In C++, you can access elements from a tuple using the `std::get` function, specifying the index of the desired element.
#include <tuple>
#include <iostream>
int main() {
std::tuple<int, char, double> myTuple(1, 'a', 3.14);
std::cout << std::get<1>(myTuple); // Accessing the second element: 'a'
return 0;
}
Understanding Tuples in C++
What is a Tuple?
A tuple is a fixed-size collection of elements, each of which can be of a different type. This makes tuples a powerful tool in C++, allowing for the grouping of heterogeneous data into a single unit. Unlike arrays or vectors, which require elements to be of the same type, tuples provide a way to store varied types while maintaining type safety.
Declaring Tuples
To declare a tuple, you use the `std::tuple` class included in the `<tuple>` header. Here’s how you declare a tuple that contains multiple types:
#include <tuple>
std::tuple<int, float, std::string> myTuple(1, 3.14, "example");
In this example, `myTuple` stores an integer, a float, and a string. This structure is compact and helps in managing related data efficiently.
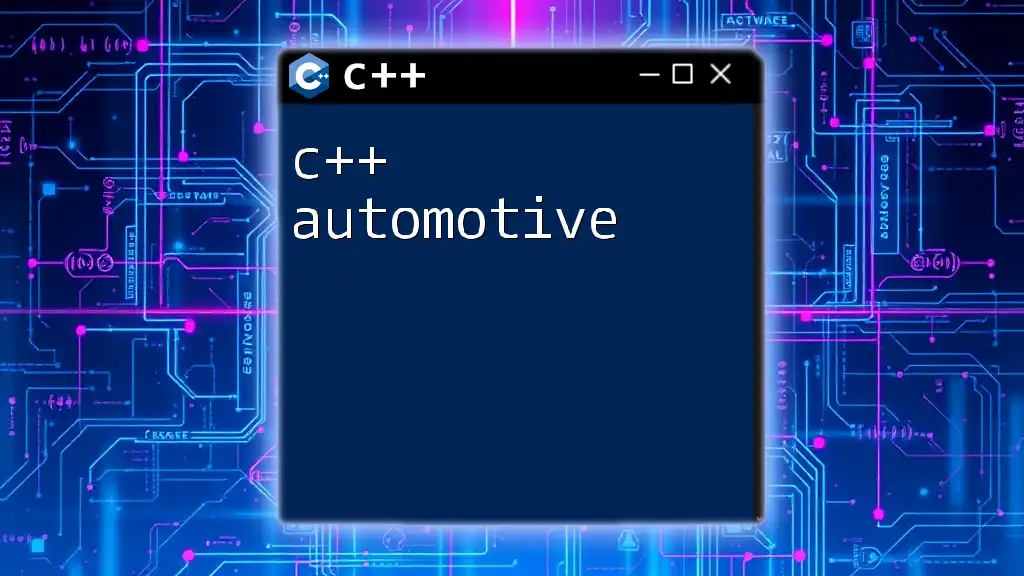
Getting Values from Tuples
Using std::get
To extract values from a tuple, you utilize the `std::get` function provided by the C++ Standard Library. This function allows you to retrieve the value of a specific position within a tuple based on its index.
int value = std::get<0>(myTuple);
This code snippet retrieves the first element from `myTuple`, which in this case is `1`.
Getting the First Element
When you specifically want the first element of the tuple, you can directly use the following syntax:
int firstValue = std::get<0>(myTuple);
// Output: 1
This is straightforward and efficient, allowing you to access the first value exactly as required. The key here is understanding that tuple indices start from zero.
Accessing Elements by Index
You can also access other elements in the tuple via their respective indices with `std::get`. For example, if you want to retrieve the second element (the floating-point number), use:
float secondValue = std::get<1>(myTuple);
// Output: 3.14
Pros: Using index-based access is clear and concise, making it easy to extract specific elements without confusion.
Cons: However, you must remember the indices, which can sometimes lead to errors if miscounted.
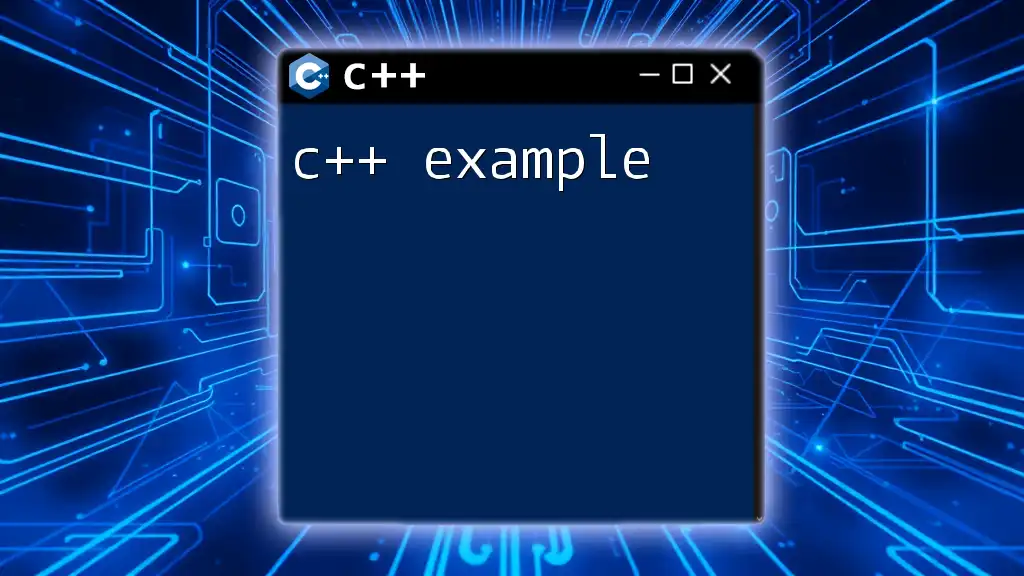
Alternative Ways to Get Tuple Elements
Using std::tie
An alternative method to get multiple values from a tuple is through `std::tie`. This method allows you to unpack values into individual variables while ignoring any elements you don't need.
int intVal;
float floatVal;
std::tie(intVal, floatVal, std::ignore) = myTuple;
Here, `std::ignore` is used for the string element. This way, you can directly get values efficiently without explicitly fetching each by index.
Pros: This method improves code readability by reducing the need for multiple `std::get` calls.
Cons: It can be less efficient in scenarios where you don’t need to unpack all values.
Structured Binding (C++17 and Later)
Structured binding is a feature introduced in C++17 that simplifies tuple value extraction significantly. By declaring multiple variables in a single statement, you can intuitively unpack all elements from a tuple.
auto [intVal, floatVal, strVal] = myTuple;
This reads like a natural language statement and enhances code clarity. You do not need to specify types or indices, making it less error-prone and much easier to maintain.
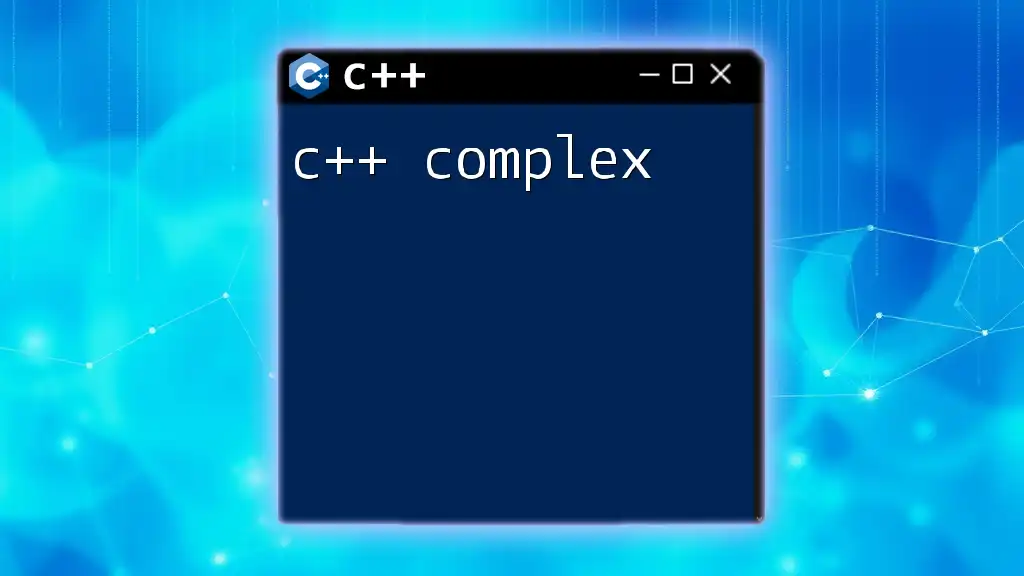
Practical Applications of Getting Values from Tuples
Use Case Scenarios
Tuples are particularly useful in scenarios where you want to return multiple values from a function. For instance, a function that calculates both the sum and the average can naturally return a tuple containing both results:
std::tuple<int, float> calculateSumAndAverage(int a, int b) {
return std::make_tuple(a + b, (a + b) / 2.0);
}
Performance Considerations
When working with tuples, it's important to understand memory usage. Tuples can store a variety of types without the need for complex data structures. The access via `std::get` is often optimized, making it a performant option compared to more dynamic structures like vectors.
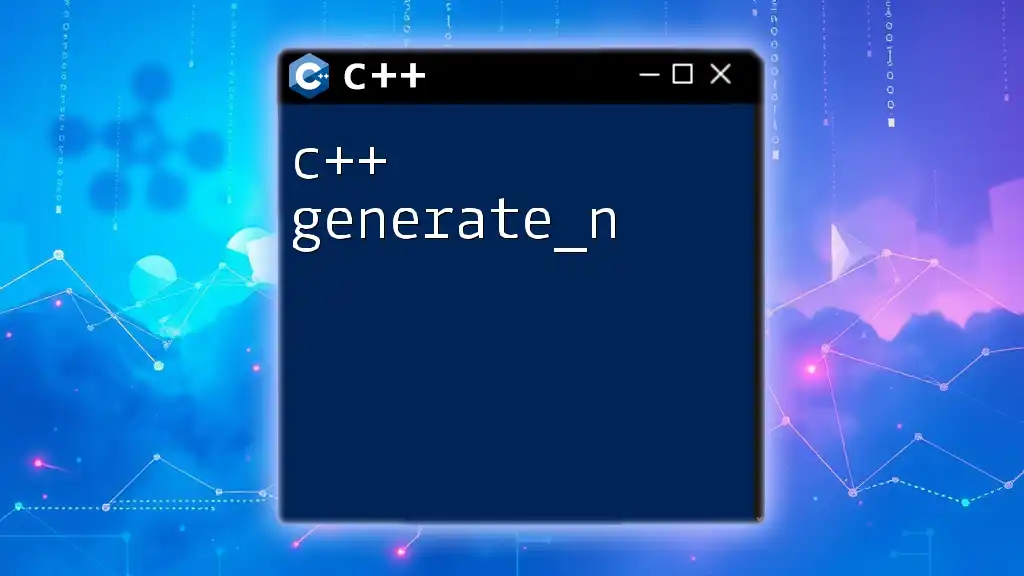
Best Practices for Working with Tuples
When to Use Tuples
Tuples should be used when you need to return multiple values of different types or when you want to group together heterogeneous data into a single entity. They are preferable over structures when you have a small set of associated values that don’t require the overhead of a more complex structure.
Common Pitfalls
One common mistake is using incorrect indices, which can lead to runtime errors or unexpected behavior. Always ensure the index used in `std::get` is in range relative to the number of elements in the tuple.
Another pitfall is assuming the values of a tuple will maintain their original types when extracted. Familiarize yourself with type inference, especially when using functions like `std::tie` or structured binding.
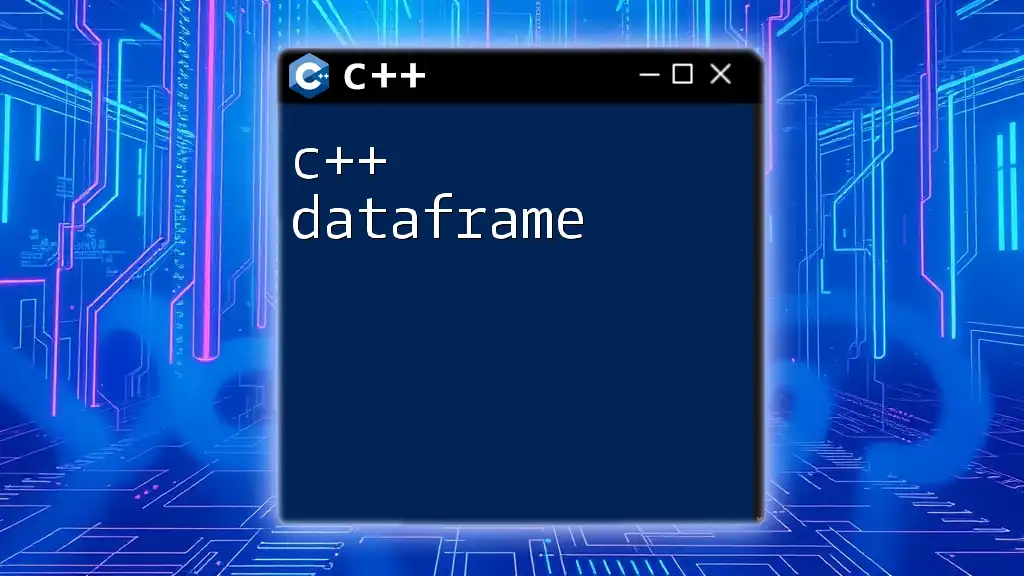
Conclusion
In conclusion, knowing how to c++ get from tuple using `std::get`, `std::tie`, and structured bindings allows for great flexibility in managing multiple return values. These methods enhance both code efficiency and readability. As you continue your journey through C++, mastering tuple management will open new avenues for structuring your data effectively.
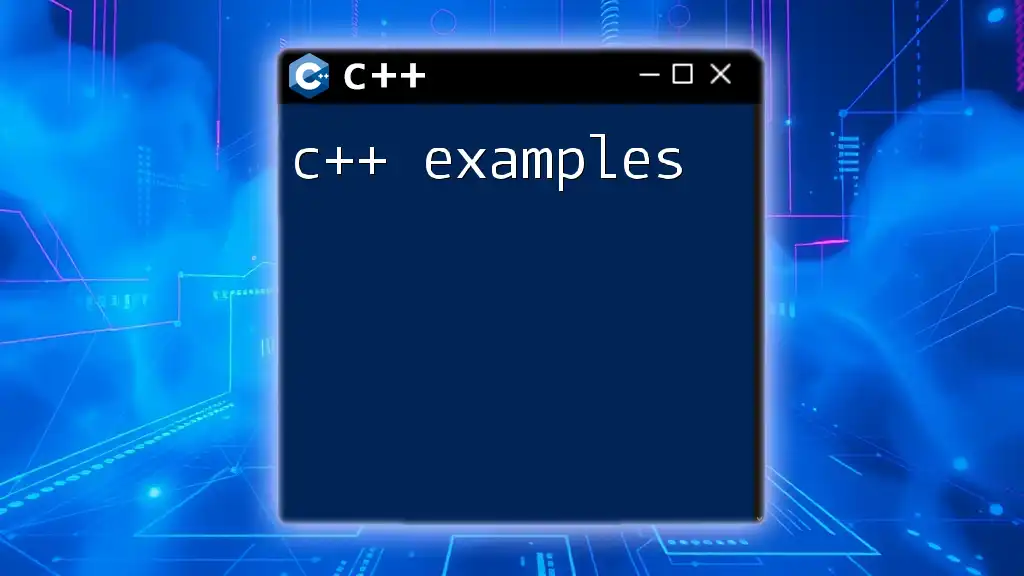
Additional Resources
Further Reading
- Visit the official C++ documentation for deeper insights into tuples and their operations.
- Explore online tutorials focused on C++ standard libraries and their applications in modern development.
Suggested Tools
- Consider using Integrated Development Environments (IDEs) like Visual Studio, CLion, or Code::Blocks for effective C++ programming.