C++ graphing involves using libraries such as Matplotlib for C++ or other graphical libraries to visually represent data in the form of charts and graphs.
Here's a simple code snippet demonstrating how to plot a graph using the Matplotlib C++ interface:
#include <matplotlibcpp.h>
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x = {1, 2, 3, 4, 5};
std::vector<double> y = {1, 4, 9, 16, 25};
plt::plot(x, y);
plt::title("Simple Graph");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
Understanding Graphing in C++
What is Graphing?
Graphing is the visual representation of data, essential for making sense of complex datasets. Through graphs, developers can show relationships, distributions, and trends, allowing stakeholders to grasp information quickly. In programming, graphing often serves to illustrate mathematical functions, data distributions, or algorithm behavior.
Why Use C++ for Graphing?
C++ is renowned for its performance capabilities and efficient memory management. When it comes to graphing, C++ can handle large datasets and complex computations with ease. Moreover, the extensive ecosystem of libraries for C++ makes it a powerful tool for various kinds of graphing tasks. C++ allows developers to create high-performance applications, which are especially beneficial in scientific computing scenarios where speed and responsiveness are paramount.
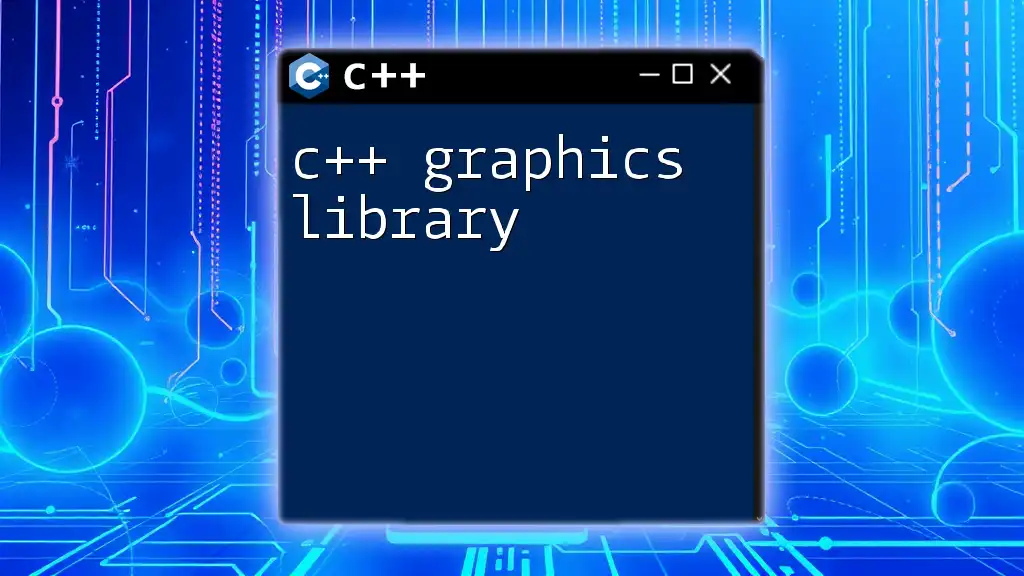
Getting Started with C++ Graphing
Setting Up Your C++ Environment
To dive into C++ graphing, you first need to set up an appropriate development environment. Recommended IDEs include Visual Studio and Code::Blocks, which provide robust support for C++ programming. After selecting an IDE, install the necessary tools and libraries to facilitate graphing.
Essential Libraries for Graphing
Matplotlib-cpp
Matplotlib-cpp is a C++ wrapper for the popular Python plotting library, Matplotlib. It provides a simple interface for generating plots directly from C++. To use Matplotlib-cpp, you first need to install it. Here is an example code snippet to include Matplotlib-cpp in your project:
// Example code to include Matplotlib-cpp in your CMake project
find_package(PythonLibs)
include_directories(${PYTHON_INCLUDE_DIRS} include)
link_directories(${PYTHON_LIBRARY})
SFML (Simple and Fast Multimedia Library)
SFML is another versatile library that excels in creating 2D graphics. While it’s primarily aimed at game development, its features can be employed for graphing as well. When using SFML, consider it for interactive graphical applications. An example of a basic setup for a graph using SFML is shown below:
#include <SFML/Graphics.hpp>
// Example code demonstrating SFML basics for graphing data points
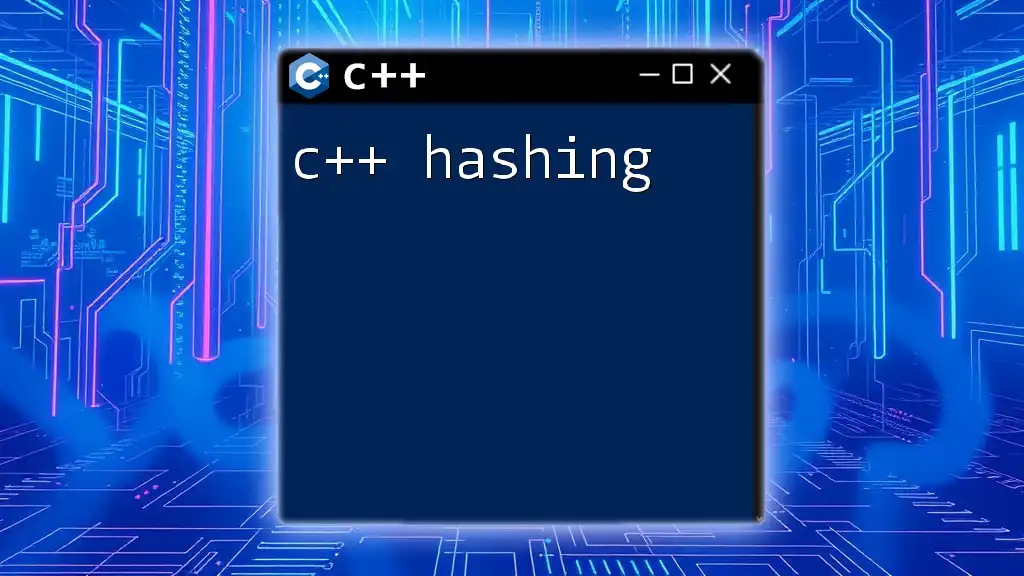
Basic Plotting in C++
Creating Your First Plot
Once your environment is set up, it's time to plot some data. A great starting point is creating a simple plot, such as a sine wave. Here's how you can achieve this using the Matplotlib-cpp library:
#include "matplotlibcpp.h"
namespace plt = matplotlibcpp;
int main() {
std::vector<double> x(100), y(100);
for (int i = 0; i < 100; ++i) {
x[i] = i / 10.0;
y[i] = sin(x[i]); // Computing sine of x
}
plt::plot(x, y);
plt::title("Sine Wave");
plt::xlabel("X-axis");
plt::ylabel("Y-axis");
plt::show();
return 0;
}
In this code, a vector of x-values is generated, and the sine of each is plotted, producing a clear sine wave.
Customizing Your Plots
Adjusting Titles and Labels
An essential aspect of graphing is clarity. Adding informative titles and axis labels enhances understanding. Using Matplotlib-cpp, you can simply customize your graphs as shown in the previous example, demonstrating the ease with which titles and labels can be included.
Modifying Graph Styles
Graph styles can significantly impact the presentation. C++ allows you to modify line styles, colors, and markers easily. Here’s how to change the line style and color in your plot:
plt::plot(x, y, "r--"); // 'r' is for red color, '--' is the dashed line style
This adds clarity and aesthetic appeal to your graphs, making them more engaging.
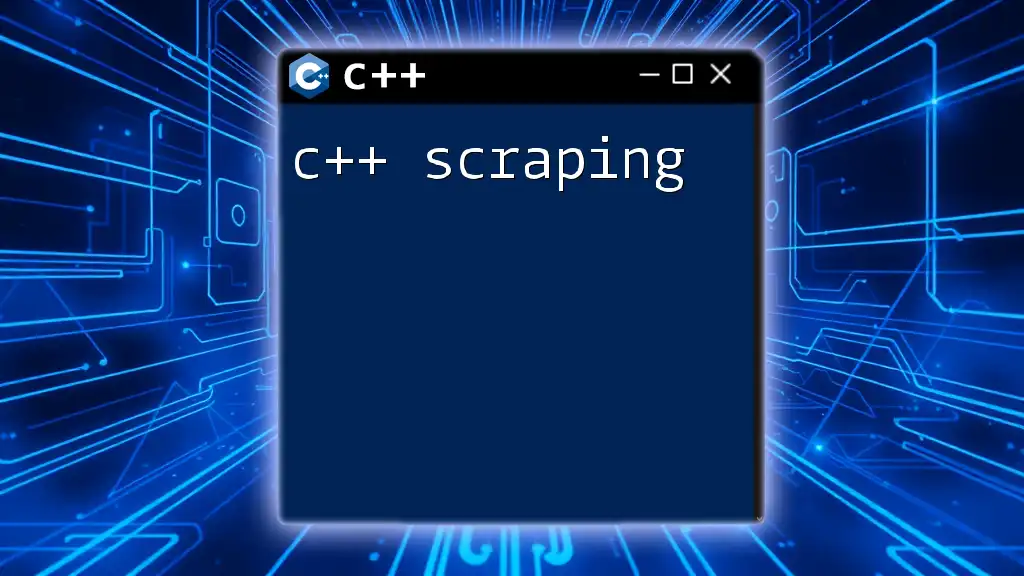
Advanced Graphing Techniques
3D Graphing in C++
3D plots provide more dimensions for data representation. While C++ lacks built-in support for 3D plotting, using libraries like OpenGL can bridge the gap. OpenGL’s capability allows developers to create intricate visualizations. Consider integrating it if your application requires detailed data representation. A basic example might look like this:
// Sample code snippet for creating a 3D plot
#include <GL/glut.h>
// OpenGL setup and rendering code here
Real-Time Graphing
Real-time graphing is crucial for applications such as monitoring systems or trading platforms. C++ allows for efficient real-time plotting using the SFML library. By continuously updating the graph based on incoming data, you can create dynamic, live visualizations. Here’s an example code snippet illustrating how to implement real-time plotting:
#include <SFML/Graphics.hpp>
// Code to create a window and update a graph based on input data
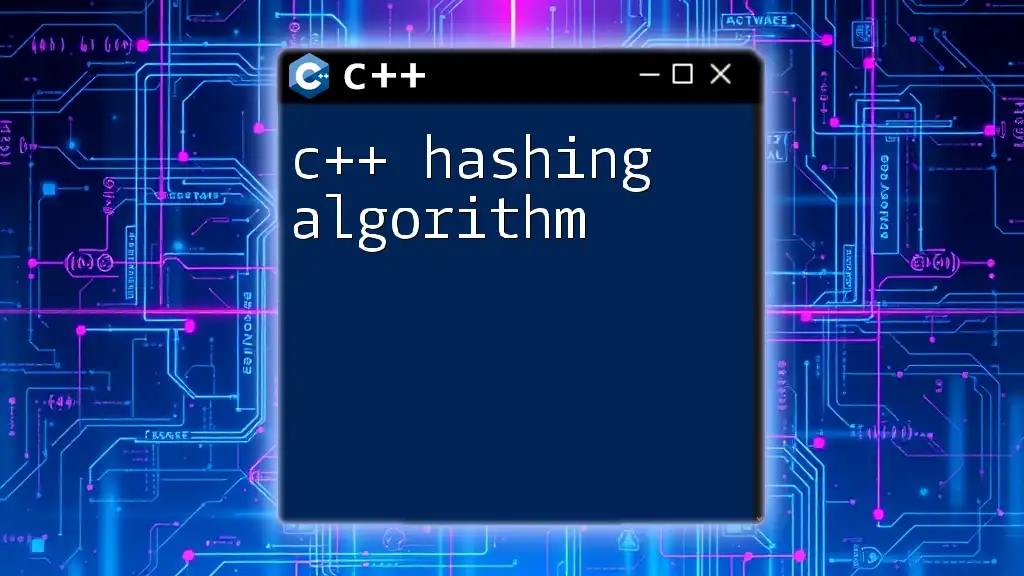
Common Graphing Challenges in C++
Performance Optimization
Performance is often a concern when dealing with extensive datasets or complex computations. Optimize your graphing applications by minimizing redraws, utilizing efficient algorithms, and implementing multi-threading for calculations. Leveraging C++'s support for multi-threading can dramatically improve graph rendering speed.
Debugging Common Issues
When creating graphs, developers may face issues such as rendering problems or incorrect data representation. Common pitfalls include mismanagement of data types or improper library usage. Debugging techniques include checking data inputs, validating library function calls, and ensuring that graphical contexts are correctly set.
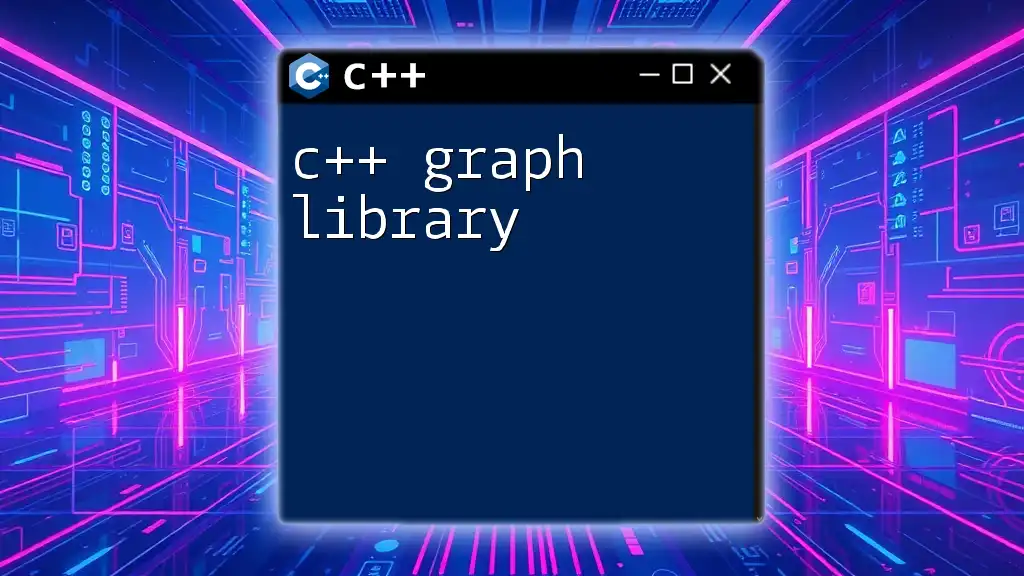
Conclusion
As illustrated, C++ graphing can be an immensely powerful tool when effectively utilized. With its robust libraries and customizable options, C++ graphing allows developers to visualize data comprehensively, making it an essential skill in modern software development. Whether you're plotting simple data or implementing complex visualizations, the flexibility and power of C++ will serve you well in your graphing endeavors.
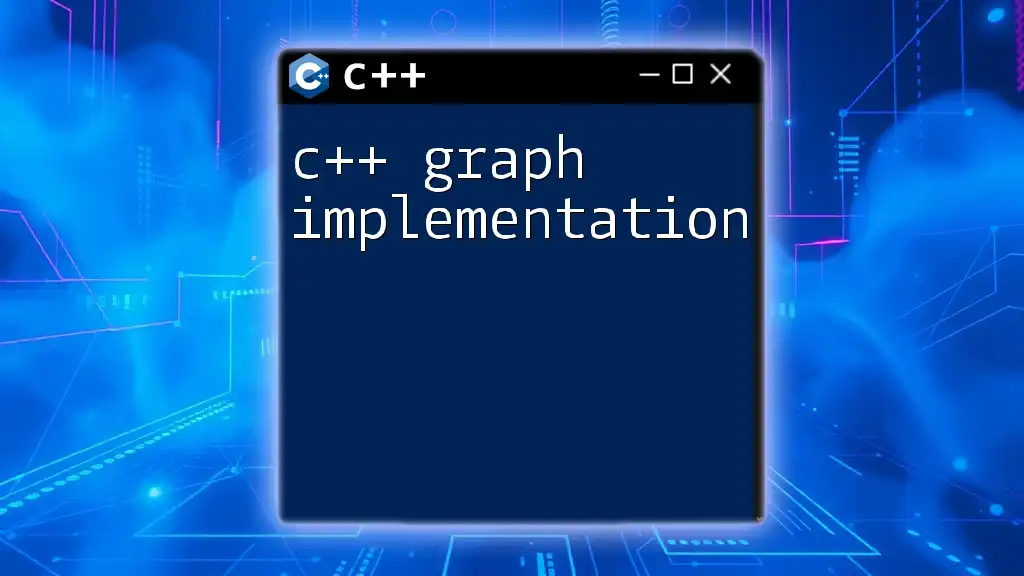
Additional Resources
For further exploration, refer to the documentation for Matplotlib-cpp and SFML. Online resources such as Stack Overflow and GitHub provide a wealth of knowledge and community support for troubleshooting and identifying best practices in C++ graphing.