A "cpp test" typically involves writing a simple C++ program to demonstrate basic command usage or syntax in the language. Here's an example of a basic C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Commands
Definition of C++ Commands
C++ commands refer to the instructions that a programmer can give to the C++ compiler, allowing the execution of various tasks. These commands dictate the behavior of the program, such as how to perform calculations, manage memory, or handle user input. They play a crucial role in constructing logical flows and operations within the application.
Categories of C++ Commands
C++ commands can be categorized into two main types:
-
Built-in Commands: These are predefined commands provided by the C++ language. They allow developers to perform common operations without the need for extensive coding. For example, commands like `cout` for output and `cin` for input are built-in features of C++.
Example of a simple built-in command:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
-
User-defined Commands: Unlike built-in commands, user-defined commands are created by programmers to encapsulate specific functionalities. This can enhance code reusability and clarity. Functions in C++ serve as the primary means of creating user-defined commands.
Example of a user-defined command:
#include <iostream> void greet() { std::cout << "Welcome to C++ Programming!" << std::endl; } int main() { greet(); return 0; }
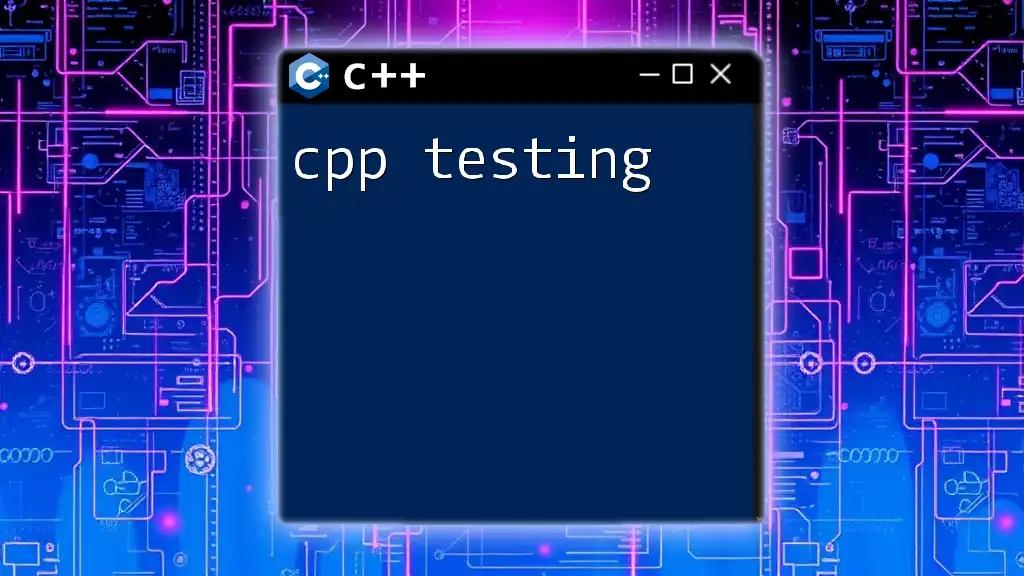
What is a C++ Test?
Understanding C++ Test Concepts
A cpp test is a structured approach to validate the behavior and performance of a C++ program. Its primary objective is to ensure that the application meets its specifications and behaves as expected under various conditions. Testing is an integral part of software development that reduces bugs and enhances software quality.
Different Types of C++ Tests
-
Unit Testing: This type of testing focuses on individual components of the software, known as units. The goal is to validate that each unit operates as designed.
Example of a simple unit test using a framework like Google Test:
#include <gtest/gtest.h> int add(int a, int b) { return a + b; } TEST(AdditionTest, PositiveNumbers) { EXPECT_EQ(add(3, 4), 7); EXPECT_EQ(add(5, 2), 7); }
-
Integration Testing: After unit tests, integration testing comes into play by checking how well different units work together. It helps to identify issues arising from the interaction between components.
-
Functional Testing: This testing verifies the software against functional specifications. It examines the system in a manner that mimics user behavior.
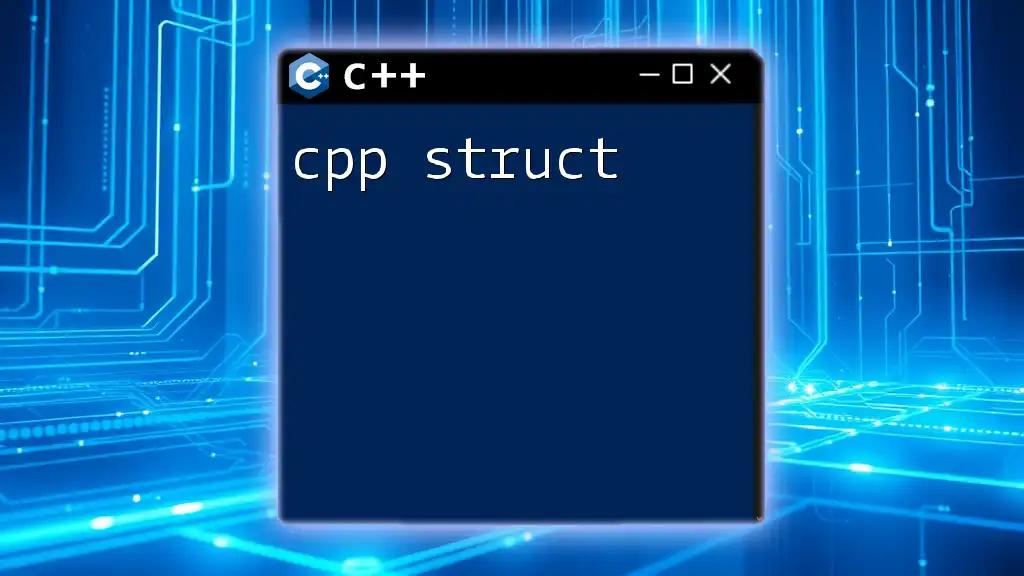
Preparing for a C++ Test
Setting Up Your Environment
To begin testing in C++, it’s essential to set up an adequate development environment. Popular IDEs like Visual Studio, Code::Blocks, and CLion offer integrated testing solutions and debugging tools that ease the process of writing tests.
To write your first test program, consider structuring your code properly and leveraging testing framework features. For example, installing Google Test and setting up your project to include necessary test files is a critical first step.
Study Materials for C++ Exams
Preparing for a cpp exam requires a solid understanding of both theoretical and practical aspects of C++. Recommended resources include:
-
Books: Look for titles focusing on C++ fundamentals and testing methodologies, such as "C++ Primer" and "Effective C++."
-
Online Courses: Platforms like Coursera and Udemy offer courses specifically tailored to C++ testing.
-
Practice Exercises: Hands-on coding is essential. Websites like HackerRank and LeetCode provide excellent resources for practice.
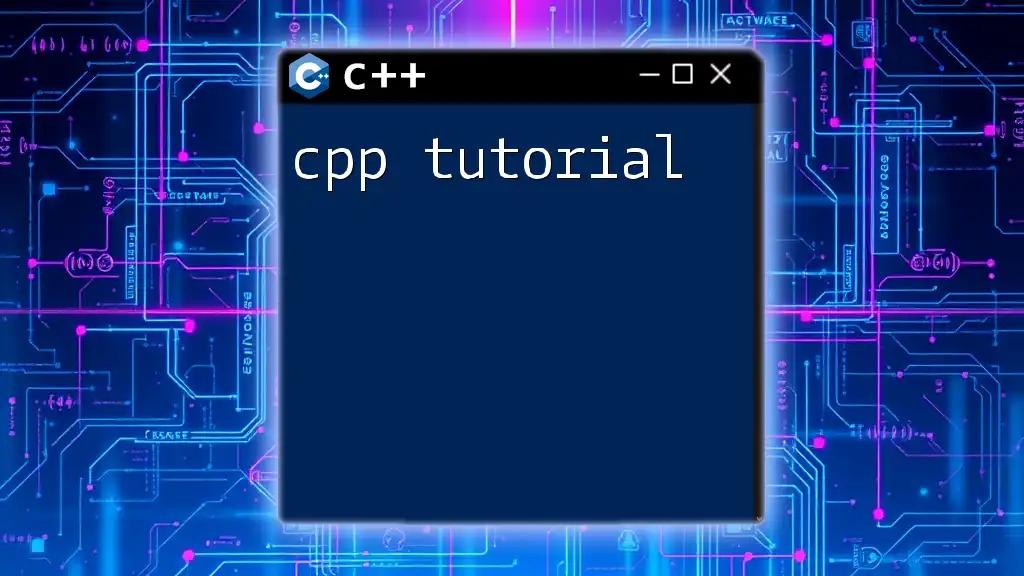
Writing Efficient C++ Tests
Best Practices for Testing in C++
Writing effective tests requires adherence to several best practices:
-
Code Readability and Maintainability: Write tests that are easy to understand. This not only aids in debugging but also helps new team members acclimate quickly.
-
Importance of Naming Conventions: Adopt clear and consistent naming conventions for test functions, classes, and variables to improve comprehensibility.
Common C++ Test Frameworks
Several frameworks simplify the testing process in C++.
-
Google Test: One of the most widely used libraries for C++ testing. It provides rich functionalities, including assertions and test fixtures.
Example of setting up a basic Google Test:
#include <gtest/gtest.h> TEST(SampleTest, AssertionTrue) { ASSERT_TRUE(true); }
-
Catch2: Known for its simple syntax and ease of integration. It requires no additional installations, allowing for quick setup.
Basic test example using Catch2:
#define CATCH_CONFIG_MAIN #include <catch.hpp> TEST_CASE("Basic operations") { CHECK(1 + 1 == 2); }
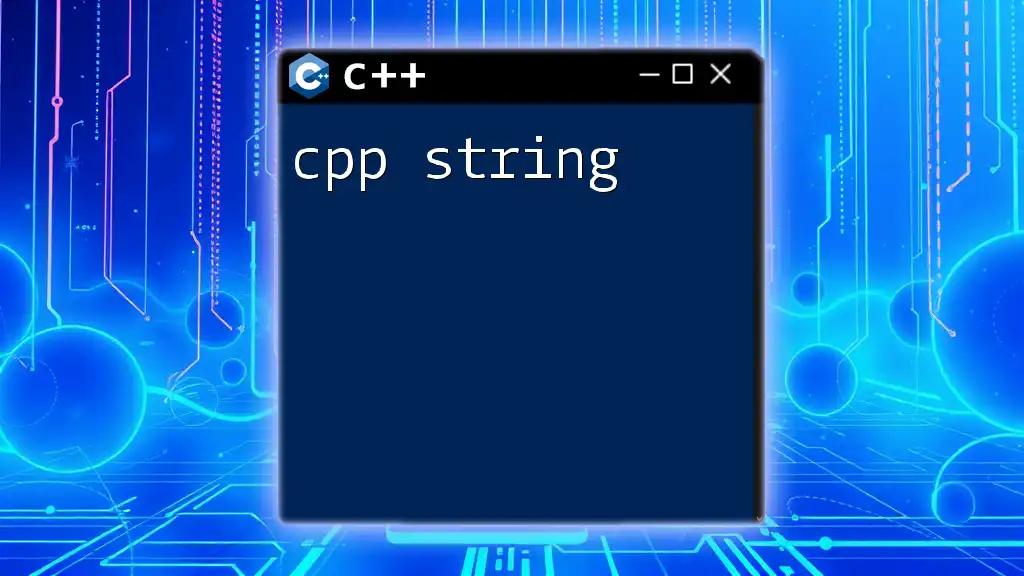
Evaluating and Analyzing Test Results
Interpreting C++ Test Outcomes
Once tests are executed, it's vital to analyze the results critically. Common outputs include pass, fail, and timeout, each indicating whether the code operates as expected. Analyzing the failure reasons is essential for effective improvements. Tools for measuring test coverage can provide insights into which parts of the codebase lack sufficient testing.
Debugging Failed Tests
Debugging is an unavoidable process when tests fail. Common pitfalls may include logical errors, syntax mistakes, or unexpected behavior from external libraries. Using debugging tools available in your IDE, such as breakpoints and watch expressions, can help isolate the problematic code.
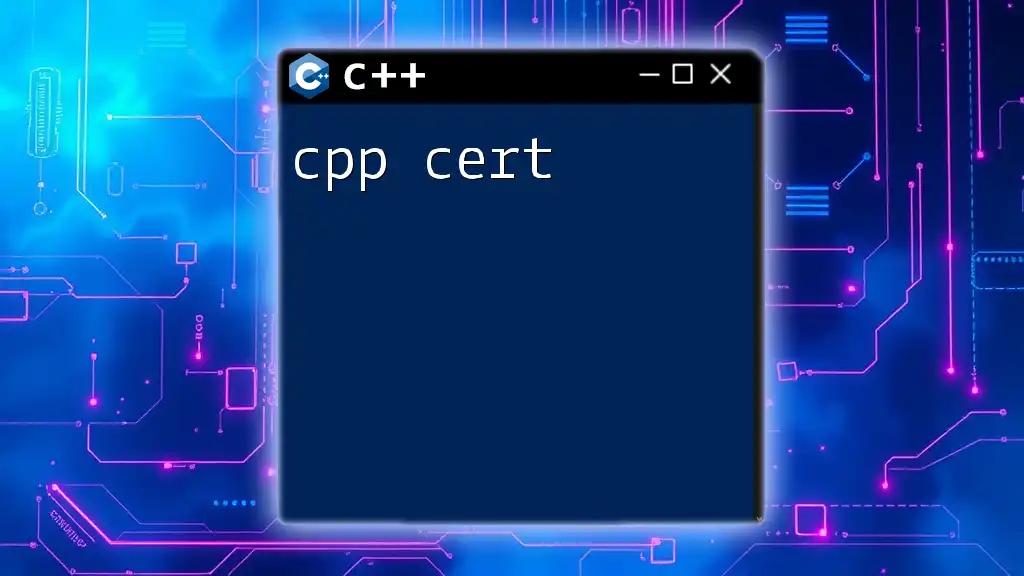
Real-World Applications of C++ Testing
Case Studies
Major tech companies leverage C++ testing to ensure quality and reliability in their software products. For instance, game development studios use rigorous testing protocols to verify that game mechanics function seamlessly, enhancing user experience.
Tips for Applying Testing in Your Projects
Incorporate a testing routine within your development workflow. Make a habit of performing tests after completing each feature or bug fix. Continuous testing helps in maintaining code quality and identifying issues earlier in the development cycle.
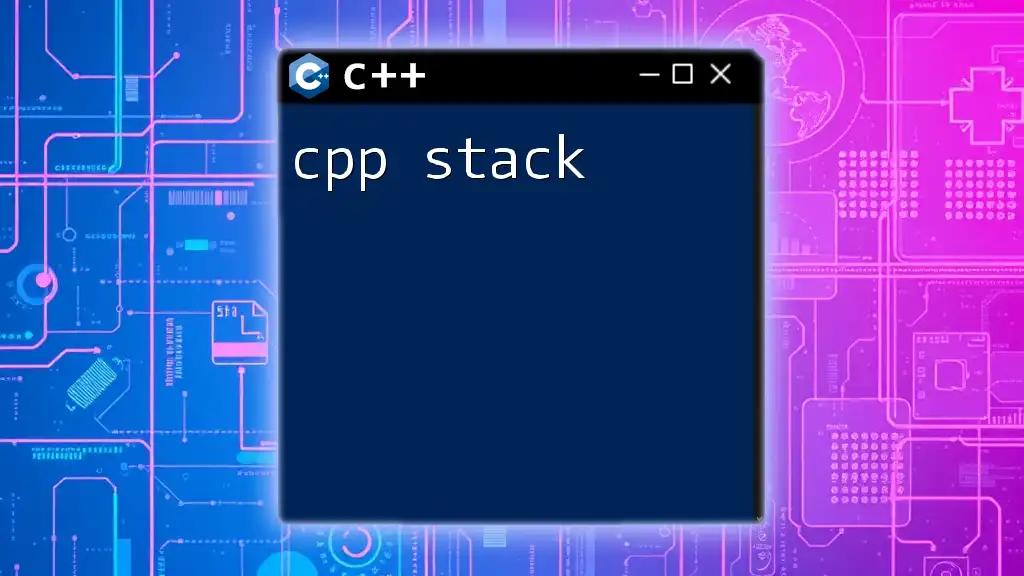
Preparing for Your C++ Exam
Strategies for Success
Develop a structured study plan that includes both theoretical learning and practical coding. Set aside time to review concepts and attempt practice exams. Group studies can also provide additional insights from peers.
Example Questions
Familiarize yourself with the types of questions likely to appear on a cpp exam. Sample questions might include:
- Explain the difference between unit testing and integration testing.
- Write a unit test for a function that computes the factorial of a number.
- Describe how to interpret the output from a failed test.

Conclusion
Understanding cpp tests is fundamental not only for passing exams but also for improving your software development skills. Consistent practice, application of best practices, and active participation in C++ communities can drastically enhance your ability to write reliable code and develop robust applications. Prepare diligently, and remember that testing is an ongoing journey in software development.