The `std::vector` is a dynamic array in C++ that can resize itself automatically as elements are added or removed, providing a convenient way to manage collections of data.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adds an element to the end of the vector
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
What is std::vector?
The `std::vector` is a powerful sequence container in C++ that encapsulates dynamic arrays. It allows developers to work with collections of data that can be resized automatically when elements are added or removed. This functionality makes `std::vector` extremely valuable in programming tasks that require flexibility and ease of use.
A few common use cases for `std::vector` include managing lists of items, implementing dynamic data structures, and handling variable-length data collections.
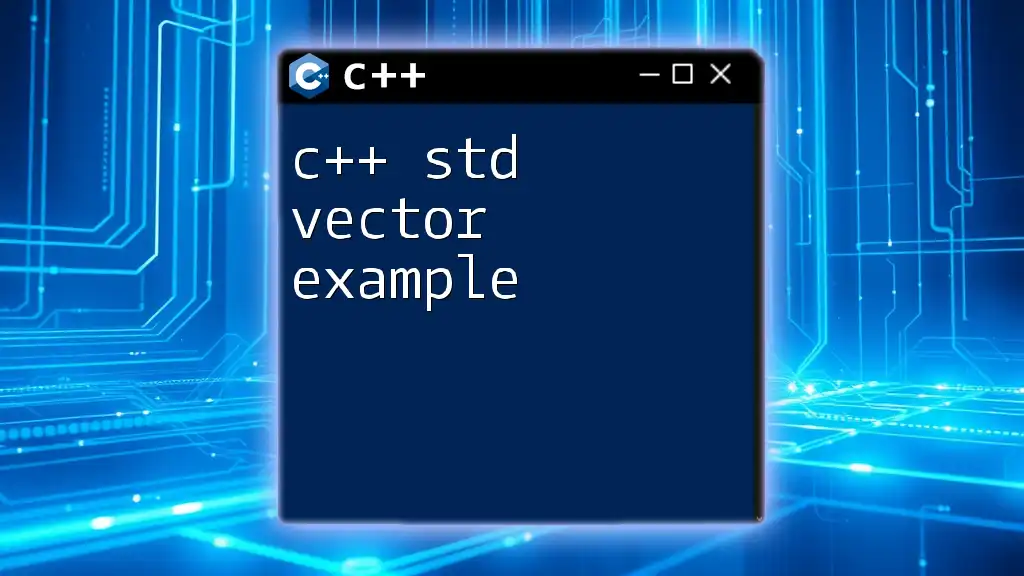
Advantages of Using std::vector
There are several significant advantages to using `std::vector`. First, its dynamic sizing capability means that you don't have to worry about allocating a fixed size upfront. As you add or remove elements, the vector will automatically resize itself to accommodate these changes, making coding cleaner and more efficient.
Ease of use is another compelling factor. With a variety of built-in functions, operating on vectors (such as adding, accessing, and removing elements) is straightforward, which can significantly reduce the amount of boilerplate code needed compared to traditional arrays.
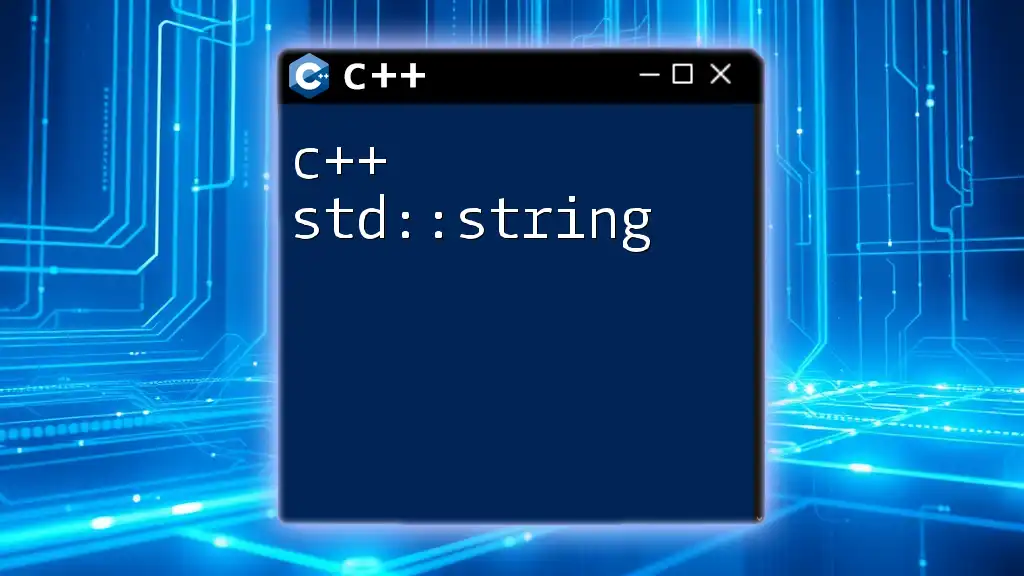
Including the Necessary Header
To use `std::vector`, you need to include the following header in your C++ code:
#include <vector>
This inclusion provides access to the full functionality of the vector container.
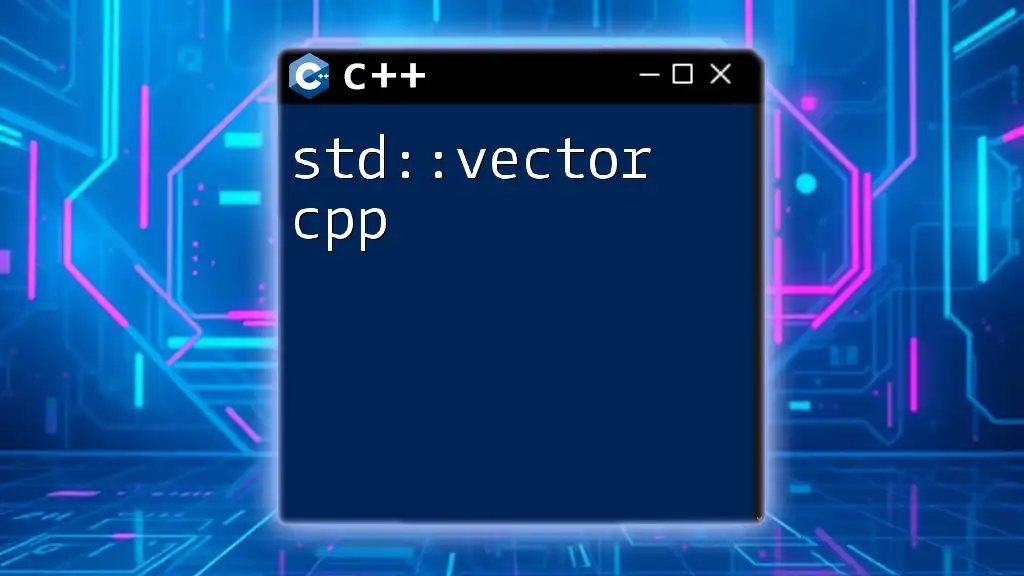
Creating a std::vector
Creating a `std::vector` is simple. You can declare an empty vector like this:
std::vector<int> numbers;
This creates an empty vector of integers. You can also initialize a vector with predefined values or specific sizes.
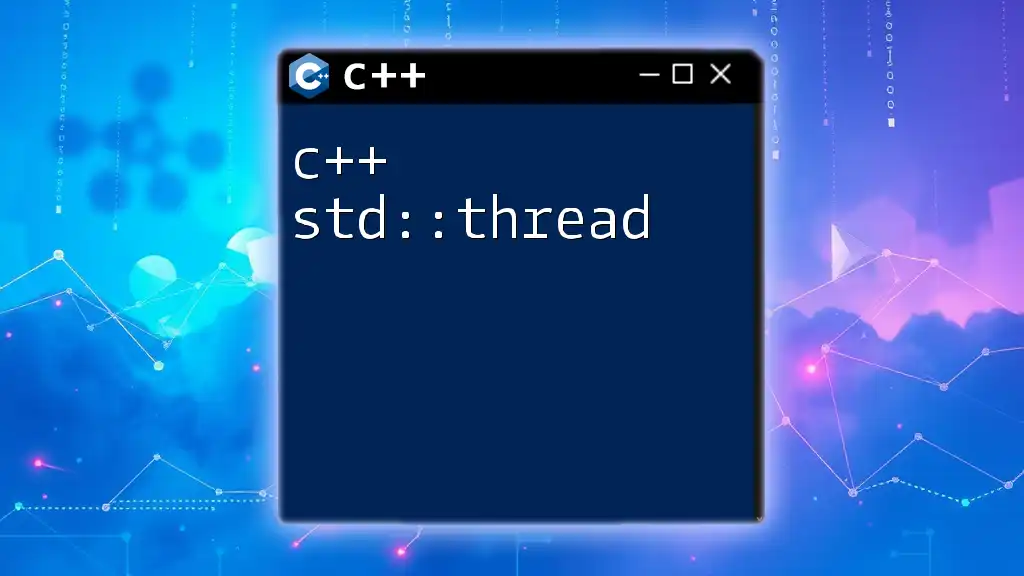
Initializing a std::vector
There are multiple ways to initialize a `std::vector`:
-
Using initializer lists: You can initialize a vector with a comma-separated list of values:
std::vector<int> initializedVector = {1, 2, 3, 4, 5};
-
Specifying size and default values: You can also specify a size and a value to initialize all elements:
std::vector<int> sizedVector(5, 10); // Creates a vector of size 5, all initialized to 10
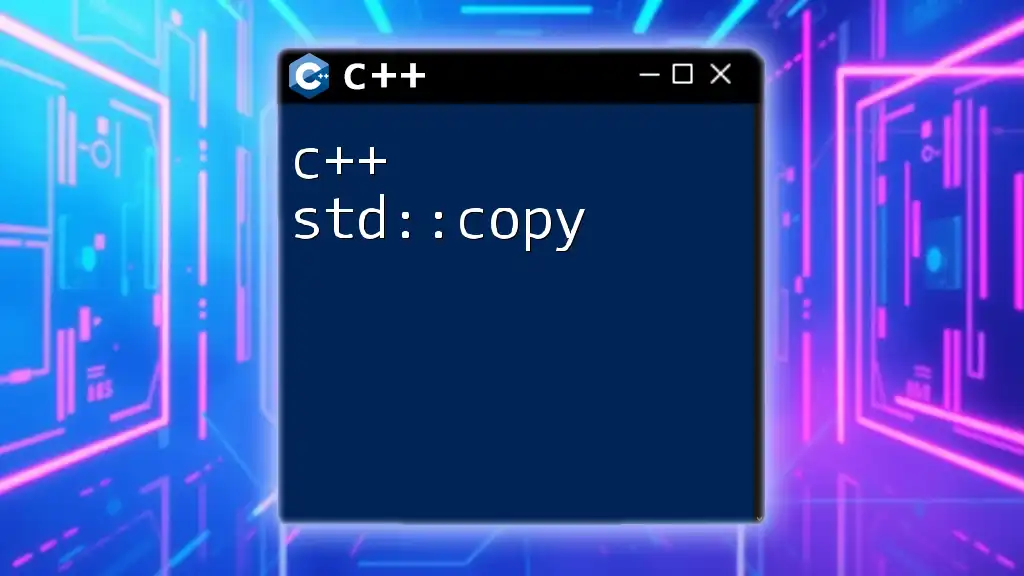
Commonly Used std::vector Member Functions
Understanding the available member functions is crucial for efficiently utilizing `std::vector`.
Adding Elements
-
push_back(): This function adds an element to the end of a vector.
numbers.push_back(10);
-
insert(): To add an element at a specific position, use the `insert()` function. This can be done as follows:
numbers.insert(numbers.begin() + 1, 20); // Inserts 20 at index 1
Accessing Elements
Accessing elements can be achieved using two main methods:
-
at(): This function provides bounds checking, ensuring that you don't access an out-of-bounds element:
int value = numbers.at(0); // Retrieves the first element
-
operator[]: This operates without bounds checking and should be used when you're certain of valid indexing:
int value = numbers[0];
Modifying Elements
You can easily modify the contents of a `std::vector` by assigning new values to its elements using the subscript operator:
numbers[0] = 15; // Changes the first element to 15
Removing Elements
To manage the contents of a vector, you can remove elements using these methods:
-
pop_back(): This will remove the last element in the vector:
numbers.pop_back(); // Removes the last element
-
erase(): To remove an element at a specific position, use `erase()`:
numbers.erase(numbers.begin() + 1); // Removes the element at index 1
Clearing the Vector
You can remove all elements from a vector with the `clear()` function, which effectively empties it:
numbers.clear(); // Empties the vector
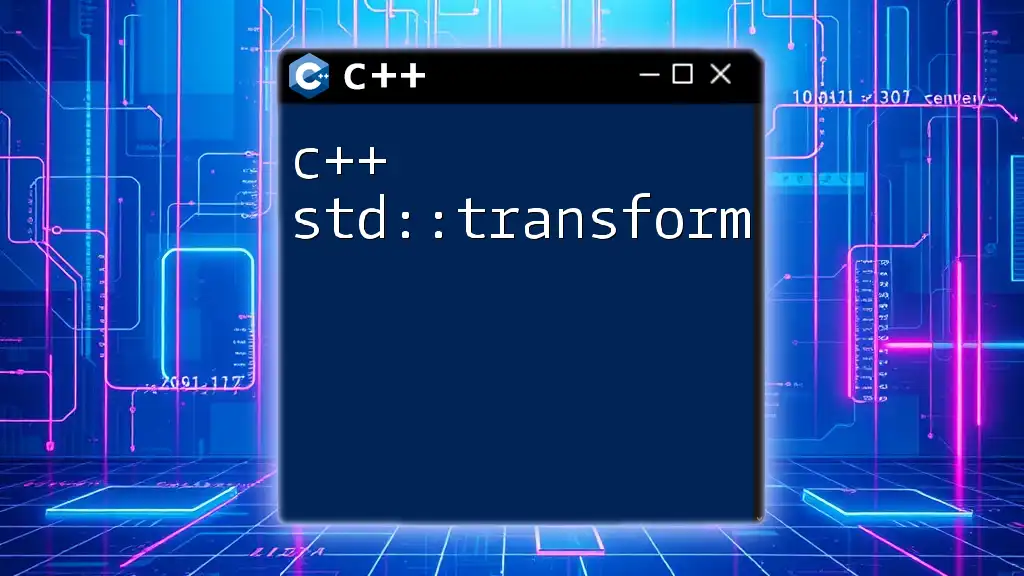
Iterating Over std::vector
Iterating over a vector can be performed in multiple ways, with two of the most common methods being range-based for loops and iterators.
Using Range-based for Loop
This modern C++ feature simplifies iteration, allowing you to write clean code:
for (const auto& number : numbers) {
std::cout << number << " ";
}
Using Iterator
Iterators provide a more granular approach to traversing elements:
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
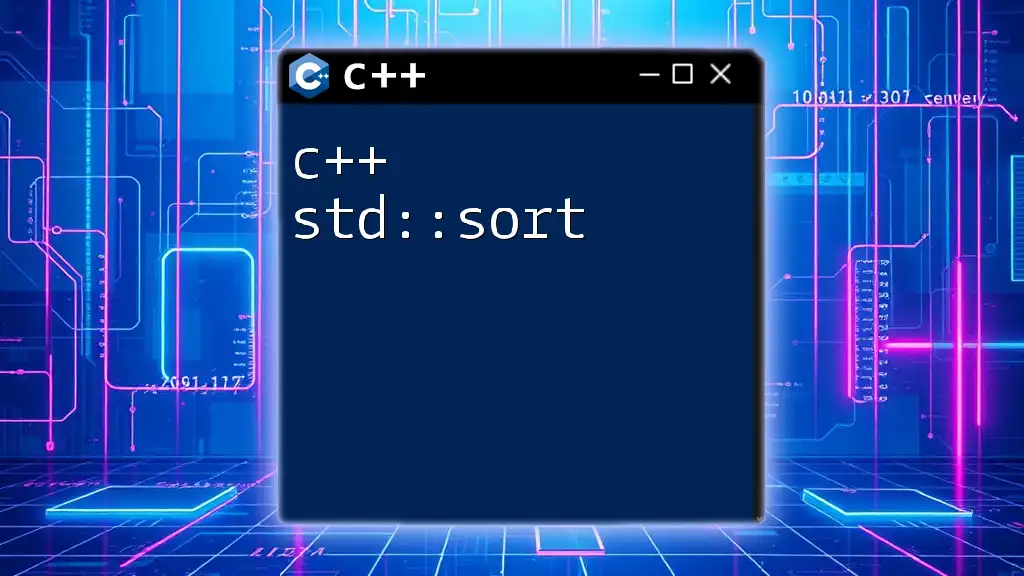
Advanced Topics
Resizing a std::vector
You can change the size of a vector at any time using the `resize()` method:
numbers.resize(10); // Resizes the vector to contain 10 elements
This adds default-initialized elements if the new size is larger than the current one or truncates the vector if smaller.
Capacity and Size
It's essential to understand the difference between size and capacity. The size refers to the number of elements in the vector, while capacity is the amount of storage space allocated for the vector. You can access both with:
std::cout << "Size: " << numbers.size() << "\n";
std::cout << "Capacity: " << numbers.capacity() << "\n";
The Importance of std::vector when Managing Resources
Using `std::vector` helps manage resources effectively, further reinforcing its suitability for various applications. However, careful usage is crucial in performance-sensitive situations, particularly concerning memory usage and sizing.
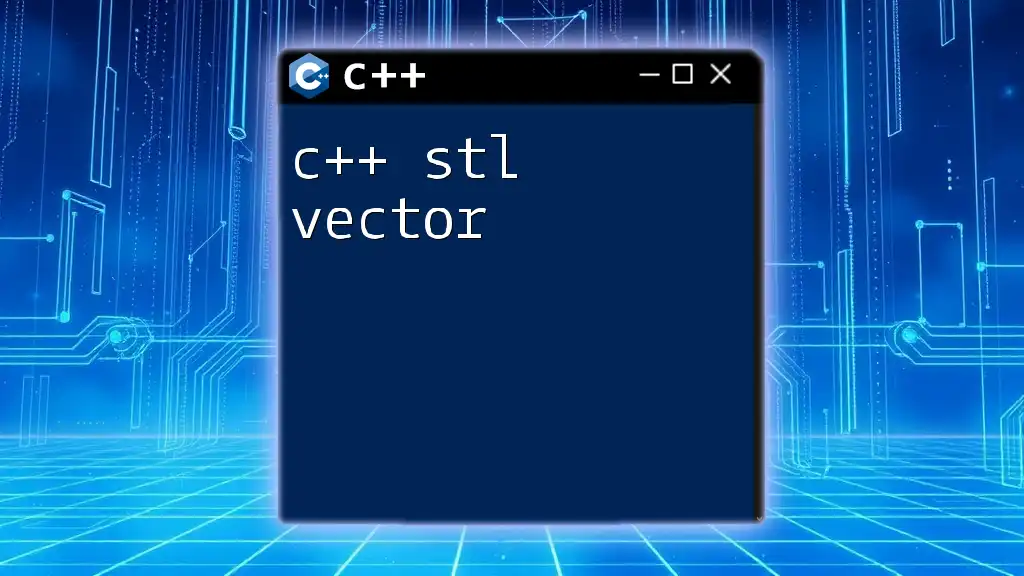
Best Practices for Using std::vector
When to Use std::vector
Consider using `std::vector` when you need a dynamic array that can grow or shrink while providing quick access to elements. For situations requiring frequent insertions and deletions at random positions, other data structures, like `std::list`, might be more efficient.
Performance Considerations
When working with larger datasets, it is prudent to reserve capacity upfront to avoid the overhead of multiple reallocations. This is particularly important in performance-critical applications where speed and memory usage are compounded.
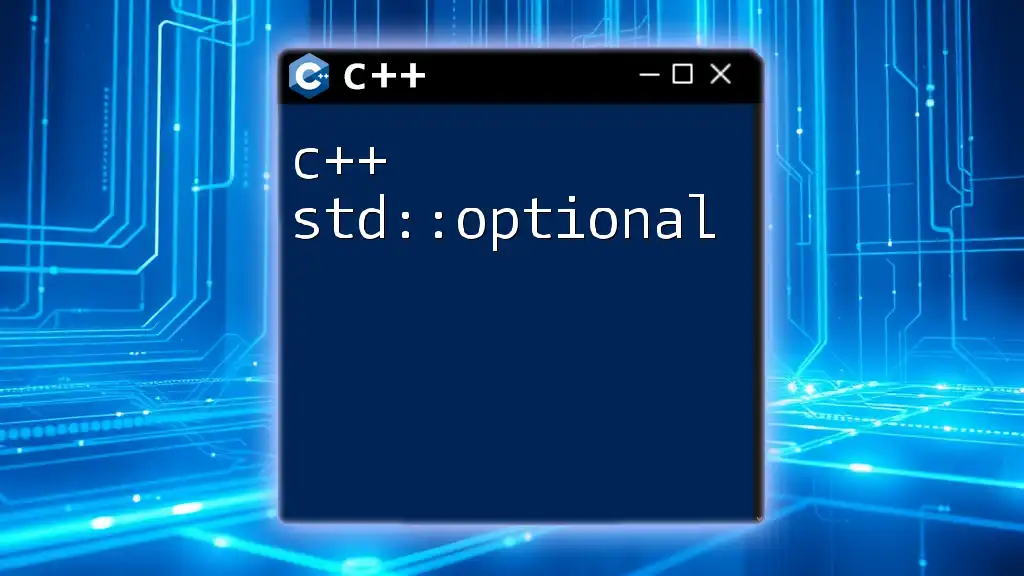
Recap of std::vector Benefits
In conclusion, `std::vector` is a powerful and flexible tool in C++. Its dynamic sizing, ease of operations, and availability of multiple member functions make it indispensable in modern C++ programming.
Encouragement to Practice
Success with `std::vector` comes with practice and exploration. Engage with coding exercises and additional resources to deepen your understanding and mastery of this critical C++ feature.
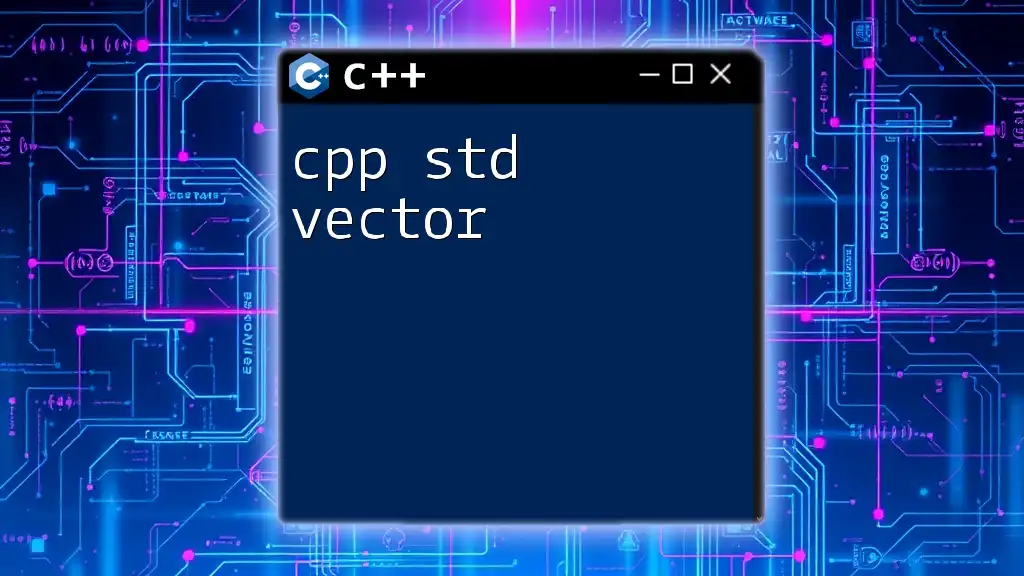
Additional Resources
- For further reading, you can explore the official C++ reference documentation at cppreference.com.
- Consider checking out recommended books or online tutorials that delve deeper into C++ and its standard library, ensuring you gain a well-rounded knowledge base.