In C++, you can unpack a tuple into individual variables using the `std::tie` function or structured binding introduced in C++17.
Here's an example using structured bindings:
#include <iostream>
#include <tuple>
int main() {
std::tuple<int, double, std::string> myTuple = {1, 2.5, "Hello"};
auto [a, b, c] = myTuple; // Unpacking the tuple
std::cout << "Integer: " << a << ", Double: " << b << ", String: " << c << std::endl;
return 0;
}
What is a Tuple?
A tuple in C++ is a fixed-size collection of elements, potentially of different types. It is defined in the `<tuple>` header and can encapsulate various data types together, providing an efficient way to return multiple values from functions or group related data.
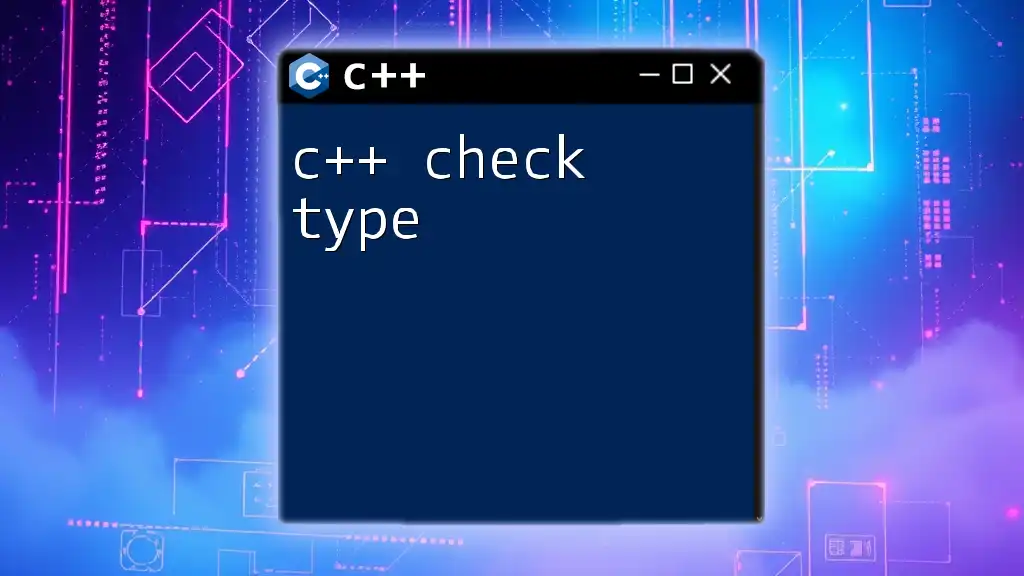
What Does Unpacking Mean?
Unpacking refers to the process of extracting individual elements from a collection—in this case, a tuple. It allows you to leverage the stored values without having to access each element by index repeatedly. This makes code cleaner, easier to read, and reduces chances of errors.
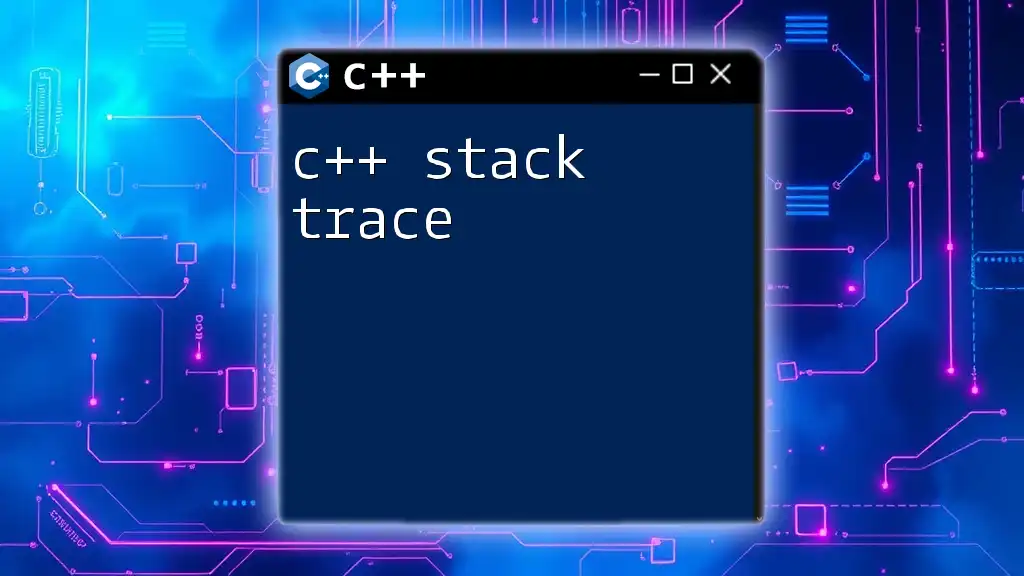
Understanding Tuples in C++
Creating a Tuple
You can create a tuple using the `std::tuple` class. The syntax is quite straightforward:
#include <tuple>
std::tuple<int, double, std::string> myTuple(1, 2.5, "Hello");
In this example, we define a tuple named `myTuple` that holds an integer, a double, and a string. This single line packs three variables into one composite variable.
Accessing Tuple Elements
To access specific elements within a tuple, you can use the `std::get` function. This function requires the index of the element you want:
int a = std::get<0>(myTuple); // Access the first element (1)
double b = std::get<1>(myTuple); // Access the second element (2.5)
std::string c = std::get<2>(myTuple); // Access the third element ("Hello")
Keep in mind that the indices in C++ are zero-based, so `std::get<0>` refers to the first element.
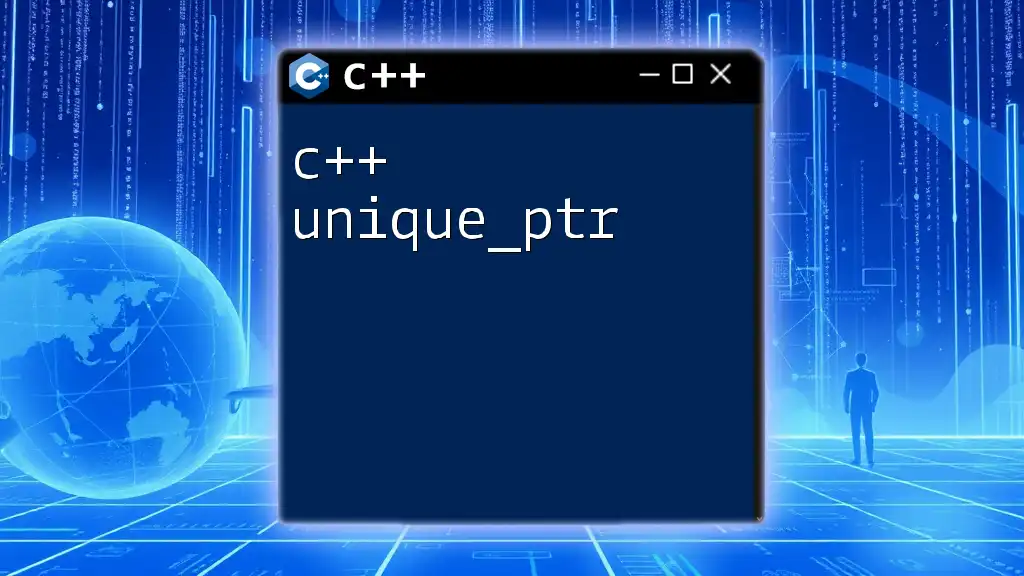
The Concept of Unpacking
What is Tuple Unpacking?
Tuple unpacking is the process of directly assigning the individual elements of a tuple to separate variables. For instance, instead of accessing each element at a specific index, you can extract them all at once, which greatly enhances readability and maintainability of the code.
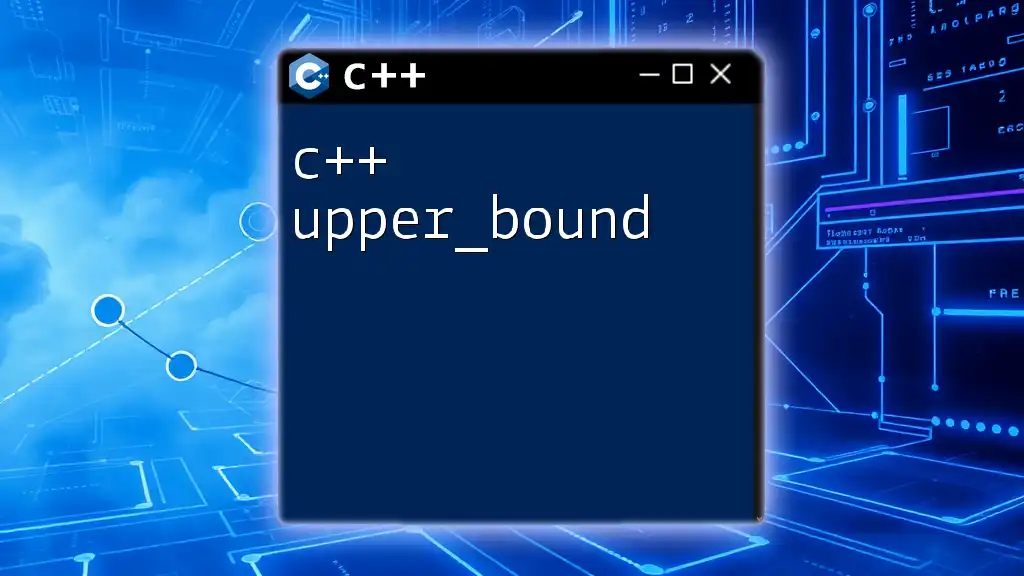
How to Unpack a Tuple in C++
Using `std::tie`
One traditional method to unpack a tuple is by using `std::tie`. This function creates references to the variables passed, allowing them to be assigned values from the tuple:
int x;
double y;
std::string z;
std::tie(x, y, z) = myTuple;
In this example, `x`, `y`, and `z` will be directly populated with the respective values from `myTuple`. Using `std::tie` is particularly useful when you want to manage unpacked variables’ values without having to declare them simultaneously.
Structured Binding (C++17 and Later)
With the introduction of C++17, a more elegant and concise way to unpack tuples has been introduced through structured bindings. This enables you to unpack the tuple like this:
auto [x, y, z] = myTuple;
The `auto` keyword automatically deduces the types of `x`, `y`, and `z`, making the code cleaner, more readable, and intuitive. Structured bindings allow for seamless unpacking without the added complexity of managing references.
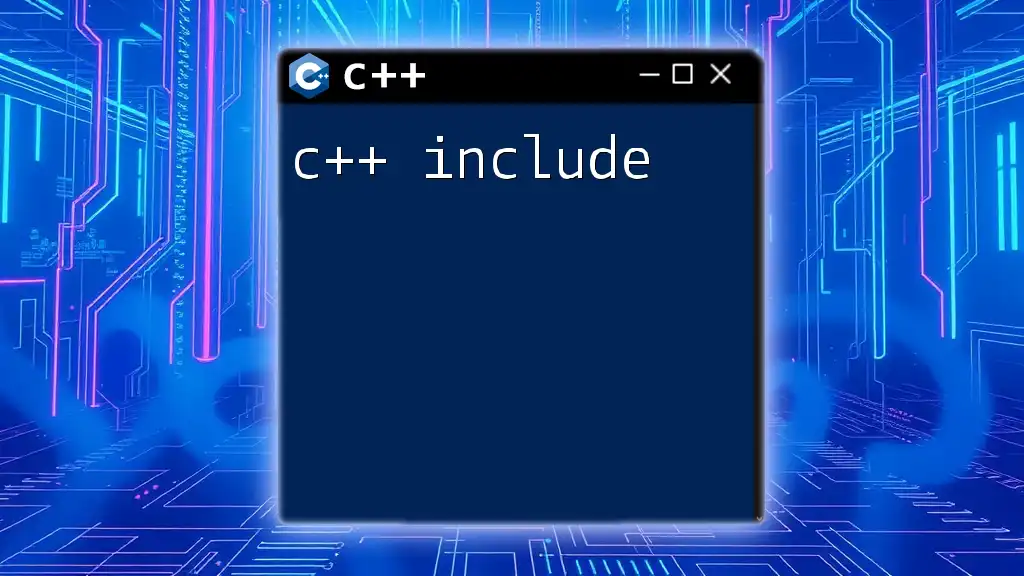
Practical Examples of Tuple Unpacking
Example 1: Function Return Values
Unpacking tuples shines when returning multiple values from a function. Here’s a simple example:
std::tuple<int, double> getCoordinates() {
return std::make_tuple(5, 10.5);
}
auto [xCoord, yCoord] = getCoordinates();
In this function, `getCoordinates` returns a tuple containing an integer and a double. The structured binding from the return allows us to extract `xCoord` and `yCoord` directly.
Example 2: Real-World Applications
Consider a scenario where you fetch user data from a database:
std::tuple<std::string, int, std::string> fetchUserDetails() {
return std::make_tuple("Alice", 30, "Engineer");
}
auto [name, age, job] = fetchUserDetails();
Here, the function `fetchUserDetails` returns a tuple with a user's name, age, and job title. Unpacking it directly into meaningful variable names enhances code readability and usability, especially when managing multiple data fields.
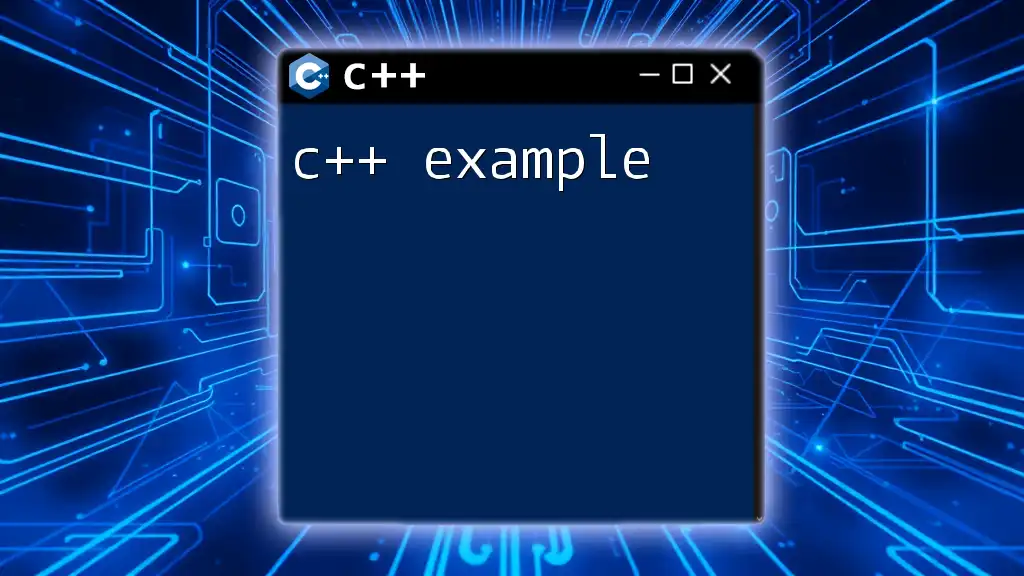
Best Practices for Using Unpacking
Readability and Maintainability
When unpacking tuples, it's crucial to choose clear and descriptive variable names. This ensures that anyone reading the code can easily understand the context of what each variable represents. For example, unpacking using `auto [n, a, j]` is less informative than `auto [name, age, job]`.
Performance Considerations
While unpacking tuples is usually efficient, consider the cost of creating complex tuples within performance-sensitive sections of your code. In cases where simplicity isn’t directly beneficial, prefer using simpler data structures if the performance impact outweighs the benefits of unpacking.
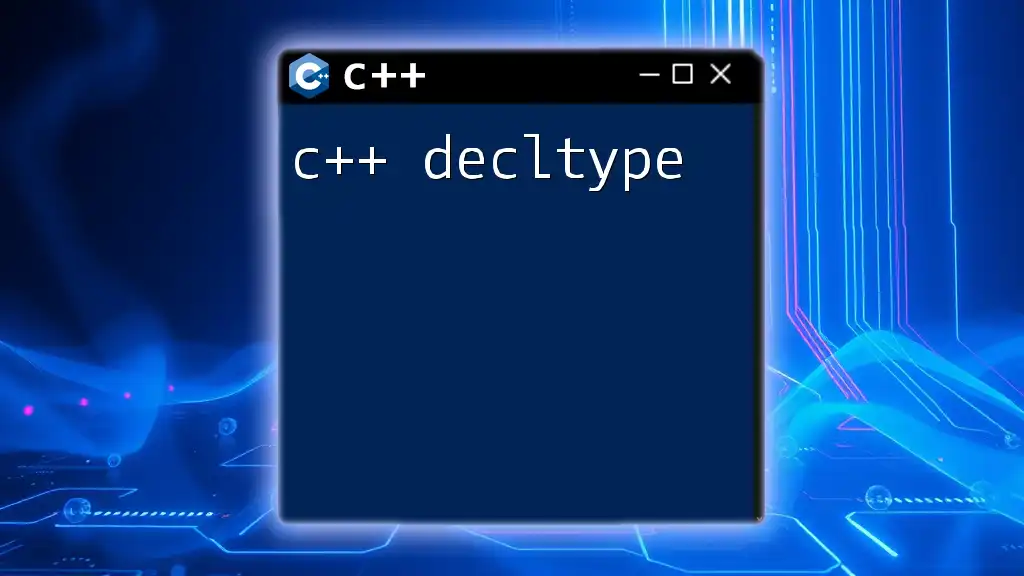
Conclusion
In summary, the ability to unpack tuples in C++ is an effective way to streamline how we manage grouped data. Using tools like `std::tie` and structured bindings significantly enhances code clarity and reduces the boilerplate typically associated with accessing individual elements. Understanding and leveraging tuple unpacking can lead to more maintainable and efficient code.
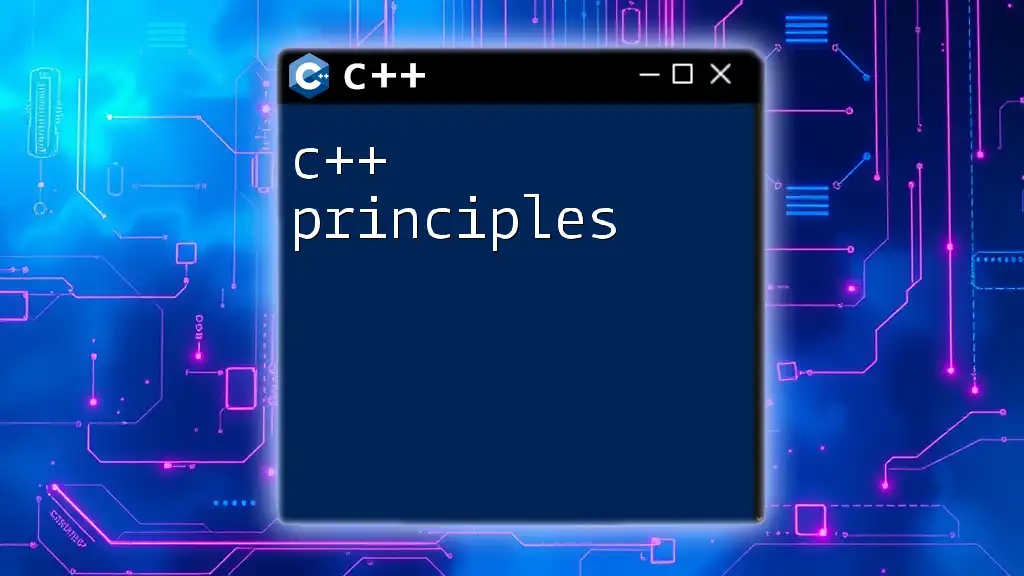
Additional Resources
For further reading and practical exercises, explore C++ documentation on tuples, and consider diving into recommended books or online courses that delve deeper into the intricacies of C++ programming. These resources will be instrumental in refining your understanding and mastery of tuples and their unpacking techniques.