In C++, you can read a file line by line using an `ifstream` object and a `getline` function within a loop to process each line individually. Here's a code snippet demonstrating this:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
Understanding File I/O in C++
File Input/Output (I/O) is a crucial aspect of programming in C++. It allows programs to interact with external data stored in files. File I/O can be categorized into two main types: text files and binary files.
Text files contain human-readable content, while binary files store data in a format that is not directly readable by humans. In this article, we’ll focus predominantly on reading text files since they are commonly used for configuration settings, logs, and data exchanges.
C++ provides a robust mechanism for file operations through its Standard Library. The primary libraries for handling file I/O are `<fstream>`, `<iostream>`, and `<string>`. These libraries provide classes that simplify the process of reading from and writing to files.
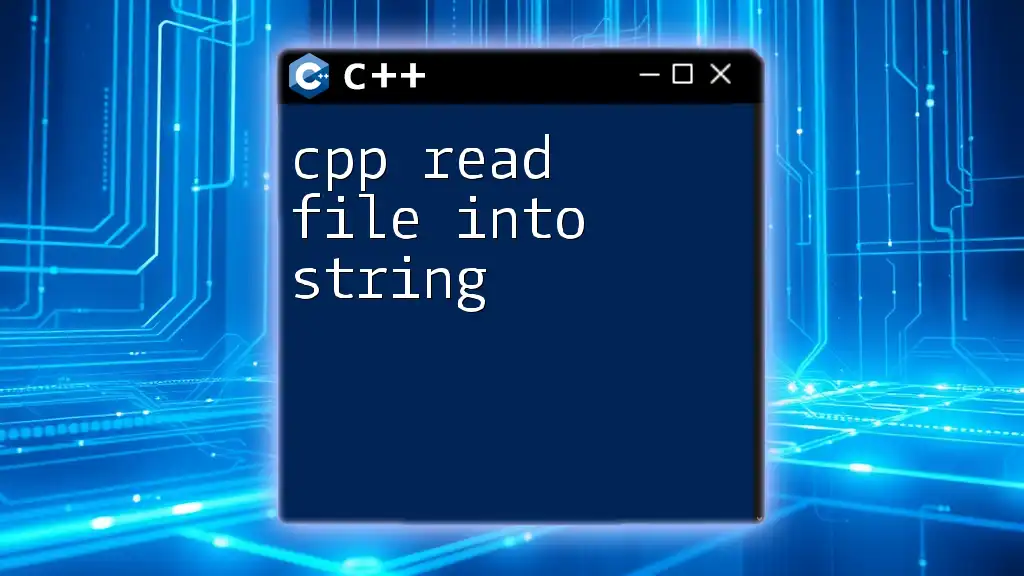
Opening a File in C++
To read a file line by line in C++, you first need to open it. This is done using file stream classes, specifically `ifstream` (input file stream) for reading files. Here's how you can open a file:
#include <fstream>
#include <iostream>
int main() {
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cerr << "Error opening file." << std::endl;
return 1;
}
// File operations
file.close();
return 0;
}
In the code above, we attempt to open `example.txt`. The `is_open()` method is used to check if the file was successfully opened. If not, it outputs an error message.
Checking if the File is Open
It's critical to ensure that the file has opened correctly before proceeding with any reading operations. This step avoids runtime errors that may arise from trying to read a non-existent or inaccessible file. Always include error handling, as shown in the example above, to make your application more robust.
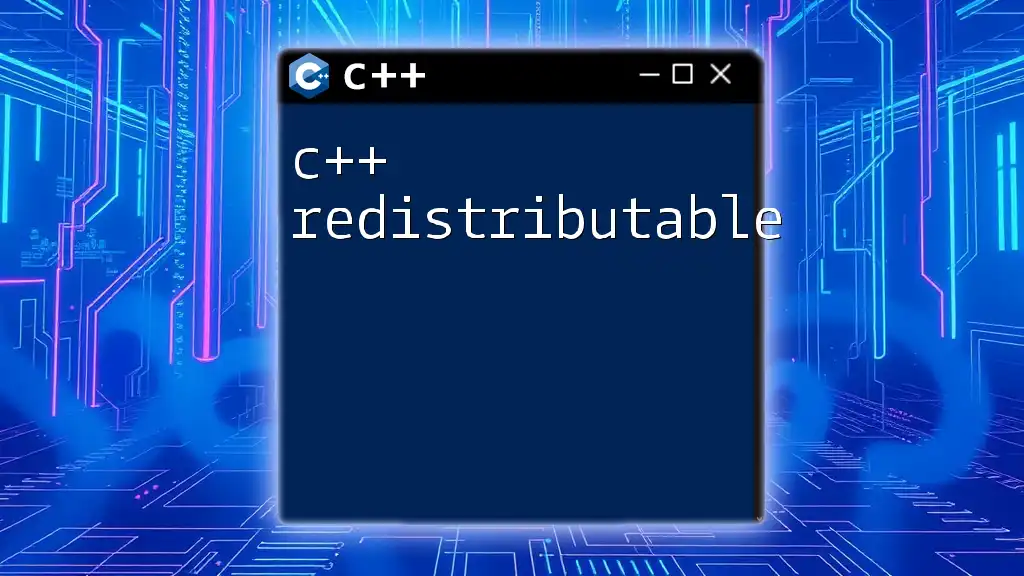
Reading from a File Line by Line
Once the file is open, you can read its contents line by line using the `std::getline()` function. This function reads characters from the input stream and stores them into a string variable until it encounters a newline character.
Here’s an example of using `std::getline()` to read a file:
std::string line;
std::ifstream file("example.txt");
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
In this snippet, each line from `example.txt` is read and printed to standard output. The loop continues until the end of the file (EOF) is reached.
Why Read Line by Line?
Reading files line by line helps manage memory more effectively, especially when working with large files. Instead of loading an entire file into memory, line-by-line reading allows you to process one line at a time. This approach is particularly useful for applications like log file processing, data analysis, and reading configuration files.
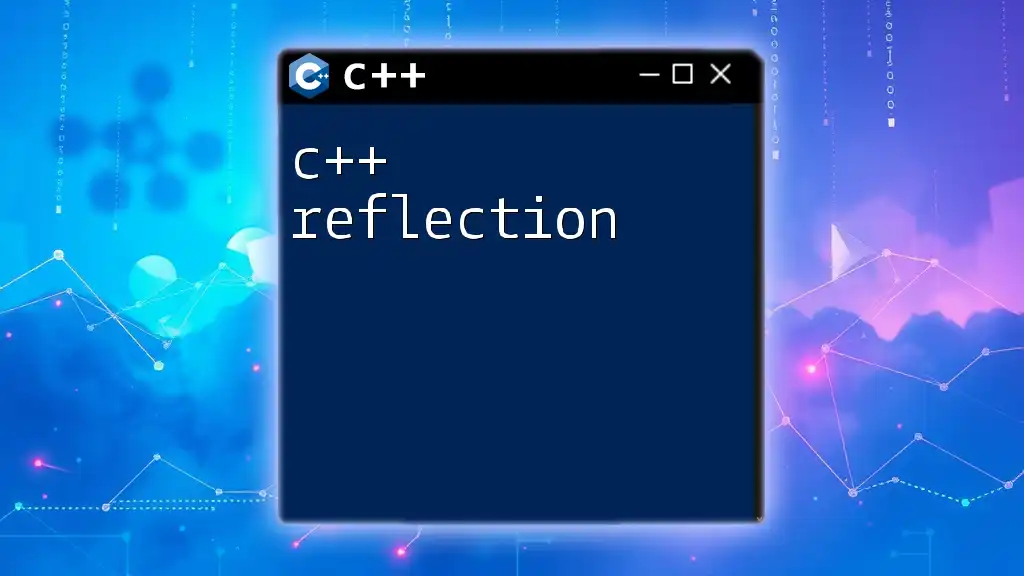
Error Handling While Reading Files
When dealing with file input, several potential errors can arise, such as reaching the end of the file or attempting to read corrupted data.
Common Errors when Reading Files
It’s essential to handle cases like:
- End of file: When you reach the last line, `std::getline()` will return false.
- Empty lines: Sometimes you might encounter lines that do not contain any data.
Here is an improved implementation that checks for these errors:
#include <fstream>
#include <iostream>
int main() {
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cerr << "Error: Could not open the file." << std::endl;
return 1;
}
std::string line;
while (std::getline(file, line)) {
if (line.empty()) {
std::cout << "Empty line encountered." << std::endl;
continue;
}
std::cout << line << std::endl;
}
file.close();
return 0;
}
In this code, we check if the line read is empty and print a message if it is. This way, we can skip any unwanted empty lines without halting the process.
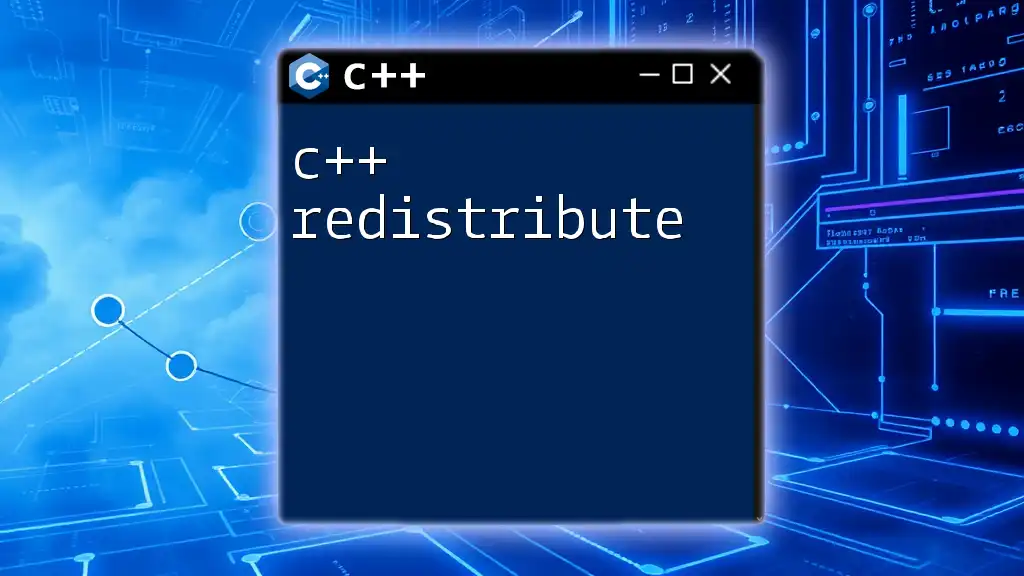
Advanced Techniques
Reading Specific Lines
You may sometimes want to read only a specific number of lines from a file. Here’s an example of reading the first five lines:
#include <fstream>
#include <iostream>
int main() {
std::ifstream file("example.txt");
std::string line;
int line_count = 0;
while (std::getline(file, line) && line_count < 5) {
std::cout << line << std::endl;
line_count++;
}
file.close();
return 0;
}
In this snippet, the loop is set to iterate until it has read five lines or the end of the file is reached, enabling more precise control over the reading process.
Storing Lines in a Container
If you want to store the lines you've read for further processing, you can use a `std::vector` to hold the lines. This technique is useful when you want to manipulate or analyze the lines later on.
Here’s how you can do this:
#include <fstream>
#include <iostream>
#include <vector>
#include <string>
int main() {
std::ifstream file("example.txt");
std::vector<std::string> lines;
std::string line;
while (std::getline(file, line)) {
lines.push_back(line);
}
// Displaying stored lines
for (const auto& l : lines) {
std::cout << l << std::endl;
}
file.close();
return 0;
}
In this example, we push each line into a vector named `lines`. After all lines are read, we iterate over the vector to display them. This approach allows you to perform additional operations on the lines after reading them.
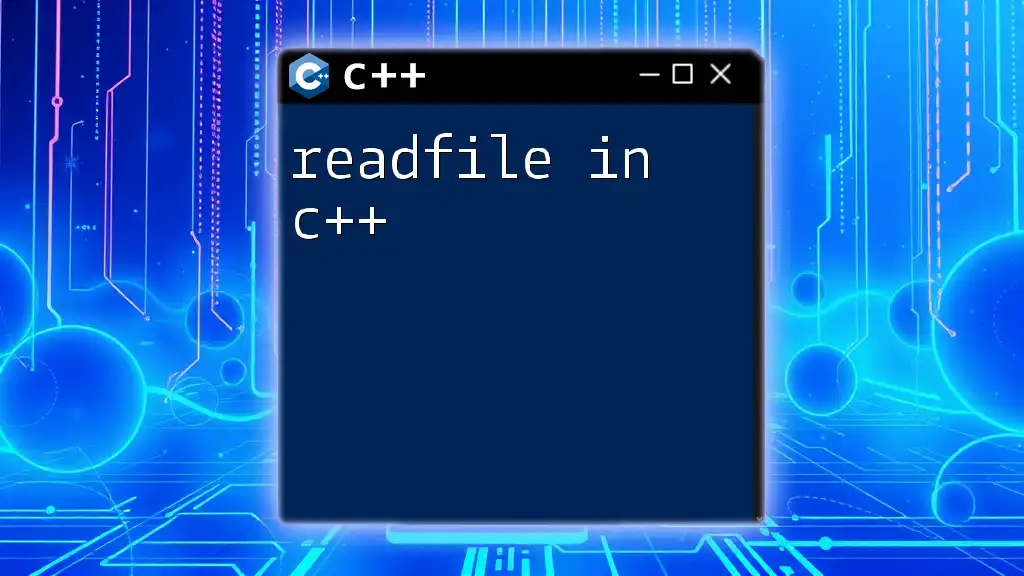
Conclusion
In this article, we’ve explored how to c++ read file line by line effectively. We discussed the importance of file opening and error handling, along with techniques for reading files while managing memory usage efficiently.
Understanding file I/O in C++ is not just about reading or writing; it's about ensuring that applications can handle data while maintaining reliability and performance. By implementing these strategies, you can better manage file data in your C++ applications.
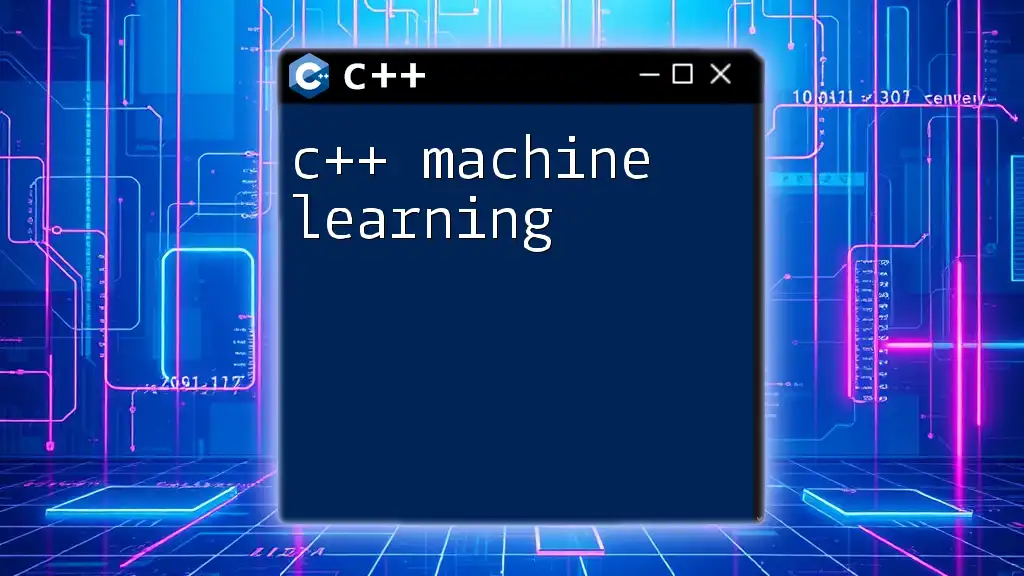
Additional Resources
For those looking to deepen their understanding of file I/O in C++, consider exploring the official C++ documentation. Additionally, numerous books and online tutorials can provide further insights into advanced techniques and practices.
Finally, don’t hesitate to engage with interactive challenges to solidify your knowledge and skills in reading files line by line in C++.