To read the contents of a file into a string in C++, you can use the following code snippet:
#include <iostream>
#include <fstream>
#include <sstream>
std::string readFileToString(const std::string& filename) {
std::ifstream file(filename);
std::stringstream buffer;
buffer << file.rdbuf();
return buffer.str();
}
Understanding File I/O in C++
What is File I/O?
File Input/Output (I/O) refers to the process of reading data from and writing data to files. In C++, file I/O is achieved using file stream classes, including `ifstream` for reading from files, `ofstream` for writing to files, and `fstream` for both reading and writing. These classes enable developers to handle files effectively and manipulate their content within a C++ program.
Why Read into String?
Reading file content into a string can be beneficial in various scenarios:
- Ease of Manipulation: Strings are flexible and can easily be modified. You can manipulate the contents in a variety of ways, such as searching, concatenating, or formatting the data.
- Better Readability: When you store file content in a string, it's easier to work with and reference, making your code cleaner and more readable.
- Data Storage: Using strings allows you to quickly store and retrieve text data without the need to manage raw byte arrays or additional buffers.
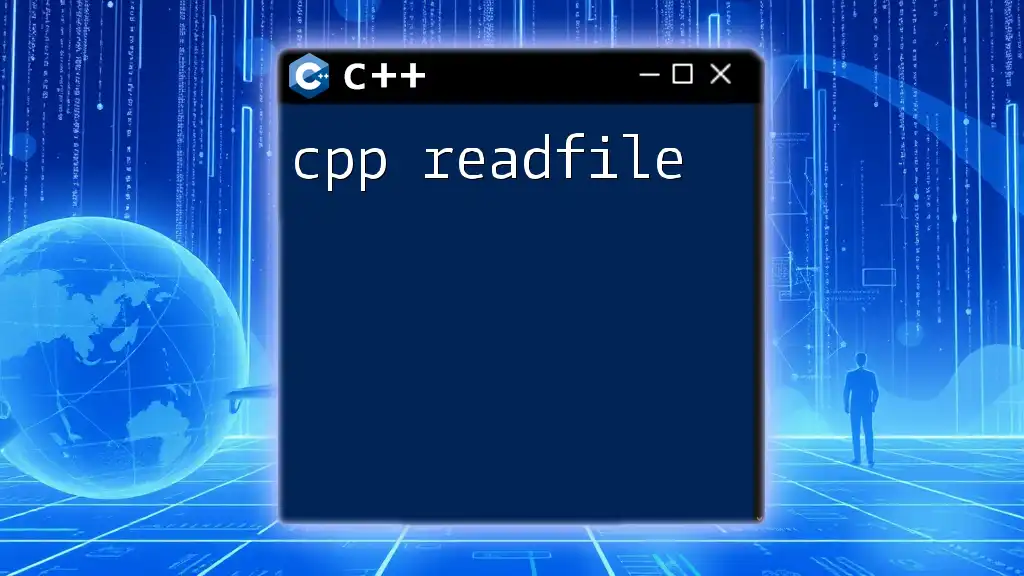
Setting Up the Environment
Required Libraries
Before you start coding, you need to include the necessary library headers. For reading files, you typically require:
#include <iostream>
#include <fstream>
#include <string>
These headers provide the functionality needed for file handling, console I/O, and string management.
Sample File Creation
To demonstrate the process of reading a file into a string, you'll need a sample text file. Create a file named `example.txt` in the same directory as your code, and add the following content:
Hello, World!
Welcome to C++ file handling.
This is a test file for reading into a string.
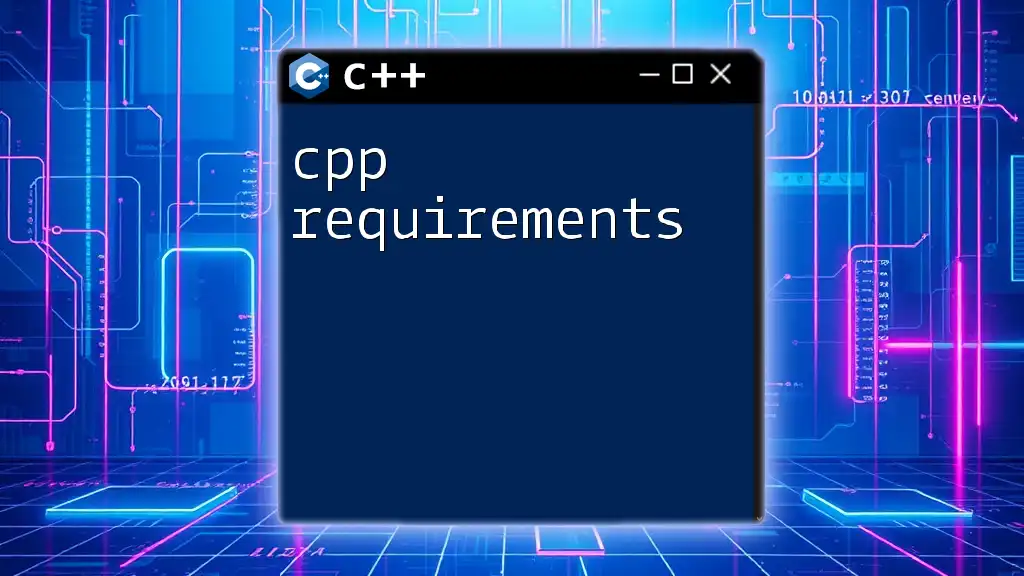
Reading File into String in C++
Basic Method Using ifstream
One of the most straightforward ways to read a file into a string in C++ is using the `ifstream` class. Below is a simple implementation.
Code Example
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string fileContent;
if (file) {
fileContent.assign((std::istreambuf_iterator<char>(file)),
std::istreambuf_iterator<char>());
std::cout << fileContent;
} else {
std::cerr << "Error opening file.";
}
return 0;
}
Explanation
In this example:
- We first create an `ifstream` object named `file` and open `example.txt`.
- We check if the file is successfully opened using the `if (file)` condition.
- The expression `std::istreambuf_iterator<char>(file)` creates an iterator that reads characters from the file until the end.
- `fileContent.assign()` collects the entire content into a string.
- Finally, we print the string to the console. If the file cannot be opened, an error message is displayed.
Alternative Method Using std::getline
Another way to read a file into a string is to use the `std::getline()` function. This method reads the file line by line, which can be more manageable depending on your needs.
Code Example
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
std::string fileContent;
while (std::getline(file, line)) {
fileContent += line + "\n";
}
std::cout << fileContent;
return 0;
}
Explanation
In this version:
- A loop is used to read the file line by line with `std::getline()`.
- Each line is appended to `fileContent`, followed by a newline character to preserve the original formatting.
- This method is particularly useful when processing large files or when you need to manipulate each line separately.
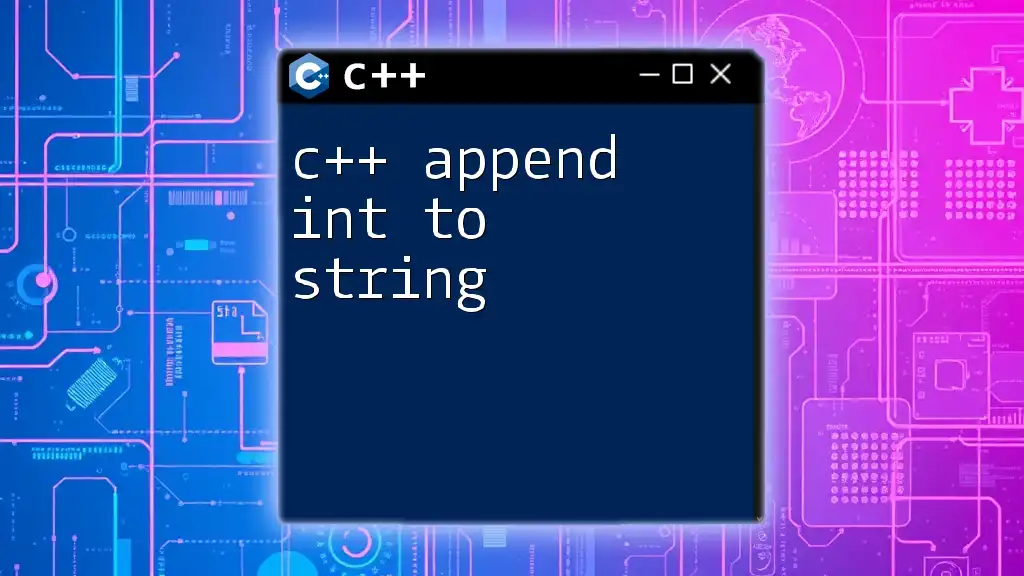
Handling Errors While Reading Files
Common Errors Encountered
When dealing with file I/O, various errors can arise, such as:
- File Not Found: The specified file path does not exist.
- Permission Issues: The program may lack the necessary permissions to read the file.
- End-of-File Handling: If not managed properly, unexpected EOFs can lead to incomplete reads.
Error Checking Techniques
To ensure robust code, it is crucial to handle potential errors effectively. The following code snippet illustrates a basic error-checking mechanism:
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cerr << "Error: Unable to open file.\n";
return 1;
}
In this example, before proceeding with reading the file, we check if it has been successfully opened. If not, an error message is displayed, and the program exits gracefully.
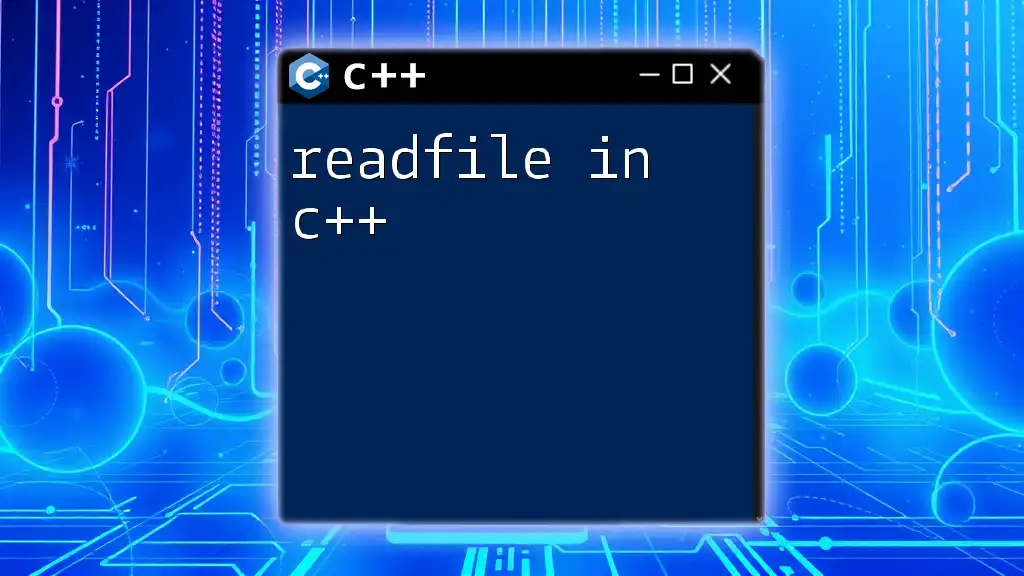
Special Considerations when Reading Files
Large Files
When dealing with large files, performance becomes a critical aspect of reading operations. Here are some tips:
- Buffering: Consider reading the file in chunks instead of loading the entire content at once.
- Memory Management: Be aware of memory usage, as large strings can consume a significant amount of memory.
Different File Formats
Reading non-text files (binary files) can be handled similarly but requires a few adjustments. When processing binary data, you may opt for different types of streams and ensure data is handled properly, often using `fstream` instead of `ifstream`.
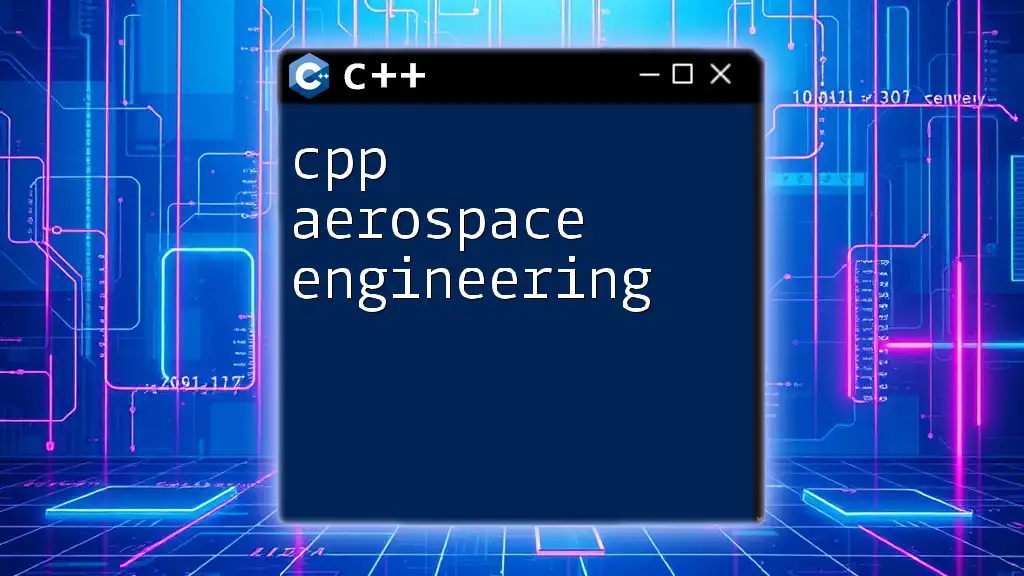
Conclusion
In summary, learning how to cpp read file into string can greatly enhance your C++ programming skills. Understanding various methods of reading files, handling different scenarios, and managing errors are crucial components to mastering file I/O in C++. We encourage you to explore these techniques and experiment with your own implementations.
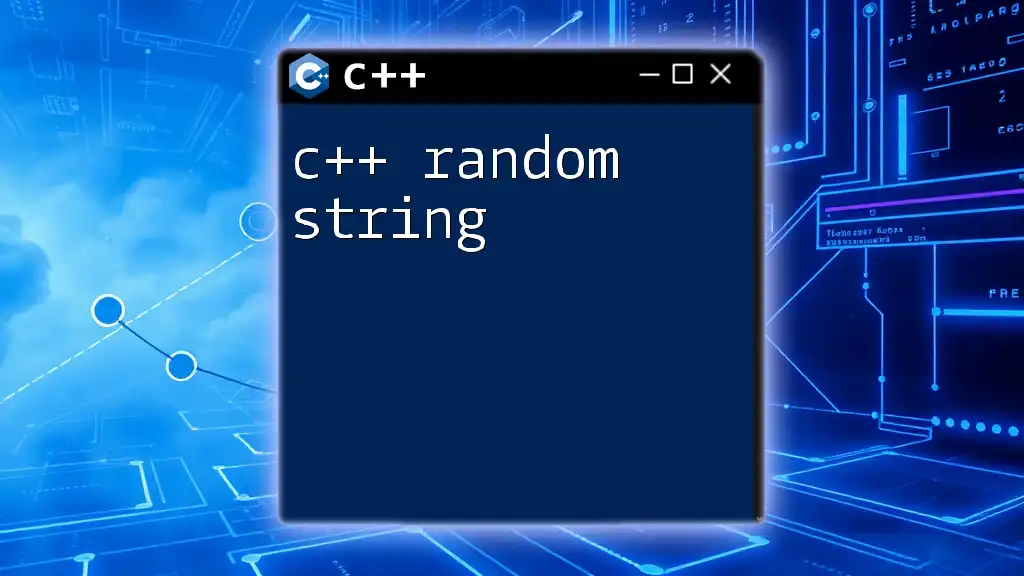
Resources and Further Reading
For those wanting to dive deeper, here are additional resources:
- [C++ Documentation: File Streams](https://en.cppreference.com/w/cpp/io/basic_filebuf)
- [Learn C++](https://www.learncpp.com/) for a broader understanding of C++ file handling and programming concepts.
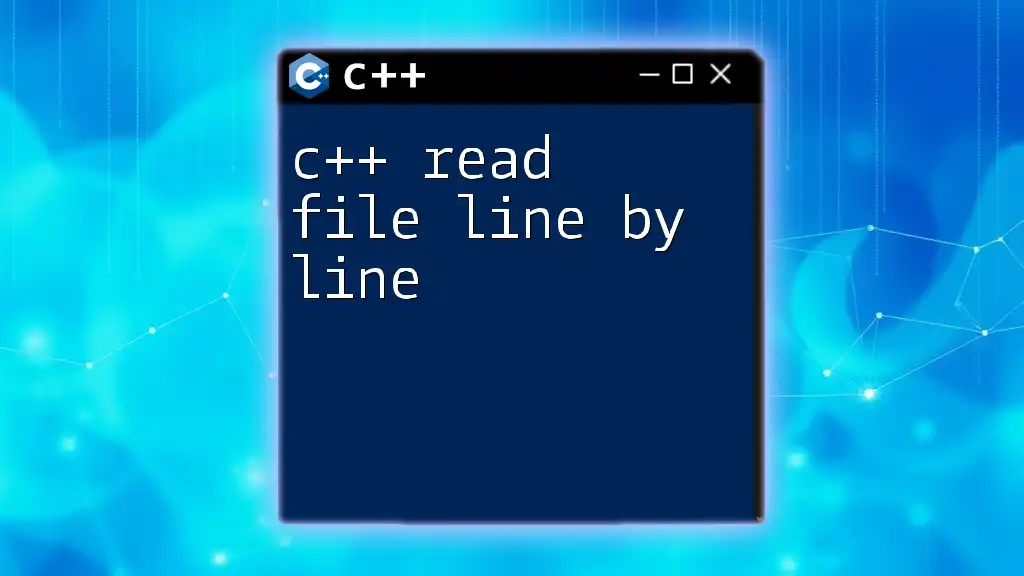
FAQs
What if my file contains special characters?
If your file includes special characters or is encoded in a specific format, make sure to handle character encoding properly. You can use libraries such as `<codecvt>` for advanced character conversions.
Can I read multiple files into a single string?
Yes, you can concatenate the contents of multiple files by opening each file consecutively and appending their content to a single string.
Does reading a file into a string use a lot of memory?
Reading large files into a string can consume a significant amount of memory. It is recommended to process large files in manageable chunks or use more efficient data structures based on your requirements.