In C++, a string variable can be declared using the `std::string` type, which is part of the Standard Library and allows for dynamic string manipulation.
#include <string>
std::string myString = "Hello, World!";
Understanding C++ String Types
The C++ String Class
A string variable in C++ can be represented by the `std::string` class, which is part of the C++ Standard Library. The `std::string` class simplifies string manipulation, providing a wide range of functionalities, such as concatenation, substring operations, and searching.
In contrast, C-style strings are arrays of characters that end with a null terminator (`'\0'`). While C-style strings are powerful and used in legacy C programs, they lack the safety and ease-of-use provided by the `std::string` class. Understanding the difference enables programmers to choose the right string type according to their needs.
C-style Strings
A C-style string is an array of characters declared using the `char` type. You can declare and initialize a C-style string as follows:
char cString[] = "Hello, World!";
Proper memory management is required with C-style strings, as they do not automatically manage their own memory. For example, when using `strcpy` to copy strings, developers must ensure that the destination has enough allocated space.
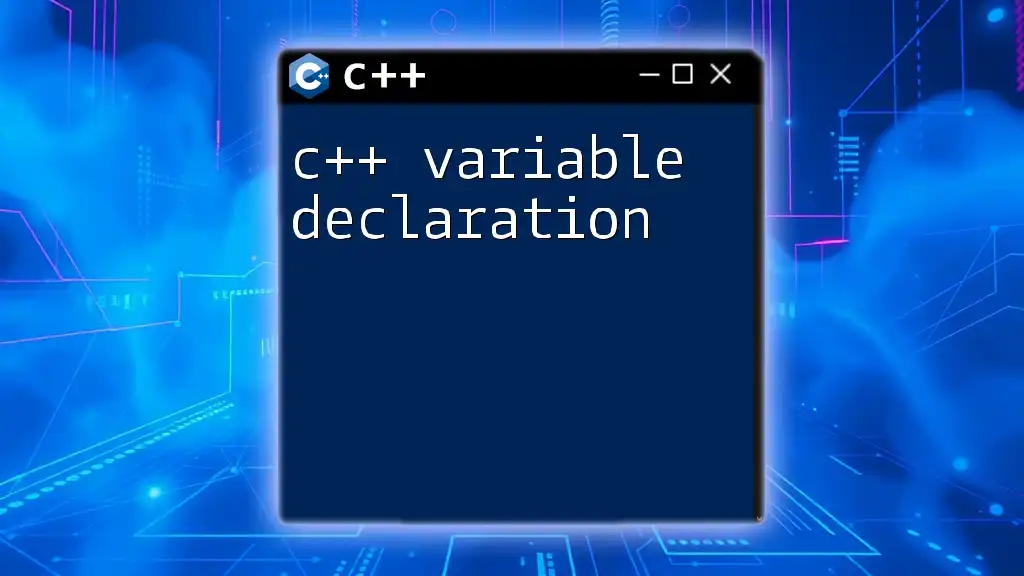
How to Declare a String Variable in C++
What Does It Mean to Declare a String Variable?
Declaring a string variable means allocating space for it and specifying its type. In C++, declaring variables is crucial for type safety and memory management. An undeclared variable leads to compilation errors, as the compiler does not know the type or how much space to allocate.
Basic Syntax for Declaring a String Variable
To declare a string variable using `std::string`, the syntax is straightforward:
std::string myString;
This line of code creates an instance `myString` of type `std::string`, ready for use.
Example Code Snippet
#include <iostream>
#include <string>
int main() {
std::string myString; // Declaring a string variable
std::cout << "String variable declared successfully!" << std::endl;
return 0;
}
In this example, `myString` is declared but not initialized, meaning it will default to an empty string.
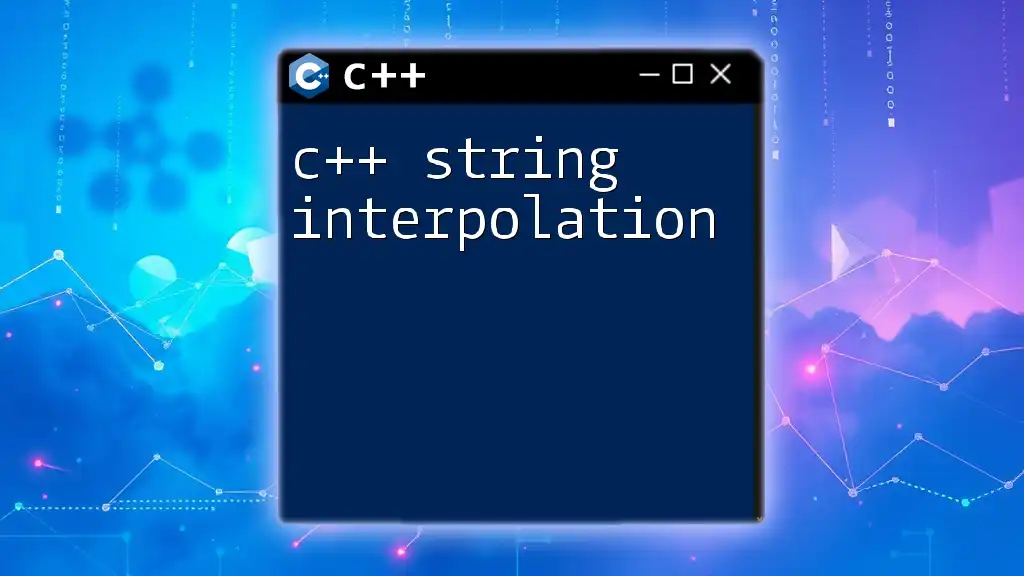
Different Ways to Declare a String in C++
Declaring and Initializing a String Variable
You can declare and initialize a string variable in one step, which is often more convenient:
std::string greeting = "Hello, World!";
In this example, `greeting` is declared and immediately initialized with the value `"Hello, World!"`.
Using the `std::string` Constructor
Another method for initializing a string variable is to use the constructor of the `std::string` class:
std::string name("John Doe");
This syntaxexplicitly uses the constructor, which can make the code clearer and more versatile.
Empty and Default Initialization
If you want to declare a string variable without assigning it any value, you can do so using default initialization. This creates an empty string:
std::string emptyString; // Default initialization to an empty string
Using an empty string is common when you plan to fill it with user input or other data later in the program.
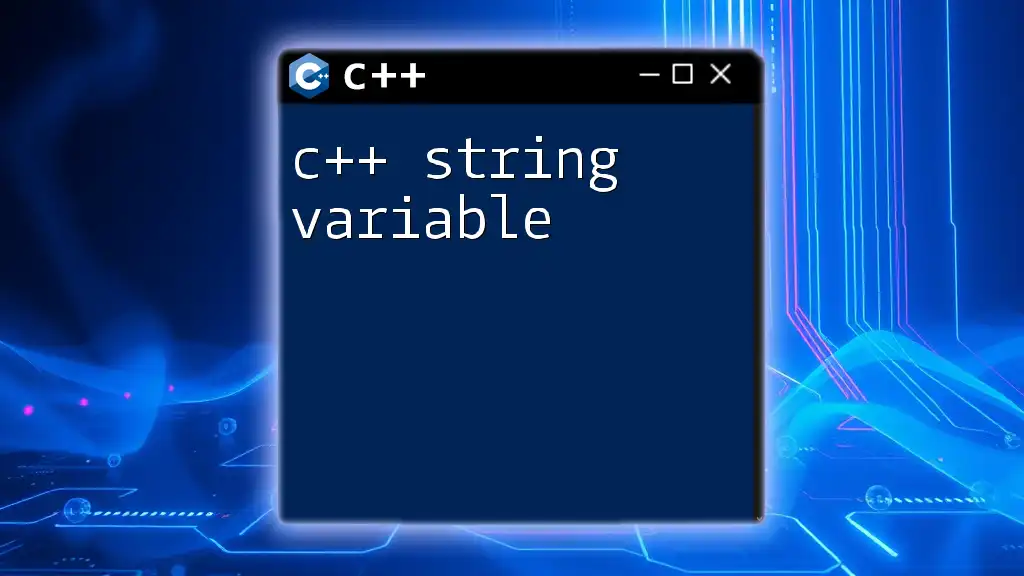
Common Mistakes While Declaring String Variables in C++
Forgetting to Include the String Header
One common mistake when working with `std::string` is forgetting to include the header file. Without:
#include <string>
the compiler will not recognize `std::string`, leading to compilation errors. Always make sure your code includes necessary headers.
Mixing C-style String with std::string
Another frequent error involves mixing C-style strings with `std::string`. For example:
std::string myString = "Hello";
char cString[10];
cString = myString; // This will cause an error
C-style strings and `std::string` are incompatible types. To convert between them safely, use the `.c_str()` method for `std::string`:
const char* cString = myString.c_str(); // Convert string to C-style
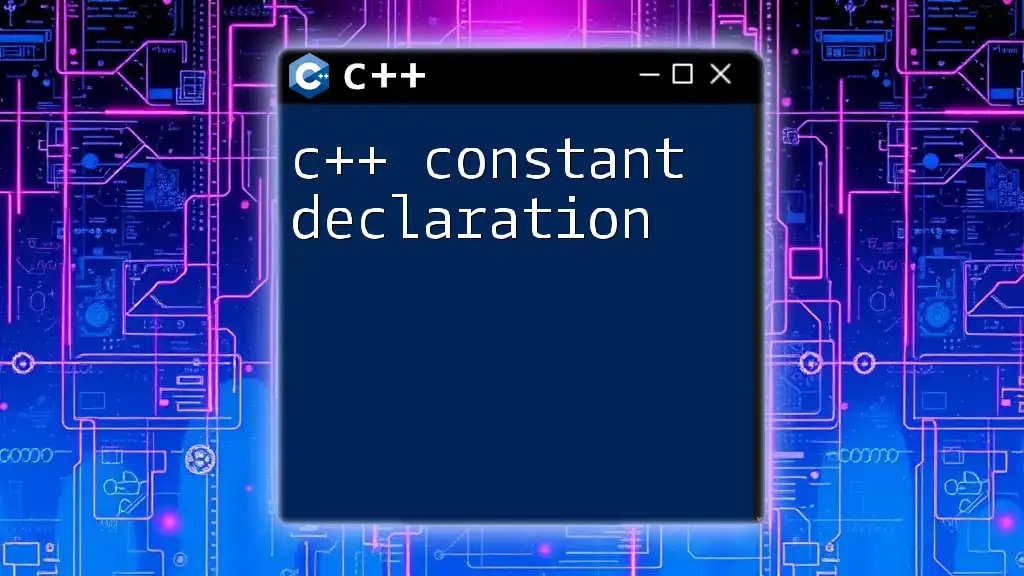
Practical Examples of Declaring String Variables in C++
Example: Taking User Input
String variables are ideal for capturing user input. Here's how you can use a declared string variable to receive input:
std::string userInput;
std::cout << "Enter your name: ";
std::getline(std::cin, userInput);
std::cout << "Hello, " << userInput << "!" << std::endl;
In this example, `std::getline` captures an entire line of text, including spaces, and stores it in `userInput`.
Example: String Concatenation
You can also utilize string variables for concatenation, combining multiple strings together seamlessly:
std::string firstName = "Jane";
std::string lastName = "Doe";
std::string fullName = firstName + " " + lastName;
std::cout << "Full Name: " << fullName << std::endl;
Here, the `+` operator is used to concatenate `firstName` and `lastName`, separated by a space, forming a complete name.
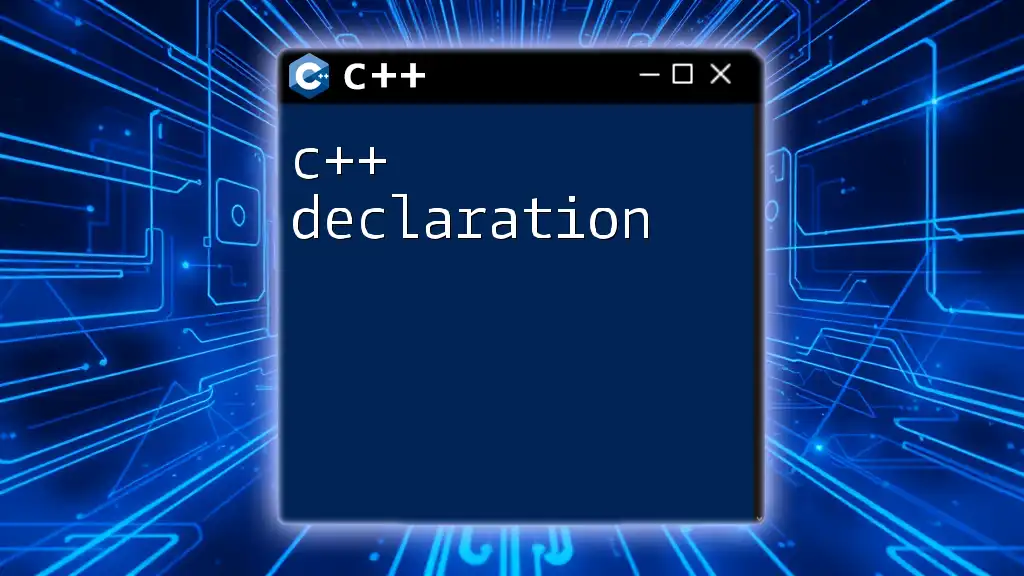
Best Practices for Declaring String Variables in C++
Choosing Descriptive Names
When declaring string variables, it is vital to choose descriptive names that convey purpose. Instead of generic names like `s` or `str`, opt for something meaningful, such as `userName` or `errorMessage`. This improves code readability and maintainability.
Understanding Scope and Lifetime of String Variables
Understanding the scope and lifetime of string variables helps prevent memory issues. Local string variables declared within a function only exist in that function's scope. Declaring them outside of any function grants them a global scope.
Being aware of the lifetime of your variables is critical because memory used by local variables is automatically released at the end of their scope, whereas global variables remain until the program terminates.
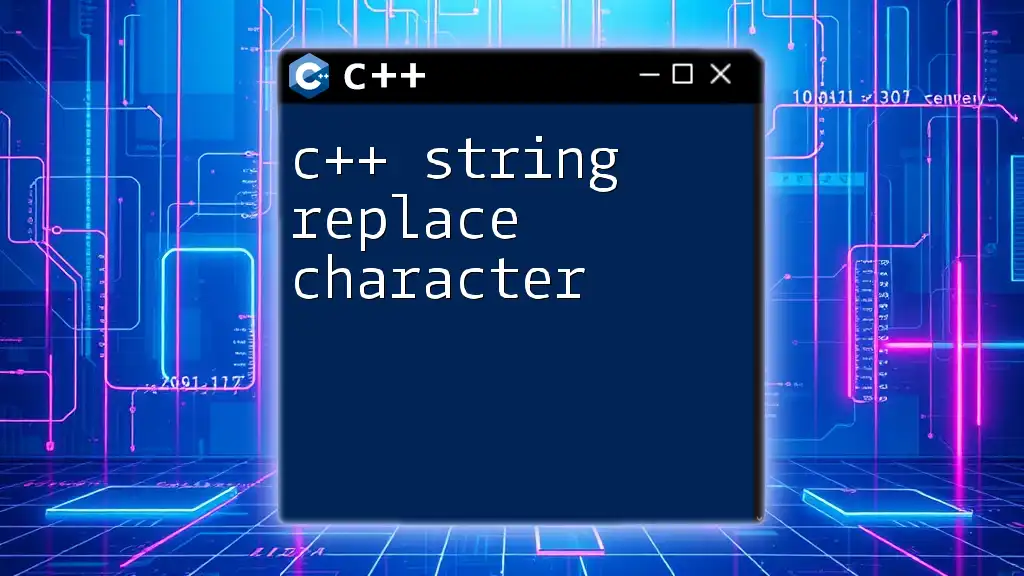
Conclusion
In summary, the C++ string variable declaration process is straightforward, requiring only the correct use of the `std::string` class, along with appropriate initialization methods. It's essential to avoid common pitfalls such as failing to include relevant headers and mixing string types, which can lead to frustrating errors.
By practicing these techniques and implementing the best practices discussed, you can become proficient in string operations in C++. Don’t hesitate to experiment with different string manipulations, as hands-on practice is key to mastering the art of C++ programming.
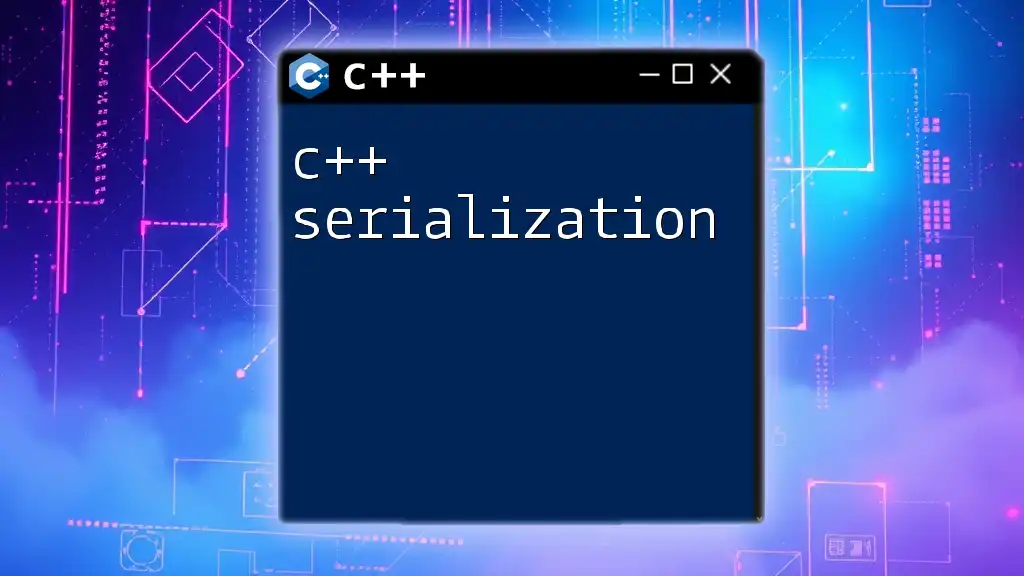
Additional Resources
For further reading, consider accessing C++ documentation and recommended learning platforms that offer tutorials, challenges, and interactive coding environments. Engaging with additional materials will solidify your understanding and enhance your skills in using strings effectively in C++.