The `substr` function in C++ is used to extract a substring from a given string, defined by the starting position and the length of the substring.
Here's a code snippet demonstrating its use:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string subStr = str.substr(7, 5); // Extracts "World"
std::cout << subStr << std::endl; // Outputs: World
return 0;
}
Understanding C++ Substring Manipulation
What is a substring?
A substring is any sequence of characters that forms part of a larger string. For example, in the string "Hello, World!", the substrings can range from single characters like "H" to full segments like "World".
Importance of substrings in programming
Substrings play a crucial role in various programming scenarios. They might be used in text processing, data parsing, or even manipulating user inputs. Understanding how to effectively use substrings can enhance your ability to perform complex string manipulations efficiently.

Overview of the cpp substr Function
What is the substr function in C++?
The cpp substr function is part of the C++ Standard Library, specifically within the `<string>` header. It allows developers to extract a portion of a string, making it essential for various string manipulation tasks.
Where to find the substr function
To utilize the substr function, ensure you include the required header at the beginning of your code:
#include <string>

Syntax of the C++ substr Function
The syntax for using the substr function is straightforward:
string substr (size_t pos = 0, size_t len = npos);
- Parameters Explained:
- pos: This parameter indicates the starting position from which the substring will be extracted. It is zero-indexed, meaning that a position of `0` refers to the first character of the string.
- len: This optional parameter specifies the number of characters to be included in the substring. If not provided, the function extracts all characters from the starting position to the end of the string.
Return value of the substr function
The function returns a new string object containing the requested substring. If the parameters exceed the limits of the original string, it may either return an empty string or result in an exception being thrown.
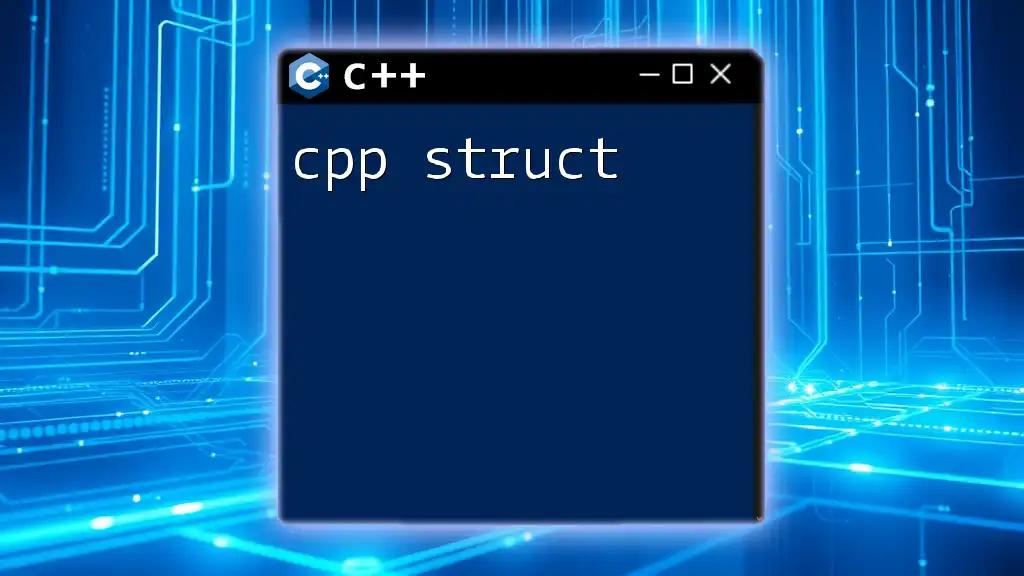
How to Use substr in C++
Using the cpp substr function is quite intuitive. Here’s a simple example to illustrate its basic usage:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string sub = str.substr(7, 5); // Extract "World"
std::cout << sub << std::endl;
return 0;
}
In this code snippet, we declare a string `str`. By calling `str.substr(7, 5)`, we extract five characters starting from the eighth character (noted by position 7, because of zero indexing).
Explaining the Example
In the example, the substring "World" is successfully extracted from the original string. This demonstrates how simple it is to use the cpp substr function to manipulate and retrieve specific portions of text.
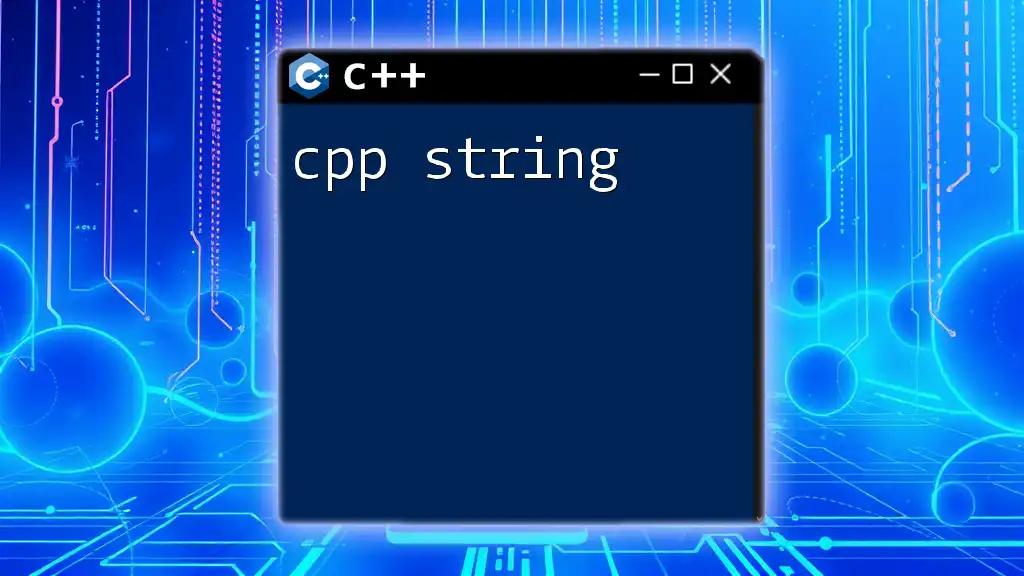
Common Use Cases for C++ String Substring
Extracting a part of a string
One of the most common scenarios for using the cpp substr function is extracting specific parts of a string. This is especially useful in applications such as:
- Extracting file extensions: Accessing the file type from a file name (e.g., "document.txt" would yield "txt").
- Tokenizing strings: Breaking a sentence into manageable pieces, useful in processing user inputs.
Case Study Example
Consider a scenario where we need to parse command-line arguments. The substr function can help extract specific flags and values from a complex input string.
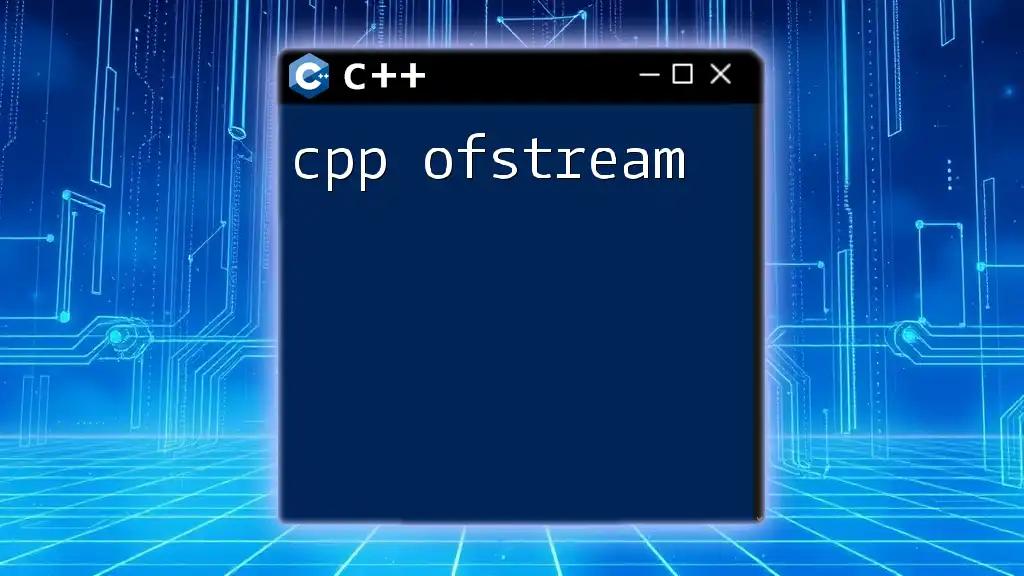
Error Handling with substr Function
What happens when the provided position is out of bounds?
When using the substr function, it’s imperative to manage bounds effectively. If the position specified exceeds the length of the string, an `std::out_of_range` exception will be thrown.
Here's an example of error handling:
#include <iostream>
#include <string>
int main() {
try {
std::string str = "Hello";
std::string sub = str.substr(10, 5); // This will trigger an exception
} catch (const std::out_of_range& e) {
std::cerr << "Out of range error: " << e.what() << std::endl;
}
return 0;
}
Best practices for using substr function safely
To ensure the safe usage of the substr function, it is prudent to check the length of the string before calling the function:
if (position < str.length()) {
// Call substr
}
This conditional check ensures that you do not encounter unintended errors during execution.
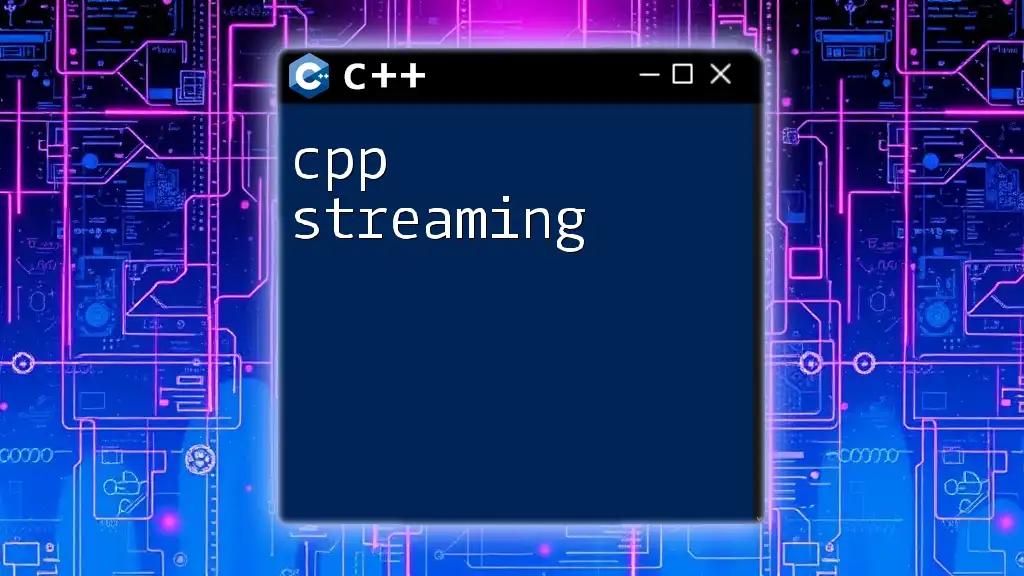
Advanced Techniques with substr in C++
Chaining substr calls
You can also chain multiple calls to the substr function for more complex operations. For example:
std::string str = "Welcome to C++ programming";
std::string sub = str.substr(11).substr(0, 2); // Result: "C+"
In this case, the first `substr` call navigates to the start of "C++ programming" before the second call extracts "C+".
Combining substr with other string functions
The substr function works exceptionally well when paired with other string manipulation functions, such as `find()`, `append()`, or `replace()`. Here is an instance of combining substr with find:
std::string text = "The quick brown fox jumps over the lazy dog";
size_t pos = text.find("fox");
std::string sub = text.substr(pos, 3); // Extracts "fox"
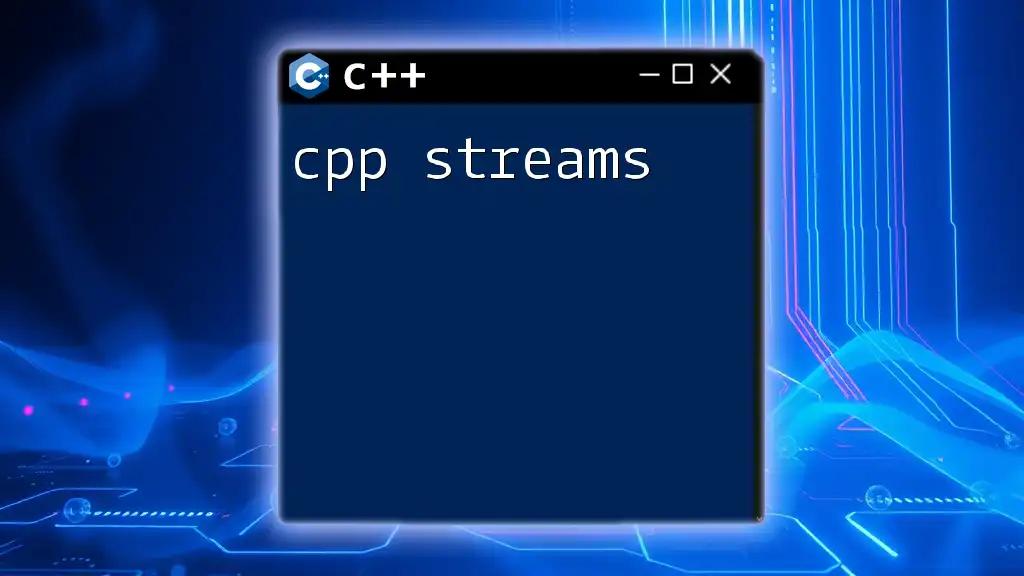
Performance Considerations
When to use substr for large strings?
Performance can become a concern when processing large strings. Each call to substr creates a new string object, which consumes memory. If substringing is performed frequently on large datasets, consider optimizing your implementation to minimize overhead.
Optimizing substring operations in C++
To boost efficiency, explore methods to minimize allocations, such as reusing string objects where appropriate, or utilizing string views (in C++17 and later) for read-only access to string substrings.
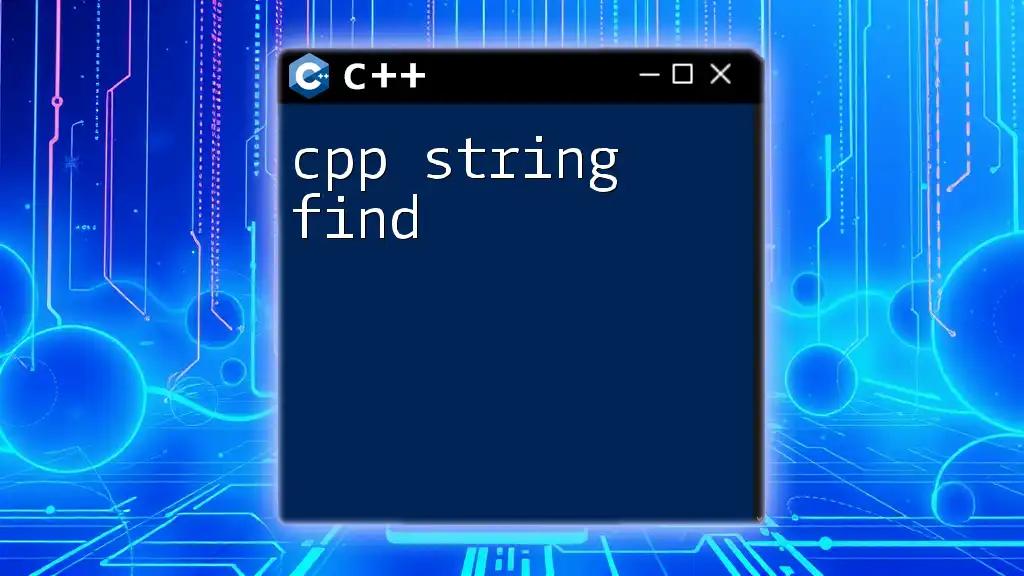
Comparing substr with Other String Manipulation Functions
How does substr differ from other functions like erase(), insert(), and replace?
The substr function focuses solely on extraction, while other functions serve different purposes:
- erase(): Removes characters from a string.
- insert(): Adds characters to a string at a specified position.
- replace(): Substitutes characters in a string with different characters.
Understanding these differences helps in choosing the right function for string manipulations in your code.
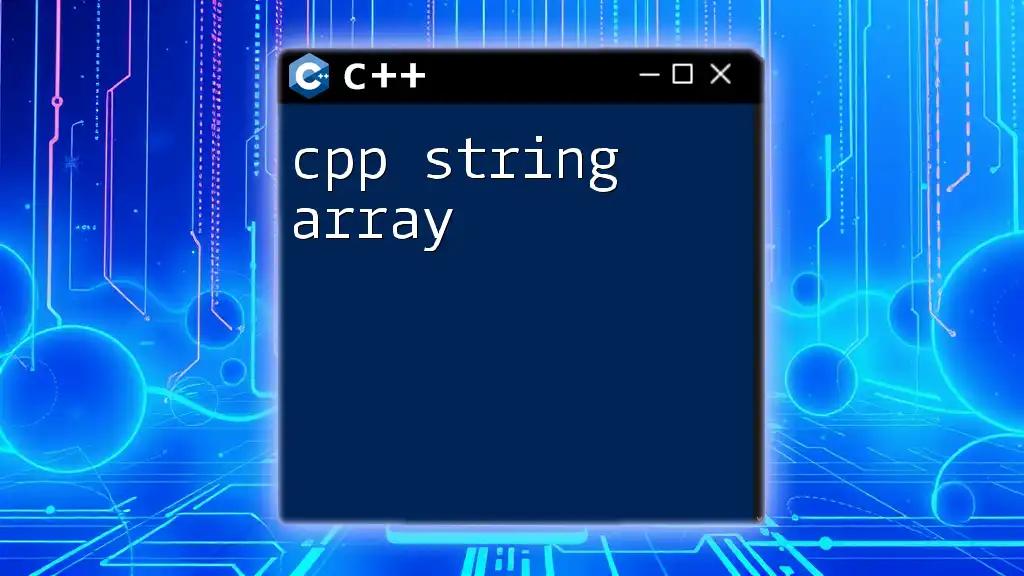
Conclusion
Mastering the cpp substr function unlocks numerous possibilities for efficient string manipulation. The ability to extract specific portions of a string is invaluable across various domains, from data analysis to user input processing.
As you dive deeper into your coding journey, don't hesitate to explore, experiment, and practice using substr. The more you work with it, the more adept you will become at leveraging this powerful tool in your C++ programming arsenal.
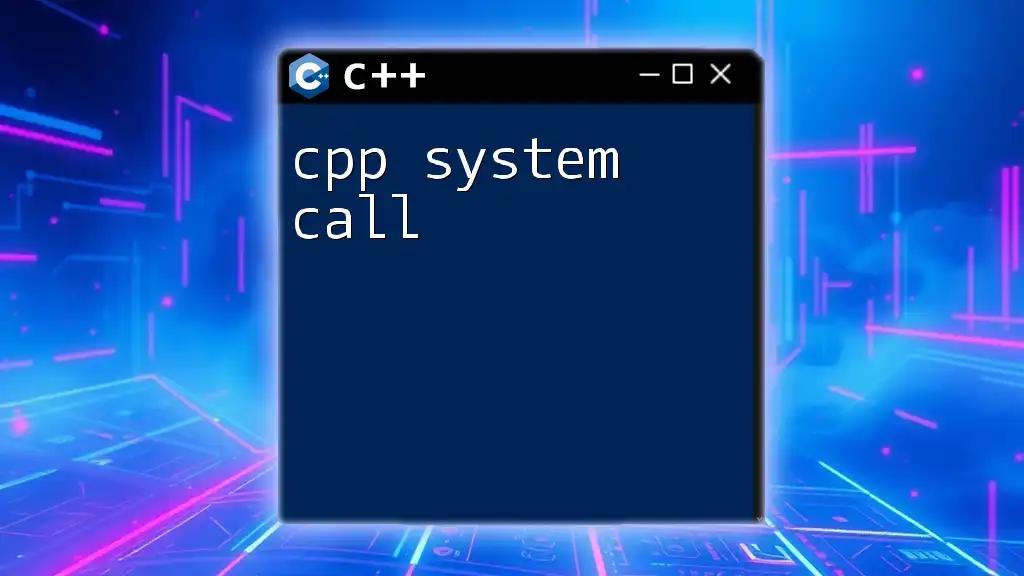
Additional Resources
- Consider checking the official C++ documentation for the latest updates and advanced concepts.
- Supplement your learning with recommended books and online tutorials focused on C++ programming and string manipulations for a well-rounded understanding.