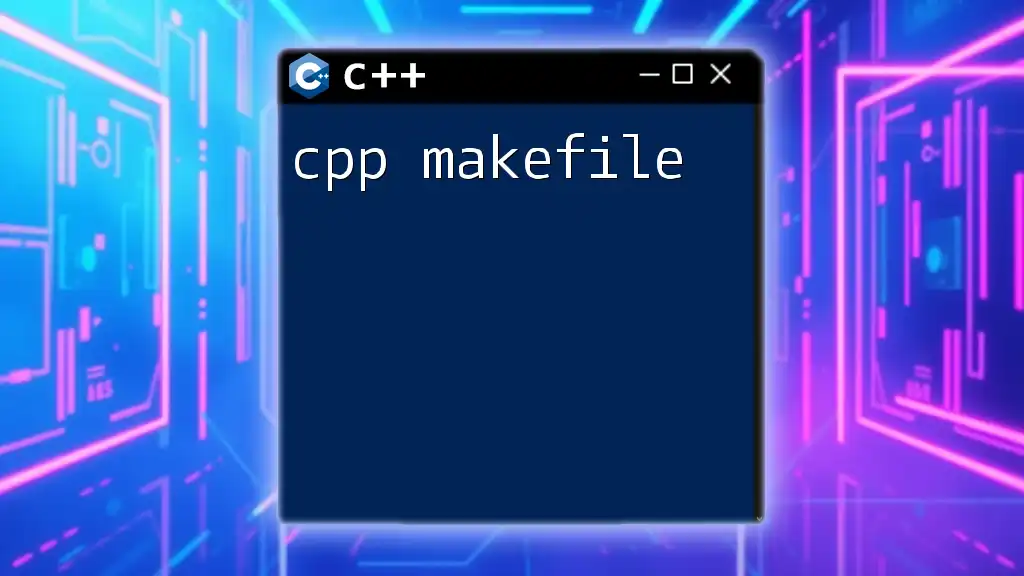
Common Mistakes and Troubleshooting
When working with file operations, several common issues may arise. Here are some frequent mistakes to be aware of:
- Forget to open the file: Always ensure that the file is opened before attempting to read.
- Not checking for errors: Always implement error checking after file operations.
- Ignoring the differences between text and binary modes: Choose the correct mode based on the file type.
- Forgetting to close files: Ensure to close files once operations are complete.
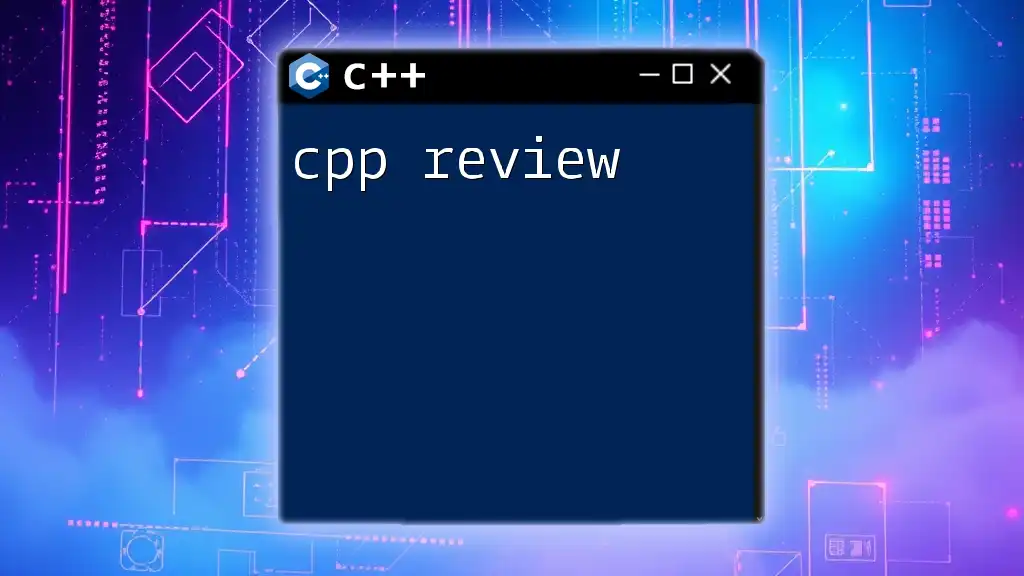
Conclusion
Through this comprehensive guide on C++ readfile operations, you now have a solid understanding of how to read files efficiently in C++. By mastering these techniques, you can enhance your programming skills and better handle file data in your applications. Practice reading various file formats, explore data validation, and always remember the importance of error handling. Happy coding!
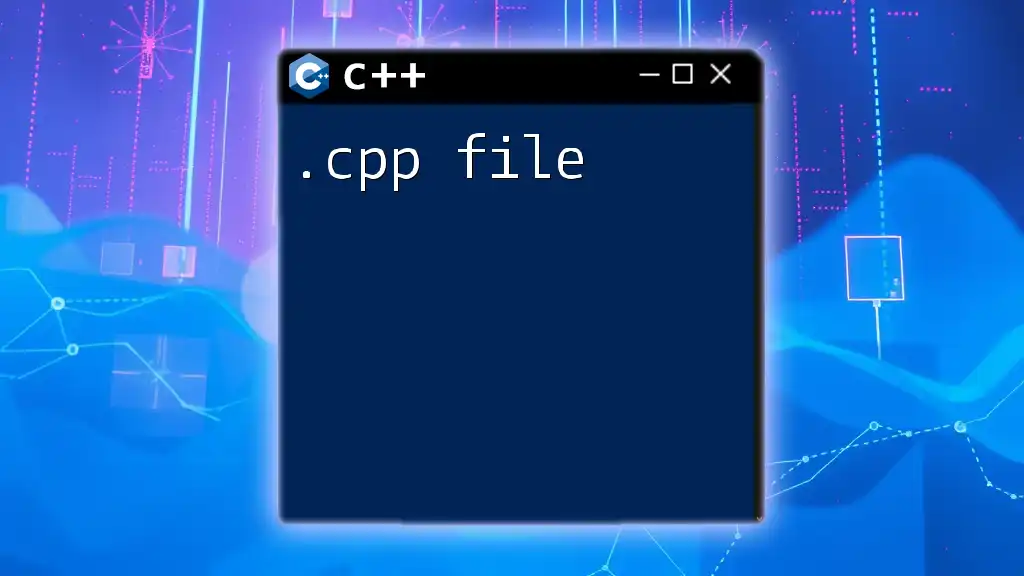
Additional Resources
To deepen your understanding of file operations in C++, consider exploring further through books, online tutorials, and community forums. Engaging with others can help solidify your knowledge and expose you to advanced topics in C++.