C++ unit tests are automated tests written to verify that individual units of source code work as intended, ensuring reliability and correctness in your codebase.
Here's a simple example using the Google Test framework:
#include <gtest/gtest.h>
// Function to be tested
int add(int a, int b) {
return a + b;
}
// Test case
TEST(AdditionTest, HandlesPositiveInput) {
EXPECT_EQ(add(2, 3), 5);
}
int main(int argc, char **argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
Introduction to C++ Unit Testing
What are Unit Tests?
Unit tests are essential components of software development that validate the functionality of specific sections of code, typically individual functions or methods, in isolation from the rest of the application. The main purpose of unit tests is to ensure that each part of the software behaves as intended. When you create unit tests, you gain confidence that your code is working correctly and can handle expected inputs and edge cases.
Benefits of C++ Unit Testing
Implementing unit tests in your C++ projects offers numerous advantages:
- Enhances code quality and maintainability: By regularly running unit tests, you ensure that your code remains robust and manageable over time.
- Early bug detection and resolution: Unit tests allow you to catch issues at an early stage, significantly reducing the time and cost associated with bug fixing.
- Facilitates easier refactoring: When changes are made to the code, unit tests can help confirm that existing functionality remains intact, fostering more fearless refactoring.
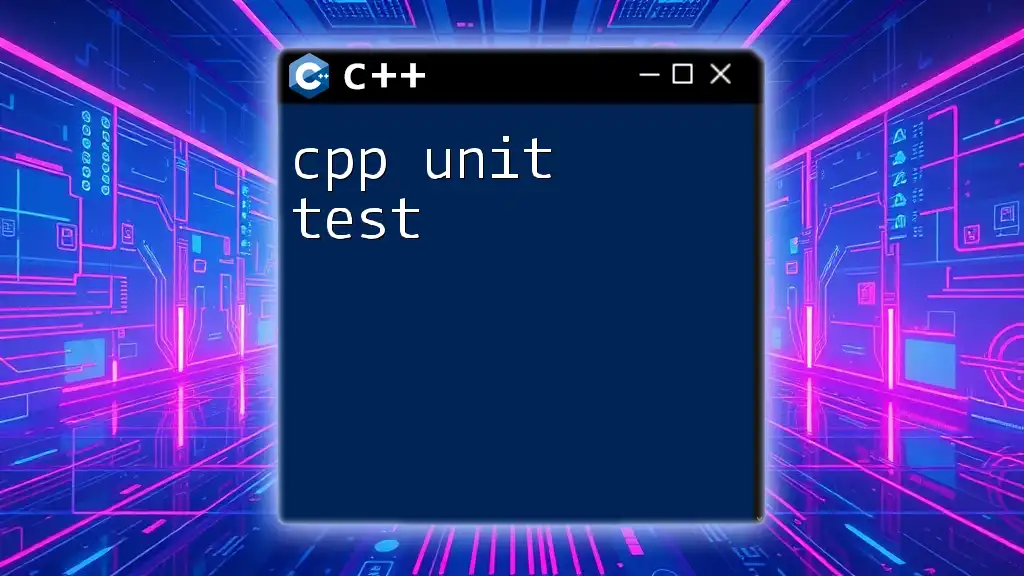
Setting Up a C++ Unit Testing Environment
Choosing a C++ Unit Testing Framework
There are several frameworks available for C++ unit testing, with Google Test and Catch2 being among the most popular. When selecting a framework, consider factors such as community support, ease of use, and compatibility with your development environment.
Installation and Configuration
To get started with unit testing using Google Test:
-
Overview of Google Test
Google Test provides a simple API for writing unit tests in C++. It is well-documented and widely used, making it a great choice for both beginners and experienced developers. -
Step-by-step Installation Guide for Google Test
- Windows: Use vcpkg, CMake, or download the source from GitHub and build it manually.
- Linux: Generally, you can install it via the package manager (e.g., `apt install libgtest-dev`) and then compile it.
- MacOS: You can install Google Test using Homebrew with `brew install googletest`.
-
Configuration Tips
For Visual Studio, after installing Google Test, make sure to add the appropriate include and library directories in your project settings. If you use CMake, include the following in your `CMakeLists.txt`:find_package(GTest REQUIRED) include_directories(${GTEST_INCLUDE_DIRS})
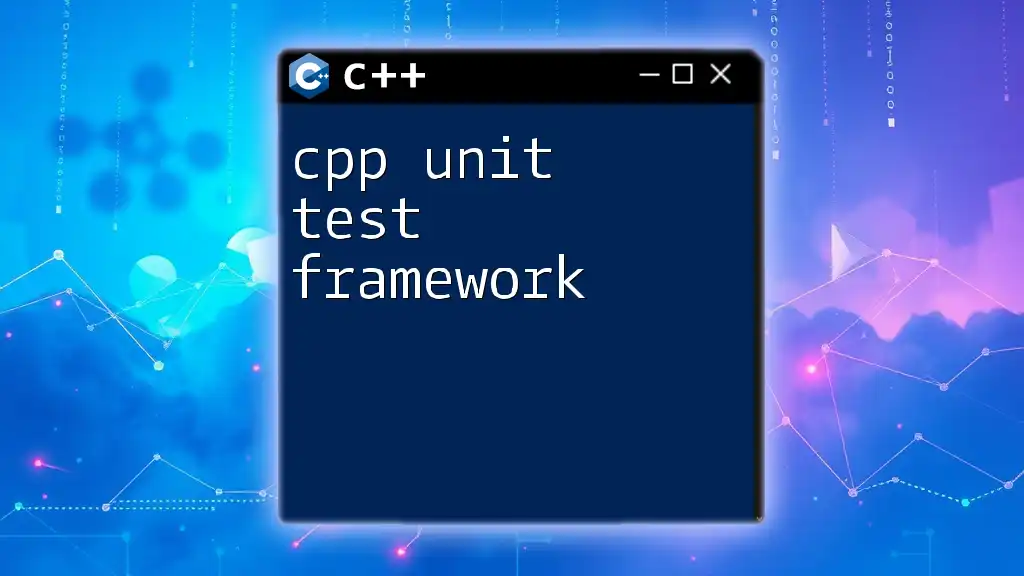
Basics of Writing C++ Unit Tests
Structure of a Unit Test
A typical unit test contains three main parts:
- Test Case: Defines what you are testing and sets up the environment.
- Assertions: Check if the output of the code matches the expected output.
- Teardown: Cleans up any resources that are not needed after the test completes.
Here’s a basic example of a unit test:
#include <gtest/gtest.h>
int Add(int a, int b) {
return a + b;
}
TEST(AddTest, PositiveNos) {
EXPECT_EQ(Add(1, 2), 3);
EXPECT_EQ(Add(3, 4), 7);
}
In this example, the `Add` function is being tested to ensure it produces the correct results for different inputs.
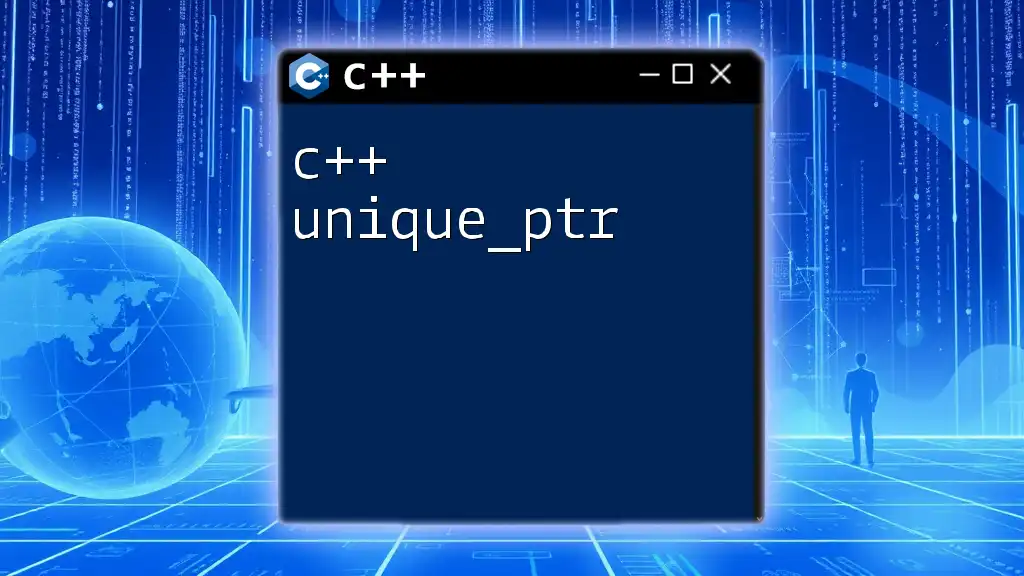
Writing Effective Unit Tests
Best Practices for C++ Unit Testing
To maximize the effectiveness of your unit tests, adhere to several best practices:
- One assertion per test: Each test should validate a single condition to simplify debugging.
- Naming conventions for test cases: Use descriptive names that clearly indicate what the test is validating.
- Keeping tests independent: Tests should not rely on the outcome of others to avoid cascading failures.
Common Pitfalls to Avoid
Newcomers to unit testing often make mistakes that can hinder the testing process. Some pitfalls to avoid include:
- Overly complex tests: Simple tests are easier to write, read, and maintain. If a test does too much, break it down into simpler tests.
- Relying on external dependencies: Strive to isolate your tests; they should run irrespective of database states or network calls.
- Ignoring edge cases: Consider scenarios that could potentially lead to failures and write tests to validate that your code handles them properly.
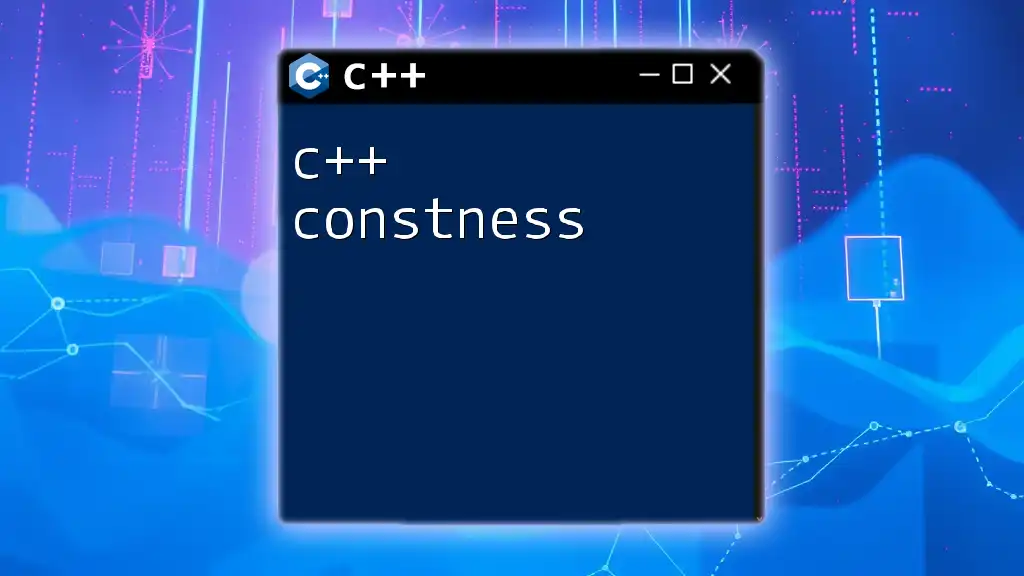
Advanced Unit Testing Concepts
Mocking and Stubbing
Mocking is a technique used in unit testing to simulate behavior of complex objects or systems that are not part of the unit being tested. Stubs provide fixed responses to calls made during the test and can help isolate unit tests from external components.
Here’s an example of using mocks with Google Mock:
class Database {
public:
virtual int GetUserCount() = 0;
};
class MockDatabase : public Database {
public:
MOCK_METHOD(int, GetUserCount, (), (override));
};
In this case, `MockDatabase` replaces actual database interactions, allowing tests to be conducted without relying on a real database.
Parameterized Tests
When certain tests require the same logic with different inputs, parameterized tests are beneficial. They allow you to run the same test multiple times with varying parameters.
Here’s a simple example using Google Test:
TEST_P(AddTest, HandlesVariousInputs) {
EXPECT_EQ(Add(GetParam().first, GetParam().second), GetParam().result);
}
INSTANTIATE_TEST_SUITE_P(AdditionTests, AddTest,
testing::Values(std::make_tuple(1, 1, 2), std::make_tuple(2, 3, 5)));
This allows you to efficiently run a series of tests with different sets of data without duplicating code.
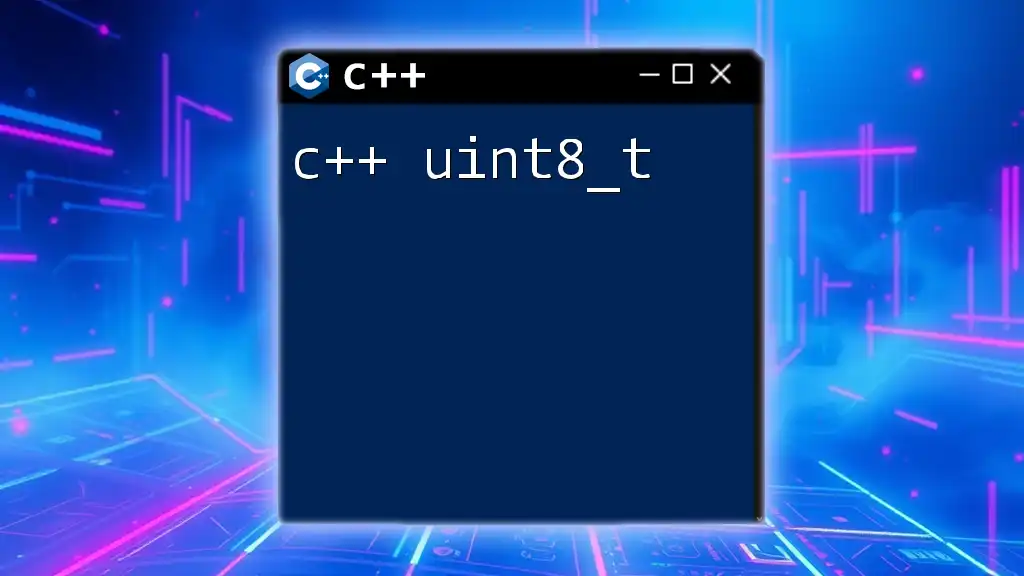
Running and Managing C++ Unit Tests
Executing Unit Tests
Running your tests can be done in various ways. Command-line execution is straightforward:
./your_test_binary
Alternatively, most development environments, like Visual Studio, allow you to run your tests directly from the IDE. Understanding how to interpret the output from these tests is crucial. Typically, barring any assertions failing, you’ll see a summary report indicating a pass or fail status for each test case.
Continuous Integration for Unit Testing
Integrating unit tests into your CI/CD process ensures that every code change is automatically tested. This practice significantly enhances software quality and reduces the likelihood of bugs in production. Tools like GitHub Actions or Travis CI can be configured to run your unit tests whenever code gets pushed or a pull request is created. This ensures that any issues are caught early in the development cycle.
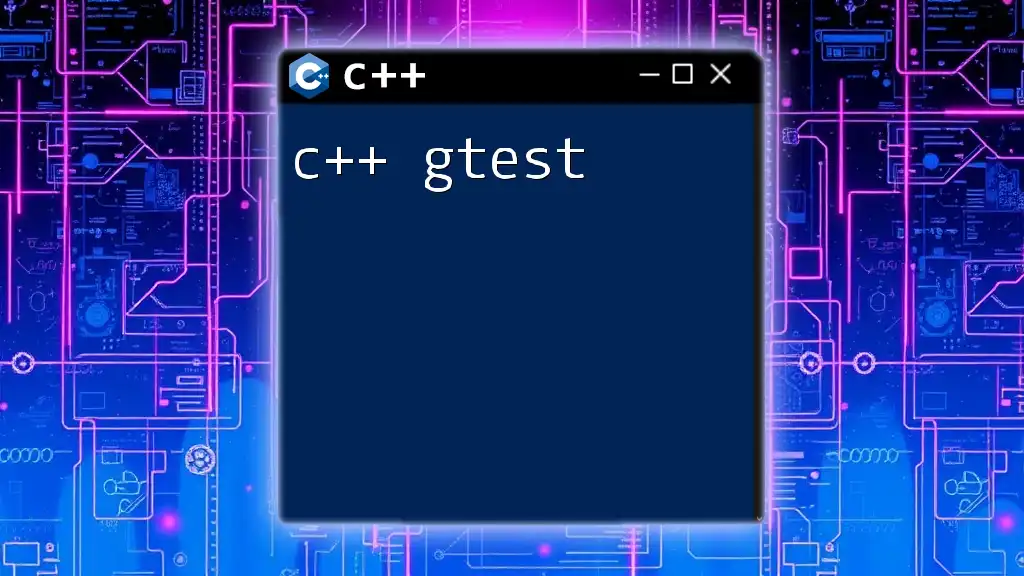
Conclusion
In this article, we explored the fundamentals of C++ unit tests, covering everything from the basic concepts to more advanced techniques like mocking, stubbing, and parameterized tests. Leveraging unit tests effectively not only boosts the quality of your code but also fosters a culture of continuous improvement and confidence in your software’s reliability. Now is the perfect time to start integrating C++ unit tests into your development workflow to reap the long-term benefits.
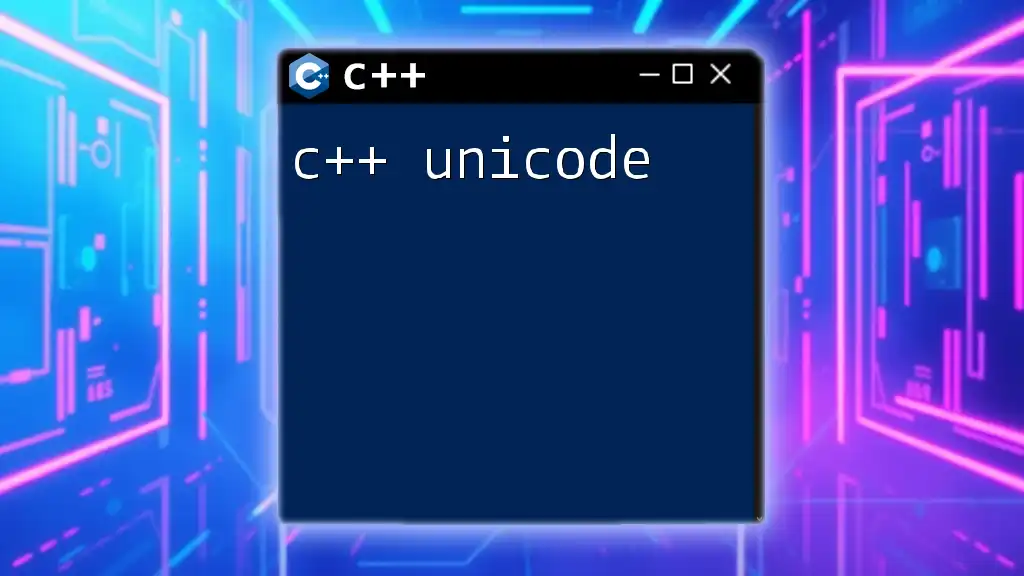
Additional Resources
For further reading, consider exploring recommended books and online courses on C++ testing methodologies. Participating in community forums can also enhance your understanding and enable you to connect with other developers who share your passion for C++.