C++ starting code typically refers to the basic structure of a C++ program which includes necessary headers, the main function, and essential syntax to create a functioning application. Here’s a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful, general-purpose programming language that has evolved significantly since its creation in the late 1970s. Often referred to as an extension of the C programming language, C++ incorporates both high-level constructs and low-level memory manipulation abilities, making it suitable for a diverse range of applications, from system software to game development.
Why Learn C++?
Learning C++ offers numerous advantages. Its versatility allows developers to build robust applications and systems. Additionally, C++ serves as a fundamental stepping stone for learning other programming languages, enhancing your understanding of programming concepts and paradigms. By mastering C++, you'll gain valuable skills that can be applied widely across the technology landscape.
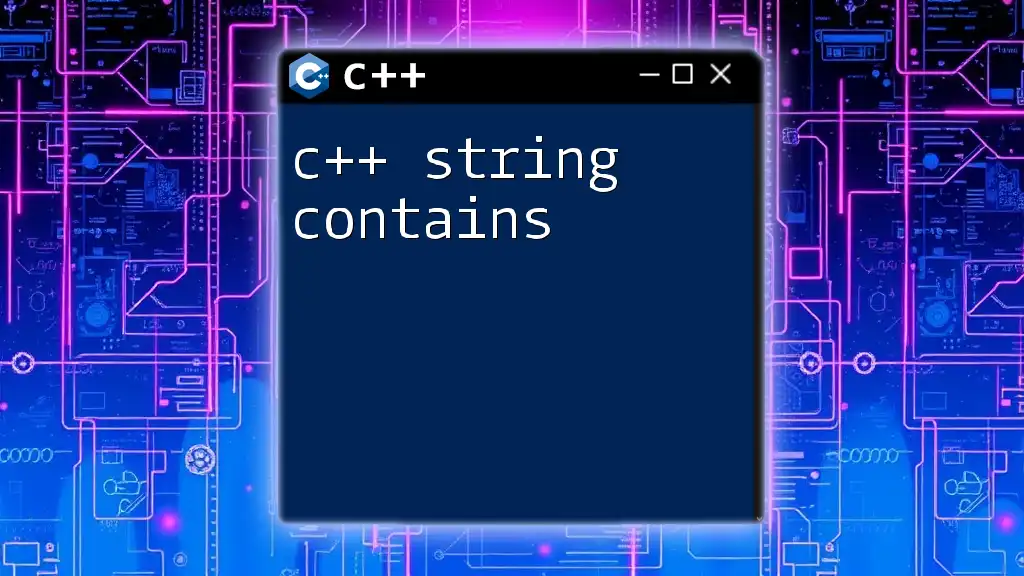
Setting Up Your Environment
Choosing a C++ Compiler
A C++ compiler translates the code you write into machine language that the computer can understand. Here are some popular options:
- GCC (GNU Compiler Collection): A free, open-source compiler that runs on various platforms.
- Clang: A compiler that is known for producing fast executables and having a user-friendly interface.
- Microsoft Visual C++: A part of the Visual Studio suite, ideal for Windows-based development.
For beginners, GCC is often a recommended choice due to its simplicity and extensive documentation.
Installing an Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) provides tools to write, compile, and debug C++ code efficiently. There are several IDEs to consider:
- Code::Blocks: A free, open-source IDE that supports multiple compilers.
- Visual Studio: A feature-rich IDE from Microsoft that is especially powerful for Windows development.
- CLion: A commercial IDE provided by JetBrains, famous for its code analysis features and user-friendly interface.
Step-by-Step Installation Guidelines: Choose an IDE based on your needs, download it, and follow the installation instructions on the official website for a seamless setup.
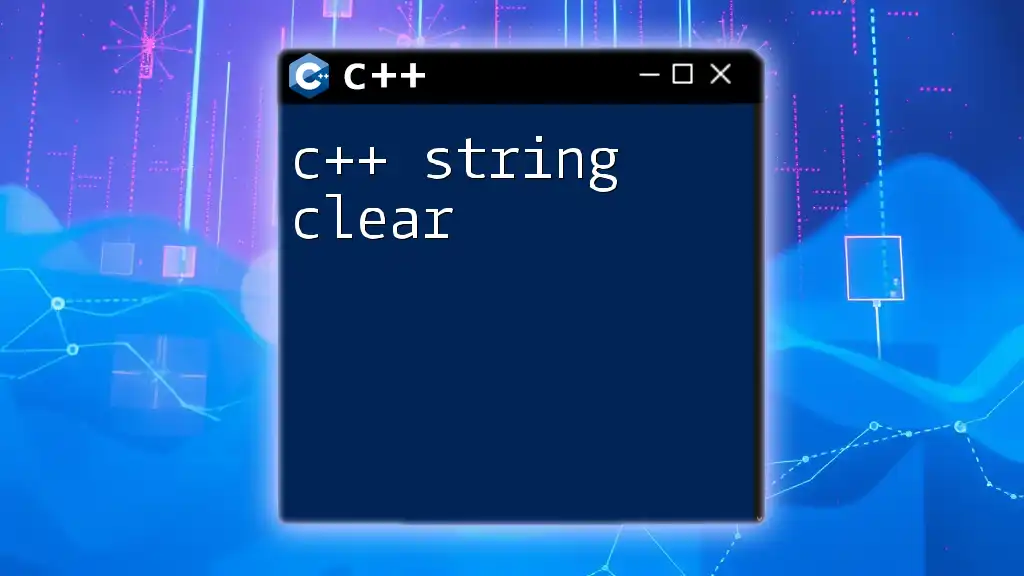
Understanding the Structure of C++ Code
Basic Syntax Overview
The syntax of C++ should be understood to write effective code. Here's a brief overview of key concepts:
- Keywords: Reserved words that have special meaning, such as `int`, `return`, and `include`.
- Identifiers: Names given to entities like variables and functions.
- Data Types: Specify the type of data a variable can hold, for example, `int` for integers, `float` for floating-point numbers, and `char` for characters.
One of the key elements of C++ syntax is the use of semicolons to terminate statements and braces to define blocks of code.
The Main Function
In C++, the program entry point is the `main()` function. Every C++ program must include this function. Below is the basic structure:
int main() {
// Your code here
return 0;
}
The `return 0;` statement indicates successful execution of the program.
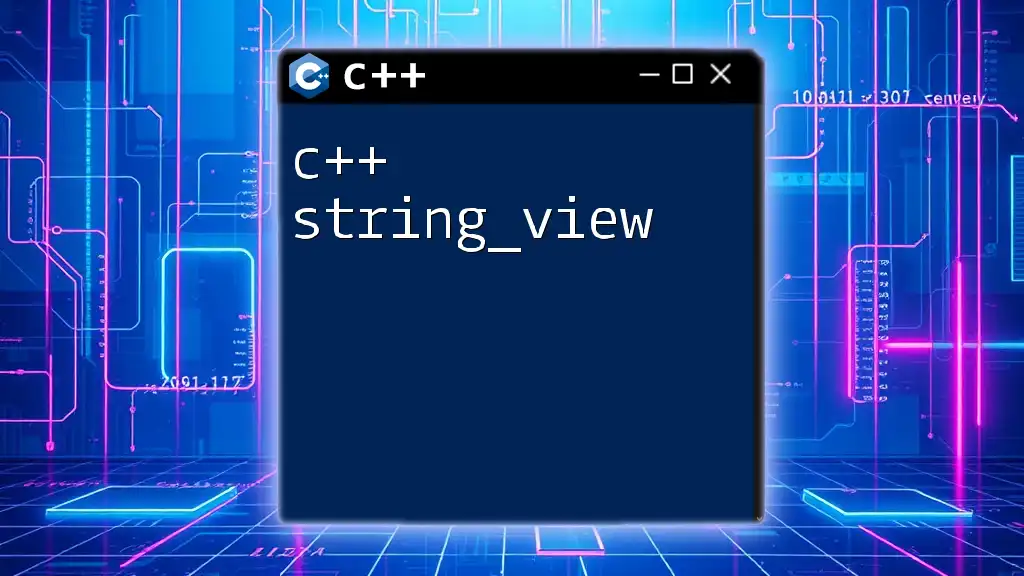
Writing Your First C++ Program
Hello World Program
The "Hello, World!" program is a commonly used demonstration in learning any programming language. It serves as an introduction to the syntax used to produce output.
Here’s how it looks in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Breaking Down the Code
- `#include <iostream>`: This line includes the Input/Output stream library, which is necessary for using `std::cout`.
- `std::cout`: This is the standard output stream used for printing text to the console.
- `std::endl`: It adds a new line after the string output and flushes the output buffer.
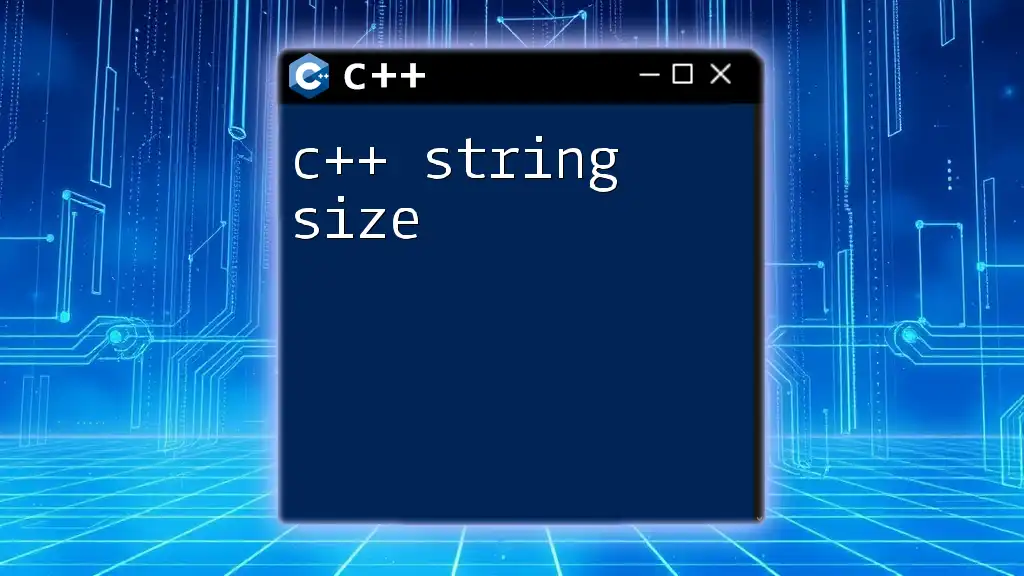
Compiling and Running Your C++ Code
Understanding Compilation
Compilation is the process where code written in a high-level language is converted into machine code. This consists of two main stages: compiling and linking.
How to Compile Using Command Line
If you are using GCC, you can compile and run your program using the command line. Here’s how:
g++ hello.cpp -o hello
./hello
This command compiles `hello.cpp` into an executable named `hello`, which can then be run in the terminal.
Running C++ Programs in an IDE
In an IDE like Code::Blocks or Visual Studio, you can compile and run your program with just a few clicks. Generally, you simply load your project and hit the "Run" or "Build and Run" button.
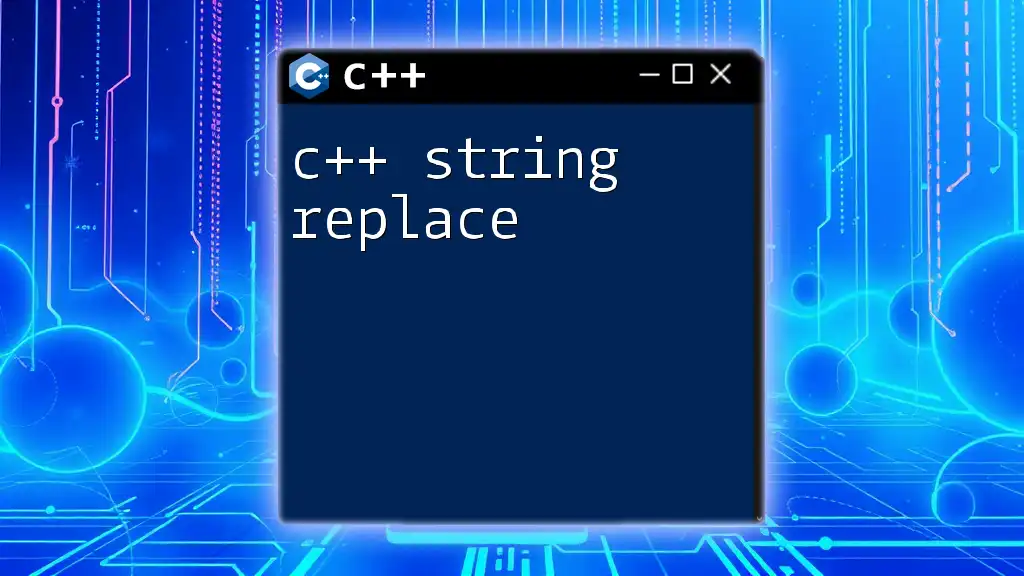
Common C++ Syntax Errors and Troubleshooting
Identifying Syntax Errors
As a beginner, you'll likely encounter syntax errors. These are mistakes like missing semicolons or mismatched braces. Recognizing them early can streamline your programming experience.
Using Compiler Warnings
Many compilers allow you to enable warnings that help identify potential problems before you compile your program fully. Always review warning messages as they can save you time during debugging.
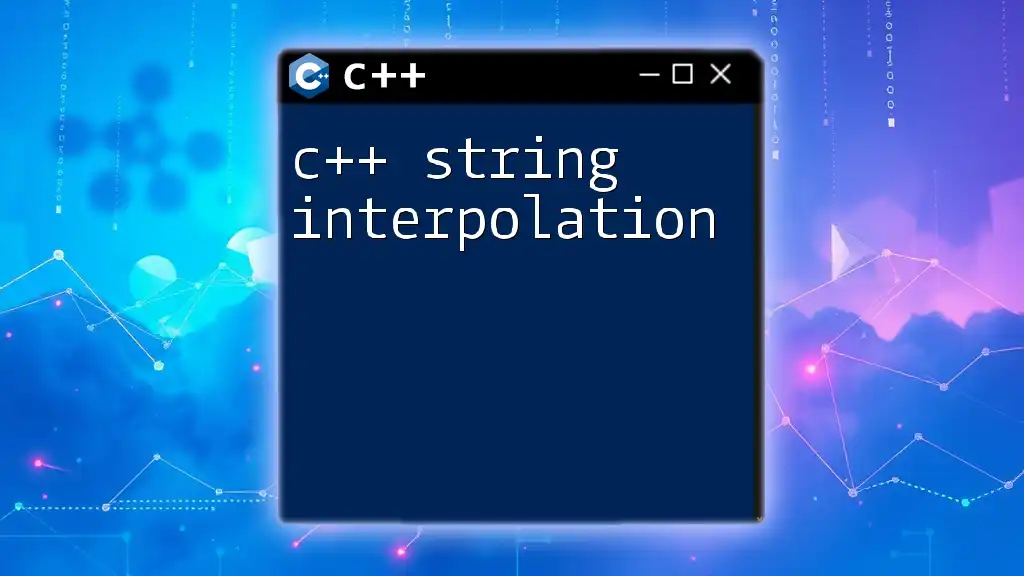
Enhancing Your Code
Comments and Documentation
Writing clear comments in your code is crucial for improving code readability. Comments explain the purpose of the code and make it easier for others (or yourself) to understand later. In C++, you can write single-line comments using `//` or multi-line comments using `/* comment here */`.
Variables and Data Types
Understanding variables and data types is fundamental in C++. Here’s an illustration:
int age = 30; // Integer data type
float height = 5.9; // Floating-point data type
char initial = 'A'; // Character data type
This shows how to declare and initialize variables of different types, which are essential for storing data.
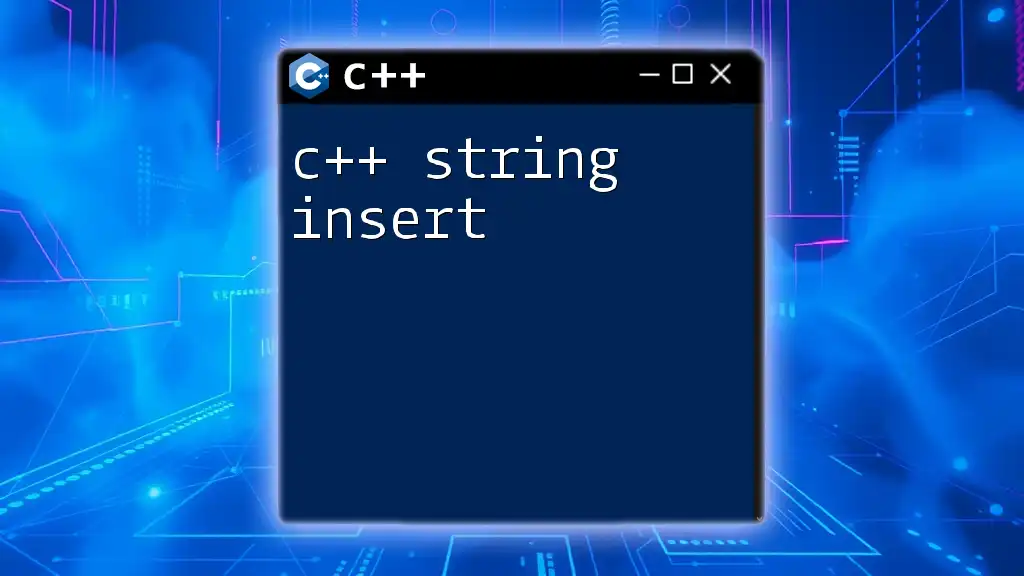
Best Practices for Writing C++ Code
Code Readability
Clean and organized code is easier to read and maintain. Follow best practices such as consistent indentation, meaningful variable names, and structuring your code logically.
Modularity
Modular code, which breaks down functions into smaller, reusable components, is a cornerstone of writing robust programs. Here’s an example of a simple function that prints a greeting:
void greet() {
std::cout << "Welcome to C++ Programming!" << std::endl;
}
This function can be called whenever you want to display the greeting, promoting code reuse.
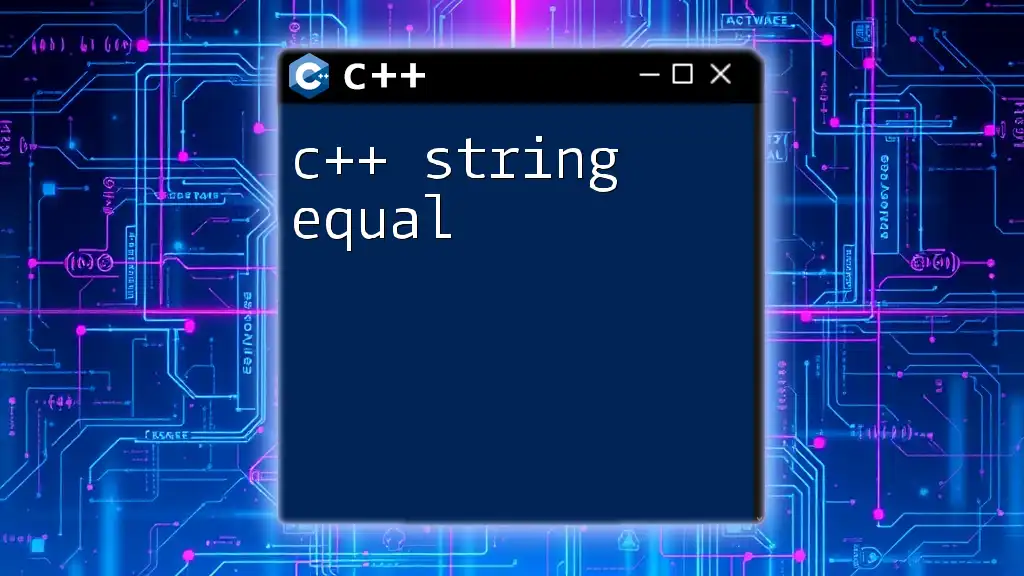
Conclusion
In this guide, we explored essential concepts regarding C++ starting code, from setting up your development environment to writing and running your first program. Understanding these basics is vital as you continue your journey into the rich world of C++ programming.
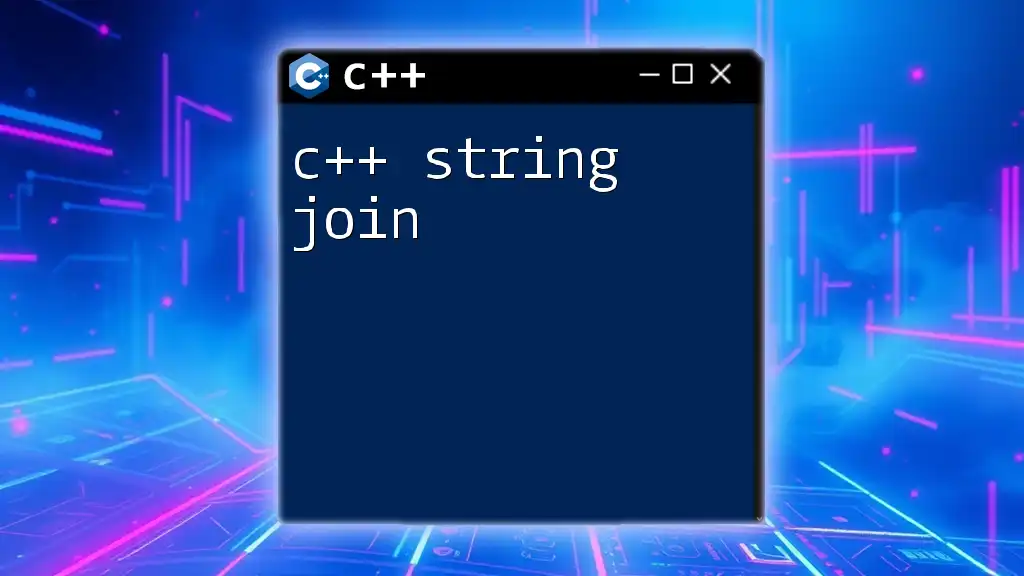
FAQs
What is the easiest way to start coding in C++?
Choose a simple IDE or text editor, install a compiler, and follow beginner tutorials to gradually increase your competence.
What resources are recommended for learning C++?
Consider books like "C++ Primer" and online platforms like Codecademy or Coursera.
How long does it take to become proficient in C++?
It varies by individual, but with consistent practice, you can gain a solid foundation in a few months.
Can C++ be used for web development?
Yes, although it is more commonly used for system-level programming, game development, and applications requiring high performance, there are web frameworks compatible with C++.
Embrace the learning process and continue coding! The more you practice, the more proficient you'll become in writing C++ starting code.