C++ unit testing involves writing small test cases to validate the functionality of individual units of code, ensuring they perform as expected.
Here's a simple example of a unit test using the Google Test framework:
#include <gtest/gtest.h>
// Function to be tested
int add(int a, int b) {
return a + b;
}
// Unit test for the add function
TEST(AddTest, PositiveNumbers) {
EXPECT_EQ(add(2, 3), 5);
EXPECT_EQ(add(10, 15), 25);
}
TEST(AddTest, NegativeNumbers) {
EXPECT_EQ(add(-2, -3), -5);
EXPECT_EQ(add(-10, 5), -5);
}
int main(int argc, char** argv) {
::testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
Introduction to C++ Unit Testing
What is Unit Testing?
Unit Testing is a software testing technique where the smallest testable parts of an application, known as units, are individually and independently assessed for proper operation. This process aims to validate that each unit of the code performs as expected, enhancing reliability and ensuring a strong foundation for the software.
Unit testing brings significant benefits to developers, such as:
- Early Detection of Bugs: By running tests frequently during development, you can identify issues at an early stage.
- Simplified Debugging: When a test fails, it narrows the scope to the specific unit, making it easier to locate the source of the problem.
- Documentation: Unit tests serve as live documentation for the behavior of your code, showing how classes and methods are intended to be used.
Importance of C++ Unit Testing
C++ unit testing is particularly crucial due to the complexities and intricacies of C++ programming. It aids in:
- Ensuring Code Reliability: Consistently running unit tests ensures that the code remains functional after any modifications or enhancements.
- Facilitating Code Refactoring: When refactoring code, existing unit tests provide a safety net, assuring that changes do not introduce new errors.
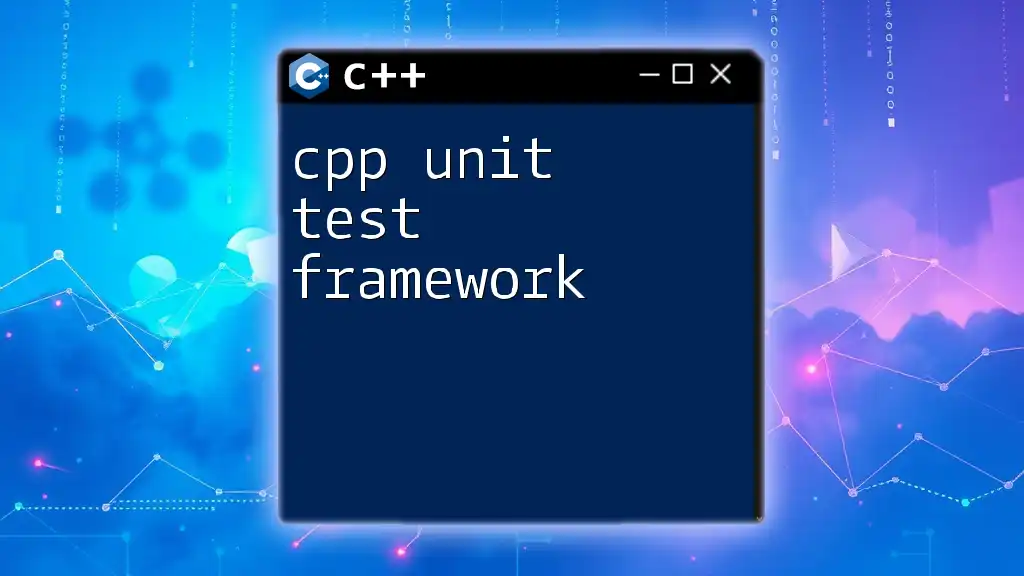
Setting Up Your C++ Testing Environment
Why Use a Testing Framework?
Utilizing a framework for unit testing offers structured methods for defining and running tests, allowing developers to focus on writing effective tests rather than worrying about the underlying mechanics of executing them.
Popular C++ Testing Frameworks
Several robust frameworks exist for C++ unit testing, each catering to different needs and preferences:
- Google Test: A widely-used testing framework that provides a rich set of assertions and supports test fixtures, parameterized tests, and mocking.
- Catch2: An easy-to-use single-header framework that is known for its expressive syntax and excellent integration with CI.
- Boost Test Library: Part of the larger Boost Libraries, this framework integrates well for projects already using Boost.
Installation Guide
For this guide, we'll focus on Google Test, as it’s one of the most prevalent frameworks in C++ development.
Step-by-Step Installation for Google Test
-
Clone the repository:
git clone https://github.com/google/googletest.git cd googletest
-
Build the library:
cmake . make
-
Install the library:
sudo make install
Now that your testing environment is set up, you can start writing your first C++ unit test.
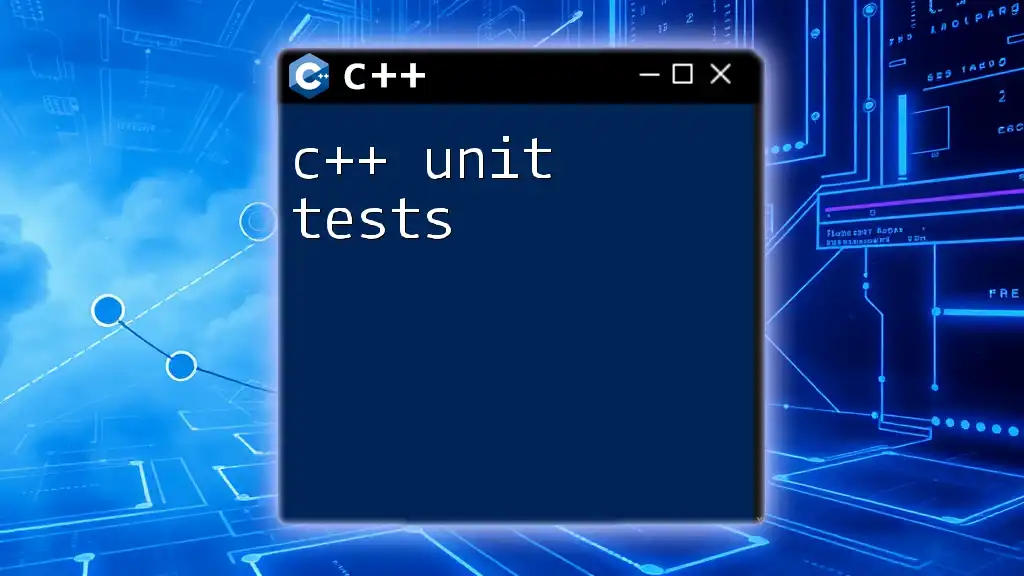
Writing Your First C++ Unit Test
Understanding Test Cases
A test case encapsulates a specific behavior you expect from a unit of code. Here’s a straightforward example of a test case using Google Test.
Code Snippet: Basic Test Case with Google Test
#include <gtest/gtest.h>
TEST(SampleTest, SampleAssertion) {
EXPECT_EQ(1, 1);
}
In this example, `TEST` is a macro that defines a test named `SampleTest`, and within it, the assertion checks if `1` is equal to `1`. This is a fundamental structure for writing tests.
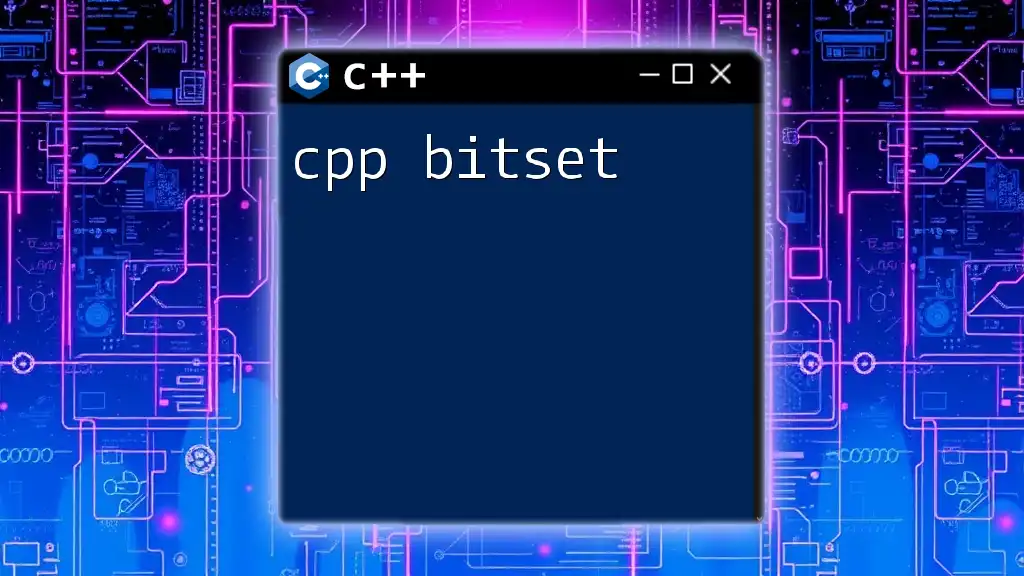
Structuring Your Test Code
Organizing Test Files
It’s crucial to maintain a clear structure in your test code. A recommended practice is to mirror your source code directory structure. For example:
/project
/src
my_code.cpp
my_code.h
/tests
test_my_code.cpp
Naming Conventions for Tests
Implementing clear and consistent naming conventions helps maintain clarity. Test names should indicate the functionality being tested, the scenario, and the expected outcome.
Example: A test for a function `add` could be named `Add_WhenGivenTwoPositiveNumbers_ReturnsTheirSum`.
Code Snippet: Structuring Tests in a C++ File
#include <gtest/gtest.h>
TEST(FunctionalityX, Test1) {
// test logic here
}
TEST(FunctionalityY, Test2) {
// test logic here
}
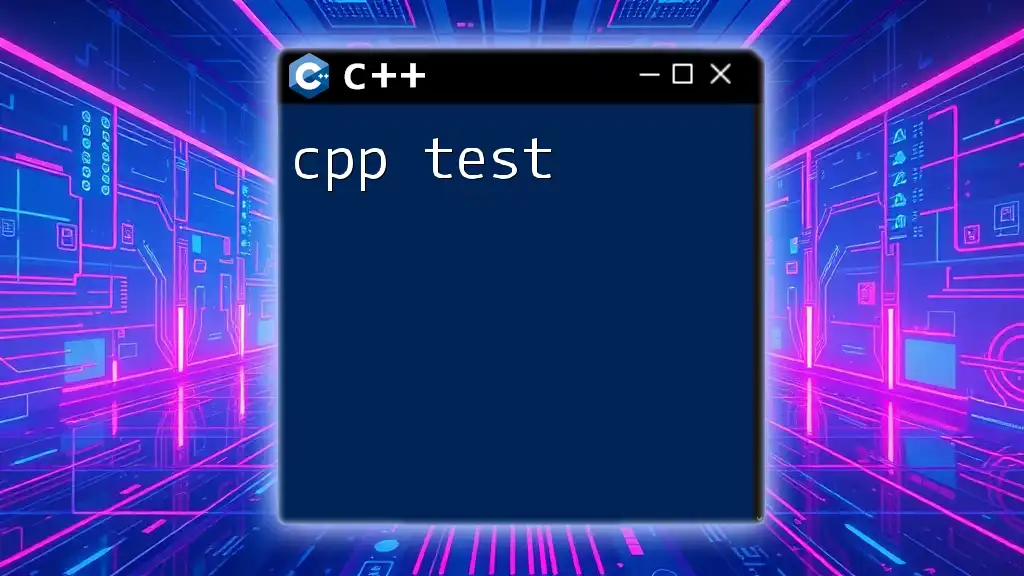
Advanced Unit Testing Concepts
Test Fixtures
Test fixtures are essential when multiple tests require a common setup. They provide a way to initialize state and manage resources for multiple tests.
- What are Test Fixtures?: A test fixture sets up a known environment for running tests.
Code Snippet: Using Test Fixtures
class MyTestFixture : public ::testing::Test {
protected:
void SetUp() override {
// Initialize your objects before each test
}
void TearDown() override {
// Clean up after each test
}
};
TEST_F(MyTestFixture, TestWithFixture) {
// test logic using shared setup
}
In the above code, the `MyTestFixture` class handles preparation and cleanup, streamlining test execution.
Mocking in Unit Tests
Mocking is the practice of simulating the behavior of complex objects. It helps isolate the unit being tested, especially when it interacts with external components.
- Importance of Mocking: Ensures that your tests do not rely on the actual implementation of dependencies, allowing for precise control over the test’s environment.
Code Snippet: Mocking an Interface
class MockFoo : public Foo {
public:
MOCK_METHOD(void, Bar, (int), (override));
};
In this example, `MockFoo` simulates behavior for methods defined in `Foo`, allowing the test to focus solely on the logic of the unit being tested.
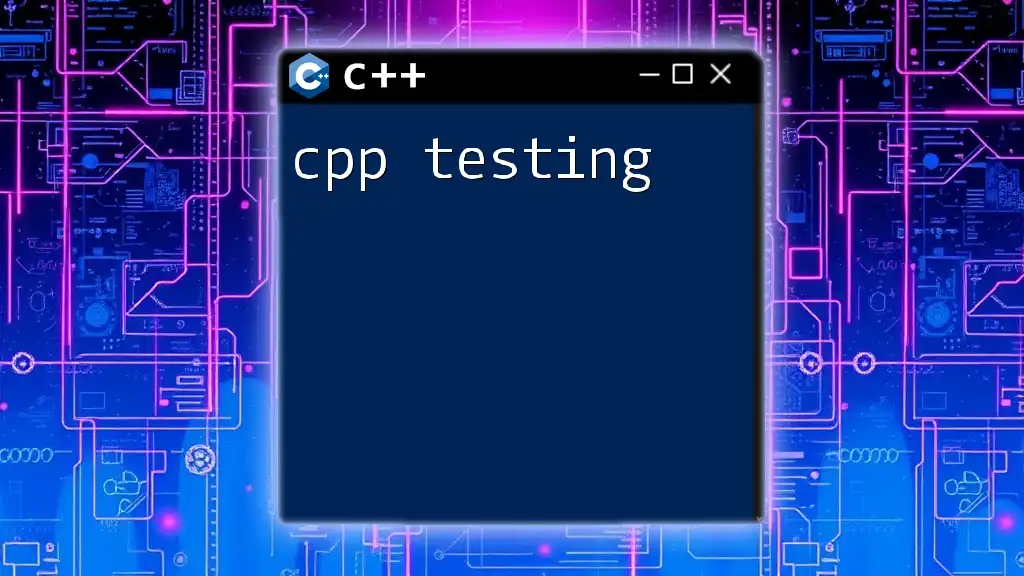
Running Your C++ Unit Tests
Executing Tests in Google Test
Google Test provides a simple command-line interface for running tests. To execute your tests, compile your test files and run the generated executable.
Generating Test Reports: Google Test can also produce output in various formats, such as XML, which is useful for integration with CI tools.
Continuous Integration (CI) and Testing
Incorporating unit tests into your CI/CD pipelines ensures automated testing every time code is pushed. Instruments like Jenkins, Travis CI, and GitHub Actions can be configured to run your C++ tests automatically upon code changes, improving code quality and checking for regressions.
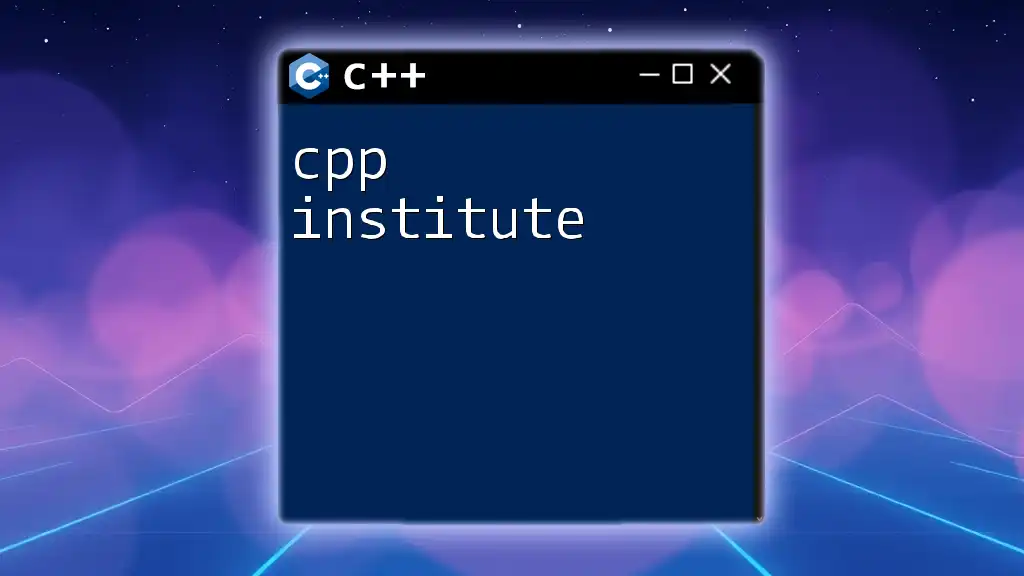
Best Practices for C++ Unit Testing
Writing Effective Test Cases
Effective unit tests should focus on a single aspect of behavior. This approach adheres to the Arrange, Act, Assert pattern:
- Arrange: Set up necessary preconditions
- Act: Execute the action under test
- Assert: Verify that the outcome matches expectations
Maintaining Your Tests
Regularly review and refactor your tests alongside your code changes. Keeping your tests maintained ensures that they remain relevant and effective over time, facilitating continued integration and deployment processes.
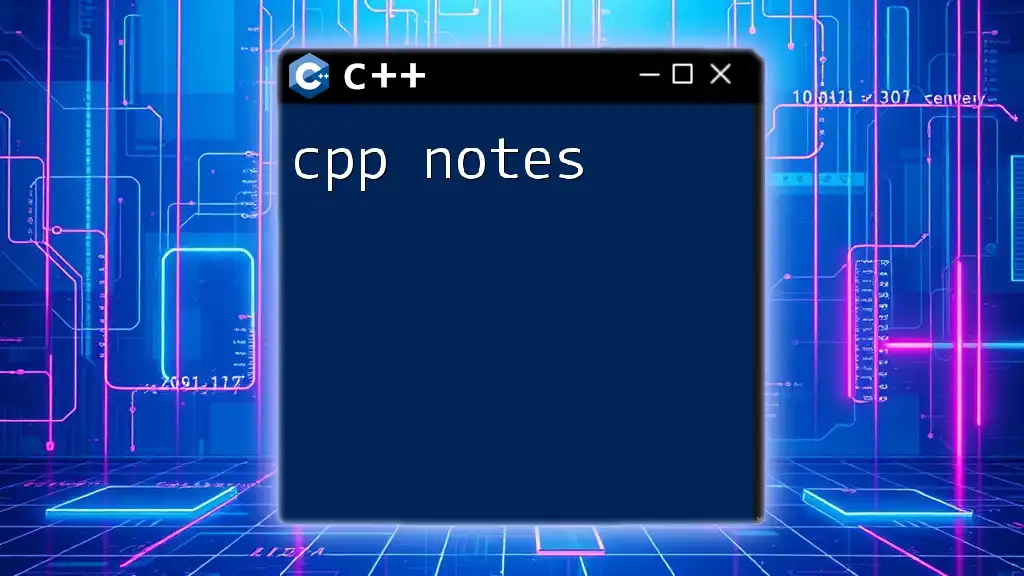
Common Pitfalls in C++ Unit Testing
Ignoring Edge Cases
It’s essential to test not only average scenarios but also edge cases. Neglecting edge cases can lead to significant bugs in production, where input varies widely from standard conditions.
Overlooking Dependencies
Ensure to isolate tests from their dependencies. Utilize measuring tools and practices that allow assessment of the unit and its behavior without reliance on complex external systems.
Not Running Tests Regularly
Incorporate a routine for running tests frequently during the development cycle. The sooner you catch a potential issue, the less impact it will have on the overall project.
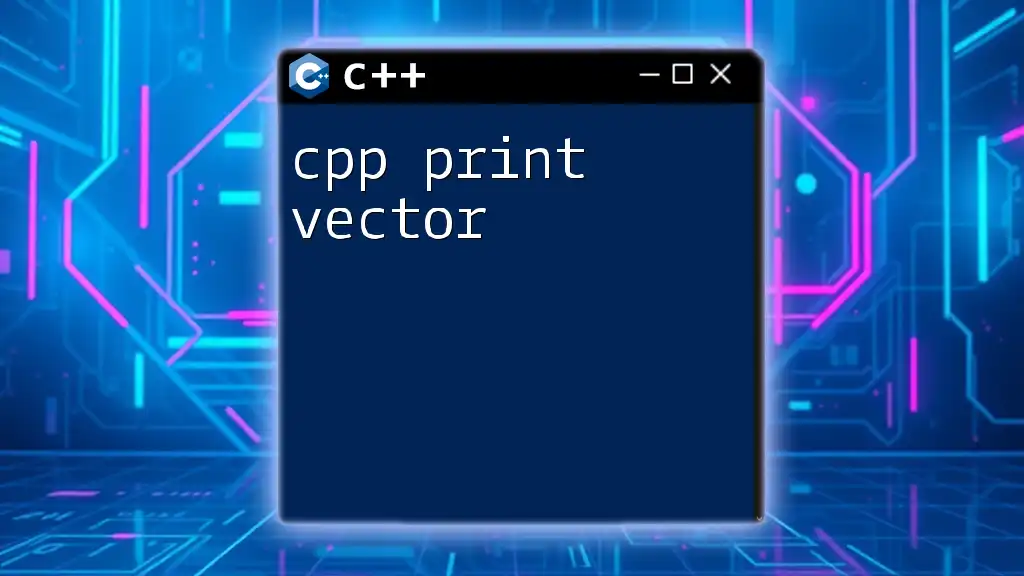
Conclusion
C++ unit testing is a foundational skill for any software developer working with the language. By prioritizing the discipline of unit testing, you can not only improve your code quality but also streamline your development process. Whether you're just starting or looking to refine your testing habits, embracing the concepts covered in this guide will enhance your programming capabilities. Never underestimate the value of well-structured tests; they're an investment that pays dividends in software quality and maintainability.
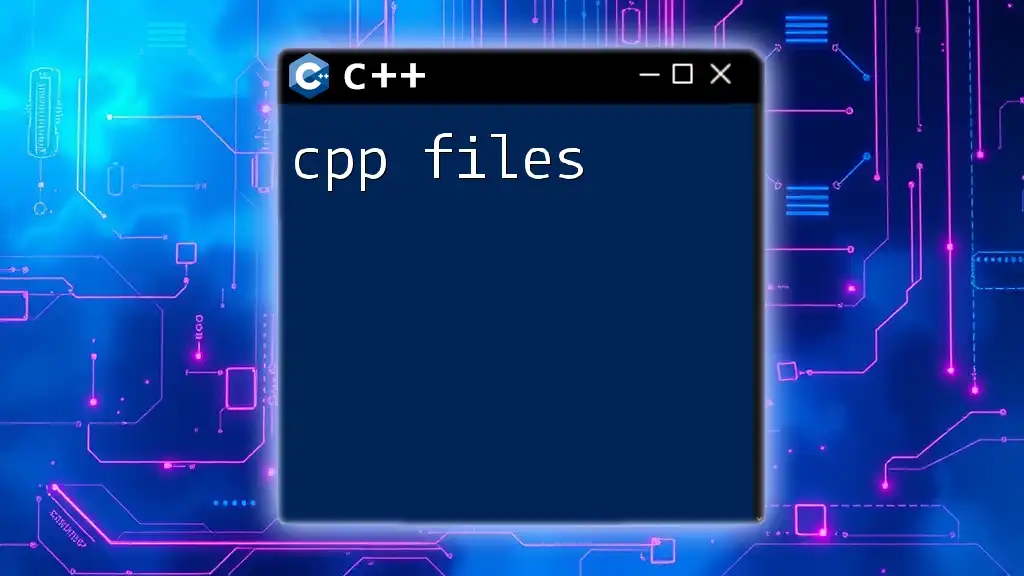
Additional Resources
Further Reading
Explore books and online courses dedicated to C++ testing techniques to deepen your understanding and skills.
Community and Support
Join online forums and support groups for C++ developers to share knowledge, troubleshoot, and grow your expertise in unit testing and beyond.