The `setenv` command in C++ is used to set the value of an environment variable within the program's execution context.
#include <cstdlib>
int main() {
setenv("MY_VARIABLE", "my_value", 1);
return 0;
}
Understanding Environment Variables
What are Environment Variables?
Environment variables are dynamic values that can affect the behavior of processes on a computer. They serve as a way to pass configuration information to running applications. For instance, they might contain details about the system's operating environment, such as the user’s home directory, the location of executables, or system-wide variables critical for configuration.
Common Use Cases for Environment Variables
-
Configuration settings: Many applications use environment variables to store configuration details. This allows users to customize the behavior of the application without modifying the code.
-
System path variables: Environment variables often include paths to executable files. The PATH variable, for example, defines where the system looks for executable files, enabling easier command execution.
-
User preferences: User-specific environment variables can hold preferences that alter how applications behave for individual users.
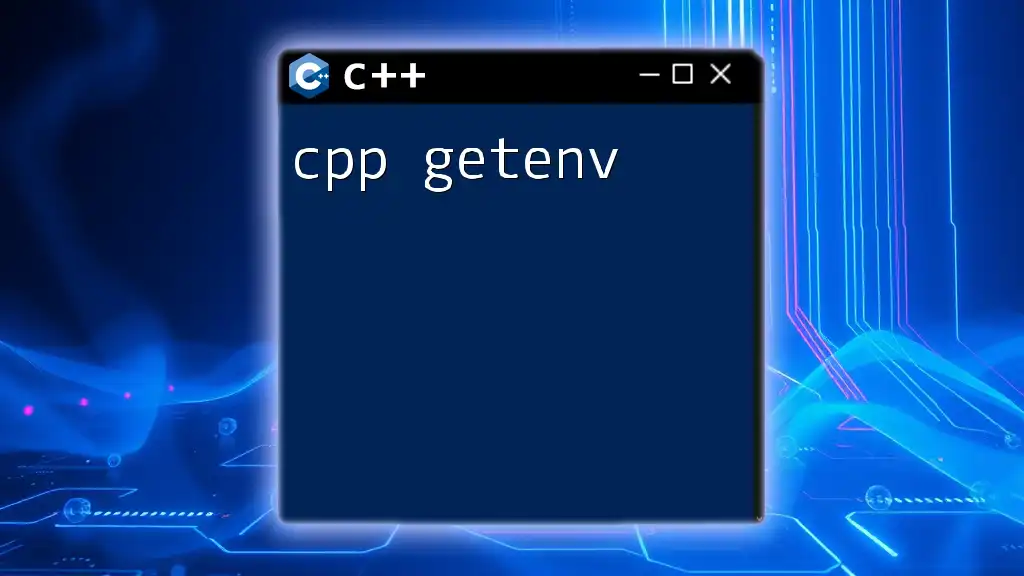
The `setenv` Function
What is `setenv`?
In C++, the `setenv` function allows you to create or modify an environment variable within your program. When you use `setenv`, you're effectively saying, "I want this variable to be available in the environment for this application and potentially for child processes."
Syntax of `setenv`
The syntax for the `setenv` function is as follows:
int setenv(const char *name, const char *value, int overwrite);
Parameters Explained
- name: This is a pointer to the name of the environment variable you want to set. It must be a string.
- value: This is a pointer to the string value you want to assign to the environment variable.
- overwrite: An integer flag that indicates whether to overwrite an existing variable:
- `0`: Do not overwrite if the variable already exists.
- `1`: Overwrite it if it exists.
Return Value
The function returns `0` on success. If the operation fails, it returns `-1`, indicating an error occurred while attempting to set the environment variable.
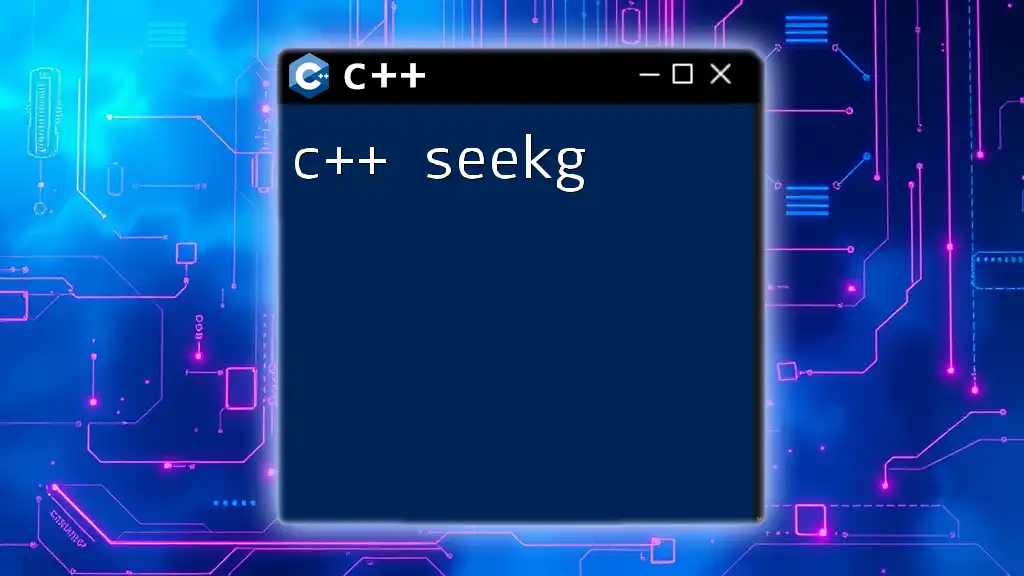
Including Necessary Header Files
To use the `setenv` function, you need to include the appropriate header file. In this case, you should include:
#include <cstdlib>
Including this header is essential as it declares the necessary functions for manipulating environment variables. Without it, you may encounter compilation errors.
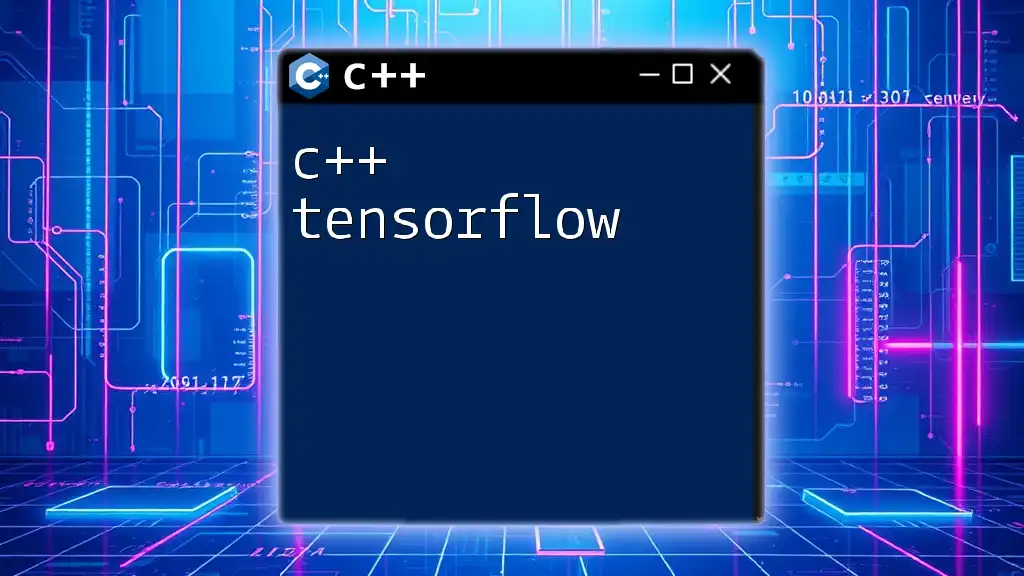
Example Usage of `setenv`
Basic Example
Here's a simple example that demonstrates setting and retrieving an environment variable using `setenv`:
#include <cstdlib>
#include <iostream>
int main() {
// Set an environment variable
setenv("MY_VAR", "Hello World", 1);
// Retrieve and print the variable
std::cout << "MY_VAR: " << getenv("MY_VAR") << std::endl;
return 0;
}
In this code:
- We set an environment variable named `MY_VAR` with the value `"Hello World"`.
- We then retrieve the value of `MY_VAR` using `getenv`, which fetches the value of the specified environment variable, and print it out.
Setting Multiple Environment Variables
You can easily set multiple environment variables in a single program:
#include <cstdlib>
#include <iostream>
int main() {
setenv("VAR1", "Value1", 1);
setenv("VAR2", "Value2", 1);
std::cout << "VAR1: " << getenv("VAR1") << std::endl;
std::cout << "VAR2: " << getenv("VAR2") << std::endl;
return 0;
}
In this example, we created two environment variables: `VAR1` and `VAR2`. After setting them, we retrieve and print their values to confirm they are set correctly.
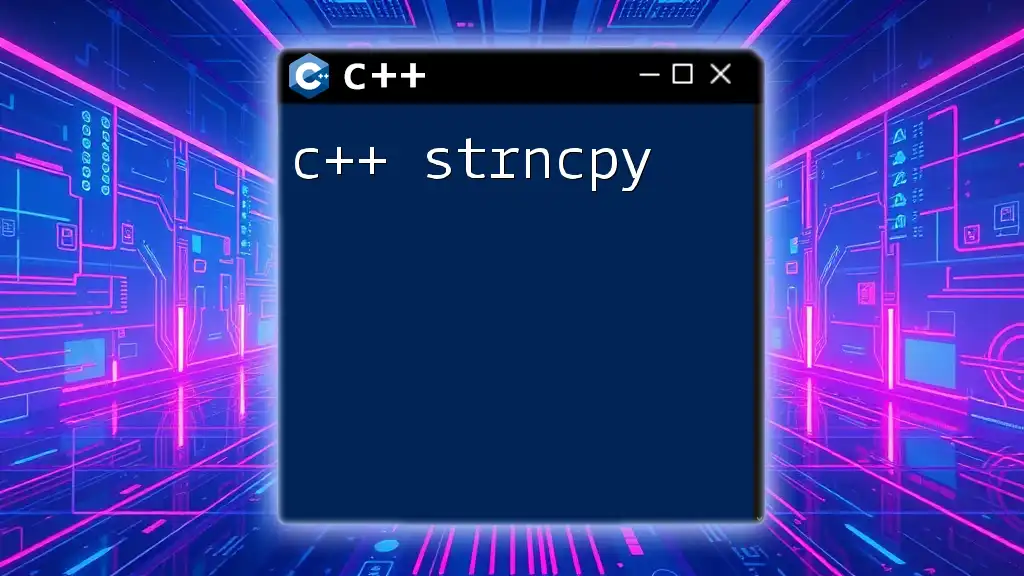
Overwriting Existing Environment Variables
Example with Overwrite
The `setenv` function is powerful because it allows you to overwrite existing environment variables if necessary. Here’s how:
#include <cstdlib>
#include <iostream>
int main() {
setenv("MY_VAR", "Initial Value", 1);
std::cout << "Before Overwrite, MY_VAR: " << getenv("MY_VAR") << std::endl;
// Overwriting MY_VAR
setenv("MY_VAR", "New Value", 1);
std::cout << "After Overwrite, MY_VAR: " << getenv("MY_VAR") << std::endl;
return 0;
}
In this snippet:
- We first set `MY_VAR` to "Initial Value", then print it.
- Next, we overwrite `MY_VAR` with "New Value" and print it again. This illustrates how setting the overwrite flag allows us to change the value of an existing environment variable.
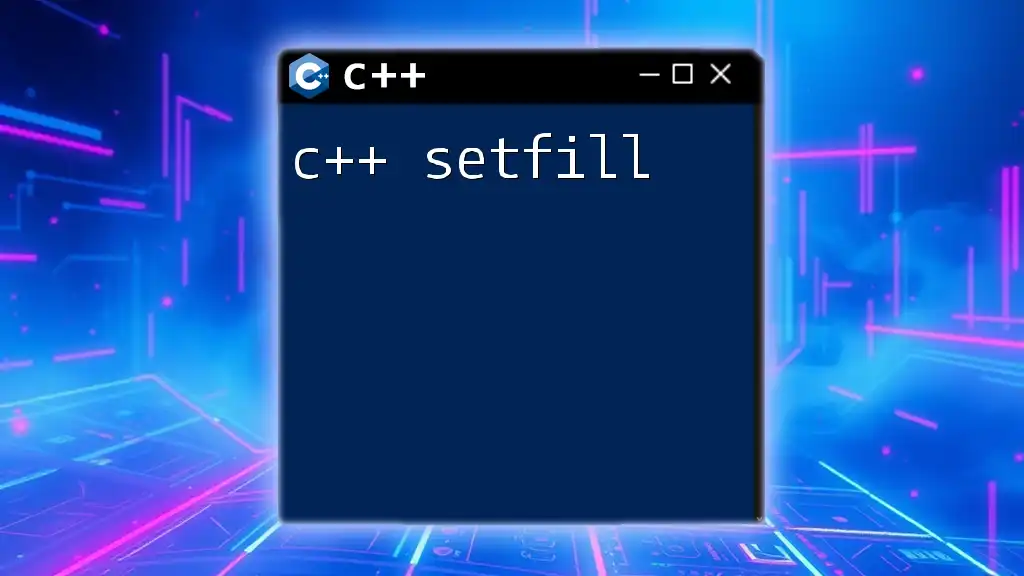
Error Handling with `setenv`
Common Errors and Their Causes
When using `setenv`, it's crucial to handle potential errors. Common issues that may arise include:
- Invalid parameter values where `name` or `value` are `NULL`.
- Resource limitations that prevent the creation of new variables.
Example of Error Checking
Incorporating error checking into your code helps ensure reliability:
#include <cstdlib>
#include <iostream>
int main() {
if (setenv("MY_VAR", "Some Value", 1) != 0) {
perror("setenv failed");
}
return 0;
}
In this snippet, we call `setenv` and immediately check the return value. If it’s not `0`, indicating an error, we use `perror` to print a descriptive error message. This practice improves software robustness by identifying issues promptly.
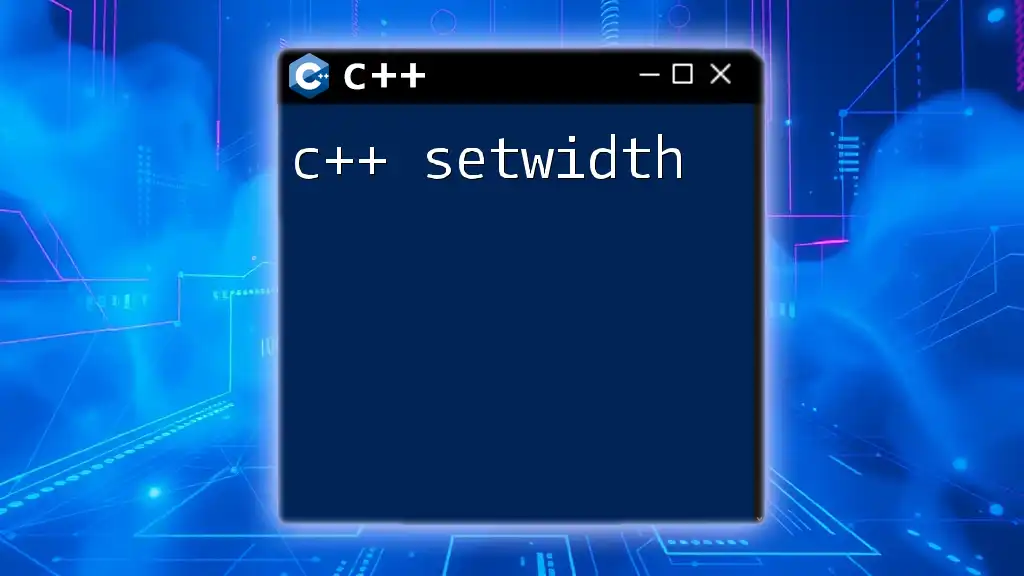
Using `getenv` with `setenv`
Interplay Between `getenv` and `setenv`
The `getenv` function is used to retrieve the values of environment variables set by `setenv`. Understanding this interplay is essential for managing environment state effectively within your application.
Example of Using `getenv`
#include <cstdlib>
#include <iostream>
int main() {
setenv("MY_VAR", "Our Value", 1);
const char* value = getenv("MY_VAR");
if (value) {
std::cout << "The value of MY_VAR is: " << value << std::endl;
} else {
std::cout << "MY_VAR is not set." << std::endl;
}
return 0;
}
In this code:
- We set an environment variable `MY_VAR`, then retrieve its value using `getenv`.
- A check is performed to verify if the retrieval is successful. This helps ensure your program handles scenarios where the variable might not exist.
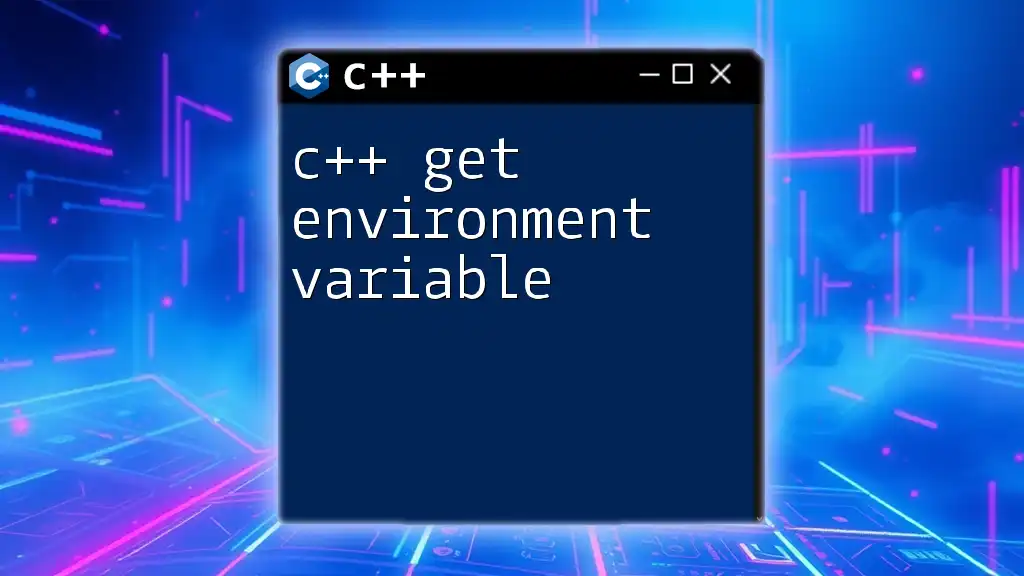
Best Practices for Using `setenv`
Tips for Effective Use
- Keep variable names consistent and meaningful: Using clear and descriptive names reduces confusion and improves code readability.
- Avoid exposing sensitive information in environment variables: Be cautious as environment variables can often be accessed by other processes. Avoid storing sensitive data.
When Not to Use `setenv`
While `setenv` is powerful, it's not always the best solution. For instance, if you need to pass configuration options that don't require runtime changes to environment variables, consider using config files or command-line arguments instead.
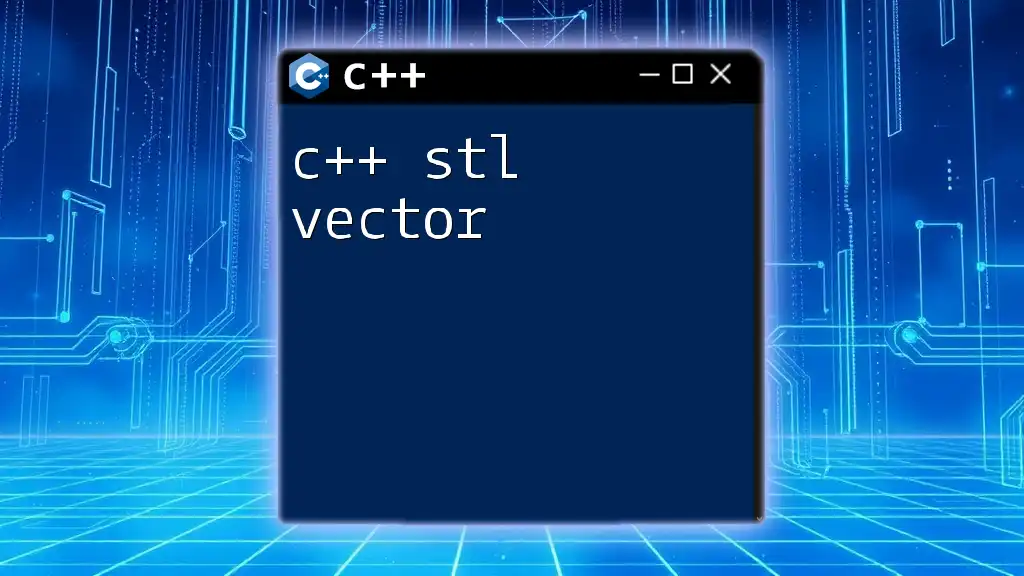
Conclusion
Understanding how to use `setenv` in C++ expands your ability to manage environment variables effectively. This function is vital for configuring operating environments dynamically, and mastering it can enhance your programming toolkit.
Explore the provided code examples to see `setenv` in action, and consider how environment variables could improve your applications' flexibility and configuration. Practice with these examples and consult additional resources to deepen your knowledge of `c++ setenv`.
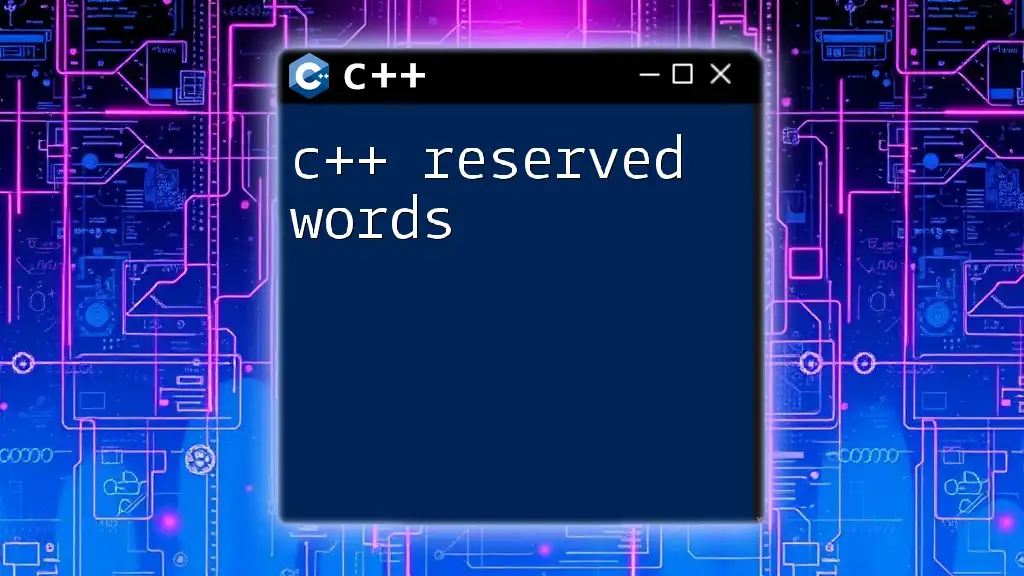
Additional Resources
For further learning, consider exploring the official C++ documentation on environment variables, along with recommended programming books and tutorials that focus on effective practices in using environment variables within C++.