A "cpp advisor" is a guide or resource that helps users quickly understand and apply C++ commands and syntax effectively.
Here's a simple example of a C++ command to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is CPP?
Definition and Purpose
CPP refers to the C preprocessor, a vital part of C++ programming that acts as an intermediary between the source code and the compiler. Its primary role is to process directives before the actual compilation occurs. This can involve file inclusion, macro definitions, and conditional compilation, among other tasks. Understanding how to use cpp effectively allows developers to customize their compilation process, making it more efficient and tailored to specific needs.
Importance of Learning C++ Commands
Mastering cpp commands opens numerous doors for aspiring developers. It not only enhances one's ability to manipulate code at a pre-compilation level but also serves as a foundation for better understanding compilation processes. This knowledge is crucial for debugging and optimizing code, which can significantly improve overall project efficiency and performance.
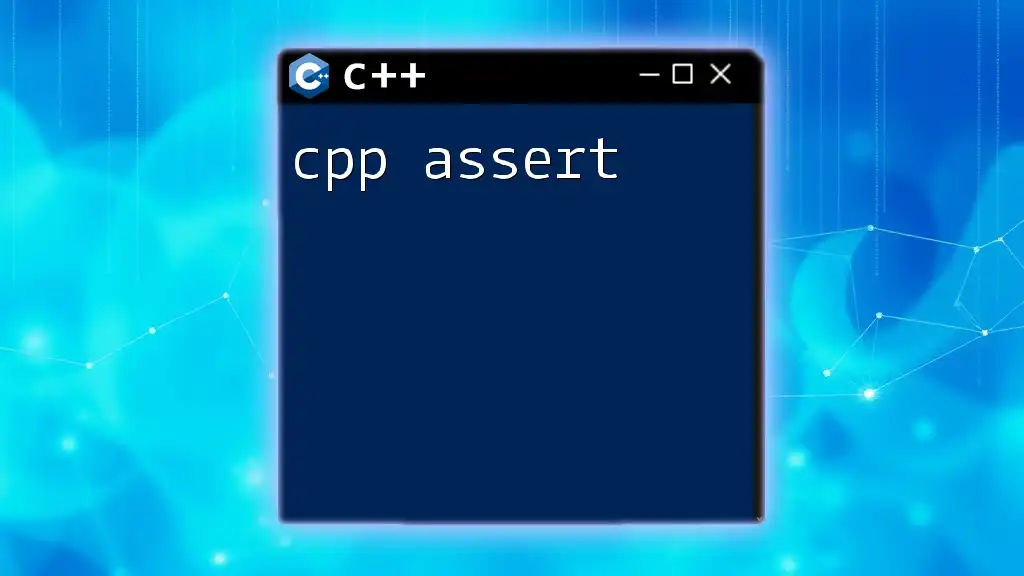
Understanding C++ Commands
Overview of C++ Structure
CPP commands typically follow a strict syntax structure. The general form is:
cpp [options] [file]
Where `[options]` can include various flags and parameters that dictate how the cpp should behave, and `[file]` is the source code file you wish to process. This structure demonstrates the flexibility and adaptability of cpp commands, suitable for different environments and situations.
Types of C++ Commands
Basic Commands
Every C++ developer should be familiar with essential cpp commands used for fundamental tasks, such as compiling code. A common command is:
cpp file.cpp
This command compiles `file.cpp`, outputting the result to the standard output or an executable, based on the platform and configuration.
Advanced Commands
As developers grow more proficient, they can explore more advanced cpp commands. An example of an advanced command includes specifying the output name for the compiled binary:
cpp -o my_program file.cpp
This command compiles `file.cpp` and names the output executable `my_program`, providing greater control over the compilation process.
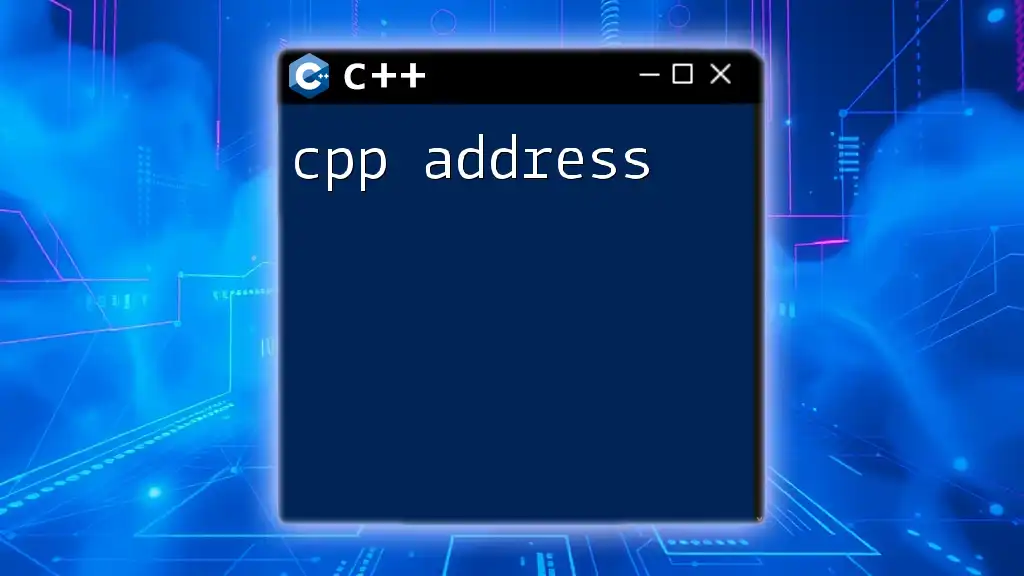
How to Set Up C++ in Your Development Environment
Choosing a Compiler
Selecting the right compiler is crucial for harnessing the full potential of cpp commands. Popular options include:
- GCC (GNU Compiler Collection): Known for its portability and compatibility across various platforms.
- Clang: Highly favored for its performance and user-friendly error messages.
- MSVC (Microsoft Visual C++): Often used in Windows environments, offering extensive integration with development tools.
Researching and selecting the appropriate compiler based on your project requirements can significantly enhance your coding experience.
Installation Guide
To start using cpp commands, you must install a C++ compiler. The following steps outline a general installation process:
- Download the Compiler: Visit the official website of your chosen compiler and download the setup file.
- Run the Installer: Follow the instructions to install the compiler. Ensure that you select the option to add the compiler to your system's PATH for easy command-line access.
- Verify Installation: Open your command-line interface and type `g++ --version` (for GCC) to confirm successful installation.
If any issues arise during this process, checking online forums and the documentation specific to your compiler can help resolve them.
Writing Your First C++ Command
Hello World Example
Now that your environment is set up, let’s write a classic "Hello, World!" program to see cpp commands in action. Create a file named `hello.cpp` with the following content:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this program using cpp commands, navigate to your command-line interface, and run:
cpp hello.cpp
This command will compile the `hello.cpp` file. To execute the program, type `./a.out` on Unix systems or `a.exe` on Windows. You should see the output:
Hello, World!
This simple example illustrates how cpp commands serve as the initial step in turning code into executable programs.
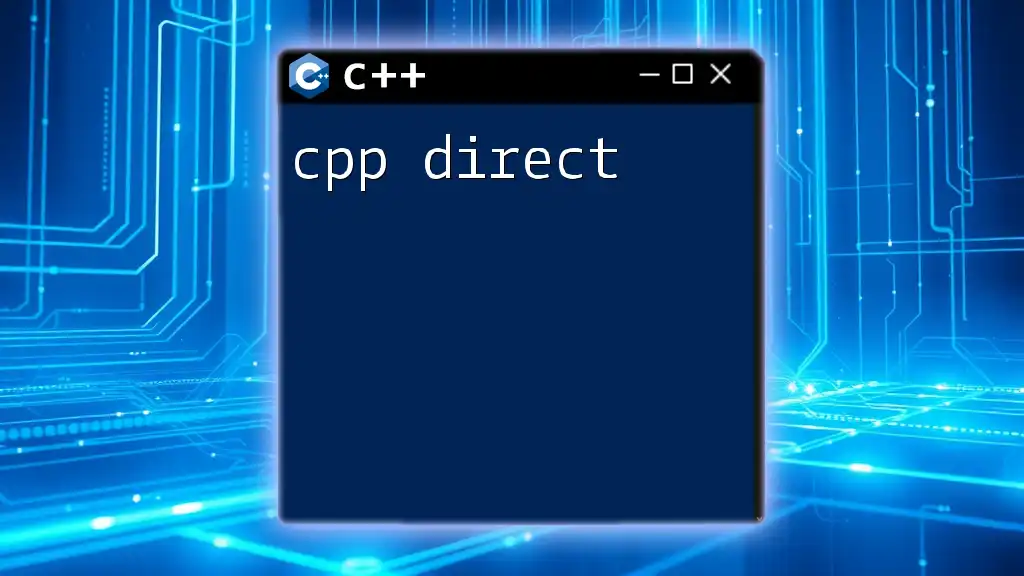
Common C++ Command-Line Options
Compiler Flags
CPP commands support several flags, which facilitate specific functionalities during the compilation process. Some common flags include:
- `-Wall`: Enables all compiler warnings, helping you catch potential issues early.
- `-O2`: Activates an optimization level that helps improve performance without significantly increasing compilation time.
By using these flags, developers can enhance the quality and performance of their C++ projects.
Optimization and Debugging
Utilizing cpp commands effectively for optimization and debugging is paramount:
- `-g`: This flag generates debug information, useful for debugging applications with tools like GDB.
- `-O3`: This option applies a higher level of optimization, which can greatly enhance execution speed.
Incorporating these options into your cpp command usage can streamline the development and debugging process.
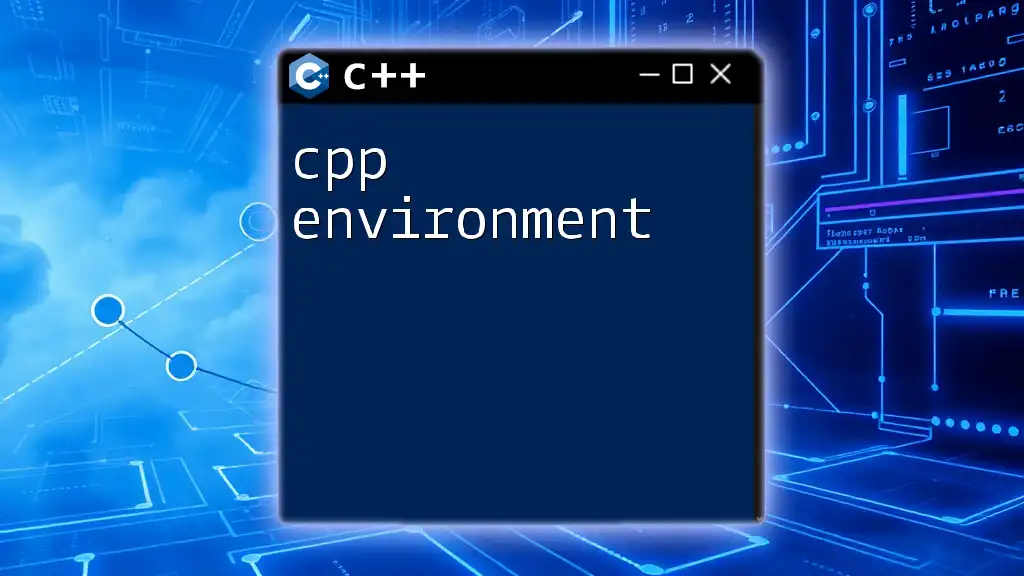
Practical Use Cases of C++ Commands
Project Management
CPP commands not only compile individual files but also facilitate entire project management. By creating a makefile that includes cpp commands, developers can automate the build process for larger projects. This not only saves time but also reduces errors related to manual compilation.
Automation Scripts
CPP commands can play a key role in automation. By integrating cpp commands into scripts, you can automate complex build processes, reduce manual workload, and improve consistency across builds.
Performance Analysis
CPP commands can also be utilized in profiling C++ applications. By compiling your program with specific flags like `-pg`, you can generate profiling data, which aids in identifying bottlenecks and optimizing performance.
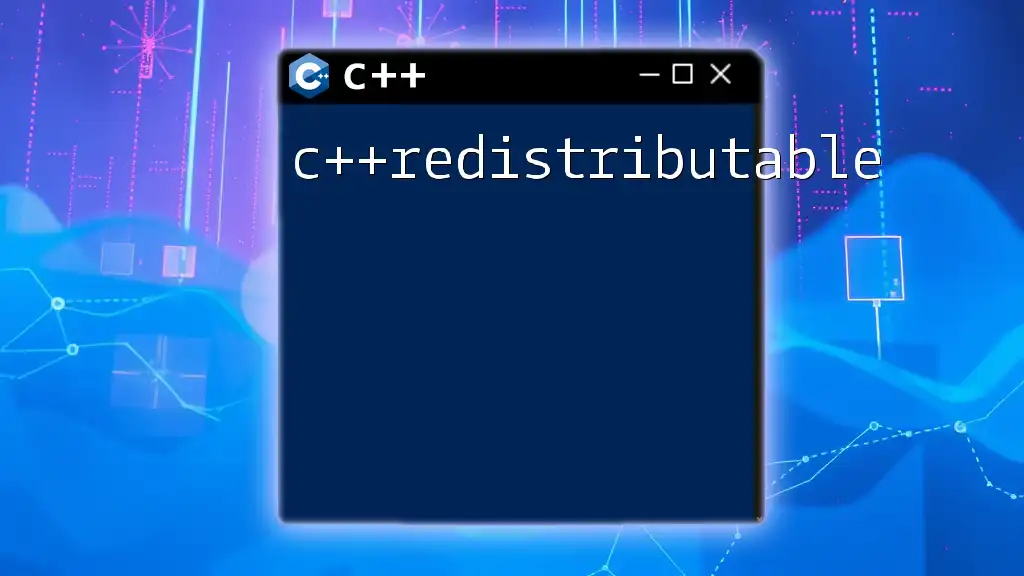
Frequently Asked Questions (FAQs)
What are the differences between cpp commands and standard C++ syntax?
CPP commands primarily operate at the pre-compilation stage and involve handling directives such as `#include` and `#define`, while standard C++ syntax comprises the actual code structure and programming logic used in creating applications.
Can I use cpp commands on Windows, Linux, and macOS?
Yes, cpp commands can be utilized across various platforms. While the command syntax remains consistent, ensure the correct compiler and setup specific to your operating system are in place.
How do cpp commands enhance productivity for developers?
By mastering cpp commands, developers reduce repetitive tasks, streamline compiling, manage projects effortlessly, and focus more on coding rather than troubleshooting compilation issues.
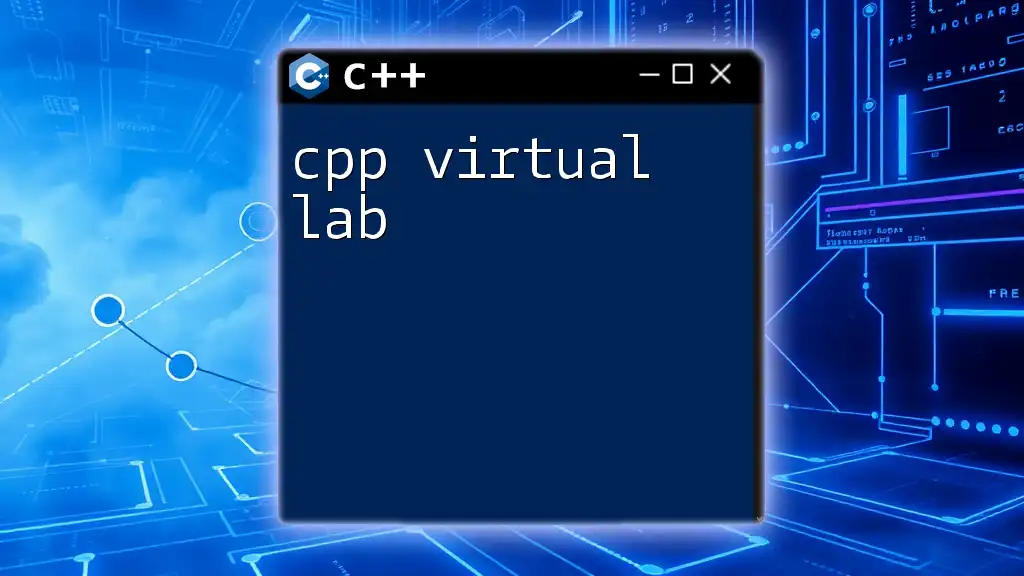
Conclusion
Understanding cpp commands is essential for mastering C++ programming. It empowers you to optimize your coding workflow, automate processes, and ultimately build better software. The journey of learning cpp commands will enhance not only your technical skills but also your overall approach to software development.
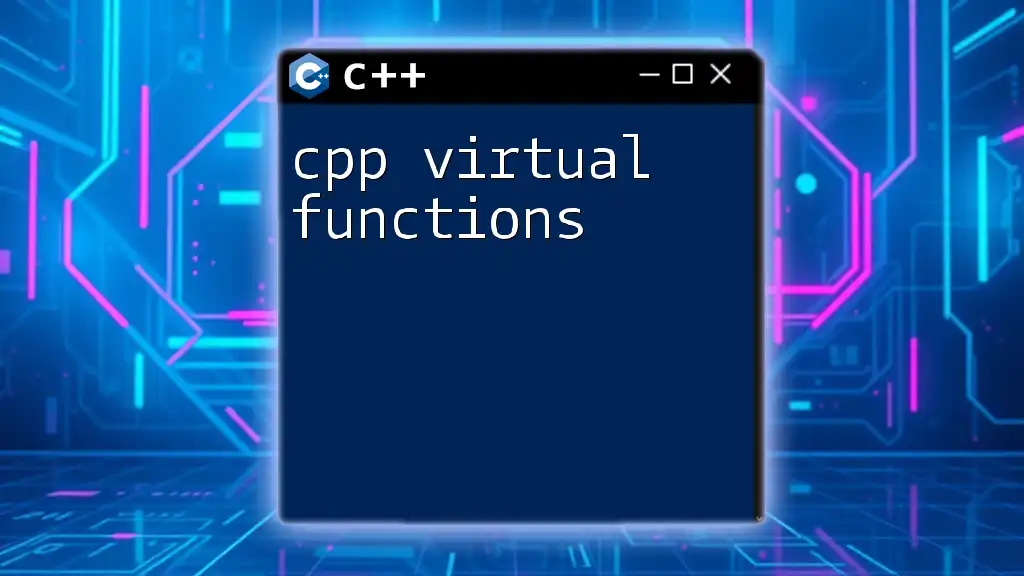
Further Resources
Recommended Books and Online Resources
For those who wish to delve deeper into C++ and cpp commands, consider exploring classical textbooks like "C++ Primer" by Lippman or online resources on platforms like GeeksforGeeks, CPPReference, and official documentation sites.
Online Courses and Tutorials
Platforms like Coursera, Udacity, and Udemy offer an array of courses specifically focused on C++ programming, providing structured guidance through video tutorials, exercises, and projects.
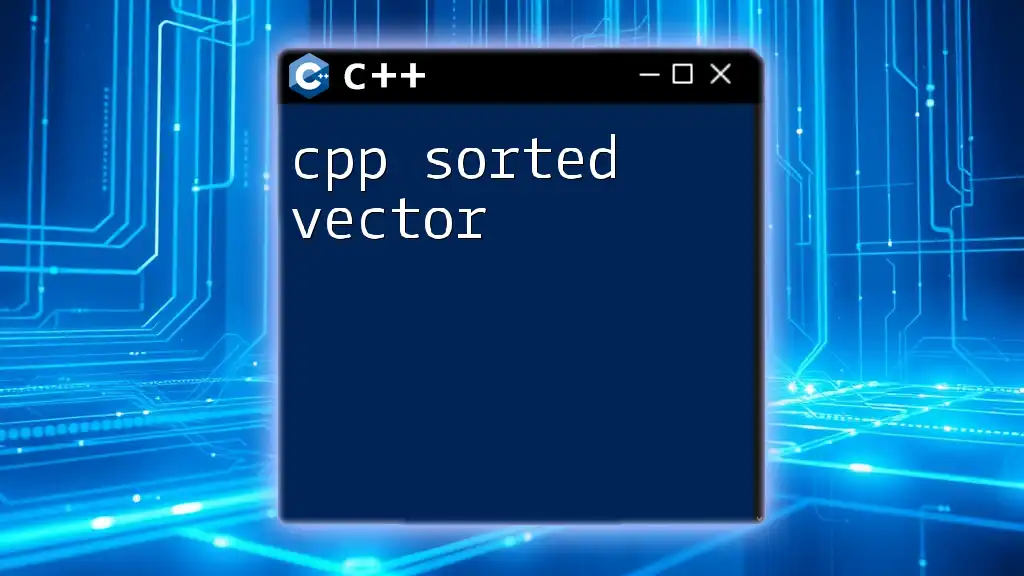
Call to Action
I encourage you to experiment with cpp commands and share your discoveries. Following our company’s tutorials will help you refine your skills and keep up with best practices in C++ programming!