C++ Unicode allows developers to handle multi-language text support by utilizing the `char32_t` type for Unicode code points and string manipulation functions, thereby ensuring proper representation of characters from various languages.
Here’s a code snippet demonstrating how to define and print a Unicode character in C++:
#include <iostream>
#include <string>
int main() {
char32_t unicodeChar = U'😊'; // Unicode code point for the smiling face emoji
std::wcout << L"The Unicode character is: " << static_cast<wchar_t>(unicodeChar) << std::endl;
return 0;
}
Understanding Unicode
Unicode is a universal character encoding standard that assigns a unique number, known as a code point, to every character in virtually every language. It aims to provide a consistent representation and handling of text, regardless of the platform or language. This is particularly important in our increasingly globalized world where applications often need to support multiple languages.
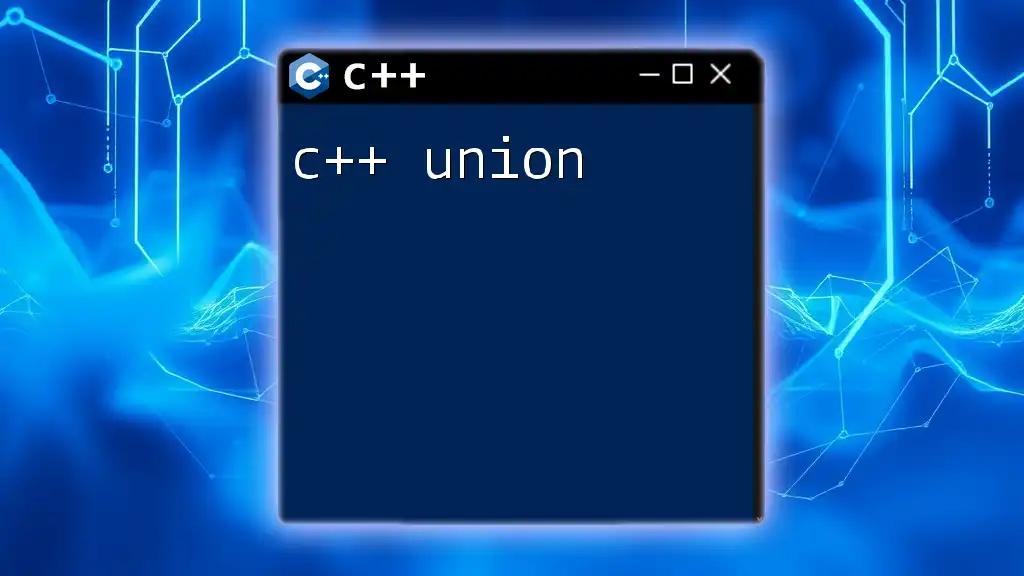
Importance of Unicode in C++
When developing applications in C++, the importance of Unicode cannot be overstated. Providing support for Unicode not only helps ensure that your program can display text in various languages but also avoids errors and misinterpretations of data. Internationalization and localization have become essential practices in software development, and Unicode helps to meet these requirements seamlessly.
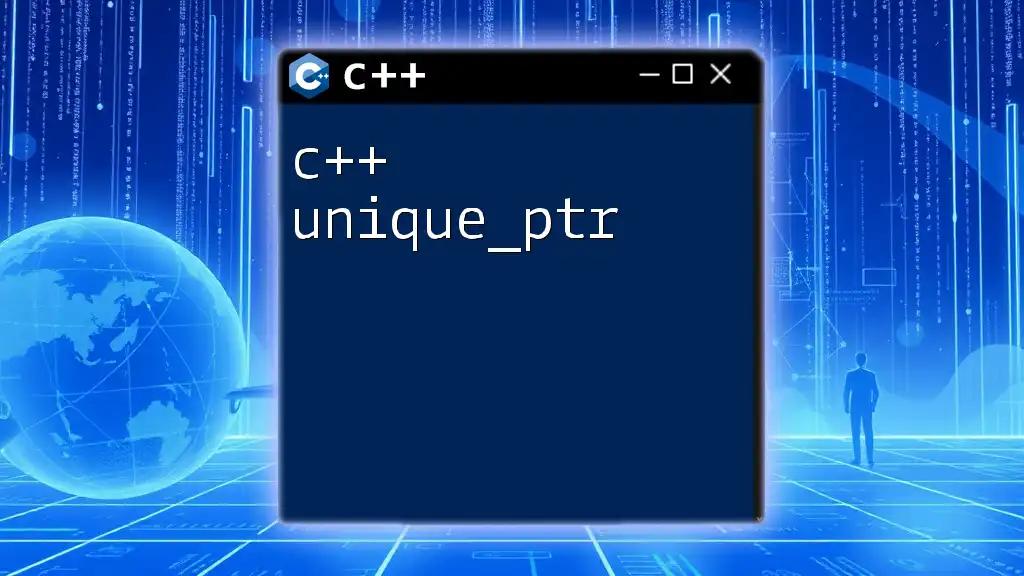
The Basics of Character Encoding
Character Encoding Schemes
Character encoding systems are essential for enabling computers to handle text data. They define how characters are represented in bytes. Some of the most common encoding systems include:
- ASCII: This utilizes 7 bits for each character, allowing for 128 unique symbols, which include English letters and basic punctuation. However, it falls short for non-Latin characters.
- UTF-8: A variable-length encoding that uses 1 to 4 bytes per character. It is backward compatible with ASCII, meaning that original ASCII characters remain encoded in a single byte, thus enabling broader language support.
- UTF-16: It uses 2 bytes for most common characters but can extend to 4 bytes for others.
- UTF-32: A fixed-length encoding that uses 4 bytes for every character, making it easier to calculate character positions but also more memory-intensive.
Comparing ASCII and Unicode
Limitations of ASCII: ASCII is insufficient for modern applications that require support for international characters. Its character set is limited primarily to the English language, which means it cannot adequately represent text in languages with different scripts, such as Arabic, Chinese, or Hindi.
Advantages of Using Unicode Over ASCII: Unicode overcomes ASCII's limitations by providing a vast character set that includes virtually all scripts and symbols. This enables developers to create truly global applications that can be used by people around the world.
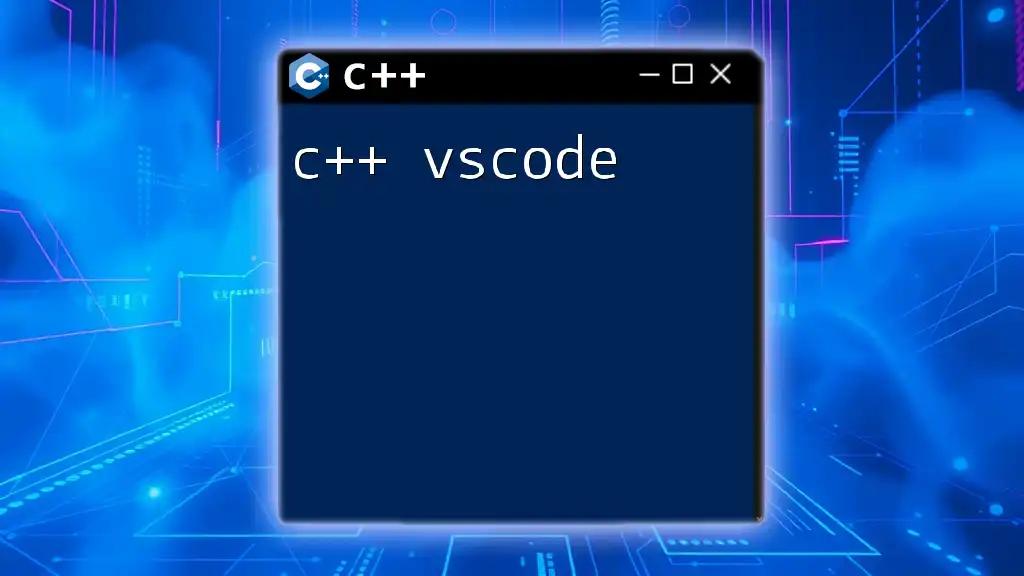
C++ and Unicode Support
Standard C++ and Unicode
C++ offers modular support for Unicode, primarily through libraries and language features. This allows programmers to utilize Unicode efficiently in their code, notwithstanding the complexity that it can sometimes introduce.
Using `<codecvt>` Header
The `<codecvt>` header facilitates converting between different character encodings. This is vital when you need to handle data from varied sources.
Code Snippet: Basic Conversion Example
#include <iostream>
#include <string>
#include <codecvt>
#include <locale>
int main() {
std::wstring_convert<std::codecvt_utf8<wchar_t>> converter;
std::string utf8_string = converter.to_bytes(L"こんにちは");
std::cout << utf8_string << std::endl; // Output in UTF-8
return 0;
}
In this example, we are utilizing the `std::wstring_convert` facility to convert a wide string (Japanese for "Hello") into a UTF-8 encoded string.
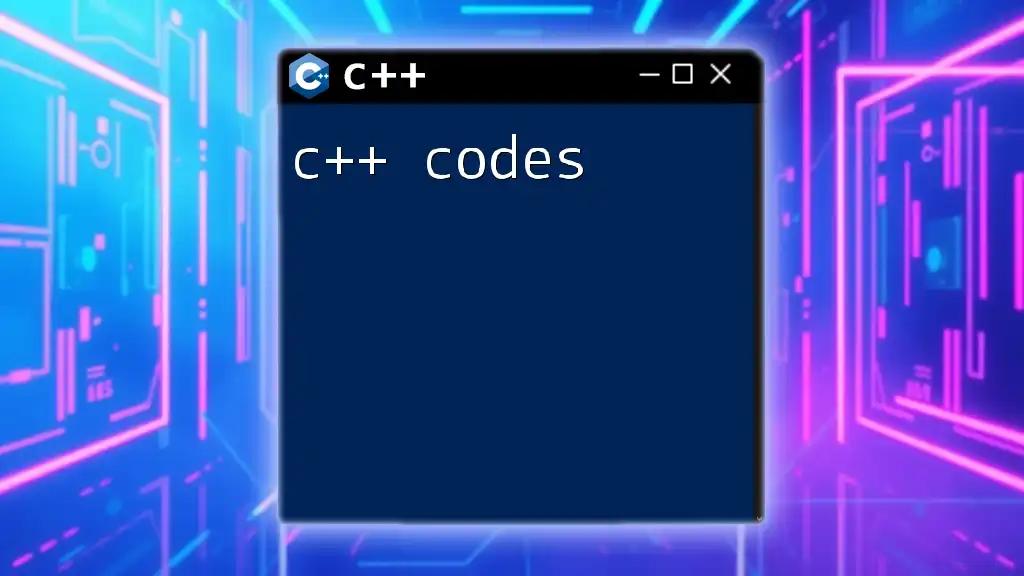
Working with Unicode Strings in C++
Using `std::wstring` for Wide Characters
In C++, the `std::wstring` type can store wide characters (16 or 32 bits). This allows for a more comprehensive representation of Unicode characters, making it an excellent choice for applications that need to support various languages.
String Literals
Understanding how to define Unicode string literals is vital for any application. C++ provides mechanisms for both UTF-8 and UTF-16 string literals.
- UTF-8 Literal: Use `u8"Your string here"`.
- Wide String Literal: Use `L"Hello, 世界"`.
Example of Wide String Literal
const wchar_t* wide_str = L"Hello, 世界";
This string literal can contain characters beyond those covered by ASCII, enabling developers to work with a more diverse set of characters.

Unicode Character Types in C++
Defining Unicode Characters
C++ treats Unicode characters uniquely through types like `char32_t` and `char16_t`. These types allow for direct manipulation of Unicode code points.
Code Point Representation
You can represent Unicode code points using these types, which is helpful when you need to work with specific characters.
Example: Defining a Unicode Character
char32_t smiley_face = U'\U0001F600'; // Grinning Face
Here, the character for a grinning face is defined using its Unicode code point.
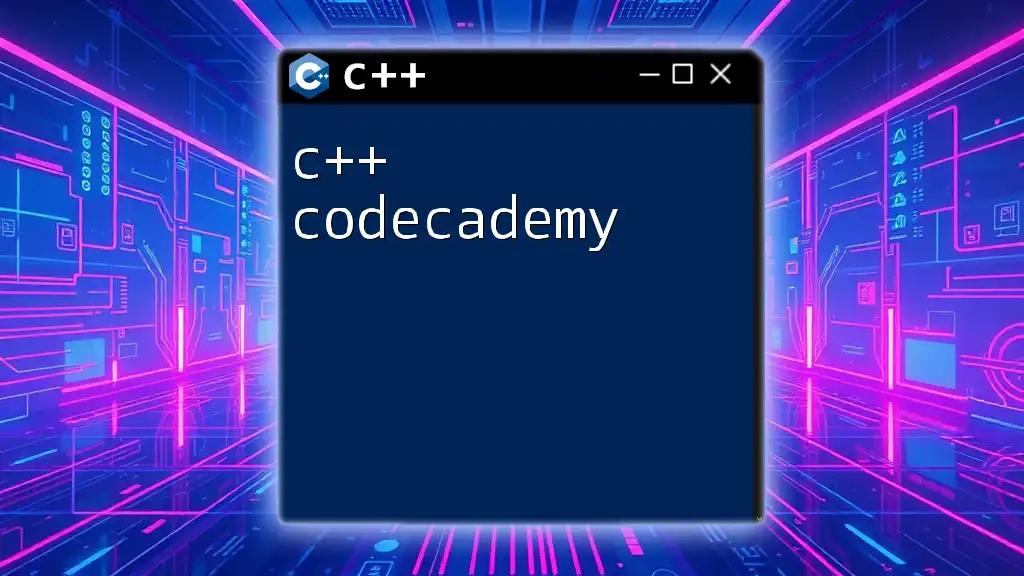
Working with UTF-8 Strings
How to Read and Write UTF-8 Strings
Handling UTF-8 encoded strings in C++ is relatively straightforward. Most modern C++ libraries support UTF-8, making it easier for developers to manage text data.
Library Support for UTF-8
Libraries like `iconv` and `libiconv` offer robust solutions for converting between different character encodings. They can handle a wide variety of formats, making multicultural application development smoother.
Code Snippet: Basic UTF-8 Handling
std::string utf8_string = "Hello, world!";
std::cout << utf8_string << std::endl;
In this snippet, a simple UTF-8 encoded string is printed directly to the console without any additional conversions.

Common Issues in Unicode Handling
Character Misinterpretation
One common issue when working with Unicode in C++ is character misinterpretation. This often arises when different parts of an application expect or process different encodings, leading to mishandled input or output.
Debugging Unicode Issues
To effectively debug Unicode issues, consider the following:
- Ensure consistent character encoding across your application.
- Use utilities to check the encoding type of input strings.
- Leverage C++ debugging and logging functionalities to analyze character representations.

Real-world Applications of Unicode in C++
Internationalization of Applications
Many successful applications have successfully harnessed the power of Unicode for internationalization. Companies like Google and Microsoft implement robust Unicode support to serve their diverse user bases.
User Input Handling
Effective handling of user input from various languages is crucial. By validating and normalizing user input, developers can create systems that prevent the entry of malformed text. For instance, stripping out unusual characters can help maintain data integrity.

Conclusion
In summary, C++ provides a robust and flexible framework for incorporating Unicode into your applications. By understanding the character encoding landscape and leveraging C++'s Unicode support features, developers can build applications that cater to a global audience. The incorporation of Unicode not only enhances user experience but also future-proofs your application in today's diverse technological landscape.
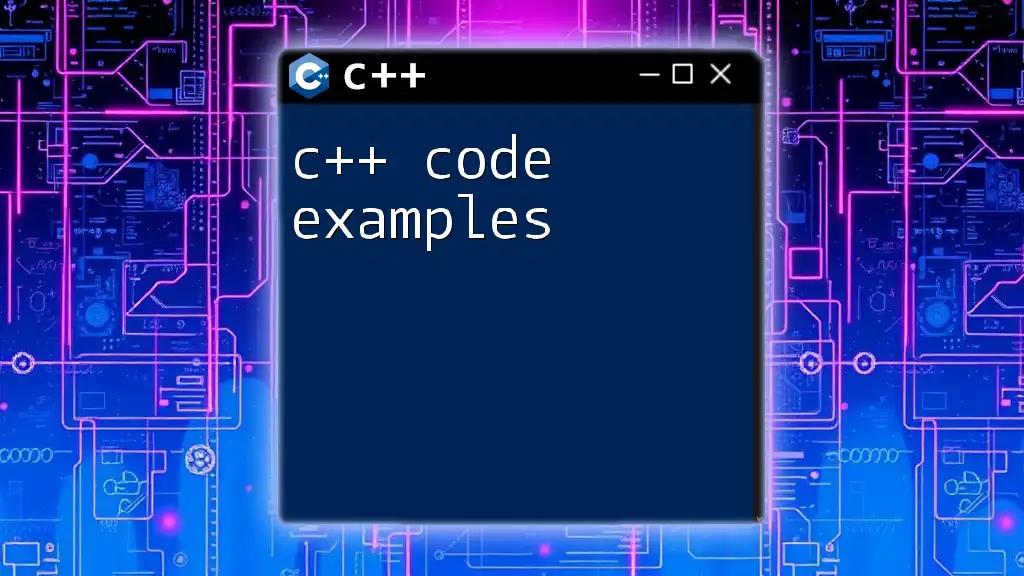
Call to Action
If you're looking to dive deeper into C++ Unicode, don’t hesitate to start exploring! This knowledge will empower you not only to write better code but also to make meaningful connections with users worldwide.