The `getenv` function in C++ retrieves the value of an environment variable, returning a pointer to a string if found or `nullptr` if not.
#include <cstdlib>
#include <iostream>
int main() {
const char* value = std::getenv("HOME");
if (value) {
std::cout << "HOME: " << value << std::endl;
} else {
std::cout << "HOME environment variable is not set." << std::endl;
}
return 0;
}
What is `getenv`?
The `getenv` function is a standard library function in C++ that retrieves the value of an environment variable. Environment variables are dynamic values that can affect the way running processes will behave on a computer. They can provide configuration data, user preferences, file paths, and more, and are especially useful in building portable applications.

Why Use `getenv` in Your C++ Program?
Using `getenv` is essential for developing applications that require flexibility and adaptability to different environments. The ability to access environment variables means that a program can adjust its behavior based on the environment in which it's running. For example, you can store sensitive information like API keys or database credentials in environment variables, allowing your application to remain secure and easily configurable.

What Are Environment Variables?
Environment variables are key-value pairs that are part of the environment in which a process runs. They can be set at various levels (system-wide, user-specific, etc.) and provide an accessible way to configure system settings.
For instance, some common environment variables include:
- PATH: This variable tells the system where to find executable files.
- HOME: Represents the path to the current user's home directory.
- USER: Contains the name of the current user.

How Environment Variables Work
Environment variables are stored in the operating system's environment and are accessible to any process running in that environment. When a program starts, it inherits a copy of the environment variables from its parent process. This way, the program can use `getenv` to fetch the values of these variables.
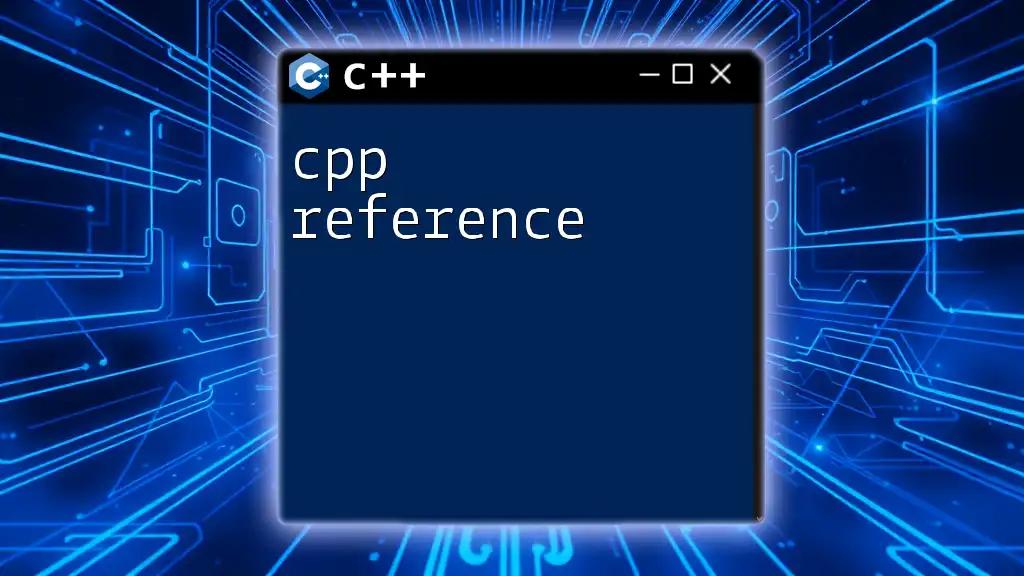
Function Prototype
The `getenv` function prototypes as follows:
char* getenv(const char* name);
Parameters and Return Value
-
Parameters: The function takes a single argument, `name`, which is a pointer to a string representing the name of the environment variable you want to access.
-
Return Value: If the specified environment variable exists, `getenv` returns a pointer to the value associated with that variable. If the variable does not exist, it returns a null pointer (`nullptr`).
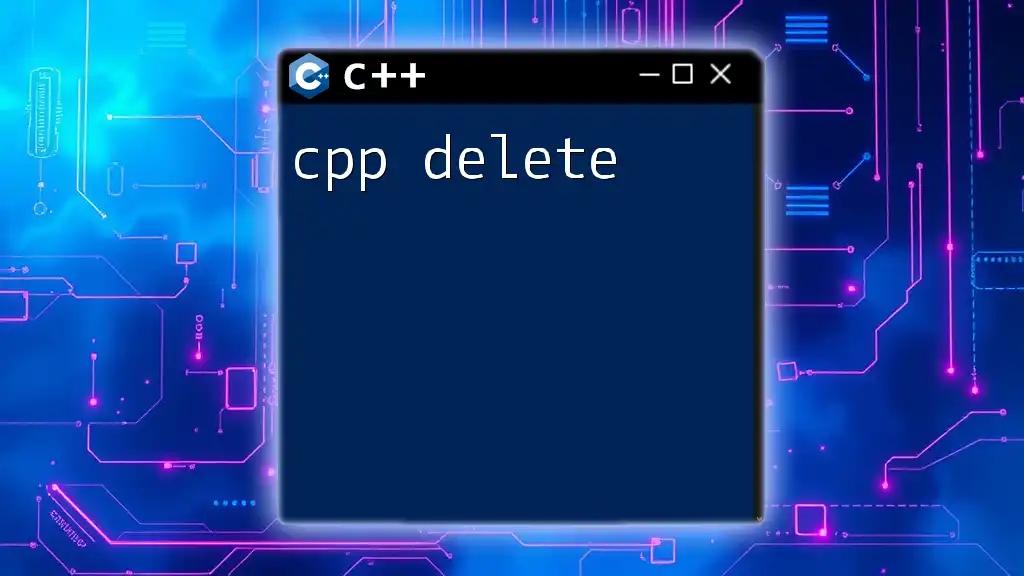
Basic Usage of `getenv`
To effectively use `getenv`, follow the basic pattern of calling the function and checking its return value. Here's a simple example:
#include <iostream>
#include <cstdlib>
int main() {
const char* path = getenv("PATH");
if (path) {
std::cout << "PATH: " << path << std::endl;
} else {
std::cout << "Environment variable 'PATH' not found." << std::endl;
}
return 0;
}
In this example, the program tries to access the `PATH` environment variable. If it exists, the program prints its value; otherwise, it displays a message indicating that the variable was not found.

Error Handling with `getenv`
Proper error handling is essential when using `getenv`. If you're trying to access an environment variable that may not be set, you should always check for a null return value. Here's an example of how to handle such situations:
const char* home = getenv("HOME");
if (!home) {
std::cerr << "HOME environment variable not set!" << std::endl;
}
This code checks if the `HOME` variable is available, gracefully informing the user if it is not. This is crucial for applications that depend on user-specific configurations.
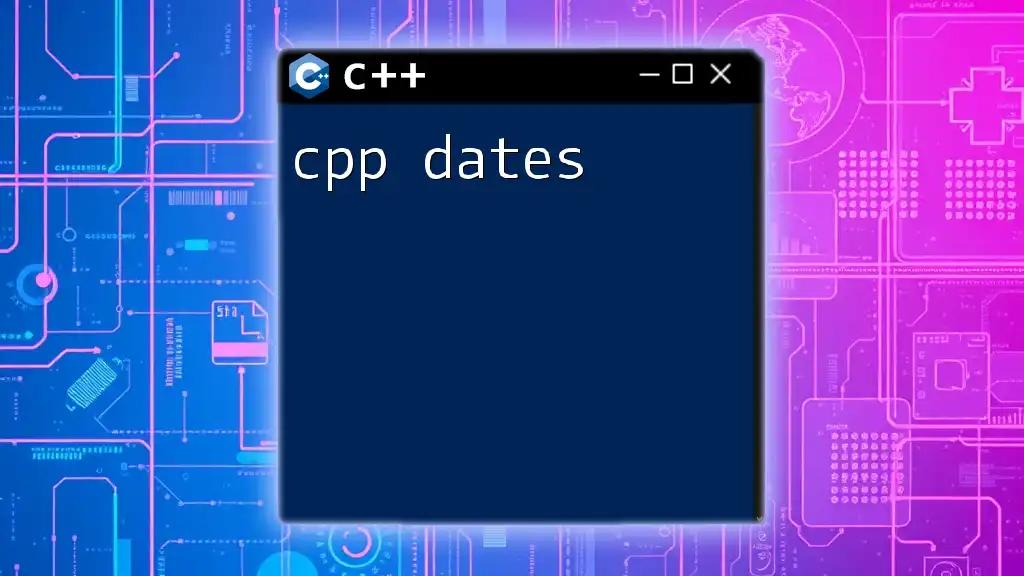
Using `getenv` with User-Defined Variables
While `getenv` usually accesses system and user environment variables, you can also use it to retrieve user-defined variables. This entails prior configuration of the respective variables in the operating system. The process of defining such variables varies by OS. For example, on Unix-like systems, you can define an environment variable in the shell with:
export MY_VAR="Hello, World!"
Then, you can access it in your C++ program:
const char* myVar = getenv("MY_VAR");
if (myVar) {
std::cout << "MY_VAR: " << myVar << std::endl;
}
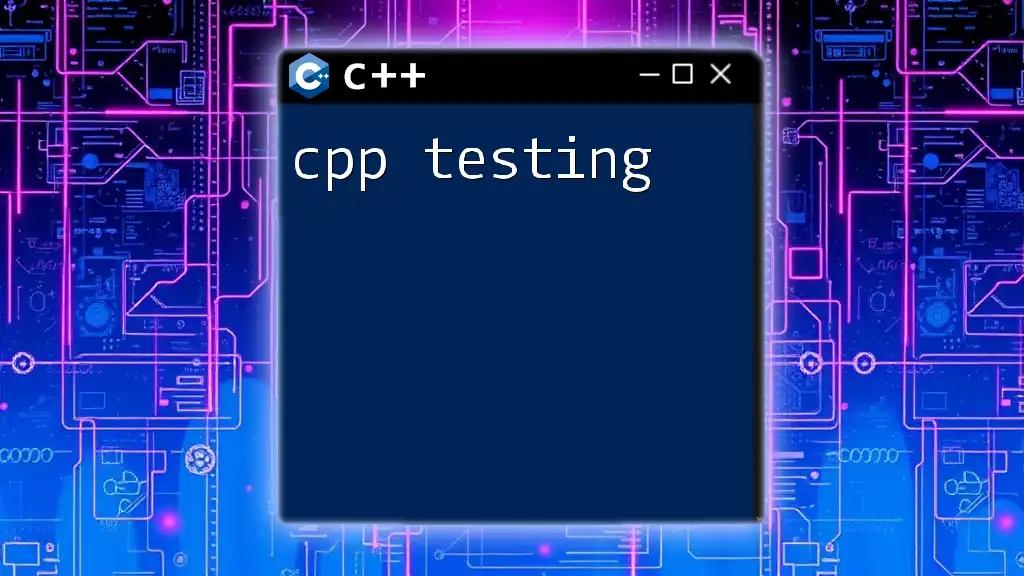
The Importance of `const char*` Type
The return type of `getenv` is `char*`, specifically, `const char*`, which means the returned string should not be modified. This distinction is critical for string safety, preventing accidental alterations to string literals stored in memory. By keeping the integrity of the data intact, you minimize the risk of bugs and stability issues in your applications.

Cross-Platform Behavior of `getenv`
It's important to note that the behavior of `getenv` can vary across operating systems. For example, while Unix-like systems treat environment variable names in a case-sensitive manner (e.g., `HOME` is different from `home`), Windows does not. Consequently, developers must remain aware of these platform-specific variations to ensure consistent behavior across different environments.
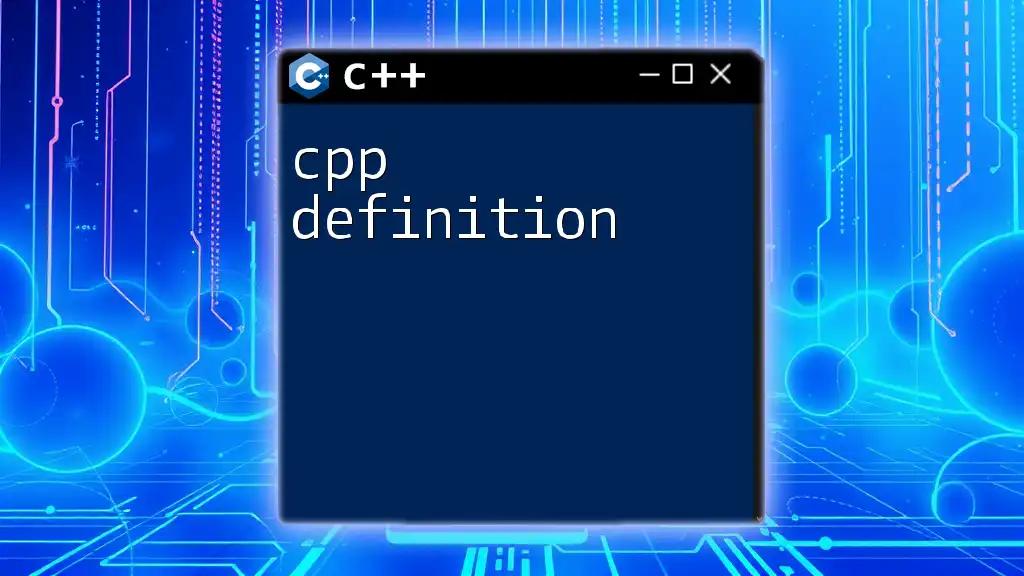
Configuration Management in C++ Applications
Environment variables are particularly useful for configuration management in applications. Storing sensitive data such as database credentials or API keys as environment variables allows you to keep such information private and secure rather than hardcoding them into the source code. For example, you may extract an API key during runtime using:
const char* apiKey = getenv("API_KEY");
In your deployment, you can configure the API key as an environment variable, enhancing both security and flexibility.
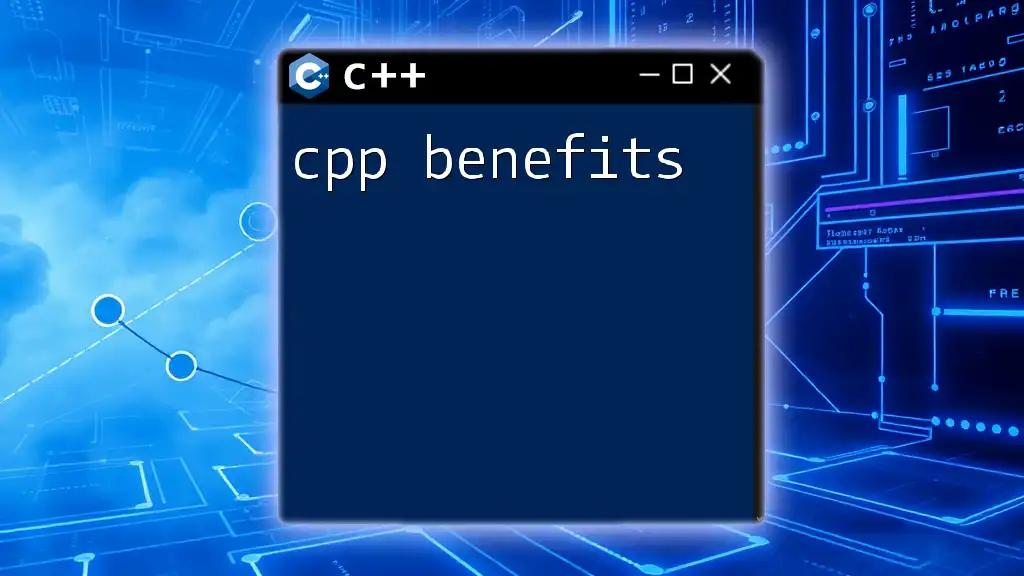
Enhancing Portability of Applications
By using `getenv`, applications become inherently more portable. They can run seamlessly across different environments without needing code changes, provided the necessary environment variables are set. This practice is vital for maintaining application consistency during development, testing, and production stages.

Avoiding Common Mistakes
A common mistake when working with `getenv` is assuming that an environment variable exists. Many developers forget to check if the returned pointer is null. Always implement checks to verify the existence of the variable you are trying to access.
Best Practices for Environment Variables
Additionally, consider the following best practices:
- Security: Avoid exposing sensitive information through environment variables, especially in environments where they may be logged or displayed.
- Naming Conventions: Use clear and consistent naming conventions for environment variables to ensure maintainability and clarity.

Recap of `getenv` Usage in C++
In summary, `getenv` provides a valuable way for C++ applications to access environment variables. By understanding its usage, error handling, and implications in application design, developers can leverage `getenv` to create flexible, portable, and secure applications.

Final Thoughts
Exploring the power of environment variables and their management through `getenv` can significantly enhance your programming toolkit. Consider incorporating this practice into your projects to build adaptable and efficient applications.
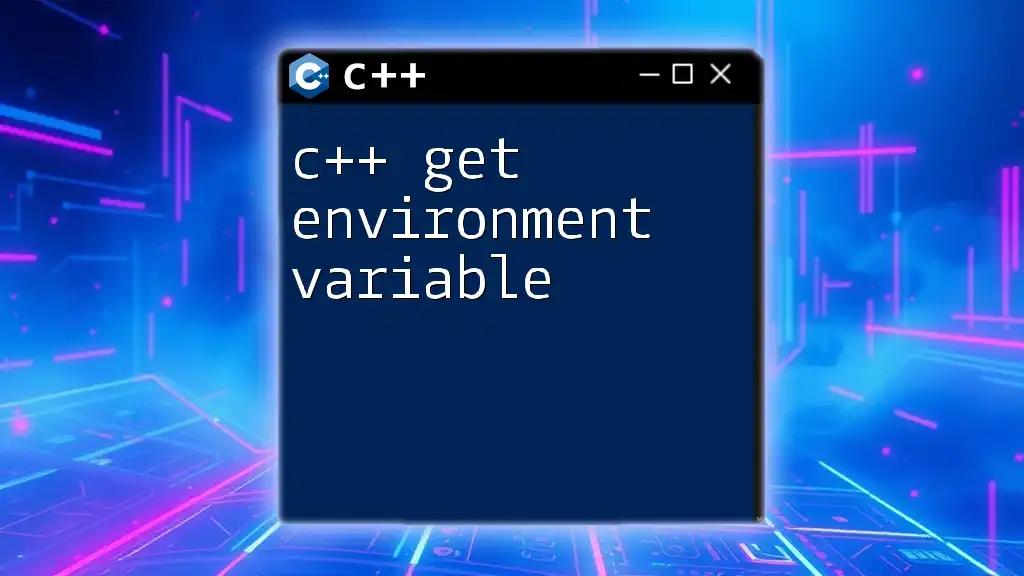
Additional Resources
For those looking to delve deeper, refer to the official C++ documentation and explore related topics to gain a comprehensive understanding of effective C++ programming techniques.