In C++, you can read input from the standard input (stdin) using the `std::cin` stream, which allows users to enter data that your program can process.
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter some text: ";
std::getline(std::cin, input);
std::cout << "You entered: " << input << std::endl;
return 0;
}
Understanding stdin
What is stdin?
Standard Input (stdin) is a predefined stream that allows input from the user or another program. In C++, stdin is typically associated with the keyboard, and it plays a central role when you want to gather data during the execution of a program.
Understanding stdin is crucial as it enables developers to create interactive applications that can respond to user inputs in real-time. It also paves the way for more complex input mechanisms like reading from files or network sockets.
Why Use stdin?
There are several scenarios where reading from stdin is preferred:
- Interactive User Input: In console applications, real-time user inputs are common. For example, asking users to enter their names or preferences.
- Efficient Testing: During debugging, you can provide input directly from the console rather than modifying files.
- Dynamic Input: Responding to user input can change the flow of a program, allowing for more dynamic execution.
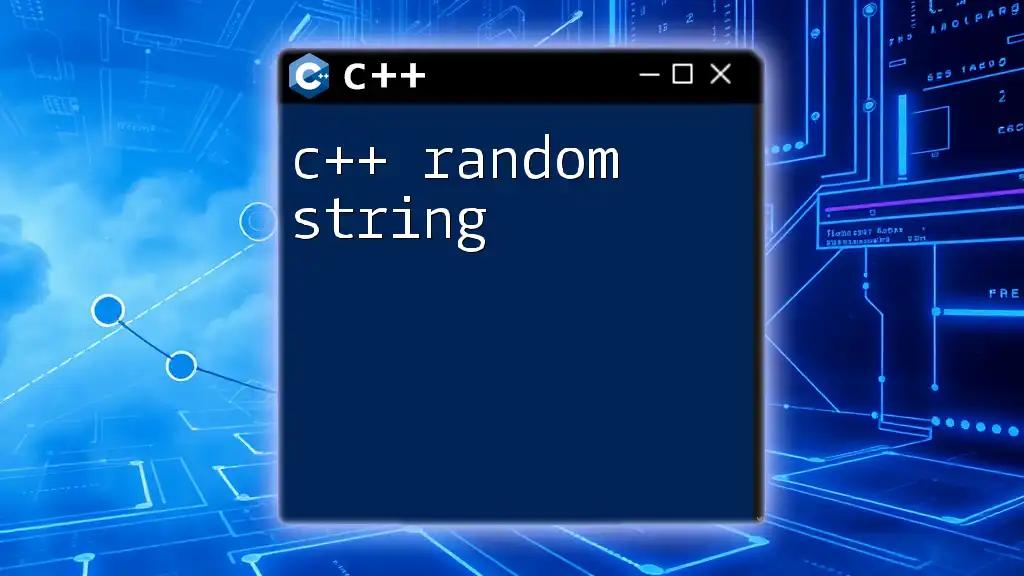
Setting Up Your Environment
Required Tools and Compilers
To effectively work with C++ and stdin, you need a C++ compiler. Some of the most popular choices include:
- g++ (GNU Compiler Collection) for Linux and Windows.
- Visual Studio for Windows developers.
- Xcode for macOS users.
Ensure you have your development environment set up correctly. Proper installation of these tools allows you to run and test your programs effectively.
Writing Your First C++ Program
The basic structure of a C++ program includes:
- Header files: Necessary libraries that your program will use.
- A main function: The entry point of any C++ program.
- Syntax statements: To execute your desired operations.
Here's a simple example, the famous "Hello World" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
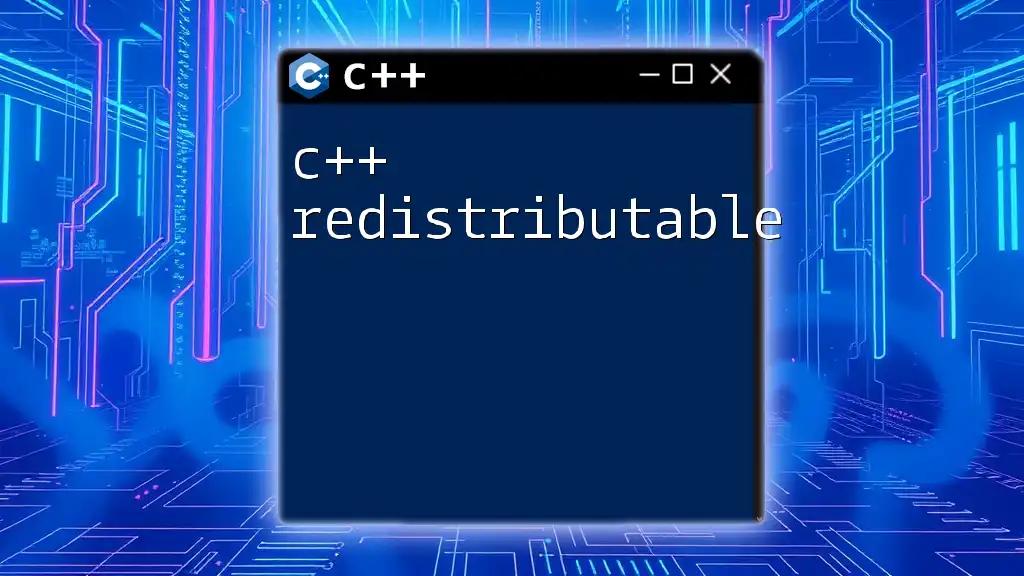
Reading from stdin in C++
Using `cin` for Input
The `cin` object in C++ is designed for reading formatted input. It is a part of the `<iostream>` library and serves as the standard method for input operations.
The basic syntax for using `cin` is straightforward. You can read various data types with a simple syntax:
cin >> variable;
Example: Basic Input
Let’s look at a practical example where we ask a user to enter a number:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
In this example, when the user enters a value, `cin` captures it and stores it in the variable `number`. The program then confirms the input by displaying it back.
Reading Multiple Inputs
You can read multiple variables at once using the `cin` object as well. This can significantly simplify the code when you have several inputs.
Example: Multiple Variables
#include <iostream>
using namespace std;
int main() {
int num1, num2;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
cout << "You entered: " << num1 << " and " << num2 << endl;
return 0;
}
Here, we prompt the user to enter two integers. Both inputs are taken in a single line, efficiently capturing user input in a neat way.
Input Types
Reading Strings
When it comes to reading text inputs, `cin` can read strings. However, keep in mind that it stops reading at whitespace. For example, if a user inputs "John Doe", only "John" is captured.
Example: Reading a String
#include <iostream>
#include <string>
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
cin >> name;
cout << "Hello, " << name << "!" << endl;
return 0;
}
In this case, only the first word is captured. If you want to read full names or sentences, you'd need a different approach.
Reading Entire Lines
For capturing entire lines of input, including spaces, use the `getline()` function. This method is more versatile for string input.
Example: Reading a Full Line
#include <iostream>
#include <string>
using namespace std;
int main() {
string line;
cout << "Enter a line of text: ";
getline(cin, line);
cout << "You wrote: " << line << endl;
return 0;
}
This example will read everything until the newline character, allowing for full sentences or lines of text. It effectively captures all user input without cutting off at whitespace.
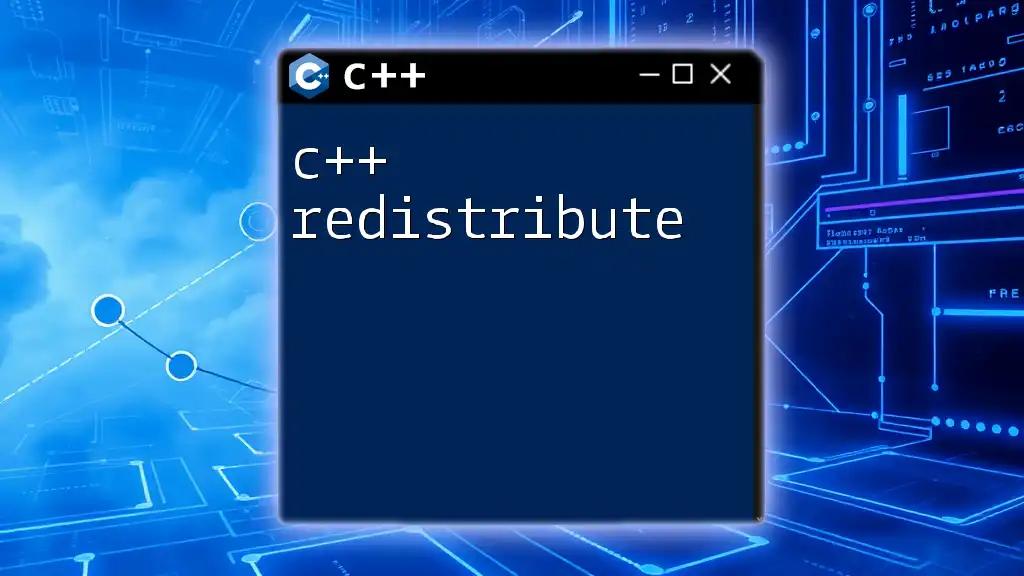
Error Handling During Input
Input Validation Techniques
When working with user input, it's essential to validate that the input is what you're expecting. Ensuring robust input validation will prevent your program from crashing due to unexpected data types or formats.
Example: Input Validation
Here's a simple demonstration of input validation, where we continuously prompt the user until a valid integer value is provided:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
while (!(cin >> number)) {
cout << "Invalid input. Please enter an integer: ";
cin.clear(); // Clear the error flag
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Discard invalid input
}
cout << "You entered: " << number << endl;
return 0;
}
In this code, if the user enters an invalid input (like a letter), the program prompts them until they provide a valid integer, demonstrating a good practice of handling input errors gracefully.

Advanced Techniques
Using `std::cin` with Custom Types
You can also read user-defined types from stdin. This usually involves creating a custom class or struct that requires a tailored input approach, often using operator overloading.
Example: Custom Input
#include <iostream>
using namespace std;
struct Point {
int x;
int y;
};
istream& operator>>(istream& is, Point& p) {
is >> p.x >> p.y;
return is;
}
int main() {
Point p;
cout << "Enter coordinates (x y): ";
cin >> p;
cout << "Point coordinates: (" << p.x << ", " << p.y << ")" << endl;
return 0;
}
In this example, we define a `Point` structure and overload the `>>` operator to handle input directly into the structure, allowing users to input x and y coordinates seamlessly.
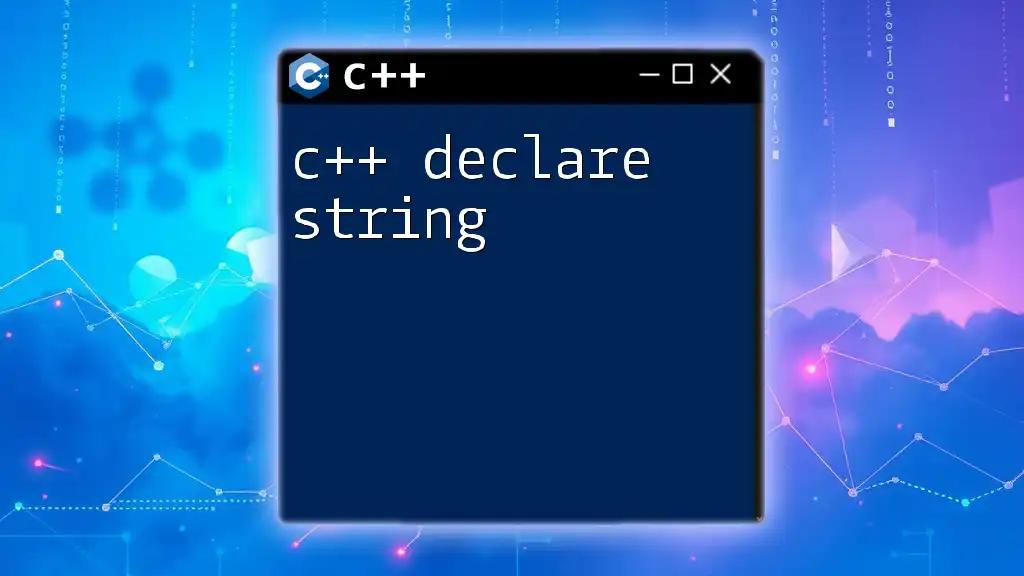
Common Pitfalls and Best Practices
Common Issues When Reading from stdin
One common issue developers face with `cin` is letting whitespace cause complications. For instance, reading a string with spaces or unexpected characters can lead to incomplete data capturing.
Another problem arises with type mismatches when the expected data type does not match the input, causing the program to enter an error state.
Best Practices to Remember
- Always validate your input to ensure that it matches expected formats and types.
- Use `getline()` for strings that might contain whitespace or special characters.
- Implement robust error handling to manage invalid inputs gracefully, keeping your program running smoothly.
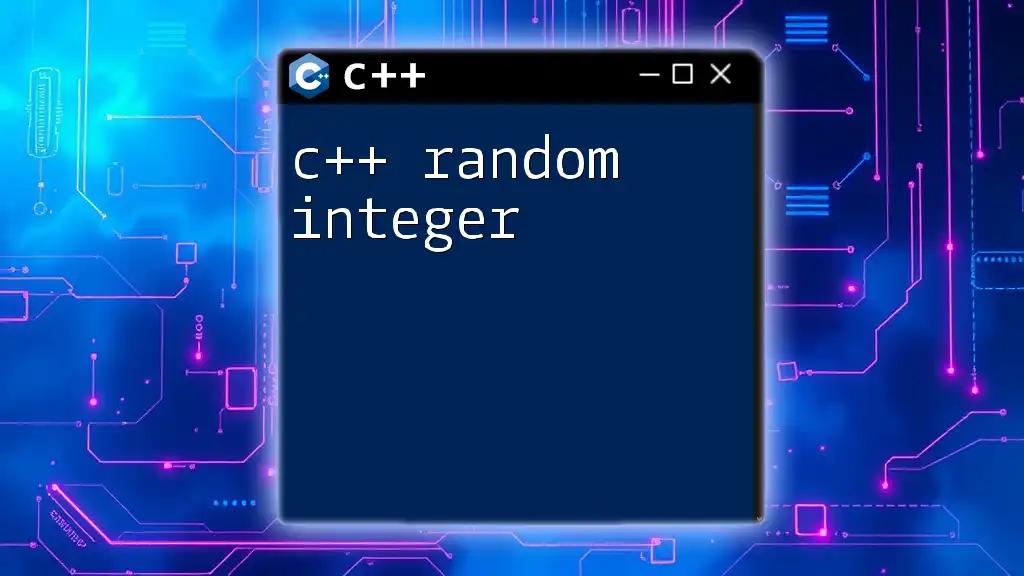
Conclusion
Working with stdin in C++ opens up a world of interactive programming. Understanding how to read from stdin effectively not only enhances user experience but also lays the foundation for robust application design. By practicing the techniques outlined in this guide, you can develop your skills in capturing and managing input in C++, whether you are creating simple applications or sophisticated systems.
Remember to consistently validate user input and optimize your code for efficiency and clarity. With these tools at your disposal, the endless possibilities of data interaction await!