The `clear()` function in C++ is used to remove all characters from a string, effectively emptying it.
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
myString.clear(); // This will empty the string
std::cout << "String after clear: '" << myString << "'" << std::endl; // Output will be empty
return 0;
}
What is the C++ String Class?
The C++ string class, part of the Standard Template Library (STL), is a sophisticated and versatile way to manage text data. Unlike C-style strings, which are simple arrays of characters terminated by a null character, the C++ string class offers various built-in functionalities that enhance ease of use and flexibility.
Advantages of Using C++ Strings
- Memory Safety: C++ strings automatically manage memory allocation and deallocation, reducing the chances of memory leaks.
- Dynamic Resizing: Unlike fixed-size arrays, C++ strings can grow or shrink as needed, allowing for better memory usage.
- Rich API: The string class provides numerous methods for string manipulation, comparison, searching, and more.
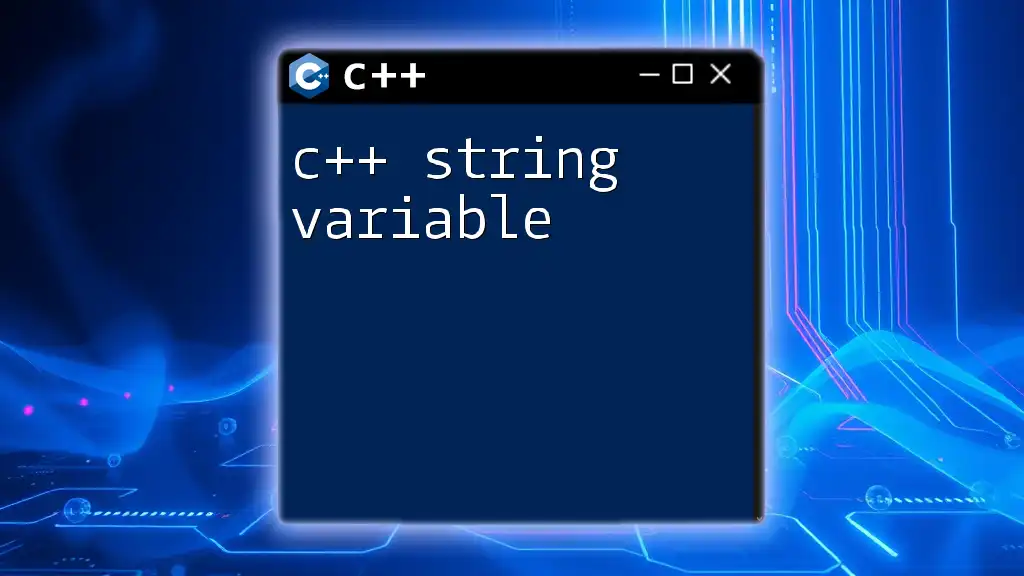
Understanding `clear()` Method
The `clear()` method of the C++ string class is a straightforward way to empty the contents of a string. Calling `clear()` effectively makes the string's size zero without destroying the string object itself.
Purpose of the `clear()` Method
The primary purpose of `clear()` is to remove all characters from a string, leaving it empty but still valid for further operations. This is particularly useful for reusing string variables instead of creating new ones, which can be more resource-intensive.
Syntax of `clear()`
The syntax for using the `clear()` method is simple:
string str;
str.clear();
This method does not take any parameters and returns no value.
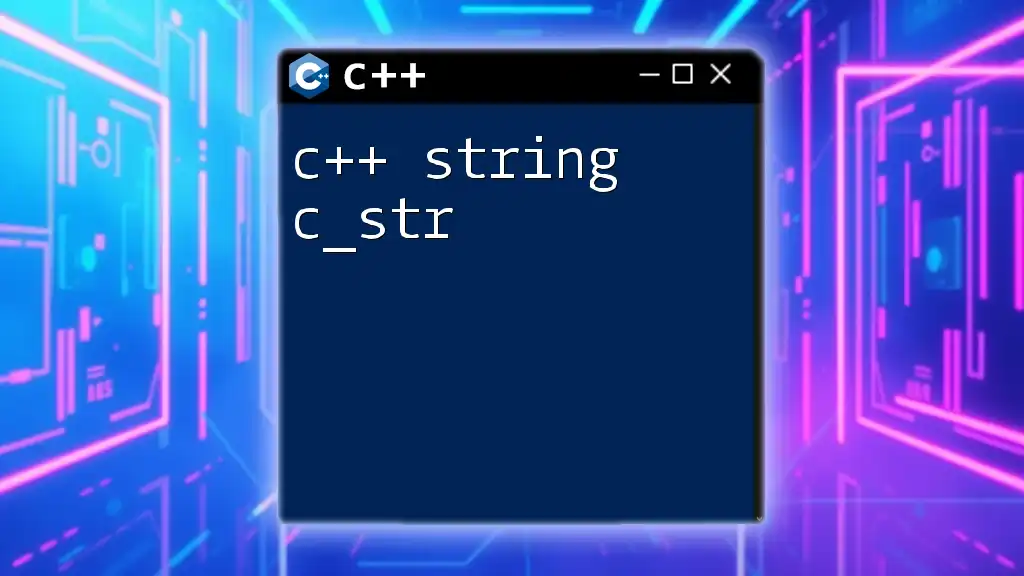
How to Use `clear()` Method
Example of `clear()` in Action
To illustrate the `clear()` method, consider the following code snippet:
#include <iostream>
#include <string>
int main() {
std::string myString = "Hello, World!";
std::cout << "Original string: " << myString << std::endl;
myString.clear(); // Emptying the string
std::cout << "String after clear: \"" << myString << "\"" << std::endl;
return 0;
}
In this example, the output will first display "Original string: Hello, World!", then after calling `clear()`, it will show "String after clear: """, indicating that the string is now empty.
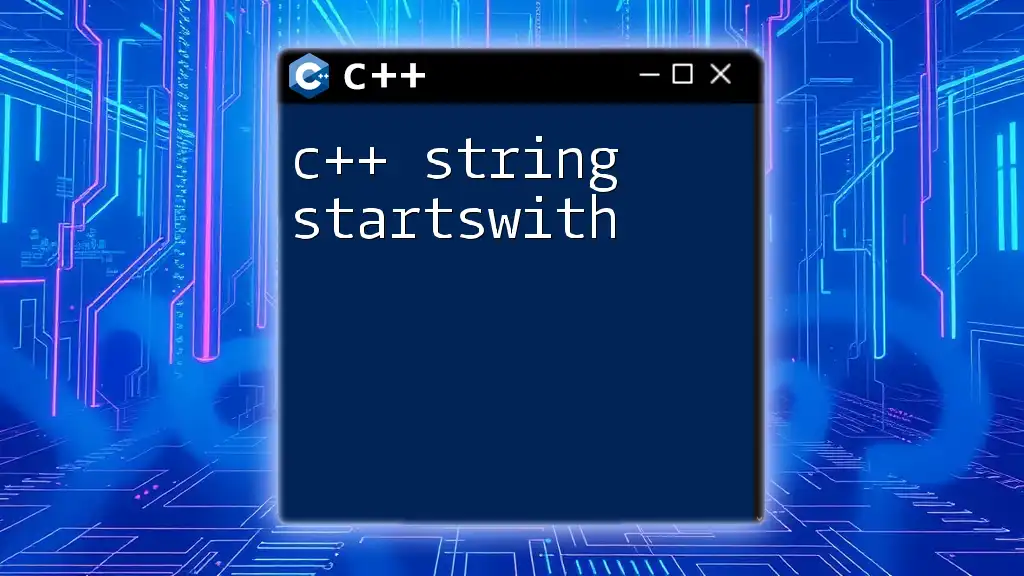
Benefits of Using `clear()`
Memory Management
One of the significant advantages of using the `clear()` method is its contribution to efficient memory management. When you call `clear()`, the string's size is reset to zero, freeing up resources associated with the string's characters. This is particularly useful when dealing with large strings or when you need to process a string multiple times.
Avoiding Undefined Behavior
Using `clear()` can also help prevent undefined behavior. For example, if you clear a string that is referenced elsewhere in your program but forget to handle its new state, it may lead to errors or crashes. By ensuring you call `clear()` when necessary, you can maintain better control over your string data.
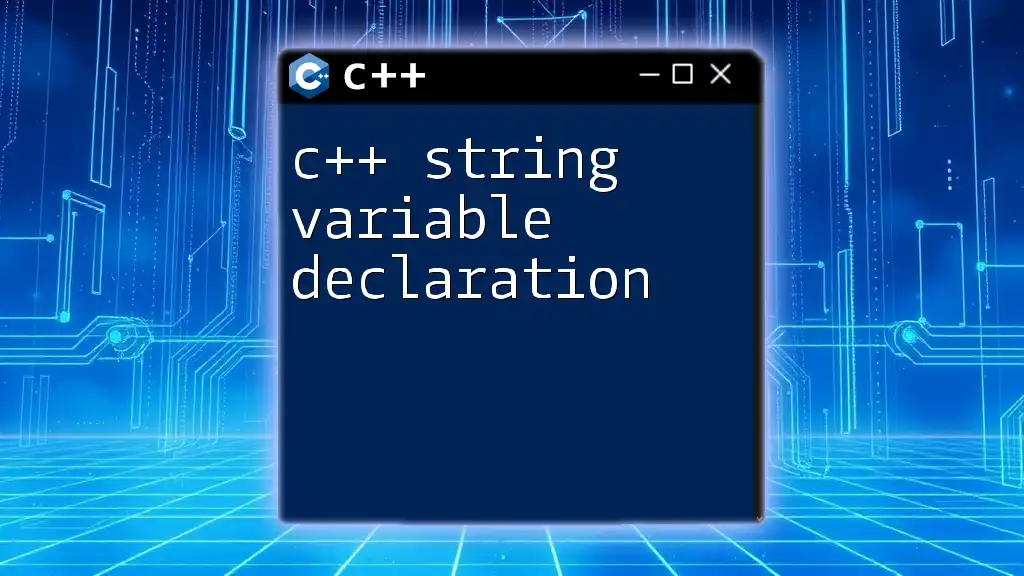
Common Mistakes When Using `clear()`
Forgetting to Check String Status
One common oversight is failing to check the state of the string after calling `clear()`. Always consider using the `empty()` method to verify if the string is indeed empty:
if (myString.empty()) {
std::cout << "String is now empty." << std::endl;
}
This practice ensures that your code behaves as expected and helps avoid issues related to string states.
Overusing `clear()`
While `clear()` is handy, overusing it can lead to unnecessary calls, especially if you are already managing the content of strings efficiently. In many cases, you may not need to clear a string if you're simply overwriting its contents.
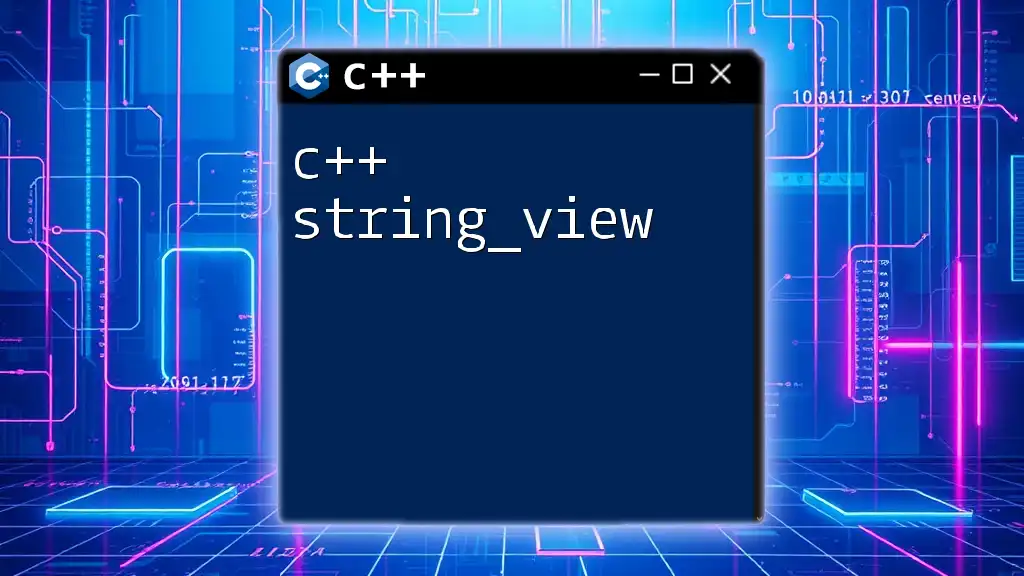
Comparing `clear()` with Other String Methods
`erase() vs. clear()`
While both `clear()` and `erase()` can be used to remove characters from a string, they serve different purposes. `clear()` completely empties the string, while `erase()` allows for more granular control by removing specific parts of the string:
std::string myString = "Hello, World!";
myString.erase(5, 7); // Removes ", World"
In this example, only the specified portion of the string is removed, leaving the rest intact.
`assign() vs. `clear()`
Another alternative to `clear()` is to use `assign()` to change the string's contents directly:
myString.assign("");
This can be useful if you want to reset the string while also providing the option to overwrite it with new data in a single step.
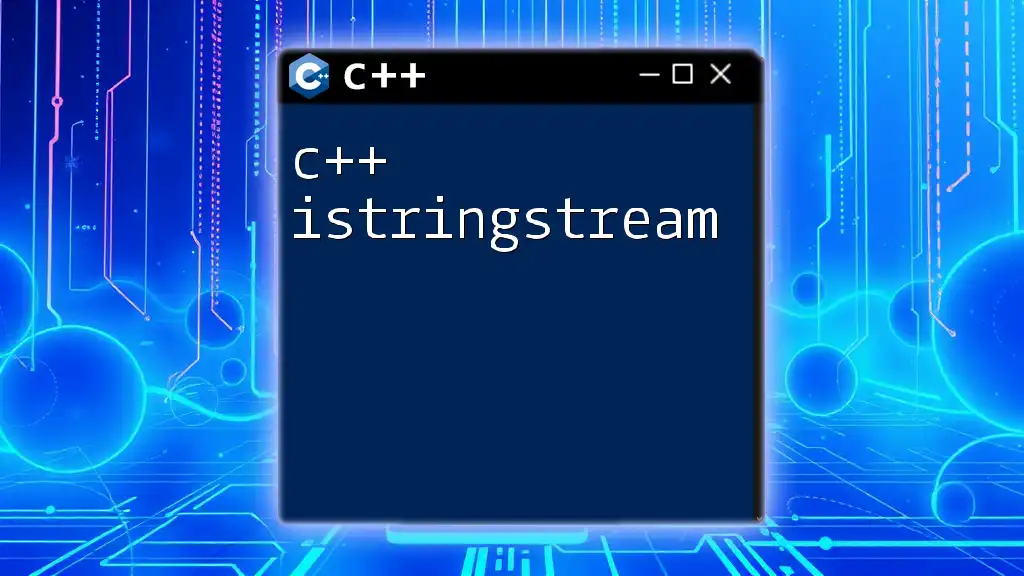
Best Practices for String Management in C++
To effectively manage strings in C++, follow these best practices:
- Prefer using `clear()` when you want to reset a string without creating a new one.
- Utilize `empty()` to check the string's status after clearing it.
- Avoid excessive calls to `clear()`; consider reusing strings to enhance performance.
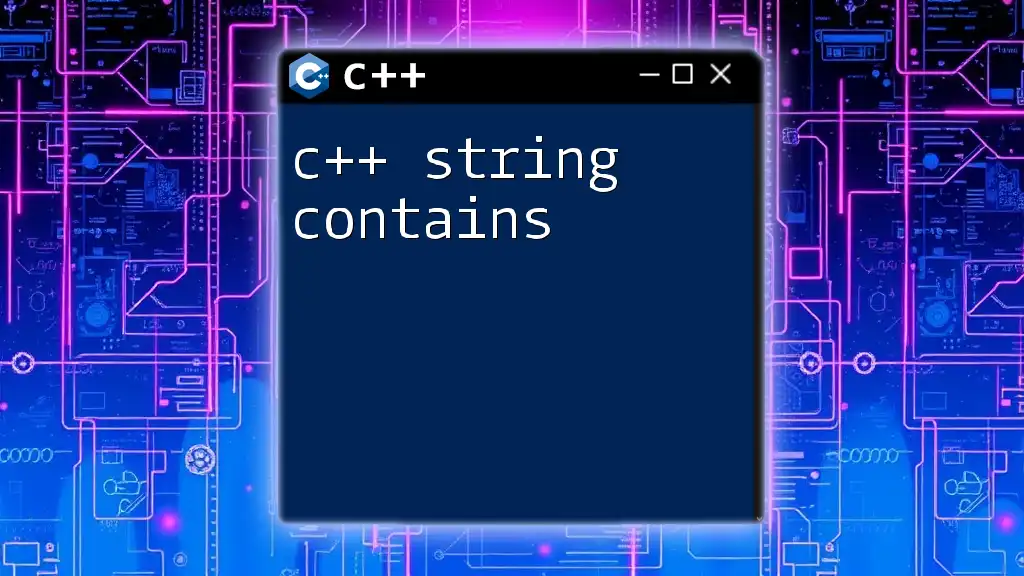
Conclusion
Understanding the C++ string clear method is crucial for efficient string manipulation and memory management. The `clear()` method not only facilitates easier coding by allowing you to reset strings, but it also helps prevent potential issues with undefined behavior. Practicing effective string management techniques, including the appropriate use of `clear()`, will greatly enhance your programming capabilities in C++.
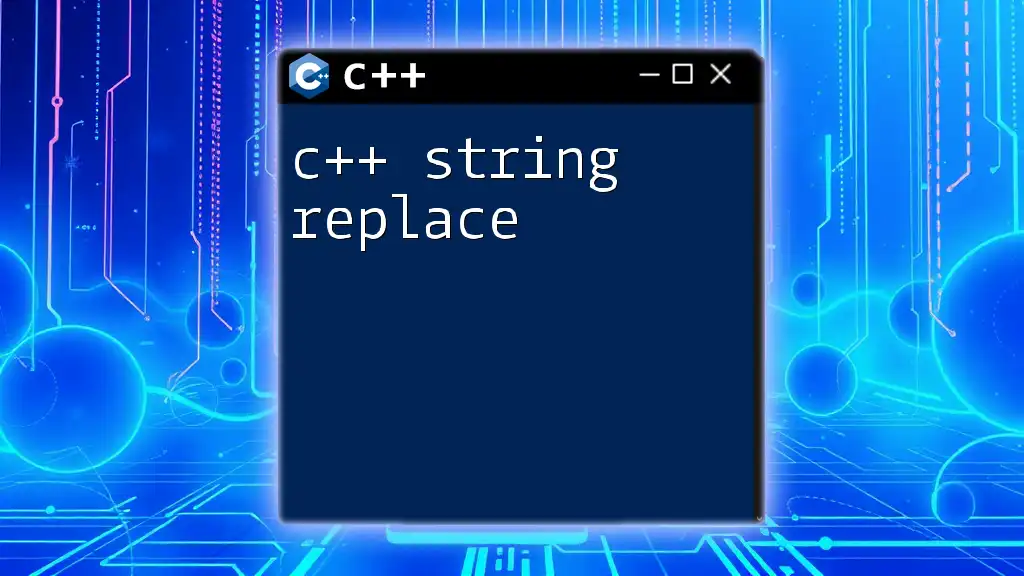
Additional Resources
For further exploration, consider reviewing the official C++ documentation concerning string manipulation and the `clear()` method. There are also a multitude of excellent books and online tutorials aimed at helping you master C++ strings.
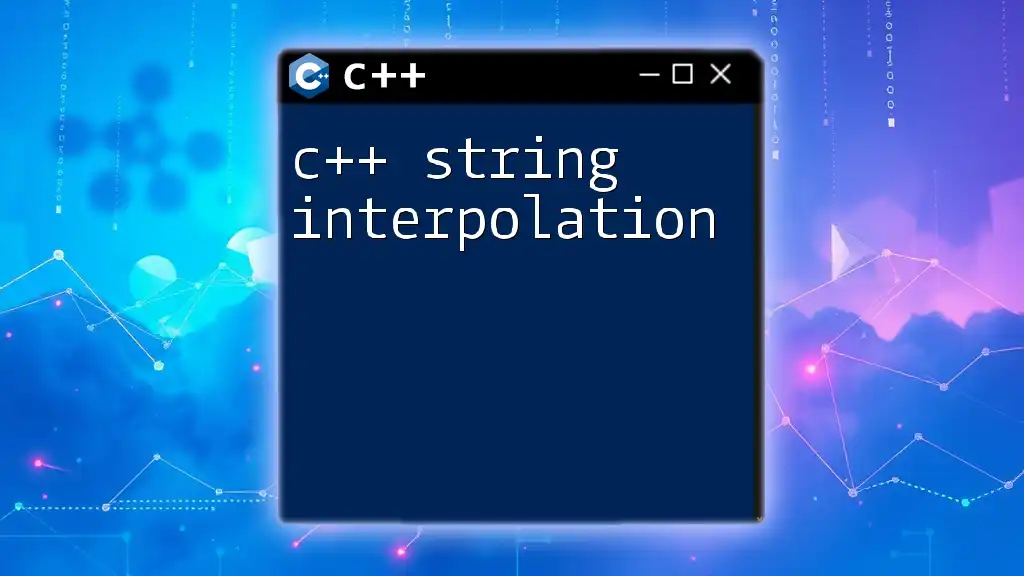
Call to Action
Experiment with the `clear()` method in your coding projects, and don't hesitate to share your experiences or questions about string management. Follow our blog to stay updated on more C++ programming tips and tricks!