The C++ `std::vector` is a dynamic array that can resize itself automatically when elements are added or removed, providing flexibility and ease of use for managing collections of data.
Here's a code snippet demonstrating the usage of `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Initialize a vector with elements
numbers.push_back(6); // Add an element to the end of the vector
for (int num : numbers) { // Iterate through the vector
std::cout << num << " ";
}
return 0;
}
What is `std::vector`?
`std::vector` is a part of the C++ Standard Template Library (STL) and represents a dynamic array that can change size during runtime. Unlike traditional arrays, which are of fixed size, `std::vector` automatically manages memory allocation and deallocation, allowing for more flexibility in code. When using `std::vector`, programmers benefit from vigorous functionalities such as quick append operations, seamless access to elements via indices, and built-in methods for manipulating the data.
Advantages of using `std::vector` include:
- Automatic Memory Management: You don't have to manually allocate or release memory.
- Dynamic Resizing: You can easily increase or decrease the size of the vector as needed.
- Versatile Access: Elements can be accessed using indices, and it provides various methods for element manipulation.
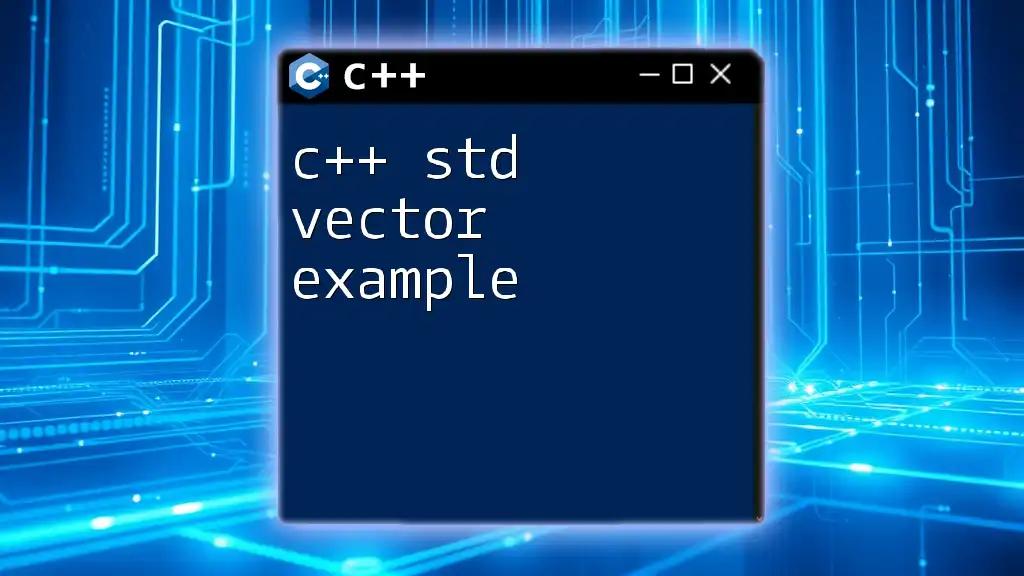
Getting Started with `std::vector`
Including the Vector Header
To use `std::vector`, you must include its header in your C++ program:
#include <vector>
This inclusion is essential because it allows access to all the functionalities available within the `std::vector` class.
Basic Syntax for Creating a Vector
Declaring and initializing a `std::vector` is straightforward. Here's how you can do it:
std::vector<int> myVector = {1, 2, 3, 4, 5};
In this example, `myVector` is a vector of integers initialized with five elements. The `std::vector` template can also accommodate other data types, including strings and user-defined types.
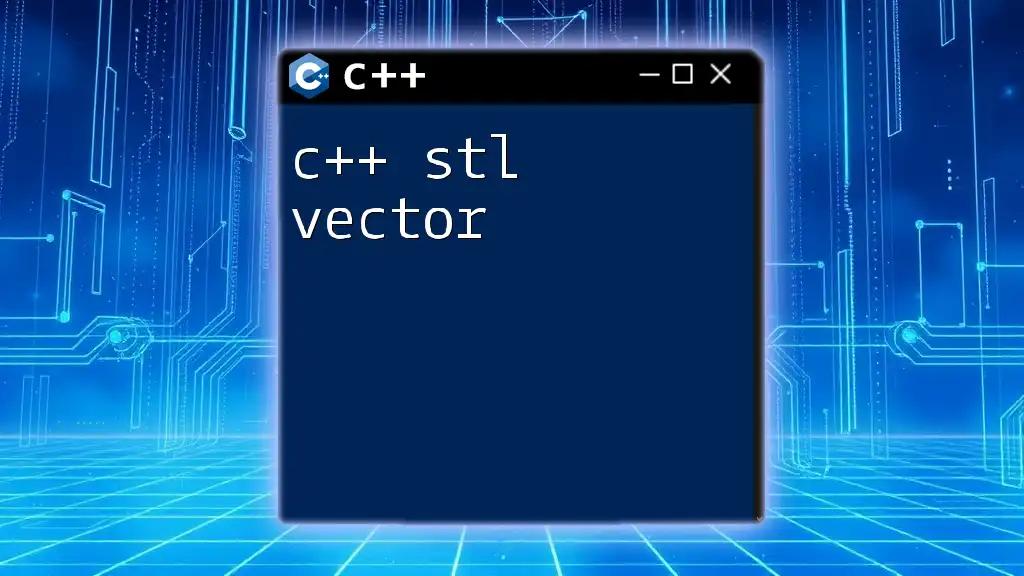
Key Features of `std::vector`
Dynamic Sizing
One of the hallmark features of `std::vector` is its ability to resize dynamically. When you add more elements than the allocated capacity, the vector automatically increases its size to accommodate them, reallocating memory as necessary. This behavior simplifies the management of collections of data.
Memory Management
With `std::vector`, you don’t need to worry about memory leaks or manual allocation. The internal memory management is taken care of, and when elements are added or removed, `std::vector` performs the necessary adjustments behind the scenes.
Element Access Methods
Accessing elements in a vector can be done using either the index operator (`[]`) or the `.at()` method.
-
Using `operator[]`: This method directly accesses the element at the specified index without bounds checking, potentially leading to undefined behavior if the index is out of range.
int firstElement = myVector[0]; // Using operator[]
-
Using `.at()` method: This method provides bounds checking and throws an exception if the index is out of range, ensuring safer coding practices.
int secondElement = myVector.at(1); // Using .at() method

Modifying Vectors
Adding Elements
Adding elements to a vector can be done easily using methods like `push_back()` and `insert()`.
-
`push_back()`: This method appends an element to the end of the vector.
myVector.push_back(6); // Adds 6 to the end of the vector
-
`insert()`: This method can insert elements at any position in the vector.
myVector.insert(myVector.begin(), 0); // Inserting at the beginning
Removing Elements
You can also remove elements using methods such as `pop_back()` to remove the last element or `erase()` to remove specific items.
-
Using `pop_back()`:
myVector.pop_back(); // Removes the last element
-
Using `erase()`:
myVector.erase(myVector.begin()); // Erases the first element
Clearing the Vector
To remove all elements from the vector, you can use the `clear()` method. This is efficient for resetting your vector:
myVector.clear(); // Clears all elements
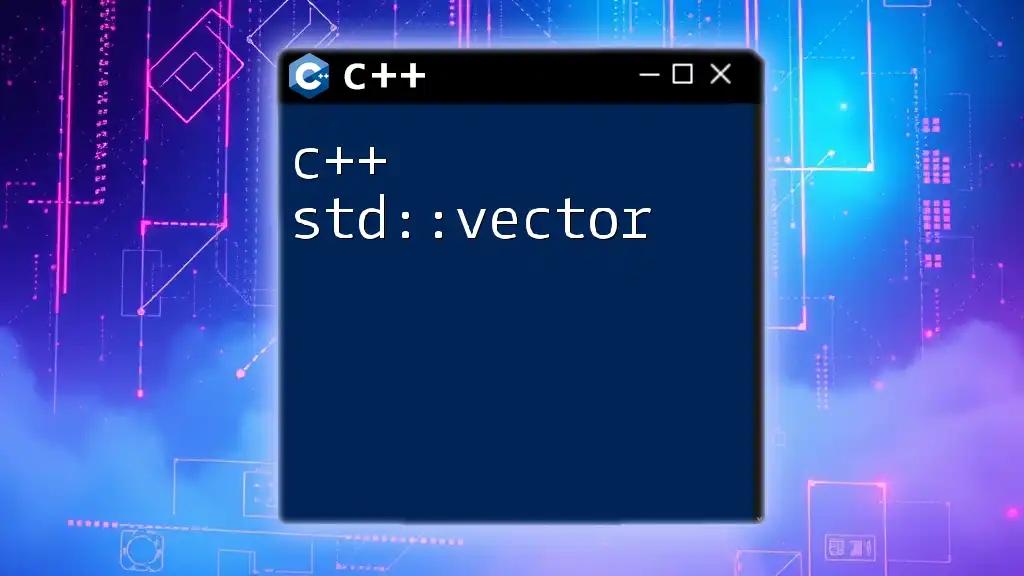
Iterating Through Vectors
Using Range-Based For Loops
Range-based for loops offer a simple syntax for iterating through all elements in a vector while providing ease of readability:
for (const auto& element : myVector) {
std::cout << element << " ";
}
This approach is efficient and concise, automatically handling the beginning and end of the vector for you.
Using Iterators
Iterators provide another way to traverse a vector. An iterator is a pointer-like object that points to an element within a range. Here's an example of using an iterator to access all elements in the vector:
for (auto it = myVector.begin(); it != myVector.end(); ++it) {
std::cout << *it << " ";
}
This method grants fine control over the vector's traversal and allows for modification during iteration, although it requires more syntax.
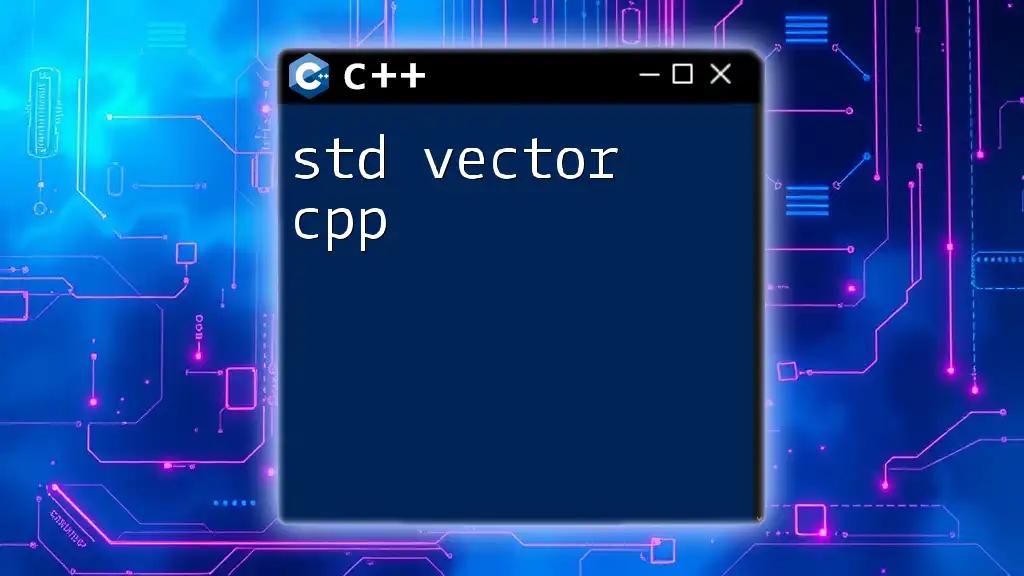
Advanced Features of `std::vector`
Capacity Functions
`std::vector` provides several methods to check its state, including:
- `size()`: Returns the current number of elements in the vector.
- `capacity()`: Returns the total number of elements that can be held without needing to resize.
- `empty()`: Checks whether the vector has no elements.
You can use these functions like this:
std::cout << "Size: " << myVector.size() << std::endl;
std::cout << "Capacity: " << myVector.capacity() << std::endl;
std::cout << "Is Empty? " << (myVector.empty() ? "Yes" : "No") << std::endl;
Resizing and Shrinking Vectors
If you need to change the size of a vector, you can utilize the `resize()` method:
myVector.resize(10); // Resizes vector to hold 10 elements
You can also reclaim unused memory using `shrink_to_fit()`, which reduces the capacity to fit the current size of the vector:
myVector.shrink_to_fit(); // Reduces capacity to fit size

Common Use Cases for `std::vector`
Storing Collections of Data
`std::vector` is particularly beneficial for scenarios where the collection of data fluctuates in size or where frequent additions and deletions are required. For instance, you might use a vector to maintain a dynamic list of customer orders or to gather user inputs in a survey application.
Working with Algorithms
C++ Standard Library algorithms are designed to work seamlessly with `std::vector`. You can use standard algorithms such as `std::sort()` directly on vectors, ensuring your data is organized effectively:
#include <algorithm>
std::sort(myVector.begin(), myVector.end()); // Sorts the vector in ascending order
This compatibility signifies a strong advantage, allowing for efficient data manipulation and organization.

Conclusion
In summary, `cpp std vector` stands out as an essential component of modern C++ programming. It provides programmers with a powerful, flexible, and efficient data structure capable of managing collections of data dynamically while simplifying memory management. With its comprehensive functionalities, `std::vector` is often the go-to choice for storing collections, offering ease of use in various applications.
Embrace this tool in your C++ projects and harness its capabilities to enhance your software solutions effectively.

Frequently Asked Questions
What is the difference between `std::vector` and arrays?
The primary difference lies in the dynamic sizing capability of `std::vector`, in contrast to the fixed size of traditional arrays. Additionally, `std::vector` manages memory automatically, whereas array management requires manual intervention.
When should I use `std::vector`?
You should use `std::vector` when you need a dynamic array that can grow or shrink as needed, ensuring efficient storage and retrieval of data without the hassle of manual memory management.
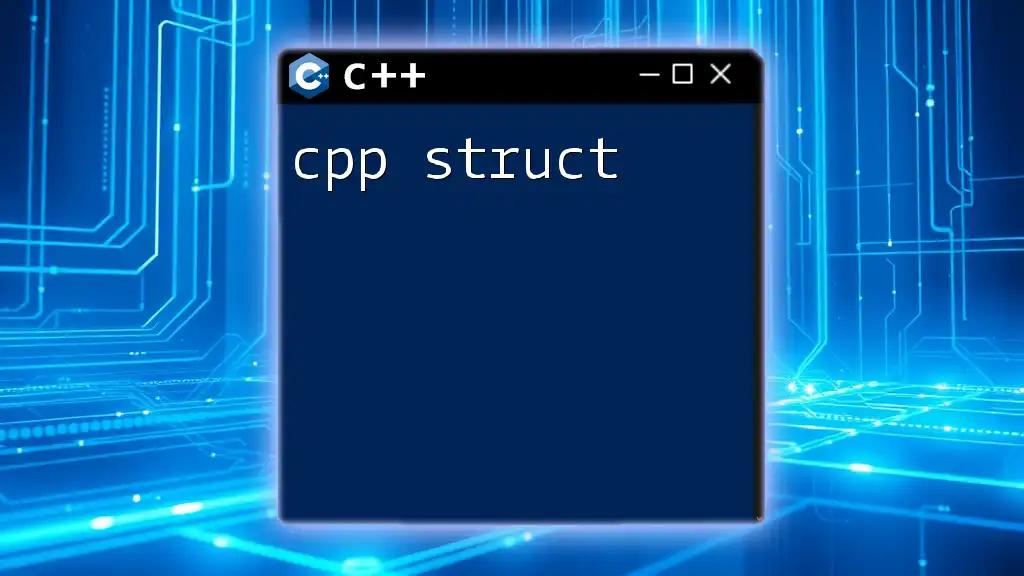
Call to Action
Join our learning community to delve deeper into C++ programming. Stay updated with interactive tutorials, resources, and expert guidance on harnessing the full potential of C++ and its various functionalities like `std::vector`.