C++ graph implementation involves using data structures like adjacency lists or matrices to represent and manipulate graphs, enabling efficient algorithms for traversal and pathfinding.
#include <iostream>
#include <vector>
#include <list>
class Graph {
int V; // Number of vertices
std::list<int>* adj; // Adjacency list
public:
Graph(int V); // Constructor
void addEdge(int v, int w); // Function to add an edge
void printGraph(); // Function to print the graph
};
Graph::Graph(int V) {
this->V = V;
adj = new std::list<int>[V];
}
void Graph::addEdge(int v, int w) {
adj[v].push_back(w); // Add w to v’s list
}
void Graph::printGraph() {
for (int v = 0; v < V; v++) {
std::cout << "Vertex " << v << ":";
for (int w : adj[v])
std::cout << " -> " << w;
std::cout << std::endl;
}
}
int main() {
Graph g(5);
g.addEdge(0, 1);
g.addEdge(0, 4);
g.addEdge(1, 2);
g.addEdge(1, 3);
g.addEdge(1, 4);
g.addEdge(2, 3);
g.addEdge(3, 4);
g.printGraph();
return 0;
}
What is a Graph?
A graph is a fundamental data structure used in computer science to model pairwise relationships between objects. It consists of vertices (or nodes) and edges connecting these vertices. Graphs can represent a myriad of real-world scenarios such as social networks, road maps, and data organization. Understanding graphs is crucial, as they underpin algorithms used in search, optimization, and navigation.
Types of Graphs
Graphs can be categorized into several types based on their characteristics:
- Directed vs. Undirected Graphs: In a directed graph, edges have a direction, indicating a one-way relationship, while undirected graphs imply a two-way relationship.
- Weighted vs. Unweighted Graphs: Weighted graphs assign a cost to each edge, whereas unweighted graphs treat all edges equally.
- Cyclic vs. Acyclic Graphs: Cyclic graphs contain at least one cycle, while acyclic graphs do not, which makes them crucial in representing hierarchical structures or dependencies.

Graph Data Structure Implementation in C++
Why C++ for Graph Implementation?
C++ is an excellent choice for graph implementation due to its ability to provide fine-grained control over system resources and memory. Its performance advantages, such as lower-level memory manipulation and faster execution compared to languages like Python, make it particularly appealing for graph algorithms that require efficiency and speed. Additionally, C++ supports object-oriented programming, making it easy to organize complex data structures.
Basic Components of Graph Data Structure
Vertices (Nodes)
Vertices represent the entities in the graph. For example, in a social network, each user can be a vertex. The cardinality of a graph refers to the total number of vertices it contains, which is crucial in understanding the potential complexity of algorithms acting on the graph.
Edges
Edges are the connections between vertices. Each edge may possess a weight indicating the cost or distance associated with traversing from one vertex to another. The choice of representation for edges—whether weighted or unweighted—depends on the nature of the problem being solved.
Choosing the Right Data Structure for Graphs
Adjacency Matrix
An adjacency matrix is a 2D array where each cell represents the presence (1) or absence (0) of an edge between nodes. It is particularly efficient for dense graphs where the number of edges is close to the maximum possible edges.
Advantages:
- Fast access to check if an edge exists.
- Simple to implement.
Disadvantages:
- Space complexity can be high, especially for sparse graphs.
Here’s a simple implementation in C++:
#include <iostream>
#include <vector>
class Graph {
public:
int vertices;
std::vector<std::vector<int>> adjMatrix;
Graph(int v) : vertices(v) {
adjMatrix.resize(v, std::vector<int>(v, 0));
}
void addEdge(int src, int dest, int weight = 1) {
adjMatrix[src][dest] = weight;
}
};
Adjacency List
An adjacency list consists of an array of lists or vectors, where each entry at index `i` contains a list of all vertices adjacent to vertex `i`. This representation is more memory efficient for sparse graphs.
Advantages:
- Lower space complexity for sparse graphs.
- Easier to iterate over the neighbors of a vertex.
Disadvantages:
- Checking for the existence of an edge is less efficient compared to an adjacency matrix.
Here’s how to implement an adjacency list in C++:
#include <iostream>
#include <list>
class Graph {
public:
int vertices;
std::list<int> *adjList;
Graph(int v) {
vertices = v;
adjList = new std::list<int>[v];
}
void addEdge(int src, int dest) {
adjList[src].push_back(dest);
adjList[dest].push_back(src); // For undirected graph
}
};
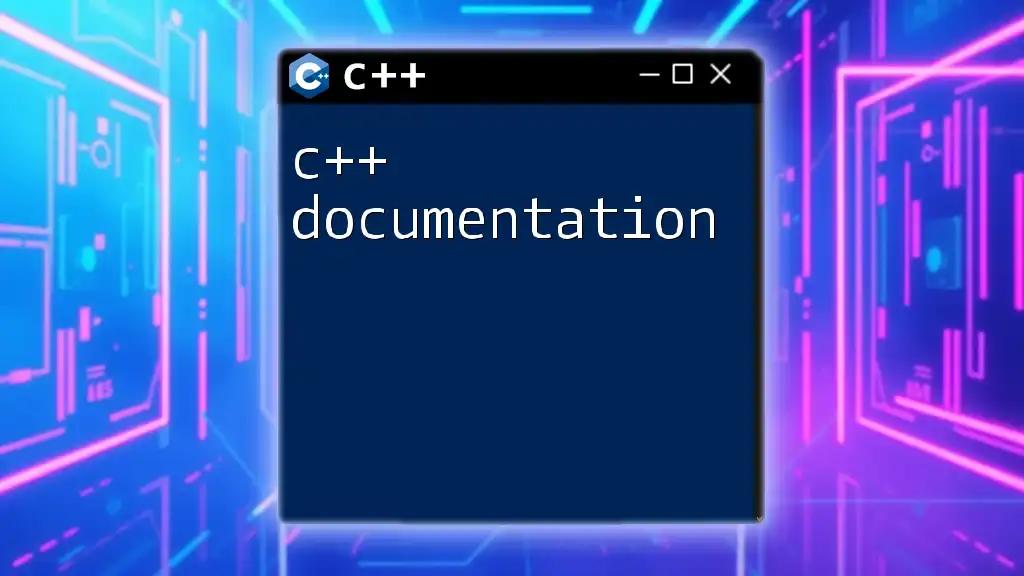
Graph Implementation in C++: Key Algorithms
Depth-First Search (DFS)
Depth-First Search (DFS) is a classic graph traversal method that explores as far into the graph as possible before backtracking. DFS is particularly useful for tasks such as pathfinding, topological sorting, and detecting cycles in a graph.
To implement DFS, we can use a recursive approach:
void DFS(int v, bool visited[], Graph &g) {
visited[v] = true;
std::cout << v << " ";
for (int neighbor : g.adjList[v]) {
if (!visited[neighbor]) {
DFS(neighbor, visited, g);
}
}
}
Breadth-First Search (BFS)
Breadth-First Search (BFS) explores all neighbors of a vertex before moving on to the next level. BFS is particularly useful in finding the shortest path in unweighted graphs.
Here’s an example of BFS implementation:
void BFS(int startNode, Graph &g) {
std::vector<bool> visited(g.vertices, false);
std::queue<int> queue;
visited[startNode] = true;
queue.push(startNode);
while (!queue.empty()) {
int node = queue.front();
queue.pop();
std::cout << node << " ";
for (int neighbor : g.adjList[node]) {
if (!visited[neighbor]) {
visited[neighbor] = true;
queue.push(neighbor);
}
}
}
}

Advanced Graph Techniques
Dijkstra’s Algorithm for Shortest Paths
Dijkstra’s Algorithm is a greedy algorithm for finding the shortest path from a source node to all other nodes in a weighted graph. It is formulated as follows:
- Assign a tentative distance value for every vertex: set it to zero for the initial vertex and infinity for all other vertices.
- Set the initial vertex as the current vertex.
- For the current vertex, consider all of its unvisited neighbors and calculate their tentative distances through the current vertex. Update the neighbor’s distance if this path is shorter.
- Once all neighbors have been considered, mark the current vertex as visited. A visited vertex will not be checked again.
- Select the unvisited vertex with the smallest tentative distance as the next “current vertex” and repeat the process until all vertices are visited.
Here's how you could implement Dijkstra’s Algorithm in C++:
#include <queue>
#include <vector>
#include <limits>
void dijkstra(int src, Graph &g) {
std::vector<int> dist(g.vertices, std::numeric_limits<int>::max());
dist[src] = 0;
std::priority_queue<std::pair<int, int>, std::vector<std::pair<int, int>>, std::greater<>> pq;
pq.push({0, src});
while (!pq.empty()) {
int node = pq.top().second;
pq.pop();
for (int neighbor : g.adjList[node]) {
int alt = dist[node] + 1; // Assuming uniform weight for simplicity
if (alt < dist[neighbor]) {
dist[neighbor] = alt;
pq.push({alt, neighbor});
}
}
}
}

Best Practices for Graph Implementation in C++
Memory Management
When implementing graphs, especially with dynamic structures like adjacency lists, proper memory management is crucial to avoid memory leaks. Always ensure to deallocate any dynamically allocated memory to maintain efficient resource usage.
Efficiency Considerations
Selecting the appropriate graph representation largely depends on the nature of the graph. For example, if a graph is sparse, using an adjacency list is recommended to save space and improve performance. A good understanding of the trade-offs between adjacency matrices and lists is essential for optimizing the performance of graph algorithms.
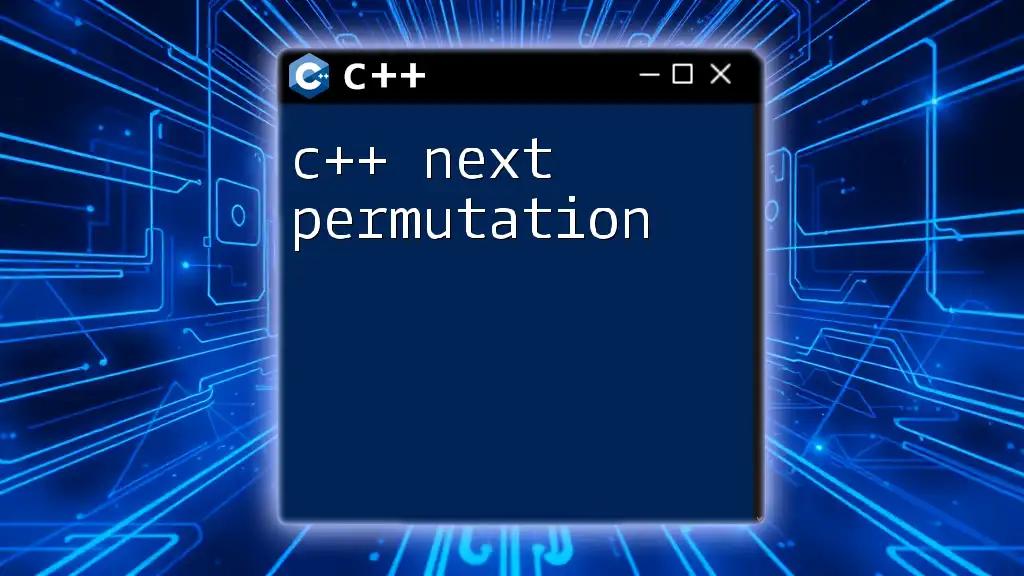
Conclusion
In this guide, we've covered the essentials of C++ graph implementation. From understanding the basic graph components to implementing critical algorithms, you should now have a strong foundation to work with graphs in C++. As you delve deeper, consider exploring libraries like Boost Graph Library for advanced functionalities and optimizations.
Call to Action
Now it’s your turn to put this knowledge into practice. Develop your own graph applications! Engage with the community, share your projects, or ask questions as you continue to learn about this critical data structure.
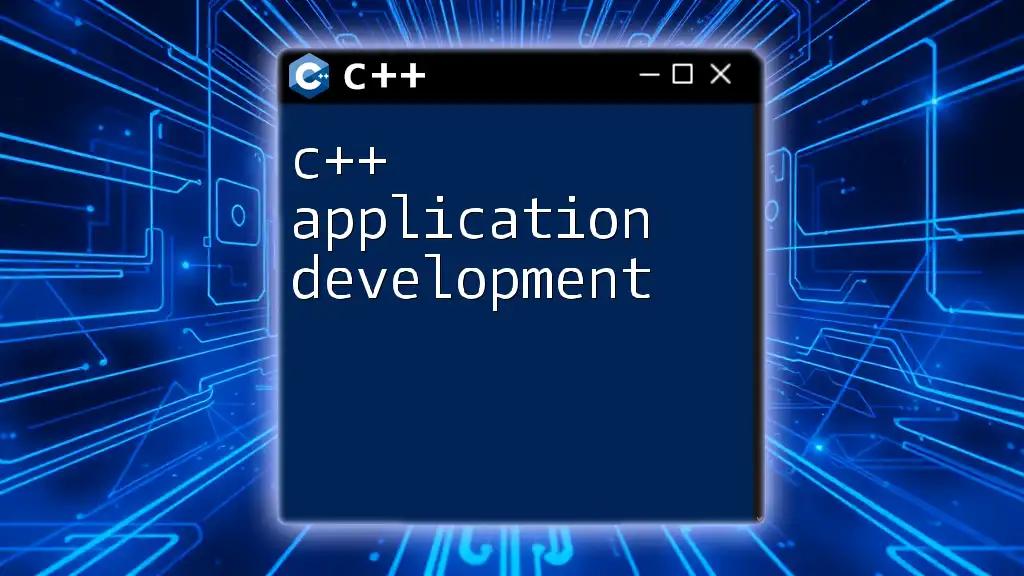
FAQ
Common questions related to graph implementation often revolve around performance comparisons between different representations, the applicability of certain algorithms to specific graph types, and tips for optimizing algorithms in large datasets. As you continue learning, remember that each graph-related challenge offers valuable opportunities to enhance problem-solving skills and deepen your understanding of data structures.