A C++ string array is an array that stores multiple string elements, allowing you to manage a collection of text data easily.
Here's a code snippet demonstrating how to declare and initialize a string array in C++:
#include <iostream>
#include <string>
int main() {
std::string fruits[] = {"Apple", "Banana", "Cherry"};
for (const auto& fruit : fruits) {
std::cout << fruit << std::endl;
}
return 0;
}
Understanding the Basics of Arrays and Strings in C++
What is an Array?
In C++, an array is a collection of elements of the same type, stored in contiguous memory locations. This structure allows for easy access and manipulation of collections of data. The syntax for declaring an array is as follows:
data_type array_name[array_size];
Arrays are beneficial when you need to handle multiple values of the same type, such as storing a list of names or values.
What is a String in C++?
A string in C++ is an object of the `std::string` class from the Standard Library. Unlike C-style strings (character arrays), `std::string` offers numerous functionalities, including string concatenation, substring search, and automatic memory management. A simple example of a string declaration is:
std::string name = "John Doe";
Combining Arrays and Strings: The String Array in C++
When we talk about a cpp string array, we refer to the use of an array to store multiple strings. This combination allows handling multiple strings while maintaining the advantages of array indexing. String arrays can be particularly useful in scenarios where you need to store a list of names, phrases, or any other textual data efficiently.
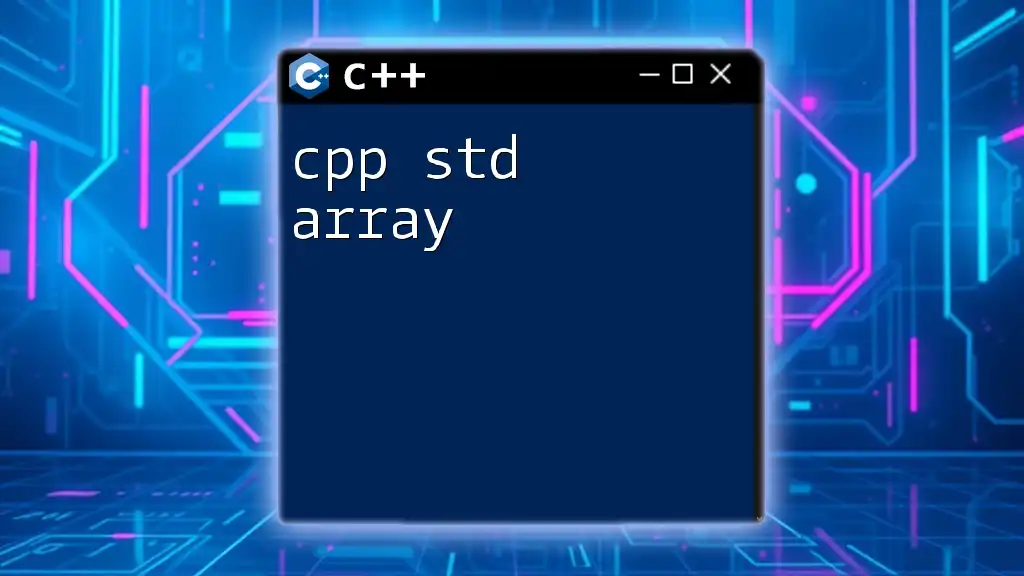
Declaring String Arrays in C++
Syntax for String Array Declaration
The declaration of a string array in C++ is straightforward. You can define a string array using the following syntax:
std::string array_name[array_size];
This simply creates an array capable of storing `array_size` strings.
Initializing String Arrays
You can initialize a string array during its declaration. Here's an example illustrating this:
std::string fruits[] = {"Apple", "Banana", "Cherry"};
This initializes an array named `fruits` containing three string elements.
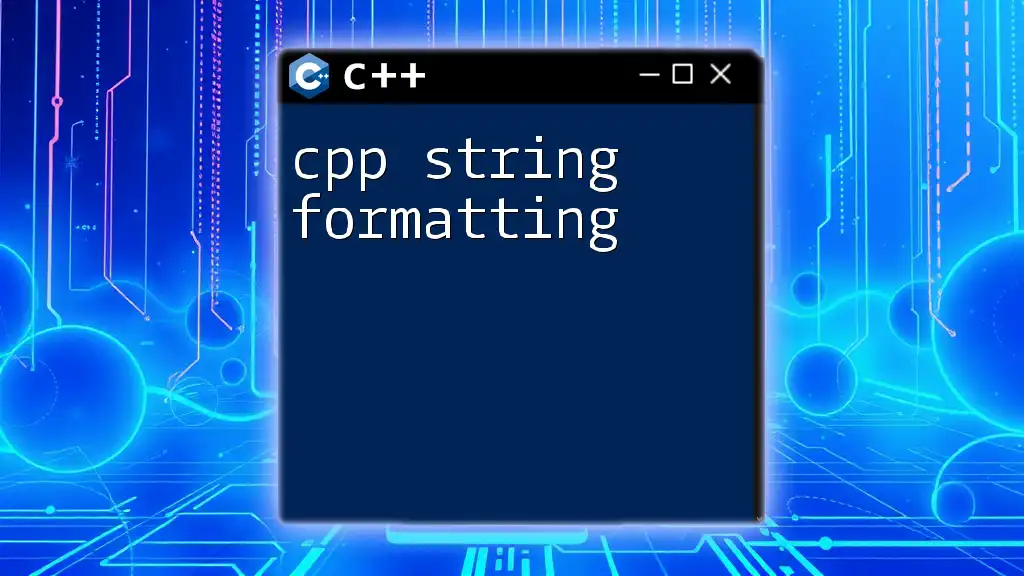
Accessing Elements in a String Array
Access syntax and methods
Each element in a string array can be accessed using the index corresponding to its position. Remember that indexing starts at zero. For example, to access the first element:
std::cout << fruits[0]; // Output: Apple
Iterating Over String Arrays
You can efficiently traverse a string array using loops. Here’s an example using a simple `for` loop to iterate over our `fruits` array:
for (int i = 0; i < 3; i++) {
std::cout << fruits[i] << " ";
}
This loop prints each fruit in the array, separated by spaces.
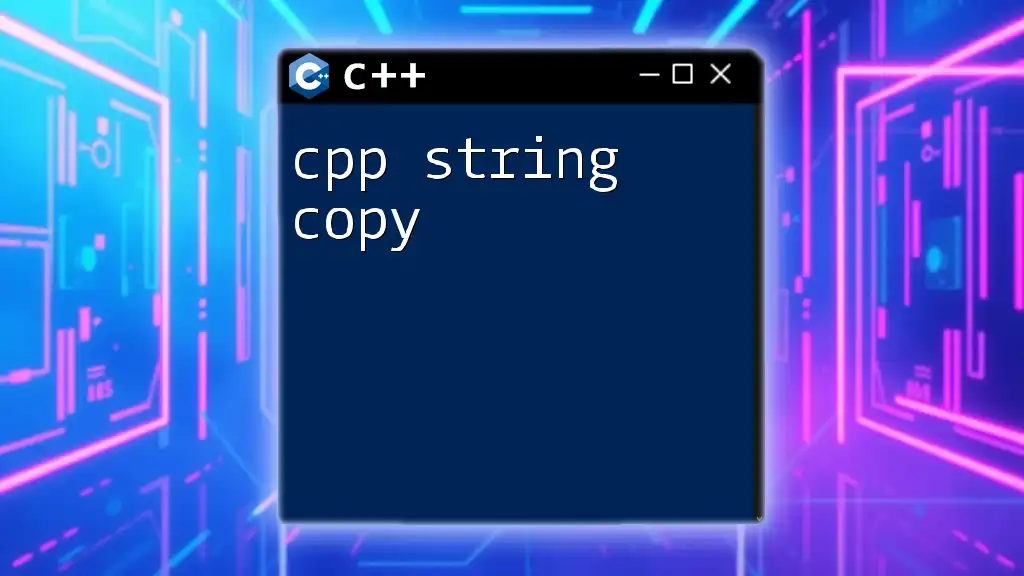
Common Operations on C++ String Arrays
Modifying Elements in a String Array
To modify an element in a string array, you can directly assign a new value using the index. For example, if you want to change "Banana" to "Blueberry":
fruits[1] = "Blueberry";
This operation directly modifies the second element of the `fruits` array.
Finding the Size of a String Array
To determine the number of elements in a string array, you can use the `sizeof` operator in conjunction with the size of a single element. Here's how you can do it:
int size = sizeof(fruits) / sizeof(fruits[0]);
This simple calculation yields the total number of elements in the `fruits` array.
Sorting String Arrays
Sorting the strings in an array can be performed using the `std::sort` function from the `<algorithm>` header. Here's a quick example:
#include <algorithm>
std::sort(fruits, fruits + size);
This will sort the `fruits` array in alphabetical order.
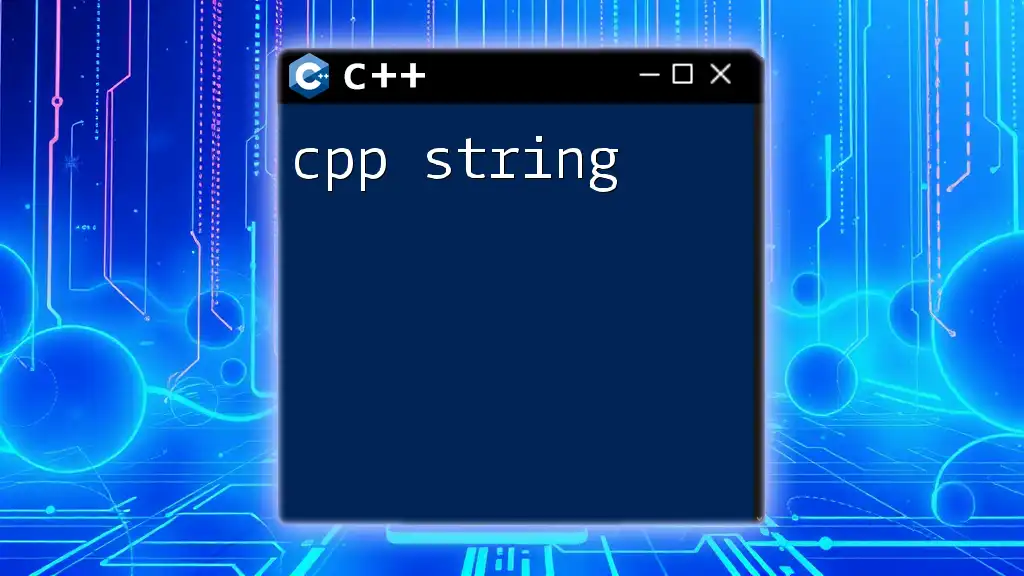
Best Practices for Using String Arrays in C++
Choosing Between String Arrays and Other Data Structures
While string arrays can be useful, they do have limitations, especially with regards to size flexibility. If you need to frequently change the number of elements or if you prioritize convenience and functionality, consider using `std::vector<std::string>` instead of a raw array. Vectors provide dynamic resizing capabilities and a rich set of built-in functions that simplify your code.
Memory Management Considerations
It is crucial to manage memory properly when working with arrays. Although C++ handles memory for `std::string` objects elegantly, remember that if you're using raw arrays of characters, you'll need to ensure proper memory allocation and deallocation to avoid memory leaks. Always prefer the standard libraries, as they help mitigate these issues automatically.
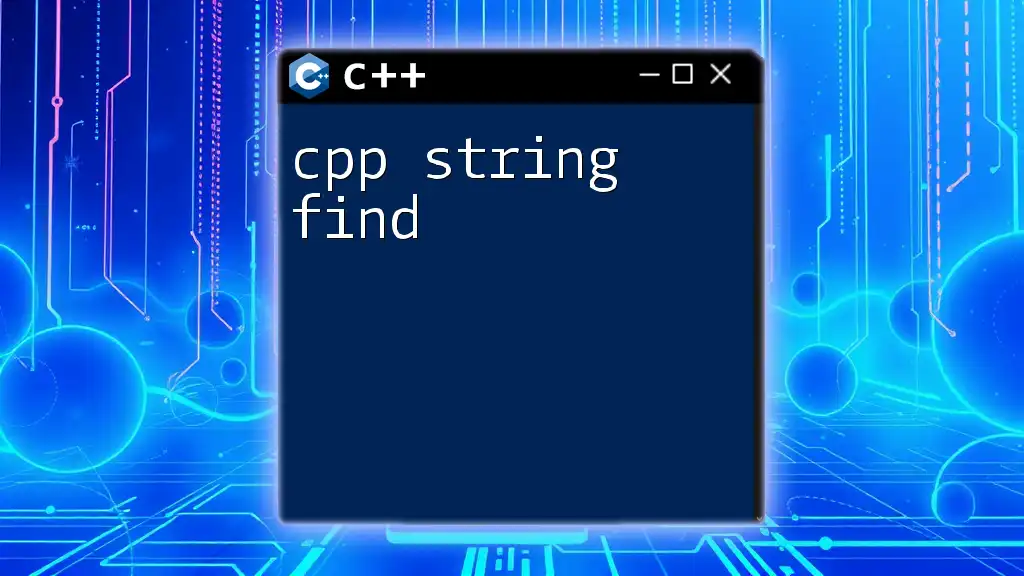
Conclusion
In this comprehensive exploration of the cpp string array, we've delved into its fundamentals, including declaration, initialization, and common operations. The versatility of string arrays makes them invaluable in various applications. Whether you’re traversing arrays, modifying elements, or sorting strings, mastering these concepts will enhance your C++ programming skills. As you grow more comfortable, consider advancing your knowledge to more complex data structures and their applications!
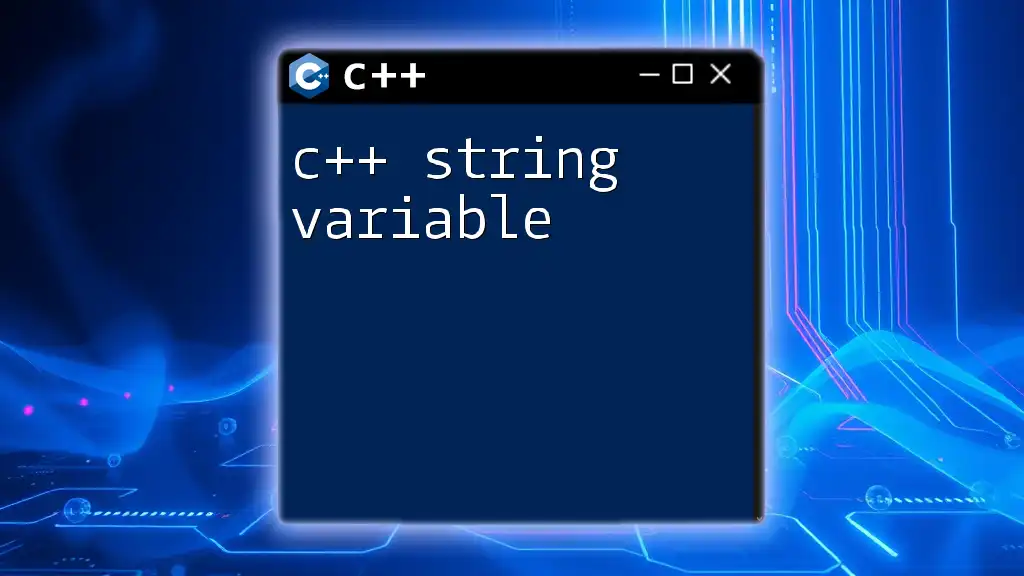
Additional Resources
For further exploration of arrays and strings in C++, you can consult the official C++ documentation. Additionally, consider browsing recommended books and tutorials that delve deeper into advanced C++ topics.
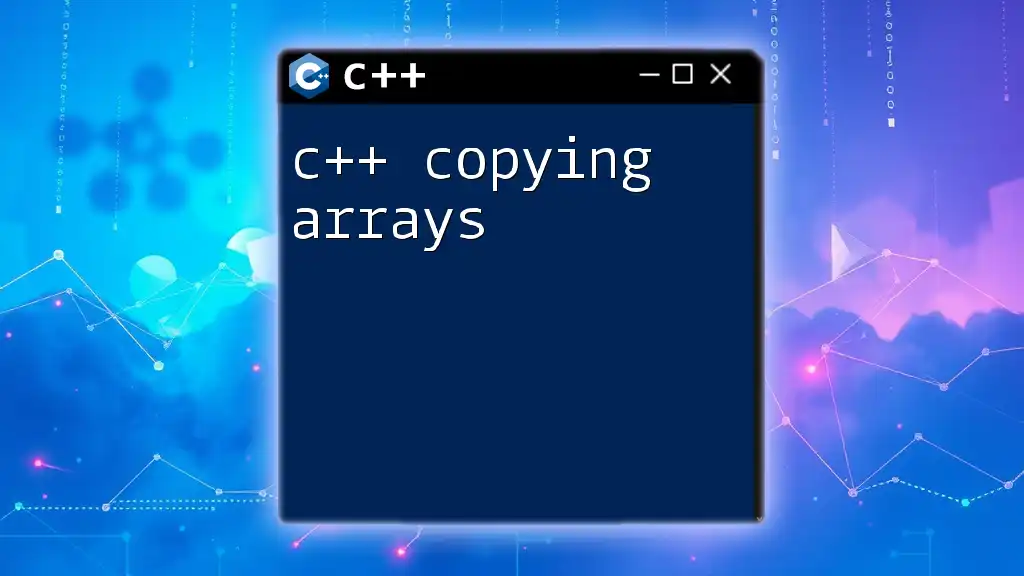
Frequently Asked Questions (FAQs)
What is the difference between a string and a string array in C++?
A string is a single instance of a text sequence, while a string array stores multiple strings, allowing for the management of a collection of text sequences.
How do I convert a string array to a single concatenated string?
You can concatenate strings in an array by iterating through the array elements and accumulating them into a single string variable.
Can I use string arrays with multiple dimensions in C++?
Yes, you can define multi-dimensional string arrays, which resemble matrices, to accommodate more complex data structures. However, they require careful indexing.